Unraveling the Intricacies of Callbacks in Programming: A Detailed Overview
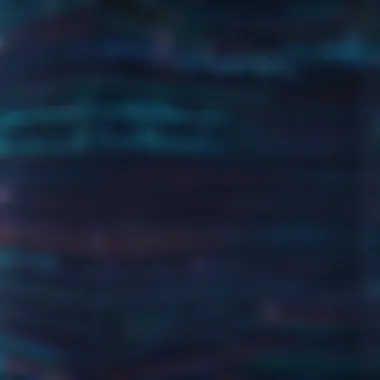
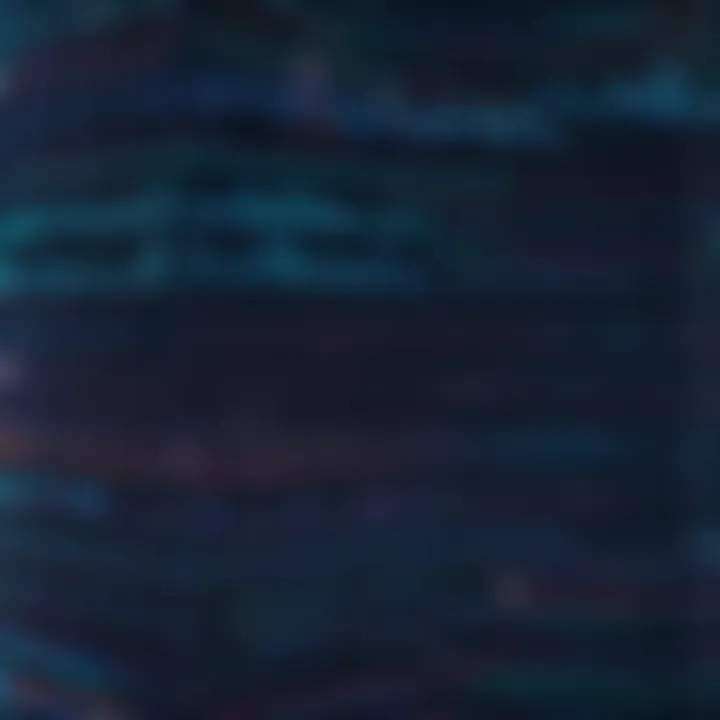
Overview of Callbacks in Programming
Callbacks are a fundamental concept in programming, crucial for asynchronous operations. Understanding callbacks is paramount for anyone in software development, especially for IT professionals delving into the intricacies of programming structures. At its core, a callback is a function passed as an argument to another function, allowing it to be executed after a specific task is completed. This in-depth exploration of callbacks will shed light on their significance, functionality, and applications in diverse programming scenarios.
Definition and Importance of Callbacks
In the realm of programming, callbacks serve as a mechanism for handling tasks that take time to complete, like fetching data from a server or processing user input. They play a pivotal role in event handling, enabling developers to write more efficient and responsive code. The importance of callbacks lies in their ability to facilitate non-blocking operations, enhancing the overall performance of software applications.
Key Features and Functionalities
Callbacks empower developers to implement asynchronous operations seamlessly, allowing programs to continue executing while waiting for specific tasks to finish. By leveraging callbacks, programmers can achieve parallelism and concurrency, essential for optimizing program efficiency. Additionally, callbacks promote modularity and code reusability, leading to more maintainable and scalable software solutions.
Use Cases and Benefits of Callbacks
In practical terms, callbacks find widespread application in scenarios like handling user interactions on web applications, executing database queries, and managing event listeners in graphical user interfaces. By embracing callbacks, developers can create responsive and interactive user experiences, improve application performance, and design robust systems capable of handling a variety of tasks simultaneously.
Prologue to Callbacks
Callbacks play a crucial role in programming, serving as a mechanism that allows functions to be passed as arguments to other functions. In this intricate world of software development, understanding the essence of callbacks is paramount. This section sets the foundation for comprehending their significance and applications throughout the programming landscape. As we delve deeper into the realm of callbacks, we will unravel their inner workings, benefits, and challenges, providing a holistic view for software developers and tech enthusiasts.
Definition of Callbacks
Understanding the fundamental concept
Within the intricate web of programming, the fundamental concept of callbacks stands as a pivotal element. By grasping this foundational idea, developers can harness the power of asynchronous operations effectively. The distinctiveness of callbacks lies in their ability to execute functions once a specific task is completed, making them an indispensable tool in modern programming paradigms. While offering flexibility and efficiency, callbacks also pose challenges related to callback hell and code readability.
Callbacks in the context of programming languages
Callbacks in the context of programming languages provide a versatile approach to handling events and asynchronous operations. Their adaptability across various programming languages ensures a robust foundation for software development. Integrating callbacks into programming languages enhances modularity and code reusability but demands a thorough understanding of callback mechanisms to avoid potential pitfalls. Striking a balance between leveraging callbacks' power and mitigating callback-induced complexities is key to optimizing their utility.
Purpose of Callbacks
Enabling asynchronous operations
Enabling asynchronous operations through callbacks empowers developers to execute tasks concurrently, enhancing program responsiveness. By decoupling functions and allowing non-blocking operations, callbacks streamline the flow of program execution. However, managing callback chaining and maintaining code readability pose challenges that developers must navigate skillfully.
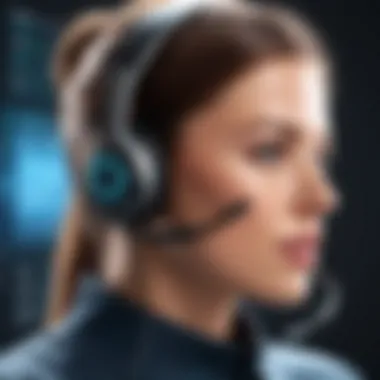
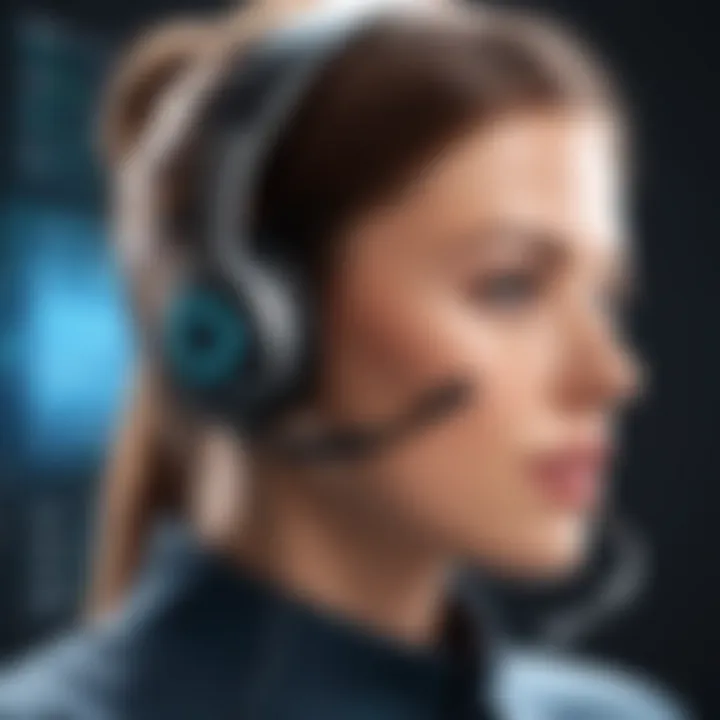
Implementing event handling
Implementing event handling using callbacks revolutionizes the processing of user interactions and system events. Callbacks serve as a linchpin for event-driven programming, enabling seamless communication between various components. While offering a structured approach to event management, callbacks require meticulous design considerations to avoid callback nesting and maintenance complexities.
Key Components of Callbacks
Callback functions
Callback functions act as the cornerstone of callback implementations, enabling dynamic behavior and extensibility within programs. Their flexibility allows for customizability and responsive event handling, contributing to code elegance. Nevertheless, over-reliance on callback functions may lead to callback hell scenarios, warranting judicious planning and design.
Higher-order functions
Embracing higher-order functions elevates callback mechanisms to a sophisticated level, offering enhanced abstraction and functional composition. By treating functions as first-class citizens, higher-order functions empower developers to encapsulate complex behaviors concisely. However, mastering higher-order functions demands a comprehensive understanding of functional programming principles and design patterns to harness their full potential.
Implementing Callbacks in Programming Languages
In the realm of programming, implementing callbacks in various programming languages holds a pivotal role. Understanding how to effectively utilize callbacks is crucial for developing asynchronous operations and event handling mechanisms. Implementing callbacks empowers software developers to create responsive and efficient programs by allowing functions to be passed as arguments to other functions. This flexibility is particularly beneficial when working with tasks that may take an undetermined amount of time to complete. By implementing callbacks, developers can streamline their code, enhance modularity, and improve code maintainability.
JavaScript Callbacks
Examples of Callback Functions in JavaScript
Diving into the realm of JavaScript callbacks, it becomes evident that callback functions play a significant role in enabling asynchronous operations. By utilizing callback functions, developers can execute code after a certain task is completed, thereby preventing blocking operations and enhancing overall program efficiency. One key characteristic of callback functions in JavaScript is their ability to ensure non-blocking behavior, allowing multiple tasks to execute concurrently. While callback functions offer efficiency and flexibility, they can sometimes lead to callback hell, a nested structure that hinders code readability. Understanding how to structure callback functions effectively is essential for leveraging their full potential.
Error-first Callbacks in Node.js
Within the context of Node.js, error-first callbacks are a common paradigm for handling asynchronous operations. This approach involves passing an error object as the first parameter to a callback function, allowing developers to efficiently manage errors within their code. The key characteristic of error-first callbacks is their structured error-handling mechanism, ensuring that errors are properly caught and handled during asynchronous tasks. While error-first callbacks promote robust error management, they also introduce a verbose syntax that may impact code readability. Striking a balance between error handling and code clarity is crucial when incorporating error-first callbacks in Node.js.
Python Callbacks
Utilizing Function Callbacks in Python
In the realm of Python programming, function callbacks serve as versatile tools for implementing event-driven functionality. By utilizing function callbacks, developers can define custom actions to be executed when specific events occur, enhancing the responsiveness of Python applications. A key characteristic of function callbacks in Python is their ability to promote modular code development, allowing developers to encapsulate event-specific functionality within callback functions. While function callbacks offer enhanced flexibility and code organization, improper implementation may lead to callback dependency issues, impacting overall code maintainability. Striving for a cohesive and modular approach to function callback utilization is essential for optimizing Python application performance.
Event-Driven Programming with Callbacks
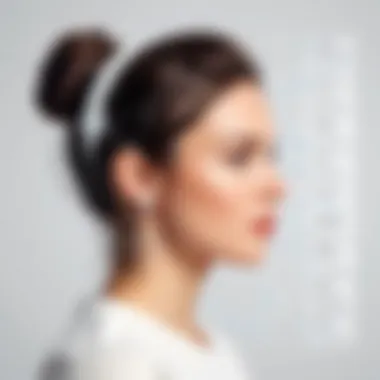
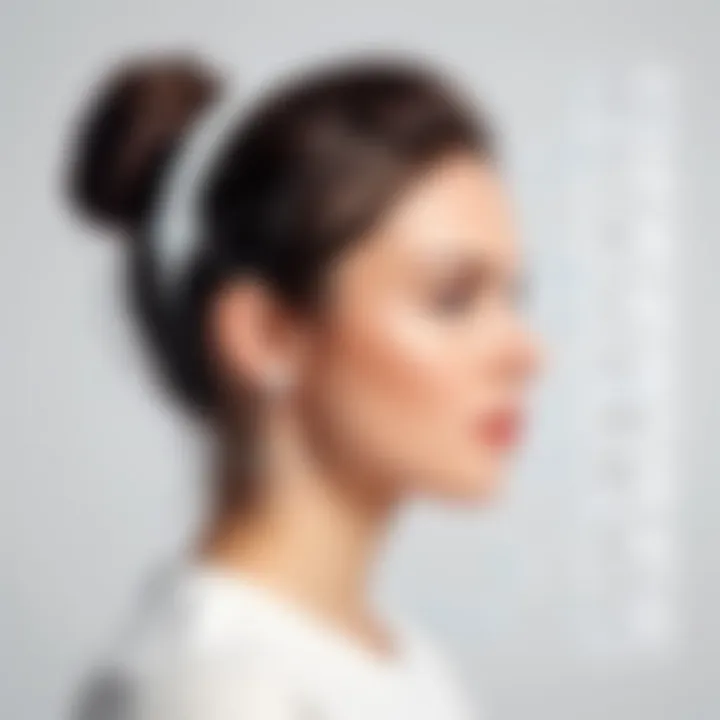
Event-driven programming with callbacks in Python enables developers to create responsive applications that respond to user interactions effectively. By implementing callback functions tied to specific events, developers can design interactive applications that engage users dynamically. A key characteristic of event-driven programming with callbacks is the establishment of callback bindings between events and functions, enabling seamless event handling within Python applications. While event-driven programming boosts application interactivity, excessive callback usage may lead to callback spaghetti, complicating code management. Balancing event-driven design with code simplicity is crucial for maximizing the benefits of callback-based event handling.
Java Callbacks
Callback Interfaces in Java
Java embraces callback interfaces as a fundamental component for achieving asynchronous processing and event-driven functionality. By defining callback interfaces, developers can establish clear communication channels between components, facilitating seamless data exchange and event propagation. A key characteristic of callback interfaces in Java is their abstraction layer, allowing developers to decouple event triggers from their associated actions. While callback interfaces enhance code modularity and reusability, they may introduce complexity when managing multiple callback implementations. Adhering to cohesive design principles and interface segregation can mitigate callback interface complexities, fostering efficient Java application development.
Asynchronous Processing Using Callbacks
Harnessing callbacks for asynchronous processing in Java empowers developers to execute tasks concurrently without blocking the main thread. By leveraging callback functionalities, developers can initiate multiple operations simultaneously, improving application responsiveness and performance. A key characteristic of asynchronous processing using callbacks is the utilization of callback chains, which enable sequential task execution and result handling. Although callback-based asynchronous processing offers efficiency, extensive callback chaining may result in callback hell, complicating code logic. Implementing structured callback patterns and leveraging callback libraries can streamline asynchronous processing in Java applications, ensuring optimal performance and scalability.
Best Practices for Using Callbacks
Callbacks play a crucial role in programming, and understanding the best practices for their utilization is paramount in ensuring efficient and maintainable code. In this section, we will delve into key elements, benefits, and considerations regarding the best practices for using callbacks. Emphasizing the importance of structuring callback functions in a clear and concise manner can lead to improved code readability and overall functionality. By adhering to best practices, developers can mitigate common pitfalls associated with callback usage and enhance the robustness of their applications.
Avoiding Callback Hell
Strategies to prevent nested callbacks
When it comes to avoiding the infamous 'Callback Hell,' employing strategies to prevent nested callbacks is indispensable. By implementing techniques such as modularization, parallelization, and refactoring, developers can effectively manage callback complexity and streamline the flow of asynchronous operations. This approach not only enhances code organization but also reduces the likelihood of encountering callback-related issues, promoting code maintainability and scalability in the long run.
Utilizing Promises or async/await
In the realm of asynchronous programming, leveraging Promises or async/await is a prevalent approach to circumvent callback hell. By adopting these constructs, developers can handle asynchronous tasks more elegantly, writing cleaner and more readable code. Promises simplify the chaining of asynchronous operations, while async/await introduces a synchronous feel to asynchronous code, making it easier to comprehend and debug. While these methods offer significant advantages in reducing callback complexity, developers should be mindful of potential drawbacks such as Promise chaining and error handling intricacies.
Error Handling with Callbacks
Managing errors effectively in callback functions
Efficient error handling is a critical aspect of utilizing callbacks in programming. By implementing mechanisms to manage errors within callback functions, developers can preemptively address and capture exceptions, ensuring graceful error propagation and response. Proper error management not only enhances the reliability of the codebase but also contributes to a more robust and resilient application architecture.
Error propagation and handling
In the context of callback-based systems, error propagation and handling are integral components of ensuring program integrity and fault tolerance. By establishing clear error propagation mechanisms and implementing systematic error-handling strategies, developers can anticipate and address potential failures effectively. Handling errors gracefully not only enhances the user experience but also fortifies the application against unforeseen issues, fostering a more resilient and stable software environment.
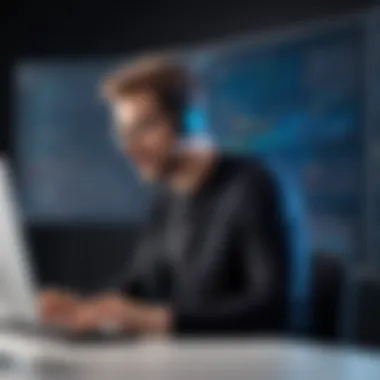
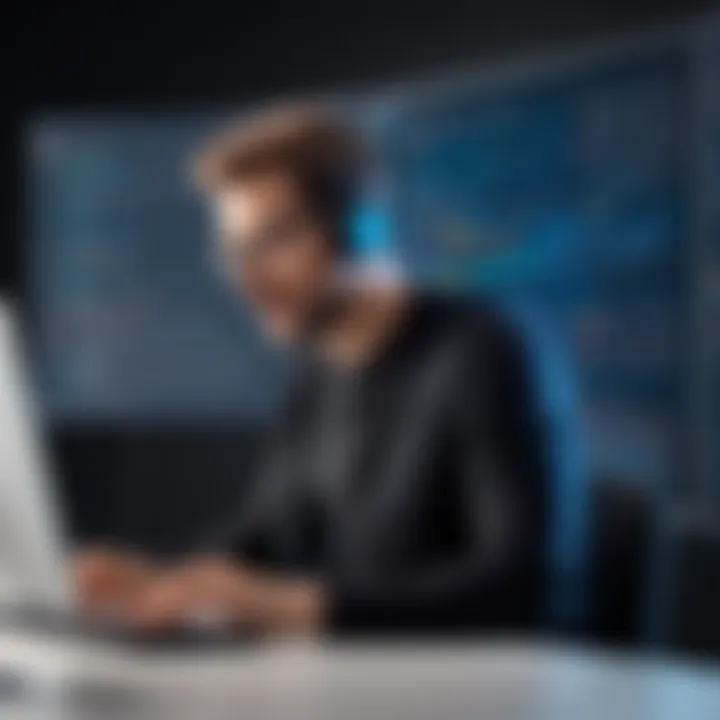
Callback Design Patterns
Common patterns for callback implementation
The adoption of common design patterns for callback implementation can streamline the development process and promote code consistency. By following established patterns such as the Observer pattern or the Command pattern, developers can encapsulate callback functionality in a structured and reusable manner, enhancing code modularity and extensibility. Leveraging these patterns facilitates code maintenance and comprehension while promoting best practices in callback utilization.
Choosing the right pattern for a given scenario
Selecting the appropriate design pattern for a specific scenario is crucial in optimizing callback usage. Depending on the nature of the application or the complexity of the functionality, developers must evaluate and choose the most suitable design pattern to ensure coherence and efficiency in callback implementation. By aligning the design pattern with the requirements of the project, developers can maximize code reusability, maintainability, and scalability while minimizing potential code inconsistencies and redundancies.
Applications of Callbacks in Real-World Scenarios
Callbacks in real-world scenarios hold immense importance for developers and IT professionals. They enable efficient event-driven programming, asynchronous operations, and modular code development. These applications are pivotal in enhancing code maintainability and scalability, fostering a well-structured and adaptable codebase. By leveraging callbacks, developers can handle user interactions in web applications, fetch data from APIs asynchronously, and process large datasets without blocking the main thread. The versatility of callbacks in real-world scenarios makes them indispensable tools for creating robust and flexible software solutions.
Event-Driven Programming
Handling user interactions in web applications
Handling user interactions in web applications plays a crucial role in enhancing user experience and functionality. By utilizing callbacks, developers can respond dynamically to various user actions, such as clicks, scrolls, and inputs. This responsiveness enables real-time updates and interactive features, enriching the overall user engagement. Implementing callback functions for user interactions allows for seamless communication between the user interface and the underlying logic, ensuring a smooth and intuitive user experience.
Broadcasting and subscribing to events
Broadcasting and subscribing to events facilitate efficient communication between different components of a system. By employing callbacks for event handling, developers can establish clear channels for broadcasting events and subscribing to specific notifications. This pub/sub model boosts system scalability and modularity, enabling independent components to interact seamlessly without direct dependencies. Although event-driven programming using callbacks offers enhanced flexibility and scalability, it may introduce complexity in managing event flows and subscriptions, necessitating careful planning and design.
Asynchronous Operations
Fetching data from APIs asynchronously
Asynchronous data fetching through callbacks enhances application performance by eliminating delays caused by synchronous requests. By leveraging callback functions, developers can initiate API calls without blocking the main thread, allowing other operations to continue in parallel. This approach streamlines data retrieval processes, improves responsiveness, and optimizes resource utilization. However, asynchronous operations using callbacks require careful error handling and callback chaining to manage dependencies and ensure data consistency.
Processing large datasets without blocking the main thread
Efficient processing of large datasets without blocking the main thread is vital for maintaining a responsive and fluid user interface. Callbacks enable developers to execute time-consuming tasks in the background, preventing UI freezes and improving overall application responsiveness. By utilizing callbacks for asynchronous processing, developers can implement efficient data chunking, parallel processing, and progress tracking, ensuring smooth performance even when handling extensive data sets.
Modular Code Development
Creating reusable and modular code components
Developing reusable and modular code components using callbacks fosters code reusability and extensibility. By structuring code into separate modules with well-defined interfaces, developers can encapsulate functionality and promote code reuse across different parts of an application. Callbacks enable effective inter-module communication, allowing components to interact without tight coupling, enhancing code modularity and flexibility. However, excessive reliance on callbacks for code modularity may lead to callback hell, a situation where nested callbacks complicate code readability and maintenance.
Enhancing code maintainability and scalability
By enhancing code maintainability and scalability, callbacks contribute to the long-term viability and growth of software projects. Modular code development with callbacks enables easier maintenance, debugging, and feature expansion, as changes in one module do not necessitate modifications in unrelated parts of the codebase. This modular approach streamlines code updates and facilitates collaborative development, enhancing team productivity and project sustainability. Despite these benefits, overusing callbacks in modular code development can introduce callback pyramid issues, where excessive callback nesting impairs code comprehensibility and maintainability.