Understanding C Programming: An In-depth Exploration
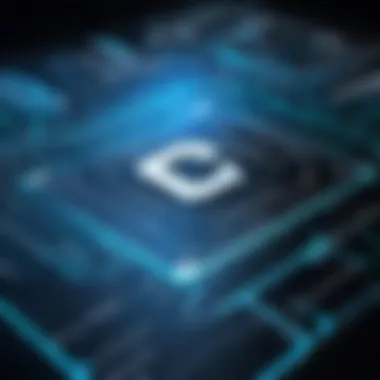
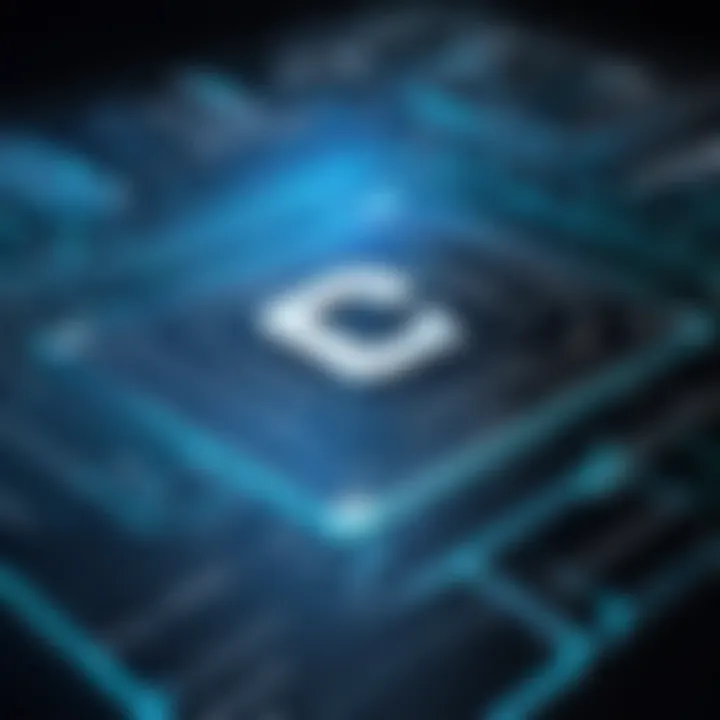
Intro
The C programming language plays a crucial role in the landscape of modern computing. Developed in the 1970s, its significance extends beyond its age, shaping various programming languages and influencing countless systems. C remains a foundation upon which many technologies and frameworks are built. This section serves as a brief introduction to why understanding C is vital for software development and other technical domains.
While many newer languages have emerged, C's efficiency and control continue to make it relevant. It offers a unique balance of low-level access and high-level abstraction. Whether working with operating systems, embedded devices, or performance-critical applications, C proves to be a preferred choice among developers.
The exploration of C programming provides essential learning for both novices and experienced professionals. It covers syntactic rules, structural insights, and practical applications, giving readers a comprehensive perspective on C's enduring legacy in today’s tech environment.
Understanding C not only enhances coding skills but also helps in grasping core programming concepts that are applicable across many modern programming languages. As we delve into the specifics, we will examine the foundational aspects that offset the need for C's role in the ever-evolving tech industry.
Prelims to Programming
C programming is a fundamental topic essential for understanding modern computing. Its significance transcends mere syntax and semantics; it embodies the principles of structured programming and serves as the precursor to many languages used today. Knowing C can unlock a deeper comprehension of programming concepts, enabling developers to tackle more complex languages with ease.
History and Evolution
Developed in the early 1970s by Dennis Ritchie at Bell Labs, C was conceived as an enhancement of the B programming language. By establishing a more familiar syntax and incorporating low-level access to memory, C quickly gained traction in system programming. Notably, its role in operating system development, starting with UNIX, positioned C as a transformative force in computer science. Over the years, the language has undergone various updates, resulting in the standardization under ANSI in 1989 and ISO in 1999, which preserved its relevance in an evolving technological landscape. The legacy of C is not limited to its original form; it has inspired many modern programming languages, including C++, C#, and Objective-C.
Significance in Computing
In the realm of computing, C programming remains critical due to several factors:
- Performance and Speed: C is a compiled language, enabling it to execute programs rapidly. This efficiency is crucial for high-performance applications, such as operating systems and real-time systems.
- Memory Management: C provides direct control over memory allocation and deallocation, which is essential for systems programming and developing performance-sensitive applications.
- Portability: Code written in C can easily be adapted to different platforms. This characteristic contributes to its widespread adoption in various fields, from embedded systems to game development.
- Foundation for Other Languages: Many higher-level languages borrow concepts from C, making it easier to learn new programming paradigms if one has a solid grasp of C programming.
C programming is not just a historical artifact; it continues to shape the future of computing. Understanding C and its fundamental principles prepares developers for any future challenges in software development.
Syntax and Structure
Understanding syntax and structure is pivotal when working with C programming. The way code is formatted dictates not only its functionality but also its clarity and maintainability. Proper syntax ensures that the compiler can read the instructions accurately, while a well-thought-out structure aids programmers in organizing their code logically.
By easily identifying and understanding different parts of the code, developers can troubleshoot issues more effectively and collaborate with others smoothly. Syntax errors can halt a program in its tracks, making a comprehensive understanding of syntax not just beneficial but necessary for efficient problem-solving.
Basic Syntax Rules
In C programming, adhering to basic syntax rules is essential for successful coding. Each line typically ends with a semicolon, indicating the termination of a statement. Comments can be written using for single-line comments or for multi-line comments, helping in documenting code without affecting its execution.
Here are some fundamental points to keep in mind:
- Each statement must end with a semicolon.
- Proper indentation is critical for readability, although it does not affect functionality.
A sample of basic syntax:
Understanding these rules allows developers to write clear and efficient code that can be easily read and maintained.
Data Types and Variables
Data types and variables are crucial constructs in C programming. They define the nature of data and how it can be manipulated. C supports several basic data types such as , , , and . Each type serves distinct purposes and occupies different memory sizes, impacting performance and storage.
Variables in C must be declared before they are used, specifying their data type. This requirement enforces type safety, ensuring that operations on these variables are valid. Here’s a brief overview of common data types:
- int: Stores integers (whole numbers), often used for counting.
- float: Represents floating-point numbers, suitable for precise calculations.
- char: Holds a single character, useful in text processing.
- double: Similar to float but with double precision.
Example of variable declaration:
Understanding data types helps developers choose appropriate types for their variables, optimizing memory usage and performance.
Control Structures
Control structures dictate the flow of execution in C programs, allowing for decision-making and repetition. They are fundamental to creating efficient and dynamic applications. The primary control structures in C include conditionals (such as , , and ) and loops (like , , and ).
- If Statements: Used to execute code blocks based on conditions. This is critical for branching logic.
- Switch Statements: Allow for multiple conditions without deep nesting, enhancing code clarity.
- For Loops: Ideal for scenarios with a known number of iterations.
- While Loops: Best for situations requiring indefinite iteration until a condition is met.
Example of a control structure using an statement:
Control structures empower developers to build logic into their applications, making them not just reactive but proactive in their function.
Core Concepts of Programming
The core concepts of C programming form the backbone of the language, providing developers with the necessary tools to create efficient and effective software. Understanding these concepts is essential not only for writing C code but also for grasping programming principles that can be applied across various languages. This section sheds light on key elements such as functions, pointers, memory management, arrays, and strings, ensuring a solid foundation in C programming.
Functions and Scope
Functions are fundamental building blocks in C programming. They allow developers to encapsulate code into reusable and self-contained units. By defining functions, programmers can organize their code in a logical and coherent manner. This organization enhances readability and maintainability.
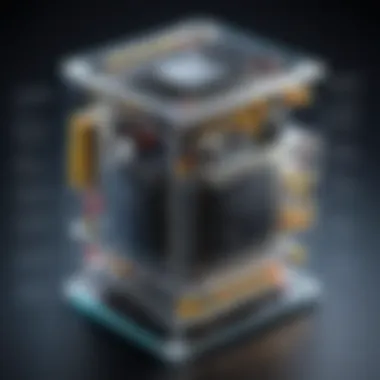
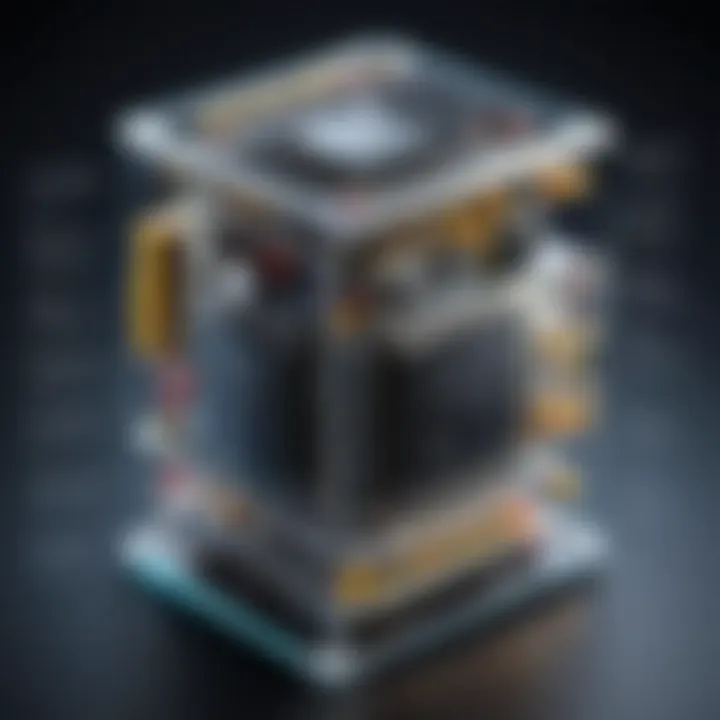
Scope, on the other hand, refers to the visibility and lifetime of variables defined within a function. In C, there are different types of scope including local and global. Local variables are accessible only within the function they are defined in, while global variables can be accessed throughout the program. This distinction is crucial for managing data and avoiding unwanted side effects.
Functions also promote modular programming. By breaking down complex tasks into simpler functions, developers can improve collaboration and testing processes. Programmers can develop and test functions independently before integrating them into larger projects.
Pointers and Memory Management
Pointers are a unique feature of C programming that allows for direct memory access and manipulation. A pointer holds the address of a variable, providing powerful capabilities for dynamic memory management. Understanding pointers is crucial for efficient programming, especially in system-level applications.
Memory management in C is manual. Programmers use functions like , , and to allocate and deallocate memory dynamically. This control can improve performance but comes with risks such as memory leaks if not managed correctly. Developers should be proficient in proper memory management techniques to optimize resource usage and application stability.
Memory management is an art and a science. Mastering it will distinguish competent developers from remarkable ones.
Arrays and Strings
Arrays are a collection of elements of the same type. They enable developers to store multiple values in a single variable, which simplifies data handling. Arrays can be static or dynamic, with dynamic arrays allowing for greater flexibility.
Strings, which are arrays of characters, are fundamental in C. The language does not have a dedicated string data type. Instead, strings are handled as character arrays terminated by a null character (). This convention allows strings to be manipulated with functions like and , which perform operations on strings effectively.
Understanding how to use arrays and strings efficiently can save time and increase the performance of applications. Proper usage of these structures is vital for tasks ranging from basic data storage to complex algorithms.
In sum, mastering the core concepts of C programming is essential for any software developer or tech enthusiast. By understanding functions, pointers, memory management, arrays, and strings, one can build robust applications and systems that adhere to the principles of efficiency and clarity.
Advanced Topics in Programming
Advanced topics in C programming serve as a bridge between mastering the basics and tackling complex programming challenges. They allow developers to optimize their code for efficiency and maintainability. Understanding these topics is essential for anyone seeking to harness the full power of C, particularly in system-level programming and performance-critical applications. Focusing on advanced features, developers can improve their code's readability while also enhancing its functionality.
Structures and Unions
Structures and unions are fundamental data types that enable developers to create complex data models. A structure groups different data types into a single unit, while a union uses the same memory space for multiple types, but only one can be active at a time.
In C programming, structures are defined with the keyword. They are ideal for representing entities with multiple attributes. For instance, a can be used to define a with fields like , , and :
Conversely, unions are defined using the keyword. Since all members share the same memory location, unions are memory-efficient but come with limitations. The following code shows how to use a union:
When deciding to use structures or unions, consider data requirements and memory cost. Structures are suitable when all members need to maintain their values simultaneously. Unions, however, are preferable when the memory footprint is crucial, for example, in embedded systems.
A crucial consideration is that using a union requires careful management, as overwriting one member affects the integrity of the data in the others.
File /O Operations
File input/output (I/O) operations are essential for persistent data storage. C provides standard library functions for handling files through a simple but effective syntax. Understanding how to perform these operations allows developers to read from and write to files, making data manipulation far more versatile.
To work with files, one must first declare a file pointer using the structure. The function opens a file based on the specified mode, for example, read () or write (). Here's a brief example:
Once the file is open, various functions can be employed. For writing, can be used, while reading can utilize . When finished, it's crucial to close the file with to release resources:
File I/O operations require attention to error handling starting from the file opening process. Ensure to check if the pointer returned by is , indicating any issues that occur:
Practical Applications of Programming
C programming transcends simple coding lessons; its practical applications span across various domains in technology. Understanding these applications is vital as they illustrate C's real-world relevance. This section breaks down the key areas where C programming plays an indispensable role, addressing both benefits and considerations.
Operating Systems Development
C is often considered the language of operating systems. Many popular operating systems, including Unix, Linux, and Windows, are largely written in C. This is due to its ability to interact directly with the hardware while maintaining a relatively high-level syntax that is readable for developers.
- Performance: C provides fine control over system resources, enabling efficient memory usage and fast execution speeds. This is crucial for operating systems that must manage multiple processes simultaneously.
- Portability: C programs can be compiled on numerous architectures with minimal modification. This aspect fosters the development of operating systems that can run on various hardware, enhancing adaptability and usage.
- System-level Access: The language allows programmers to write low-level code, giving access to hardware interrupts and memory management, which is necessary for core functionality of an operating system.
In operating systems development, understanding pointers and memory management is essential due to the need for handling hardware resources efficiently.
Embedded Systems Programming
Embedded systems represent a significant sector where C is omnipresent. These systems are specialized computing devices part of larger systems, often designed to perform specific tasks. Variants of embedded systems range from household appliances to medical devices.
- Real-time Performance: In embedded programming, C helps develop applications that meet strict timing constraints. Quick and deterministic responses are often required, making C an ideal choice for such environments.
- Direct Hardware Manipulation: Many embedded systems require direct interaction with hardware components. The capacity of C to manipulate data at a low level gives it an edge in this domain.
- Resource Constraints: Embedded devices frequently operate under tight resource limitations. C's capacity for fine-tuned memory and performance management aligns well with the needs of these restrictive environments.
Embedded systems programming can range from simple tasks in a washing machine to complex operations in autonomous vehicles. Thus, a deep understanding of C is vital for professionals in this field.
Game Development
Another area where C programming shines is game development. While many might associate higher-level languages with easier game design, C remains a critical component for performance-centric games.
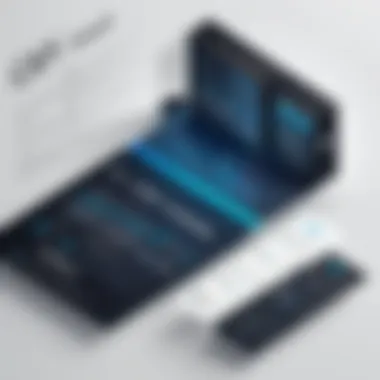
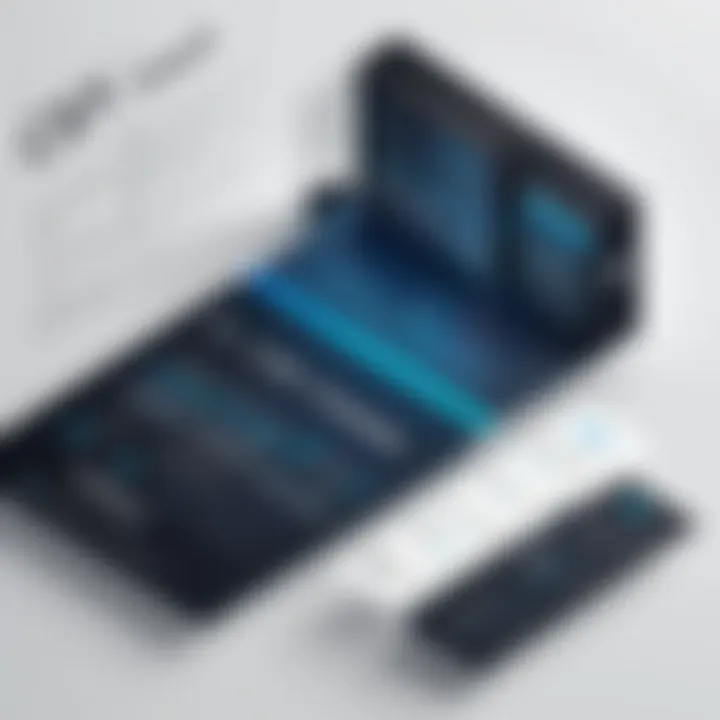
- Speed and Efficiency: Game engines often require swift execution to maintain high frame rates and responsive controls. C’s capabilities in optimization allow developers to leverage it for performance-critical sections of their code.
- Graphics Libraries: Many graphics APIs, such as OpenGL and DirectX, provide C bindings for low-level access to graphics hardware. This enables game developers to create visually impressive and interactive experiences.
- Cross-Platform Development: C supports the development of games that can operate on different platforms without significant rewrites. This portability allows games to reach broader audiences across devices.
In summary, the applications of C programming are diverse and impactful, particularly in fields such as operating systems development, embedded systems programming, and game development. By mastering C, software developers and IT professionals position themselves favorably to tackle complex challenges in these sectors.
C programming continues to serve as a foundation for all forms of software development, enabling efficiency and versatility across multiple domains.
C programming's relevance isn't limited to its syntax; it extends to enhancing students’ and professionals' careers for a lifetime.
Programming Across Platforms
The versatility of C programming is a significant factor in its enduring popularity. By facilitating cross-platform compatibility, C allows for the development of software that can run on various systems with minimal modification. This section will delve into how C operates across different platforms, highlighting its role in system software, application software, and web development.
in System Software
One of the pivotal areas where C programming shines is in system software development. Operating systems such as Windows, Linux, and macOS rely heavily on C due to its proximity to hardware and its efficiency. The ability to manipulate memory and handle low-level tasks gives developers a powerful tool to create robust systems.
The advantages of using C in system software include:
- Performance: C programs exhibit fast execution speeds, which is critical when managing system resources.
- Control: Developers have fine-grained control over system hardware, making it easier to optimize performance and resource management.
- Portability: C’s relatively simple standards mean that code can often be ported to different systems with little effort.
Popular operating systems, such as the Linux kernel, are predominantly written in C, showcasing the language's efficiency and reliability in handling essential system processes. The combination of its speed, performance, and elegance makes C a prime choice for any system-level programming.
in Application Software
In the realm of application software, C provides a reliable foundation for a wide array of programs ranging from simple utilities to complex enterprise applications. Many software applications, especially those needing high performance and resource efficiency, are developed using C.
Some key points about C’s use in application software include:
- Highly scalable: Developers can create applications that perform well under different loads, making C an excellent choice for server-side applications.
- Compatibility with other languages: C serves as a bridge between other high-level languages. For instance, many Python libraries contain components written in C for performance optimization.
- Community and resources: A robust community supports the C programming language, providing developers an abundance of libraries and frameworks that simplify application development.
This adaptability makes C an attractive option for building application software that requires speed and optimal performance.
in Web Development
With the rise of web technologies, the role of C in web development has evolved. While languages like JavaScript, PHP, and Python dominate web development, C still contributes significantly in certain areas—especially on the server side or in the development of web server infrastructure.
Consider the following aspects related to C in web development:
- Web server performance: C can be utilized in the development of high-performance web servers. Nginx, for instance, is written in C and is known for its ability to handle concurrent connections efficiently.
- Handling data: C can be used for backend processes where processing speed is paramount, such as in algorithms that handle large amounts of data.
- Infrastructural support: Many web-based technologies rely on C for their lower-level components, such as web browsers and database servers.
In summary, while it may not be the first choice for all web development scenarios, C's efficiency and performance make it an essential underpinning for various web applications and services.
"C programming language is fundamental in various applications, providing the tools and performance necessary for today’s technological demands."
Programming Libraries and Frameworks
C programming libraries and frameworks play a crucial role in streamlining the development process. These tools allow programmers to utilize pre-written code, which can increase efficiency and reduce the likelihood of errors. Understanding both standard and third-party libraries is key for any C programmer. This section elaborates on their significance and presents considerations for effective use.
Standard Libraries
Standard libraries in C programming are built-in sets of functions and macros that provide a robust framework for tasks such as input-output operations, string manipulation, and memory allocation. The core standard library, known as the C Standard Library, is accessible via the standard header files such as , , and . These libraries are essential for any programmer as they save time and effort by providing commonly needed functionality.
- Key Benefits of Standard Libraries:
- Efficiency: They are optimized and tested by the community, ensuring better performance.
- Portability: Code utilizing standard libraries is often more portable across different platforms.
- Ease of Use: Functions within these libraries have well-defined behaviors and are documented comprehensively.
One common example is the function from , which simplifies output to the console. By leveraging this function, a developer can easily print formatted text.
By using standard libraries, developers do not need to code everything from scratch. This leads to faster development cycles. Moreover, the community is always updating and maintaining these libraries, which means they are a secure choice in many cases.
Third-party Libraries
While standard libraries offer many functionalities, third-party libraries expand the capabilities of C programming. These libraries are often created by other developers or organizations and can be integrated into projects to add additional features.
- Advantages of Third-party Libraries:
- Specialization: Many libraries focus on specific tasks, such as graphics rendering or network communication.
- Active Development: Many have vibrant communities that regularly update and enhance their offerings.
- Innovation: They often introduce new features and methodologies, keeping programmers at the cutting edge.
Examples of popular third-party libraries include GTK for creating graphical user interfaces and libcurl for transferring data with URLs. These libraries enable developers to implement complex functionalities without the need to manually code them.
When choosing third-party libraries, it is important for developers to evaluate factors such as documentation, community support, and compatibility with existing code. Missteps in this selection process can lead to integration issues and increased maintenance burdens.
"Utilizing libraries frees developers from the mundane, allowing them to focus on the unique logic of their applications."
For further reading on libraries and frameworks in C, you may visit Wikipedia or check community discussions on Reddit.
Common Challenges in Programming
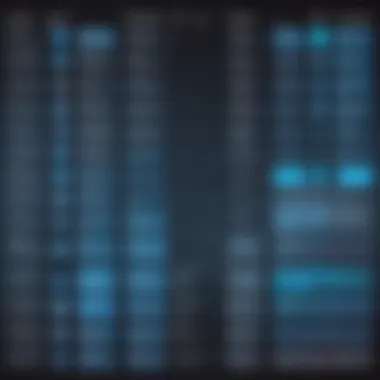
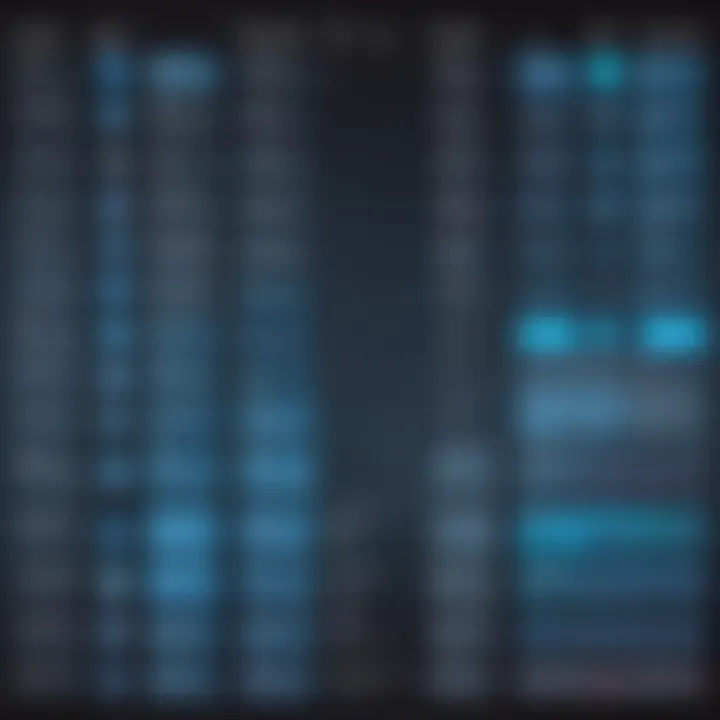
C programming presents various challenges that can be daunting for both novices and experienced developers. Understanding these common obstacles is crucial as they can significantly impact the development process. Addressing these challenges can enhance the quality of code produced and increase efficiency. Among the prominent challenges faced by programmers in C are debugging and memory management, particularly memory leaks. Engaging deeply with these aspects aids in developing robust software applications.
Debugging Techniques
Debugging is an essential skill in programming. The process involves identifying and resolving errors or bugs within the code. C's low-level nature can complicate debugging. To effectively debug C programs, developers often rely on various techniques:
- Print Debugging: This method includes inserting print statements into the code to inspect variable values at different execution stages. It is straightforward but may disrupt the program flow.
- Using GDB: GDB, the GNU Debugger, is a powerful tool in C programming. It allows programmers to execute their code step-by-step, set breakpoints, examine memory, and control the execution flow. Familiarity with GDB expands the debugging toolkit.
- Static Analysis Tools: These tools analyze code without executing it. They detect potential issues, such as syntax errors and pinpoint unreachable code.
- Dynamic Analysis Tools: Running the program and observing its behavior is crucial. These tools help identify runtime errors and resource leaks that static tools may miss.
Utilizing a combination of these techniques is advisable for effective debugging. By being thorough in debugging practices, developers can ensure code runs smoothly and adheres to the desired functionality. This approach enhances confidence in software quality and reliability.
Memory Leaks and Solutions
Memory management is vital in C programming due to the absence of automatic garbage collection. A common issue that surfaces is memory leaks. A memory leak occurs when a program allocates memory but fails to release it after use, leading to decreased performance over time. Understanding how to identify and rectify memory leaks is paramount for maintaining resource efficiency.
Some strategies to combat memory leaks include:
- Allocating and Freeing Memory: Developers should always match each or with a corresponding statement. This practice is the foundation of proper memory management in C.
- Tools for Detection: Valgrind is a prominent tool in detecting memory leaks. It monitors memory use and signals where leaks occur. Regularly using such tools can greatly reduce memory leak occurrences.
- Code Review Practices: Peer reviewing code can help identify potential leaks. A fresh set of eyes may catch mistakes that the original developer overlooked.
- Creating Allocation Patterns: By establishing consistent patterns for allocating and freeing memory, developers can reduce the likelihood of leaks. Developing habits such as initializing pointers to aids in catching uninitialized pointer dereferences.
In summary, encountering challenges in C programming is unavoidable; however, understanding and addressing them can lead to better software practices. Debugging techniques and vigilance regarding memory management are critical components for success in this domain. The ability to troubleshoot and optimize code is what distinguishes proficient developers from their peers.
"In C programming, attention to detail is vital. Small mistakes can lead to significant issues."
By equipping themselves with the knowledge and tools to overcome these challenges, programmers can significantly enhance their coding practices.
The Future of Programming
The C programming language holds a unique position in the landscape of computer science. Emerging from a time when hardware resources were limited, it has proven its resilience and adaptability over decades. The future of C programming is not merely an extension of its past; it is being shaped by evolving standards and the integration of new technologies. Understanding these developments is crucial for software developers, IT professionals, data scientists, and tech enthusiasts who wish to remain relevant in the fast-paced industry.
C's simplicity and efficiency allow it to be used in a variety of contexts, from system programming to applications in data science and artificial intelligence. As technology advances, it becomes increasingly important to recognize how C can thrive alongside modern innovations.
Evolving Standards
ISO C, often referred to as C11 or the newer C18, continues to evolve to incorporate features that meet the demands of contemporary programming. New standards aim to make C safer and more efficient. The standardization process not only addresses issues of portability but also emphasizes strong type checking, improved multithreading capabilities, and enhanced support for Unicode.
Keeping up with these standards is essential. As developers continue to embrace best practices in software development, the evolution of C reflects a growing need for language features that cater to modern programming paradigms. Some critical areas of focus include:
- Improved error handling processes
- New library functions for better performance
- Compatibility with concurrent programming contexts
A commitment to learning and applying these new standards can significantly influence a developer's effectiveness and versatility.
Integration with Emerging Technologies
C's role in emerging technologies is becoming increasingly significant. As Internet of Things (IoT) devices proliferate, C remains a primary language for embedded systems. Its low-level capabilities allow for close interaction with hardware, making it ideal for the performance constraints of IoT devices.
In fields such as machine learning and data science, C also acts as a foundation for performance-sensitive components of larger frameworks. Libraries like TensorFlow are often written in C or C++ to maximize their speed and efficiency.
Moreover, C's relevance in systems programming is unchallenged. Many operating systems, including Linux, are predominantly written in C, and maintaining such software ensures a continuous demand for skilled C programmers.
"The future of C programming is bright, not because it is outdated, but due to its foundational role in new technologies, ensuring its relevance for years to come."
Educational Resources for Programming
In the realm of programming, continuous education is vital for both skill enhancement and adapting to new technologies. C programming is no exception to this necessity. Educational resources play a crucial role in guiding learners through the complexities of C. They bridge gaps in knowledge and empower individuals at various stages of their career, fostering both proficiency and confidence.
Identifying the right resources can significantly impact a learner's development. Various forms of educational materials exist, including online courses, tutorials, books, and publications. They cater to different learning styles, offering both structured and flexible learning paths. Benefits of these resources encompass a thorough understanding of concepts, practical applications, and a strong foundation for more advanced studies.
Furthermore, resource quality must be considered; reputable sources can provide accurate theories and code examples, while subpar materials may lead to confusion. It is therefore imperative to approach educational content critically, discerning which materials will genuinely enhance your understanding of C programming.
"Learning never exhausts the mind." - Leonardo da Vinci
Below are essential types of resources:
- Online Courses: Perfect for those seeking structured learning.
- Books and Publications: Excellent for deep dives into subjects and reference materials.
- Tutorials and Articles: Great for quick guides on specific topics.
Online Courses and Tutorials
Online courses have emerged as a favored method to learn C programming. They offer structured environments, guided by experienced instructors to lead learners through the language's fundamentals and intricate details. Interactive coding exercises allow for immediate application of knowledge.
Many platforms deliver high-quality content. Websites like edX or Coursera often partner with leading universities, providing not just tutorials but complete courses with certification options. They focus on practical projects, enabling students to build a portfolio alongside their knowledge. For those self-motivated, platforms like Codecademy or freeCodeCamp offer excellent resources at little to no cost.
Benefits of Online Learning
- Flexible scheduling accommodates varied lifestyles.
- Interactive elements enhance engagement and retention of information.
- Access to diverse instructors with differing teaching methodologies.
To gain the most from online courses, engage actively with the materials. Participate in discussions, complete exercises diligently, and seek out peer feedback. This approach encourages community learning and deeper insights.
Books and Publications
Books and publications remain fundamental for deepening knowledge about C programming. While online courses provide great interactive experiences, books often present comprehensive coverage of principles and theories. They allow self-paced study and can be revisited for reference.
Notable books include "The C Programming Language" by Brian W. Kernighan and Dennis M. Ritchie, often considered an authoritative reference. Another essential read is "C Programming: A Modern Approach" by K. N. King, which covers C with clarity and depth.
Considerations When Choosing Books
- Ensure that the publication is current to reflect recent updates in C standards.
- Look for well-reviewed books that offer practical examples alongside theoretical knowledge.
- Opt for books that include exercises to reinforce learning.
Investing time in quality books can greatly enhance one’s understanding of C programming. This continual engagement is the key to mastering the language and applying its principles in real-world scenarios.