Java Inheritance and Polymorphism Explained
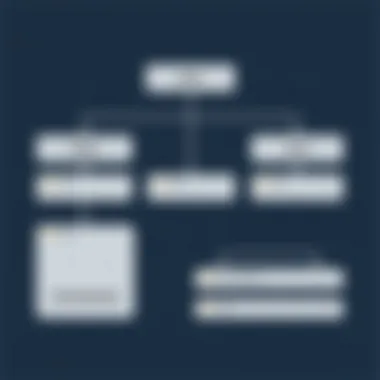
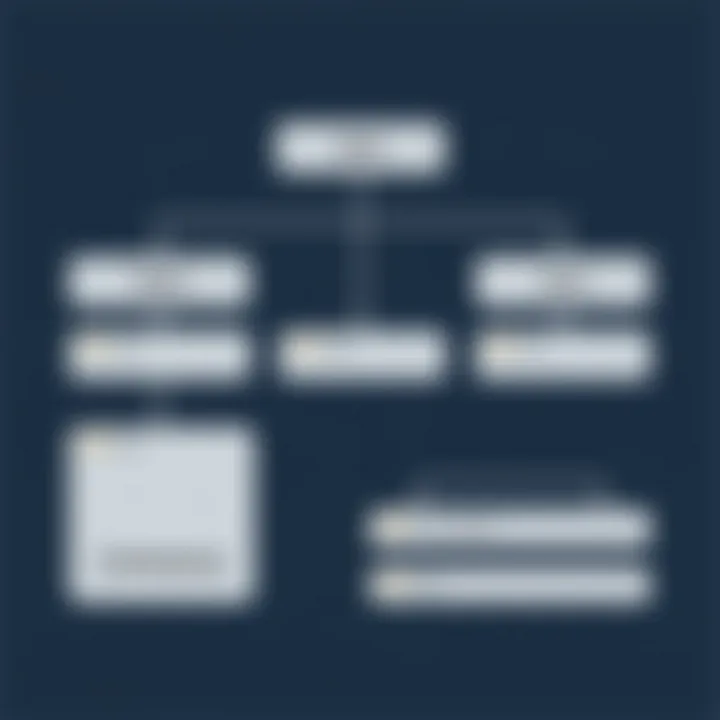
Intro
In a rapidly evolving world of software development, the ability to grasp core principles is indispensable. Among the essential concepts within object-oriented programming, inheritance and polymorphism stand out as fundamental pillars in Java. Their significance is echoed through everyday development practices, leading to enhanced code efficiency and maintainability.
Inheritance allows developers to craft new classes based on existing ones, promoting code reuse. This means getting a head start by leveraging previously defined properties and methods. For example, if we consider a basic class like , we can define subclasses like and , inheriting general attributes while adding specific behaviors unique to each.
On the other hand, polymorphism introduces flexibility to method behaviors, enabling objects to take on multiple forms. Imagine a scenario where various animal types might respond differently to a command like . With polymorphism, each class can implement this method in its own way, ensuring that behavior is contextual to the specific object.
Understanding these concepts isn't just about theory; it's about implementing them in real-world applications to unlock powerful efficiencies. The ongoing discussion throughout this article aims to shine light on practical applications, common pitfalls, and advanced techniques that enhance your Java programming prowess.
As technology continues to advance, holding a firm grasp on inheritance and polymorphism will ensure that you can adapt and thrive. Let's venture deeper into these crucial concepts and their applications.
Foreword to Object-Oriented Programming
In the fast-paced world of software development, understanding fundamental concepts is crucial for building robust applications. One of these cornerstones is Object-Oriented Programming (OOP). OOP is not just a method of structuring code; it represents a paradigm that shifts how developers approach problem-solving and system design. Its principles enable greater efficiency, flexibility, and scalability in software projects.
Defining the Core Principles
At its core, OOP revolves around several key principles that guide how objects interact within a program. Encapsulation allows developers to bundle data and methods that operate on the data within one unit, or class, while restricting outside access. This is like putting valuables in a safe, where only designated keys (methods) can access or alter them.
Inheritance enables new classes to inherit attributes and behaviors from existing classes, promoting code reuse and a hierarchical organization of concepts.
Polymorphism, as the term suggests, refers to the ability of different objects to respond to the same method call in different ways. This principle underlies the flexibility of OOP, allowing one interface to control access to a wide range of functionalities.
Lastly, abstraction abstracts away complex realities while exposing only the necessary parts to the user. It's akin to driving a car without needing to understand the intricacies of its engine. Together, these principles form a cohesive framework that elevates the programming experience.
Significance of Object-Oriented Concepts
The significance of OOP can't be overstated. For one, it mirrors how we perceive the real world, thus making it more intuitive for developers who are tasked with converting complex systems into understandable models.
- Improved Code Maintainability: Focusing on objects and their interactions, instead of procedural sequences, simplifies the tracking of changes and debugging. Developers can modify a single class without affecting others, provided the interface remains consistent.
- Enhanced Collaboration: In team settings, OOP allows multiple developers to work on different classes simultaneously without stepping on each other's toes. Each developer can take ownership of a class, refining it as per specific requirements while the rest of the project continues to evolve.
- Scalability: OOP designs pave the way for systems to expand and evolve over time. New functionalities can often be added with minimal adjustments to existing code, making it easier to keep pace with changing demands.
"Object-oriented programming is an exceptionally powerful approach for organizing one’s code in a manageable format that deeply mirrors thought processes."
Understanding Inheritance in Java
Inheritance sits at the core of object-oriented programming, and in Java, it brings a host of advantages that stretch the capabilities of plain old classes. When you grasp the ins and outs of inheritance, it paves the way for organized code structure and efficient reuse. It's not just a matter of typing out rules and regulations; it's about understanding how to build upon existing foundations.
By letting developers create a new class based on an existing one, inheritance fosters a relationship where the child class inherits properties and methods. This not only minimizes redundancy in code but also aligns with the real-world relationships among objects, forging a more intuitive design pattern. With inheritance, fresh classes can tap into existing functionalities, which streamlines modifications and enhances maintainability—all vital elements in the life of a software developer.
Basic Concepts of Inheritance
To kick things off, let's unpack the foundational ideas. Inheritance establishes a parent-child connection between classes. The parent class, often called the superclass, is the one that offers characteristics, while the child class, referred to as the subclass, garners those traits.
- The superclass can be a general parent, like , that might define methods common to all animals, and the subclass, for instance, , can inherit these methods while possibly adding its own specialities.
This benefits the coding process immensely. Consider an example where a system needs separate classes for different types of vehicles. Instead of rewriting code for common features like or , a programmer can create a base class and derive other classes like or from it, which will inherit those shared methods.
Types of Inheritance
Delving deeper, we find multiple forms of inheritance, each with its own flair and functionalities. Understanding these varied types helps in selecting the right structure for specific tasks.
Single Inheritance
Single inheritance is like having a dedicated coach guiding one athlete. A subclass can inherit only from one superclass. This approach is straightforward and reduces complexity, making it relatively easy to trace where specific properties come from.
The key characteristic here is clarity. When a subclass derives from one parent class, there's minimal confusion about method resolution and property access. This simplicity makes single inheritance a popular foundation for most Java applications.
- The principal benefit is its ease of understanding and management. However, its limitation lies in the inability to inherit from multiple sources, which can hinder code reuse in complex scenarios.
Multilevel Inheritance
Next up is multilevel inheritance. This is like a family tree, where a child class inherits from a parent, and that parent is also a child of another class. It’s a kind of stacking that exemplifies hierarchy effectively.
- A unique feature is how it demonstrates a clear lineage of classes. For instance, you might have as the parent, as the intermediate child, and as the derived child. Each class can introduce new methods relevant to its scope.
- The advantage here is enhanced specialization. However, over-relying on layers can sometimes muddle method access and lead to complications, especially when each level introduces methods that can clash.
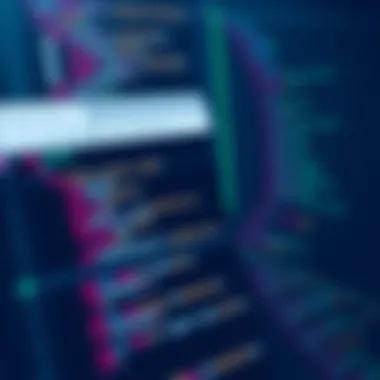
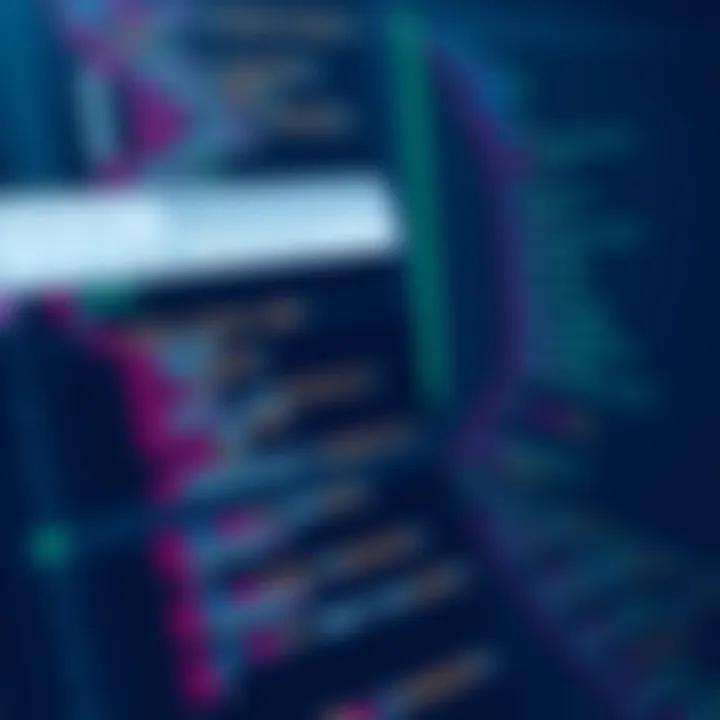
Multiple Inheritance Through Interfaces
Java avoids the snake pit of multiple inheritance directly within its class structures. However, it offers a workaround through interfaces. Basically, a class can implement multiple interfaces, thus gaining the ability to incorporate behaviors from various sources, resembling multiple inheritance.
The key characteristic is flexibility. A class can mix and match functionalities from various interfaces, which allows developers to create rich and versatile object models.
- The repurposed advantage of multiple inheritance through interfaces is high code reusability without the messy complications that often come with it. On the flip side, maintaining clarity on which interface provides which method can become confusing if not documented properly.
Benefits of Using Inheritance
Inheritance brings a treasure trove of benefits to the table. Most notably, it promotes code reuse. Once a class is defined, it can serve as a template for many others without requiring additional effort. This leads to less code duplication, ultimately making development faster and less prone to errors.
- It supports maintainability. Changes in the superclass automatically reflect in all the subclasses, saving developers from churning out changes for each individual class.
- Furthermore, it enhances logical structure, aligning code closely with real-world relationships, making it easier to understand and manage.
Common Inheritance Pitfalls
Despite its advantages, using inheritance can lead to a few potholes. One common mistake is creating deep inheritance hierarchies. They seem elegant initially, but if poorly managed, they become labyrinthine and hard to navigate. Understanding where to actually use inheritance vs. composition can be quite tricky.
Inheritance vs Composition
On the topic of composition, it's important to note that relying solely on inheritance can be a double-edged sword. Composition allows combining simpler objects to form more complex ones. This can lead to greater flexibility and better encapsulation, often resulting in more manageable code than ever-increasing class hierarchies. It’s a classic case of considering all options before diving into a specific solution.
Being aware of these considerations will lead to a more robust, flexible, and maintainable application. This section about inheritance gears the understanding that inheritance in Java is not just about making it work but about making it smart.
"Inheritance is a powerful mechanism, but overuse can lead to rigid systems that are hard to change and maintain."
In this journey through inheritance, the key is to find a balance that supports growth while avoiding unnecessary complications.
Exploring Polymorphism
In the realm of Java, polymorphism stands out as a critical concept, offering versatility and dynamic behavior in code execution. This section unwraps the layers of polymorphism, shedding light on its significance and the subtle nuances that make it a cornerstone of object-oriented programming. By grasping polymorphism, you can enhance code flexibility, improve readability, and foster a more intuitive coding environment. It’s like having a Swiss Army knife in your programming toolkit; the more you understand, the better you can adapt to different situations.
Defining Polymorphism
Polymorphism can be understood as the ability of a variable, function, or object to take on multiple forms. In simpler terms, it allows one interface to be used for different underlying data types. This versatility is essential for building scalable applications, as it enables developers to write code that can operate on various classes and objects seamlessly. Essentially, polymorphism simplifies code management and enhances its adaptability, ensuring that a single function can process different data types without unique implementations for each type.
Types of Polymorphism
Polymorphism in Java comes in two primary flavors: compile-time polymorphism and runtime polymorphism, each serving unique purposes and offering distinct advantages.
Compile-Time Polymorphism
Compile-time polymorphism, often associated with method overloading, is a mechanism where the method to be invoked is determined at compile time. This sharp distinction and early determination of method bindings can lead to improved performance since decisions are made ahead of time rather than at runtime.
A key characteristic of compile-time polymorphism is its reliance on method signatures. That means methods with the same name but different parameter lists can exist within the same class without conflict.
This approach is popular among Java developers, mainly because it adds a layer of clarity to the codebase and allows for cleaner code structure.
Advantages:
- Efficient performance due to early binding
- Provides a clear, organized structure to the code
Disadvantages:
- Can lead to method confusion when many overloads exist
The unique feature of compile-time polymorphism lies in its predictability; however, it requires careful planning to avoid overwhelming situations with too many overloaded methods.
Runtime Polymorphism
On the other hand, runtime polymorphism is tied closely to method overriding. This type of polymorphism occurs when a call to an overridden method is resolved at runtime rather than compile time. The situation allows for dynamic changes in behavior with the same method call based on the object invoking it.
A vital aspect of runtime polymorphism is that it enhances code scalability and flexibility. This means you can create more generic methods which can operate on various subclasses without knowing their specifics at compile time.
Advantages:
- Greater flexibility and adaptability in code
- Supports dynamic method invocation, which is crucial for complex applications
Disadvantages:
- Slightly less efficient than compile-time polymorphism due to dynamic binding
Runtime polymorphism is advantageous for developing applications that require dynamic behavior, allowing developers to write more generic and reusable code.
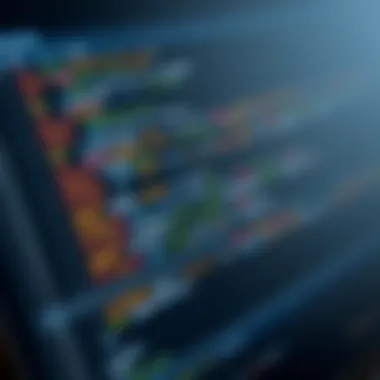
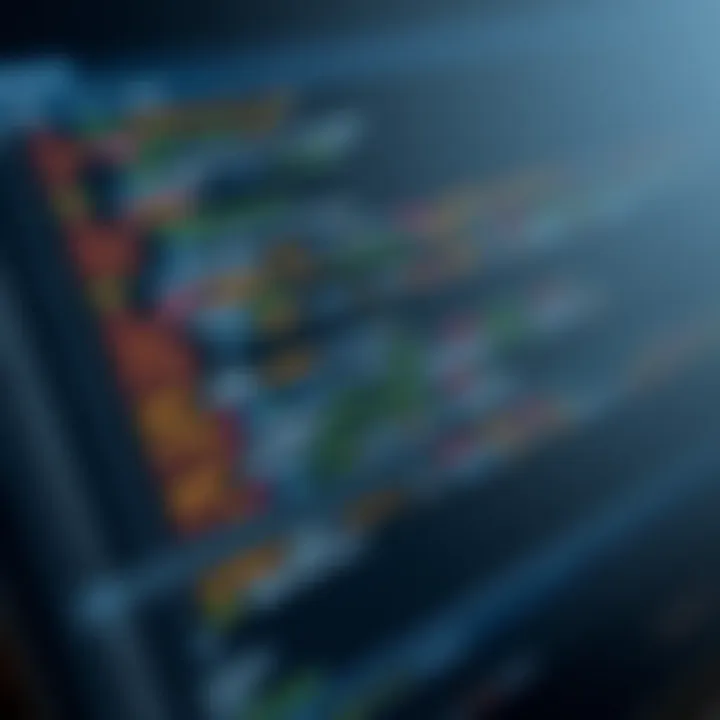
Method Overloading Explained
Method overloading is a classic example of compile-time polymorphism, where multiple methods share the same name but differ in their parameter types or counts. For instance, consider a simple class that has two methods named . One method might take two integers, while the other takes three doubles. This flexibility allows for an intuitive and user-friendly design, letting developers use the same method name for similar functionality without confusion.
Method Overriding Explained
Method overriding features in runtime polymorphism as it allows a subclass to provide a specific implementation of a method that is already defined in its superclass. This concept is a game changer in creating functionalities where behavior can differ significantly between the base class and its derived classes. The annotation ensures that the superclass method exists and is intended to be overridden, preventing silent errors that could emerge in large codebases.
Applications of Polymorphism
The practical applications of polymorphism are vast and varied. Here are a few scenarios where it shines:
- User Interfaces: Handling different types of user input through a common interface.
- Gaming: Creating diverse game entities (like players and enemies) that share a basic set of properties but have different behaviors.
- Data Management: Allowing processing of different data types through a unified method call, making code simpler and reducing redundancy.
By leveraging polymorphism, Java developers can construct highly maintainable systems that are both flexible and robust, catering to a steadily evolving set of requirements and enhancing the overall programming experience.
Practical Examples Demonstrating Inheritance
In the realm of programming, understanding inheritance is vital. It’s like grasping the strings of a finely crafted instrument; without this knowledge, achieving harmony is challenging. Inheritance is not just a concept; it’s a robust structure that allows for creating scalable and maintainable code. With it, developers can form relationships between classes, fostering code reuse and reducing redundancy. In this section, we delve into practical examples of inheritance to shed light on its functionality and real-world application.
Simple Class Hierarchy
Creating a simple class hierarchy can often feel like drawing a family tree. Each class represents a different generation and exhibits specific traits inheriting characteristics from previous generations. Let's illustrate this with a straightforward example involving animals.
Consider a class called . It could have attributes like , , and a method like . Here’s how this works in code:
Next, you can create subclasses like and , which inherit from but also add their distinctive behaviors:
Now you have a simple hierarchy. and share properties from but can also express their unique methods. This way, you’re not muddling through multiple definitions of sound-making methods; modifying one class leads to changes across the tiered system, enhancing efficiency.
Use Case Scenarios
Use cases showcase the practical application of inheritance in everyday programming tasks. They serve as a blueprint for real-world scenarios that a developer might encounter while coding. For instance, let’s say we’re developing a game where various characters have specific roles:
- Game Character Classes:
- Transportation Systems:
- Base Class: could share common attributes like , , and methods like .
- Derived Classes: You could have , , and , each with their peculiar features while using shared attributes. This saves time and keeps the code cleaner.
- Base Class: An class might define methods like and .
- Derived Classes: From , you can have , , and . Each subclass can enhance the base class functionality, perhaps by adding methods like for or for .
In both cases, inheritance simplifies the management of properties and behaviors, minimizing the likelihood of errors and boosting maintainability.
"Inheritance is like sharing the family recipe; each generation adds its twist while keeping the core ingredients.”
In summary, practical examples of inheritance not only illustrate its functioning but also demonstrate its significance in promoting organized and efficient programming. From creating simple class hierarchies to developing extensively linked use case scenarios, inheritance stands out as a cornerstone of effective code architecture. Instead of reinventing the wheel each time, leveraging inheritance allows developers to focus on innovation without getting lost in the maze of redundancies.
Practical Examples Illustrating Polymorphism
Polymorphism plays a vital role in Java programming, allowing objects to be treated as instances of their parent class rather than their actual class. This is especially crucial when building large systems where flexibility and maintainability are desired. By employing polymorphism, developers can write more dynamic and reusable code, which leads to easier modifications and upgrades. Throughout this section, we will dive into practical examples that illuminate the intricacies of method overloading and method overriding, showcasing how they promote a robust coding environment.
Demonstrating Method Overloading
Method overloading is a cornerstone of compile-time polymorphism. The idea is simple yet powerful: you can create multiple methods with the same name in a class, as long as they have different parameter lists. This allows the programmer to use the same method name to apply to different types of inputs without worrying about the method name cluttering the code.
For instance, consider a class called . It could have multiple methods, capable of handling different types and numbers of arguments. Here’s a practical illustration:
In the example above, the class has three overloaded methods. Depending on the parameters you provide, Java will automatically pick the appropriate method. This capability offers both clarity and succinctness in your code — no need to remember a unique method name for each addition variant. As a result, method overloading enhances readability and simplifies the learning curve for new developers.
Demonstrating Method Overriding
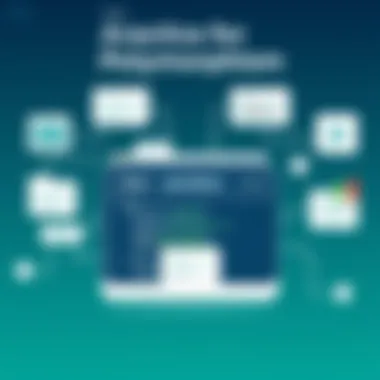
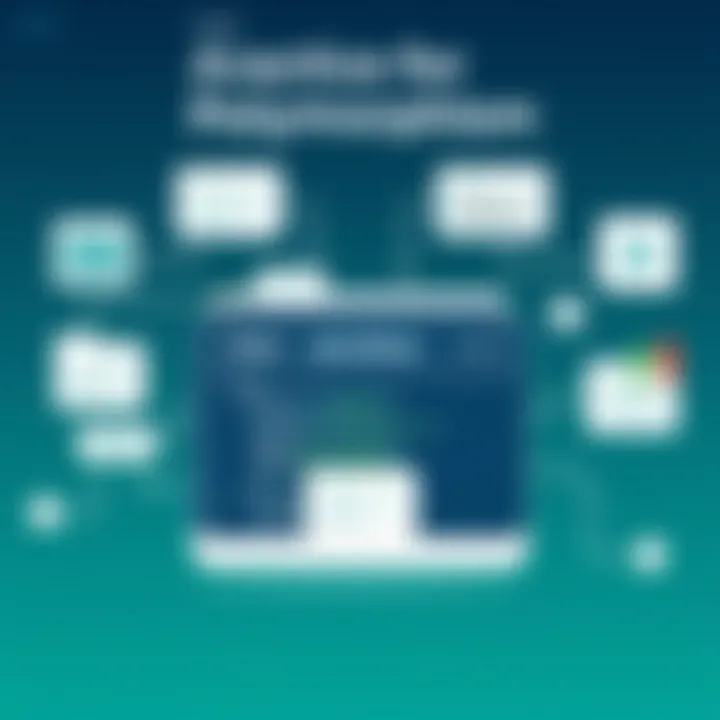
Method overriding takes a different approach. This technique comes into play when a subclass provides a specific implementation of a method already defined in its parent class. With this, the subclass can enhance or replace the behavior of that method, allowing for dynamic method resolution during runtime.
Let’s consider a base class called and a derived class that overrides the method. This is how it might look:
In this snippet, we define a general class with a method . The class, which inherits from , overrides this method to provide a more specific implementation. When we create an instance of and assign it to an reference, calling the method leads to the 's implementation executing. This behavior is known as runtime polymorphism, where the method that gets executed is determined at runtime, based on the object type rather than the reference type.
Polymorphism enables one interface for multiple implementations, promoting flexibility and reducing code complexity.
In summary, both method overloading and overriding illustrate the power of polymorphism in Java. They enable developers to write cleaner, more intuitive, and less redundant code. When used judiciously, these techniques foster a programming approach where extensions and modifications can be performed with minimal friction, essentially preparing the ground for robust software design.
Best Practices in Using Inheritance and Polymorphism
In the realm of object-oriented programming, the concepts of inheritance and polymorphism stand as fundamental pillars. Employing these features effectively can lead to more robust and maintainable code. Understanding and implementing best practices regarding inheritance and polymorphism not only enhances code flexibility but also promotes reusability. When faced with the intricate idiosyncrasies of these concepts, following certain guidelines can help to avoid common pitfalls.
Guidelines for Effective Inheritance
When it comes to inheritance, simplicity rules the roost. Keeping your class hierarchy shallow can prevent a slew of complications. Here are a few points to lay down some guidelines:
- Favor Composition over Inheritance: Often, you'll find that relying on composition (using objects of other classes) can be more beneficial than deep inheritance hierarchies. This also aids in code clarity and maintainability.
- Use Abstract Classes Wisely: Abstract classes provide a partial implementation that can be shared. They serve as a great foundation, but they should not become the crutch that everyone depends on. It’s prudent to limit their use to true abstractions.
- Avoid the Fragile Base Class Problem: Changes in a base class can inadvertently affect derived classes. Thus, be careful when modifying the base class. Occasionally, a new derived class may be a better choice rather than altering existing ones.
- Limit the Visibility of Constructors: Constructors in base classes should be protected or private whenever possible. This way, they can only be accessed within their family, avoiding unintentional misuse.
- Document Inheritance Relationships: Maintaining clear documentation about the inheritance structure can clarify how classes relate to one another. This practice aids in understanding and navigating the codebase more efficiently.
"Well-structured inheritance can lead to clean, understandable code, while overusing it can turn a manageable project into a tangled mess."
Staying aligned with these guidelines can significantly boost the efficacy of your inheritance implementation.
Leveraging Polymorphism for Code Flexibility
Polymorphism offers the flexibility to work with multiple forms of an object, expanding the ability to adapt to changing requirements. By embracing polymorphism, developers can write more generic, reusable code, thus enhancing adaptability. Here’s how to do it:
- Embrace Interface-Driven Design: Define interfaces that describe expected behaviors rather than specifying concrete implementations. By programming to an interface, you gain flexibility in how classes are implemented.
- Utilize Method Overriding: This allows a derived class to provide a specific implementation of a method already defined in its base class. Employ this feature strategically to tailor the inherited behavior, making your classes more versatile.
- Combine with Dependency Injection: Implementing dependency injection with polymorphic behavior can enhance testability and separation of concerns. It aids in creating more loosely coupled systems.
- Test for Behavioral Consistency: When using polymorphism, ensure that subclasses adhere to the expected behavior of their superclasses. By maintaining a consistent contract, you reduce the risk of unexpected behavior in your application.
By aligning these practices, developers can cultivate a coding environment where inheritance and polymorphism are not just concepts to understand but are tools that propel projects forward, enabling both change and continuity in the software development process.
Comparative Analysis of Inheritance and Polymorphism
In the realm of software development, inheritance and polymorphism stand as foundational concepts in object-oriented programming. Their practical applications, when deftly understood and implemented, can significantly enhance the efficiency and maintainability of code. In this segment, we will unravel the intricate relationship between these two concepts, highlighting the benefits they bring to developers and the considerations they pose.
Understanding Their Interdependence
At a glance, inheritance and polymorphism may seem like independent entities within Java's framework. However, a deeper examination reveals a synergy that cannot be overlooked. Inheritance allows one class to inherit fields and methods from another, creating hierarchies that can aid in code organization. Polymorphism, on the other hand, provides a mechanism to invoke methods dynamically, enabling objects to process actions in a manner tailored to their specific implementations.
For instance, consider a base class called Animal, which has a method speak(). Different animal classes such as Dog and Cat can inherit from the Animal class, overriding the speak() method.
When you call the speak() method on an object of type Animal, the actual method executed depends on the object type rather than the reference type. This dynamic behavior encapsulates the essence of polymorphism and showcases how inheritance lays the groundwork for it. Such interdependence emphasizes the importance of structuring classes wisely and recognizing that well-implemented inheritance can unlock robust polymorphic capabilities.
Common Misconceptions and Clarifications
Despite their importance, misconceptions about inheritance and polymorphism abound. It is crucial to address these myths to pave the way for a clearer understanding among developers.
- Misconception 1: Inheritance always leads to better code reusability.
- Misconception 2: All polymorphism is about inheritance.
- While inheritance enhances code reuse, it also introduces complexities. Over-reliance on inheritance may lead to tightly coupled classes, making maintenance tedious. In such cases, composition might be a more prudent choice.
- Not necessarily. While compile-time polymorphism is achieved through method overloading within the same class, runtime polymorphism relies on establishing a parent-child relationship via inheritance. Understanding both types can lead to better coding habits.
End and Future Perspectives
In closing, the exploration of inheritance and polymorphism within Java sets the stage for a deeper understanding of object-oriented programming. These concepts are not just design choices but vital tools that enhance code reusability, scalability, and readability. For software developers, grasping these principles means writing code that is not only efficient but also easier to maintain and extend over time.
Key Takeaways on Inheritance and Polymorphism
- Enhances Reusability: Inheritance allows developers to create new classes based on existing ones, leading to a reduction in redundancy. By leveraging a simple parent-child hierarchy, one can extend or modify behavior without rewriting code.
- Increases Flexibility: Polymorphism provides the ability to define a single interface and have multiple implementations. This means, for example, that a method can accept objects of different types, enriching the code's adaptability to future requirements.
- Encourages Best Practices: Both inheritance and polymorphism promote a cleaner, more organized codebase. By adhering to design principles like SOLID, developers can avoid common pitfalls such as the fragile base class problem, where a minor change can cascade through a system and cause breakage.
- Interoperable Systems: With these principles, systems can communicate effectively. The relationship built through inheritance allows various classes to work together harmoniously, while polymorphism ensures that objects can interact in predictable ways.
Emerging Trends in Object-Oriented Programming
As the landscape of software development evolves, so do the practices surrounding object-oriented programming. A few trends worth noting include:
- Increased Use of Functional Programming: While still respecting principles of inheritance, many developers are blending object-oriented paradigms with functional programming techniques, leading to more expressive and concise code.
- Focus on Microservices: As organizations shift toward microservices architecture, the concepts of inheritance and polymorphism play a crucial role in designing services. They help manage shared code across multiple services while maintaining clear interfaces.
- Rise of Static Typing: Languages that enforce strong typing, while also allowing inheritance and polymorphism, are on the rise. Typescript, for instance, offers a type system with class-based inheritance that helps reduce runtime errors, enhancing code safety.
In summary, mastery of inheritance and polymorphism is no longer an optional skill in a developer’s toolkit but a necessity. With the changing tides of technology and best practices, staying current with these concepts will continue to be pivotal for building modern, robust applications.