Understanding Asynchronous Programming: Concepts and Applications
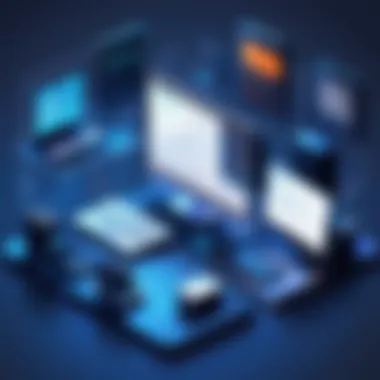
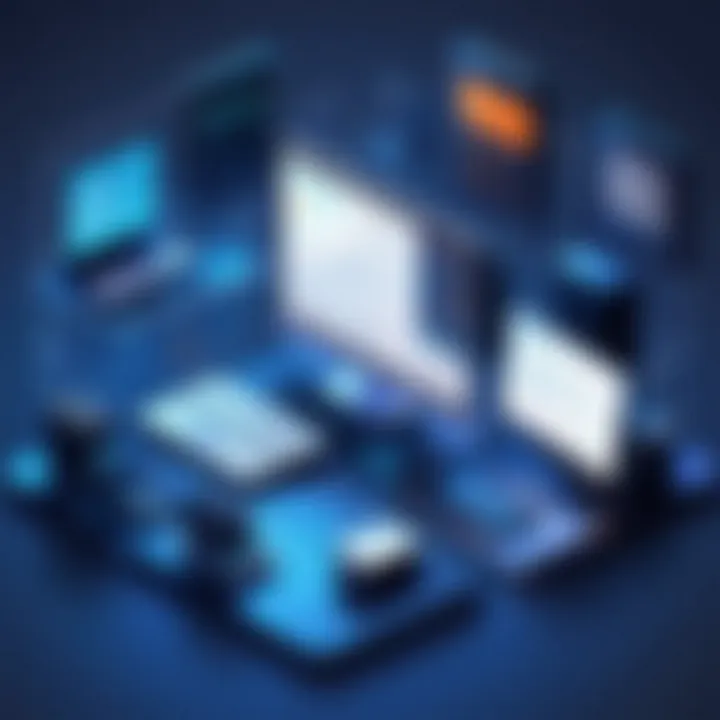
Intro
Asynchronous programming is a pivotal aspect of modern software development. As applications evolve, demands on performance and user experience intensify. This method allows programs to perform operations without waiting for a prior task to finish. It ensures smooth interaction, particularly in environments like web applications where speed is crucial.
The rise of cloud computing and the proliferation of data analytics underscore the importance of such programming techniques. These technologies necessitate handling multiple processes simultaneously, enhancing the overall efficiency of applications. Asynchronous programming meets this demand and addresses common challenges of concurrency, making it an indispensable tool in any developer’s arsenal.
Implications of Asynchronous Programming in Software Development
Software development has moved towards more complex architectures. The shift has been driven by the need for responsiveness and scalability in applications. Asynchronous programming allows developers to create more interactive applications. This is particularly relevant in areas such as data streaming, API interaction, and real-time processing.
The importance of asynchronous programming grows in the context of cloud computing. With services like Amazon Web Services and Microsoft Azure, applications are expected to handle large volumes of requests efficiently. Utilizing asynchronous techniques means that developers can better manage resources while improving the speed of data processing.
In data analytics, the ability to execute many tasks concurrently accelerates the analysis of large datasets. It simplifies operations like data fetching and processing, enhancing productivity and unlocking insights in real-time.
Overall, asynchronous programming represents a shift towards more effective and efficient coding practices, recognizing the need for performance in a fast-paced digital environment.
Prologue to Asynchronous Programming
Asynchronous programming is a critical aspect of contemporary software development. It has become increasingly important due to the rise of high-performance applications and the need for enhanced user experiences. This section aims to establish the foundational elements of asynchronous programming, emphasizing its significance in various programming contexts.
Defining Asynchronous Programming
Asynchronous programming is a programming paradigm that enables a program to perform tasks without waiting for previous tasks to complete. In simpler terms, it allows multiple operations to occur simultaneously, yielding better utilization of resources. The core idea is to prevent blocking operations, enabling applications to remain responsive, particularly in user interface design.
For instance, when a web application fetches data from a server, it can do so asynchronously. This means the user can continue interacting with the app while the data is being retrieved. Not only does this approach enhance user experience, but it also improves efficiency by allowing the CPU to handle other tasks instead of idling while waiting for an operation to finish.
Historical Context and Evolution
The evolution of asynchronous programming can be traced back to the early days of computing. Initially, programs operated in a sequential manner. As application demands grew, especially with the introduction of graphical user interfaces, the need for non-blocking behavior became apparent. Early implementations often relied on threads or processes to achieve concurrency.
In the late 1990s and early 2000s, Java introduced the concept of Futures and Callables, laying groundwork for modern asynchronous techniques. Meanwhile, JavaScript, particularly with the advent of Ajax, began to adopt asynchronous programming methods to enhance the performance of web applications.
With the introduction of Promises in ECMAScript 6 and Async/Await syntax in later versions, JavaScript further simplified asynchronous programming for developers. Other languages, such as Python with its asyncio library, and C# with async/await keywords, followed suit, drawing attention to the need for better management of asynchronous code.
Asynchronous programming is not merely a trend; it is a fundamental shift toward creating responsive, efficient applications in a connected world.
Understanding the historical context provides insights into how asynchronous programming emerged and why it is indispensable today. The demand for optimized performance and fluid user experiences drives the ongoing evolution of this programming approach.
The Need for Asynchronous Programming
Asynchronous programming is increasingly crucial in the realm of software development, primarily due to the demands of modern applications and user expectations. Applications today require efficiency, scalability, and responsiveness. Prioritizing these elements helps to enhance the overall user experience. Asynchronous programming allows developers to execute tasks without waiting for previous operations to complete, significantly improving application throughput.
User Experience Considerations
User experience is at the heart of web and mobile application design. Asynchronous programming plays a vital role in maintaining a seamless interaction between users and applications. For example, when users initiate a request—such as loading online content or submitting a form—an application can continue running other functions in the background. This prevents the user interface from freezing and provides real-time feedback. If the system must pause for an operation to complete, it can result in frustration among users, leading them to abandon the application entirely.
By allowing certain processes to continue running, developers can create a more fluid interaction. Techniques such as lazy loading and background data fetching rely heavily on asynchronous capabilities to optimize performance and keep users engaged. A well-executed asynchronous strategy is essential in delivering a satisfactory user experience.
Performance Optimization Techniques
Performance optimization is a significant concern for developers. Asynchronous programming introduces various techniques that help achieve this goal. Here are some of the most relevant strategies:
- Concurrency Control: Breaking down tasks into smaller, manageable pieces lets multiple operations occur simultaneously. This reduces wait times and increases resource utilization.
- Using Promises: Promises are a cornerstone of modern asynchronous programming. They provide a clear structure for handling asynchronous operations, leading to more organized and maintainable code.
- Utilizing Async/Await Syntax: This approach simplifies promise handling, enabling developers to write asynchronous code that appears synchronous, thus improving readability.
- Throttling and Debouncing: These techniques help control the frequency of function calls, especially when dealing with user input or events, leading to significant performance gains in high-interaction environments.
Employing these strategies can lead to substantial improvements in application performance, enabling systems to handle increased loads while providing a smoother, more responsive user experience.
"In the world of software development, efficiency is not just about speed, it's about providing a framework that keeps users engaged while performing backend operations."
By understanding the need for asynchronous programming and employing these techniques, developers can create high-performing applications that adapt to users' expectations and needs.
Core Concepts in Asynchronous Programming
Asynchronous programming is a critical concept in modern software development. It allows for more efficient execution of tasks, especially when dealing with I/O operations, which can often be time-consuming. Understanding the core concepts of asynchronous programming lays a foundation not only for better performance but also for enhanced user experience in applications. Key elements include the event loop, callbacks, promises, and the async/await syntax. Each of these components plays a vital role in how applications manage concurrency and resource usage.
Event Loop Mechanism
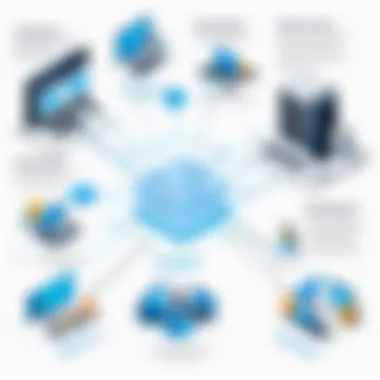
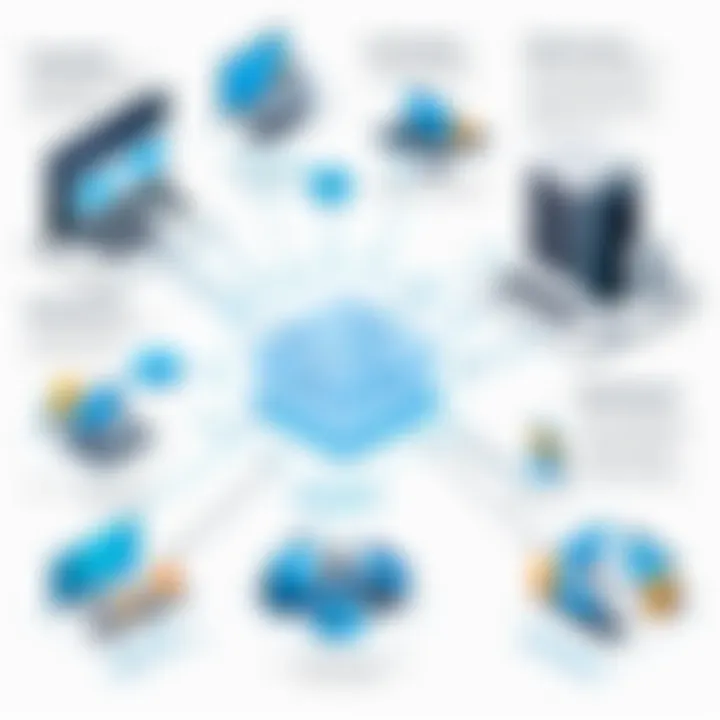
The event loop is a fundamental aspect of asynchronous programming. It enables a program to perform non-blocking operations, effectively allowing the execution of multiple tasks concurrently. The event loop processes callbacks and events in a single-threaded environment, making it possible to handle numerous I/O operations without freezing or crashing the application.
- How it works: The event loop constantly checks a queue of messages and executes the top-most task. It only processes one task at a time, ensuring that the main thread remains responsive.
- Importance: This mechanism is especially significant in environments like JavaScript, where traditional multithreading is avoided in favor of a more efficient model. It simplifies concurrent programming, making it easier for developers to handle multiple asynchronous tasks without complex thread management.
Callbacks and Event Handlers
Callbacks are a foundational building block in asynchronous programming. They are functions passed as arguments to other functions and are executed at a later time, usually in response to an event or a signal indicating that a task has completed.
- Usage: Callbacks are extensively used in event-driven programming, especially in JavaScript, where they allow developers to write non-blocking code. After a request for data is made, the function can return immediately, letting the rest of the code run while awaiting the response. Once the data is available, the callback function is triggered.
- Considerations: While callbacks are powerful, they can lead to complex code structures known as "callback hell" if not properly managed. This can reduce code readability and maintainability. It is crucial to structure callbacks wisely and consider alternative methods like promises or async/await to enhance clarity.
Promisification of Functions
Promisification refers to the process of converting functions that use callbacks into functions that return promises. Promises are objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value.
- Benefits: This approach helps streamline error handling and makes the code more manageable. Instead of dealing with nested callbacks, developers can chain promises, leading to cleaner and more readable code.
- Implementation: For example, a function that reads a file could be promisified to return a Promise, allowing the function to either resolve with the file data or reject with an error if the operation fails. This clear separation enhances the maintainability of the code.
Async/Await Syntax in Modern Languages
The async/await syntax is a significant advancement in asynchronous programming, offering a more straightforward way to work with promises. This syntax allows developers to write asynchronous code that looks synchronous, enhancing readability and reducing complexity.
- Async functions: Declaring a function with the keyword enables the use of the keyword within. This effectively pauses the execution of the function until the awaited promise settles.
- Reduction of complexity: This contrasts significantly with traditional promise chaining, making it easier to read and maintain code. As a result, developers can handle asynchronous code in a linear fashion, which is often easier to follow.
"The async/await syntax helps in managing promises more intuitively, reducing callback complexity and making code easier to understand."
In summary, these core concepts of asynchronous programming form a crucial basis for developing responsive and efficient applications. Understanding how the event loop operates, how to effectively utilize callbacks, and the advantages of promises and async/await syntax will empower developers to write cleaner and more effective asynchronous code.
Asynchronous Programming in Different Languages
Asynchronous programming plays a crucial role in modern software development, impacting how applications manage tasks and respond to various events. This section explores how different programming languages integrate asynchronous concepts, showcasing unique features and benefits. Understanding the implementation of asynchronous programming in various languages enriches developers' aptitude to write efficient, responsive applications.
JavaScript and Node.js Approaches
Basics of Async in JavaScript
JavaScript has become synonymous with asynchronous programming, especially in web development. The primary characteristic of asynchronous behavior in JavaScript is its non-blocking nature. This allows long-running tasks, such as network requests, to run in the background while the main thread continues processing other code. This behavior enhances user experience by preventing the browser from freezing during heavy computations.
A unique feature of JavaScript's approach to async is its event loop. It handles executing code, collecting events, and processing messages, allowing for smooth interactions. The advantages include improved load times and responsiveness. However, JavaScript's asynchronous framework can lead to complexity, especially when dealing with multiple asynchronous operations, often referred to as "callback hell."
Using Async/Await with Node.js
The syntax in Node.js offers a more manageable way to work with asynchronous code. Its key characteristic is the ability to write asynchronous code in a way that resembles synchronous code, which aids readability. This method allows developers to write cleaner, more maintainable code by reducing the need for deeply nested callbacks.
An essential feature of using in Node.js is error handling. It simplifies capturing errors in asynchronous functions using blocks. This approach significantly improves the debugging experience compared to traditional callback methods. However, developers must be cautious about not mixing synchronous and asynchronous code without understanding the implications, which could lead to unexpected behaviors.
Python: The Asyncio Library
Python offers asynchronous programming through its library. This library allows for the creation of asynchronous tasks using coroutines. The primary advantage here is the integration of async functions, which allow for concurrent execution without threads, thereby minimizing overhead. The uniqueness of lies in its event loop, handling I/O-bound operations efficiently. Yet, developers must ensure proper understanding of the event-driven model to avoid pitfalls regarding blocking code.
and Asynchronous Programming
C# has embraced asynchronous programming with the introduction of the and keywords. These keywords simplify asynchronous coding and resemble JavaScript's approach. This similarity in syntax acknowledges the growing need for clarity in concurrent programming. The primary advantage of C#'s implementation is the seamless integration with the .NET framework, which provides robust tools for managing asynchronous tasks. That said, misunderstandings around task management can lead to issues, particularly in long-running applications.
Go Lang and Concurrency Support
Go Lang offers built-in support for concurrent programming through goroutines. These lightweight threads allow developers to launch concurrent functions easily. The important aspect of this method is its simplicity and efficiency in managing thousands of concurrent tasks without significant memory overhead. The Go runtime manages the scheduling of these goroutines, which results in a clean concurrency model. However, programmers must pay attention to synchronization issues that can arise, particularly when handling shared data.
Best Practices for Asynchronous Programming
Asynchronous programming introduces unique benefits but also comes with challenges. Implementing best practices can enhance reliability, maintainability, and performance of applications. Understanding and applying these practices is crucial for developers in building robust software solutions, especially in environments that demand high responsiveness.
Error Handling in Asynchronous Code
Error handling in asynchronous programming is particularly challenging due to the non-linear flow of execution. Traditional synchronous error handling using try-catch blocks is less effective. Developers must instead adopt specific strategies to ensure that errors are caught and handled properly.
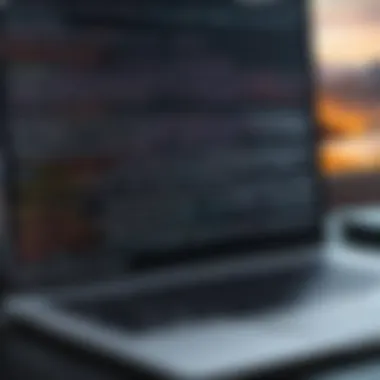
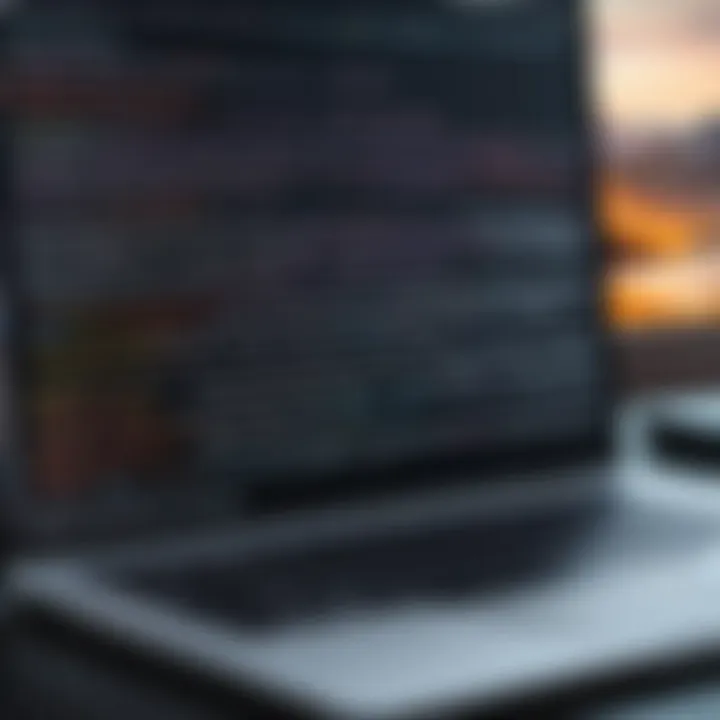
Consider using promises effectively. Each promise can be followed by a method, which is a good way to handle errors for specific operations.
Here’s an example in JavaScript:
Using async/await can further streamline error handling. With this syntax, the developer can use try-catch blocks as in synchronous code. This makes the implementation more readable:
It's essential to catch and log all errors at every level of your asynchronous chain, ensuring that no failures go unnoticed. Consistency in error handling leads to more resilient applications.
Managing State in Asynchronous Applications
Managing state in asynchronous applications can be complex. As operations become non-blocking, the state of an application might change unexpectedly, leading to inconsistencies. Proper state management techniques are vital to maintain harmony between asynchronous events and the overall application structure.
One common approach is to use state management libraries. In JavaScript, for example, Redux can be employed to manage state alongside asynchronous actions. This allows developers to separate application state from UI state, making the codebase easier to maintain.
Another technique is to leverage observable streams, such as RxJS. This can help manage state changes as events occur over time.
"Managing state effectively in asynchronous applications is key to providing a seamless user experience."
Remember to maintain a predictable state, making it easier to debug and test the application.
Performance Monitoring and Profiling
Performance monitoring and profiling are crucial components of developing asynchronous applications. Without proper monitoring, it can be difficult to pinpoint bottlenecks or performance issues that arise from non-blocking code execution. Identifying slow asynchronous processes can significantly improve user experience and application performance.
Utilize tools like Chrome DevTools or Node.js built-in monitoring to analyze your application's performance. They provide insights into how your asynchronous code executes. Pay attention to:
- The completion time of asynchronous operations
- The memory usage of your application
- Network requests and their response times
Incorporating performance profiling as part of your development cycle will promote a focus on efficiency. Continuous performance monitoring can help to identify lagging areas in code execution post-deployment as well.
In summary, mastering error handling, state management, and performance monitoring will greatly enhance your ability to develop effective asynchronous applications. These best practices are crucial for anyone aiming to excel in asynchronous programming.
Real-World Applications of Asynchronous Programming
Asynchronous programming has become a key element in the design and development of modern software applications. Its potential to handle multiple operations without blocking resources leads to enhanced performance and improved user experience. The practical implications of these benefits are evident across various domains, particularly in web and cloud computing.
Implementing asynchronous programming effectively allows systems to become more responsive. This is crucial in real-world applications where user interaction is expected at all times. Asynchronous patterns facilitate handling events or actions in a timely manner, which is an urgent requirement in today’s fast-paced technological landscape.
Web Development Frameworks and Async Benefits
In web development, frameworks such as Express.js, Django, and Flask leverage asynchronous programming to manage user requests and responses without significant lag. Users expect immediate feedback, especially in applications requiring real-time data interaction. By adopting asynchronous patterns, developers can achieve this responsiveness while managing I/O operations efficiently.
- Non-blocking I/O: Async programming allows applications to continue executing tasks while waiting for I/O operations to complete. This means that database queries, file reads, and API requests can be handled without hindering the main execution thread.
- Scalability: Asynchronous frameworks can manage more concurrent connections. This enhances the capability of applications to serve many users simultaneously without degrading performance.
- Reduced Latency: With async, the response time can be minimized, creating a smoother user experience. When a web application remains responsive, users are more likely to engage further.
Data Fetching and Processing
Data-intensive applications often face the challenge of processing large datasets. Asynchronous programming offers robust solutions for data fetching and processing operations. Traditional synchronous processing can lead to bottlenecks. On the other hand, async techniques allow for data to be fetched from multiple sources concurrently, significantly reducing overall processing time.
For instance, when collecting user data from disparate APIs, an application can launch several asynchronous calls simultaneously. This means the application spends less time waiting for responses.
- Example Implementation: In a JavaScript-based application, asynchronous functions can be written using the API to retrieve data from a remote server. Here is a basic example:
- Streamlined User Interfaces: Users can interact seamlessly with an application because backend data fetching does not block the frontend experience. As data loads gradually, applications can present loading indicators or update sections incrementally.
Microservices Architecture Implementation
Microservices represent a shift from monolithic architecture, where applications are built as a single unit, to a more modular approach. In microservices architecture, small, independent services communicate over a network, and asynchronous programming is vital for ensuring effective service interaction.
- Inter-service Communication: Asynchronous message queues (like RabbitMQ or Kafka) facilitate communication between microservices, enabling them to process requests without waiting for each other. This leads to a system that is not only quick but also resilient.
- Error Handling and Reliability: With the support of async patterns in microservices, error handling can be more effective. Services can retry failed operations or fallback to alternative systems without affecting users.
- Independent Deployment: Each service can be developed, deployed, and scaled independently, resulting in more agile development practices. Since functions can operate asynchronously, teams can focus on building and maintaining individual components without redundancy.

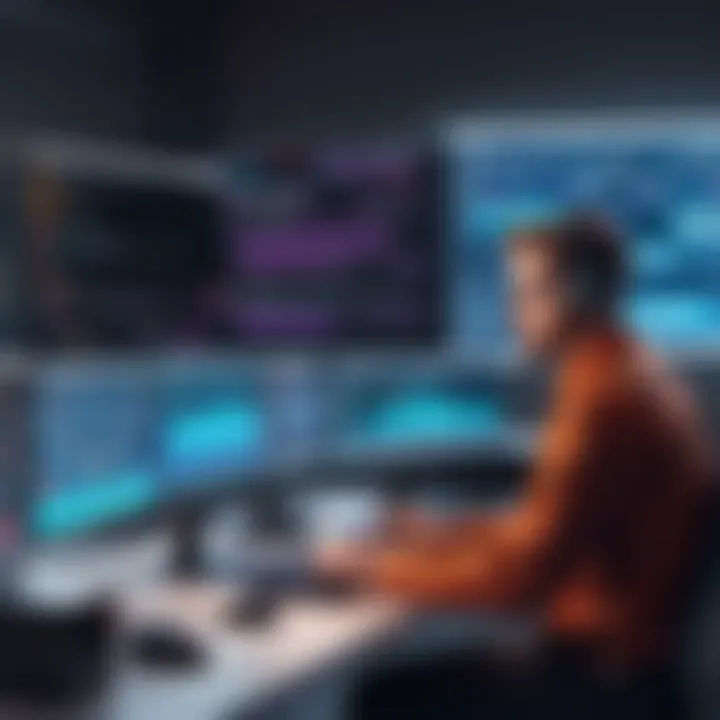
Challenges and Considerations
Asynchronous programming is not without its challenges. Understanding these challenges is vital for developers seeking to create efficient and robust applications. This section highlights critical elements within this domain, focusing on debugging and code organization.
Debugging Asynchronous Code
When working with asynchronous code, debugging can be notably more complicated than with synchronous operations. The non-linear nature of asynchronous tasks means that the flow of execution may not follow a straightforward path. Errors might occur far from their source. This can make it difficult to trace the origin of bugs. Common tools like breakpoints may not behave as expected. Consequently, developers should employ specialized debugging strategies.
Some effective techniques include:
- Use of modern debugging tools: Tools like Chrome DevTools can help in tracking asynchronous actions. They allow you to pause script execution and inspect the call stack.
- Implementing logs: Adding console logs at various execution points can shed light on the flow of operations and reveal where issues arise.
- Error handling mechanisms: Promises and async/await syntax provide built-in ways to manage errors. Ensure you are using on promises and blocks with async functions. This will help in catching errors where they happen, allowing for more straightforward solutions.
"Debugging is not just about finding errors. It is about understanding the flow of your program."
Avoiding Callback Hell
Callback hell refers to the situation in which callbacks are nested within each other, leading to poorly structured and difficult-to-read code. This often occurs when multiple asynchronous operations depend on one another. Each subsequent operation needs to wait for the previous one to complete, leading to deep nesting.
To mitigate this issue, consider these approaches:
- Flat structure: Always keep your code as flat as possible. This can be helped by breaking code into small functions that handle specific tasks. By doing so, you limit the depth of chaining.
- Use of Promises: Promises simplify asynchronous code writing. They enable you to handle asynchronous operations in a more linear fashion and reduce embedding callback functions.
- Async/Await Syntax: This modern syntax allows developers to write asynchronous code that reads almost like synchronous code. It also makes error handling considerably easier. When using async/await, you can organize your code logically without sacrificing readability.
Following these practices can lead to cleaner, more maintainable code in asynchronous programming.
Future Trends in Asynchronous Programming
Asynchronous programming is an essential aspect of modern software development, especially as applications become more complex and user expectations heighten. Understanding the future trends in this field is crucial for developers, IT professionals, and tech enthusiasts striving to remain competitive. This section discusses emerging technologies, the growing impact of distributed systems, and what these trends mean for future software projects.
Emerging Technologies
The rise of new technological paradigms and frameworks is reshaping how asynchronous programming is approached. One of the key emerging technologies is serverless computing. In this model, developers can deploy code without worrying about the underlying infrastructure. Serverless architectures often rely on async programming to manage event-driven processes efficiently, allowing developers to focus on application logic rather than server management.
Furthermore, microservices architecture has gained popularity. This architecture enables applications to be broken down into smaller, manageable services that communicate over a network. Each service can operate asynchronously, improving resource utilization and scalability. Developers utilizing microservices must understand the nuances of async programming to avoid pitfalls related to inter-service communication.
Other notable advancements include WebAssembly. This technology allows code to run in the browser with near-native performance, facilitating asynchronous operations in web development. Utilizing WebAssembly can lead to faster load times and a smoother user experience.
"The efficient use of resources in software development lies in the power of asynchronous programming and emerging technologies."
Impact of Distributed Systems
The evolution of distributed systems has profound implications for asynchronous programming. In a distributed environment, multiple systems interact, and the ability to perform tasks asynchronously becomes vital. This is because traditional synchronous methods can lead to significant latency, disrupting user experience. As these systems span across geographies, network issues and varying latencies make it essential to adopt asynchronous practices.
In distributed systems, event-driven programming models are increasingly relevant. Events can be handled independently from the main program execution flow, allowing for more responsive applications that can scale across numerous nodes or services. Technologies like Apache Kafka enable reliable event streaming, promoting decoupling between producers and consumers, which is key for building robust applications.
Additionally, distributed databases and storage solutions rely heavily on asynchronous programming to manage data integrity and availability without sacrificing performance. As data is stored in multiple locations, asynchronous methods ensure that operations remain responsive to users, even amidst potential network disruptions.
In summary, the future of asynchronous programming is intertwined with technological advancements and the evolution of distributed systems. Understanding these trends will empower software developers and IT professionals to design more efficient, scalable, and resilient applications in an increasingly complex digital landscape.
Closure
In the realm of software development, asynchronous programming stands as a critical pillar that influences both the performance and user experience of applications. This conclusion draws together the essential elements discussed throughout the article, outlining how asynchronous programming reshapes modern technology landscapes.
Asynchronous programming facilitates non-blocking operations, enabling systems to execute tasks concurrently. This feature is indispensable for applications that require real-time data processing and responsiveness. For example, during intensive computations or data retrieval, traditional synchronous programming can lead to bottlenecks, resulting in poor application performance. By embracing asynchronous methodologies, developers can ensure smoother user interactions and more efficient resource utilization.
Reflecting on the key points, it is clear that mastering asynchronous programming is not merely beneficial; it is essential for any software professional aiming to stay relevant in today’s competitive environment. Proper understanding of concepts such as event loops, callbacks, and async/await syntax fosters enhanced application design and promotes coding practices that reduce errors and debugging complexities.
In summary, asynchronous programming is a powerful tool that, when applied correctly, can significantly enhance both the functionality and the user satisfaction of applications.
Continuing to innovate in this area allows developers to not only meet current demands but anticipate future requirements. As systems evolve and the need for rapid, scalable solutions becomes more pronounced, asynchronous programming will undeniably remain an essential component of effective software development.
Summary of Key Takeaways
- Asynchronous programming enables concurrent task execution, preventing application blocking.
- It enhances performance, improving user experiences through responsive interactions.
- Mastery of concepts like event loops and async/await is crucial for modern software development practices.
- Proper implementation reduces complexities in debugging, leading to cleaner, maintainable code.
- Continuous innovation in asynchronous methodologies can promote adaptability in evolving tech landscapes.
The Role of Asynchronous Programming in Future Development
As we look ahead, asynchronous programming will continue to gain importance in various fields of software development. Emerging technologies, especially in cloud computing and microservices, rely heavily on asynchronous paradigms to handle vast amounts of data efficiently and effectively.
The transition towards distributed architectures demands that applications maintain robust performance levels under variable loads. Asynchronous programming allows for seamless integrations and data exchanges, which are increasingly vital for systems that communicate in real time.
Additionally, as machine learning and artificial intelligence applications grow, the need for efficient asynchronous processing becomes even more pronounced. These applications often need to handle multiple tasks simultaneously, demanding effective asynchronous coding practices.
To conclude, the role of asynchronous programming in future development signifies not just a trend, but a foundational shift in how applications are built for reliability and performance. Embracing these techniques will be crucial for any developer wishing to thrive in a landscape where efficiency and speed are paramount.