Mastering SendGridMessage in C#: A Complete Guide
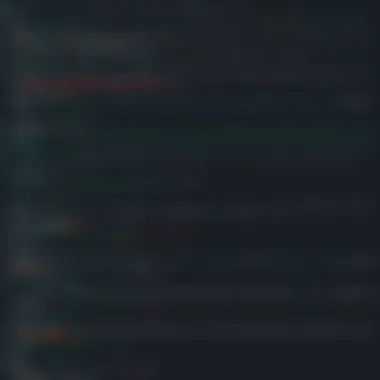
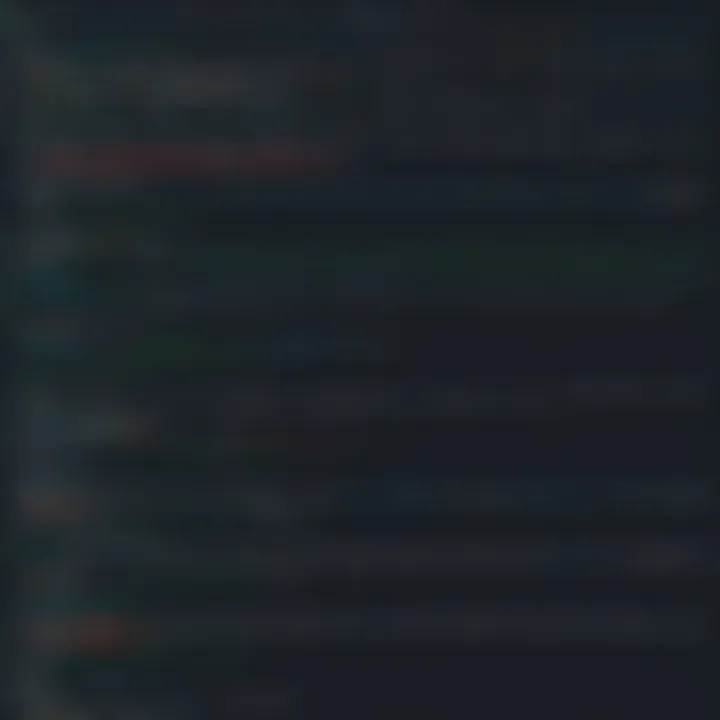
Intro
In today’s digital landscape, email remains a crucial communication tool. This article delves into SendGridMessage in C#. As online communication multiplies, developers face the challenge of efficient, reliable email delivery. Utilizing SendGrid simplifies this task. It streamlines the process of sending unsolicited or common transactional emails through a robust API.
By understanding how to effectively implement SendGridMessage, both beginners and seasoned professionals can enhance their software applications. This article aims to provide valuable insights into setting up SendGrid, configuring the SendGridMessage class, and adhering to best practices.
Overview of Software Developmet
Software development is multifaceted. It considers various components such as applications, interface creation, and backend systems. In this realm, cloud computing also plays an essential role. Cloud computing permits developers to deploy applications without complicated infrastructure concerns. This accelerates productivity and flexibility in email handling through platforms like SendGrid.
The integration of SendGridMessage in C# aligns with this efficiency. The significance of utilizing cloud-based technologies becomes apparent as organizations increasingly opt for scalable solutions. Incorporating SendGrids capabilities into an application unlock significant benefits in email communications.
Best Practices
Implementing SendGridMessage is facilitated by understanding effective strategies. Here are essential practices:
- Authenticate Your Domain: Verify your domain to enhance deliverability. This act can significantly boost trustworthiness with recipients.
- Utilize Dynamic Templates: This tool allows for personalization in communication. Leveraging templates can save development time while improving user engagement.
- Implement Tracking and Analytics: Monitor metrics with SendGrid’s dashboard. Evaluating user interaction is crucial for refined communication strategies.
Common pitfalls may include neglecting proper error management or bypassing encryption protocols. Always ensure secure handling of sensitive data in line with industry standards.
Case Studies
Several companies showcase successful SendGridMessage implementations:
- Zoom used SendGrid for its email campaigns, resulting in effective scaling and communication.
- Airbnb leveraged SendGrid messages to notify hosts and travelers about updates, achieving higher user satisfaction.
These examples highlight that with meticulous implementation, significant outcomes are possible.
Latest Trends and Updates
The email communication landscape is evolving. Recent trends focus on artificial intelligence and automation within email marketing. SendGrid and similar services explore new AI-driven capabilities to enhance user engagement and personalize content delivery.
How-To Guides and Tutorials
Beginners must engage in thorough educational resources. Here is a practical basic guide:
- **Setupstrong: Register for a SendGrid account and obtain API keys.
- NuGet Installation: Install the SendGrid NuGet package in your C# project using:
- Configure Message: Create your SendGridMessage and set the TO, FROM, Subject, and Body.
- Sending the Email: Utilize the SendGrid service for dispatching the message.
This structured step-by-step guide helps transform novices into intermediate users proficient in SendGrindMessage implementations.
For further information, SendGrid's documentation is a valuable resource: SendGrid
Refer to Wikipedia for a broader context on cloud computing tools in software development.
Preamble to SendGrid
Email communication has become a backbone for businesses and organizations striving to connect with clients and stakeholders efficiently. Understanding the tools that can enhance this communication is vital. This section introduces SendGrid, a powerful service in the email landscape. The integration of SendGrid into programming allows developers to streamline email functionalities in their applications.
Overview of Email Services
Email services are essential for all sorts of online interactions. These services enable users to send, receive, and manage email messages. Different services provide varying features, like email marketing or transactional email features. An efficient email service provides reliability, speed, and scalability, elements crucial for businesses that depend on effective communication.
What is SendGrid?
SendGrid is a cloud-based service that specializes in email delivery. Initially gaining attention for its email marketing features, it now focuses on transactional and bulk emails. Users can send emails programmatically using SendGrid’s API, integrating smoothly into applications developed across platforms like C#. With its dynamic templates, webhook features, and tracking options, SendGrid extends functionality beyond basic emailing.
Benefits of Using SendGrid
Deploying SendGrid presents numerous advantages:
- Scalability: SendGrid is designed to accommodate varying volumes of emails without a hitch. Whether sending a few emails or scaling up to thousands, its infrastructure handles load effectively.
- Delivery Optimization: The service monitors sending reputation and optimizes delivery rates, ensuring that emails land in recipient inboxes rather than being marked as spam.
- Real-time Analytics: SendGrid provides insightful metrics on sending performance. This data includes open rates, bounce rates, and link clicks, feeding into business decisions related to email strategies.
- Quick Integration: The flexible API allows developers to quickly connect to applications, streamlining the process to integrate email services with existing software.
In summary, knowing SendGrid and its advantages is fundamental as we explore its mechanisms in future sections, particularly for software developers, IT professionals, and others focused on data-driven solutions.
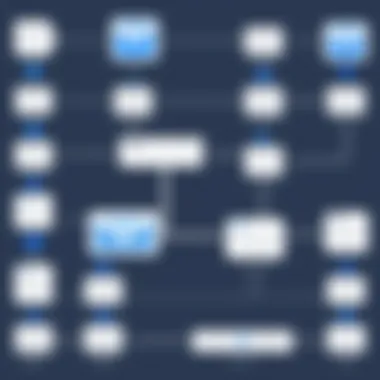
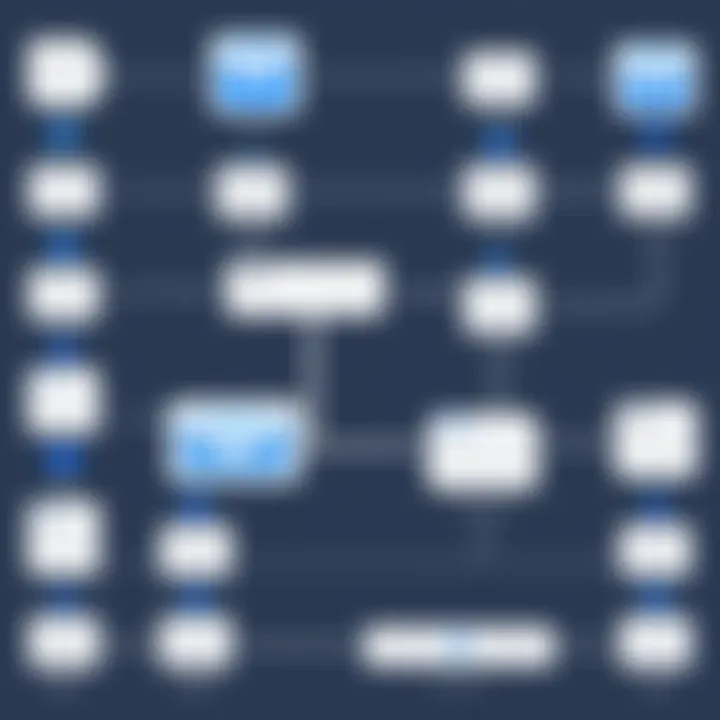
Prerequisites for Using SendGridMessage
Before diving into the implementation of the SendGridMessage class in C#, it's essential to establish a solid groundwork. This section outlines the necessary steps and considerations that will facilitate a smoother experience while using SendGrid's email capabilities. Understanding these prerequisites can significantly enhance the efficiency and efficacy of your email setup.
Creating a SendGrid Account
The first step is to create a SendGrid account. This process is simple and straightforward. Go to the SendGrid website and sign up. Depending on your requirements, you can choose between different service plans—free or paid options are available. Creating an account grants you access to a variety of features, including the SendGrid API.
Once your account is set up, it is important to verify your email and complete any necessary setup procedures as prompted by SendGrid. By doing so, you ensure that your account can send and manage emails without any issues. Seting your priorities at the account level will lay a solid foundation for further integration.
Obtaining API Key
After creating your account, obtaining the API key is crucial. The API key acts as a unique identifier that authenticates your C# application with the SendGrid services. To generate your API key, navigate to the API Keys section in your SendGrid dashboard. Here, you can easily create a new key. It is advisable to use a descriptive name for your key, as well as limit its permissions based on your usage requirements.
Your API key should be protected at all costs. It is best practice never to expose it in public repositories.
Document the generated key and store it securely. You’ll need this key to integrate SendGridMessage within your code. Remember that you can regenerate your key at any time if necessary, but doing so might affect existing applications that rely on it.
Setting Up a
Development Environment
Setting up a compatible C# development environment is the next significant step. If you have not already done so, install a suitable Integrated Development Environment (IDE) like Microsoft Visual Studio or Visual Studio Code. These tools provide the functionality needed to write, compile, and run your C code efficiently. Adjusting your IDE settings might also be beneficial for the tasks you are about to undertake.
After installing your IDE, ensure that you have the .NET framework compatible with the libraries and functionalities you plan to use. Most commonly, developers opt for .NET Core or .NET 5/6 as they are trendier and embrace cross-platform capabilities. Familiarizing with essential libraries that support SendGrid integration allows easier access and minimizes tackle while coding.
Finally, it's imperative to integrate the SendGrid library into your C# project. This can typically be done using a package manager like NuGet. Here’s a simple command you might run in the Package Manager Console:
In summary, having a well-configured SendGrid account, obtaining your API key, and preparing your C# development environment lay the groundwork for implementing the SendGridMessage class effectively.
Understanding SendGridMessage Class
The SendGridMessage class is celebrated for its efficiency and flexibility within the context of the SendGrid email service. As a software developer or IT professional, understanding this class is critical because it embodies the core functionality required to send emails through the SendGrid platform. Failure to grasp its structure and features can complicate email implementation within applications, leading to wastage of time and resources. This class streamlines common email-related tasks, making it an essential component of your email development toolkit.
Structure of SendGridMessage
The SendGridMessage is designed with several components that together allow users to create, format, and send emails. Here are the fundamental parts:
- Header Information: Identify the sender, recipient, subject line, and any custom headers needed for advanced features like tracking.
- Email Body: Contains both HTML content and plain text alternate versions to ensure cross-email-client compatibility.
- Attachments: Allows files to be appended for transmission along with the email.
The class simplifies access and modification to these components, presenting a user-friendly interface for developers. For instance, when creating a new instance of SendGridMessage, you generally perform operations in the following manner:
Understanding its structure allows programmers to manage emails more effectively by utilizing its comprehensive feature set without being overwhelmed by extraneous functionality.
Key Properties and Methods
The SendGridMessage class is equipped with several vitial properties and methods that elevate its utility:
Properties
- Subject: Retrieves or sets the subject line of the email.
- HtmlContent: Holds the main body content in an HTML format.
- PlainTextContent: Provides the plain text version of the email, ensuring all recipients can view the message.
- From: Determines the email sender's attributes, including address and display name.
Methods
- AddTo(EmailAddress email): Sends an email to the specified recipient.
- AddCc(EmailAddress email): Adds a recipient as a carbon copy (CC) recipient.
- AddBcc(EmailAddress email): Includes a blind carbon copy (BCC) recipient, maintaining their confidentiality.
- AddAttachment(File file): Attaches a file to the email, which is essential for sharing documents and images.
These properties and methods enable a thorough customization of email elements, allowing developers to build tailored messages. Understanding these aspects ensures you can properly leverage the class when developing applications that include email functionalities.
To maximize effectiveness and deliverability, make full use of these properties and methods based on the application requirements.
Implementing SendGridMessage in
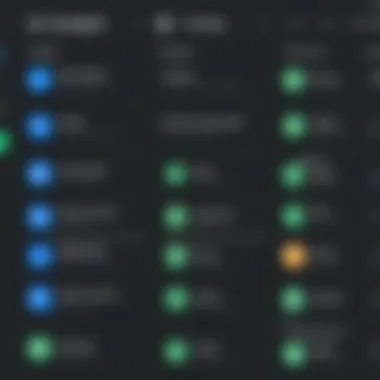
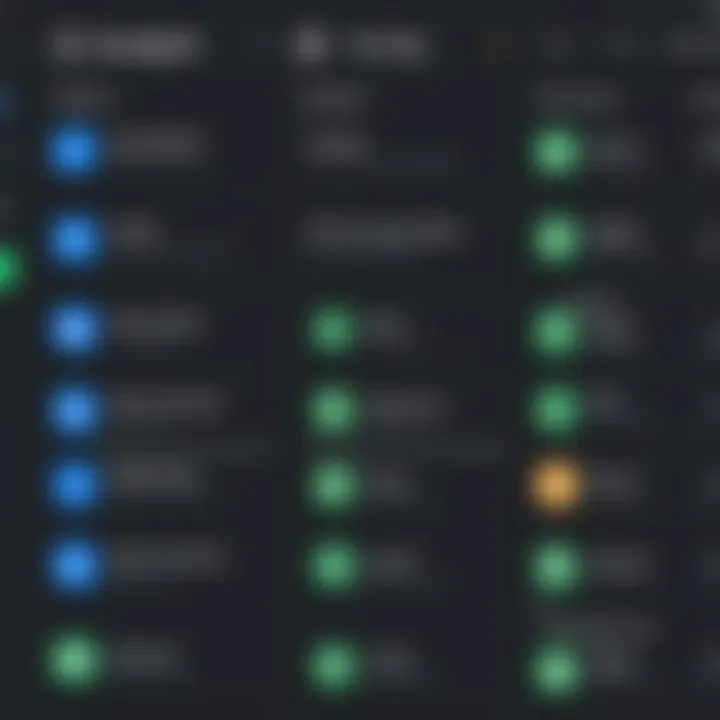
Implementing SendGridMessage in C# is a critical step for effective email communication within applications. The SendGrid API simplifies the process of sending transactional and marketing emails, enabling developers to focus on functionality rather than the intricacies of email protocols. As a widely adopted email delivery solution, SendGrid ensures reliable delivery, which is pivotal in maintaining engagement with users through consistent communication.
Integrating SendGridMessage allows developers to harness a variety of features such as real-time analytics, templates, and ease in managing contacts. The relevance of this topic goes beyond basic email dispatching, focusing on modern software development needs. Utilizing tools like SendGrid not only streamlines workflows but also enhances the overall application experience. Moreover, understanding the implementation details also paves the way for better email marketing strategies, which increase user retention and satisfaction.
Creating a Simple Email
Creating a simple email using SendGridMessage involves defining essential elements such as the sender's information, subject, and content. The first step in crafting this email is to instantiate the SendGridMessage class and populate it with necessary values.
Here’s an example of how to build a basic email:
In this snippet, the email is sent from a specified address and includes a simple HTML content. Building robust emails is crucial for establishing effective communication, and SendGridMessage simplifies this by offering a flexible structure.
Adding Recipients and /BCC
Adding recipients in the SendGridMessage class is straightforward. Inclusion of main recipients can be done with minimal effort:
Utilizing CC (carbon copy) and BCC (blind carbon copy) provides structure to email distribution while maintaining privacy among recipients. This is important when sending emails to multiple users who may not know each other. Having clear visibility into email delivery can ensure tailored experiences for different groups.
Including Attachments
Including attachments with SendGridMessage enhances the utility of messages. It allows developers to send documents or media files seamlessly. The process is simple, requiring just a few lines of code. For example:
This code snippet demonstrates how to attach files to an email. Ensuring that important documents reach recipients enhances communication reliability. Presence of attachments can facilitate discussions and decision-making in both formal and informal exchanges. Each of these implementation aspects contributes to a fuller utilization of the SendGridMessage class, assuring that emails sent fulfill their intended purpose effectively.
Best Practices for Sending Emails
To establish an effective communication strategy through email, integrating best practices is essential. The significance of adhering to best practices in email sending can not be overstated, especially when using a service like SendGrid. Good practices enhance user engagement, increase deliverability rates, bolster brand reputation, and build trust with your audience. The right strategies can prevent common pitfalls that lead to low success in email campaigns and communication failures.
Knowledge of best practices equips software developers and IT professionals to utilize SendGrid’s capabilities optimally. Here are some key domains to enhance your email communication.
Email Content and Formatting
Creating clear, well-structured email content is critical. Consider the following aspects:
- Subject Lines: Choose concise and informative subject lines. Aim to pique interest while also informing the recipient about the email's purpose.
- Personalization: Tailor emails to resonate with recipients. Personalization can increase open and conversion rates significantly.
- Clear and Relevant Content: Content should be to the point and informative. The main points should be easy to find. !
- Formatting: Utilize headings, bullet points, and proper spacing to make emails easy to read. Avoid large blocks of text as they can overwhelm the reader and may cause important information to get overlooked.
- Call to Action: Clearly state what you want recipients to do next. Whether it’s clicking a link or replying, a direct call to action is crucial.
Using HTML for formatting can help improve the visual presentation but be careful with overly complex designs.
Managing Bounce and Spam Reports
Bounces and spam reports can critically impact sender reputation. Thus managing these can not be ignored:
- Understanding Bounces: Bounces can be 'hard' or 'soft'. Hard bounces are permanent failures due to invalid addresses, while soft bounces may happen due to temporary issues.
- Measures to Manage Bounces: Regularly clean your email lists to remove invalid addresses. Implement automated checks on subscription forms to minimize errors.
- Monitoring Spam Reports: High spam complaints can tarnish your reputation as a sender. Utilize SendGrid statistics to track and assess the quality of your emails. Engage with feedback loops to understand why your emails are not being well-received.
Following these practices ensures that you minimize negative responses that influence future deliveries, establishing sustainable communication strategies.
Monitoring Email Deliverability
Understanding deliverability involves gaugeing how many emails actually reach their intended recipient folders as opposed to ending up in spam. Here are some useful tactics:
- Regular Performance Assessments: Consistently monitor analytics provided by SendGrid. Use these insights to understand open rates, click rates, and bounce rates.
- List Hygiene: Implement a method for automatically removing unengaged users from your lists. Usually, users are unresponsive after several emails.
- Use of Authentication Techniques: Deploy DMARC, DKIM, and SPF records to authenticate your emails. This ensures that your emails consented reaching user inboxes and strengthen your domain's credibility.
- Feedback Comprehension: Gather feedback directly from your audience. Use survey tools to learn what readers think about content and delivery.
Troubleshooting Common Issues
When utilizing the SendGridMessage class, it is crucial to understand how to troubleshoot common issues that may arise. Sending emails can sometimes be unpredictable, with delivery problems being a frequent occurrence. Addressing these issues promptly and efficiently ensures seamless email communication. Longitude between a functioning integration and a systematic breakdown often lies within proper troubleshooting protocols. Here, we will delve into two prevalent problems: failed deliveries and API key issues.
Failed Deliveries and Errors
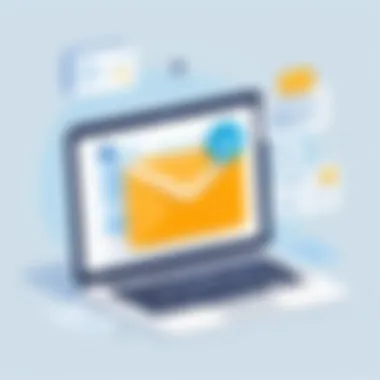
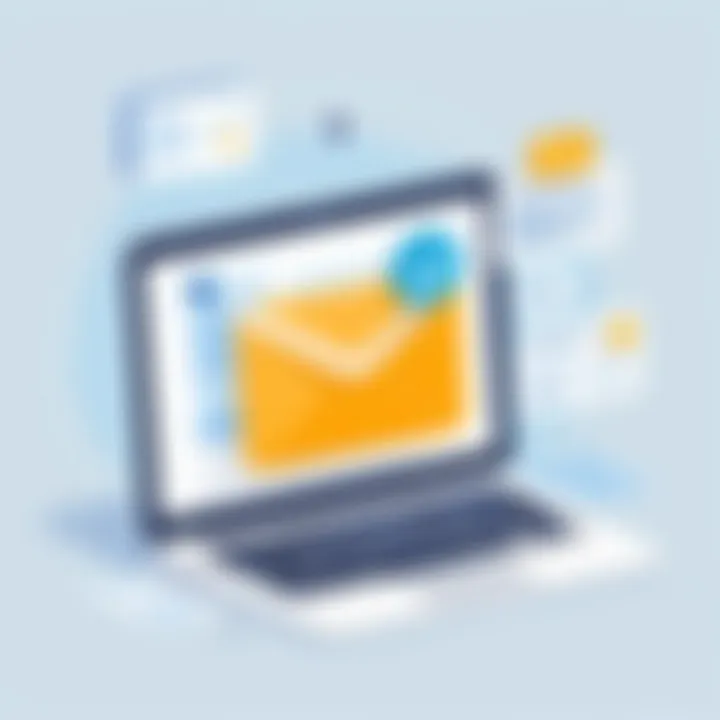
Failed deliveries can result from several factors. Understanding these factors can help in easily navigating such hurdles. Possible causes include erroneous email addresses, incorrect configuration, or issues with SendGrid’s services.
- Invalid Email Address: One of the most common reasons emails fail to deliver is to send to an incorrect address. A typo in the recipient's email can prevent successful delivery.
- Spam Filters: Often, delivered emails fail at the feet of reason filters created by recipient servers. Your outreach may be flagged as spam, leading to significant gaps in content outreach.
- Carrier Issues: Sometimes, the receiving email service may simply experience outages or issue processing incoming email paths.
When dealing with failed deliveries:
- Double-check the recipient's email address.
- Monitor SentGrid's status page for service disruptions.
- Implement feedback loops and monitoring from SendGrid to clarify where the issues are.
"Common detection and avoidance will enhance the reliability throughout email operations"
API Key Issues
The API key is a fundamental component needed for authenticating with the SendGrid API. Issues here can moot your ability to send any emails. API-related complications ground your efforts in integrating SendGrid’s capabilities effectively. There are several potential problems associated with API keys.
- Regenerating the API Key: If you're using an old API key, regenerating it may resolve connectivity issues with SendGrid.
- Incorrect Scopes: Make sure your API key has the correct permissions. Some tweets require particular access outside the default range.
- On-ramp and Deprecation Ensuring: Check if there is an upgraded version or novel endpoints when integrating your service thus keeping synergy.
For rectifying API key issues:
- Confirm your key configuration in your application or project environment.
- Consider creating user-friendly UI for sending error notifications on your application to enhance troubleshooting.
- Document the freshest sets of your API limitations during project updates or modifications in scope.
Overall, troubleshooting these common issues with vigilance enhances effectiveness and minimizes unnecessary disruptions. Expertise in this area is not simply useful but expects as standard in employing the SendGridMessage class in C#. When users can troubleshoot efficiently, they foster an environment with better trust and higher performance from their email management strategies.
Security Considerations
The topic of security considerations is essential when working with email services like SendGrid. As you develop applications that send emails, multiple security threats exist that can compromise your data and your users' information. A flawed implementation creates vulnerabilities such as exposing your API keys. Additionally, ensuring your communications are reliable prevents less undesirable email events like phishing, which can harm reputations.
Integrating effective security measures promotes trust and enhances user experience. By addressing potential risks upfront, you can reduce negative impacts and foster a secure atmosphere for email communication. Let’s delve into specific elements to focus on in this region.
Storing API Keys Securely
API keys represent credentials that give applications access to SendGrid's services. Storing them securely is fundamental. If these keys get exposed, unauthorized individuals may send emails from your account, leading to spam abuse or even data breaches. Thus, it is necessary to follow a set of best practices:
- Use Environment Variables: Store these keys in environment variables instead of hardcoding them into your application files. This keeps sensitive information out of the source code.
- Configuration Files: If you opt for config files, make sure your .gitignore file excludes these files from your version control system.
- Key Rotation: Regularly changing your API keys minimizes risk. Automating this as part of your security protocol can be a worthwhile long-term measure.
Here is an example of setting an API key in an environment variable:
By following these practices, you substantially lower your risks of exposing sensitive information.
Preventing Email Spoofing
Email spoofing is when unauthorized parties send emails appearing to come from a legitimate sender. This tactic undermines the reliability of email communications. To mitigate such scenarios, certain measures should be adopted:
- Use SPF and DKIM: These protocols help ensure that your emails are sent from verified sources. Setting these up complicates spammers' endeavors to impersonate your domain, safeguarding recipients from potential phishing scams.
- Monitor Bounce Back Reports: Regularly check bounce back messages for suspected spoof occurrences. Early identification curtails potential damages.
- Enable DMARC: Implementing DMARC (Domain-based Message Authentication, Reporting, and Conformance) builds an additional security layer. It tells receiving servers what to do with messages that fail SPF or DKIM checks, giving you greater control over your email communications.
By instilling these tactics, you not only protect your email, but also enhance your overall security framework.
Emphasizing security measures while implementing email services protects you and the users relying on those communications. The importance is undeniable in today's digital ecosystem.
The End
The conclusion of this article on using the SendGridMessage class in C# is vital for summarizing the key insights covered. A accessible yet in-depth look at effective email communication is essential for software developers and IT professionals alike. Sending emails through an API may sound simple, but ensuring their reliability and security requires diligence and proper understanding. In this section, we encapsulate the knowledge acquired, reinforcing the relevant practices to maximize the effectiveness of SendGrid.
Recap of Key Points
In reviewing the significant aspects discussed, we can outline a few core elements:
- Creating a SendGrid Account: This was detailed in the prerequisites. An account is necessary for utilizing any of SendGrid's features.
- API Key Usage: The prominence of a secure API key has been highlighted. Storing and handling this key with care is essential to prevent unauthorized access.
- Structure of SendGridMessage: Understanding this class was crucial for effective implementations. It was emphasized how to properly set up email components like recipients, subjects, and bodies.
- Best Practices: From content segmentation to email formatting, these practices promote better interaction with recipients.
- Security Considerations: Careful measures against common vulnerabilities, such as spoofing, were discussed. Protecting sensitive credentials remains paramount.
Ultimately, each topic correlates to the finesse and reliability one must embrace when working with SendGrid’s infrastructure. These principles enable both novice and experienced C# developers to cultivate an effective email-sending strategy.
Future of Email Communication
The avenues for email communication continue to evolve, too. With rapid technological advancements, the future appears to grasp areas like automation, machine learning, and enhanced security protocols.
- Automation: Expect more features that automate responses and enable personalized communication without heavy manual input.
- Machine Learning: As networks and user behavior analyze, optimized communication will likely become the norm.
- Security Improvements: With more threats emerging, rigorous security implementations will become standard rather than a luxury.
Adaptation in response to changing user experiences means a continuous evaluation of tools like SendGrid. Future email handling should prioritize both engagement and safety. Staying informed about the industry enhances your skills, ensuring effective communication channels for audiences globally. By embracing these changes, the foundation for successful email interactions is solidified.
Maintaining simplicity and focus in email communication allows for better connection with audiences, enhancing both engagement and response rates.