Understanding GC Collect: Mechanisms and Best Practices
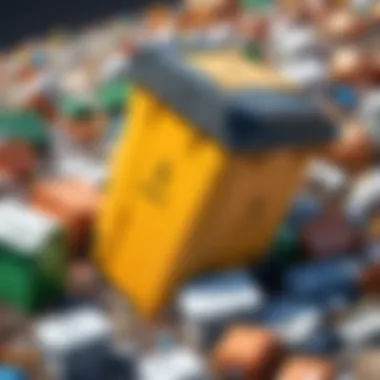
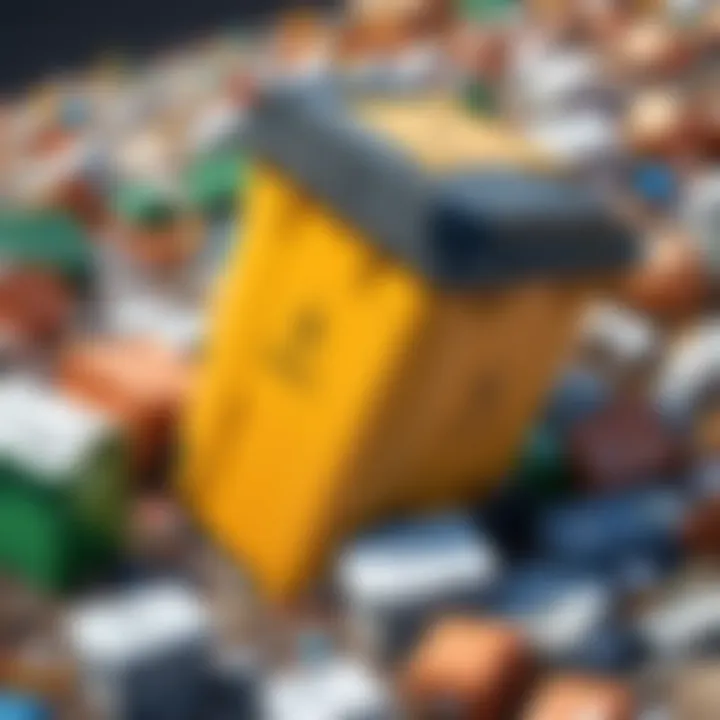
Intro
Garbage collection is an essential aspect of modern programming, particularly in high-level languages. The process helps manage memory automatically, which can significantly ease development overhead. Among various garbage collection techniques, the GC collect process plays a crucial role in ensuring efficient memory usage. Understanding its mechanisms is not just beneficial for system performance but also for maintaining healthy application behavior.
As applications grow in complexity, the implications of ineffective memory management become more pronounced. Memory leaks, performance bottlenecks, and application crashes can all stem from inadequate understanding of garbage collection. This article explores the intricacies of GC collect, examining how it functions, its performance implications, and best practices for developers aiming to optimize memory management within their applications. The following sections will provide a detailed analysis of algorithms, trade-offs, and common challenges in garbage collection, ultimately offering a comprehensive guide to effective usage of GC collect.
Preface to Garbage Collection
Memory management is a critical aspect of software design and development. Proper memory management ensures the effective utilization of system resources, impacts application performance, and enhances the overall user experience. Garbage collection plays a central role in memory management by automating the process of reclaiming memory that is no longer in use. This prevents memory leaks and fragmentation, which can severely degrade performance over time.
Garbage collection simplifies the coding process for developers by relieving them from the burden of manual memory management. However, this technological convenience comes with certain costs, primarily in performance overhead. Understanding how garbage collection operates, its implications for application performance, and best practices for its optimization is essential for developers striving to create efficient code.
The Importance of Memory Management
Memory management is fundamental to programming and system design. Every application requires memory to operate effectively. Poor memory management can lead to a range of issues such as crashes, performance bottlenecks, and inefficient resource usage. These problems can stem from memory leaks, where allocated memory is not properly released, leading to eventual exhaustion of memory resources.
In today’s computing landscape, where applications demand more memory due to complexity and functionality, maintaining optimal memory usage is crucial. Garbage collection addresses these challenges by automating the detection and reclamation of unused memory. This represents a significant advantage, particularly for complex applications that manage large datasets, as it reduces the risk of human error and enhances reliability.
Overview of Garbage Collection
Garbage collection refers to the automatic process of identifying and freeing memory that is no longer needed by a program. Without it, developers would need to manually manage memory allocation and deallocation, which can introduce errors and efficiency issues. Garbage collectors work in the background to monitor objects and references in memory, freeing up resources that are unreachable.
There are various garbage collection algorithms, each with its own principles and methodologies. These algorithms vary in their efficiency and the timing of collection processes, affecting application responsiveness and performance. By understanding the different types of garbage collection mechanisms, developers can make informed decisions on which methods align best with their application needs and performance objectives.
In summary, a robust understanding of garbage collection is vital for developers. It equips them with the knowledge to optimize memory management, improve application performance, and create more efficient, reliable software.
What is GC Collect?
In the realm of programming and application development, understanding the mechanics behind GC Collect is crucial. This segment explains the definition and purpose of GC Collect, as well as how this process operates. Grasping these elements not only aids developers in optimizing their applications but also enhances the overall performance and efficiency of memory usage.
Definition and Purpose
In simple terms, GC Collect refers to the Garbage Collection process used by various programming languages and platforms to manage memory automatically. The primary purpose of GC Collect is to reclaim memory that is no longer in use, thus freeing up resources for new objects and preventing memory leaks.
Memory leaks occur when the application continues to use memory that is no longer needed. This leads to performance degradation over time, sometimes causing applications to crash or slow down significantly. GC Collect helps in mitigating these risks by regularly identifying and releasing memory held by objects that are no longer referenced.
Understanding GC Collect is not just for theoretical knowledge; it has practical implications in real-world application development. By allowing developers to focus on writing code instead of managing memory manually, GC Collect facilitates the creation of more reliable and stable software. Yet, it also calls for an understanding of when the garbage collector runs, as this can affect application performance. For example, if an application relies on GC Collect at critical points, it might suffer from spikes in latency during collection cycles.
How GC Collect Works
The operation of GC Collect hinges on an intricate set of algorithms that identify unused memory and recover it. The most prevalent algorithms include mark-and-sweep and reference counting. The working of these algorithms can depend heavily on the programming language being used.
With mark-and-sweep, the garbage collector first 'marks' all the objects that are still in use. It then 'sweeps' through memory, reclaiming space from objects that were not marked. This process can be resource-intensive, often leading to noticeable latency during application execution.
In contrast, reference counting involves keeping track of the number of references to an object. When no references exist, the garbage collector can immediately reclaim the associated memory. While this technique is more efficient in terms of immediate memory recovery, it does not handle circular references well.
Properly deploying GC Collect involves understanding the dynamics between system resources and application performance. Developers must gauge when to utilize manual memory management versus relying on automatic garbage collection.
The careful balance between memory management strategies can significantly influence application reliability and performance.
Ultimately, GC Collect serves as a vital tool in robust software development, enabling better memory management and application efficiency. By comprehensively understanding GC Collect’s definition, purpose, and operational mechanisms, developers can systematically optimize their applications and reduce common pitfalls associated with manual memory allocation.
Types of Garbage Collection Algorithms
Understanding the various types of garbage collection algorithms is crucial for software developers and IT professionals who want to manage memory effectively. Each algorithm comes with its own set of benefits and downsides. Selecting the right one can significantly affect the performance of applications, influencing factors such as speed, memory usage, and overall responsiveness.
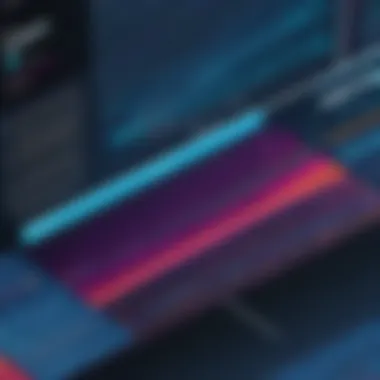
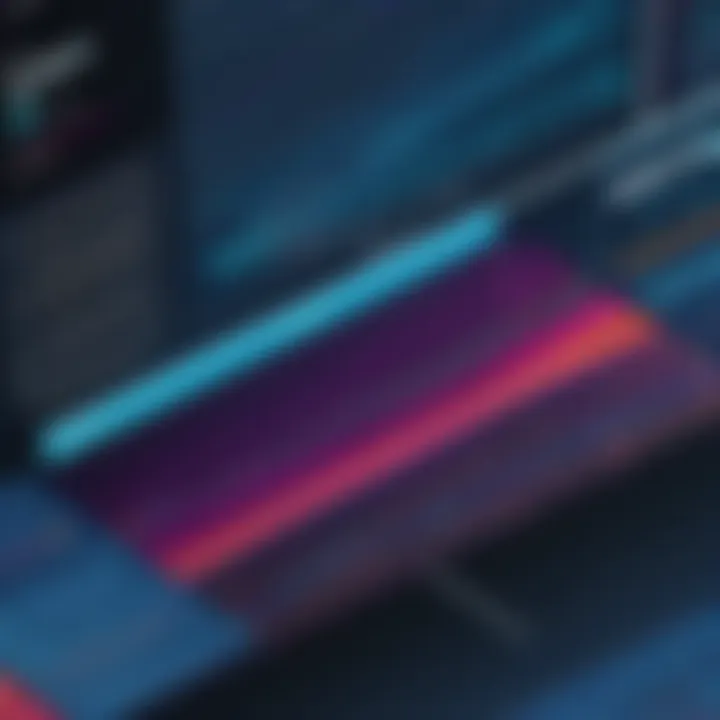
Mark-and-Sweep
Mark-and-sweep is a fundamental garbage collection algorithm characterized by its straightforward approach. During the first phase, known as the mark phase, the algorithm identifies reachable objects in the memory from root references like global variables or active threads. Objects that can be accessed are marked. After this, the algorithm moves to the sweep phase, where it traverses the memory and collects unmarked objects for reclamation.
This method has notable benefits. For instance, it can effectively manage memory without fragmenting it, as all reclaimable space can be coalesced into larger blocks. However, it does have some downsides. The algorithm may lead to long pause times since it needs to traverse the entire heap to mark and sweep, affecting application performance, especially in real-time systems.
One must also consider the overhead during the marking process, which can slow down applications that require consistent response times.
Generational Garbage Collection
Generational garbage collection is based on the hypothesis that most objects die young. This strategy divides the heap into two or more generations. Young generations hold newly allocated objects, and older generations store long-lived objects. The algorithm frequently collects the young generation since many objects become obsolete quickly.
The benefit of this approach is that it improves performance significantly by reducing the need to scan the entire heap frequently. It minimizes pauses by only focusing on newly created objects, which are often more numerous in terms of allocation. Moreover, by promoting objects to older generations, the algorithm minimizes the effort spent on them in future collection cycles.
However, the generational garbage collection does have some trade-offs. The promotions between generations can lead to overhead, and if many objects survive the young generation collection, the older generation may become cluttered, leading to longer collection pauses in the future.
Reference Counting
Reference counting is a technique that keeps track of the number of references to each object in memory. When an object is referenced by another, its count is incremented. Conversely, when a reference is deleted, the count is decremented. Once an object's reference count hits zero, it is deemed unreachable and can be collected.
This algorithm provides immediate reclamation of memory, which can lead to better performance in scenarios where short-lived objects are common. Additionally, it can allow more predictable memory usage, as the reclaimed memory happens without needing long pauses.
However, there is a significant limitation. Circular references among objects can lead to memory leaks since the reference counts may never reach zero. As a result, programmers must often employ strategies to manage such cases, which can add complexity to code management.
Performance Implications of GC Collect
Understanding the performance implications of GC collect is crucial for any developer working with memory management. Garbage collection, while invaluable for automating memory handling, often introduces performance overhead. It can affect the responsiveness of applications, which can be particularly detrimental in performance-critical environments such as gaming or financial services. Developers must strike a balance between memory safety and application performance to ensure that applications run smoothly.
Impact on Application Performance
The impact of GC collect on application performance can be wide-ranging. One primary concern is the potential for "stop-the-world" events. During these events, the application pauses while garbage collection occurs, which can disrupt user experience. Depending on the frequency and duration of these pauses, users may perceive the application as laggy or unresponsive.
When evaluating the impact on performance, consider the following factors:
- Memory Footprint: A larger memory footprint can lead to more frequent collections. Heap size directly correlates with GC activity; if an application uses memory heavily, it triggers GC to free unused objects, possibly leading to more frequent interruptions.
- Allocation Rate: Higher rates of memory allocation can increase pressure on the garbage collector. High allocation rates can cause increased GC cycles, leading to measurable performance degradation.
- Application Architecture: Some architectures lend themselves better to optimized garbage collection. Leveraging better design can reduce the impact on performance.
To mitigate adverse effects on performance, developers often need to perform careful profiling, including measuring the pauses introduced by GC. Utilizing tools like GC log analyzers can offer insights into memory usage patterns and potential areas for improvement.
Latency Considerations
Latency is a critical metric when considering GC collect implications. The very nature of garbage collection can introduce unpredictable latency, depending on how well the collector is tuned for specific workloads.
Developers must think about how GC latency affects overall latency metrics for their applications. High latency during critical operations can lead to negative user experiences. Here are some common latency considerations:
- Real-time Requirements: Applications that require real-time performance must minimize GC latency. This often involves using specialized algorithms or collectors designed for low-latency scenarios, such as the Z Garbage Collector (ZGC) implemented in Java.
- Tune GC Behavior: Adjusting settings such as heap size or the frequency of collections can help balance latency with performance. Finding the right configuration can vary significantly based on workload characteristics and should be performed with profiling in mind.
- Testing and Monitoring: Continuous monitoring of GC pauses can provide insights into latency issues. Employing monitoring solutions that track garbage collection behavior in production can help identify when latency peaks that disrupt the user experience.
In summary, GC collect has direct implications for application performance and latency. A deeper understanding of these implications allows developers to architect applications in a way that minimizes negative impacts, ensuring smoother user experiences.
Optimizing Garbage Collection Performance
Optimizing garbage collection performance is crucial for ensuring that applications run efficiently and effectively. Proper optimization can lead to significant improvements in speed and responsiveness, particularly in memory-intensive applications. By understanding the nuances of garbage collection, developers can fine-tune their applications, thereby minimizing the adverse effects that can arise from improper memory management.
Tuning GC Parameters
Tuning GC parameters is one of the most effective ways to enhance garbage collection performance. Different programming environments provide various options for configuring garbage collection behavior. Understanding the available parameters is essential for achieving optimal performance. Here are some strategies to consider:
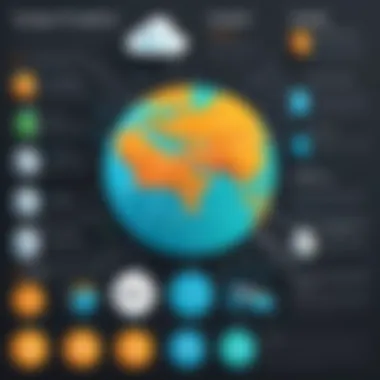
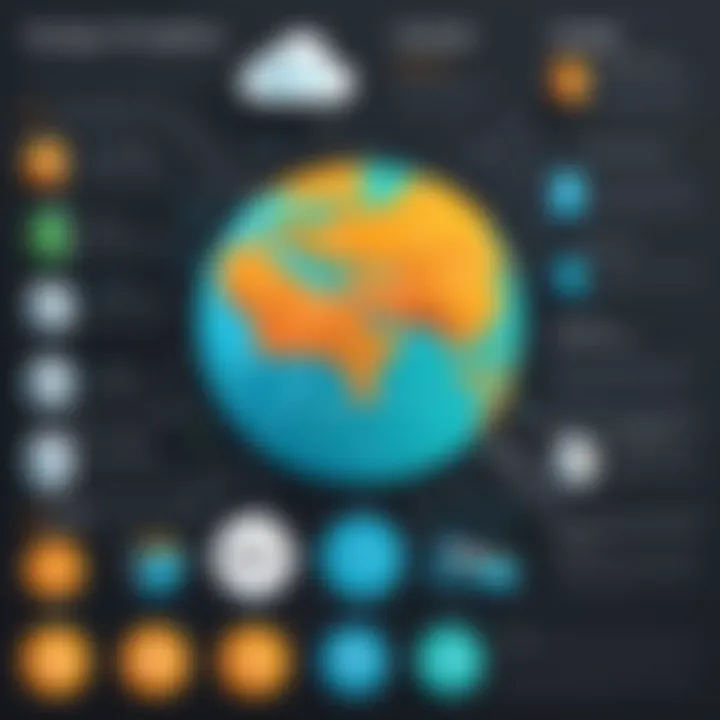
- Heap Size Management: Adjusting the heap size can influence how frequently garbage collections occur. A larger heap can reduce the frequency of collections, while a smaller heap might lead to more frequent but shorter collections.
- GC Algorithm Selection: Each application has unique needs, making it essential to choose the right garbage collection algorithm. For instance, applications that require lower latency might benefit from using concurrent garbage collectors that minimize pause times.
- Pause Time Goals: Setting explicit goals for pause times can guide the tuning process. Developers can leverage profiling tools to measure the impact of their settings and make adjustments based on empirical data.
By systematically experimenting with these parameters, developers can tailor garbage collection to align with their application's specific needs.
Memory Allocation Strategies
Memory allocation strategies play a significant role in optimizing garbage collection performance. Effective memory management reduces the load on the garbage collector, improving application responsiveness. Consider the following strategies:
- Object Pooling: Reusing existing objects can prevent excessive allocation and deallocation, minimizing the frequency of garbage collections. This method is particularly useful for objects that are expensive to create and can be reused across multiple requests.
- Size and Lifetime Awareness: Understanding object size and expected lifespan allows for more informed allocation decisions. If certain objects are short-lived, it makes sense to allocate them in a way that reduces the garbage collector's workload.
- Avoiding Temporary Objects: Frequent creation of temporary objects can lead to increased garbage collection pressure. Developers should be cautious about creating unnecessary temporary instances, especially in high-frequency code paths.
Adopting a thoughtful approach to memory allocation can lead to less strain on the garbage collector, improving overall application performance.
Optimizing garbage collection requires an understanding of both GC parameters and memory allocation strategies. These two aspects work hand in hand to create a more efficient memory management environment.
Common Challenges and Solutions
Understanding the challenges associated with GC collect is crucial for developers and IT professionals alike. These challenges not only affect application performance but also impact user experience. Addressing these issues effectively can lead to smoother applications and optimized resource management. This section will cover two major challenges: long GC pause times and memory leaks.
Long GC Pause Times
One significant challenge inherent in garbage collection is long GC pause times. During a garbage collection cycle, the application may become unresponsive as the system reclaims memory. These pauses can be especially pronounced in applications requiring real-time processing or handling large datasets.
- Implications: Long GC pauses can lead to delayed transactions, increased latency, and ultimately, user dissatisfaction. Applications that demand high performance must manage these pauses to ensure seamless operation.
- Factors Contributing to Long GC Pauses:
- Heap Size: A larger heap can lead to longer garbage collection times due to increased complexity in identifying unreachable objects.
- Object Allocation Rate: A high rate of object creation can increase the frequency of garbage collection cycles.
- Algorithm Choice: Different garbage collection algorithms have varying techniques and trade-offs regarding pause times.
Ways to mitigate long GC pauses include tuning GC parameters to optimize the process, using generational garbage collection algorithms that can reduce pause times, and implementing better memory allocation strategies. By doing so, developers can create applications that are responsive and aware of user needs.
Memory Leaks
Memory leaks pose another critical challenge to garbage collection strategies. These occur when allocated memory is not properly released even after it is no longer in use. Consequently, the application continues to hold onto this memory, leading to inefficient memory use and eventual application crashes.
- Causes of Memory Leaks:
- Impact on Applications: Over time, memory leaks can exhaust system resources, causing the application to slow down or crash altogether. The implications are severe in long-running applications, such as web servers or background processing systems.
- Poor Reference Management: Holding references to objects unnecessarily can prevent proper garbage collection.
- Static Field References: Objects retained in static fields can lead to memory leaks since they are never eligible for garbage collection.
Strategies to Combat Memory Leaks:
- Code Review: Regularly auditing the code can help identify sections where references are not released.
- Using Weak References: Employ weak references to allow objects to be garbage collected when memory is required.
- Profiling Tools: Utilizing profiling tools, such as VisualVM or Eclipse Memory Analyzer, can aid in detecting memory leaks before they escalate.
By recognizing and addressing these challenges, developers can enhance the performance and reliability of their applications, making them more robust against the potential pitfalls of poor memory management.
Measuring Garbage Collection Impact
Measuring the impact of garbage collection is crucial for developers and IT professionals. It serves as a means to gauge how effectively memory is being managed in applications. A well-measured GC process not only identifies performance bottlenecks but also informs decisions about optimization. Understanding this impact aids in refining applications to meet performance goals while ensuring resource efficiency.
The assessment of garbage collection involves examining various factors. These factors play a significant role in the overall application performance. By measuring garbage collection, developers gain insights into:
- Memory Usage: Understanding how much memory is utilized and when it is freed can help in optimizing applications.
- Pause Times: Having awareness of how long the GC process interrupts application execution is vital for maintaining user experience.
- Allocation Patterns: Recognizing allocation rates can reveal the efficiency of memory utilization.
Tools and Techniques for Measurement
There are numerous tools and techniques available for measuring garbage collection impact. Employing these can greatly simplify the process for developers. Here are some of the notable tools:
- Java VisualVM: This tool provides insights into JVM performance, including memory utilization and garbage collection events. It is particularly useful to profile applications in real-time.
- GC Viewer: GC Viewer is an analysis tool that visualizes garbage collection logs. It helps in understanding the behavior of the GC over time.
- JProfiler: This is a commercial tool that offers detailed profiling capabilities, including garbage collection metrics, and CPU analysis.
- YourKit: Similar to JProfiler, YourKit is another powerful profiler that allows users to analyze memory usage and detect potential memory leaks.
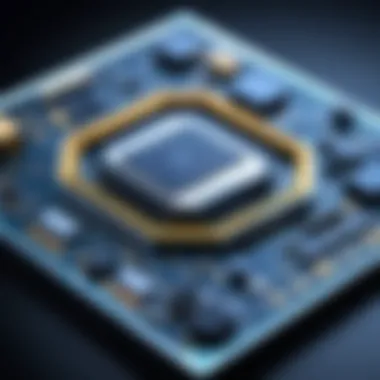
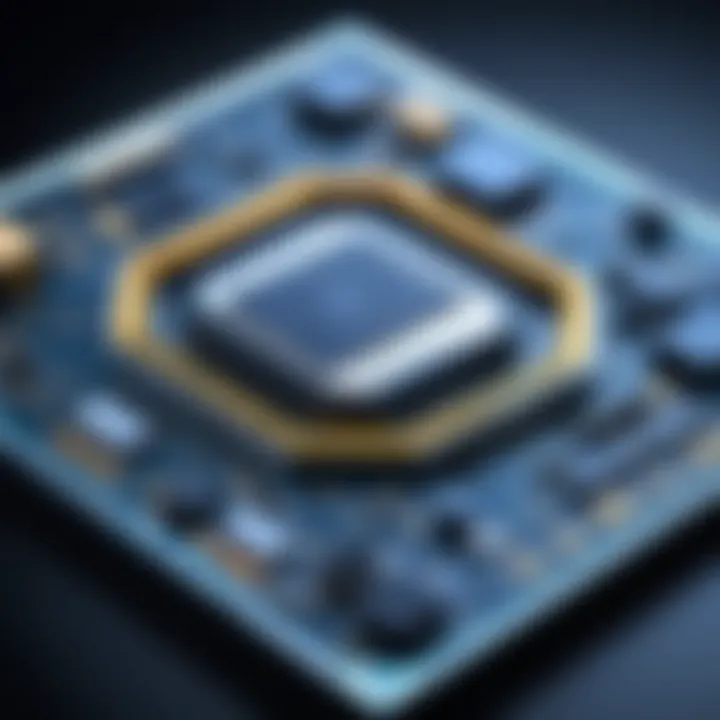
These tools provide various data points such as frequency of garbage collection, size of the heap before and after collections, and duration of pauses, among others.
Developers should choose the right tools based on their specific needs and the programming environment they are working in.
Analyzing GC Logs
The analysis of garbage collection logs provides deeper insights into the efficiency and effectiveness of the garbage collector. A common approach is to enable detailed GC logging, allowing developers to trace GC activity over time.
When examining these logs, the following aspects are important:
- GC Event Types: Distinguishing between minor and major collections can help in understanding memory management better.
- Pause Duration: Observing how long each collection pauses application threads is essential to gauge performance impact.
- Memory Utilization Trends: Tracking changes in memory usage before and after collections can reveal how well the GC is performing its job.
In a sample log format, the output may include:
Here, one can identify the memory status before and after the garbage collection has occurred and the time it took to complete.
Analyzing GC logs systematically helps to identify patterns and recurring issues, ultimately leading to informed tuning and optimization efforts.
In summary, measuring garbage collection impact is a vital aspect of maintaining high-performance applications. With the right tools and methodologies, developers can optimize the garbage collection process, ensuring efficient memory management and a seamless user experience.
Future Trends in Garbage Collection
The evolution of garbage collection (GC) is vital as programming paradigms and application demands change. With increasing complexity in software and the push towards more efficient resource utilization, understanding future trends in garbage collection becomes crucial for developers and IT professionals. This section will explore emerging algorithms and techniques in GC, as well as their integration into cloud computing environments. By recognizing these trends, developers can make informed decisions that enhance the performance and efficiency of their applications.
Emerging Algorithms and Techniques
As the landscape of software application continues evolving, new challenges arise that traditional garbage collection methods may not adequately address. Emerging algorithms aim to tackle these challenges effectively. One significant trend is the focus on concurrent garbage collection. This approach allows garbage collection to occur simultaneously with application threads, minimizing pause times and improving overall throughput.
Another approach gaining traction is the use of machine learning in garbage collection algorithms. These algorithms can learn from application behavior over time, optimizing memory management based on patterns. For example, predicting memory allocation and deallocation can lead to more efficient resource allocation and quicker GC cycles.
Hierarchical garbage collection is also emerging. This technique organizes memory into multiple layers, optimizing the collection process by prioritizing areas of memory that are more likely to be reclaimed. Different areas of memory can be collected based on specific criteria, helping to minimize impact on the performance level of the application.
Developers should keep an eye on these algorithmic developments, as they offer potential enhancements to memory management efficiency, which can contribute to the overall performance of applications.
Integration with Cloud Computing
The rise of cloud computing presents unique challenges and opportunities for garbage collection. Cloud environments often operate at scale, employing distributed systems that require efficient memory management across different nodes. Future trends in garbage collection must consider these architectures.
One significant change is the need for garbage collection strategies that are optimized for cloud-native applications. In this context, techniques such as sharding and partitioning can be helpful. By dividing memory management tasks across distributed services, the pressure on single nodes is alleviated, resulting in better overall system performance.
Additionally, serverless computing requires a rethink of garbage collection. In these environments, applications may spin up and shut down rapidly. Garbage collection in such contexts must be agile, adapting to the instantiation and disposal of functions effectively. As more applications shift to serverless architectures, optimizing GC for these use cases will become essential.
Furthermore, as multi-cloud strategies become prevalent, understanding how different cloud providers implement garbage collection can help organizations choose the right architectures and configurations for their needs.
"The transition to cloud computing not only changes how applications are built but also significantly influences how these applications manage resources, including memory."
Epilogue
Garbage collection is a crucial aspect of memory management that impacts software performance and resource utilization. The conclusion of this article serves as a reflective summary on the importance and implications of GC collect. It reinforces the necessity for software developers and IT professionals to grasp the mechanisms and strategies associated with this process.
Understanding garbage collection allows developers to write more efficient code by minimizing memory leaks and reducing application downtime. With optimizing memory allocation strategies and tuning GC parameters, professionals can significantly enhance the efficiency of their applications.
This article synthesizes key concepts and practices involved in GC collect, allowing readers to form coherent strategies in their work. Recognizing the balance between the algorithmic choices for garbage collection and their corresponding performance impacts is more than beneficial; it is essential in the development of modern software applications.
Recap of Key Points
- Importance of Memory Management: Memory management plays a vital role in ensuring applications run smoothly and efficiently.
- Understanding GC Collect: GC collect is a process designed to reclaim unused memory, which directly impacts software performance.
- Garbage Collection Algorithms: Various algorithms, such as Mark-and-Sweep and Generational Garbage Collection, have unique strengths and weaknesses that affect their implementations.
- Performance Implications: Garbage collection can cause application pauses that may disrupt user experience, making latency considerations crucial.
- Optimization Techniques: Developers can enhance GC performance by tuning parameters and employing effective memory allocation strategies.
- Challenges and Solutions: Addressing long GC pause times and memory leaks is critical for optimal application performance.
- Measuring Impact: Tools for measuring garbage collection impact aid in understanding application behavior and optimizing performance.
- Future Trends: The future holds potential for advanced algorithms and integration with cloud technologies enhancing GC mechanisms.
Final Thoughts on GC Collect
In summary, GC collect is not merely an execution detail but a fundamental pillar of effective memory management in modern programming. Its merits and pitfalls must be understood deeply. The trade-offs between various algorithms should be evaluated in context. For developers, this means staying current with trends, challenges, emerging techniques, and best practices.
The key takeaway is to approach garbage collection with a proactive mindset. Adequate knowledge of GC mechanisms and implications empowers professionals to anticipate challenges and implement solutions effectively.