Mastering Security: Securing ASP.NET Core 3.1 Web API with Bearer Token Authentication
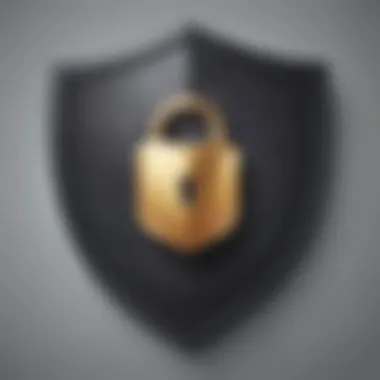
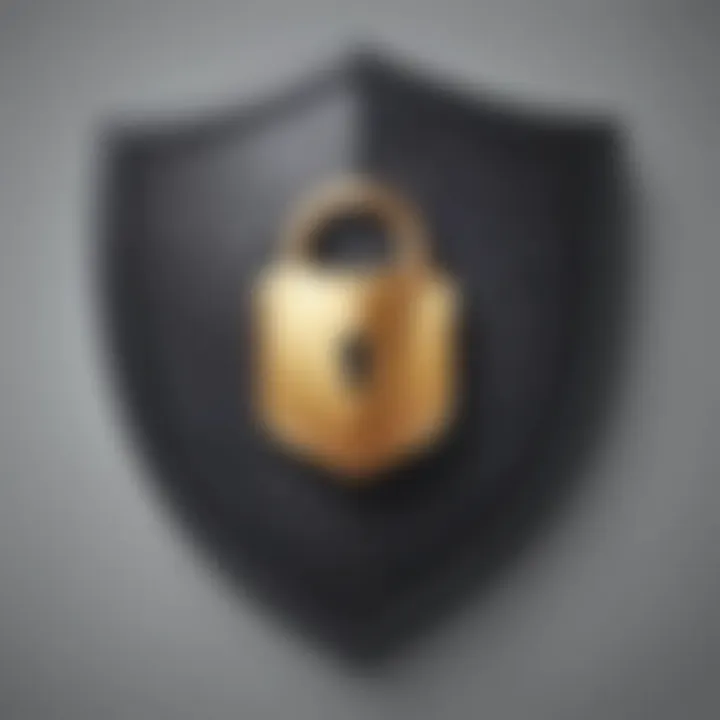
Overview of Securing ASP.NET Core Web API with Bearer Token Authentication
ASP.NET Core 3.1 Web API is a powerful framework utilized for developing secure web applications. By implementing Bearer Token Authentication, developers can enhance the security of their applications significantly. This method provides a way to authenticate API requests using tokens, ensuring that only valid users can access protected resources. It is crucial to understand the fundamentals of Bearer Token Authentication to reinforce the security mechanisms of ASP.NET Core 3.1 Web API.
- Key features and functionalities:
- Use cases and benefits:
- Token-based authentication
- Authorization of API requests
- Enhanced security measures
- Secure access to API endpoints
- Prevention of unauthorized access
- Simplified authentication process for users
Best Practices for Securing ASP.NET Core Web API with Bearer Token Authentication
Implementing Bearer Token Authentication in ASP.NET Core 3.1 Web API requires adherence to industry best practices to ensure optimal security. Developers must pay attention to key aspects to maximize efficiency and productivity while avoiding common pitfalls that could compromise the application's security. By following these best practices, developers can create a robust security framework for their web applications.
- Industry best practices for implementing Bearer Token Authentication:
- Tips for maximizing efficiency and productivity:
- Common pitfalls to avoid:
- Token validation and expiration management
- Encrypted token transmission
- Role-based access control
- Regularly update token validation logic
- Monitor and log authentication attempts
- Implement multi-factor authentication for added security
- Storing tokens in insecure locations
- Failing to revoke compromised tokens
- Neglecting token expiration policies
Case Studies of Secure ASP.NET Core Web API Implementations
Real-world examples showcase successful implementation of Bearer Token Authentication in ASP.NET Core 3.1 Web API. Industry experts provide insights into their experiences, highlighting the lessons learned and outcomes achieved through the adoption of this security measure. These case studies offer valuable learnings for developers looking to bolster the security of their web applications.
- Lessons learned and outcomes achieved:
- Insights from industry experts:
- Enhanced security posture
- Improved user authentication experience
- Mitigation of potential security threats
- Recommendations for seamless implementation
- Best practices for maintaining secure authentication
- Future trends and advancements in web application security
Latest Trends and Updates in ASP.NET Core Web API Security
Stay informed about the upcoming advancements and trends in ASP.NET Core 3.1 Web API security. By understanding the current industry landscape and forecasts for web application security, developers can prepare for upcoming innovations and breakthroughs. Keeping pace with the latest trends is essential for maintaining robust security measures in ASP.NET Core 3.1 Web API.
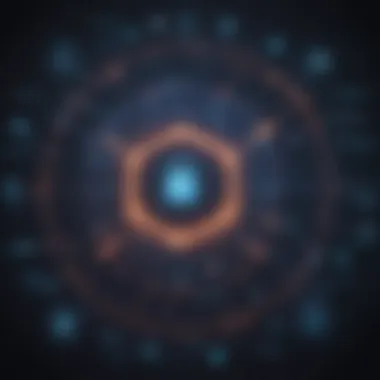
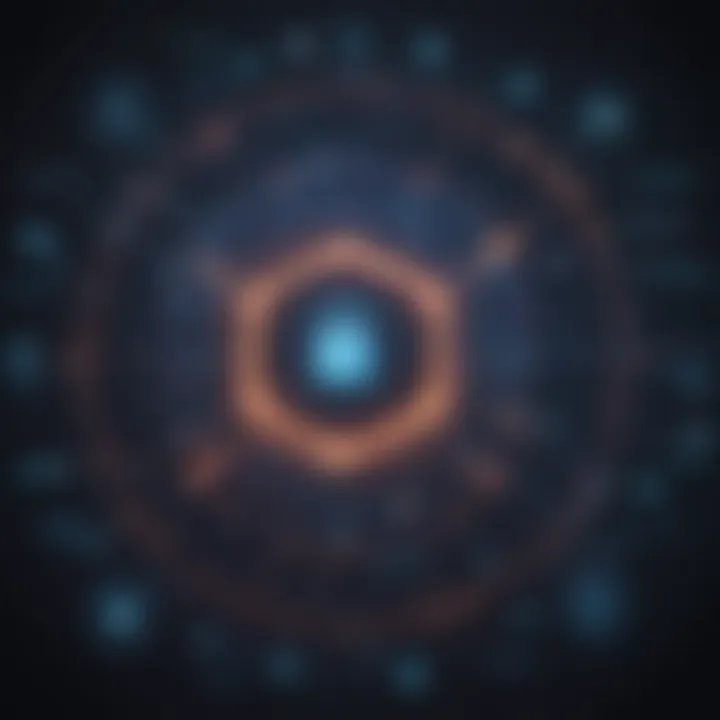
- Current industry trends and forecasts:
- Innovations and breakthroughs:
- Integration of machine learning for anomaly detection
- Continuous advancements in token encryption techniques
- Evolution of security protocols for enhanced protection
- Implementation of biometric authentication
- Adoption of blockchain technology for secure token management
- Integration of threat intelligence for proactive security measures
How-To Guide for Implementing Bearer Token Authentication in ASP.NET Core Web API
A step-by-step guide for developers to effectively implement Bearer Token Authentication in ASP.NET Core 3.1 Web API. This tutorial caters to both beginners and advanced users, offering practical tips and tricks to streamline the authentication process and enhance the security of web applications. By following this comprehensive guide, developers can fortify their ASP.NET Core 3.1 Web API with robust security measures.
- Hands-on tutorials for beginners and advanced users:
- Practical tips and tricks for effective utilization:
- Generating and validating bearer tokens
- Configuring token-based authentication middleware
- Implementing role-based access control
- Managing token expiration policies
- Securing token transmission over HTTPS
- Logging and monitoring authentication activities
Securing ASP.NET Core Web API with Bearer Token Authentication
Introduction
Securing an ASP.NET Core 3.1 Web API with Bearer Token Authentication is a critical aspect of modern web development. In today's interconnected digital landscape, where cyber threats loom large and data breaches are rampant, implementing robust security measures is non-negotiable. Bearer Token Authentication serves as a shield, fortifying your Web API against unauthorized access and safeguarding sensitive information. Understanding the nuances of this authentication method is paramount for developers looking to bolster the defense mechanisms of their applications, instilling trust and confidence in their users.
Understanding ASP.NET Core Web API
Overview of ASP.NET Core
At the core of ASP.NET Core 3.1 lies a framework renowned for its versatility and performance. Leveraging the latest advancements in web development technology, ASP.NET Core 3.1 empowers developers to build scalable and efficient Web APIs. Its modular design and robust architecture provide a solid foundation for creating applications that are not only secure but also high-performing. By understanding the intricacies of ASP.NET Core 3.1, developers can harness its full potential, delivering seamless user experiences and optimizing productivity.
Role of Web APIs in Modern Applications
Web APIs play a pivotal role in the interconnected web ecosystem, serving as the communication bridge between various software applications. In modern applications, Web APIs facilitate seamless integration, enabling different systems to interact and share data effectively. By embracing Web APIs, developers can streamline processes, enhance connectivity, and promote interoperability across diverse platforms. Understanding the significance of Web APIs equips developers with the tools needed to build agile and responsive applications that meet the demands of today's dynamic digital landscape.
Importance of Authentication in Web Applications
Ensuring Data Security
Data security is a fundamental concern in web applications, especially in an era governed by stringent privacy regulations and growing cybersecurity threats. By implementing robust authentication measures, developers can safeguard sensitive information, prevent unauthorized access, and uphold the integrity of their applications. Ensuring data security instills confidence in users, fostering trust and loyalty towards the application and its services.
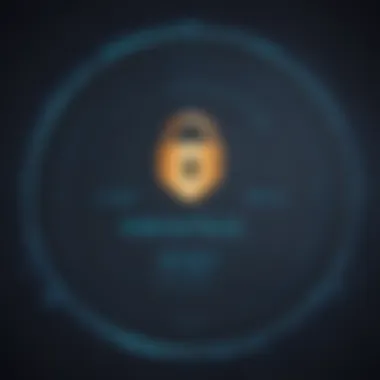
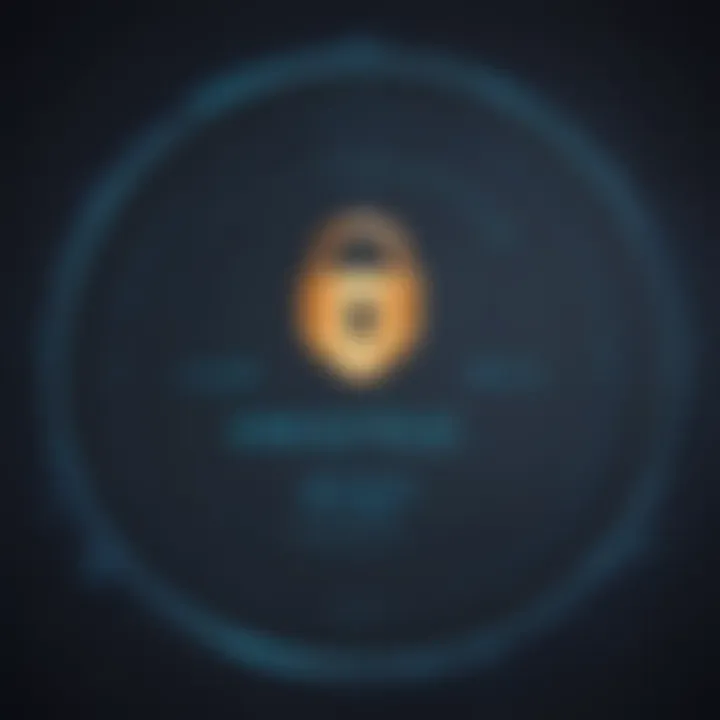
Protecting User Privacy
User privacy is sacrosanct in the digital realm, and protecting it should be a top priority for developers. By enforcing stringent privacy measures, developers can prioritize user confidentiality, comply with data protection regulations, and instill a sense of transparency in their interactions with users. Protecting user privacy not only mitigates the risk of data breaches but also enhances the overall user experience, fostering long-term relationships built on trust and mutual respect.
Overview of Bearer Token Authentication
Definition and Functionality
Bearer Token Authentication operates on the principle of bearer tokens, which serve as access tokens granted to authenticated users. This authentication method validates the identity of users based on the possession of a unique token, enabling secure access to resources within the Web API. The simplicity and efficiency of bearer token authentication make it a popular choice for developers seeking a scalable and reliable security mechanism for their applications.
Advantages over Other Authentication Methods
In comparison to traditional authentication methods, such as session-based or cookie-based authentication, bearer token authentication offers several advantages. By decoupling the authentication process from the session state, bearer tokens promote stateless communication, improving scalability and performance. Additionally, bearer tokens are less vulnerable to cross-site request forgery (CSRF) attacks, enhancing the overall security posture of the application. Embracing bearer token authentication empowers developers to adopt a modern and robust security approach, elevating the protection of their Web APIs and ensuring a seamless user authentication experience.
Implementation
In the realm of securing ASP.NET Core 3.1 Web API, the implementation phase emerges as a pivotal component. It stands as the bridge between theory and practice, where concepts materialize into actual security measures. Implementation encompasses the translation of theoretical knowledge into tangible actions, ensuring that the proposed security mechanisms are effectively put into operation. This article illuminates the significance of implementation, delving into specific elements like setup, integration, and validation processes that are indispensable for fortifying the security posture of ASP.NET Core 3.1 Web APIs.
Setting Up ASP.NET Core Web API Project
Creating a New Project
Embarking on the journey of securing an ASP.NET Core 3.1 Web API involves the foundational step of creating a new project. This initial setup lays the groundwork for the entire security architecture, defining the structural skeleton within which authentication mechanisms will be integrated. By creating a new project, developers establish the basic structure where authentication middleware and token management systems will be implemented. This process proves beneficial by providing a clean slate to build security layers upon, allowing for efficient organization and scalability of security features in the Web API.
Configuring Startup.cs
Configuration of the Startup.cs file plays a critical role in shaping the behavior of ASP.NET Core 3.1 Web API projects. By configuring Startup.cs, developers can define how the application handles incoming requests, including those related to authentication and authorization. This setup determines the sequence of middleware components, such as authentication middleware, which intercept and process HTTP requests. The pivotal advantage of configuring Startup.cs lies in its ability to centralize the setup logic, simplifying the management of authentication-related configurations across the entire Web API. While this approach streamlines the initialization process, it also demands careful attention to detail to ensure that the configured settings align with the intended security objectives.
Integrating Bearer Token Authentication
Installing Required Packages
One of the fundamental aspects of integrating Bearer Token authentication is the installation of required packages. These packages contain pre-built functionalities that streamline the implementation process, offering ready-to-use components for token generation, validation, and management. By installing the necessary packages, developers can expedite the integration of authentication mechanisms into the ASP.NET Core 3.1 Web API, reducing development time and effort. The advantage of leveraging packages for authentication lies in their robustness and compatibility with the ASP.NET Core framework, ensuring seamless integration and interoperability with other security features.
Configuring Authentication Middleware
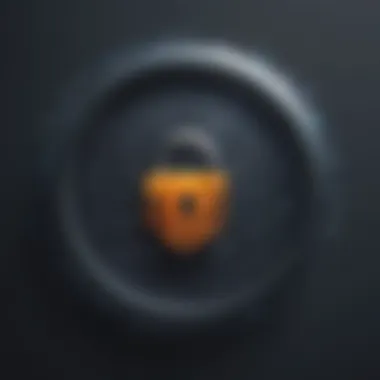
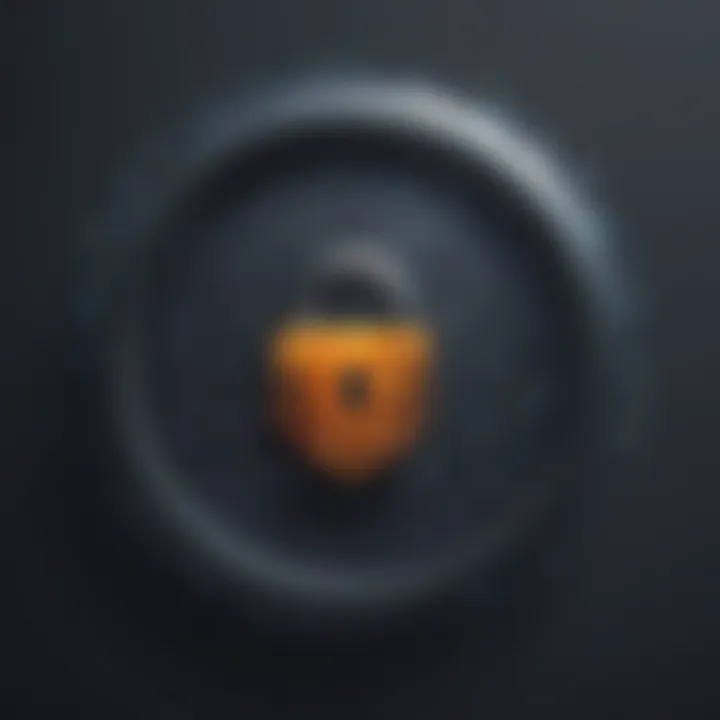
Configuring Authentication Middleware involves setting up middleware components that are responsible for authenticating incoming requests based on bearer tokens. This configuration dictates how the Web API will verify the validity of tokens and authorize access to protected resources. The key characteristic of this process is the establishment of a secure communication channel between clients and the API server, where token-based authentication adds an additional layer of security to the data exchange. By configuring Authentication Middleware effectively, developers can enhance the overall security posture of the Web API, safeguarding sensitive information and ensuring proper access control.
Generating and Validating Tokens
Token Generation Process
The token generation process is a pivotal aspect of Bearer Token Authentication, where tokens are created to authenticate and authorize client requests. This process involves the creation of unique tokens that hold encrypted information about the client's identity and permissions. The key characteristic of token generation lies in its ability to produce cryptographically secure tokens that are resistant to tampering and forgery, ensuring the integrity of the authentication process. By implementing a robust token generation process, developers can establish a secure authentication mechanism that verifies the authenticity of clients and prevents unauthorized access to protected resources.
Token Validation Mechanisms
Token validation mechanisms form the backbone of Bearer Token Authentication, verifying the authenticity and integrity of tokens presented by clients. These mechanisms validate the digital signatures of tokens, decrypt their contents, and perform validity checks to ensure that the tokens are genuine and unaltered. The unique feature of token validation lies in its ability to detect forged or expired tokens, preventing security breaches and unauthorized access attempts. By implementing rigorous token validation mechanisms, developers can fortify the security of their ASP.NET Core 3.1 Web API, mitigating the risks associated with unauthorized access and data breaches.
Best Practices
Securing Token Transmission
Utilizing HTTPS for Secure Communication:
Within the context of Bearer Token Authentication, the utilization of HTTPS emerges as a fundamental aspect of ensuring secure communication channels. HTTPS, Hypertext Transfer Protocol Secure, encrypts data during transit, safeguarding it from unauthorized access or tampering. By encrypting the information exchanged between the client and server, HTTPS thwarts malicious interception attempts, thereby upholding data integrity and confidentiality. Its integration within the authentication process bolsters the overall security posture of the application, instilling trust among users and mitigating the risks associated with data breaches.
Avoiding Token Exposure in URLs:
The practice of avoiding token exposure in URLs serves as a critical measure to prevent sensitive information from being vulnerable to exploitation. When tokens are included in URLs, they are susceptible to being logged in various servers, proxies, or browsers, jeopardizing their confidentiality. By refraining from exposing tokens in URLs, developers can mitigate the risks of token leakage and unauthorized access. This practice augments the security landscape of the application, ensuring that sensitive authentication data remains protected from prying eyes and potential security threats.
Token Expiration and Renewal
Setting Token Expiry Periods:
Setting token expiry periods is a strategic approach to enhancing security and minimizing the window of vulnerability within the authentication process. By defining specific time limits for token validity, developers can restrict the timeframe during which a token remains active. This not only reduces the likelihood of unauthorized access but also ensures that outdated tokens cannot be exploited by malicious actors. Setting appropriate expiry periods aligns with the principle of least privilege, allowing users access only for the required duration, enhancing overall security posture.
Issuing Refresh Tokens:
The issuance of refresh tokens presents a mechanism to facilitate seamless and secure token renewal processes. Refresh tokens, distinct from access tokens, are used to obtain new access tokens without requiring the user to re-enter their credentials. This approach minimizes the exposure of access tokens, reducing the potential impact of unauthorized token access. By incorporating refresh tokens into the authentication flow, developers can streamline the token renewal process while maintaining robust security measures.
Role-Based Access Control
Defining User Roles and Permissions:
The establishment of user roles and permissions forms the bedrock of role-based access control, empowering developers to organize and manage user privileges efficiently. By categorizing users into specific roles with predefined permissions, access control becomes more granular and tailored to the requirements of the application. Defining user roles allows for the enforcement of least privilege principles, granting users access only to resources essential for their tasks. This targeted approach enhances security by limiting unauthorized access attempts and potential breaches.
Implementing Role Checks in Controllers:
The implementation of role checks in controllers reinforces the role-based access control mechanism by validating user permissions at specific endpoints. By conducting role checks before executing controller actions, developers can ensure that users possess the requisite privileges to perform certain operations. This granular verification enhances the security framework of the application, preventing unauthorized access attempts and maintaining data confidentiality. Role checks in controllers serve as a proactive measure to uphold security standards and enforce access control policies effectively.