Mastering App Selection and Debugging on Android
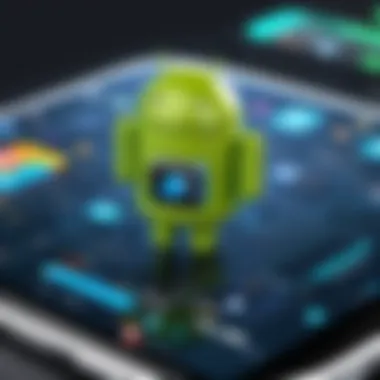
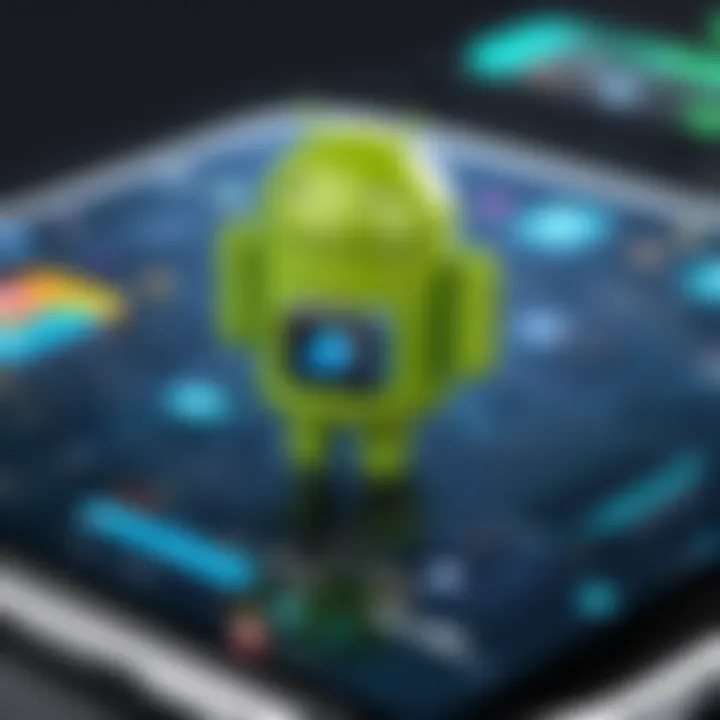
Intro
In the landscape of modern software development, Android stands out as a dominant platform for mobile applications. The selection and debugging processes are crucial for developers aiming for optimal performance and user satisfaction. This guide underscores the significance of understanding how to effectively select and troubleshoot Android applications. With the rapid evolution of technology, developers must stay abreast of tools and methodologies that can enhance their workflow.
Overview of Software Development for Android
Selecting and debugging apps on Android is not merely a technical necessity; it serves as the backbone of quality software development. The Android ecosystem encompasses a diverse array of devices and user environments. A well-designed application must consider these factors to ensure compatibility and user engagement.
Definition and Importance of Testing Tools
Testing tools are software applications that assist developers in identifying, diagnosing, and fixing issues within their Android applications. Their importance lies in the ability to enhance software quality and reliability. Utilizing efficient testing tools can lead to a smoother user experience, reducing the chances of negative reviews and enhancing the application's reputation.
Key Features and Functionalities
- Automated testing capabilities: Tools like Espresso and Robot Framework allow developers to automate tests, saving time and minimizing errors during the testing phase.
- Real-time debugging: Integrated Development Environments (IDEs) such as Android Studio provide real-time debugging features, enabling developers to track issues as they arise.
- Performance monitoring: Capabilities to monitor app performance can identify bottlenecks or crashes, essential for maintaining a high-quality user experience.
Use Cases and Benefits
These tools are beneficial in various scenarios, including:
- Pre-release validation: Ensuring the app is functional and stable before launching.
- Ongoing support and updates: Continuously tracking application performance post-release to safeguard against new issues.
- Collaboration among teams: Facilitating better communication and coordination within development teams through shared debugging observations.
Best Practices
Industry Best Practices for Implementation
Successful app selection and debugging involve adhering to established industry practices:
- Define clear criteria for app selection: Consider usability, performance, and security before development begins.
- Incorporate user feedback: Utilize insights gained from user experience to make data-driven adjustments.
Tips for Maximizing Efficiency
- Leverage automation: Implement automated testing processes to free up human resources for more complex problem-solving tasks.
- Optimize builds: Use build variants efficiently to eliminate unnecessary clutter and focus on relevant test scenarios.
Common Pitfalls to Avoid
- Neglecting edge cases: Overlooking uncommon user scenarios can lead to critical application failures.
- Forgetting about updates: Regularly updating debugging tools ensures compatibility with the latest Android OS versions, enhancing development efficiency.
How-To Guides and Tutorials
Step-by-Step Guides for Using Debugging Tools
- Setting up Android Studio: Install Android Studio and configure it for your project needs.
- Implementing unit tests: Create unit tests using the JUnit framework to ensure code functionality.
- Utilizing Android Profiler: Use the Android Profiler to gain insights on CPU, memory, and network utilizations.
Hands-on Tutorials for Beginners and Advanced Users
- Beginners can explore online resources that demonstrate how to use basic testing tools effectively.
- Advanced users may look into more complex scenarios such as integrating Continuous Integration (CI) systems with automated testing tools.
Practical Tips for Effective Utilization
- Regularly consult the documentation of the tools to stay aligned with current best practices.
- Join developer forums or platforms, such as reddit.com or facebook.com, to share experiences and insights with peers.
"Effective debugging is as important as code writing; it ensures that applications not only function correctly but also provide value to the users."
This guide aims to equip software developers, IT professionals, and tech enthusiasts with the essential knowledge to excel in selecting and debugging apps for the Android platform. The insights provided can enhance development efficiency and ultimately lead to superior applications.
Understanding Android App Debugging
Debugging is a critical aspect of the software development cycle, especially for Android applications. Effective debugging helps ensure that an app not only meets its intended functionality but also performs reliably under various conditions. This section aims to provide a clearer understanding of the debugging process, its significance, and the challenges developers may face.
The Importance of Debugging in Software Development
Debugging plays a vital role in maintaining the quality of software applications. It involves identifying and resolving bugs or issues in the code. The result is a more robust application that provides users with smooth experiences. A few key benefits of debugging include:
- Improved Application Stability: Regular debugging leads to a reduction in crashes and unexpected behavior, ensuring that users have a consistent experience.
- Enhanced Performance: By locating inefficiencies and memory leaks, developers can improve an app's performance. This is essential in a competitive environment where users have high expectations.
- User Satisfaction: When issues are addressed proactively, users are more likely to remain satisfied, leading to higher retention rates.
Without a proper debugging process, developers risk releasing flawed applications into the market. This can result in negative reviews and reduced user trust. Consequently, investing time in debugging can yield long-term benefits for any software project, especially those targeting the Android platform.
Common Challenges Faced During Debugging
Debugging is not without its hurdles. Developers commonly encounter obstacles that can hinder their progress. Recognizing these challenges can help in developing strategies to overcome them. Some frequent issues include:
- Complex Codebases: As apps grow, their codebases often become more intricate, making it difficult to pinpoint the source of an error.
- Environment Differences: Bugs may only appear in certain conditions or devices, complicating their identification. This is especially true in the diverse Android ecosystem.
- Time Constraints: Tight deadlines may result in rushed debugging efforts. Developers might overlook important steps in the process.
- Limited Information: Debugging tools may not always provide concrete information on what went wrong, leaving developers with little direction.
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it."
This quote summarizes the inherent difficulty present in debugging efforts. Identifying the root cause of bugs requires a clear strategy and thorough understanding of the app's design. By anticipating and addressing these common challenges, developers can foster more effective debugging practices.
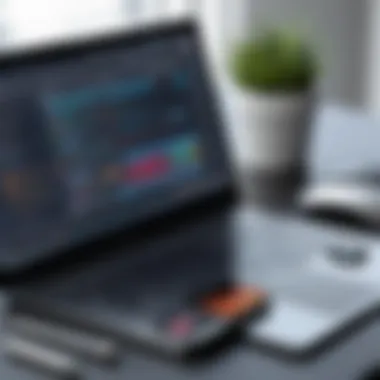
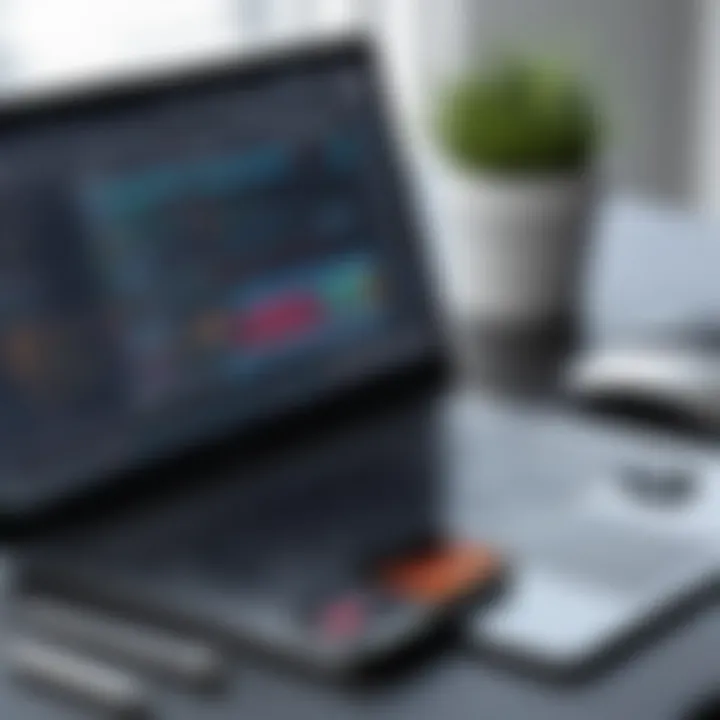
Prerequisites for Debugging Android Apps
Debugging Android applications is a crucial phase in the app development lifecycle. Before diving into the complexities of this task, it is essential to understand the prerequisites necessary for effective debugging. These prerequisites ensure developers can efficiently identify and resolve issues within their applications. Moreover, having the right system requirements and software tools significantly influences the debugging effectiveness and speeds up problem resolution.
System Requirements for Effective Debugging
Having a capable system is non-negotiable for successfully debugging Android apps. An excellent starting point is to ensure that the operating system is compatible with Android Studio and its requirements. Windows, macOS, or Linux can be used, but each has its own set of specifications. Here are general requirements to consider:
- Processor: At least a dual-core CPU, preferably Intel i5 or higher.
- Memory: A minimum of 8GB of RAM is ideal for smoother performance.
- Storage: SSDs are recommended for faster data access and application loading, with a minimum of 4GB available space for Android Studio.
- Graphics: Dedicated graphics card is not mandatory, but better rendering speed improves efficiency during resource-intensive debugging activities.
These requirements ensure that the development environment runs smoothly, minimizing the chances of crashes or freezes during the debugging process.
Essential Software Tools for Debugging
Debugging Android apps typically involves various software tools that facilitate a more effective process. Several key tools stand out, including Android Studio, ADB (Android Debug Bridge), and Emulators or Real Devices. Each of these tools serves a unique purpose in the debugging workflow.
Android Studio
Android Studio is more than just an IDE; it is a comprehensive software development environment specifically designed for Android development. Its robust debugging features make it a cornerstone tool for developers. One of the core advantages of Android Studio is its integration with various debugging tools such as the Debugger, Logcat, and Profiler.
The unique feature of Android Studio is its powerful Graphical User Interface, which allows developers to visualize their layouts and methods effectively. This interface enhances understanding and interaction with the app's components while debugging. While Android Studio is a beneficial choice, it may require a steep learning curve, especially for developers new to the platform.
ADB (Android Debug Bridge)
ADB, or Android Debug Bridge, is a command-line tool that enables communication between a computer and an Android device. It plays a crucial role in debugging Android applications by allowing developers to install apps, execute commands, and access device logs. ADB stands out due to its versatility in managing multiple devices and emulators.
A significant unique feature of ADB is its ability to run shell commands through the command line. This capability can be incredibly useful for deeper analysis and troubleshooting of issues that may not be visible through the Android Studio GUI. However, ADB commands can sometimes overwhelm new users because of their syntax and complexity.
Emulators and Real Devices
Emulators provide a virtual testing environment for Android applications. They simulate different devices, allowing developers to test apps across various screen sizes and Android versions without needing multiple physical devices. The key characteristic of emulators is their cost-effectiveness and ease of use when setting up.
On the other hand, testing on real devices offers a more authentic environment. Real devices help identify issues that may not appear in emulators, such as performance constraints or hardware compatibility problems. A unique advantage of real devices is their ability to interact with hardware features like GPS, camera, and sensor inputs directly.
Using both emulators and real devices might be ideal to ensure comprehensive testing. This hybrid approach provides a balanced view, helping identify more bugs efficiently.
"Understanding the prerequisites for debugging applications helps streamline the process, leading to successful application deployment."
Selecting the Right Android App for Debugging
Choosing the right Android app for debugging is crucial for optimizing the development process. Each application can bring unique challenges and learning opportunities. A well-selected app enables developers to implement effective debugging strategies, ensuring smooth performance and user satisfaction. This section outlines parameters for selection and emphasizes the benefits of a focused approach in debugging.
Criteria for App Selection
Functionality Testing
Functionality testing is a critical aspect because it evaluates how well an app performs its intended functions. This testing phase identifies any discrepancies between the app’s expected behavior and actual performance. Key characteristics of functionality testing include its ease of use and straightforward approach. This makes it a beneficial choice for both novices and experienced developers. By focusing on core functions, developers can ensure that the app operates correctly under various conditions.
Unique features of functionality testing involve checks for usability, data handling, and integration with other systems. While advantages include quick identification of bugs, the potential disadvantage is its limited scope. It primarily concerns the functional aspects, possibly overlooking performance or security vulnerabilities.
User Experience Evaluation
User experience evaluation centers on assessing how users interact with the app. This aspect contributes significantly to app success, as user satisfaction directly correlates with app retention rates. A key characteristic of this evaluation is its focus on intuitive interfaces and user pathways. This popularity as a criterion for selection stems from its emphasis on user-centric design.
The unique feature of user experience evaluation is its focus group testing, often involving real users in beta phases. Benefits include turns of invaluable feedback that enhances intuitive design. However, a disadvantage is it can be time-consuming, requiring diverse user demographics for accurate data collection.
Performance Metrics
Performance metrics involve quantitative measurements of app efficiency and resource usage. This evaluation is essential when aiming to develop robust applications that can handle heavy usage without lag. A primary characteristic is its ability to track various performance indicators. This makes performance metrics a popular choice as they provide actionable data allowing developers to refine their apps.
Unique features include monitoring CPU usage, memory consumption, and response times. The advantages encompass identifying bottlenecks in real-time, facilitating prompt fixes. Nevertheless, a downside can be the complexity, as excessive focus on these aspects may detract from other equally crucial areas such as accessibility.
Identifying App Dependencies and Limitations
Understanding app dependencies is vital for determining how other software components may affect the app’s functionality. Dependencies can include libraries and third-party services that are integrated into the application. Recognizing these relationships helps in troubleshooting issues that might arise due to them. Moreover, knowing limitations, such as API usage restrictions, can prevent potential pitfalls during the debugging process. This awareness is also key when planning updates or new features, making it an integral part of the overall debugging strategy.
Setting Up Android Debugging Environment
Setting up the Android debugging environment is a crucial step in the app development lifecycle. It lays the foundation for efficient debugging and testing, enabling developers to identify and resolve issues swiftly. An appropriately configured debugging environment increases productivity and reduces frustration during the development process.
Configuring Developer Options on Android Devices
To configure developer options, you first need to unlock these settings on the Android device. Here's how you can do it:
- Open Settings on your device.
- Scroll down to .
- Find the and tap it seven times. You should see a message confirming that you are now a developer.
Now, return to the main settings menu, and you will see a new option labeled . Within this section, several settings are pivotal for debugging. For example, enabling allows your device to communicate with your development machine, facilitating the transfer of data and commands. Additionally, you can adjust various settings such as window animations, background process limits, and more to optimize the performance during debugging sessions.
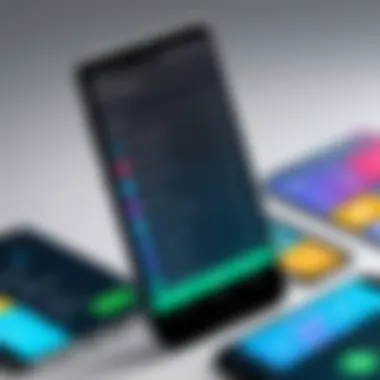
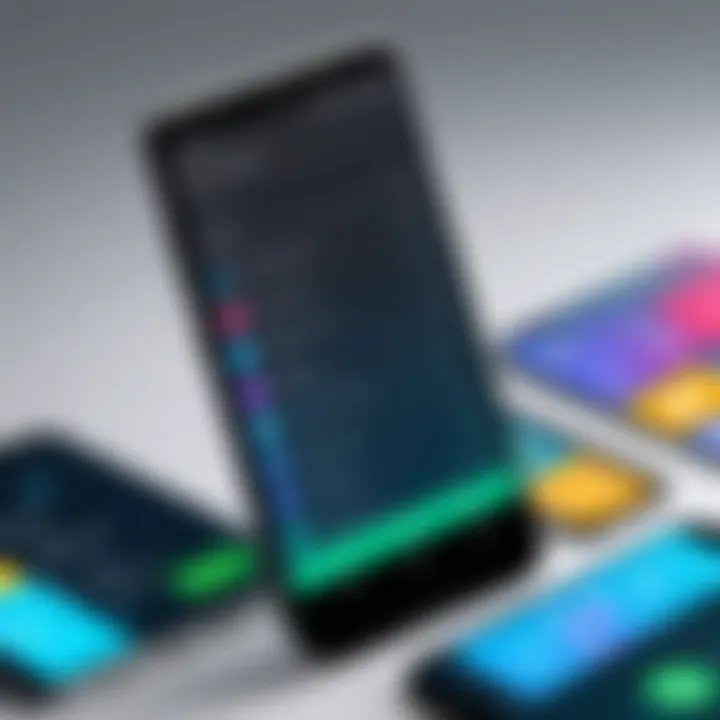
These configurations ensure that you can access the available tools designed for debugging. Missing even a small step can lead to inefficient debugging processes or hinder the connection between your device and your development environment, so attention to detail is essential.
Connecting Devices for Debugging
The connection between your Android device and your development environment is essential. This connection allows you to deploy apps directly to the device and enables real-time debugging. The basic requirement is to have a USB cable for a wired connection, or you can set up a wireless connection if needed.
Steps to connect a device:
- Via USB Cable:
- Via Wireless Debugging:
This method involves additional setup. You will need to:
- Connect your Android device to your computer using a USB cable.
- The device should prompt you to allow USB debugging. Accept this prompt.
- Ensure that your computer recognizes the device. You may need to install necessary drivers depending on your OS.
- Connect your device and computer to the same Wi-Fi network.
- Obtain the device's IP address from the Wi-Fi settings.
- Use ADB commands in the terminal to connect, such as .
- After successful connection, you can begin deploying and debugging wirelessly.
Making sure that your device is properly connected can save time and effort during the debugging process. Any misalignment in the connection can lead to disrupted workflow, such as failing to deploy applications or retrieve logs. Therefore, verifying these connections is one key part of setting up your debugging environment for Android app development.
Utilizing Debugging Tools in Android Studio
Utilizing debugging tools in Android Studio is essential for any developer aiming to deliver high-quality applications. These tools are not just helpful; they are crucial in identifying issues that affect app functionality, performance, and user experience. By leveraging the full potential of Android Studio's debugging features, developers can enhance their productivity significantly and ensure the final product meets users' expectations.
Using the Logcat Tool Effectively
The Logcat tool is an integral component of Android Studio's debugging suite. It provides a real-time log of system messages, which include stack traces when the device throws errors. Experienced developers know that using Logcat effectively can lead to a faster debugging process.
To use Logcat effectively, set the filters to only show relevant messages. Developers can filter by application name, severity level, and specific tags. This allows a focused view on the issues at hand, cutting through irrelevant data. The key characteristic of Logcat is its ability to display logs dynamically, which means developers can track issues as they happen during runtime.
Breakpoints and Stepping Through Code
Breakpoints allow developers to pause application execution at designated lines of code, enabling them to inspect the state of the application. This method gives insight into program flow, variable states, and function calls—vital elements when isolating bugs.
Setting and Removing Breakpoints
Setting breakpoints is straightforward in Android Studio. By clicking on the margin next to the line of code, a breakpoint gets established. It is easy to toggle these breakpoints on or off, allowing for flexibility depending on the debugging needs. The unique feature lies in the ability to have conditional breakpoints, which only pause execution when certain conditions are met. This can significantly cut down debugging time by focusing on specific cases instead of running through every line.
However, while beneficial, excessive breakpoint usage can complicate the debugging process if not managed properly. Too many active breakpoints can lead to confusion, making it harder to trace the actual problem.
Inspecting Variable States
Inspecting variable states during debugging is critical. This feature allows developers to see the current value of variables at each breakpoint, providing immediate insight into whether the application logic works as intended. This aspect plays a central role in diagnosing issues that arise due to incorrect variable values.
Variable inspection is popular among developers because it allows for real-time feedback on the application's state. This can rapidly inform the decision-making process when troubleshooting. A notable advantage is that developers can also modify variable values on-the-fly to test different scenarios without restarting the app.
However, the drawback might be the potential introduction of new bugs when altering values. Therefore, careful consideration is important when leveraging this feature.
Advanced Debugging Techniques
Debugging is a nuanced and essential component of Android app development. In today's landscape, where applications are increasingly complex, advanced debugging techniques must be utilized. These techniques facilitate developers in identifying troubling issues efficiently and thoroughly, thus ensuring a smoother user experience. The key in employing these techniques is understanding both their value and application in real-world scenarios.
Memory Analysis and Leak Detection
Memory leaks can severely hinder app performance, leading to crashes or slowdowns. The process of memory analysis is critical in pinpointing inefficient memory usage. Tools like Android Studio's memory profiler help developers visualize memory allocations and pinpoint leaks.
- Key Benefits:
- Reduces app crashes.
- Improves performance.
Memory analysis also involves tracking object lifecycles. Identifying objects that should have been garbage collected and remain allocated can lead to major optimizations. This understanding is invaluable for enhancing overall application reliability, particularly for resource-intensive apps.
Performance Profiling Tools
Performance profiling is another key aspect that goes hand-in-hand with debugging. With tools designed for monitoring resource use, developers can gain insights into how their applications perform under various conditions.
CPU Profiler
The CPU Profiler is integral for performance profiling. It provides a detailed breakdown of CPU usage while the user interacts with the application. One major characteristic of this tool is its ability to show real-time CPU activity, allowing for immediate and tangible analysis.
- Unique Feature: Ability to record method traces.
- Advantages: Helps identify CPU bottlenecks.
- Disadvantages: Some learning curve can be involved.
Using the CPU Profiler enables developers to see which methods are consuming the most processing power, thus facilitating targeted optimizations.
Network Profiler
The Network Profiler is another crucial tool for developers looking to ensure efficient data communication. This tool highlights how much data the app uses over both Wi-Fi and mobile networks.
- Key Characteristic: Presents a visual timeline of network traffic.
- Unique Feature: Monitors both inbound and outbound data calls.
- Advantages: Assists in optimizing data usage, which is critical for user satisfaction.
- Disadvantages: Can be overwhelming with a large volume of data.
The visual representation makes it easier to spot episodes of excessive network usage, which is often a sign of inefficient coding practices or issues with third-party libraries.
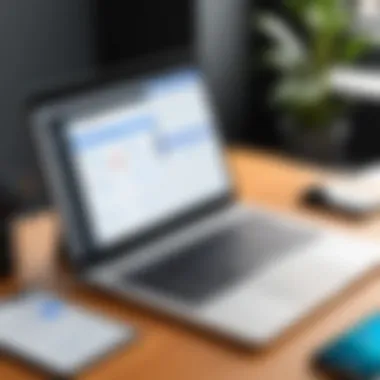
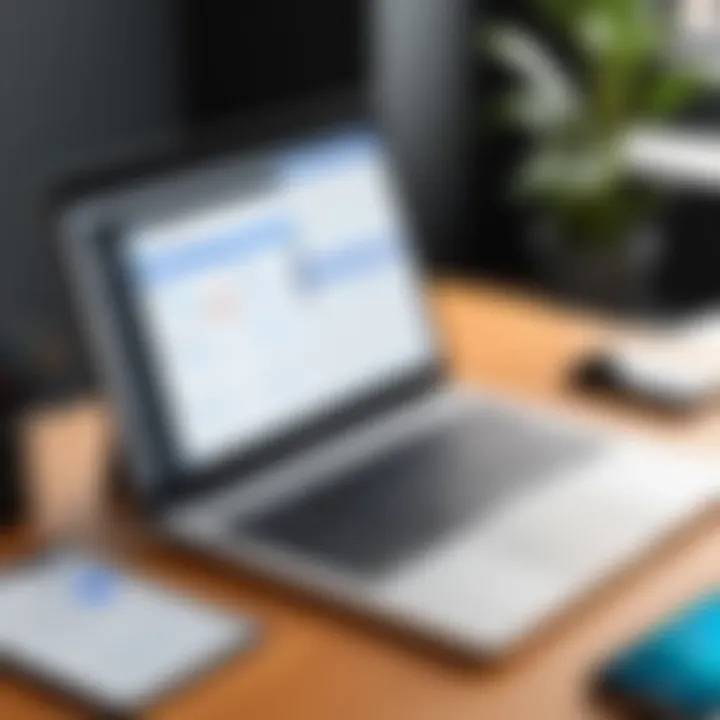
Understanding these advanced debugging techniques will not only contribute to your skill as an Android developer but also ensure that the applications you create deliver optimal user satisfaction. Leveraging tools like the CPU and Network Profilers can dramatically improve the quality and efficiency of your software.
Handling Debugging Errors
Handling debugging errors is a critical aspect of the Android app development process. When developers encounter errors, they can stall progress and diminish user experience. Understanding these errors and how to resolve them leads to smoother application performance and increased user satisfaction. This section explores common debugging errors and their implications, as well as ways to effectively manage runtime exceptions.
Common Error Messages and Their Implications
Common error messages serve as vital clues during the debugging process. Recognition of these messages can help developers quickly identify issues and implement solutions. Understanding the implications of these messages is equally important, as it aids in preventing future occurrences.
A few prevalent error messages include:
- NullPointerException: Often encountered when an application tries to use an object reference that has not been initialized. This can lead to application crashes if not handled appropriately.
- ArrayIndexOutOfBoundsException: This occurs when an attempt is made to access an index that is out of the bounds of an array. It’s crucial for developers to ensure index numbers align with the array size to avoid such errors.
- ClassCastException: Happens when an object is attempted to be cast to a subclass of which it is not an instance. This signifies a need for careful class management.
Developers must analyze the context surrounding these messages. Sometimes, they may indicate larger structural issues within the code. Proper handling of these messages can lead to a more robust application, providing a better experience for end users.
Resolving Runtime Exceptions
Resolving runtime exceptions requires a structured approach and familiarity with both the application and the debugging tools available. Runtime exceptions can hinder the functioning of an app, making timely resolution essential for maintaining usability. Here are several strategies to address these exceptions effectively:
- Reproducing the Error: Before implementing solutions, it is essential to reproduce the error consistently. This helps understand the conditions under which the error occurs.
- Reviewing Recent Changes: If a runtime exception arises after recent changes to the code, reviewing those modifications can unearth the source of the error. Often, new code introduces unforeseen complications.
- Utilizing Logcat: Logcat is a powerful tool that displays system messages, including stack traces when runtime exceptions occur. A careful examination of these logs can provide insight into where the error originated.
- Implementing Exception Handling: Proper exception handling using try-catch blocks can prevent abrupt application crashes. It allows developers to manage exceptions gracefully and provide user-friendly error messages.
- Conducting Code Reviews: Collaborative reviews of code can highlight potential pitfalls that one developer may overlook. This sharing of knowledge among team members fosters a culture of quality and attentiveness.
Testing Strategies Post-Debugging
Testing strategies following the debugging phase are vital to ensure the application meets its intended functionality. The core purpose of this phase lies in validating that not only have the bugs been effectively resolved but also that no new issues have been introduced during the debugging process. A structured approach to testing is essential, as it reduces the risk of malfunctions once the application is deployed. Developers and IT professionals must focus on several key elements, such as establishing a rigorous testing environment, implementing scenarios that mimic real-world usage, and prioritizing user feedback.
Best Practices for Testing Applications
Establishing a set of best practices serves to streamline the testing process. It provides a framework for obtaining consistent results and maximizing reliability. Here are several recommended practices:
- Define Clear Objectives: Before testing begins, establish measurable goals related to functionalities, performance, and user experience. Knowing what you want to accomplish helps maintain focus throughout the testing process.
- Automate where Possible: Automation can significantly enhance the efficiency of testing. Utilizing tools that facilitate automated tests can save time and ensure consistency across multiple testing scenarios.
- Incorporate Unit and Integration Testing: These methods help to identify bugs at an early stage. Unit testing focuses on individual components, while integration testing evaluates how well these components function together.
- Conduct Performance Testing: After debugging, it is important to examine how the app performs under various loads and user interactions. This ensures that the app can handle the expected traffic once in production.
- User Acceptance Testing (UAT): Engage actual users to test the application. Their feedback is crucial as it reflects real-world interactions and helps identify any usability issues that you might not have foreseen.
Automated Testing Frameworks to Consider
In the realm of software development, various automated testing frameworks can enhance the efficiency and coverage of your testing strategies. Here are some notable options:
- JUnit: A widely-used framework for unit testing in Java applications. It is essential for developers aiming to test their Android applications effectively.
- Espresso: A framework specifically for testing Android applications. It simplifies the process of writing UI tests, allowing you to simulate user interactions seamlessly.
- Mockito: This tool aids in creating mock objects for unit tests. It enhances the application of test-driven development by allowing developers to simulate certain scenarios without relying on the actual components.
- Spoon: This framework is used to run tests on multiple devices simultaneously. It helps in capturing screenshots and can be useful for comparative analysis between different environments.
- Robot Framework: An open-source automation framework that supports keyword-driven testing, making it flexible for both beginners and advanced users.
Utilizing these frameworks, developers can ensure a higher quality application post-debugging and streamline the testing effort. Implementing these strategies effectively lays the groundwork for a smoother deployment and an enhanced user experience.
Deploying Applications After Debugging
Once an Android application has undergone debugging, the next critical phase is deployment. This stage is where the efforts invested in debugging culminate into a functional product ready for end-users. Proper deployment is not merely the final step; it serves as a gateway to the application's real-world performance and user interactions. A successful deployment ensures that the app behaves as intended across various devices and conditions, leading to higher user satisfaction and fewer post-release issues.
Preparation for App Release
Before releasing an application to the public, various preparations are essential. These tasks help validate the application's readiness for the market. First, developers must ensure that all identified bugs are resolved, and outstanding issues are either fixed or accounted for in release notes.
Further, developers should verify that the app adheres to the platform's guidelines. This compliance guarantees that the application meets the necessary performance and usability standards set by the Android ecosystem. It is beneficial to conduct a final round of testing under different scenarios to confirm that there are no emerging issues. This round may involve running the app on multiple devices, observing its functionality across different operating systems and screen sizes.
Moreover, meticulous attention should be directed towards optimizing the app's performance. This can involve cleaning up the code, minimizing resources, and leveraging appropriate icons and graphics that won't slow down the app or consume excessive battery life. Establishing a clear understanding of app size and loading times can significantly impact user experience and retention rates.
Lastly, ensure that all necessary metadata, such as app descriptions, keywords, and screenshots for the app store, are appropriately prepared. This can enhance visibility in app stores and attract potential users effectively.
Best Practices for Deployment
Effective deployment involves adhering to several best practices, which can significantly influence the ongoing success of the application.
- Thorough Testing: Even after debugging, extensive user acceptance testing is crucial. Engage beta testers to gather feedback about usability and performance in real-world scenarios. This feedback can uncover hidden issues that internal testing may misinterpret.
- Incremental Rollout: Instead of releasing the app to the entire user base simultaneously, consider an incremental rollout. This strategy enables monitoring of the app's performance and user responses on a smaller scale before broader release, reducing risks associated with unforeseen issues impacting the entire user base.
- Monitor Post-Deployment Metrics: After launch, closely monitor app metrics such as crash reports, user engagement statistics, and performance analytics. Tools such as Firebase Crashlytics can provide valuable insights into how the app behaves post-launch.
- User Feedback and Updates: Encourage users to provide feedback through reviews and direct communication. Addressing concerns raised by users promptly can improve the app's reputation and user retention significantly.
- Documentation and Support: Prepare comprehensive documentation for users and a support system that can assist with any queries or problems they encounter with the app. This support extends the reliability of the app and fosters user loyalty.
"A well-prepared deployment can ensure that your app reaches its intended audience effectively and performs reliably in the field."
Integrating these practices will lead to a smoother deployment experience, ultimately solidifying the application’s presence in the competitive market. Well-prepared deployments maintain the integrity of the app, thus enhancing the overall user experience and satisfaction.
Ending and Future Trends in Android Debugging
The conclusion and the future trends of Android debugging are crucial components in understanding how the field is evolving. As technology advances, so do the methods and tools available for debugging applications, making it essential to stay informed about these changes.
Android developers must recognize the importance of applying modern techniques in debugging processes. The efficiency of an application can be greatly improved when developers not only understand their current debugging tools but also embrace the latest advancements. Keeping abreast of industry trends allows professionals to incorporate best practices into their workflow while enhancing their application quality.
In this section, we will summarize the key takeaways from the debugging process and discuss emerging technologies that could shape future practices.
Summarizing Key Takeaways
- Importance of Debugging: Debugging remains a fundamental aspect of software development. Effective debugging can lead to improved application performance, reduced errors, and a better user experience.
- Tools and Practices: Knowledge of tools like Android Studio and ADB is crucial. Understanding how to utilize these tools effectively can streamline the debugging process.
- Testing Post-Debugging: Engaging in thorough testing after debugging ensures that any fixes do not introduce new issues, maintaining the integrity of the application.
- Continuous Learning: The field of technology is always advancing. Continued education and adaptation to new debugging techniques is beneficial for developers.
Emerging Technologies in App Development
The landscape of app development is continually changing with the advent of new technologies. Some prominent trends to watch include:
- Machine Learning Integration: Applications utilizing machine learning can now identify bugs proactively, predicting issues before they happen and providing real-time debugging solutions.
- AI-Driven Testing Tools: Artificial intelligence is being leveraged to automate testing, reducing the amount of manual intervention required and speeding up the overall debugging process.
- Cloud-Based Solutions: Cloud technologies are allowing for more sophisticated debugging tools that can be shared among teams, enhancing collaboration and efficiency.
- Real User Monitoring (RUM): By implementing RUM, developers can gather data on how real users interact with their applications. This information offers essential insights into areas that may require debugging.
"Staying ahead of trends is essential in maintaining the effectiveness and relevance of your development practices."
As Android debugging continues to evolve, remaining adaptable and aware of new technologies will ensure higher quality applications and a more efficient development cycle.