Unveiling the Intricacies of Java Programming: An In-Depth Exploration
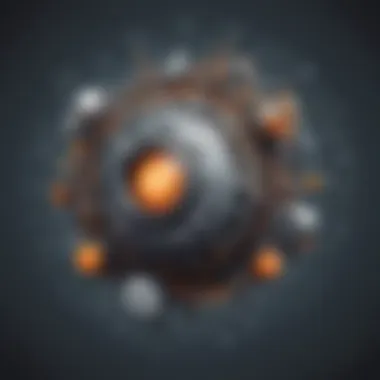
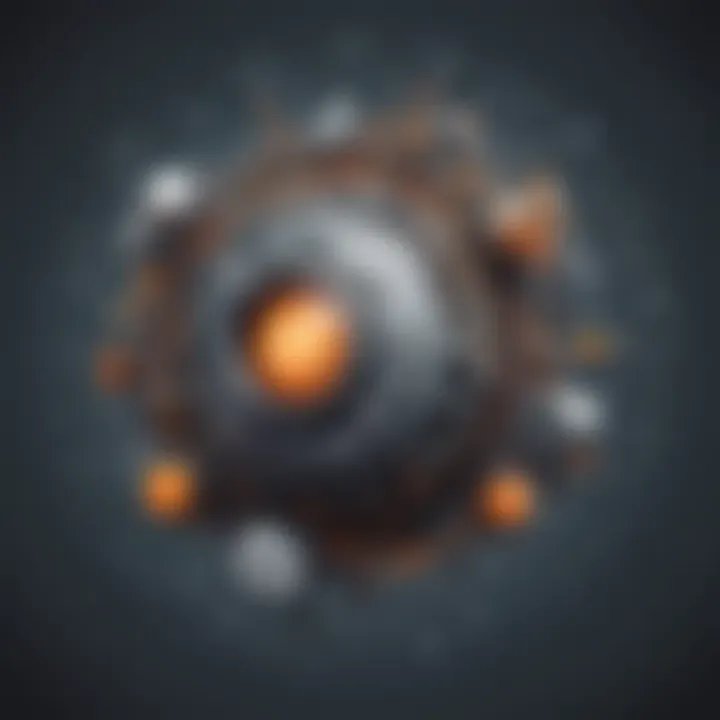
Overview of Java Programming
Java programming is a versatile language extensively used in software development, known for its scalability and robustness. Understanding the mechanisms of Java is crucial for developers to harness its full potential. From syntax intricacies to memory management techniques and object-oriented principles, delving deep into Java's core functioning unveils a world of possibilities.
Java Syntax Demystified
At the heart of Java lies its syntax, which dictates the rules for writing code in this language. Java syntax is elegant yet precise, emphasizing readability and simplicity. By mastering Java syntax, developers can write efficient and error-free code, laying a strong foundation for building complex applications.
The Art of Memory Management in Java
Memory management is a critical aspect of Java programming, enabling effective allocation and deallocation of memory resources. Understanding how Java handles memory boosts application performance and prevents memory leaks. By implementing best practices in memory management, developers can optimize their code for efficiency and stability.
Unraveling Object-Oriented Principles
Java is renowned for its robust support of object-oriented programming (OOP) principles. Encapsulating data, inheritance, polymorphism, and abstraction form the pillars of OOP in Java. Mastery of these principles empowers developers to design modular, scalable, and maintainable code structures.
Benefits of Mastering Java Mechanisms
Proficiency in Java mechanisms equips developers with a powerful toolkit to create sophisticated applications with ease. By honing their skills in syntax, memory management, and OOP principles, developers can elevate their coding capabilities and unlock a world of possibilities in the realm of Java programming.
Introduction to Java
Java, a powerhouse in the world of programming languages, serves as the bedrock for numerous applications and systems across the globe. Its versatility, reliability, and robustness make it a go-to choice for developers seeking a comprehensive language for various projects. In this section, we delve into the essential aspects of Java programming, setting the stage for a detailed exploration of its mechanisms and functionalities.
History and Evolution of Java
Origins of Java
In understanding the evolution of Java, we must first explore its inception. Java emerged in the mid-1990s, created by Sun Microsystems as a solution for developing applications for consumer electronic devices. The key charm of Java lies in its platform independence, allowing programs to run on any device with a Java Virtual Machine (JVM). This feature revolutionized the software development landscape by streamlining cross-platform compatibility.
Key Milestones in Java's Development
The development of Java has been marked by significant milestones that have shaped its current form. From the release of Java 1.0 in 1996, which introduced the Java Applet for web browsers, to the Java 8 update in 2014 that brought lambdas and the Stream API, each step represented a leap forward in enhancing Java's capabilities. These milestones showcase the continuous evolution and refinement of Java as a cutting-edge programming language.
Java Virtual Machine (JVM)
Role of JVM in Executing Java Programs
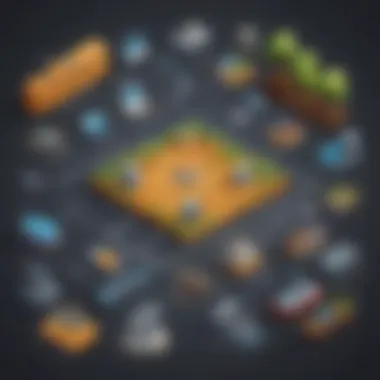
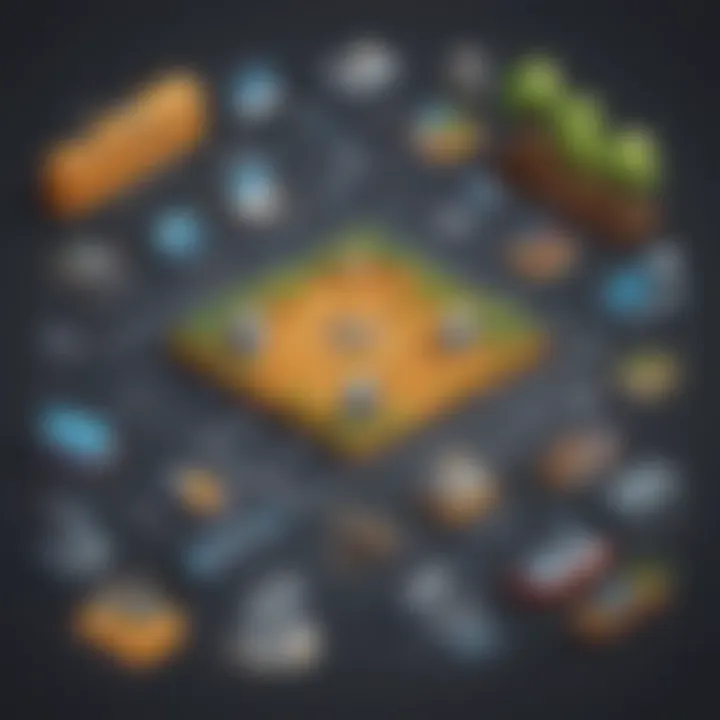
At the core of Java's functionality lies the Java Virtual Machine (JVM), a crucial component responsible for executing Java programs. The JVM acts as an interpreter, converting Java bytecode into machine code that can be understood by the underlying hardware. This abstraction layer not only ensures platform independence but also adds a layer of security through controlled execution environments.
Components of JVM
The JVM comprises several essential components that work in unison to execute Java programs seamlessly. From the Class Loader, which loads class files to the Execution Engine, which executes instructions, each part plays a vital role in the program's execution. Additionally, the JVM includes the Java API, which provides a rich set of libraries for developers to build upon, enhancing productivity and code quality.
Memory Management in Java
Memory management plays a critical role in the effective operation of Java programs. Efficient memory allocation and deallocation are essential for optimal performance and resource utilization. In this section, we will explore the intricate mechanisms involved in memory management within the Java programming language.
Stack and Heap Allocation
Key Differences Between Stack and Heap Allocation
In Java, memory is allocated in two primary areas: the stack and the heap. The key dissimilarity between these two lies in their storage mechanisms and accessibility. The stack is used to store method execution contexts and local variables, operating in a last-in-first-out manner. On the contrary, the heap is a region of memory used for dynamic memory allocation, such as objects created during runtime. Understanding the variance between stack and heap allocation is crucial for efficient memory management and preventing memory leaks.
Memory Allocation Process
The memory allocation process in Java involves dynamically assigning memory to objects created during runtime. When an object is instantiated, memory is allocated on the heap, and a reference to that memory address is stored in the stack. Java employs automatic memory management through garbage collection to reclaim memory occupied by objects that are no longer in use. By comprehending the memory allocation process, developers can optimize memory usage and enhance the performance of Java applications.
Garbage Collection
Principles of Garbage Collection
Garbage collection in Java is based on the principle of reclaiming memory from objects that are no longer reachable or in use. Java's garbage collector automatically identifies and removes unreferenced objects, preventing memory leaks and maintaining the integrity of the heap. By adhering to sound garbage collection principles, Java ensures efficient memory utilization and system stability.
Types of Garbage Collectors
Java offers different types of garbage collectors, each with its unique advantages and characteristics. From the serial and parallel collectors to the concurrent and G1 collectors, Java provides developers with options to tailor garbage collection strategies based on application requirements. Understanding the types of garbage collectors enables developers to fine-tune memory management practices and optimize the performance of Java programs.
Object-Oriented Principles in Java
Object-oriented principles form the backbone of Java programming, playing a crucial role in organizing and structuring code. By emphasizing concepts such as encapsulation, inheritance, and polymorphism, Java promotes code reusability, maintainability, and scalability. Understanding these principles is essential for developers to write efficient, modular, and easily maintainable code. Object-oriented programming allows for the conceptualization of real-world entities as objects, each encapsulating data and behavior. Java's implementation of object-oriented principles provides a powerful framework for building complex software systems.
Encapsulation
Definition and Benefits of Encapsulation
Encapsulation in Java refers to the bundling of data with the methods that operate on that data, restricting access to the internal state of an object. This fundamental concept fosters data hiding, preventing direct modification of object properties by external entities. The primary benefit of encapsulation is the enhancement of code security and maintainability. By encapsulating data within objects, Java promotes modular design and reduces interdependencies between components. This simplifies debugging and enhances code readability, leading to more robust and scalable applications.
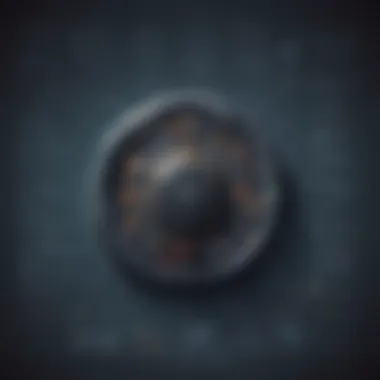
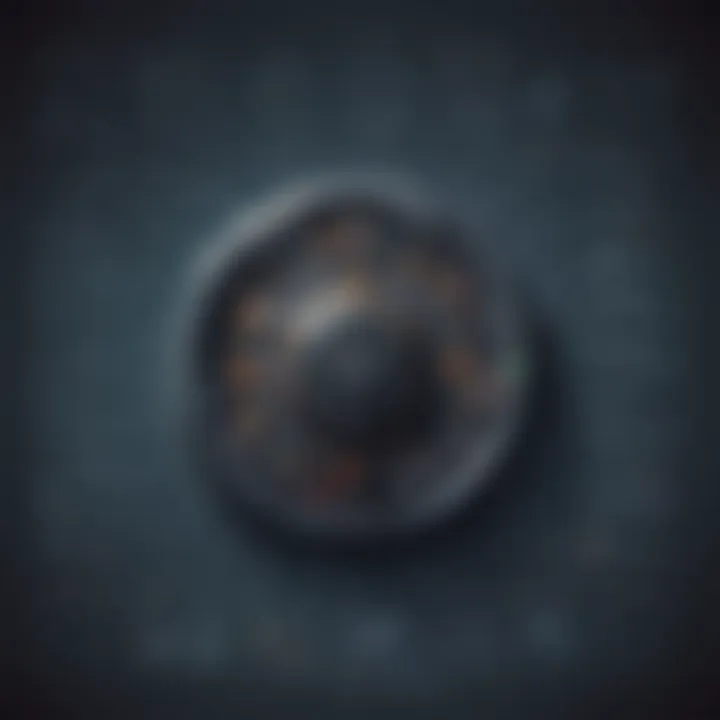
Encapsulation in Java
In Java, encapsulation is achieved through the use of access specifiers such as private, protected, and public to control the visibility of class members. The key characteristic of encapsulation in Java is the principle of information hiding, where data within an object is shielded from direct external access. Encapsulation allows developers to enforce data integrity by providing controlled access to class members. While encapsulation enhances code security and modularity, it may introduce overhead due to the need for accessor and mutator methods. However, the advantages of improved code organization and reduced complexity outweigh these minor drawbacks.
Inheritance
Concept of Inheritance
Inheritance in Java enables the creation of a new class based on an existing class, allowing the new class to inherit attributes and methods from the parent class. This promotes code reuse and establishes a parent-child relationship between classes. The key characteristic of inheritance is the ability to derive new classes with shared characteristics, reducing code duplication and enhancing code extensibility. Inheritance supports the hierarchical organization of classes, facilitating the classification of objects based on common traits.
Types of Inheritance in Java
Java supports various types of inheritance, including single inheritance, where a class inherits from only one base class, and multiple inheritance, where a class inherits from multiple base classes through interfaces. Each type of inheritance offers distinct benefits and considerations in terms of code design and complexity. Single inheritance promotes simplicity and clarity but may limit class relationships, while multiple inheritance allows for broader class hierarchies but introduces challenges such as diamond inheritance problem. Choosing the appropriate type of inheritance depends on the specific requirements of the software design.
Polymorphism
Understanding Polymorphism
Polymorphism in Java allows objects to be treated as instances of their parent class, enabling the implementation of methods that can operate on objects of multiple types. This flexibility in method invocation is a key characteristic of polymorphism, promoting code reusability and extensibility. Polymorphism simplifies code maintenance by enabling the use of generic algorithms that can process objects of diverse types. Java's support for polymorphism enhances the adaptability of code to changing requirements and promotes the development of flexible and scalable software systems.
Polymorphism Examples in Java
In Java, polymorphism can be exemplified through method overloading and method overriding. Method overloading involves defining multiple methods with the same name but different parameters within a class, allowing for the execution of different functionalities based on the method arguments. Method overriding, on the other hand, occurs when a subclass provides a specific implementation of a method defined in its parent class. These examples showcase the versatility of polymorphism in Java programming, illustrating how the language facilitates dynamic method dispatch and runtime object binding.
Exception Handling in Java
Exception handling is a crucial aspect of Java programming, playing a vital role in ensuring the robustness and reliability of applications. In this article, we will unravel the intricacies of exception handling in Java, shedding light on its significance and practical implications. By effectively managing exceptions, developers can prevent unforeseen errors and enhance the overall stability of their codebase.
Types of Exceptions
Checked and Unchecked Exceptions
Checked and Unchecked Exceptions are pivotal in Java exception handling. Checked exceptions must be either caught or declared in the method signature, compelling developers to address potential issues explicitly. On the other hand, Unchecked Exceptions need not be explicitly handled, offering more flexibility but requiring careful consideration to prevent runtime disruptions. The distinction between these exceptions lies in their handling requirements and the impact they have on code predictability and stability.
Custom Exceptions
Custom Exceptions empower developers to create tailored exception types to suit specific application requirements. By defining custom exception classes, developers can encapsulate complex error scenarios in a structured manner, improving code readability and maintainability. These custom exceptions not only enhance the clarity of error-handling logic but also enable developers to communicate specific failure conditions effectively. However, the creation and maintenance of custom exceptions require meticulous handling to avoid redundancy and ensure coherence within the codebase.
Exception Handling Mechanisms
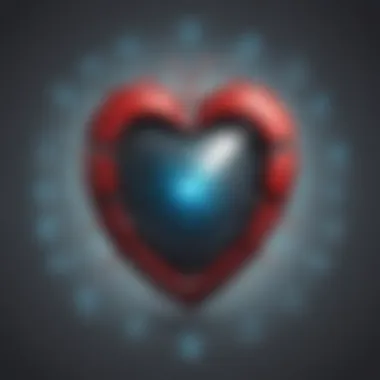
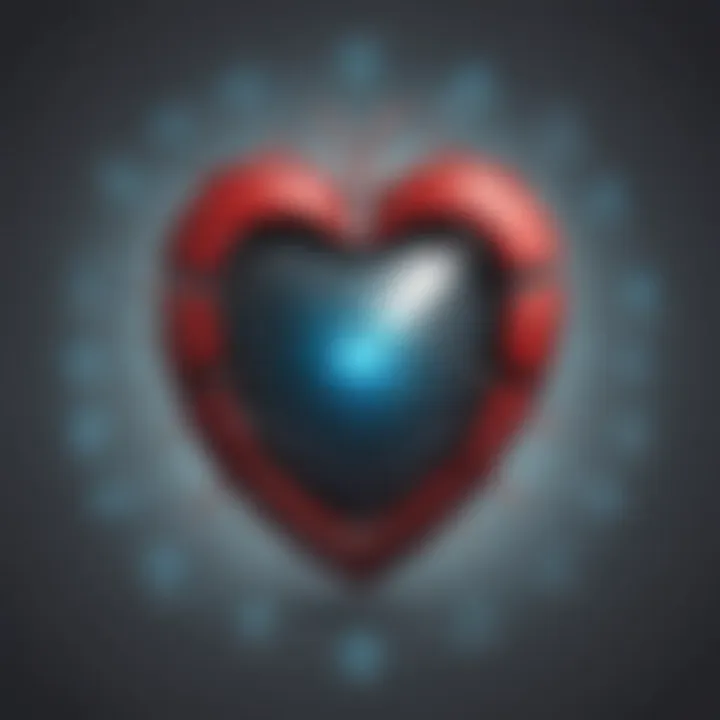
Try-Catch Blocks
Try-Catch Blocks provide a structured approach to handling exceptions in Java. By encapsulating error-prone code within a 'try' block and specifying appropriate catch blocks, developers can gracefully manage exceptions and maintain program flow. The key advantage of Try-Catch Blocks lies in their ability to segregate error-handling logic from regular code execution, promoting code organization and clarity. Nevertheless, overusing Try-Catch Blocks can lead to code bloat and obscure the underlying program logic, necessitating a balanced approach to exception handling.
Finally Block
The Finally Block complements Try-Catch Blocks by providing a mechanism to execute cleanup code irrespective of whether an exception occurs. This ensures that critical resources are released properly, enhancing the overall reliability and stability of the application. The Finally Block serves as a safety net, enabling developers to implement necessary cleanup operations without relying solely on the success or failure of specific code blocks. However, improper usage of Finally Blocks can introduce maintenance challenges and obscure the intended flow of the program, emphasizing the need for strategic placement and precise execution.
Concurrency in Java
In the realm of Java programming, concurrency plays a pivotal role in enabling tasks to run simultaneously, enhancing performance and efficiency. The significance of concurrency in this comprehensive guide lies in unraveling how multiple threads can execute sections of code concurrently, providing developers with the ability to optimize resource utilization and improve response times. With the increasing demand for responsive and scalable applications, understanding and implementing concurrency mechanisms in Java is essential for software developers seeking to elevate their programming skills.
Threads in Java
Multithreading
Multithreading, a fundamental concept in Java, allows multiple threads to execute within a single process, enabling parallel task execution and enhancing the overall performance of Java applications. The key characteristic of multithreading lies in its ability to divide a program into smaller tasks that can run concurrently, exploiting the full potential of multi-core processors. This approach proves beneficial as it accelerates task completion and improves system responsiveness. However, managing shared resources among threads and synchronizing their execution poses challenges that developers must address to avoid conflicts and ensure data integrity.
Synchronization
The synchronization mechanism in Java ensures thread safety by coordinating the access of shared resources among multiple threads. By restricting concurrent access to critical sections of code through synchronization blocks or methods, developers can prevent race conditions and maintain data consistency. The key characteristic of synchronization lies in its ability to enforce mutual exclusion, allowing only one thread to access synchronized blocks at a time. While synchronization safeguards against data corruption and concurrency issues, it can introduce performance overhead due to thread contention and context switching. Developers must strike a balance between thread safety and performance optimization when employing synchronization in Java programs.
Executor Framework
Task Execution in Java
Task execution in Java revolves around executing units of work concurrently using thread pools managed by the Executor framework. This approach facilitates efficient task distribution and resource allocation, enhancing application scalability and responsiveness. The key characteristic of task execution lies in its ability to decouple task creation from its execution, enabling seamless parallel processing of tasks without the overhead of thread creation. By leveraging executor services, developers can delegate tasks to worker threads, allowing for optimized utilization of system resources and streamlined task management.
ThreadPool Implementation
The ThreadPool implementation in Java simplifies task management by encapsulating a pool of reusable worker threads that execute submitted tasks concurrently. The key characteristic of ThreadPool implementation lies in its ability to maintain a pool of pre-initialized threads, eliminating the overhead of thread creation and teardown for each task. This approach enhances performance and reduces latency by minimizing thread creation delays. However, sizing the thread pool appropriately is crucial to avoid resource contention and optimize task throughput. By configuring thread pool parameters effectively, developers can strike a balance between resource utilization and task processing efficiency in Java applications.
Conclusion
In this comprehensive guide on Understanding the Mechanisms of Java, the Conclusion section holds immense significance. It serves as the culmination of the detailed exploration into Java's intricate workings, summarizing the key insights obtained throughout the article. By elucidating the practical applications and essential concepts discussed earlier, the Conclusion ties together the various facets of Java programming covered in the preceding sections. It reiterates the importance of understanding Java's syntax, memory management, object-oriented principles, exception handling, and concurrency to comprehend the language's core functionality fully.
Key Takeaways
Understanding Java Concepts
Delving into the realm of Understanding Java Concepts unveils a fundamental aspect critical to mastering Java programming. This section elucidates the foundational elements that underpin Java's syntax, structure, and principles. By dissecting the intricate details of Java concepts like data types, inheritance, and polymorphism, readers gain a nuanced understanding of how these elements contribute to building robust Java applications. The clarity provided in comprehending Java's core concepts empowers developers, IT professionals, and tech enthusiasts to harness Java's full potential in their projects. Understanding Java Concepts not only equips individuals with a solid foundation in Java programming but also serves as a gateway to delving deeper into advanced Java topics.
Practical Applications
Exploring Practical Applications sheds light on the real-world relevance and usability of Java programming. This section focuses on bridging the gap between theoretical knowledge and practical implementation, presenting tangible scenarios where Java's concepts and mechanisms come to life. By showcasing examples of Java applications in various industries such as software development, web development, and data analysis, readers witness firsthand the versatility and scalability of Java programming. Practical Applications provide insights into how Java can be leveraged to solve complex problems efficiently, emphasizing its role as a versatile and in-demand language in the tech landscape. Understanding the practical aspects of Java applications enables professionals to apply their knowledge effectively, driving innovation and problem-solving across diverse domains.