Mastering For Loops in Python: An In-Depth Exploration
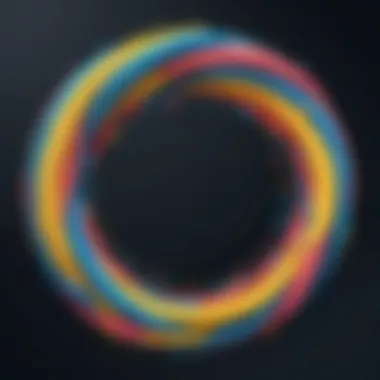
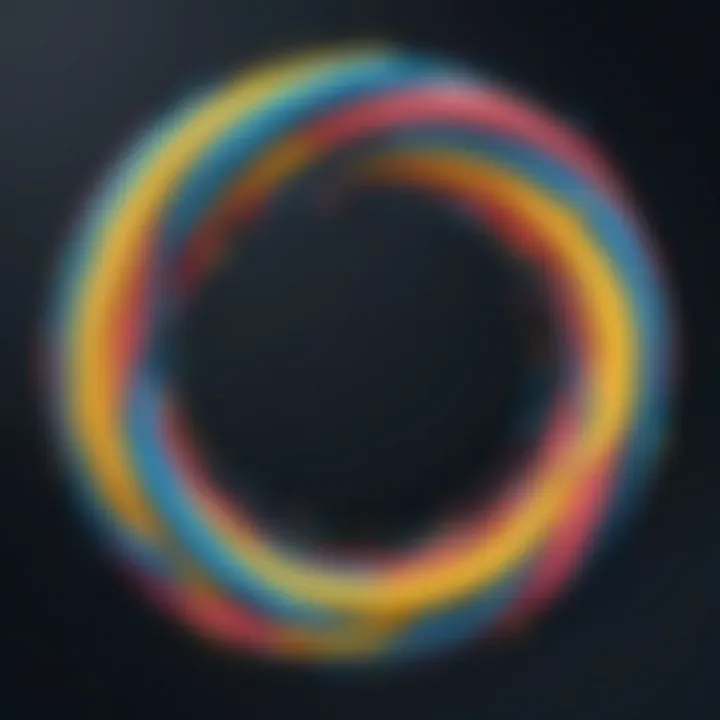
Intro
In modern programming, understanding loops is vital. They automate repetitive tasks and make code more efficient. Among these, for loops in Python are simple yet powerful. This guide will walk through their structure, usage, and understanding challenges.
For loops in Python control the flow of execution by iterating over a sequence, like a list or a range. This enables developers to handle tasks dynamically, without hardcoding values. Efficient use of for loops can enhance readability and performance in code, which are key skills in software development.
In this comprehensive exploration, we will cover everything from basic structure to complex use cases. As you read ahead, consider different scenarios where for loops make coding streamlined and clean.
Overview of For Loops
Definition and Importance
For loops bond closely with other programming languages as they elegantly handle collection iterations. In Python, their significance is paramount, simplifying loops with straightforward syntax. As developers, grasping this concept enables one to manage data seamlessly.
Key Features and Functionalities
- Readable Syntax: Python offers clear syntax for initializing and controlling loops, leading to lower error rates.
- Iterables: It works richly with all types of iterables, like strings and dictionaries, expanding its versatility.
- Control Structures: Incorporates control statements for more dynamic code flow.
Use Cases and Benefits
- Data analysis: When analyzing lists of values swiftly.
- Automation: Facilitating repetitive tasks in decisions and calculations.
- Game Development: To quickly process object states or sequences of events.
Best Practices
Adhering to best practices when implementing for loops can improve code quality drastically.
- Keep it Simple: Avoid complex nested loops. If doable, refactor the code.
- Use Enumerate: Utilize the function for obtaining index and value simultaneously.
- Test for Edge Conditions: Ensure handling of lists with different lengths gracefully.
Common Pitfalls to Avoid
- Infinite Loops: Ensure your indexes work to terminate the loop.
- Off-by-One Error: Verify that ranges are effectively controlled.
Case Studies
In software building, agile teams have successfully implemented for loops.
- Data Aggregation: Teams aggregated sales data from various months, using for loops to summarize totals. The result showed that for appropriate iterables and structures, performance was calculated efficiently and accurately.
- Simulation: Simulations of daily operations in managing inventory cycles where coders noted reduced time costs open to increased effectiveness.
Preface to For Loops in Python
For loops in Python serve as a vital aspect of the programming language, facilitating repetitive execution of code blocks with efficiency. Understanding their structure and function is crucial for any coder aiming to create optimized and robust programs. Through for loops, developers can iterate over collections, engage with lists, and manage complex tasks with relative ease.
Understanding the Concept of Loops
Loops are foundational constructs in programming. They allow the execution of a block of code multiple times, which is essential for handling repetitive tasks. The concept of looping ensures that programmers can achieve iterations without the need to recode similar lines repeatedly.
The primary forms of loops in Python include for loops and while loops. A for loop specifically is designed for iterating over iterables, the details of which will be covered in later sections. Decomposing tasks into loop structures yields cleaner, more understandable code, and experimentation with them reveals their powerful capabilities.
Importance of For Loops in Programming
For loops have a significant role in the realm of programming for several reasons:
- Efficiency: They automate repetitive actions, saving time and minimizing errors compared to manual executions.
- Readability: Code that uses loops tends to be clearer and more maintainable. Rather than cluttering scripts with repetitive code, a single loop provides a succinct declaration of actions to be performed.
- Enhances Data Processing: Coders often utilize for loops to manipulate data within collections. They are especially valuable for operations on large datasets found in data analysis and manipulation with libraries like Pandas.
By mastering for loops, programmers significantly enhance their toolkits. As we explore this article, it will be solidified how essential they are in crafting high-quality software.
Syntax of a For Loop in Python
The syntax is what differentiates a correctly functioning loop from one laden with errors. Understanding the syntax of a for loop in Python is vital for effective programming. It defines how you instruct Python to repeat actions smoothly. By mastering this syntax, developers will find loops much easier to implement and troubleshoot, enhancing their overall coding efficiency. This segment will break down the basic structural elements and explain how to effectively use the syntax when writing loops.
Basic Structure of For Loop
The very basis of a for loop in Python consists of a specific structure. Broadly, the format is:
Here, variable represents each subsequent element in the iterable as the for loop progresses through its cycle. This can stand for a list, a string, or any collection. The yields from the iterable allow the programmer to run specified operations per element. The colon denote feelings of indentation which follows this line with commands that Python needs to execute. The code beneath this line signifies commands that will operate on each variable as they are accessed.
Looping Through a Sequence
Looping through a sequence is a fundamental application of for loops. A
Iterables in Python: A Closer Look
Understanding iterables is fundamental when using for loops in Python. They enable the for loop to function efficiently by providing a sequence or collection of items that can be traversed. Recognizing what constitutes an iterable can greatly enhance the programmer's ability to manipulate data structures effectively. This section will elucidate the definition of an iterable and outline some common iterables that are particularly useful in conjunction with for loops.
What is an Iterable?
An iterable in Python refers to any object that can return its elements one at a time. Common examples include lists, tuples, sets, dictionaries, and strings. To be considered iterable, an object must implement the method or alternatively, the method. This allows Python to generate an iterator from which elements can be accessed in a sequence.
Key Characteristics of Iterables:
- Sequence Access: Iterables provide a way to sequentially access their elements.
- Memory Efficiency: Many iterables, such as generators, are memory-efficient since they generate items on-the-fly without storing the entire set in memory.
- Flexibility: They allow the use of common constructs to process, filter, and organize data, especially when used with for loops.
By comprehending iterables, developers can write more adaptable and concise code, which may lead to increased productivity and clarity in the programming process.
Common Iterables Used with For Loops
For loops make heavy use of certain iterables in practical applications. Here we examine a selection of commonly utilized iterables that programmers interact with regularly:
- Lists: One of the most prevalent data structures. Lists can store ordered collections of arbitrary items.
- Tuples: Similar to lists but immutable. They serve well for cases where data should not change.
- Dictionaries: Offer key-value mappings. It is often the case to loop through keys, values, or items in a structure.
- Sets: Collections of unique items where order does not matter. Their use in looping can make certain types of operations more efficient relative to lists.
- Strings: Being iterable, strings allow character-level iteration, immensely useful in text processing.
Understanding these common iterables enables developers to leverage the full power of for loops, regardless if their task is data manipulation, iteration, or transformation.
Iterables are at the core of effective for loops and learning these concepts can hugely boost programming efficiency.
Using For Loops with Lists
For loops offer a profound versatility when it comes to interacting with lists in Python. A list is one of the basic data types and forms the foundation for many programming tasks. Understanding how to use for loops with lists not only enhances coding efficiency but also deepens your comprehension of list structures. With effective list iteration, developers can access, manipulate, and transform data seamlessly.
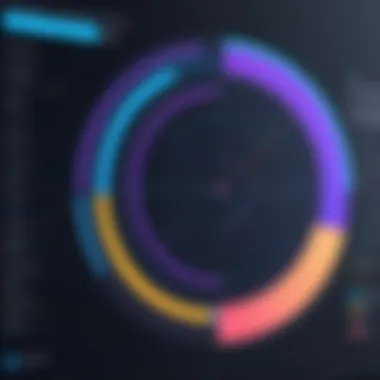
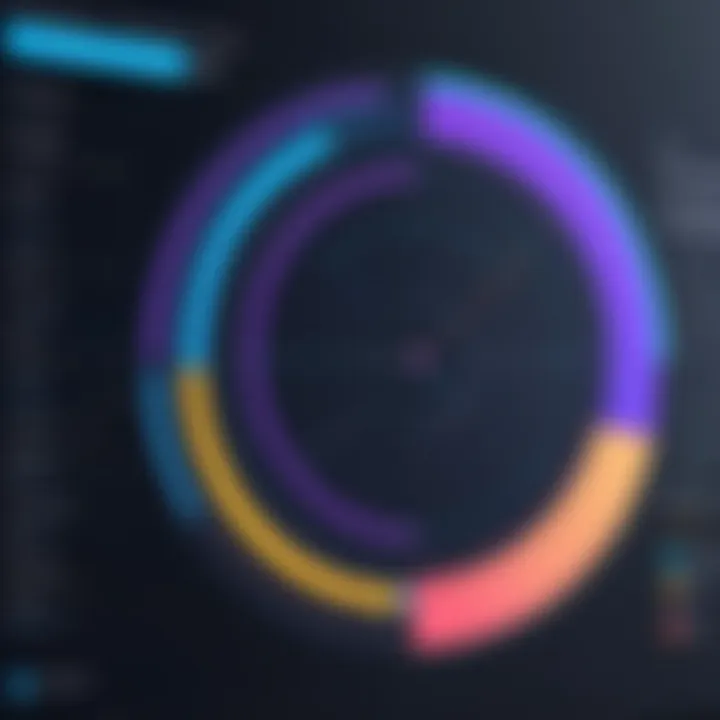
Basic List Iteration
The most straightforward application of a for loop with lists involves basic iteration. When using a for loop to iterate through a list, each element in the list becomes accessible sequentially. The syntax is clear and delivers readable code, maintaining ease of understanding.
In this snippet, the for loop simply prints each element in the list. Notice the clarity this brings; there is no ambiguity about which items are being processed. This method proves especially beneficial in numerous data manipulation tasks where efficiency is paramount.
Modifying Elements During Iteration
Modification of list elements during iteration is feasible but requires an awareness of implications. Directly altering list elements being iterated through can induce unexpected behavior. For example, consider this code:
The output will be . Utilizing the function gives access to elements by index. While this maintains the list's intended structure, care must be observed with modifying operations that affect the iteration’s inherent integrity.
Additionally, understanding loop scoping, how values are kept, is crucial. Is it possible to create a new list instead? A more Pythonic approach often resorts to list comprehensions to generate modified versions of lists without impacting the original data or creating potential side effects.
In summary, leveraging for loops with lists offers clarity and control over data. The iterative mechanics, whether for basic extraction or advanced manipulation, significantly elevate one’s programming expertise. Adhering to best practices in modification via planned approaches or employing list comprehensions can lead to more predictable code. Engaging with lists correctly informs you about larger data structure concepts that are universally applicable in Python programming.
For Loops with Dictionaries
For loops offer remarkable capabilities when working with dictionaries in Python. This section seeks to establish the significance of understanding how to utilize for loops effectively with dictionaries. The unique structure of dictionaries allows the programmer to manipulate pairs of data with ease. Employing for loops can streamline this manipulation process, ensuring efficiency and clarity.
Dictionaries are widely used in programming for storing data in a key-value format. Each key in a dictionary points to a specific value, enabling quick access and modification of the data. By mastering for loops with dictionaries, developers can enhance their ability to perform data analysis, automate repetitive tasks, and manage large datasets effectively.
Utilizing for loops with dictionaries brings several advantages, such as:
- It simplifies the process of accessing and manipulating data.
- The code becomes cleaner and more readable by removing the need for obtrusive indexing.
- It introduces the possibility for concise, repetitive operations on each key-value pair without additional complexities.
In summary, understanding for loops in the context of dictionaries reaffirms a developer's capacity to handle data efficiently.
Iterating Through Keys and Values
Iterating through the keys and values of a dictionary is a fundamental operation in Python programming. This task can be effectively performed with for loops. Python provides straightforward methods to access keys, values, or both using a for loop, allowing flexibility in how a developer handles the data stored in dictionaries.
To iterate directly through keys, the following pattern can be used:
By default, iterating through the dictionary will consider only its keys. If the user also wants to access values, it can be achieved easily with the items method:
This structure allows developers to deal with both aspects of the dictionary promptly. The clarity achieved is one of the key benefits of using for loops in conjunction with dictionaries.
Using For Loops for Dictionary Comprehension
Dictionary comprehension is an elegant and concise way to create dictionaries in Python. Using for loops in this context allows developers to construct new dictionaries from existing ones or to filter dictionaries based on conditions.
The syntax for dictionary comprehension is notably simple. Here is an example illustrating how to multiply each value in a dictionary by two:
Consequently, the new dictionary, doubled_dict, will now have values as doubles of the original data.
Moreover, applying conditions during dictionary comprehension adds a layer of versatility. Here’s an example filtering out items:
This loop efficiently constructs a dictionary with only elements meeting the specified criteria. Combining for loops with dictionary comprehension not only maintains readability but also enhances performance.
In essence, dictionary comprehension accompanied by for loops stands out as a powerful tool in a programmer's arsenal.
Nested For Loops
Nested for loops are loops within loops, allowing programmers to iterate over multi-dimensional data structures or perform repetitive actions across multiple levels of data. This section discusses how nested for loops operate, their usefulness, and key considerations for implementing them effectively in Python.
Understanding Nested Structures
Nested loops can be used when you need to work with matrices, grids, or any situation requiring the traversal of multi-layered data. Each iteration of the outer loop triggers the full sequence of iterations in the inner loop. For example, if you have a list of lists (a matrix), a nested for loop is an elegant solution for accessing each individual element.
Example:
In the example above, the outer loop iterates through each row of the matrix, while the inner loop retrieves each number, showcasing the manner in which values are extracted from a two-dimensional structure seamlessly. This structure simplifies complex data manipulation, making it easier for developers to manage intricate data relationships.
Nested for loops can simplify handling multi-dimensional structures, though they do require careful consideration of performance implications.
Consider memory efficiency and operational complexity when working with nested loops. Each additional nesting level often multiplies the execution time—leading to O(n*m) time complexity for an n by m matrix, for instance. Thus, while functionality is broadened through nesting, it can induce slower performance.
Use Cases of Nested For Loops
Understanding appropriate cases for when to deploy nested for loops can geometrically enhance coding proficiency. Some scenarios include:
- Data Analysis: Iterating through rows and columns of datasets for computations or visualizations, such as calculating averages or retrieving subsets based on conditions.
- Grid Computation: Applications involving game development, where game boards comprising multiple entities are manipulated, often need nested structures for processing.
- Multi-Dimensional Arrays: Technical environments, especially in scientific computing, often utilize multi-dimensional arrays for simulated scenarios.
Based on your project needs, saving calculations or restructuring can be applied to refine the usage of nested loops effectively. Always litter your code with clarifying comments to maintain readability, especially when nesting multiple loops increases cognitive load for anyone reviewing the code later.
efficiently implementing nested for loops can elevate the structure and functionality of your program while demanding vigilance concerning performance. When recognizing appropriate application, combined logic becomes a potent tool in a programmer's arsenal.
For Loop Control Statements
For loop control statements are vital for enhancing the flexibility and effectiveness of a loop in Python. Understanding how to utilize control statements enables programmers to manage the flow of loops efficiently. There are two primary control statements for loops: and . Each provides specific functionalities that can alter the loop’s default behavior, resulting in more robust and readable code.
Using Break to Exit a Loop
The statement is a powerful tool when you need to exit a loop prematurely. This can be particularly useful in scenarios where further iterations no longer serve a purpose. For example, if searching through a list for a specific value, once that value is found, the loop can immediate stop with a statement, improving efficiency. Here is a simplified structure of using in a loop:
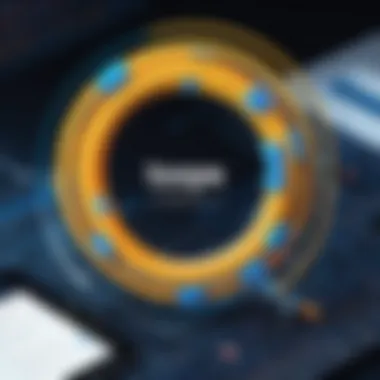
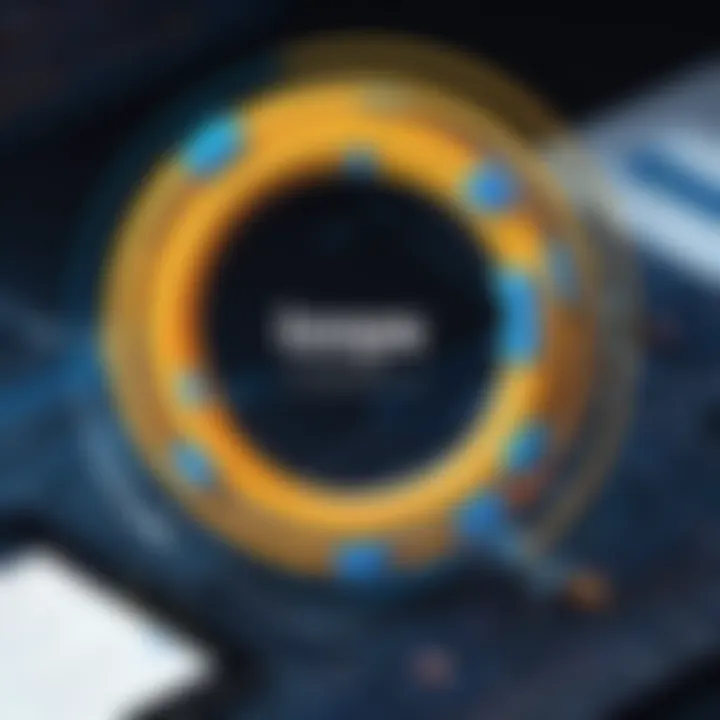
In this case, the loop prints numbers from 0 to 4 and exits once it reaches 5. Not only does this prevent unnecessary iterations, it results in cleaner output and avoids potential errors as the loop cannot proceed beyond its point of termination. In many use cases, breaking a loop early can significantly enhance performance.
Skipping Iterations with Continue
The statement serves a different purpose by instructing the loop to skip the remaining code block and proceed to the next iteration. This process can be valuable in situations where certain conditions render the rest of the loop iteration irrelevant. Careful use of can reduce complexity and make code easier to follow. Here’s a basic example:
In the above block, the loop prints only odd numbers from 0 to 9. When the number is even, the statement causes the loop to skip to the next iteration. This exemplifies wielding control statements effectively to manage functionality within loops.
Control flow statements like and help improve enough to create readable and efficient Python code. Learning when and how to implement these statements will embolden software developers in their programming journey.
Performance Considerations
Performance is a critical aspect when dealing with for loops in Python, especially in scenarios requiring a high degree of efficiency. Understanding how loops operate under the hood allows developers to optimize their code, ensuring it executes swiftly and effectively. There are several elements to examine in this discussion, including computational complexity, memory usage, and the trade-offs involved in loop structure.
Efficiency of For Loops
The efficiency of for loops hinges largely on the algorithms utilized and the nature of the data being processed. In many cases, for loops can suffer from poor performance if not designed thoughtfully. Thus, considering the size of data being iterated can make a significant difference.
For example, looping through large lists might cause more considerable delays compared to smaller datasets. Optimizing the length of the list and ensuring it is necessary to even utilize a loop can hugely affect performance. On the contrary,understanding how Python handles data types and the operations performed within loops can also impact speed.
Here are a few tips to enhance the efficiency of for loops:
- Limit Data Size: Only loop through the necessary elements.
- Minimize Function Calls: Inside loops, limit extra function calls where possible to avoid inefficiencies.
- Use Built-in Functions: Python's built-in functions like and are optimized for performance and can sometimes replace traditional loops.
In summary, being aware of how Python computes loop operations can lay the foundation for writing more efficient code.
Comparative Analysis with While Loops
Both for loops and while loops serve the fundamental purpose of iteration but perform differently in actual usage. A for loop is often more suited to iterate over a predefined range or collection. This results in clearer and often lower maintenance code due to its concise syntax. Whereas a while loop gives a developer more control over the condition being evaluated.
It’s not uncommon to analyze which loop structure performs better under specific context. While loops typically run quickly unless the loop condition sometimes results in a scenario of inconsistency, reaching infinite checks is harmful to performance; thus, they might become less efficient. For example:
Conversely, with for loops typically executing in a straightforward manner such as;
In this latter code snippet, readability remains intact as the control of the loop's boundaries lies at the forefront. It tends to encourage more predictable performances than complex while loops.
Ultimately, choosing between for and while loops depends on task requirements and also personal coding style preferences. Reflecting on how both structures manage iterations allows for more personalized performance optimizations in Python programming.
Common Pitfalls and Troubleshooting
Identifying common pitfalls and understanding troubleshooting strategies related to for loops in Python is crucial for any programmer. Such knowledge helps prevent inadvertent errors and enhances the effectiveness of code execution. This section delves into -Off-by-One Errors- and -Infinite Loops-, detailing how to recognize and resolve these issues.
Off-by-One Errors
Off-by-one errors are a frequent source of confusion in loop constructs. These mistakes often occur when the programmer miscalculates loop boundaries. For instance, if a list has five elements indexed from 0 to 4, forgetting to stop the loop at the correct point can lead to unintended behavior or errors when accessing list elements.
Common Causes
- Incorrect range specification in the loop, such as using instead of .
- Assuming one-based indexing where Python uses zero-based indexing.
- Including or excluding boundary elements improperly in conditions.
Resulting Issues
This kind of error may lead to:
- IndexError when trying to access an element outside the defined length of the sequence.
- Logic errors in the program, resulting in inaccurate data processing.
Corrective Measures
To mitigate this pitfall, consider:
- Careful boundary checking: Always validate starting and ending points of the loop.
- Using built-in functions that return lengths or counts, such as , to ensure proper loop limits.
- Performing proof-running small sets of data to catch any discrepancies quickly.
By actively applying these measures, developers can avoid common unexpected behaviors stemming from off-by-one errors.
Infinite Loops: Causes and Solutions
Infinite loops occur when a loop has no defined exit point. This can result in programs that become unresponsive or crash, leading to significant operational disruptions. Recognizing the signs of an infinite loop and identifying its causes is fundamental for efficient and effective programming.
Triggers for Infinite Loops
- Mistakes in loop termination conditions, such as with no breaks.
- Changes to loop variables that do not contribute towards meeting exit conditions.
- Erroneous conditional statements that paradoxically satisfy infinite looping.
Solutions
Confronting infinite loops requires careful inspection of the loop structure. Here are effective strategies:
- Code review: Scrutinize entire loop conditions and variable updates to align on exit criteria.
- Debugging tools: Implement debugging techniques to step through loops and observe variable values.
- Timeouts and limits: For critical applications, set maximum execution times to prevent hangs.
Ending
While encountering pitfalls is part of the development journey, adherence to robust strategies can fortify your coding practices. Avoid endless cycles and boundary miscalculations, ensuring smooth code execution and reliable outcomes in projects.
Real-World Applications of For Loops
For loops in Python are not just a theoretical concept; they hold significant importance in practical software development, data science and automation processes. Understanding where and how to apply for loops can greatly enhance the effectiveness and efficiency of programming tasks. This section explores the many real-world uses cases for for loops and provides clarity on their essential role in various domains.
Data Processing and Analysis
One prominent application of for loops is in the realm of data processing and analysis. As data manipulation becomes increasingly important across industries, for loops allow developers to handle large datasets effectively. The structure of for loops provides an organized way to iterate through data structures—be it lists, dictionaries, or complex datasets.
For example, when performing data cleaning, a for loop can be employed to examine each element within a list of values, checking for set criteria, and making modifications on-the-fly. Consider this simple scenario: removing unnecessary whitespace from strings in a list.
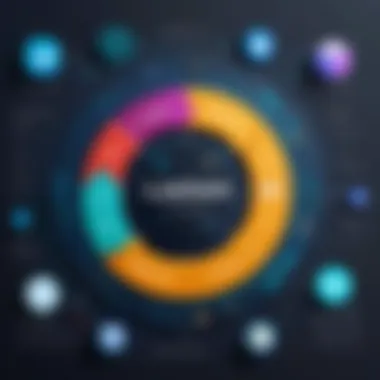
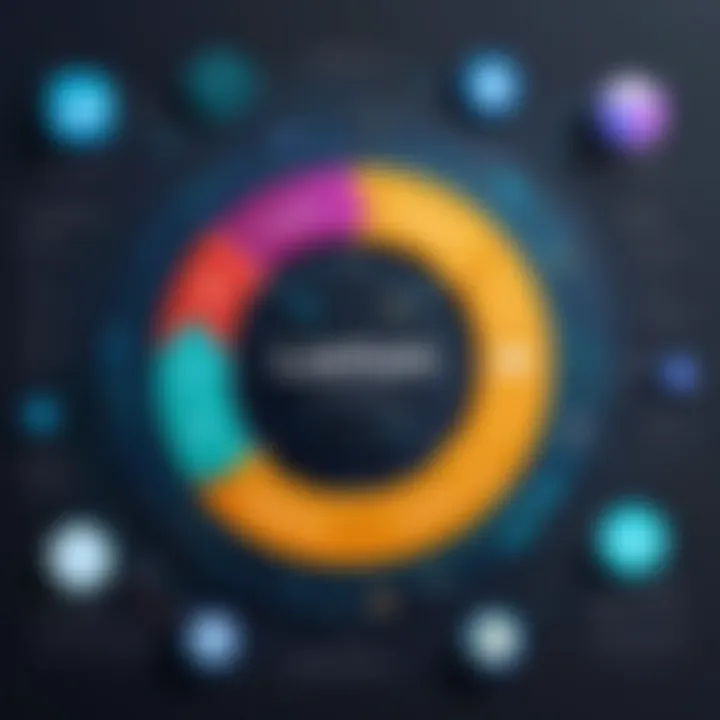
In the above snippet, the for loop processes each string, applying the method to eliminate whitespace. Without the ability granted by for loops, similarly manipulating data would be tedious and considerably more challenging.
Additionally, for loops facilitate aggregating data. For instance, summing objects based on given conditions is straightforward to accomplish using this common Python structure. The ability to efficiently process data means programmers can extract insights faster, ultimately leading to better informed decisions grounded in accurate data.
Automation in Software Development
In the field of software development, automation stands out as a critical necessity for improving productivity. For loops play an indispensable role here as well. They permit the repetitive execution of tasks without requiring individual handling for each instance. This powerful function can streamline many repetitive processes.
By utilizing for loops, developers can effectively automate tasks like file manipulation, batch processing, and application testing. For instance, consider a scenario where a developer may need to rename a batch of files. Instead of typing commands repetitively, here's how a for loop might simplify this:
The for loop above takes every file in the list and prepends “new_” to each of their names. Tasks that involve even moderate repetition can benefit from such automation.
Moreover, in testing software applications, for loops can run test cases repeatedly, which ensures coverage without manual intervention. The logical repetition provided by for loops supports greater efficiency while simultaneously reducing human error through unmonitored manual performance.
In software development, leveraging for loops’ power can save time and decrease developmental complexity.
Advanced Techniques in Looping
For loops are fundamental in Python programming, but mastering their more advanced techniques can drastically enhance one’s ability to manipulate data and implement efficient algorithms. Utilizing advanced methods such as list comprehensions and generator expressions can lead to cleaner, more readable code. These approaches improve both performance and maintainability by leveraging Python's innate capabilities for concise data handling.
List Comprehensions
List comprehensions provide a syntactically compact way to create lists based on existing iterables. This feature is highly valued for its expressiveness and efficiency. By creating lists in a single line, a programmer can reduce boilerplate code significantly while still keeping the logic clear.
For example, using a list comprehension, you can create a list of squares of numbers from an existing list. By avoiding unnecessary loops, you not only enhance performance, but also make maintenance easier due to reduced code complexity.
Here’s a simple illustration:
In this example, squares holds the squares of the first ten integers. The compact syntax allows for clear intent without cumbersome for loop syntax. It encourages an efficient coding style, fostering productivity in repetitive tasks.
The creativity offered through list comprehensions drives unique programmatic expressions. Expanding this technique with conditions results in powerful combined logic, allowing filtering and transformation to take place in clean sequences. For instance:
Here, only the squares of even numbers are captured.
Generator Expressions
Generator expressions are another advanced form of looping construct in Python. They are similar to list comprehensions, but instead of creating a full list in memory, they generate items one at a time as needed. This becomes a powerful tool for scenarios where large datasets are involved, as it uses memory more efficiently.
In establishing a generator, the syntax mirrors that of list comprehensions, with parentheses replacing square brackets. This technique proves beneficial when one only needs to iterate over data without requiring a high-cost allocation of resources.
To observe a generator in action:
Notice how this process yields, one by one, rather than building the entire collection at once. The nominal memory overhead is essential for optimal performance when processing large volumes of information without hindering system memory.
The seamless transition from list comprehensions to generator expressions emphasizes the versatility of looping in Python. Rather than generating large datasets, developers can work incrementally, retaining lean runtime efficiency. Utilizing this technique ultimately contributes to sustaining performance while effectively managing larger data sets.
Best Practices for Writing For Loops
Writing efficient and effective for loops in Python is crucial for all code you develop. A loop's structural clarity can greatly affect its addaptability and performance. This section details practical steps for enhancing your coding style while leveraging Python's dynamic capabilities.
Maintaining Readability and Clarity
Readability is vital in programming; it allows others (and yourself) to understand the code you have touching more easily. Here are a few guides for achieving clarity:
- Consistent Naming Conventions: Use descriptive variables names that indicate the data represented. This makes it easier for the reader to follow the logic of the for loop.
- Limit Loop Complexity: Avoid nesting too many loops within loops. This increasee cognitive load on the reader. If necessary, break these structures into functions or separate loops.
- Keep Operations Within The Loop Minimal: Place only essential operations inside the body of the loop. Performing few operations maintains efficiency while ensuring you retain clarity.
- Commenting: Use comments judiciously to provide context about the purpose of certain codes or loop conditions. Explaining why the loop executes a certain way can be more informative than just describing what it does.
Quote Important information:
"Clear code is a powerful asset. Readable code enhances collaboration and long-term maintenance.”
Employing these best practices helps programmers conceive of the code like a story. Drawing coherent and understandable illustrations is essential while engaging with communication between developers.
Optimizing Loop Performance
While clarity is vital in your loops, performance matters too, especially in data-heavy environments. Here are effective techniques for enhancing loop efficiency:
- Avoid Unnecessary Computation in the Loop: Move operations or calculations that stay consistent outside the loop. This way, you save computing time across multiple iterations.
- Using List Comprehensions: Python allows a more compact loop syntax that builds lists quickly. When applicable, this can improve both speed and readability over traditional for loops.
- Use the Built-In Functions: Exploit functions like , or . These functions run much faster than explicit for loops.
- Profile Your Code: Keep reviewing your loop performance with profiling tools to uncover bottlenecks. By analyzing traces, you can pinpoint where optimisations are needed.
Applying these optimisations brings clear benefits to the looping structure and performance while affirming the importance of effective coding strategies. Missteps, when copying industry standards into execution, can have cascading negative impacts in applications where speed and scale take precedence. Additionally, if large datasets must undergo repeated processes, logical structuring pays dividends in long run.
Culmination
The conclusion is an essential part of any comprehensive exploration of a topic. Here, we consolidate knowledge gained about for loops in Python. A well-rounded understanding allows programmers to effectively use loops, making code cleaner and efficient. For loops help manage collections, including lists, dictionaries, and other iterables, simplifying iteration tasks.
Summary of Key Points
For loops are a fundamental construct in Python programming. They offer several capabilities:
- Iteration through sequences: For loops can process elements of a sequence sequentially.
- Optimization: Efficient looping constructs often result in faster execution time.
- Readability: They enhance the clarity of code compared to repetitive structures.
In this guide, we addressed:
- Syntax and basic structure.
- Common pitfalls associated with for loops. Common errors such as off-by-one can lead to bugs, which are preventable by a good understanding of the topic.
- Real-world applications, where this feature proves invaluable in areas from data science to working with different APIs.
Understanding these aspects is critical for professionals working to enhance their programming skills. Familiarity with for loops leads to better practices and ultimately helps programmers succeed.
Future Trends in Python Looping
The future promises continuous evolution in programming practices. Innovations around for loops may include:
- Incorporation of AI and ML technologies: As languages like Python are used more in artificial intelligence and data science, handling complex data structures will be crucial, requiring optimized looping.
- Integration with asynchronous programming: There's growing interest in asyncio for performance improvements, potentially reshaping how loops enhance speed and efficiency.
- Expansion of built-in functions: Python's standard library may increasingly emphasize functional programming paradigms, introducing new looping constructs to streamline code efficiency even further.
Continuous learning regarding features and changing contexts will hold weight for developers in adapting to upcoming trends in Python looping. Understanding these dimensions ensures going beyond basic programming knowledge, remaining relevant in an ever-evolving technological landscape.