In-Depth Analysis of Python Syntax for All Levels
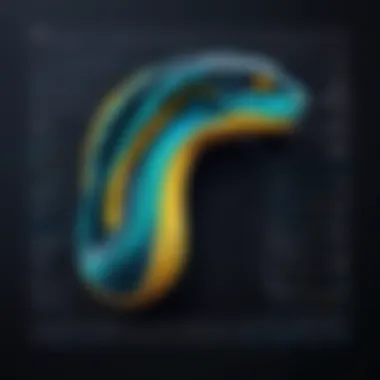
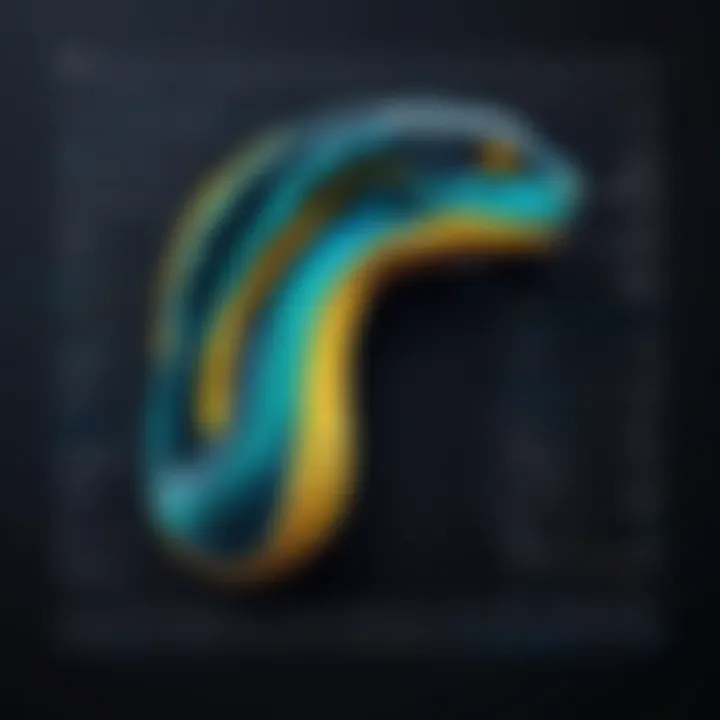
Intro
Python programming has gained remarkable traction over the years due to its simplicity and versatility. Understanding the core syntax of Python is essential, whether youâre embarking on your coding journey or are a seasoned developer looking to refine your skills. Syntax is not merely a set of rules; it is the very foundation on which Python operates, determining how code is structured and how it executes.
This guide aims to demystify the various elements of Python syntax. By unpacking its fundamental components, youâll get a clearer picture of how to write clean and efficient code. The following sections will explore everything from data types to control flow, diving into best practices that can significantly impact your coding experience.
Overview of Python Syntax
Definition and Importance of Syntax
In the realm of programming, syntax refers to the set of rules that defines the combinations of symbols and keywords in a programming language. In Python, proper syntax is crucial as it dictates how commands and statements are constructed. Getting grasp on the nuances of Python syntax can be the difference between a smoothly running program and one laden with errors.
Key Features and Functionalities
Python syntax is designed to be both intuitive and expressive, making it accessible to a wide range of users. Here are a few of its notable characteristics:
- Indentation: Unlike many languages that use braces or keywords to indicate blocks of code, Python uses indentation to define the structure. This enforces readability, making it clear where code blocks begin and end.
- Dynamic Typing: No need to declare variable types explicitly; Python infers the data type at runtime, allowing for flexibility in coding.
- Rich Libraries: Python's extensive standard library reduces the need for lengthy code by offering powerful pre-built functions.
Use Cases and Benefits
From web development to data analysis, Python's syntax allows it to be versatile across various domains:
- Web Development: Frameworks like Django and Flask utilize Python's clear syntax to build robust web applications.
- Data Science: Libraries such as pandas and NumPy thrive under Python's expressive syntax, enabling efficient data manipulation and analysis.
- Automation: Python's syntactical simplicity facilitates quick script development for task automation.
Best Practices
Implementing best practices is key to harnessing Pythonâs full potential:
- Embrace Readability: Write code that others can understand at a glance. Use meaningful variable names and descriptive comments.
- Consistent Indentation: Stick to a consistent indentation style to avoid confusion. Pythonâs reliance on indentation for structure mandates this discipline.
- Leverage Built-in Functions: Familiarize yourself with Python's rich set of built-in functions rather than reinventing the wheel; they save time and improve efficiency.
Tips for Maximizing Efficiency
- Understand the Zen of Python: Familiarize yourself with the guiding principles of Python, often referred to as "The Zen of Python." You can access this by typing in your Python shell.
- Modular Code: Break your code into functions. The smaller the function, the easier it is to test and debug.
- Error Handling: Implement try-except blocks to gracefully handle unexpected errors without crashing your program.
Common Pitfalls to Avoid
- Forgeting to Indent: Even seasoned coders can overlook indentation rules, leading to exceptions.
- Overusing Global Variables: This can lead to code that is difficult to debug and maintain.
- Ignoring Documentation: Failing to document your code can hinder future updates and collaborative efforts.
Ending
Mastering Python syntax is more than just knowing the rules; it's about understanding how to apply them effectively in real-world scenarios. As you navigate through the intricacies of Python, remember that clarity and efficiency are your best friends. The journey of learning Python syntax is ongoing, but with each line of code you write, you inch closer to becoming an adept programmer.
Understanding Python Syntax
Understanding the syntax of Python is akin to learning the rules of a game before you start to play it. Having a solid grasp of these rules not only helps in writing code that works but also in communicating effectively with others in the programming community. Syntax is the foundation upon which programming logic is built; it defines how statements should be structured. If youâve ever encountered a syntax error, you know how frustrating it can be. Itâs like trying to navigate through a maze with no clear path. Keeping an eye on syntax is crucial because it dictates how the Python interpreter reads your code, making it a vital part of software development.
When programmers embrace Python's syntax, they unlock the language's full potential. Key elements such as indentation, statements, and expressions serve as the building blocks that shape the program's flow and functionality. They help maintain clarity, allowing developers to focus on solving problems rather than getting lost in a forest of code.
Moreover, understanding Python's syntax can lead to several benefits:
- Error Reduction: Knowing the correct syntax minimizes the likelihood of errors during coding.
- Collaboration: Clear and consistent syntax allows for better teamwork, as others can easily read and understand your code.
- Maintainability: Well-structured code is easier to maintain and adapt over time.
In the realm of programming, familiarizing oneself with the syntax may seem daunting at first, especially for newcomers, yet it is undeniably rewarding and essential for effective programming.
The Importance of Syntax
To put it bluntly, syntax is the backbone of any programming language, and Python is no exception. Think of syntax as the grammar for coding. Just as grammar ensures that a sentence conveys a clear meaning, proper syntax guarantees that the interpreter comprehends the instructions your code is meant to execute.
A primary reason why syntax holds immense significance is that Python emphasizes readability through its design. It gives a nod to the idea that code should be as understandable as a book. In this light, adhering to correct syntax allows programmers not only to communicate with machines but also to express ideas clearly to their peers.
In practice, using proper syntax impacts several aspects of programming:
- When code is clean and well-structured, itâs easier to debug.
- A programmer has more ease in understanding the flow of logic when revisiting their older projects.
- Other developers who might work on your code will find it more approachable, reducing the learning curve.
Thus, maintaining a solid grasp of syntax isnât just beneficial; itâs an imperative part of being a proficient Python developer.
How Syntax Influences Readability
Readability is one of Python's most celebrated features. The language was designed to allow developers to express concepts in fewer lines of code than would be necessary in languages such as C++ or Java. It makes sense, then, that syntax plays an influential role in enhancing the readability of Python code.
To put it simply, when code is easy to read, it also becomes easier to understand, maintain, and modify. Consider the following:
- Use of Indentation: Unlike many programming languages, Python uses whitespace (indentation) to define code blocks. This design choice allows the structure of complex code to be visually apparent without additional syntax, making it straightforward to follow.
- Explicit Expressions: Python syntax encourages clear and concise expressions. For instance, using keywords like , , or acts as a clear signal to what follows, granting the code an almost sentence-like quality.
- Natural Language Elements: The use of common English phrases in Pythonâs syntax allows for self-documenting code. Much of the time, one can glean the purpose of code just by reading it.
Some popular practices to ensure readability include:
- Writing comments where necessary
- Employing descriptive naming conventions for variables and functions
- Organizing code into functions or modules when appropriate
As a side note, consider this:
"The clearer you write your code, the fewer headaches you will give yourself and others later on."
Basic Syntax Components
Understanding the basic syntax components in Python is essential for anyone stepping into the world of programming. Syntax can be seen as the grammar of a programming language, dictating how code must be structured for the interpreter to understand it. Getting a handle on these foundational elements ensures that developers can communicate effectively with Python. Solid knowledge of syntax translates to fewer errors, increased productivity, and overall better code.
The syntax in Python is designed for simplicity and clarity, making it an approachable language for beginners while still being powerful enough for experts. By focusing on key components, such as statements, expressions, and indentation, programmers can significantly enhance their coding proficiency.
Statements and Expressions
At the core of Python programming are statements and expressions. A statement is a line of code that performs an action, while an expression evaluates to produce a value. The difference might seem a bit subtle, but understanding it is pivotal.
- Statements: Examples include assignments and function calls. For instance, in the code snippet below:The first line is an assignment statement, whereas the second line is a call statement. The interpreter executes these statements sequentially, and any misstep in syntax can throw a wrench into the works.
- Expressions: These can be arithmetic operations or function calls that return a result. In the case of the following code:The expression evaluates to a new value based on the original value of . Understanding the role of expressions allows developers to manipulate data efficiently and build complex logic.
Recognizing the distinction between the two allows programmers to write code that behaves as intended while minimizing errors. This clarity also aids in debugging, as developers can trace issues back through their statements and expressions with much greater ease.
Indentation Rules
Another unique feature of Python syntax is its reliance on proper indentation. Unlike many programming languages that use braces or keywords to delineate blocks of code, Python uses whitespace as a part of its syntax. This can be both a blessing and a curse.
- Importance of Indentation: It's not just about aesthetics; indentation signifies grouping of statements. For example:In this snippet, the indentation shows which statements are part of the block and which are part of the block. Misaligning the indentation can result in , which can sometimes be puzzling for new developers.
- Best Practices: Adopt a consistent style for indentation, whether it's four spaces or a tab. Many teams opt for spaces given its wider compatibility across various editors and platforms.
In summary, mastering these basic syntax componentsâstatements, expressions, and indentationâserves as a solid foundation for more complex programming tasks. As developers dive deeper into Python, these elements help them write cleaner and more effective code, paving the way for mastering the language overall.
"In programming, clarity serves as your best ally; strong syntax transforms thoughts into code that resonates."
This clarity is critical when tackling larger projects or collaborating in teams, where misunderstanding can cost valuable time.
Happy coding!
Data Types and Their Syntax
Understanding the various data types in Python is fundamental when it comes to programming effectively. Each type serves a distinct purpose, allowing programmers to manage and manipulate data with precision and ease. When you grasp these types, you're well-equipped to handle everything from simple arithmetic to complex data structures. Having a solid understanding of data types not only bolsters your coding skills but also improves your program's performance and readability.
Numeric Types
Numeric types are perhaps the most straightforward data types youâll encounter. Python supports integers, floating-point numbers, and complex numbers, allowing you to perform a vast array of calculations.
- Integers are whole numbers without a decimal point. For example, and are integers. They're useful for counting items or performing operations requiring whole numbers.
- Floats, or floating-point numbers, are used for representing real numbers. They include decimal points, like or . Floats come into play when calculations require precision, such as in scientific computations.
- Complex numbers are written with a real part and an imaginary part, such as . Although theyâre less commonly used in everyday coding, they are indispensable in fields like engineering and physics.
Hereâs a simple code example demonstrating numeric types:
Your choice of numeric type can affect the outcome of calculations, especially when it comes to performance and memory usage. Always opt for the most fitting type to achieve optimal results.
String Formatting
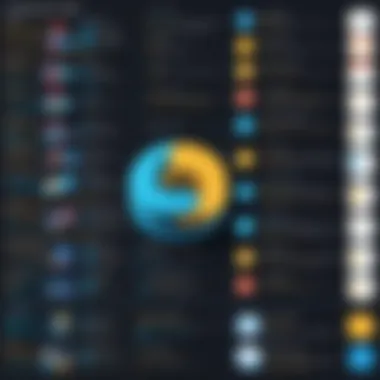
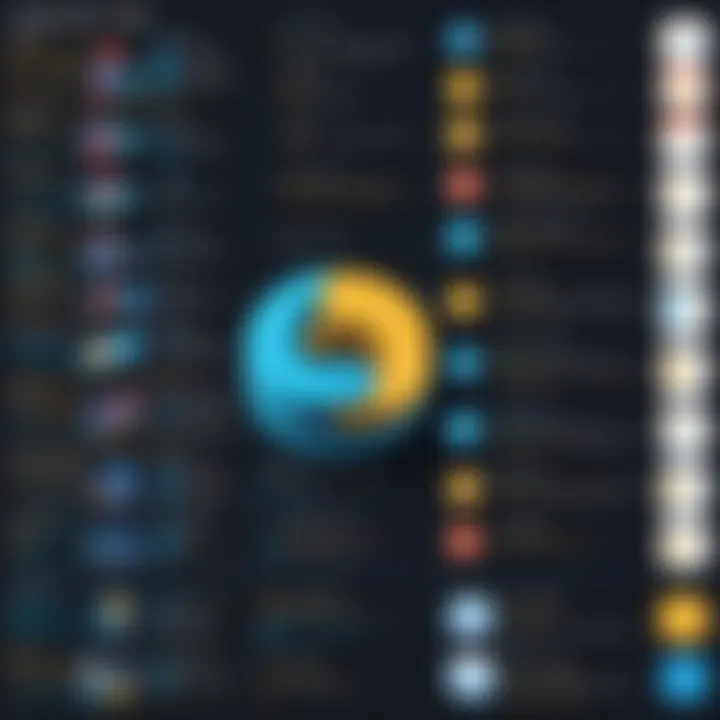
Strings are sequences of characters enclosed in quotes. They can be used extensively for representing text. Proper string formatting can dramatically improve code readability and ease of debugging. Python provides several ways to format strings, which allows developers to create dynamic and user-friendly outputs.
- Using f-strings (formatted string literals) which are prefixed with . Example:
- The method allows for more advanced formatting. Example:
- Percentage Formatting, an older method still used, looks like this:
Choosing the right string formatting method often depends on your needs. If you want readable and maintainable code, f-strings are generally the most efficient choice.
Boolean Values and Expressions
Boolean values represent one of two states: or . They form the backbone of logic operations within Python. Booleans are widely used in conditional statements, helping to control the flow of execution based on conditions specified in the code.
Here are some common aspects of boolean usage in Python:
- They result from comparisons, such as , which evaluates to .
- Logical operations combine boolean values. The , , and keywords are used to perform these operations. For example:
result = a and b# result will be False
This code snippet succinctly captures the essence of decision-making in programming. The clarity and simplicity of conditional statements contribute to Python's reputation for being beginner-friendly. Each option is readily apparent, and as codebases grow, these decisions help in maintaining clarity.
Looping Constructs
Looping constructs allow for the repetition of a particular block of code until a specified condition is met. This is incredibly useful in situations where tasks need to be performed multiple times, such as iterating through items in a list or processing data in batches. Python provides two primary types of loops: loops and loops.
for Loops
For loops are particularly favored for their ability to iterate over sequences like lists, tuples, and strings. They offer a straightforward and clean syntax that many developers appreciate. The following example demonstrates how a for loop elegantly prints each fruit in a list:
The key characteristic of for loops is their iteration over a definite sequence, making them a preferred choice when the number of iterations is known ahead of time. One might argue that their clarity and simplicity outweigh the alternatives, making for loops a common go-to for Python developers.
However, one downside to consider is that for loops can be less flexible when dealing with dynamically changing data sets, as they rely on the predefined sequence.
while Loops
While loops, on the other hand, are driven by a condition rather than a sequence. This means they will continue to execute as long as a certain condition evaluates to true. This can be particularly powerful for scenarios where the number of iterations isnât known in advance.
For example, the following while loop continues until a counter variable reaches a specified number:
The primary trait of while loops is that they are more flexible but can lead to issues if the condition never becomes false, resulting in infinite loopsâa common pitfall for novice programmers. Their ability to handle variable situations, however, often makes them indispensable in more complex algorithms and data processing tasks.
In summary, understanding control flow syntax in Python is not just about writing code that works; it's about crafting programs that are logical, efficient, and adaptable to the varying needs of users and systems. Leveraging conditional statements and looping constructs effectively enhances the performance and readability of code, contributing to a more streamlined development process.
Function Definitions
Function definitions are a cornerstone of Python programming. Essentially, they allow developers to create reusable pieces of code that can be called upon multiple times throughout a program. This not only promotes code reuse but also enhances organization and clarity. By encapsulating specific functionality within functions, programmers can keep their codebase cleaner and easier to navigate. Additionally, function definitions help prevent repetition, promoting the DRY (Don't Repeat Yourself) principle which is key in maintaining efficient code.
When discussing function definitions, a few key elements come into play:
- Function Name: The name should be descriptive. It should give a clear idea of the functionâs role. Following a consistent naming convention enhances readability.
- Parameters and Arguments: These are inputs to functions. Parameters are the variables listed in the function definition, and arguments are the actual values passed to that function when called.
- Return Statement: A function can return a value using the return statement, allowing function outputs to be used elsewhere in the program.
All these components together enable the powerful abstraction of functionality which makes Python a favorite among software developers, IT professionals, and data scientists alike.
Defining Functions
To define a function in Python, one uses the keyword, followed by the function name and parentheses which may include parameters. Below is a simple example of defining a function:
In this snippet, we've created a function called which takes a single parameter, . When called, it outputs a greeting message.
The structure is straightforward:
- introduces the function.
- Followed by a name (which ideally should be indicative of its purpose).
- Parentheses that house any parameters.
- Finally, a colon that indicates the start of the function body.
The body of the function, which is indented, contains the code that runs when the function is invoked. Itâs worth noting that Python relies heavily on indentation, and this must be adhered to strictly to avoid syntax errors.
Function Arguments and Return Values
Function arguments are essentially the inputs to functions, and they play a pivotal role in how functions operate. When defining a function, the parameters specify what inputs the function expects. But when the function is called, values are passed as arguments.
Consider this further example:
Here, the function accepts two arguments, and . It utilizes the statement to output their sum. Notably, the function can be called like this:
This will assign the value to as the outcome of our function. Each function can return only one value, but you can return multiple items as a tuple if needed. For instance:
In this case, calling will yield .
Understanding how to properly define functions and manage their arguments and return values can greatly enhance your coding prowess. It allows for greater flexibility and optimization in programs, proving crucial in making your Python projects more manageable and efficient. Writing functions with clear inputs and outputs not only boosts the code clarity but also its maintainability, thus echoing the significance of well-thought-out function definitions in Python.
Comments and Documentation
When delving into Python programming, comments and documentation serve as crucial tools that enrich code quality and maintainability. They not only aid developers in understanding the intent behind code but also facilitate collaboration in team environments. Code is often read more than it is written, so having well-placed comments can make a significant difference. Without them, itâs easy to get lost in the intricacies of logic, especially when revisiting old projects or working alongside peers who might not be familiar with your coding style. Therefore, taking the time to document your code adequately saves everyone from scratching their heads later.
Single-Line Comments
Single-line comments are like the convos you have with a friend while working through a problem. They allow you to add short notes to clarify or explain a segment of codeâa simple reminder or a flag for yourself or others later on. In Python, a single-line comment is initiated with a hash symbol (# ).
For example:
In this snippet, the single-line comment right above the function definition hints at its purpose without bogging down the reader with extraneous text. However, it's worth noting that overusing comments can lead to clutter, making the code harder to read. A good rule of thumb is to comment on why something is done rather than what is being done, as the latter seems obvious from the code itself.
Multi-Line Comments
Multi-line comments come into play when you need to express a more elaborate thought or provide details about a chunk of code. Python uses triple quotes or triple single quotes for this purpose, which also serves as docstrings if placed right under a function or class definition. Utilizing multi-line comments effectively allows for clear, organized documentation.
For instance:
In this example, the multi-line comment gives a detailed overview of what the function does, why itâs structured that way, and what to expect when it runs. In a professional setting, quality comments often become the glue that holds teams together, as they minimize misunderstandings and enable a smoother onboarding process for new team members.
Comments and documentation are not just nice-to-have features; they're essential components that contribute to the overall health of a coding project.
Keeping in mind these best practices around comments and documentation enhances readability and ultimately, the effectiveness of your code. Always aim for clarity and conciseness, and youâll create a codebase that speaks volumes, even in silence.
Multi-Dimensional Structures
In the realm of Python programming, multi-dimensional structures are essential. They allow developers to organize and manipulate data effectively, which can be especially crucial when tackling complex data sets. Whether it's lists, tuples, dictionaries, or sets, each of these structures has unique properties that serve different purposes but often complement each other.
Using multi-dimensional data structures helps manage relationships between data points. For instance, organizing student data in a classroom setting can include student names, grades, and attendance records in a list of dictionaries. This approach enhances the legibility of your code and allows for complex data representations without foraying into unnecessary confusion.
Itâs equally important to consider the mutability of these structures when electing which to utilize. While lists are mutable and allow for modifications, tuples are immutable. This feature affects how data is handled and could impact performance in larger applications.
Lists and Tuples
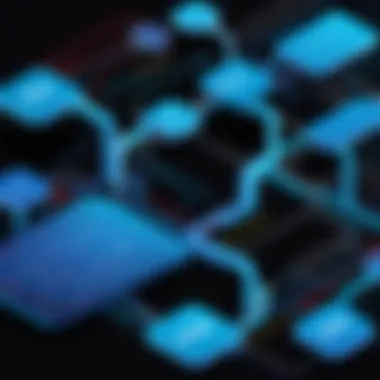
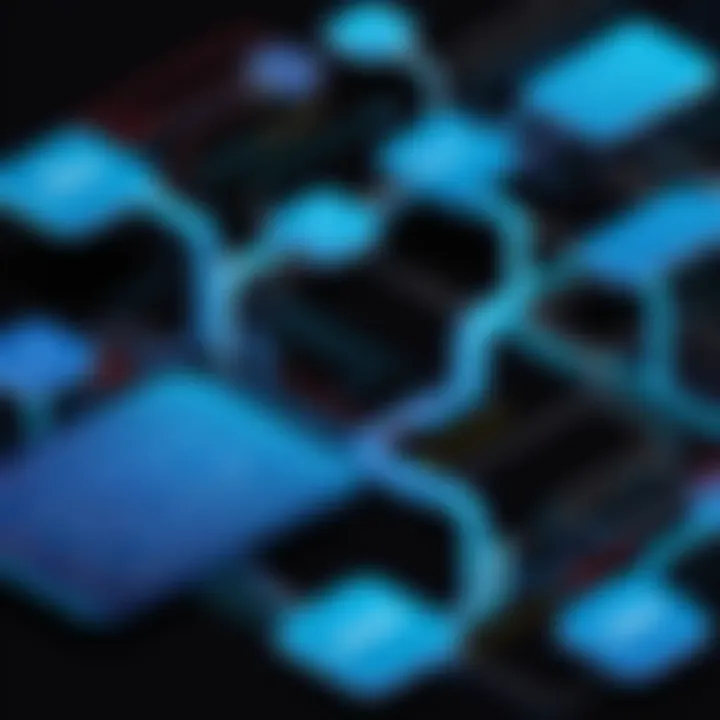
Let's start with lists and tuples, as they are the building blocks for sequential data handling in Python.
- Lists: Lists are used when you need a collection of items. They can hold multiple data types and can be modified afterwards. You can add, remove, or change items pretty easily. The versatility of lists makes them a favorite for many developers.
students.append("David")# Adds David to the list
The choice between lists and tuples often revolves around the need for safety versus flexibility. If your data should stay constant, tuples are the way to go. If you expect to make changes, then lists suit better.
Dictionaries and Sets
Next up are dictionaries and sets, which bring a unique flair to how we can process and store data.
- Dictionaries: These are collection of key-value pairs. Each key is unique and is used to access its corresponding value. This is particularly useful for scenarios where you want to associate pieces of data with unique identifiers. A classic example is using a dictionary for user profiles where usernames can lead you to their data.
print(user_profile["age"])# Outputs: 20
In summary, multi-dimensional structures like lists, tuples, dictionaries, and sets are intimately woven into the fabric of Python programming. Understanding their properties and appropriate applications allows you to write cleaner, more effective code. These structures not only make data management easier but also enhance the clarity and ingenuity of your programming solutions.
"The right choice of data structure can often mean the difference between clear and obscured logic in code."
By mastering these basic structures, developers position themselves well for the more intricate aspects of Python programming, paving the way for handling larger datasets and implementing advanced algorithms with ease.
Error Handling Syntax
Error handling is an essential factor when programming in Python. Without solid error management, even the smallest error can crash an entire application. Proper error handling adds a layer of reliability that is crucial not just for the software's functionality but also for providing users a smoother experience. Here, we'll explore two primary elements of error handling: Try and Except Blocks and Finally Clauses. Understanding these elements allows developers to anticipate issues, manage errors gracefully, and maintain control over their applicationâs flow even in dire situations.
Try and Except Blocks
A Try and Except Block is a fundamental building block in Pythonâs error-handling structure. It allows you to write code that might produce an error in a controlled way. The idea is straightforward; you place the code that might fail inside the try block, and if an error occurs, it jumps to the except block instead of crashing the program.
Consider this simple example:
In this snippet, trying to divide by zero raises a . The except block catches that specific error, allowing us to handle it gracefully rather than letting the program crash. This approach enhances both the user experience and the robustness of the code.
The benefits of using try and except blocks include:
- Graceful Degradation: Users get meaningful feedback instead of a program crash.
- Error Specificity: You can catch different types of errors and respond accordingly.
- Code Clarity: It makes the code clean and easier to maintain by isolating error-prone code.
However, rely on these blocks judiciously. Overusing them or catching overly broad exceptions can lead to hiding problems instead of solving them.
Finally Clauses
In many situations, you may need to execute final cleanup actions whether an error has occurred or not. That is where Finally Clauses come into play. A finally block follows the try and except and runs regardless of whether an error was raised. It's perfect for actions like closing files or releasing resources, ensuring that they always complete.
Hereâs an illustrative example:
In this case, if doesn't exist, Python prints a file not found message. Regardless of the outcome, the finally block ensures the file is closedâif it was opened in the first place. This practice not only keeps resources in check but also reflects good coding discipline.
To summarize, proper error handling in Python using try and except blocks coupled with finally clauses strengthens your programming. It promotes a culture of anticipating issues, creating a better coding environment. Since software often operates in real-time with users, these tools become invaluable in ensuring a smooth operation.
A well-designed error-handling strategy is like an insurance policy; you hope you never need it, but it's critical when something goes awry.
Modules and Packages
Modules and packages serve as the backbone of Python programming, allowing developers to build complex applications by organizing code into manageable segments. Understanding modules and packages not only enhances code organization but also promotes reusability and maintainability.
When working on a project, the ability to compartmentalize code into modules allows for cleaner and easier navigation. Each module can focus on a specific functionality, and by using packages, these modules can be grouped effectively under a unified namespace. This method of structuring code is paramount for larger projects, ensuring that different parts donât interfere with each other while keeping the codebase clear and coherent.
Here are some key benefits of utilizing modules and packages:
- Reusability: Once a module is created, it can be easily imported and used across different projects without needing to rewrite the code.
- Namespace Management: By using packages, itâs possible to avoid naming conflicts among modules, thus allowing for more scalable code development.
- Simplified Testing: Isolating code into modules allows for more straightforward testing and debugging processes.
The consideration when dealing with modules and packages includes ensuring that dependencies are explicitly managed, so when others or you revisit your code later, everything runs smoothly. Moreover, understanding the difference between a module and a package is crucial. A module is simply a file containing Python code, while a package is a directory that holds multiple modules and a special file.
Import Statements
Import statements are foundational in Python as they enable the use of existing modules and packages, facilitating code reuse across various applications. The import statement allows you to tap into Python's standard library and third-party libraries, leading to increased productivity.
You can employ several forms of the statement:
- To import an entire module:This method imports the entire module, allowing you to access its functions using the module name, e.g., .
- To import specific functions from a module:With this approach, you can call directly without needing to prepend the module name.
- To alias a module for convenience:Using an alias makes it easier to reference modules with long names throughout your code.
Keep in mind, each import statement can impact performance, so itâs wise to only load what you need. Being strategic with imports will help keep your application lightweight.
Creating Custom Modules
Creating custom modules is an empowering practice in Python development. It enables you to encapsulate functionality into standalone pieces, which can be easily distributed and reused. Building a custom module involves simply saving your Python code in a file.
For example, if you're creating a module for arithmetic operations, you might write:
Now you can import this module in your main application file:
Key steps in creating custom modules:
- Think about the functionalities your module will provide.
- Name your module logically based on what it does.
- Use meaningful functions and comment your code for clarity.
By following these principles, youâll develop modules that facilitate collaboration, ease maintenance, and enhance overall productivity in your programming journey.
"In Python, it is the names of directories and files that serve as namespaces; using modules helps in organizing these names and avoiding conflicts" - Python Community.
Additionally, for further information, refer to the Official Python Documentation. There, you will find guidance on module creation, usage, and best practices to keep your coding on the path to success.
Advanced Syntax Elements
In the world of Python programming, advanced syntax elements stand as powerful tools that can streamline workflows, enhance code readability, and improve overall efficiency. Understanding these elements not only allows a programmer to harness the full capabilities of Python, but also helps in crafting elegant solutions to complex problems. This section delves into two paramount components: list comprehensions and lambda functions, both of which enrich the syntax landscape of Python with their unique functionality.
List Comprehensions
List comprehensions represent a compact and expressive way to create lists. Instead of deploying a traditional loop to append items, you can condense the operation into a single readable line. This not only minimizes code clutter, but also boosts performance.
For instance:
In this example, will contain the squares of numbers from 0 to 9. The magic happens in the concise expression, which accomplishes the task in a fraction of the time it would take using standard loops.
Benefits of using list comprehensions:
- Readability: They're often clearer than traditional for-loops, especially for those familiar with the syntax.
- Performance: Due to their optimized implementation, list comprehensions can be faster than an equivalent for-loop.
- Less Code: Fewer lines result in easier maintenance and less room for bugs.
However, caution is advised. Over-complicated list comprehensions can lead to confusion, particularly for those not well-versed in this syntax. Striking the right balance between brevity and clarity is key. Maximizing benefits while minimizing potential pitfalls should be a priority.
Lambda Functions
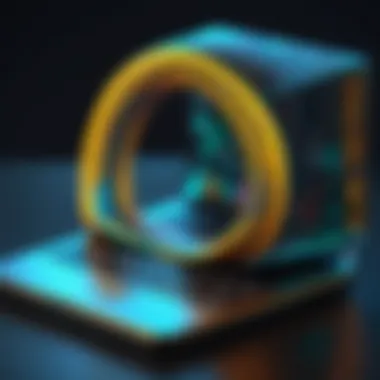
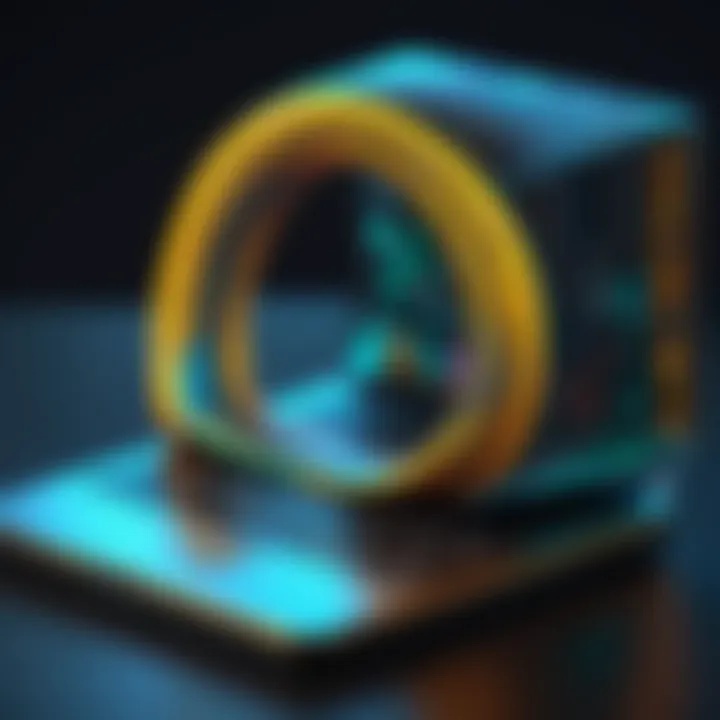
Lambda functions are another sophisticated feature of Python, often referred to as anonymous functions due to their lack of explicitly defined names. They allow you to define small, throwaway functions on the fly without the need for a full function declaration.
An example might clarify this:
Each lambda function can take any number of arguments but can only have one expression. When that expression is executed, it returns a value. This can be particularly useful in contexts requiring a quick function definition, such as sorting or filtering data.
Consider the use of a lambda function with the function:
In this case, will contain .
While lambda functions synchronize seamlessly with functional programming styles, it's important to know when to utilize them effectively. Pros include:
- Simplicity: Perfect for short, single-use functions.
- Conciseness: Cuts down on boilerplate when you need a quick function.
In contrast, they can sometimes lead to less readable code, particularly if used excessively or with complexity that obscures understanding. For most common uses, however, their appeal is strong.
"Simplicity is the ultimate sophistication." â Leonardo da Vinci
Expanding one's command over these advanced syntax elements will undeniably enhance a programmer's toolkit.
Best Practices in Python Syntax
Adhering to best practices in Python syntax is akin to following a recipe when crafting a culinary masterpiece. Just like the right ingredients yield a delightful dish, proper syntax ensures that code is not only functional but also clear and maintainable. The significance of these practices stretches beyond personal preference; they contribute directly to the overall readability and efficiency of the code, making it easier for othersâ and future youâto understand. Introducing a bit of structure ensures that developers can collaborate seamlessly, reducing the time needed for debugging and enhancing productivity.
Consistent Naming Conventions
Consistency is key when it comes to naming in Python. Think about how a bookâs index makes it easier to find chapters. Similarly, clearly defined names help programmers quickly locate and understand code functions. When you employ consistent naming conventions, it even helps to create a personal style that others can quickly recognize.
In Python, the convention for naming variables and functions often leans toward the use of lower_case_with_underscores. This convention underscores clarity and minimizes confusion.
Here are a few tips on maintaining a consistent naming convention:
- Use descriptive names: Clear variable names enhance understanding. Instead of naming a variable , use or .
- Avoid single-letter variables: Except in specific contexts, like loop counters, single-letter variables can be vague and uninformative.
- Be mindful of global and local scopes: Clearly distinguish between them to help grasp context quickly.
By adhering to consistent naming conventions, not only do you improve code readability but you also set a solid foundation for maintainable software.
Code Readability and Maintenance
Code readability is a vital aspect that significantly influences maintainability. Just as a well-structured essay flows logically from one point to another, code that rightly follows formatting and structural practices is easy to navigate. With the landscape of technology continually evolving, maintaining a codebase should be effortless.
Consider these elements to foster readability in your Python scripts:
- Use Proper Indentation: Python is indentation-sensitive, and proper indentation creates a clear visual structure. For instance:In this code, the indentation signals that is part of the function.
- Comment Wisely: Comments should clarify, not clutter. A well-placed comment can illuminate the purpose behind a complex block of logic.
- Limit Line Length: Aim for lines that don't exceed 79 characters. This is a convention that allows code to fit neatly on screen and helps in side-by-side comparisons.
The goal is to make the code self-explanatory to an extent, thus facilitating smooth transitions for anyone delving into it at a later date.
"Code is like humor. When you have to explain it, itâs bad." - Cory House
By following these guidelinesâmixing clarity, consistency, and community conventionsâPython developers can sustain a healthy codebase that will stand the test of time. Make it easy on yourself and your collaborators: read and maintain with ease!
Common Pitfalls in Python Syntax
Understanding common pitfalls in Python syntax is crucial for both beginners and experienced programmers. These pitfalls often arise from a misunderstanding of the language's rules or from overlooking certain syntactical nuances. Recognizing and addressing these issues can significantly improve the overall quality of your code. Failing to grasp these aspects may lead to unexpected behavior, bugs, or inefficient code. Through careful attention to common syntax errors and indentation misunderstandings, programmers can enhance readability and robustness in their projects.
Common Syntax Errors
Python, while being known for its readability, can still trip up developers with common syntax errors. These errors can stem from simple typographical mistakes to more complex issues related to the structure of code. Here are a few noteworthy examples:
- Missing Colons: Often, developers forget to include colons at the end of control statements like , , and . Missing a colon can halt your code execution before it even begins.
if x > 10# This will raise a syntax error print("X is greater than 10")
Each of these errors can cause unnecessary frustration, but they can be easily avoided by taking a moment to review code and utilizing good programming practices. Catching them early saves time and ensures smooth sailing down the road.
Misunderstandings with Indentation
Indentation in Python is not merely a stylistic choice; it's a fundamental part of the language's syntax. Unlike many other languages that use braces or keywords to define code blocks, Python relies on indentation to denote the structure. This can lead to confusion, especially for those new to programming or transitioning from other languages.
Key points to consider include:
- Consistency is Key: Mixed tab and space usage can lead to . Itâs imperative to choose one method and stick with it throughout your code. Most communities suggest using spaces due to their compatibility across various editors and systems.
- Visual Clarity: Good indentation enhances the readability of code. It makes it easier for someone else (or future you) to follow the program logic. Each level of indentation typically indicates a new block of code, forming a visual hierarchy.
- Common Errors: Forgetting to indent a line of code inside a loop or function will lead to unexpected output or errors. The line won't be recognized as part of the block unless it's indented correctly.
- Nesting Indentation: When working inside nested structures, keep an eye on your indentation. As you add layers of complexity, the risk of misalignment increases, which could upset program flow.
"Mistakes are a portal of discovery." â James Joyce
In summary, attention to detail in these areas can prevent many of the headaches that novices and even seasoned developers encounter. Developing a disciplined approach towards avoiding syntax errors and mastering indentation will pave the way for better Python programming and a more enjoyable coding experience.
Resources for Further Learning
In the realm of programming, continuous education is the key to staying relevant and effective. Having a solid set of resources at your fingertips can make a world of difference when mastering the intricacies of Python syntax. By tapping into high-quality materials, developersâwhether theyâre fresh faces or seasoned handsâcan deepen their understanding and keep their skills sharp. This section explores the best resources available to augment your learning experience in Python.
Official Python Documentation
The official Python documentation stands as the gold standard for learning and reference. It encapsulates everything about Python, from installation to advanced features. Whether you want to trace the historical development of a specific syntax or need code snippets for practical utilization, the documentation provides a comprehensive overview.
Here are some key benefits of using the official documentation:
- Detailed Explanations: Concepts are explained with considerable clarity, leaving no stone unturned.
- Example-driven Learning: Most sections include code examples, invaluable for grasping how theories translate into practice.
- Up-to-date Information: Since the documentation is managed by the Python Software Foundation, it reflects the most current best practices and updates.
As cited on Pythonâs official website,
"The documentation is there to help you. Always refer back to it when confused or unsure."
You can access this treasure trove at Python.org.
Recommended Online Courses
Online courses have gained tremendous popularity as they facilitate learning in a structured manner. A well-crafted course can lay out complex topics in manageable portions, often with interactive elements that enhance engagement. Here are some reputable platforms that offer valuable Python courses:
- Coursera: Offers a range of courses from basic to advanced, often featuring instructors from renowned universities.
- edX: Similar to Coursera, it partners with top educational institutions to provide courses on various topics.
- Udemy: Known for its vast selection, Udemy allows course creators to provide affordable, flexible learning options on specific topics.
- Pluralsight: Best known for catering to tech enthusiasts, this platform offers exhaustive content in programming, focusing on practical skills.
When choosing a course, consider a few key elements:
- Instructor Credibility: Look for courses taught by experienced professionals.
- Course Reviews: Feedback from past learners can guide your selection process.
- Hands-on Projects: Ensure the course includes practical assignments to cement your knowledge.
Epilogue and Future Directions
In wrapping up our comprehensive look at Python syntax, itâs essential to reflect on the journey we've taken through its core components and how they influence effective programming. Understanding the evolution of syntax is not just an academic exercise; it plays a significant role in how developers write code today and anticipates how they will write it tomorrow.
The Evolution of Python Syntax
Python has come a long way since its inception in the late 1980s. Originally designed as a language that emphasized code readability and simplicity, Pythonâs syntax has grown and adapted over the years to accommodate the needs of its users. The transition from version 2 to version 3 was monumental, introducing changes that rendered many older practices obsolete. This provides a clear reminder for developers: staying updated with syntax evolution is crucial.
- List comprehensions and generator expressions are prime examples of how Python syntax has evolved to become more concise and expressive, enabling developers to accomplish more with lesser lines of code.
- The introduction of type hints in Python 3.5 is another significant change. This feature allows for better code analysis and aids in debugging, as it explicitly indicates what type of arguments a function expects or what type it will return.
Understanding these evolutionary milestones helps developers appreciate the rationales behind certain syntax structures and prepares them for future updates. According to many industry experts, programming languages, including Python, are moving towards more expressiveness and clarity, promoting maintenance as a first-class concern.
Embracing New Features
With Python's steady evolution, embracing new features is not just beneficial but essential for any serious developer. These features not only enhance functionality but often address issues of performance and usability.
For instance, Python's introduction of asynchronous programming through the and keywords has revolutionized how developers write concurrent code, making it easier to handle I/O-bound tasks more efficiently.
Consider the following:
- Pattern matching, introduced in Python 3.10, enables more intuitive ways to handle complex data structures, thus improving code clarity and reducing potential errors.
- The tools and libraries associated with Python, like , have also seen syntactical enhancements that make data manipulation far easier and more readable than before.
Staying abreast of new additions not only enhances a developerâs toolkit but also opens doors to better performance and efficiency in code. By understanding and utilizing the latest features, developers can write cleaner, faster, and more maintainable code.
Ultimately, the commitment to mastering syntax is both a process of continual learning and adaptation. As Python continues to evolve, developers must remain proactive, ensuring they do not fall behind the curve. The growth of Python doesn't just reflect the language itself, but the dynamic landscape of programming as a whole. This ever-changing framework underlines the necessity of constantly revisiting the syntax to harness its full potential.
"To keep up with the evolving landscape of programming, one must be willing to learn continually. Python's syntax evolution is a perfect example of how adaptation leads to mastery."