Unraveling the Intricacies of the Singleton Design Pattern in Software Development
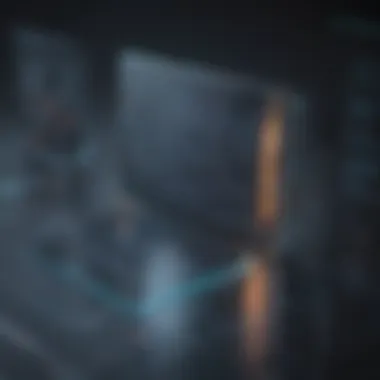
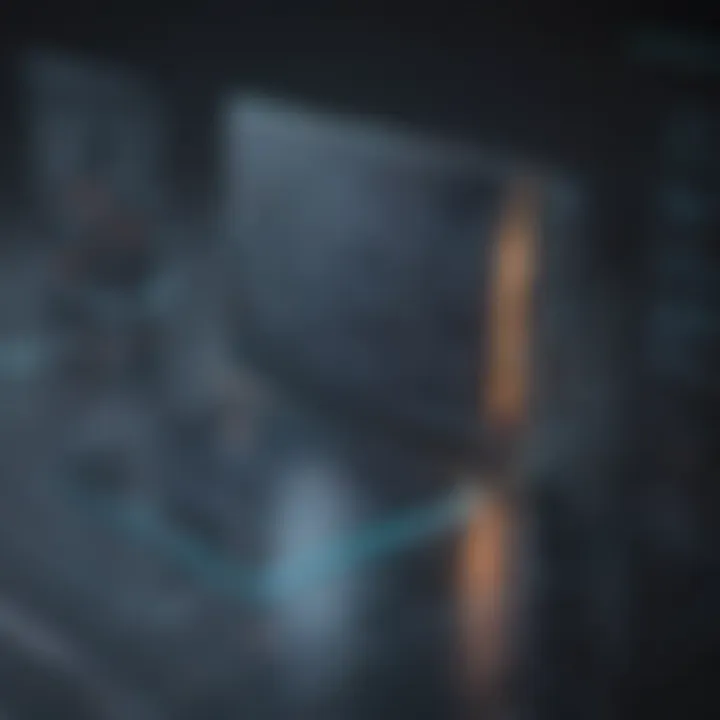
Overview of the Singleton Design Pattern in Software Development
In the realm of software development, the Singleton Design Pattern stands as a pillar of efficiency and reusability. This architectural approach involves restricting the instantiation of a class to ensure that it has only one instance, providing a globally accessible point of access to that instance. The Singleton pattern plays a crucial role in scenarios where a single object is essential for tasks such as managing configurations, logging, thread pools, caches, and more. By delving deeper into the Singleton Design Pattern, software developers can elevate their understanding of structuring and optimizing code.
Definition and Importance of the Singleton Design Pattern
The Singleton Design Pattern, categorized under the creational design patterns, serves to control the instantiation of a class by allowing only one instance to exist throughout the application. This pattern not only centralizes the management of a single object but also enhances performance by avoiding multiple instantiations. Its significance lies in streamlining object creation, promoting memory efficiency, and facilitating a global point of access to the instance, fostering simplicity and maintainability within the codebase.
Key Features and Functionalities
At the core of the Singleton Design Pattern is the static instance creation within the class itself, ensuring that only one instance is generated. Additionally, the pattern involves a private constructor to prevent external instantiation and a static method for accessing the instance globally. By employing such features, developers can enforce object uniqueness, promote resource efficiency, and enable seamless access to the Singleton instance across different parts of the application.
Use Cases and Benefits
The Singleton pattern finds application in various scenarios across software development, such as database connections, logging mechanisms, thread pools, configuration settings, and caching systems. By implementing the Singleton Design Pattern, developers can optimize resource utilization, simplify access to shared resources, enhance performance by reducing redundant object creation, and improve the overall structure and organization of their codebase.
Introduction
Software development encompasses various design patterns to facilitate efficient and scalable coding practices. One such fundamental pattern is the Singleton Design Pattern. Understanding this pattern is essential for developers aiming to optimize their codebase. Through this article, we will delve into the intricacies of the Singleton Design Pattern, exploring its definition, purpose, benefits, key concepts, and its significance in the realm of software development.
Definition of Singleton Design Pattern
The Singleton Design Pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance. This pattern restricts the instantiation of a class to a single object, which proves beneficial in scenarios where only one instance of a class is required throughout the system's lifecycle.
Purpose and Benefits
The primary purpose of the Singleton Design Pattern is to control object creation by restricting the instantiation of a class to a single instance. By doing so, it allows global access to this instance, making it convenient to access the object without the need to create multiple instances. The Singleton Pattern enhances code reusability, memory management, and performance optimization by providing a single, shared instance across the application.
Significance in Software Development
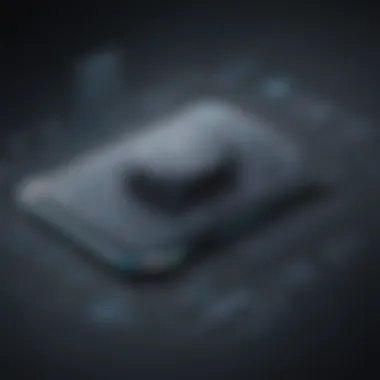
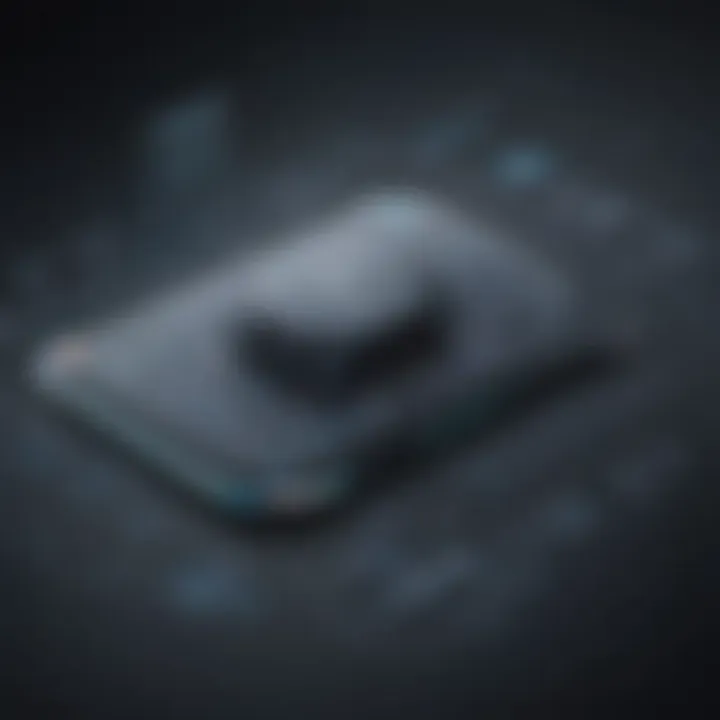
The Singleton Design Pattern plays a crucial role in software development by promoting the efficient utilization of resources and maintaining global state consistency within an application. It offers a structured approach to managing global resources and configuration settings, making it easier to control object creation and access. Understanding and implementing Singletons ensure a streamlined development process and foster code scalability and maintainability.
Key Concepts
Several key concepts underpin the Singleton Design Pattern's implementation. These include ensuring a private constructor to prevent external instantiation, utilizing a static instance variable to hold the single instance, and providing a static method for accessing this instance. By grasping these concepts, developers can effectively implement the Singleton Pattern while addressing thread safety concerns and optimizing object creation and management within their software projects.
Implementation Guidelines
In the realm of software development, the Implementation Guidelines surrounding the Singleton Design Pattern hold paramount importance. These guidelines serve as the blueprint for meticulously crafting a Singleton Class, ensuring that it functions optimally within the software architecture. By adhering to these guidelines, developers can eliminate the possibility of multiple instances, leading to more efficient memory usage and streamlined code organization. The intricacies of implementing a Singleton Class delve into various aspects, from designing a private constructor to considering thread safety measures.
Creating a Singleton Class
Private Constructor
The Private Constructor plays a pivotal role in the Singleton Design Pattern by restricting the instantiation of a class from external sources. It serves as a gatekeeper, allowing only the class itself to create an instance. This exclusivity ensures that the Singleton Class maintains a single instance throughout the software's lifecycle, preventing any unauthorized replication. The Private Constructor's inherent characteristic of privacy enhances the pattern's control over instance creation, contributing significantly to the pattern's overall effectiveness.
Static Instance Variable
A Static Instance Variable within a Singleton Class acts as the storage container for the single instance of the class. By declaring this variable as static, developers guarantee that it is accessible at the class level rather than at the instance level. This distinction is crucial in Singleton implementation, as it enables all calls to refer to the same instance, promoting consistency and coherence in the codebase. The Static Instance Variable's static nature encapsulates the essence of singularity that defines the Singleton pattern, underlining its importance in maintaining the pattern's integrity.
Static Method for Instance Access
The incorporation of a Static Method for Instance Access provides a centralized mechanism for retrieving the single instance held by the Singleton Class. This method acts as the entry point for acquiring the instance, offering a standardized approach that obviates the need for direct instantiation. By abstracting the process of instance retrieval behind a static method, developers instill a layer of indirection that enhances flexibility and extensibility. The Static Method for Instance Access represents a key component in interfacing with the Singleton Class, facilitating seamless interaction and enhancing the pattern's usability.
Thread Safety Considerations
In the dynamic landscape of software development, Thread Safety Considerations bear immense significance when implementing the Singleton Design Pattern. With the potential for concurrent access in multithreaded environments, ensuring thread safety is imperative to prevent race conditions and data corruption. Various strategies such as Lazy Initialization, Double-Checked Locking, and Enum Implementation offer solutions to mitigate thread safety risks, safeguarding the Singleton Class's integrity.
Lazy Initialization
Lazy Initialization postpones the instantiation of the Singleton Class until the moment it is accessed for the first time. By deferring creation until necessary, developers optimize resource utilization and promote efficiency. The Lazy Initialization approach minimizes overhead by initializing the instance only upon demand, striking a balance between resource conservation and responsiveness.
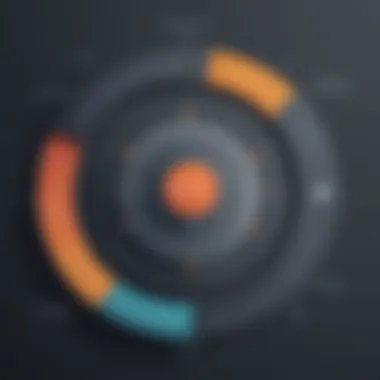
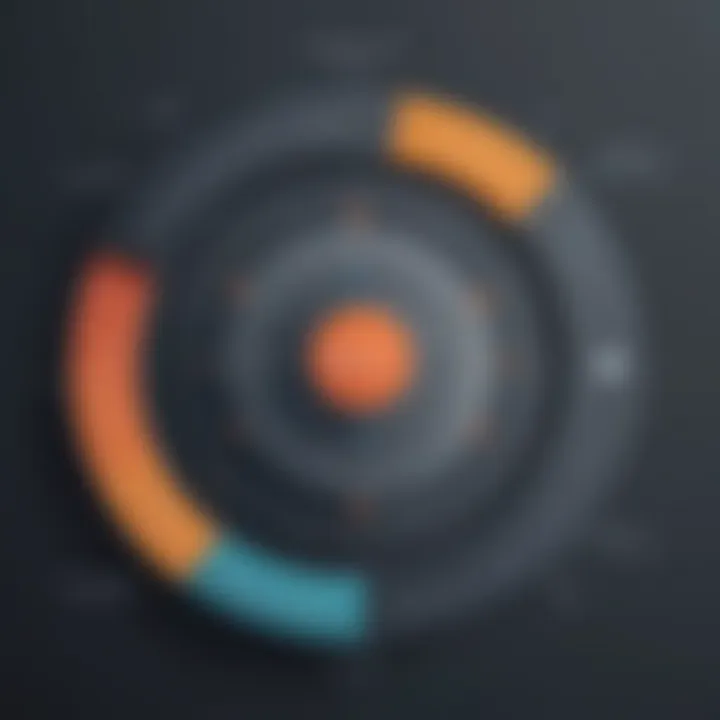
Double-Checked Locking
Double-Checked Locking presents a concurrency control mechanism aimed at improving performance in multithreaded scenarios. This method involves checking a locking condition before entering a critical section to minimize synchronization overhead. By confirming the lock status both outside and inside the critical section, developers enhance efficiency while maintaining thread safety. Double-Checked Locking stands as a sophisticated strategy for enhancing the Singleton Design Pattern's concurrency handling.
Enum Implementation
The Enum Implementation of the Singleton Design Pattern leverages Java enums to achieve singleton behavior. Enums inherently embody singularity by restricting their instances to a predefined set of constants, aligning seamlessly with the Singleton concept. This method ensures implicit thread safety, serialization, and robust implementation while succinctly expressing the Singleton pattern. Enum Implementation simplifies Singleton creation and effectively addresses thread safety concerns, making it a compelling choice for modern software development.
Serialization and Deserialization
Serialization and Deserialization present essential considerations when extending Singleton Classes to persist state or facilitate interprocess communication. Serialization enables converting data objects into a format for storage or transmission, ensuring that the Singleton instance remains intact during such operations. Deserialization, on the other hand, reconstructs serialized objects back into their original form, maintaining data integrity and instance coherence. By addressing serialization and deserialization challenges, developers can harness the full potential of Singleton pattern instances across diverse software environments.
Best Practices
In the realm of software development, adhering to best practices is the cornerstone of crafting robust and maintainable code. Specifically, when delving into the intricacies of the Singleton design pattern, employing best practices becomes paramount. By following established guidelines and principles, developers can streamline development processes, enhance code quality, and promote consistency across projects. Embracing best practices ensures that the singleton instances are managed efficiently, preventing potential pitfalls that may arise from deviating from established norms. Through meticulous attention to detail and adherence to proven methodologies, software professionals can optimize the utilization of singletons in their applications, thereby fostering scalability and resilience.
Avoiding Global State Abuse
One of the fundamental aspects of implementing the Singleton design pattern is avoiding global state abuse, which can lead to unintended consequences and make code maintenance challenging. Global state, when not managed appropriately, may introduce dependencies and obscure the flow of data within an application. By constraining the scope of singleton instances to their intended usage context, developers can mitigate the risks associated with global state abuse. Through encapsulation and judicious design choices, such as limiting access to singleton instances and minimizing cross-module dependencies, the potential for architectural conflicts and code entanglement is minimized. Upholding principles of encapsulation and information hiding is crucial in preventing global state abuse while harnessing the benefits of the Singleton pattern.
Maintaining Readability and Maintainability
Ensuring readability and maintainability in software projects involving the Singleton design pattern is indispensable for fostering collaboration and facilitating code evolution. By adopting a structured and coherent coding style, developers can enhance the comprehensibility of singleton implementations and ease the debugging and modification processes. Clear and concise documentation, coupled with adherence to naming conventions and design patterns, contributes to the overall readability of the codebase. Moreover, prioritizing modularity and separation of concerns fosters maintainability by facilitating isolated modifications without affecting the entire system. By advocating for code simplicity, clarity, and coherence, software professionals can uphold standards of excellence and facilitate seamless collaboration among team members.
Testing Strategies
Effective testing strategies play a pivotal role in validating the functionality and reliability of software systems employing the Singleton design pattern. By devising comprehensive test suites that cover various scenarios and edge cases, developers can ensure that singleton instances operate as intended across different usage scenarios. Leveraging unit tests, integration tests, and system tests enables the identification of potential issues early in the development lifecycle, thereby streamlining the debugging and optimization processes. Implementing test-driven development methodologies further enhances the robustness of singleton implementations by iteratively refining code segments based on test outcomes. Embracing a culture of continuous testing and quality assurance aids in the early detection of anomalies and instills confidence in the resilience of software systems leveraging the Singleton pattern.
Common Pitfalls
Understanding and addressing common pitfalls is crucial when working with the Singleton design pattern. By recognizing and mitigating these issues in advance, you can prevent potential problems and ensure the effectiveness of your software development process. In this section, we will explore some of the most prevalent pitfalls associated with implementing the Singleton pattern, shedding light on ways to navigate these challenges successfully.
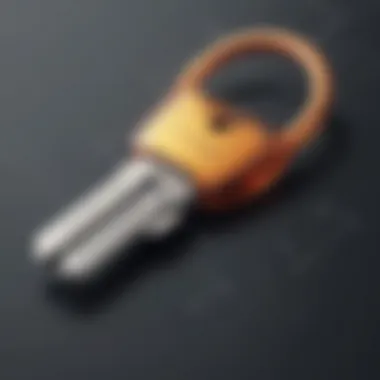
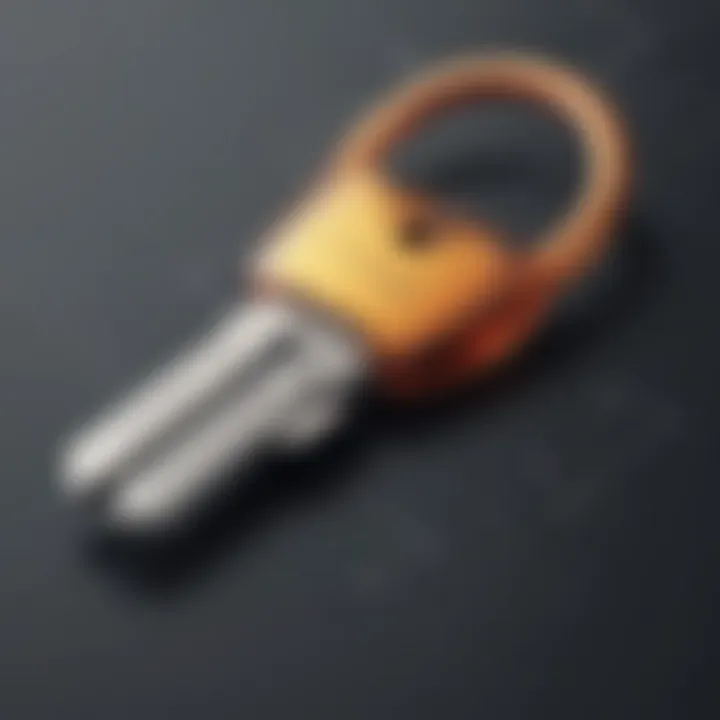
Eager Initialization
Eager initialization is a common pitfall that developers may encounter when implementing the Singleton pattern. This occurs when the single instance of a class is created eagerly, regardless of whether it is needed at that moment or not. While eager initialization may seem efficient initially, it can lead to performance issues and unnecessary resource consumption. By creating the instance preemptively, you might inadvertently increase memory usage and slow down the application's startup time. Therefore, it is essential to carefully consider the necessity of creating the Singleton instance eagerly and opt for lazy initialization when appropriate to avoid these pitfalls.
Reflection Vulnerabilities
Reflection vulnerabilities pose a significant risk when working with the Singleton pattern. Reflection in Java, for instance, allows developers to access private fields and methods of a class, bypassing encapsulation and potentially compromising the Singleton instance's integrity. Attackers can exploit reflection to modify the Singleton instance, leading to unexpected behavior and security breaches. To prevent reflection vulnerabilities, it is advisable to restrict access to critical methods and fields, ensuring that the Singleton instance remains secure and immutable.
Testing Challenges
Testing Singleton classes presents unique challenges due to their inherent design characteristics. Since Singletons maintain a single instance throughout the application's lifecycle, testing interactions with this instance can be complex. Mocking Singletons for unit testing purposes can be cumbersome, especially when Singleton classes are tightly coupled with other components. Additionally, testing multithreaded scenarios and concurrent access to the Singleton instance requires careful consideration to identify and address potential race conditions effectively. To overcome these testing challenges, developers can leverage dependency injection frameworks, use interfaces for Singleton classes, and adopt strategies like dependency inversion for seamless testing without compromising the Singleton pattern's integrity.
Real-World Applications
In the realm of software development, understanding the real-world applications of the Singleton design pattern is crucial for efficient coding practices. This pattern finds its utility in scenarios where there must be precisely one instance of a class present in a system at any given time. By restricting the instantiation of a class to a single object, Singletons offer a tailored solution to several common design challenges. Harnessing this pattern guarantees that resources are utilized optimally and dependencies are managed effectively within the software architecture, contributing towards streamlined and robust codebases. Without a doubt, the real-world applications of Singletons showcase the versatility and reliability they bring to software projects.
Use Cases in Software Frameworks
The use cases of Singleton designing pattern in software frameworks are diverse and multifaceted. Many established frameworks across various programming languages leverage Singletons to manage resources efficiently. For instance, in web development, frameworks like AngularJS employ this pattern to ensure that only one instance of a component is active at a time, promoting data consistency and enhancing performance. Similarly, in mobile application development using frameworks like React Native, Singletons aid in centralizing state management, leading to more maintainable and scalable codebases. By emphasizing the judicious application of Singletons within software frameworks, developers can harness the benefits of this pattern to optimize their coding practices and elevate the overall quality of the software they build.
Integration with Dependency Injection
The integration of Singletons with dependency injection frameworks amplifies the flexibility and extensibility of software applications. Dependency injection plays a crucial role in decoupling components and enhancing testability, and when combined with Singletons, it empowers developers to efficiently manage dependencies while ensuring that only one instance of a class is utilized throughout the application lifecycle. By seamlessly integrating Singletons with dependency injection mechanisms, developers can simplify the instantiation of objects, promote code reusability, and enhance the maintainability of their codebase. This integration stands as a testament to the adaptability and synergies that Singletons offer in modern software development practices.
Singleton vs. Static Class Usage
The comparison between Singleton and static class usage revolves around the core principle of managing object instances and class behavior within a software system. While static classes offer convenient accessibility and maintain a global state, Singletons provide a more structured approach by enforcing a single point of access for class instances. Understanding the nuances between Singleton and static class usage is essential for choosing the appropriate design pattern based on the specific requirements of a project. Singletons excel in scenarios where a single instance of a class is imperative, whereas static classes thrive in situations that demand shared behavior across multiple instances. By carefully deliberating the implications of Singleton versus static class implementation, developers can make informed decisions that align with the design goals and objectives of their software projects.
Conclusion
Summary of Key Points
In summary, the Singleton design pattern in software development plays a vital role in ensuring that a class has only one instance and provides a global point of access to that instance. Key points covered include the definition of singletons, their purpose, benefits, significance in software development, key implementation concepts such as creating a singleton class, thread safety considerations, and serializationdeserialization. Additionally, best practices to avoid global state abuse, maintain readability and maintainability, and implement effective testing strategies were discussed. Common pitfalls like eager initialization, reflection vulnerabilities, and testing challenges were highlighted, along with real-world applications, such as use cases in software frameworks, integration with dependency injection, and comparisons with static class usage. By understanding these key points, developers can leverage the Singleton design pattern efficiently in their projects to enhance code quality and ensure robust software architecture.
Future Trends in Singleton Usage
Looking ahead, the future trends in singleton usage are likely to focus on enhancing thread safety mechanisms, promoting more efficient serialization and deserialization techniques, and integrating singletons with emerging technologies like microservices and cloud computing. As software development practices evolve, there is a growing emphasis on scalability, distributed systems, and containerization, which will influence how singletons are implemented and utilized in modern applications. Furthermore, advancements in programming languages and frameworks may introduce new patterns or methodologies that impact the traditional usage of singletons. It is essential for developers to stay abreast of these evolving trends to adapt their singleton implementation strategies accordingly and ensure their codebases remain optimized for performance and flexibility.