Unlocking the Depths of Python Code: A Comprehensive Exploration
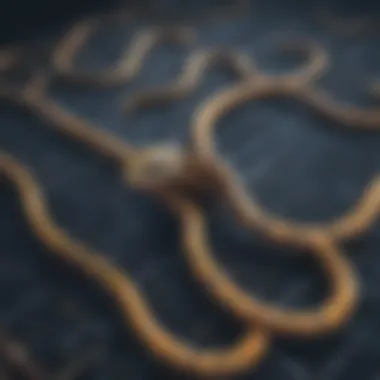
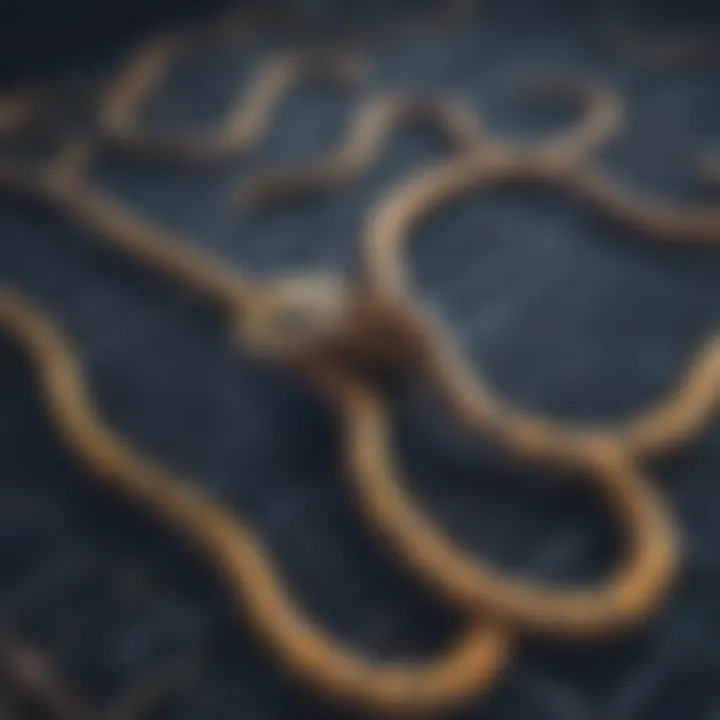
How-To Guides and Tutorials for Python
For beginners and experienced developers seeking to enhance their Python skills, detailed guides and tutorials offer valuable insights. Let's explore some practical tips and tricks:
- Step-by-Step Guides: Starting with setting up a Python development environment, understanding data structures, and progressing to advanced topics like decorators and generator functions, step-by-step guides cater to learners at various proficiency levels.
- Hands-On Tutorials: Interactive tutorials on web scraping with Beautiful Soup, building RESTful APIs with Flask, and implementing machine learning models with scikit-learn provide hands-on experience in real-world Python applications. Practical examples and coding exercises reinforce learning outcomes.
- Tips for Effective Utilization: Leveraging Jupyter notebooks for interactive data analysis, exploring Python libraries for data visualization, and optimizing code performance with profiling tools are essential tips for effective utilization of Python in diverse projects. Embracing Pythonic idioms and patterns enhances code clarity and functionality.
Introduction to Python Code
Python is a versatile programming language that has garnered significant attention in the tech community for its simplicity and flexibility. In this section of the article, we set the stage, introducing readers to the fundamental aspects of Python code. Understanding the syntax and structure allows developers to grasp the essence of Python's power and elegance. Exploring Python code lays a strong foundation for tackling more complex topics in the subsequent sections.
What is Python Code?
Definition and Purpose of Python Code
Python code serves as a medium for developers to communicate with computers effectively. Its readability and concise syntax make it a preferred choice for projects ranging from simple scripts to large-scale applications. Python's versatility enables programmers to write code for various purposes efficiently. This section will delve into the specifics of how Python code functions, outlining its role in the programming landscape.
Characteristics of Python Programming Language
The characteristics of Python set it apart from other programming languages. Its dynamic typing and easy-to-understand syntax contribute to its popularity among beginners and experienced programmers alike. Python's extensive standard library and community support enhance its utility for a wide range of applications. Understanding these characteristics provides insight into why Python is a go-to language for tasks such as web development, data analysis, and automation.
History and Evolution
Origins of Python Language
Python's origins trace back to the late 1980s when Guido van Rossum developed the language as a hobby project. Its emphasis on readability and clear coding practices attracted early adopters seeking an alternative to existing languages. Exploring the roots of Python sheds light on its design principles and the philosophy that guides its development.
Milestones and Versions
Python's journey has been marked by significant milestones, with each version introducing new features and improvements. The evolution of Python reflects the dynamic nature of programming languages and the community's commitment to enhancing the language's capabilities. Tracking the milestones and versions provides a historical perspective on Python's growth and adaptation to changing trends in technology.
Basics of Python Code
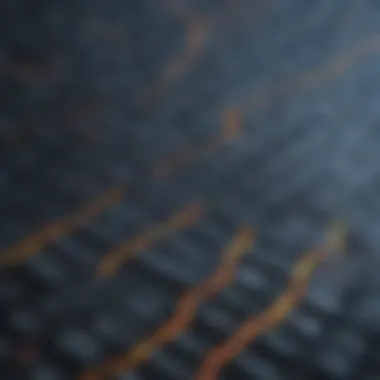
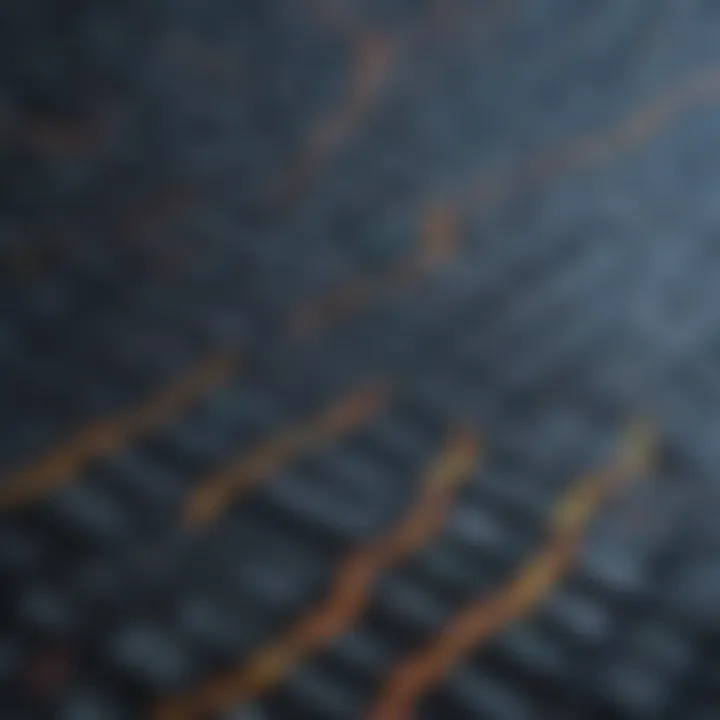
In this section of the comprehensive guide to understanding Python code, we delve into the fundamental building blocks that form the backbone of any Python program. The Basics of Python Code are essential for both novice programmers and seasoned developers, as they lay the groundwork for mastering more advanced concepts. Understanding the syntax, structure, and principles of Python is crucial for writing efficient, clear, and functional code that can achieve various tasks and solve complex problems. Without a firm grasp of the basics, programmers may struggle to progress and harness the full power of Python's capabilities.
Syntax and Structure
Indentation and Formatting Rules
Indentation and formatting rules in Python play a pivotal role in determining the structure and readability of code. Unlike many other programming languages that use braces or keywords to indicate blocks of code, Python relies on indentation. The consistent use of indentation not only enforces clarity but also serves as a visual guide for understanding the logical flow of the program. By requiring developers to adhere to strict indentation rules, Python promotes well-organized and visually appealing code, making it easier to identify errors and maintain codebases effectively.
Variables, Data Types, Operators
Variables, data types, and operators form the backbone of Python programming, allowing developers to store and manipulate data efficiently. Understanding how variables work, the different data types available (such as integers, floats, strings, lists, and dictionaries), and how operators can be used to perform operations on these data types is essential for competent Python programming. Variables provide a means of storing information, data types define the kind of values that can be used, and operators enable actions to be performed on these values. Mastering these foundational concepts lays the groundwork for more complex computations and algorithms in Python programming.
Control Flow
Conditional Statements
Conditional statements are integral to Python programming, as they allow developers to create branches in the code based on specified conditions. By using keywords like 'if,' 'elif,' and 'else,' programmers can control the flow of their programs, executing different blocks of code depending on whether certain conditions are met. Conditional statements enable developers to build interactive and responsive applications, handle edge cases, and make decisions within their code, enhancing the flexibility and functionality of Python programs.
Loops and Iterations
Loops and iterations are essential for performing repetitive tasks and processing collections of data in Python. By using constructs like 'for' and 'while' loops, developers can iterate over sequences, such as lists or strings, to perform operations on each element consistently. Loops offer efficiency by reducing code duplication and enabling dynamic data manipulation, while iterations provide a systematic way to access and process elements within data structures. Mastery of loops and iterations is crucial for automating tasks, processing large datasets, and implementing algorithmic logic in Python programs.
Functions and Modules
Defining Functions
In Python, functions play a crucial role in structuring code, encapsulating logic, and promoting reusability. By defining functions, developers can modularize their programs, breaking down complex tasks into smaller, manageable units. Functions accept inputs, perform specific operations, and return outputs, allowing for efficient code organization and maintenance. The ability to define custom functions tailored to specific requirements enhances code readability, promotes code reuse, and simplifies debugging and refactoring processes in Python development.
Importing and Using Modules
Python's modular nature is one of its key strengths, enabling developers to extend the language's functionality by importing and utilizing external modules. Modules are collections of functions, classes, and variables that can be imported into a Python program to leverage pre-written code and enhance functionality. By importing modules, developers can access libraries for tasks like mathematical operations, file handling, networking, and more, without having to reinvent the wheel. This modular approach promotes code scalability, encourages code reuse, and fosters collaboration within the Python community, making it easier to build robust and feature-rich applications.
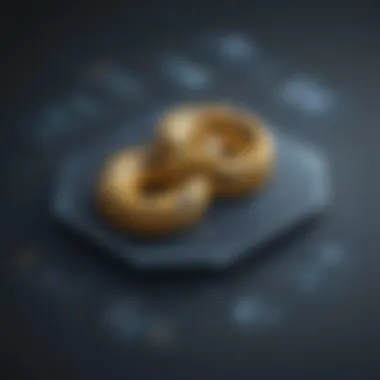
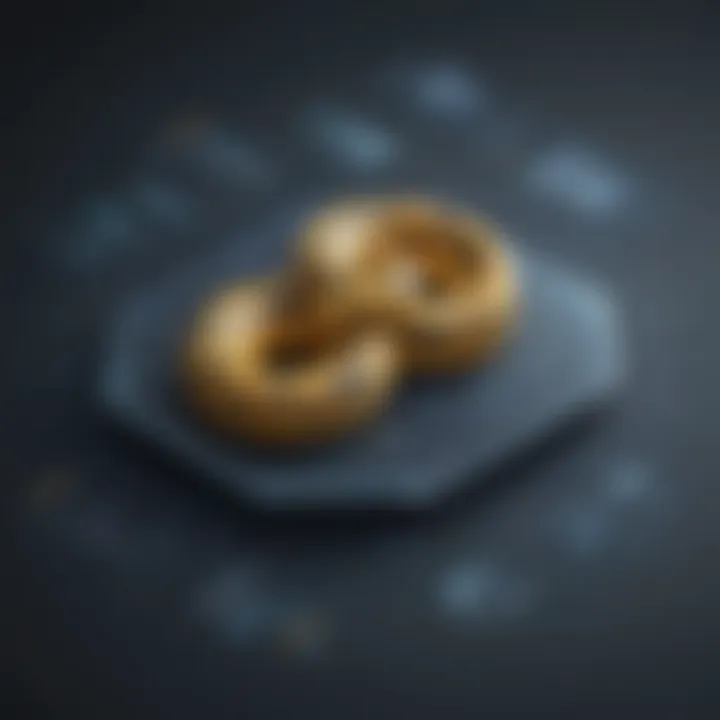
Advanced Concepts in Python
When delving into the intricate world of Python programming, understanding advanced concepts is paramount to mastering the language. In this section, we will explore some of the essential elements that elevate Python to a powerful and versatile tool in software development. From Object-Oriented Programming to Exception Handling and File Handling, these advanced topics provide developers with the expertise to create robust and scalable applications.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a key paradigm in Python that facilitates code reusability and organization. Classes and Objects lie at the core of OOP, allowing developers to structure code efficiently. Classes act as blueprints for creating objects, encapsulating data and methods within a single entity. Objects, on the other hand, are instances of classes, representing tangible entities in the program. The key advantage of Classes and Objects is their ability to promote modularity and abstraction, leading to more maintainable code bases.
Inheritance and Polymorphism further enhance the flexibility of OOP in Python. Inheritance enables the creation of new classes based on existing ones, fostering code reuse and extension. Polymorphism, on the other hand, allows objects of different classes to be treated uniformly, promoting code clarity and flexibility. However, while Inheritance and Polymorphism enhance code structure, they can also introduce complexities if overused or misapplied, requiring developers to strike a balance in their usage.
Exception Handling
Exception Handling is crucial in ensuring the robustness and reliability of Python code. Try-Except Blocks provide a mechanism to anticipate and manage errors gracefully during program execution. By enclosing potentially error-prone code within Try-Except Blocks, developers can prevent abrupt program termination and provide alternative pathways for error resolution. This proactive approach to error handling enhances the user experience and reinforces the stability of Python applications.
Handling Errors Gracefully complements Try-Except Blocks by defining explicit strategies for addressing specific error scenarios. By incorporating targeted error-handling mechanisms, developers can accurately diagnose and resolve issues, improving the fault tolerance of their programs. However, excessive error handling can lead to code bloat and diminish readability, underscoring the importance of striking a balance between thoroughness and conciseness.
File Handling
File Handling operations are integral to interacting with external data sources and persisting information in Python. Reading and Writing Files enable the retrieval and storage of data from and to files, facilitating seamless data manipulation. By mastering these operations, developers can integrate file-based functionalities into their applications, expanding the scope of their projects.
Working with File Streams provides a streamlined approach to handling file operations, offering efficient methods for data transmission and manipulation. File Streams enhance the performance of file-related tasks by optimizing resource utilization and minimizing data processing overhead. However, improper management of file streams can lead to resource leaks and potential security vulnerabilities, necessitating diligent oversight during file handling operations.
Applications of Python Code
Python is exceedingly versatile, finding application across varied domains, making it an indispensable tool. In this comprehensive guide, the relevance of Python applications will be thoroughly explored, shedding light on its significance in modern programming landscapes.
Web Development (250- words)
Frameworks like Django and Flask
Frameworks such as Django and Flask play a pivotal role in web development, offering streamlined solutions for building robust web applications. Django, known for its high-level design patterns, emphasizes rapid development, enabling developers to create feature-rich websites efficiently. Conversely, Flask's lightweight nature provides flexibility, making it a favorable choice for smaller projects or prototypes. While Django thrives on its all-inclusive approach, Flask excels in its simplicity, catering to developers seeking minimalistic frameworks.
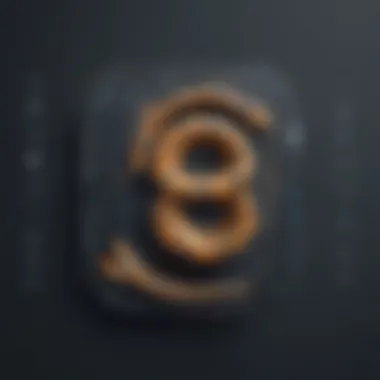
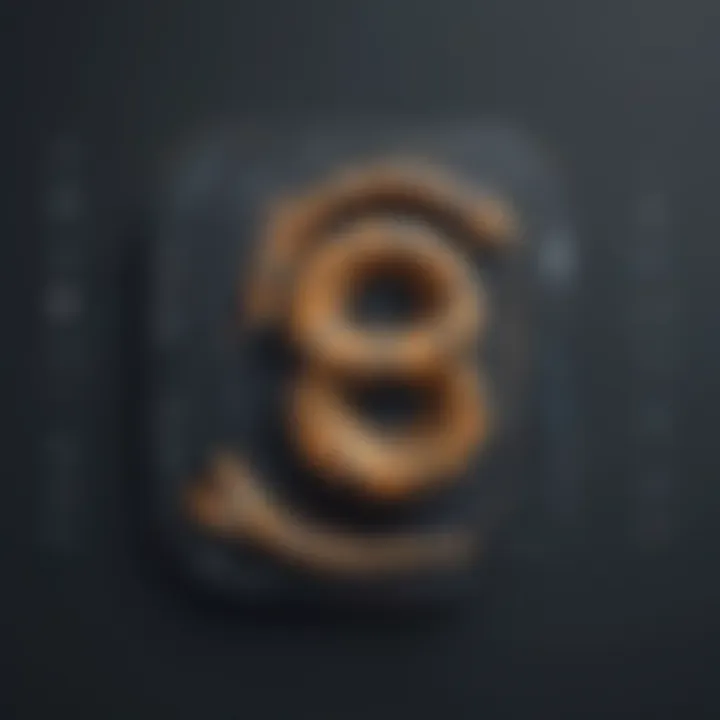
Server-side Scripting
Server-side scripting is paramount in web development, allowing dynamic generation of web pages tailored to user interactions. Python's server-side scripting capabilities empower developers to create interactive and personalized web experiences. By processing requests on the server, Python enhances web application performance, exhibiting reliability and scalability. Although server-side scripting requires server resources, Python's efficiency minimizes resource consumption, ensuring optimal website operation.
Data Science and Machine Learning (250- words)
Libraries such as NumPy and Pandas
NumPy and Pandas are essential libraries in data science, facilitating efficient data manipulation and analysis. NumPy's array operations and mathematical functions expedite complex calculations, crucial for scientific computing tasks. Conversely, Pandas simplifies data structuring and analysis through its DataFrame object, enabling swift data handling and transformation. Together, these libraries bolster Python's capabilities in data science, enabling data professionals to derive valuable insights efficiently.
Building Models
Python's prowess in machine learning manifests through model building, leveraging libraries like scikit-learn for diverse ML algorithms. Building ML models in Python is intuitive and accessible, with scikit-learn offering a comprehensive suite of tools for model training and evaluation. Python's extensive ML ecosystem fosters innovation in model development and deployment, accelerating advancements in artificial intelligence.
Automation and Scripting (250- words)
Task Automation
Python automates repetitive tasks through scripts, enhancing productivity and efficiency in various industries. Task automation streamlines workflows by executing predefined sequences of actions, reducing manual intervention and potential errors. Python's simplicity and readability make it an ideal choice for automation, enabling users to automate intricate processes with ease.
Shell Scripting
Shell scripting with Python grants users command-line control, automating system administration tasks efficiently. Python's shell scripting capabilities enable users to interact with the operating system, executing commands seamlessly. While shell scripting enhances system automation, users must adhere to best practices to ensure script reliability and security. Python's robust error handling mechanisms mitigate risks associated with automated scripting, ensuring system stability.
Conclusion
In wrapping up this comprehensive guide on Understanding Python Code, it is crucial to underscore the significance of grasping the insights shared throughout this article. Python is not merely a programming language but a powerful tool that underpins a vast array of applications across various industries. By delving into the intricacies of Python code, readers can enhance their problem-solving skills, streamline their workflow, and unlock new avenues for innovation. Understanding Python code goes beyond syntax and semantics; it opens doors to the world of software development, data science, machine learning, and web development, empowering individuals to create robust solutions to real-world challenges.
Future of Python Programming
Trends and Innovations
Embarking on an exploration of the Trends and Innovations within Python programming illuminates the dynamic nature of this language. Python's adaptability and versatility have made it a top choice for developers seeking to stay ahead in the ever-evolving tech landscape. From the integration of AI and machine learning to advancements in web development frameworks, Python continues to redefine the boundaries of innovation. Its user-friendly syntax, extensive library support, and cross-platform compatibility make it a preferred option for both novice programmers and seasoned veterans alike. While Trends and Innovations bring about exciting possibilities, it is essential to tread carefully and evaluate each new trend's practicality and long-term viability within specific projects, ensuring that the chosen innovations align with the project's objectives and requirements.
Role in Emerging Technologies
Within the realm of Emerging Technologies, Python plays a pivotal role in shaping the future of software development and technological advancements. Its prominence in fields such as artificial intelligence, data analysis, and automation underscores its relevance in enabling cutting-edge solutions across diverse sectors. Python's scalability, ease of integration, and robust community support contribute to its standing as a cornerstone of emerging tech trends. By leveraging Python's capabilities within new technologies, developers can harness its power to drive innovation, enhance efficiency, and propel digital transformation. However, while Python's role in Emerging Technologies offers unparalleled opportunities, it is imperative to assess the practical implications and potential challenges associated with integrating this language into complex tech ecosystems, ensuring seamless adoption and sustainable growth.