Integrating Redis with C#: A Comprehensive Guide
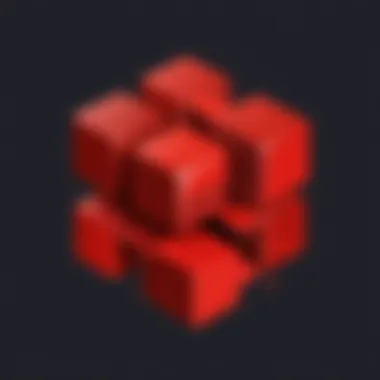
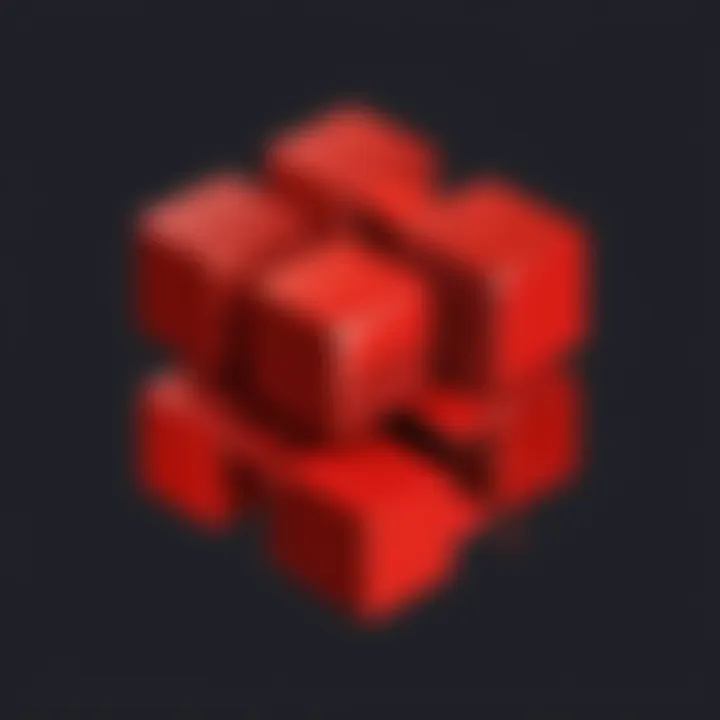
Intro
In the ever-evolving landscape of software development, a solid understanding of data management is essential. As applications grow in complexity and data volume, the need for efficient, scalable solutions becomes apparent. Redis has emerged as a powerful tool, especially suited for developers working within the C# environment of the .NET framework. Its unique characteristics make it an alluring option when developing high-performance applications.
Overview of Redis and
Integration
Redis is an in-memory data structure store that not only supports various types of data but operates with impressive speed. In contrast, C# is a versatile programming language adept at crafting a wide range of applications, from desktop to web-based. Together, they form a synergy that enhances performance, making them a favorite among developers.
Definition and Importance of Redis
Redis, fundamentally a key-value store, excels in scenarios that require rapid access and manipulation of data. Its importance lies in its ability to handle massive data loads effortlessly, particularly in real-time applications such as gaming leaderboards, chat systems, and even caching mechanisms. By maintaining data primarily in memory, rather than on disk, Redis ensures that read and write operations occur without delay.
Key Features and Functionalities
Redis comes packed with features that set it apart from traditional databases:
- Data Structures: Supports strings, lists, sets, hashes, sorted sets, and more.
- Persistence Options: Offers snapshotting and append-only file mechanisms to safeguard data.
- Pub/Sub Messaging: Supports publish/subscribe patterns for real-time message broadcasting.
- Atomic Operations: Enables complex transactions that can be executed atomically.
Use Cases and Benefits
The integration of Redis with C# opens a plethora of opportunities for developers. Some notable use cases include:
- Caching: Speeding up data retrieval processes, particularly for frequently accessed data.
- Real-Time Analytics: Processing and analyzing data streams as they occur.
- Session Management: Storing user sessions for web applications efficiently.
The benefits of using Redis in a C# context are tangible. With faster access times and advanced data handling, applications become more responsive, improving user satisfaction.
Best Practices
When working with Redis in conjunction with C#, adhering to best practices helps in harnessing its full power. Here are some pertinent points to keep in mind:
Industry Best Practices for Implementing Redis
- Use Connection Pooling: Efficiently manage connections to avoid excessive resource consumption.
- Cache Wisely: Only store data that is frequently accessed. This will help optimize memory usage.
- Track Performance: Use monitoring tools to analyze query performance and make adjustments as needed.
Tips for Maximizing Efficiency and Productivity
- Keep Data Structures Simple: Complex data structures can lead to increased latency. Choose the right type for your data.
- Limit TTL Usage: Setting a time-to-live on cached data can help manage memory, but set these judiciously to prevent data loss.
Common Pitfalls to Avoid
- Ignoring Persistence Settings: Relying solely on in-memory storage without proper persistence configurations can lead to data loss.
- Inadequate Testing: Always validate your Redis setup under various workloads to ensure it scales properly under loads.
Case Studies
Looking at real-world applications provides insight into the practical benefits of integrating Redis with C#. Here are notable examples:
Successful Implementations
Online Gaming Platforms: Many gaming platforms have integrated Redis to manage in-game data, such as player stats and leaderboards. This ensures players experience minimal lag during gameplay.
E-Commerce Applications: Online stores utilize Redis for managing shopping carts. This allows for faster retrieval of user sessions, enhancing the shopping experience.
Lessons Learned and Insights from Experts
Industry leaders emphasize the necessity of proper data modeling when working with Redis. The way data is structured profoundly impacts application performance.
Latest Trends and Updates
As technology rapidly evolves, staying abreast of current trends is vital. Here are some of the latest developments in the integration of Redis and C#:
Upcoming Advancements
The Redis community is continuously improving its core functionalities. The inclusion of multi-threading capabilities is on the horizon, which could yield higher throughput.
Current Industry Trends
The growing demand for microservices architecture has led to a surge in Redis usage, where it acts as a dependable data store across various services.
Innovations and Breakthroughs
There are ongoing exploration efforts into combining Redis with machine learning frameworks, paving the way for more intelligent data handling solutions.
How-To Guides and Tutorials
To effectively utilize Redis with C#, a hands-on approach can be invaluable. Here’s a brief overview of some essential guides:
Step-by-Step Guides
- Setting Up Redis: Detailed steps to install Redis on various platforms and configure it for C# applications.
- Creating a Simple C# Application: A guide on connecting a C app to Redis, including code snippets for common operations.
Practical Tips
- Use the StackExchange.Redis Library: This library simplifies the integration process and offers extensive functionality.
- Experiment with Redis Data Types: Proactively try out different data structures to see what works best for your specific use case.
In summary, learning to integrate Redis with C# not only boosts application performance but also equips developers with an arsenal of tools to tackle modern data challenges effectively. As you navigate through this guide, the knowledge gained will broaden your horizons in software development.
Foreword to Redis and
In the fast-evolving world of software development, knowing how to efficiently manage and manipulate data is crucial. That's where Redis, a high-performance in-memory data structure store, comes into play. Combining it with C#, a versatile language in the .NET framework, can create powerful applications that handle data with ease. This section will lay the groundwork for understanding how Redis complements C# and the avenues it opens for developers.
Defining Redis
At its core, Redis is more than just a database; it’s an in-memory key-value store that provides extensive data structure support. This means you can not only store simple strings but also hashes, lists, sets, and so on. Why does this matter? Well, when speed is of the essence, and you require rapid data access, Redis is a natural choice. It enables microseconds-long data retrieval, making it suitable for applications that demand speed and efficiency.
Consider scenarios such as real-time analytics or caching frequently accessed data. In these cases, the ability to manage data quickly transforms the user experience and the overall performance of applications. Redis, with its pub/sub messaging system, can also handle real-time updates effortlessly, contributing to interactive interfaces without the lag.
Overview of
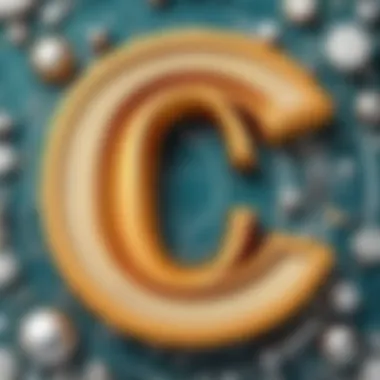
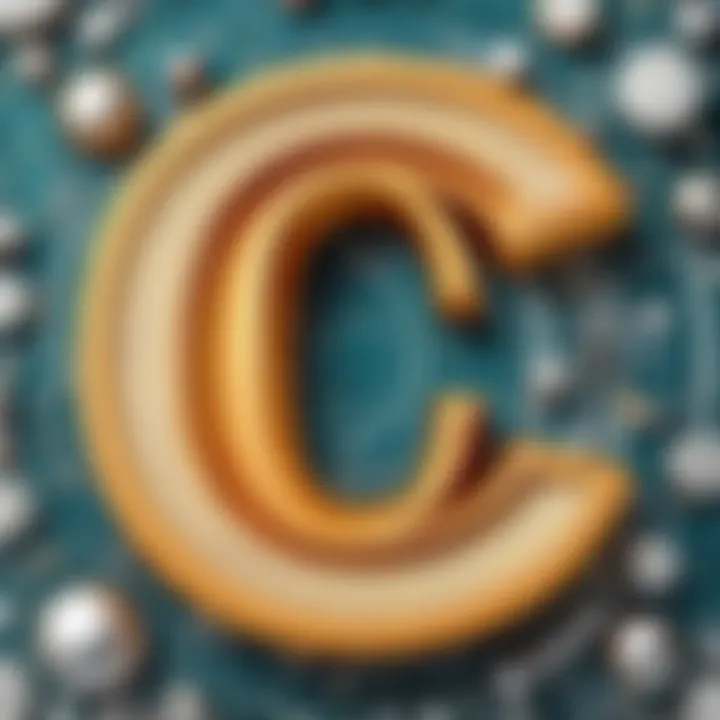
Moving on to C#, it stands firmly in the realm of programming languages designed for building a range of applications on the .NET platform. Its syntax is straightforward, making it accessible; however, its capabilities run deep. C# supports object-oriented programming, which encourages code reusability and maintainability.
The seamless integration of C# with powerful tools and frameworks allows developers to create robust applications across various domains—from web services to desktop applications and even mobile platforms. When one thinks of C#, the idea of building scalable applications often comes to mind. This capability, especially when paired with Redis, opens doors to designing efficient systems that manage large datasets without breaking a sweat.
In essence, the interplay of C# and Redis creates a formidable duo, tailoring software solutions that are both responsive and capable of handling complex data interactions. It's about leveraging the best features of both technologies to enhance application performance and deliver a richer user experience.
"Combining the speed of Redis and the flexibility of C# can lead to innovations that set new standards in application performance."
In summary, grasping both Redis and C# lays a solid foundation for developers looking to build high-performance applications in a competitive landscape. As we delve deeper into how these technologies integrate, we will explore the framework for setting them up and the practicalities of working with this dynamic combo.
The Synergy of Redis and
When it comes to modern application development, the need for speed and efficiency cannot be overstated, particularly for data-intensive applications. Here, Redis shines as a go-to solution. When coupled with C#, known for its versatility within the .NET ecosystem, this combination provides a formidable approach to handle various data challenges. This section will discuss the significant benefits and considerations of integrating two powerful technologies: Redis and C#.
Merging Redis with C# allows developers to harness both an in-memory key-value store capable of lightning-fast data retrieval and a mature programming language that supports complex application structures. This synergy is not just about speed; it’s also about the capability to build scalable and resilient applications. Here’s a closer look at some of the specific elements that make this integration appealing:
- Performance: Redis operates primarily in memory, offering much quicker read/write times compared to traditional databases that rely heavily on disk storage. This can dramatically enhance the performance of C# applications, especially those that require real-time data access.
- Scalability: As user demands grow, ensuring that your application can scale is crucial. Redis can handle high volumes of transactions seamlessly, which aligns perfectly with C#’s capability to manage one or multiple threads effectively.
- Flexibility: Redis supports various data structures, including strings, lists, and hashes, providing C# developers with the tools to implement complex data hierarchies without extensive overhead. Developers can manipulate these structures effectively to suit application needs.
Understanding these benefits illustrates why many developers turn to Redis when building applications in C#. Now, let’s explore some compelling reasons to choose Redis for your C# applications.
Why Choose Redis for
Applications?
Turning to Redis for C# applications brings forth some distinctive advantages:
- Speed and Efficiency: As mentioned, Redis is blazing fast. For C# applications that depend on rapid data access—like gaming or live analytics—Redis provides a performance edge.
- Ease of Use: Redis's simple API integrates well with C#, leading to a smoother development experience. Most C# developers can hit the ground running without cumbersome setup processes.
- Community and Support: Both Redis and C# have extensive community support. There are numerous resources available for troubleshooting or enhancing your integration. This community can provide valuable insights and updates on best practices, making it easier to stay current.
- Persistence Options: While Redis is primarily an in-memory store, it offers various persistence options. With features like RDB snapshots and AOF (Append Only File), data can be safely stored, minimizing risks of loss.
"Choosing Redis for your C# applications means investing in speed, flexibility, and a strong support network—a trifecta that today’s developers crave."
These reasons illustrate why combining Redis with C# isn’t just a trend, but a strategic consideration for building high-performance applications. Let’s dig into some common use cases of Redis in the context of C#.
Common Use Cases
Understanding how to implement technologies effectively requires practical examples to draw insights from. Integrating Redis with C# can serve several purposes across various industries and applications. Here are some of the most common use cases:
- Session Management: In web applications, managing user sessions efficiently is critical. Redis can store user session data temporarily, allowing quick access to user states, which enhances user experience.
- Real-Time Analytics: Applications requiring real-time data processing—think web traffic monitoring or social media analysis—thrive with Redis due to its speed and ability to handle large datasets.
- Caching Mechanism: Often, the answer doesn’t need to be pulled from the database every time. By caching database queries in Redis, developers can vastly improve application response times.
- Leaderboards or Scoring Systems: In gaming or competitive applications, Redis allows for real-time updates to leaderboards, providing a seamless experience for users.
Setting Up Redis with
Setting up Redis with C# is a crucial step that lays the foundation for effective data management within your applications. The importance of this process cannot be overstated, as it serves as the gateway to the myriad features and performance gains Redis offers. When we talk about setting up Redis, it’s not merely about installing Redis server or libraries; it involves understanding how these components will interact within the .NET framework, ensuring optimized data handling, and anticipating future scalability needs.
By the end of this section, readers will grasp not just the procedural steps involved, but also the underlying benefits of seamless integration. This knowledge becomes vital when deciding how to structure your data and optimize access patterns, which are crucial aspects in developing modern, responsive applications.
Installing Redis Server
To begin the integration process, the first step is installing the Redis server. Redis operates as a standalone server, and its quick installation is one of the many reasons developers favor it for quick data access. Depending on your operating system—Windows, Linux, or macOS—the installation process may differ slightly.
- Windows: Although Redis was initially designed for Linux, it is possible to run it on Windows via the Windows Subsystem for Linux (WSL).
- Linux and macOS: Both have native support for Redis, making installation straightforward.
- Start the Redis service once installed:
- Enable WSL in your Windows features settings.
- Download a Linux distribution like Ubuntu from the Microsoft Store.
- Open Ubuntu and run and .
- Start the server using command.
- For Ubuntu, use:
- For macOS, utilizing Homebrew simplifies the process:
- for Linux.
- For macOS, you could start it with:
Setting up a Redis server is generally uncomplicated, but once you venture into configuration, it’s essential to ensure the specifics align with your project’s needs, like memory allocation and persistence settings.
Installing Required Libraries in
With your Redis server in place, the next pivotal step involves installing the necessary libraries in C#. One of the most popular libraries for C# is . It's a robust, efficient client that not only simplifies communication with Redis but also supports advanced features like connection pooling and pub/sub messaging.
- Using NuGet Package Manager:
- Alternatively, use Package Manager Console:
- Verify the installation by checking your project references to confirm appears properly.
- Open your Visual Studio project.
- Go to Tools -> NuGet Package Manager -> Manage NuGet Packages for Solution.
- Search for .
- Click on Install.
- Go to Tools -> NuGet Package Manager -> Package Manager Console.
- Execute:
Equipped with the Redis server running and the necessary libraries installed, you're now strategically positioned to develop applications that can leverage the high-speed capabilities of Redis. This setup ensures efficient data handling and creates a strong backbone for your C# applications' needs.
Connecting
to Redis
Connecting C# to Redis is a crucial step for developers looking to leverage the power of in-memory data storage for their applications. Redis stands out for its speed and efficiency, which is especially beneficial in scenarios requiring rapid data retrieval and manipulation, such as real-time analytics or session management. Integrating C with Redis not only enhances application performance but also simplifies data handling.
With powerful libraries available for .NET, the connection process becomes straightforward, making it more accessible to developers regardless of their expertise level. The main advantage here is that rather than dealing with complex protocols, C# developers can use simple method calls to interact with their Redis instance. Thus, understanding how to establish this connection is fundamental for any developer aiming to build scalable and high-performance applications.
Establishing a Connection
To begin, you need to ensure that both Redis and your C# application are set up correctly. Once you have confirmed that Redis is running, you can use libraries like StackExchange.Redis to create a connection. Here's a brief rundown on how to establish a connection to your Redis server:
- Install the Redis client library: In Visual Studio, you can easily add the StackExchange.Redis package via NuGet. This library provides everything you need to communicate with Redis in an efficient manner.
- Create a ConnectionMultiplexer instance: This is the core class used to manage connections. You can instantiate it in your code like this:Here, "localhost:6379" refers to the default address where Redis is typically hosted. Adjust as needed if you have Redis on a different server or port.
- Access Redis databases: Once you have the connection established, you can access different databases within Redis. For instance:This line pulls in the default database for you to start working with.
Establishing a connection may seem simple, but it's the first and most important step in using Redis effectively.
Connection Parameters and Configuration
When connecting C# to Redis, you can customize your connection through various parameters. Fine-tuning these can make a significant difference in performance and reliability. Some of the notable parameters include:
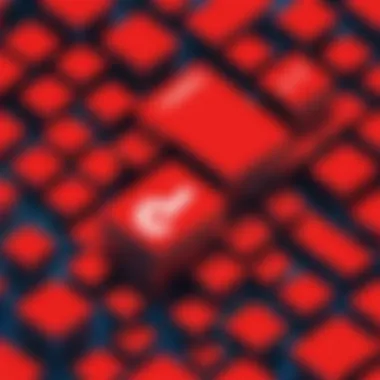
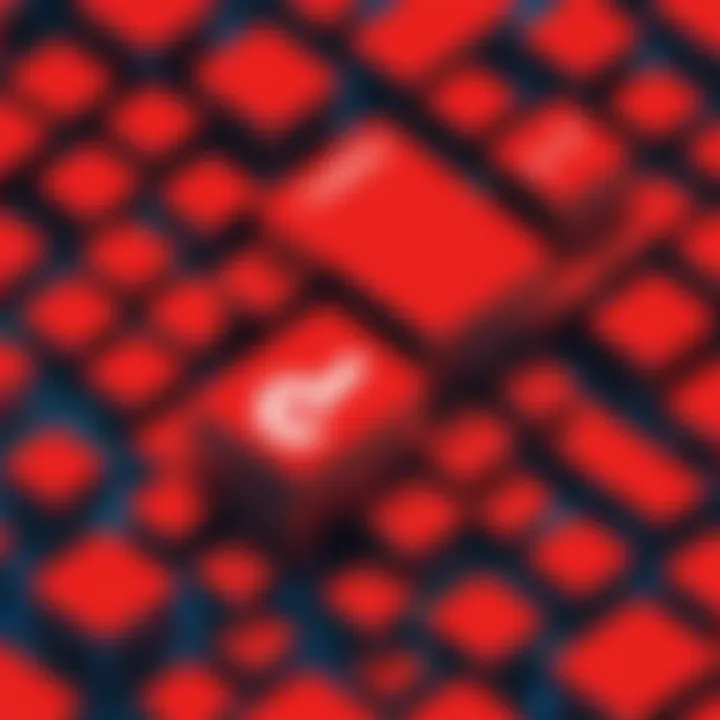
- Timeout: This sets the time limit for establishing a connection. If your connection attempt exceeds this time, it will fail. For example:
- Password: If your Redis instance is password-protected, include this parameter in your connection string to authenticate successfully.
- SSL: For enhanced security, you can enable SSL, particularly if Redis is accessed over the internet.
Choosing the right configuration settings can minimize connection issues and ensure a smoother interaction with Redis. In the world of high-performance applications, making these considerations can save you grief down the line and ensure your app runs like a well-oiled machine.
"A properly configured connection is the foundation of smooth data operations with Redis."
In sum, the process of connecting C# to Redis is clear-cut once you understand the basic structure. Establishing the connection, along with tweaking parameters to fit your needs, sets the stage for the data manipulation you'll conduct further on.
Data Manipulation with Redis in
Data manipulation is at the heart of any application, and when it comes to integrating Redis with C#, this topic takes on particular significance. In this segment, we will delve into how Redis enables efficient data operations while highlighting specific benefits, considerations, and what differentiates Redis from traditional databases.
Working with Redis means dealing with in-memory data structures that are designed for quick access and manipulation. This capability is crucial for developers who are looking to enhance application performance and user experience. From creating new data to deleting existing entries, understanding the nuances of data manipulation can significantly affect your application's structure and efficiency.
The use of Redis allows developers to adopt a more agile approach to handling data. Here are some essential benefits:
- Speed and Efficiency: Because Redis operates primarily in memory, it provides data retrieval and storage at lightning speed, making it ideal for high-performance applications.
- Flexibility: Redis supports various data types, including strings, hashes, lists, and sets. This variety enables developers to choose the most suitable type for their specific needs.
- Scalability: Redis can handle large volumes of data, which is essential in dynamic environments where data is continually being created and updated.
In the following subsections, we will break down the specific operations that can be performed with Redis in C#. Let’s explore how CRUD (Create, Read, Update, Delete) operations come into play in this integration.
Advanced Data Structures in Redis
In the realm of data storage and manipulation, Redis stands out not just as a simple key-value store, but as a versatile database that supports several advanced data structures. Understanding these structures is crucial for developers who want their applications to be both performant and scalable. This section aims to shed light on why these advanced data structures matter and how they can be effectively leveraged in C# applications.
Understanding Redis Data Types
Redis possesses a rich palette of data types, and these are not merely for variety's sake. Each type serves a distinct purpose and offers unique advantages depending on the application requirements. Here’s a brief overview of the primary Redis data types:
- Strings: The most basic type, useful when you need to store textual information or binary data.
- Hashes: Ideal for storing objects and related information under a common key, allowing for easy retrieval of data fields without needing to unpack an entire object.
- Lists: These are collections of ordered elements which can be great for maintaining ordered feeds or playlists.
- Sets: Unordered collections that prevent duplications, perfect for managing unique items or tags.
- Sorted Sets: Similar to sets but with associated scores, enabling quick retrieval and ranking functionalities.
Each type has its strengths and knowing when to use each can enhance performance dramatically. For instance, if you're building a social media application, utilizing Sets for unique user tags can simplify many operations compared to traditional relational databases. This flexibility is a powerful ally for C# developers who want to build complex data models with ease.
Using Hashes, Lists, and Sets
Hashes, Lists, and Sets are amongst the most commonly employed data structures in Redis. Their applications can be numerous, and understanding their functionality can significantly optimize how data is handled within your C# applications.
Hashes
Hashes in Redis work like dictionaries, storing key-value pairs. This is particularly useful for saving objects where you want to access attributes individually without dealing with the entire object. For example:
In the above code, the user object is stored in a Redis hash. Each field can be updated independently, which leads to less data modification overhead.
Lists
Lists are great for maintaining an ordered collection of items. They can be used for tasks such as managing playlists:
This snippet adds songs to a playlist efficiently. With lists, you can add, remove, or access elements based on their order, creating a dynamic setup for real-time applications.
Sets
Redis Sets are perfect for storing unique collections, like user tags. For example:
This approach guarantees that each tag is unique to the user. Retrieving tags or performing operations like intersections and unions with other sets can be incredibly fast, thanks to how Redis optimizes set operations.
"The right tools in the data management toolbox can enhance performance and reduce complexity. Redis’s advanced data structures empower C# developers to create efficient and scalable applications."
By skilfully integrating these advanced data structures into your C# projects, you can not only handle data effectively but also create robust solutions capable of meeting modern application demands. The interplay between Redis's capabilities and C#’s functionality can lead to impressive, data-driven applications that are both reliable and easy to maintain.
Error Handling and Performance Optimization
Error handling and performance optimization are critical components in the integration of Redis with C#. Both aspects ensure that applications are not only robust but also efficient in managing data. Without proper error handling, an application can misbehave in unpredictable ways—sometimes leading to a complete data loss or corruption. Meanwhile, performance optimization can be the cherry on top, ensuring the application runs snappily without hogging resources.
Common Errors and Solutions
When using Redis with C#, various errors may surface depending on the application complexity and Redis interactions. Here are some of the common pitfalls along with solutions:
- Connection Issues: Often, developers grapple with connection timeouts or failures. This can be due to incorrect configuration or network setbacks. The solution is to thoroughly check the connection strings and parameters.
- Data Serialization Errors: Redis expects data in a certain format. When sending complex objects, you might encounter serialization issues. To deal with this, ensure you use appropriate serializers like JSON.NET or ServiceStack. Adapt your model to simpler structures where needed.
- Command Misuse: Sometimes, commands may be used incorrectly, leading to runtime exceptions. Stay updated with Redis command documentation and validate command usage within the application.
- Memory Issues: If not monitored properly, Redis can fill up server memory leading to performance degradation. Always configure appropriate memory limits and eviction policies in your Redis settings to manage this.
"Error handling is like a safety net for developers, catching the unforeseen and enabling better user experiences."
When these errors crop up, having fallback strategies and error handling mechanisms like logging allows developers to address and rectify issues before they can cause significant damage.
Strategies for Optimizing Performance
Performance can make or break an application. Slow data retrieval or updates can lead to frustrated users and missed opportunities. Below are some effective strategies:
- Use Pipelines: By batching commands, you send multiple commands to Redis at once rather than one at a time. This drastically reduces the round-trip time. For example:
- Choose the Right Data Types: Redis offers various data structures—only use what fits your needs best. For instance, when needing a leaderboard, leverage Sorted Sets rather than Lists or Hashes.
- Minimize Network Latency: Running Redis and the application server in close proximity can lower network delays. Deploying Redis in the same region or virtual network as the application can help mitigate latency issues.
- Configure Redis for Your Workload: Understand the expected workload of your application—whether it’s read-heavy or write-heavy. Adjust Redis configurations like maxmemory and maxclients accordingly.
- Use Caching Wisely: Implement cache expiry strategies to prevent memory leakage. Leveraging Redis as a cache can offload your primary database and improve performance significantly.
By integrating these strategies correctly, developers can better harness the capabilities of Redis while using C#. This not only enhances performance but also sets the foundation for a smoother user experience.
Testing and Debugging
Testing and debugging are pivotal not just in any software development journey, but specifically when integrating Redis with a C# application. The quicker you can catch errors or oversights, the smoother your development cycle becomes. Working with Redis, a fast and efficient in-memory data store, offers its advantages, but it also introduces unique challenges that need addressing. If not tackled properly, these challenges can lead to performance bottlenecks or, worse, data inconsistencies. Testing allows developers to ensure that everything operates as intended before hitting the deployment button. Likewise, debugging helps to identify the roots of issues when things don’t work out as planned.
The benefits of solid testing and debugging practices include:
- Early Detection of Issues: By running tests throughout the development process, potential errors can be caught early; this saves both time and resources.
- Improved Code Quality: A robust testing strategy ensures higher quality code, which streamlines future modifications.
- Reduced Risk of Data Loss: Considering Redis is often used for critical data operations, ensuring its integration is flawless minimizes the risk of losing important data.
While working with Redis and C#, several factors should be taken into account, like connection latency and data consistency. These can hugely impact the efficacy of both your testing and debugging processes.
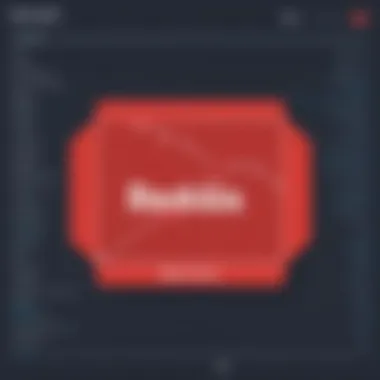
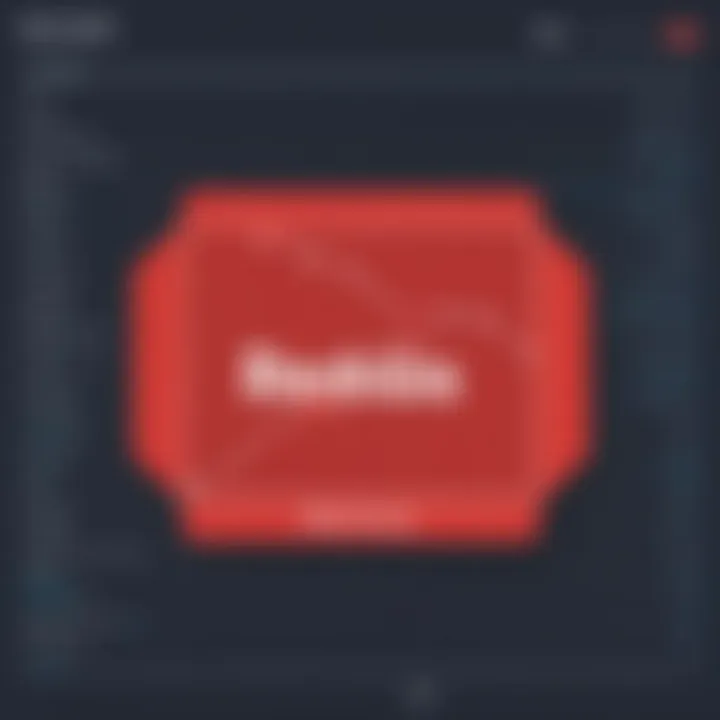
Important Testing Frameworks
When you venture into testing C# applications that integrate with Redis, certain frameworks stand out for their functionality and ease of use. Here’s a closer look at some essential frameworks that developers often employ:
- xUnit: Highly extensible and easy to use, xUnit is a preferred choice for many in the .NET community. It’s not just about writing tests but also about writing them efficiently with a rich set of features.
- NUnit: A solid, well-documented framework that offers extensive support for unit testing. NUnit is known for allowing you to organize your test cases well, making it easy to manage.
- MSTest: This tool is integrated into Visual Studio, simplifying the testing process for C# developers. Its seamless integration allows you to write, run, and manage tests easily within the familiar Visual Studio environment.
- FluentAssertions: While not a testing framework in itself, FluentAssertions enhances the readability of tests written with frameworks like NUnit and xUnit. It offers a more expressive way to assert conditions.
Selecting the right framework can have a direct impact on the ease of your testing processes. Make sure to evaluate the specific needs of your project before choosing your tools.
Best Practices in Debugging
Debugging is an art in itself. Knowing how to tackle issues when they arise can greatly influence overall application performance. Here are some recommended best practices:
- Use Meaningful Logging: Implement logging at strategic points within your application. This helps in tracing issues once they occur without unnecessarily cluttering the output.
- Test Different Scenarios: Don’t just focus on the happy path. Explore edge cases and testing under various conditions to uncover hidden bugs.
- Break Down Problems: When something goes wrong, it helps to isolate the problematic section. This can involve commenting out parts of code to pinpoint where things are going awry.
- Use Debugging Tools: C# provides debugging tools in Visual Studio that can tremendously simplify your debugging tasks. Make use of breakpoints and step-through debugging to observe the live application state.
- Document Findings: Keeping notes of common issues and their resolutions can serve later as a valuable guide for both yourself and your team.
"An ounce of prevention is worth a pound of cure."
In the realm of software integration, this adage rings especially true. Approaching testing and debugging methodologies with clarity and intention can set up a solid foundation for seamless Redis integration with C#.
Taking the time to lay down good routines in testing and debugging pays off in spades, allowing you to concentrate on what truly matters: enhancing the performance and functionality of your applications.
Real-World Applications and Case Studies
Understanding how Redis integrates with C# takes on a new layer when we consider real-world applications. This section seeks to illustrate the practical applications of Redis within diverse industries, as well as highlighting specific case studies showcasing successful integrations. Real-world examples help ground the theoretical concepts and show developers, IT professionals, and tech enthusiasts how to truly harness the capabilities of Redis and C#.
In today's fast-paced digital landscape, quick access to data can be the difference between success and failure for an application. Redis contributes to this speed, making it a favorite for developers aiming to optimize performance.
Industry Use Cases
Redis finds its way into various sectors, each with distinct requirements and outcomes. Below are some notable examples:
- E-commerce Websites: Online retailers often deal with a massive influx of user data, especially during sales or special promotions. Using Redis for caching product details and user sessions can significantly reduce server load and improve response times.
- Social Media Platforms: With a high volume of interactions, social media sites benefit from Redis’s ability to manage session states and real-time messaging features. Messages or content can be stored in Redis quickly, manipulating user interactions seamlessly.
- Game Development: Multiplayer games employ Redis to manage user sessions, leaderboard scores, and game state data. This allows for real-time updates and enhances the gaming experience by reducing lag.
- Financial Services: In environments where every millisecond counts, financial applications utilize Redis for transaction processing, market data analysis, and alerting systems, ensuring performance meets strict industry regulations.
By leveraging Redis in these contexts, developers can create applications that are not only fast but also capable of handling substantial amounts of data effortlessly.
Case Study: Successful Integration
To illustrate the impact of Redis in a practical setting, let’s look at an integration undertaken by TechIdeas Inc., a fictitious software development company that created an online learning platform for coding tutorials. Initially, the platform relied on traditional relational databases. As user traffic surged, response savings weren't acceptable, and the user experience suffered.
To tackle these challenges, TechIdeas Inc. chose to integrate Redis with their C# application. The following steps helped streamline the process:
- Caching Mechanism: They implemented Redis as a caching layer to store frequently accessed data such as course material and user profiles.
- Session Management: Instead of using database sessions, they utilized Redis to handle user sessions, reducing the burden on their SQL database.
- Real-Time Data: By leveraging Redis' Pub/Sub feature, they created a system for real-time notifications about course updates, keeping users engaged and informed.
As a result, page load times improved significantly; users reported an average increase in speed of nearly 200%. Moreover, user engagement metrics showed a marked uptick, as users could now access content almost instantaneously. Thus, their application transitioned into a scalable and efficient system.
End and Future Directions
In wrapping up our exploration on integrating Redis with C#, it’s paramount to acknowledge the significant role this union plays in the modern development landscape. Both Redis and C# are powerful tools in their own right, and together, they elevate application performance, making it a smooth ride for developers aiming for efficiency and scalability.
Summary of Key Points
Throughout this guide, we have uncovered some key elements to keep in mind when considering the integration of Redis with C#:
- Performance: Redis acts as an in-memory data structure store that markedly accelerates data retrieval and improves server response time. This is vital for applications requiring swift data access.
- Ease of Use: C# developers can easily adopt Redis for its straightforwardness. With numerous libraries available, connecting and manipulating Redis data becomes a breeze.
- Data Management Flexibility: From caching to session storage, Redis offers flexibility through its diverse data structures, including lists, sets, and hashes, allowing developers to tailor their data handling strategies effectively.
- Robust Community Support: Both Redis and C# boast strong community backing, which means developers can frequently find solutions to issues or enhancements through forums like Reddit or official documentation.
- Optimized Performance Strategies: Utilizing the right patterns for managing connections and data allows for heightened performance, an essential consideration for large-scale applications.
Trends to Watch in Redis and
Integration
As technology continually evolves, certain trends are emerging in the integration of Redis with C# that are worth keeping an eye on:
- Increased Use of Microservices: As companies shift towards microservices architecture, Redis is becoming a go-to choice for managing transient data between microservices in a C# environment, facilitating seamless data sharing and caching.
- Growing Adoption of Serverless Architectures: Redis is being more frequently utilized in serverless computing frameworks, where quick access to data is crucial. This trend is particularly prominent in cloud-native applications.
- Focus on Real-Time Data Handling: Industries requiring real-time analytics are increasingly leveraging Redis with C# to deliver instant insights, triggering new opportunities for businesses across various sectors.
- Enhanced AI and Machine Learning: Using Redis as a backbone for AI applications developed in C# is gaining traction. Fast data retrieval aligns perfectly with the requirements of machine learning algorithms that rely on speed and efficiency.
To sum it up, if you are navigating the waters of application development with C#, leveraging Redis will not only enhance your application's performance but also keep you at the forefront of technological advancements. Keep your ear to the ground, as the future of this integration continues to unfold.
Resources for Further Learning
In the ever-evolving landscape of technology, staying informed and up-to-date is vital, especially when integrating tools like Redis with C#. Understanding Resources for Further Learning can provide developers and IT professionals with a solid foundation, helping them grasp concepts more deeply and apply them effectively. This section will navigate through significant resources that can support your journey in mastering this integration.
Rather than swimming in a sea of information, focusing on specific resources allows for a more organized approach to learning. These resources serve not only as reference points but also as avenues for continuous growth in knowledge and skills.
Books and Tutorials
Books and tutorials play a crucial role in the realm of programming. They offer structured content that’s often more digestible than scattered online material. When it comes to Redis and C#, several resources stand out:
- Redis in Action by Josiah L. Carlson – This book does a fantastic job of covering core principles and use cases of Redis, providing real-world examples.
- C# in a Nutshell by Joseph Albahari – While not specifically focused on Redis, this resource is invaluable for mastering C fundamentals.
- Online platforms such as Pluralsight and Udemy provide targeted courses that cover Redis, often including C# integration as part of their curriculum. They often feature hands-on projects which are essential for practical understanding.
By diving into these materials, developers can not only grasp how Redis operates within a C# environment but also obtain insights into best practices and optimization strategies. In turn, this will significantly enhance one's coding prowess and problem-solving skills.
Online Communities and Forums
Connecting with others in the field can be as crucial as self-study. Online communities and forums facilitate collaboration, idea exchange, and support systems. Here are some noteworthy spaces where developers gather to discuss Redis and C#:
- Reddit: The r/programming and r/dotnet subreddits are vibrant platforms where users frequently share insights, tips, and ask questions, providing a rich resource for ongoing discussion.
- Stack Overflow: For any queries regarding integration challenges, Stack Overflow is arguably the go-to site. Users can read previously solved issues or post their own, tapping into a wealth of collective knowledge.
- Facebook Groups: Groups like .NET Developers and Redis Users allow for targeted discussions and networking among peers, where you can share experiences and learn from successes and failures.
Engaging in these communities not only enhances learning but also builds connections that can lead to career opportunities.
Answers to Common Questionss on Redis with
In today's fast-paced tech world, developers often seek answers that help them navigate complexities. This section targets the Frequently Asked Questions on the integration of Redis with C#. Having clarity on these common issues can save time and reduce frustration.
Understanding the nuances of how Redis operates within a C# context allows for better project planning and execution. It sheds light on integration pitfalls, performance anxieties, and use-case particularities that developers frequently face, ensuring they can deploy Redis effectively.
Common Queries by Developers
When diving into Redis with C#, developers usually find themselves grappling with a plethora of questions. Here are some of the most common queries:
- How do I connect my C# application to Redis?
Establishing this connection is straightforward but can be tricky if configurations are not correctly set. Ensuring you have the right dependencies and connection strings makes all the difference. - What are the best library options for using Redis in C#?
The NuGet package is a widely-used library that offers comprehensive support for Redis commands. It's crucial to consider your project requirements before choosing a library. - Are there size limits for data stored in Redis?
Redis handles large volumes of data but does have limits based on memory. Being aware of these limits helps in planning your data structures appropriately. - What are typical performance issues when using Redis with C#?
Common problems include connection timeouts and serialization issues. Understanding connection pooling and the nuances of data serialization is essential for maintaining optimal performance. - How does Redis handle data persistence?
Redis can be configured for persistence using snapshots or journaling. This feature plays a vital role in data durability, and knowing how to best configure it is essential.
Clarifications on Usage Scenarios
Many developers are uncertain about the best contexts for utilizing Redis. Here’s a look at some scenarios where Redis excels:
- Real-time Analytics
Using Redis for real-time data analysis is advantageous due to its in-memory data structure, allowing for quick access and manipulation of data sets. It shines with high-velocity data streams. - Session Management
With its capability to store and manage user sessions efficiently, Redis acts as a great solution for web applications. It can store session data so that web servers can quickly recall user sessions without heavy database queries. - Leaderboards and Counting
Redis is perfect for gaming applications that need leaderboards or counters. Thanks to its sorted sets, it can easily track and update player scores in real-time. - Caching Layer
Implementing Redis as a caching layer can drastically improve application performance by storing frequently accessed data closer to the application, thus speeding up response times. - Pub/Sub Messaging
Redis enables a simple yet powerful pub/sub messaging pattern. Applications that require real-time messaging tasks can benefit significantly from this feature.
"Redis is not just a database; it's a tool for solving specific problems efficiently. Knowing when and how to use it can significantly boost your application's performance."
Arming oneself with these insights helps craft a robust development strategy. Recognizing these answers not only empowers developers but also reinforces confidence in utilizing Redis in their C# applications.