Unraveling the Intricacies of Debugger in PHP: A Comprehensive Guide
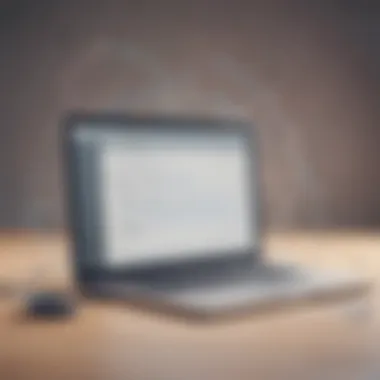
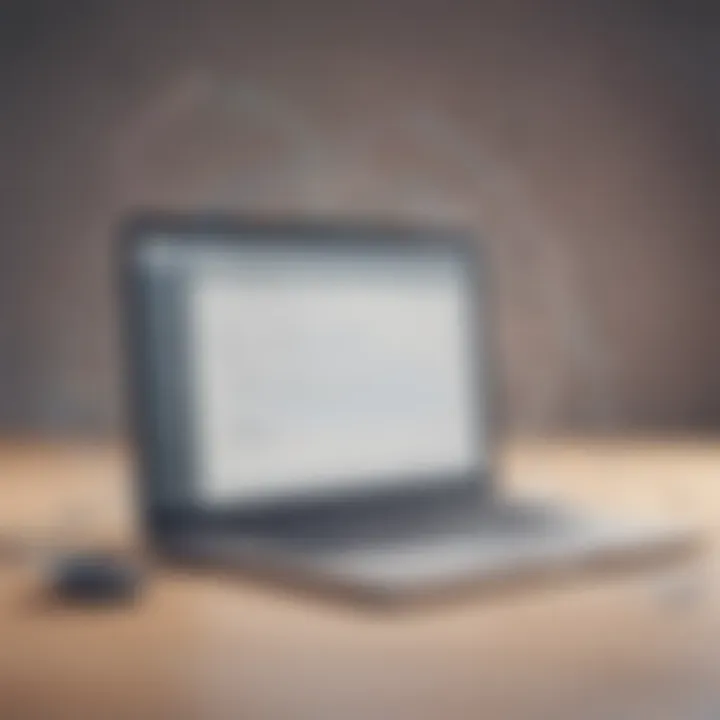
Overview of Debugger in PHP
PHP debugging is a crucial aspect of software development, ensuring code accuracy and functionality. By identifying and resolving errors, developers can streamline the coding process and enhance application performance. The debugger tool in PHP provides a systematic approach to locate and rectify issues, leading to efficient problem-solving within the codebase. Understanding the debugger in PHP equips developers with the necessary skills to create robust and reliable applications. ## t Practices ## Im enting best practices in PHP debugging is essential for maximizing efficiency and productivity. Developers should adopt a systematic approach to debugging, including setting breakpoints, inspecting variables, and tracing program execution. Effective utilization of logging and error reporting mechanisms can aid in identifying and resolving issues promptly. Avoiding common pitfalls such as overlooking error messages or neglecting thorough testing is crucial for successful debugging outcomes. ## How-T ides and Tutorials ## Beginner d advanced users alike can benefit from comprehensive how-to guides and tutorials on PHP debugging. Step-by-step instructions on setting up debugging environments, configuring tools, and executing debugging processes offer practical insights for developers. Hands-on tutorials provide real-time examples and scenarios, enabling readers to apply concepts in a tangible manner. Practical tips and tricks, including shortcut keys, debugging strategies, and code optimization techniques, enhance the effectiveness of PHP debugging practices.
Introduction to Debugging in PHP
Debugging in PHP is a critical aspect of software development, playing a pivotal role in identifying and resolving errors. It is a methodical process that aids programmers in pinpointing and rectifying issues within their code efficiently. Understanding debugger tools and techniques is paramount for PHP developers to streamline their development workflow and optimize code functionality.
Definition and Importance of Debugger
Understanding the concept of a debugger
The concept of a debugger in PHP revolves around a robust toolset designed to assist developers in tracking and fixing errors within their code. By providing detailed insights into the code execution process, a debugger enables programmers to trace the flow of their application, identify bugs, and resolve them effectively. This tool's significance lies in its ability to enhance the development process by promoting code accuracy and efficiency, thereby ensuring the creation of high-quality software products.
Significance of debugging in PHP development
Debugging holds significant importance in PHP development as it serves as a fundamental process for error identification and correction. Effective debugging practices aid in detecting and rectifying syntax errors, logical errors, and other issues that may arise during code execution. By emphasizing meticulous bug identification and resolution, debugging contributes to the overall efficiency and reliability of PHP applications, fostering enhanced user experiences and optimizing code performance.
Common Challenges in PHP Debugging
Identifying syntax errors
One of the primary challenges in PHP debugging involves identifying syntax errors, which can impede code functionality and lead to runtime issues. Detecting and rectifying syntax errors require a comprehensive understanding of PHP syntax rules and effective debugging techniques. By streamlining the error detection process and addressing syntax errors promptly, developers can ensure code accuracy and streamline the development workflow.
Dealing with logical errors
Another common challenge in PHP debugging is addressing logical errors, which often result in unexpected program behavior and output inconsistencies. Resolving logical errors necessitates a systematic approach to problem-solving, including thorough code inspection, testing, and debugging. By employing strategic debugging methods and logical reasoning, developers can effectively troubleshoot and correct complex issues within their PHP codebase.
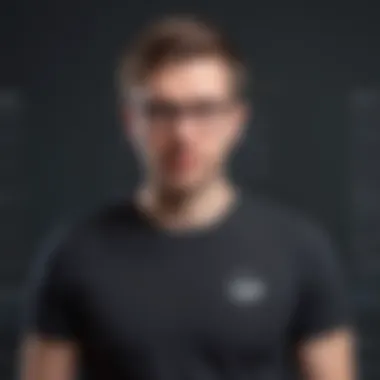
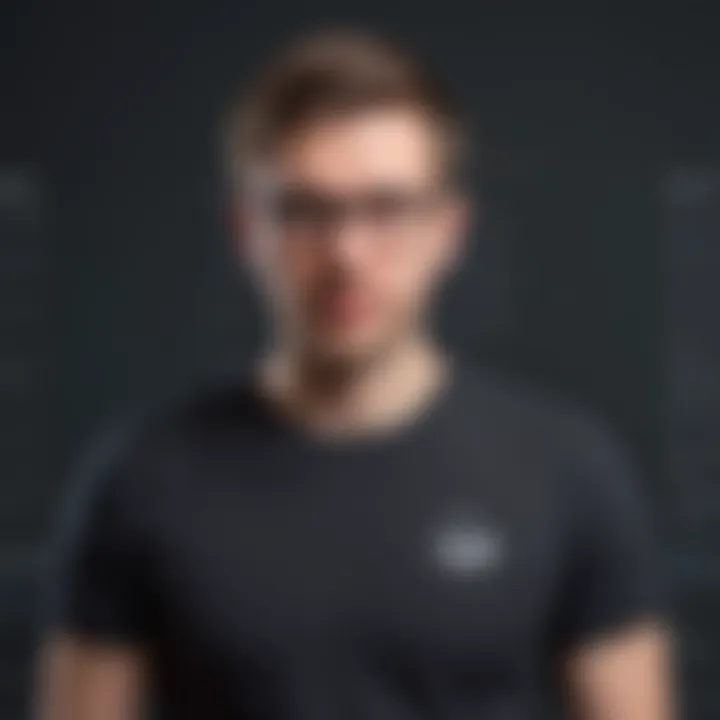
Benefits of Effective Debugging
Enhanced code quality
Effective debugging practices contribute to enhanced code quality by facilitating the identification and resolution of errors and bugs. Through meticulous bug tracking and resolution, developers can ensure code accuracy, consistency, and functionality reliability. Enhanced code quality not only promotes a seamless user experience but also fosters code maintainability and scalability, positioning PHP applications for long-term success.
Time-saving in troubleshooting
Efficient debugging techniques save developers valuable time in identifying and resolving issues within their codebase. By streamlining the debugging process and leveraging advanced debugging tools, programmers can expedite troubleshooting efforts and accelerate the development lifecycle. Time-saving in troubleshooting enables developers to focus on innovation, optimization, and product enhancement, ultimately enhancing their productivity and code efficiency.
Debugger Tools in PHP
Debugger tools in PHP play a pivotal role in the development process, aiding programmers in identifying and resolving errors efficiently. These tools are crucial for debugging PHP applications, ensuring smooth functionality and enhanced performance. By utilizing debugger tools, developers can streamline their debugging processes, leading to improved code quality and reduced time spent on troubleshooting.
Popular PHP Debugger Tools
Xdebug
Xdebug is a powerful debugging tool for PHP that offers a wide range of features to assist developers in identifying and fixing issues within their code. One of the key characteristics of Xdebug is its ability to provide detailed stack traces, making it easier to trace the execution path of a script and pinpoint errors accurately. This unique feature of Xdebug significantly enhances the debugging experience for developers, allowing for more efficient troubleshooting and problem-solving in PHP development.
Zend Debugger
Zend Debugger is another popular choice among PHP developers for its seamless integration with PHP IDEs and robust debugging capabilities. The key characteristic of Zend Debugger lies in its efficient handling of breakpoints, allowing developers to pause the execution of their code at specific points to inspect variables and evaluate expressions. This capability streamlines the debugging process and enables developers to identify and resolve issues swiftly, contributing to overall improved code quality.
PHP Debug Bar
PHP Debug Bar is a handy debugging toolbar that provides developers with valuable insights into the performance of their PHP applications. The key characteristic of PHP Debug Bar is its intuitive interface, which displays essential information such as database queries, execution time, and memory usage in a clear and organized manner. This feature enhances developers' ability to optimize their code and identify potential bottlenecks, ultimately leading to improved application performance and efficiency.
Features and Capabilities
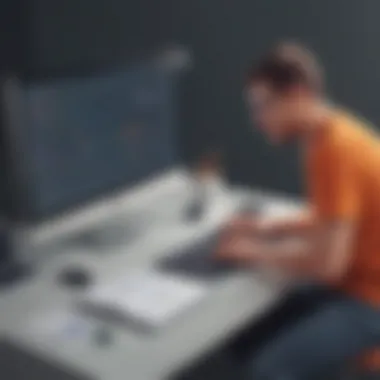
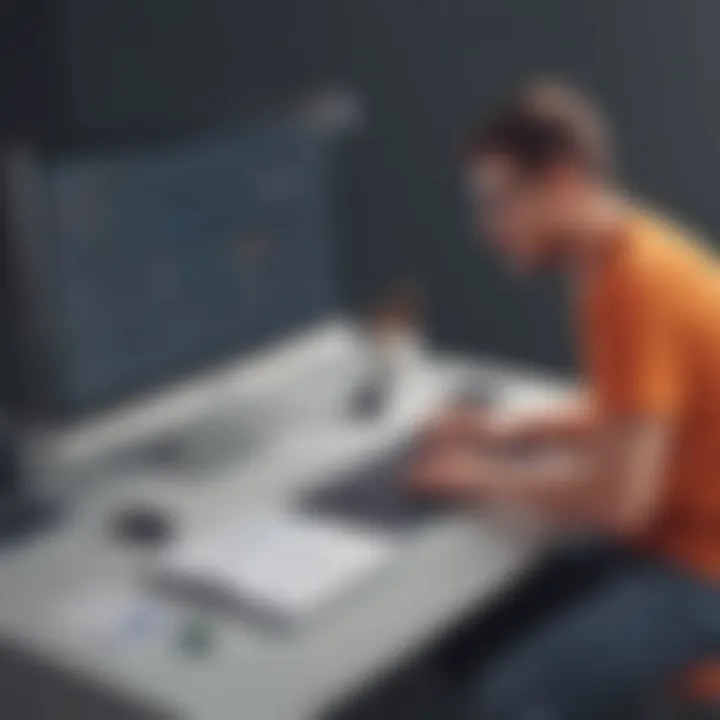
Breakpoints
Breakpoints are a fundamental feature of debugger tools in PHP, allowing developers to pause the execution of their code at specific lines to inspect variables and analyze the program's state. The key characteristic of breakpoints is their versatility in facilitating precise debugging, enabling developers to identify and rectify errors efficiently. By leveraging breakpoints, developers can expedite the debugging process and streamline their code optimization efforts, leading to enhanced code quality and performance.
Variable inspection
Variable inspection is a vital capability offered by debugger tools, enabling developers to view and evaluate the values of variables at different stages of code execution. This feature plays a crucial role in error detection and troubleshooting, allowing developers to analyze the behavior of their code and identify potential issues effectively. By utilizing variable inspection during debugging, developers can gain valuable insights into their code's functionality and make informed decisions to improve its performance and reliability.
Stack traces
Stack traces provide developers with detailed information about the sequence of function calls leading to an error or issue within their code. This feature is instrumental in helping developers trace the execution path of a script and understand the flow of program control. By analyzing stack traces, developers can identify the root cause of errors, optimize code efficiency, and enhance overall debugging effectiveness. Stack traces serve as a critical tool in the developer's arsenal, enabling them to isolate and address issues promptly, thereby improving the quality and robustness of their PHP applications.
Effective Strategies for PHP Debugging
In the realm of PHP development, mastering effective strategies for debugging is paramount to ensure the smooth functioning of software applications. This section sheds light on the pivotal role that effective strategies play in enhancing code quality and streamlining troubleshooting processes. By emphasizing the significance of implementing robust debugging techniques, developers can proactively address errors and maintain code integrity with greater efficiency. Leveraging the right strategies not only accelerates the debugging process but also fosters a more systematic approach to identifying and rectifying issues promptly.
Best Practices for Efficient Debugging
When it comes to efficient debugging in PHP, adopting best practices serves as a cornerstone for successful software development. Isolating issues stands out as a fundamental aspect within this domain, enabling developers to pinpoint specific errors or bugs within the codebase effectively. Isolating issues involves the strategic identification of problematic code segments, allowing for targeted analysis and resolution. This streamlined approach not only expedites the debugging process but also promotes a structured methodology for comprehensive issue resolution. Embracing debugging statements complements these practices by facilitating real-time tracking and monitoring of code behavior. By strategically placing debugging statements throughout the code, developers can gain valuable insights into variable values, function outputs, and program flow, enabling them to unravel complex issues with precision.
Utilizing Step-by-Step Debugging
Executing code line by line holds immense significance in the context of PHP debugging, enabling developers to delve deep into the code execution process. By executing code incrementally, developers can trace the flow of program logic, identify potential anomalies, and validate the expected output at each step. This methodical approach to debugging fosters a granular understanding of code behavior, making it easier to detect and rectify errors systematically. Furthermore, observing variable values in real-time offers developers a dynamic perspective into code variables' states and alterations during program execution. By monitoring variable values closely, developers can validate data integrity, track variable interactions, and troubleshoot issues promptly to ensure optimal code performance and functionality.
Advanced Techniques in PHP Debugging
In the realm of PHP development, mastering advanced techniques in debugging holds paramount significance. These techniques empower developers to delve deep into complex code structures, identify elusive errors, and optimize performance. By incorporating advanced debugging methods, professionals can streamline the development process, enhance code efficiency, and elevate the overall quality of their projects. Embracing advanced PHP debugging techniques is a testament to a developer's meticulous approach and dedication to producing top-notch, error-free code.
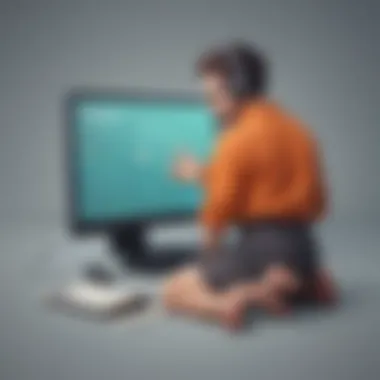
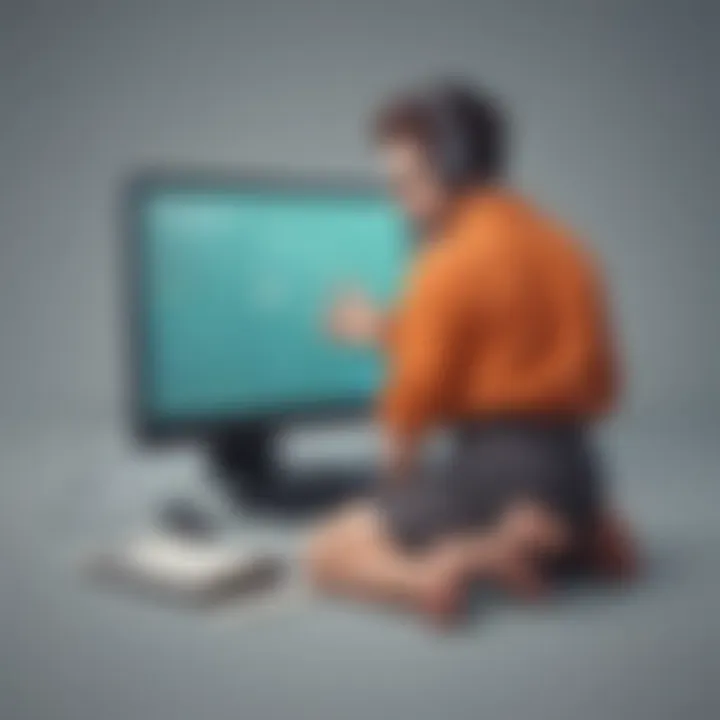
Remote Debugging
Setting up remote debugging environments
Setting up remote debugging environments revolutionizes PHP debugging by allowing developers to diagnose and rectify issues across different physical locations. This feature connects developers to servers or devices remotely, enabling real-time error detection and resolution. The seamless integration of remote debugging minimizes downtime, enhances collaboration among team members working in geographically dispersed settings, and accelerates the troubleshooting process. Remote debugging eliminates the need for developers to be physically present at the problematic location, offering unparalleled flexibility and efficiency in resolving issues promptly.
Profiling and Performance Analysis
Identifying bottlenecks
The task of identifying bottlenecks within PHP code is integral to optimizing overall performance. By pinpointing bottleneck areas, developers can address inefficiencies and enhance code execution speed. Through meticulous bottleneck identification, practitioners can fine-tune their applications for maximum efficiency, ensuring a seamless user experience. The keen focus on identifying bottlenecks underscores a developer's commitment to delivering high-performing, optimized solutions.
Optimizing code efficiency
Optimizing code efficiency involves refining PHP code to maximize performance, reduce resource consumption, and enhance overall functionality. This process entails fine-tuning algorithms, streamlining data structures, and minimizing unnecessary computations. By optimizing code efficiency, developers can boost application responsiveness, lower operational costs, and elevate user satisfaction. Embracing code optimization as a core practice embodies a developer's dedication to crafting efficient, agile solutions that resonate with users and stakeholders.
Handling Exceptions Effectively
Catching and managing exceptions
The ability to catch and manage exceptions is a hallmark of proficient PHP debugging. By effectively handling exceptions, developers can preemptively address potential errors, prevent application crashes, and maintain system stability. Catching and managing exceptions requires a vigilant approach to error detection, swift resolution, and comprehensive error reporting. This proactive stance towards exception handling showcases a developer's foresight, technical acumen, and commitment to delivering robust, error-resilient applications.
Conclusion
In the realm of PHP debugging, the conclusion serves as a crucial segment that encapsulates the essence of the entire discourse on debugging practices within PHP development. It acts as a gateway to summary and reflection, offering developers a comprehensive understanding of the significance and intricacies associated with debugging in PHP. The conclusion segment is pivotal in reinforcing the key insights derived from the preceding sections, emphasizing the importance of robust debugging methodologies in the context of software development, specifically within the PHP ecosystem. It underlines the critical role of debugging in identifying and rectifying errors, ultimately enhancing code quality and optimizing performance. Additionally, the conclusion section provides a roadmap for continuous improvement and refinement in debugging processes, reinforcing the iterative nature of debugging practices to achieve optimal results and efficiency.
Summary of PHP Debugging
Key takeaways
Delving into the key takeaways of PHP debugging unveils fundamental principles and practices that form the cornerstone of effective debugging strategies in PHP development. Key takeaways encompass essential concepts such as error identification, troubleshooting methodologies, and code optimization techniques. These key takeaways serve as actionable insights derived from practical experiences and industry best practices, empowering developers to leverage this knowledge to streamline their debugging workflows effectively. By synthesizing key takeaways, developers can enhance their problem-solving skills, refine their debugging approaches, and expedite the error resolution process, consequently boosting overall development productivity and code quality.
Importance of continuous debugging
The importance of continuous debugging cannot be overstated in the realm of PHP development. Continuous debugging epitomizes an iterative approach towards code refinement and error resolution, ensuring that software projects maintain optimal performance and stability throughout their lifecycle. By embracing continuous debugging practices, developers can proactively identify and address issues as they arise, preempting potential bottlenecks and enhancing the overall reliability of software applications. The cyclical nature of continuous debugging fosters a culture of accountability and excellence within development teams, promoting a proactive mindset towards issue resolution and software optimization. Emphasizing the value of persistent debugging efforts, developers can cultivate a responsive and adaptive development environment, poised for innovation and efficiency in PHP programming.