Unlocking the Power of Delegates: A Comprehensive Guide in C# Programming
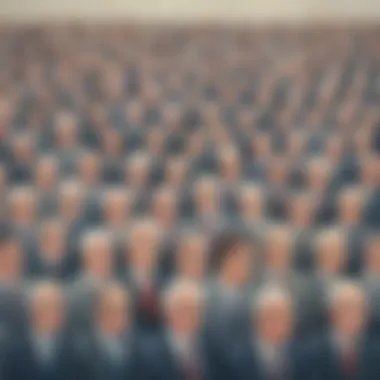
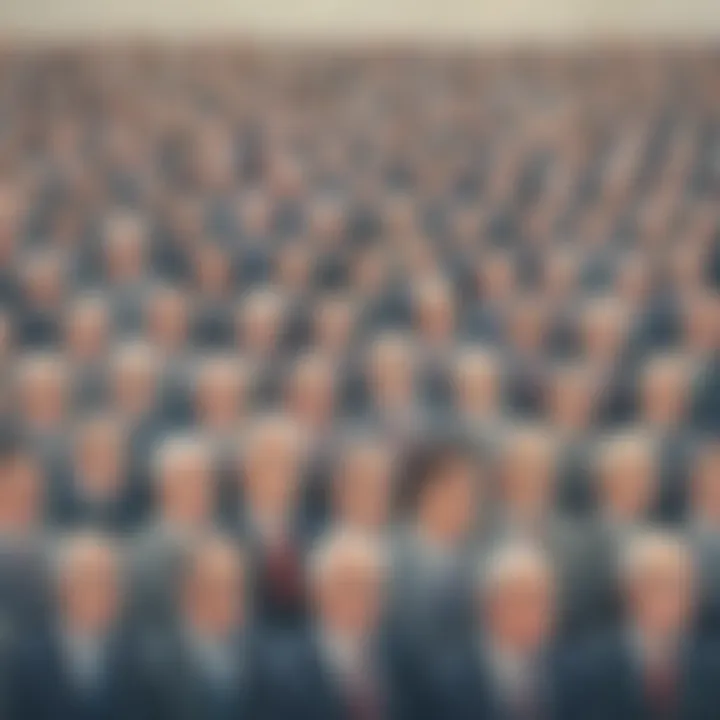
Overview of Delegates in
Programming Delegates in C# are essential for achieving flexibility and extensibility in code implementation. They serve as type-safe function pointers that enable efficient event handling and callbacks. By understanding delegates, programmers can enhance the structure and functionality of their C programs.
Best Practices Regarding Delegates
When working with delegates in C#, it is crucial to follow industry best practices for seamless implementation. Maximizing efficiency and productivity involves choosing the right delegate types and ensuring proper error handling. Avoiding common pitfalls such as circular references and memory leaks is paramount for effective delegate usage.
Case Studies on Delegates in Action
Real-world examples showcase the power of delegates in C# applications. By studying successful implementations, developers can gain insights into utilizing delegates for various functionalities. Understanding the outcomes achieved through delegate usage provides valuable lessons for enhancing coding practices.
Latest Trends and Updates in Delegate Usage
Stay updated on the evolving landscape of delegate usage in C#. By exploring upcoming advancements and current industry trends, developers can adapt their coding techniques to leverage the latest innovations. Understanding breakthroughs in delegate mechanisms is key to staying competitive in the programming sphere.
How-To Guides for Implementing Delegates
Step-by-step guides offer practical insights into incorporating delegates effectively in C# programming. From beginner tutorials to advanced user tips, these guides provide a comprehensive approach to utilizing delegates for enhanced code functionality. By following these detailed instructions, programmers can master the art of delegate implementation.
Introduction to Delegates
Delegates play a vital role in C# programming, providing a level of flexibility and extensibility that are crucial in modern software development. Understanding delegates is a cornerstone of mastering event-driven architectures and functional programming paradigms. By delving into the intricacies of delegates, developers can enhance the efficiency and versatility of their codebase.
Definition of Delegates
Understanding the role of delegates in
programming Delegates in C# serve as type-safe function pointers, allowing developers to encapsulate and reference methods within their applications. This capability introduces a higher level of abstraction, enabling decoupling of components and enhancing the overall maintainability of the codebase. Understanding how delegates function in C is essential for leveraging the full potential of this language feature.
Exploring how delegates represent references to methods
Delegates represent a significant advancement in programming by providing a mechanism to pass methods as parameters. This unique feature allows for dynamic invocation of methods, opening doors to innovative programming paradigms such as callback mechanisms and event-driven architectures. Knowing how delegates represent references to methods is key to harnessing the power of C# in building robust and scalable applications.
Delegate Declaration
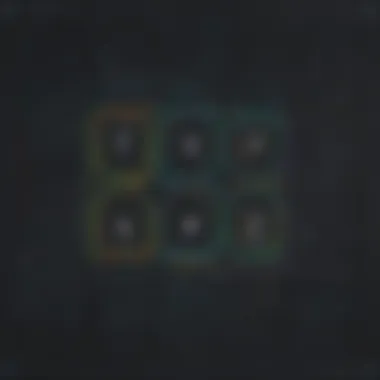
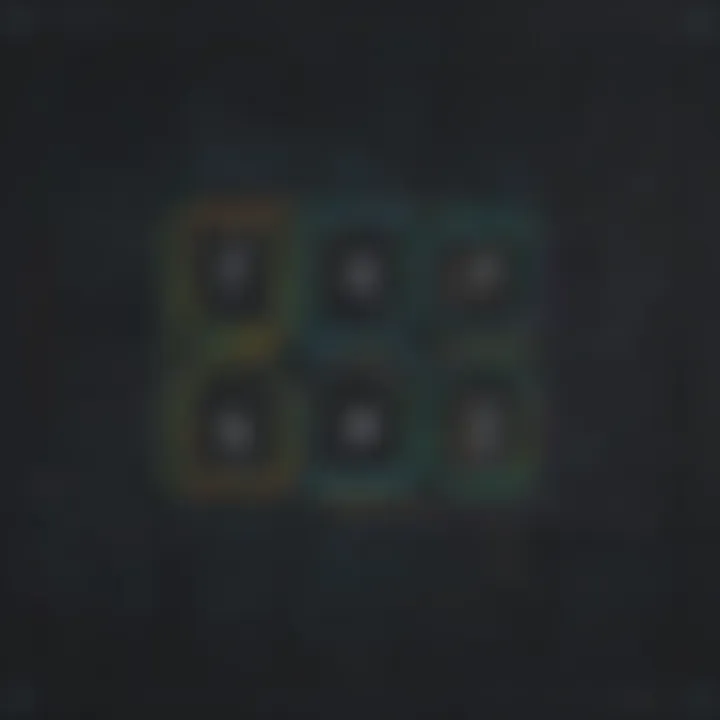
Syntax and structure of delegate declarations
The syntax and structure of delegate declarations define the blueprint for creating delegate types in C#. By understanding the intricacies of declaring delegates, developers can tailor these constructs to specific use cases, ensuring type safety and compatibility with targeted methods. Mastery of delegate declaration enables precise method signature definitions, laying the foundation for effective delegation in C# programming.
Defining delegate types for method signatures
Defining delegate types for method signatures involves specifying the parameters and return type that a delegate can encapsulate. This step is crucial in ensuring type consistency and coherence when associating delegates with methods. By defining delegate types accurately, developers enhance the robustness of their code and facilitate seamless method invocations through delegate instances.
Delegate Instantiation
Creating instances of delegates and associating with methods
Creating instances of delegates and associating them with methods enable developers to establish the connections needed for method invocation. This process involves instantiating delegates with compatible methods, paving the way for invoking these methods through delegate instances. Understanding the nuances of delegate instantiation is paramount for enabling efficient callback mechanisms and event handling in C#.
Discussing multicast delegates for multiple method invocations
Multicast delegates in C# allow for the invocation of multiple methods through a single delegate instance. This powerful feature enhances the flexibility of delegate-based programming by facilitating the execution of multiple methods in response to a single event. Exploring multicast delegates provides insights into orchestrating diverse method invocations efficiently, bolstering the adaptability of C applications.
Using Delegates in
Delegates play a crucial role in C# programming, offering a high level of flexibility and extensibility in code implementation. By using delegates, developers can easily work with functions as first-class citizens, allowing for the creation of type-safe function pointers. The ability to reference methods through delegates enhances event handling and callback mechanisms, enabling efficient and scalable solutions in application development. Understanding how to leverage delegates in C is essential for implementing modular and manageable code structures.
Delegate Invocation
Invoking delegate instances to execute related methods
Invoking delegate instances is a fundamental aspect of delegate usage in C#. It allows for the execution of methods indirectly through delegate objects, providing a level of abstraction that promotes loose coupling in code. By invoking delegate instances, programmers can achieve dynamic method calls and delegate chaining, enhancing the adaptability and reusability of code components. This capability is particularly valuable in scenarios where the specific method to be executed is determined at runtime, offering a powerful mechanism for implementing flexible and responsive applications.
Passing parameters and returning values in delegate calls
Delegate calls can pass parameters to target methods and retrieve return values seamlessly. This capability enables delegates to serve as conduits for data transfer between different components of a software system. By facilitating communication between disparate parts of an application, delegates enhance modularity and maintainability. However, excessive reliance on delegate parameters and return values may introduce complexity and potential debugging challenges, requiring careful consideration of data flow and function interactions within delegate-invoked methods.
Delegate as Callbacks
Utilizing delegates for callback functionalities
Delegates excel in supporting callback functionalities, a key paradigm in event-driven programming in C#. By assigning methods to delegates, developers can define callback routines that respond to specific events or conditions. This event-driven approach enables asynchronous processing, user interaction handling, and system notifications, fostering responsive and interactive software applications. Leveraging delegates for callbacks enhances code organization and promotes efficient event propagation within an application architecture.
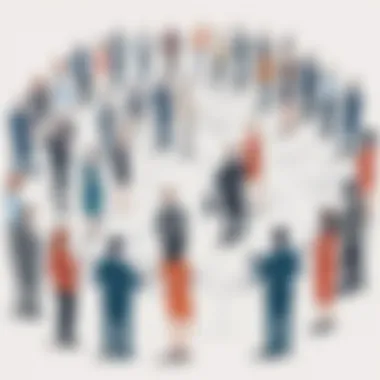
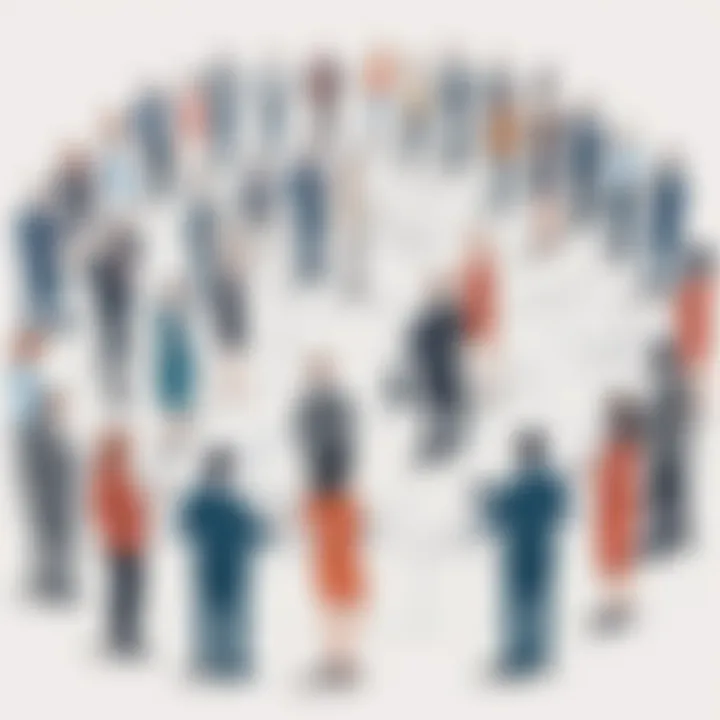
Implementing event-driven architectures with delegates
Delegates are instrumental in implementing robust event-driven architectures, providing a structured mechanism for managing and coordinating event handlers. By utilizing delegates to bind event listeners to relevant functions, developers can establish clear patterns for event subscription and notification. This declarative approach enhances code readability and simplifies the maintenance of event-driven systems, ensuring coherent handling of diverse events throughout an application's lifecycle.
Functional Programming with Delegates
Leveraging delegates for functional programming paradigms
Functional programming principles align closely with delegate usage in C#, offering a concise and expressive way to define behavior through functions. By leveraging delegates, developers can adopt a functional programming style that emphasizes immutable data and composability. Delegates enable the encapsulation of logic within method references, facilitating the creation of composable functions that promote code reuse and clarity. This functional approach enhances maintainability and testability, making codebases more resilient to change and evolution.
Exploring lambda expressions and anonymous methods
Lambda expressions and anonymous methods represent powerful syntactic sugar in C# for concise function definitions within delegate contexts. These features streamline the declaration of delegate instances, reducing boilerplate code and enhancing code readability. By embracing lambda expressions and anonymous methods, developers can create inline function definitions that improve code conciseness and maintainability. The compact and expressive nature of these constructs enhances code comprehension and supports the adoption of functional programming techniques in C development.
Real-World Examples
In this segment of the article, we delve into real-world examples of delegate usage in C#. By showcasing practical applications, readers can grasp the significance and utility of delegates in actual scenarios. Demonstrating how delegates streamline event handling and callback mechanisms in various contexts, we highlight their adaptability and extensibility in software development.
Event Handling with Delegates
Implementing event listeners using delegates
Presenting a crucial aspect of utilizing delegates, we focus on implementing event listeners. This feature plays a pivotal role in event-driven programming by facilitating the connection between events and their corresponding actions. The key characteristic of implementing event listeners using delegates lies in its ability to decouple event sources from event handlers, promoting a modular and maintainable code structure. This implementation choice proves beneficial as it allows for dynamic event subscriptions and simplifies event management within the application architecture.
Managing event propagation and handling through delegates
Discussing the importance of managing event propagation through delegates sheds light on the efficient control of event flow within an application. By leveraging delegates for event handling, developers can establish clear propagation pathways and ensure streamlined event processing. The key characteristic of this approach is its event bubbling mechanism, where events travel through a predefined hierarchy of delegates for optimized handling. While offering advantages in event delegation and parallel event processing, this method may introduce complexities in managing event propagation chains.
Callback Mechanisms in Applications
Integrating callbacks for asynchronous programming
Exploring how callbacks enhance asynchronous programming, we delve into the seamless integration of callback mechanisms using delegates. This integration empowers developers to initiate non-blocking function calls and enables efficient handling of asynchronous tasks. The key characteristic of integrating callbacks for asynchronous programming is the ability to define post-task execution procedures, optimizing resource utilization and enhancing task scheduling. While prevalent in event-driven architectures, this approach necessitates careful consideration of callback registration and execution sequencing for robust application performance.
Achieving decoupling and extensibility via delegates
Delving into the significant role of delegates in achieving decoupling and extensibility within applications, we analyze how delegates serve as intermediary entities to support flexible and modular design structures. By utilizing delegates for callback implementations, developers can establish loosely coupled components that promote code maintainability and scalability. The unique feature of achieving decoupling and extensibility via delegates lies in their ability to encapsulate method references, allowing for dynamic function assignments and extended functionalities. While advantageous in enhancing code flexibility, this approach may introduce challenges in tracking callback dependencies and managing callback contexts.
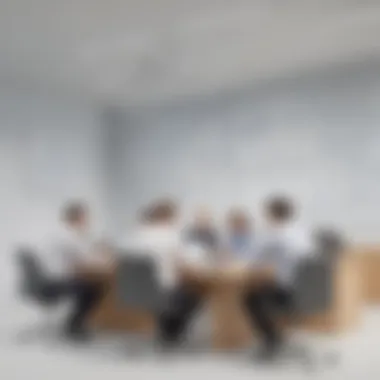
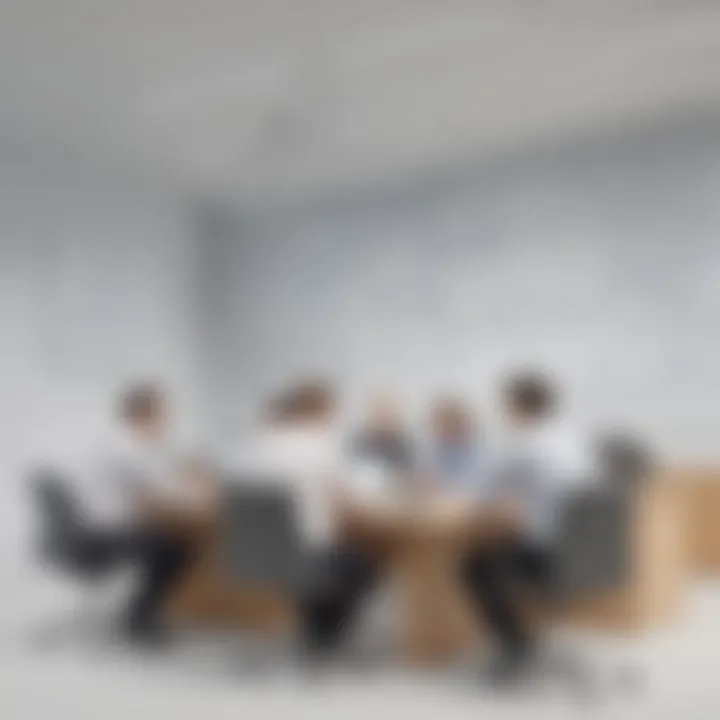
Dynamic Method Invocation
Dynamic invocation of methods through delegates
Examining the dynamic invocation capabilities enabled by delegates, we navigate through the dynamic execution of methods via delegate references. This functionality empowers developers to adapt method behaviors at runtime, offering unparalleled flexibility in method invocation. The key characteristic of dynamic invocation of methods through delegates is its ability to invoke diverse methods through a unified manner, enabling code modularity and agile functionality adjustments. Despite its advantages in runtime method selection, this approach requires meticulous method reference management to prevent runtime errors and ensure seamless method invocations.
Adapting functionality at runtime with delegate flexibility
Exploring the dynamic adaptability provided by delegates, we study how delegate flexibility enables runtime functionality adjustments in applications. By assigning and reassigning delegate instances dynamically, developers can alter application behavior on-the-fly, catering to evolving requirements. The key characteristic of adapting functionality at runtime with delegate flexibility is the capacity to substitute method implementations seamlessly, promoting code dynamism and adaptability. While instrumental in enabling runtime modifications, this approach demands careful consideration of delegate compatibility and error handling to maintain application stability and functionality coherence.
Advanced Concepts
In the realm of C# programming, the section on Advanced Concepts plays a pivotal role in enhancing the readers' understanding of delegates. By delving into intricate details and nuances, this segment sheds light on the sophisticated mechanisms that underpin delegate functionality. Understanding Advanced Concepts is paramount for developers seeking to leverage delegates to their full potential. Concepts such as Generic Delegates, Delegate Chaining, and Covariance and Contravariance are explored in depth, providing a comprehensive overview of the advanced features that elevate C programming.
Generic Delegates
Creating generic delegates for versatile functionality
The creation of generic delegates for versatile functionality offers a dynamic approach to defining delegate types. By incorporating generics, developers can encapsulate a wide range of methods under a single delegate type, paving the way for flexible and reusable code structures. This aspect of delegate utilization not only streamlines coding processes but also fosters a high degree of adaptability, allowing for seamless integration of diverse method signatures. The ability to create generic delegates enhances the extensibility and scalability of C# applications, enabling developers to cater to a multitude of scenarios without the constraints of predefined delegate structures.
Discussing type-safe generic delegate implementations
Delving into type-safe generic delegate implementations elucidates the robust nature of delegate functionalities in C# programming. By enforcing type safety at the delegate level, developers can mitigate potential runtime errors and enhance code reliability. The discussion on type-safe generic delegate implementations underscores the importance of strict type adherence, ensuring compatibility between delegates and associated methods. This approach not only promotes code consistency but also enables developers to write more predictable and error-resilient code segments. Embracing type-safe generic delegate implementations is instrumental in fostering code robustness and safeguarding against inadvertent type mismatches.
Delegate Chaining
Concatenating delegates to form chains of execution
The concept of concatenating delegates to form chains of execution revolutionizes the way methods are orchestrated within C# applications. By chaining delegates together, developers can create intricate sequences of method calls, unlocking a new realm of possibilities for code structuring. This methodical approach allows for modular and sequential execution of methods, empowering developers to build sophisticated workflows with ease. The ability to concatenate delegates streamlines the management of method invocations, offering a structured and efficient way to orchestrate complex operations within a codebase.
Understanding order and composition in delegate chaining
Understanding the order and composition in delegate chaining is imperative for designing robust and efficient code architectures. By dissecting the sequence of delegate invocations, developers can unravel the intricate interactions between methods and optimize the execution flow. This detailed understanding of delegate order and composition facilitates precise control over method execution, enabling developers to tailor the behavior of their applications with precision. Grasping the nuances of order and composition in delegate chaining empowers developers to design scalable and maintainable codebases, setting the foundation for robust software development practices.
Covariance and Contravariance
Exploring type conversions in delegate signatures
Exploring type conversions in delegate signatures delves into the subtle nuances of type compatibility within delegate assignments. By examining covariance and contravariance principles, developers can navigate the intricacies of type conversions with finesse, ensuring seamless interaction between delegate types. This exploration equips developers with the knowledge to manage type transformations effectively, facilitating smooth communication between delegates and methods. Understanding type conversions in delegate signatures is indispensable for establishing coherent and compatible coding structures, promoting code interoperability and flexibility across varying delegate types.
Handling compatibility and variance in delegate assignments
Handling compatibility and variance in delegate assignments addresses the alignment of delegate types with method signatures, emphasizing the importance of maintaining compatibility to avoid runtime errors. By assessing compatibility and managing variances in delegate assignments, developers can safeguard against potential inconsistencies and ensure smooth method invocations. This meticulous approach to handling delegate assignments not only enhances code robustness but also fosters a stable and predictable execution environment. Skillfully managing compatibility and variances in delegate assignments is instrumental in fortifying code integrity and promoting seamless interaction between delegates and methods.