Unveiling the Synergy Between Node.js and JavaScript in Advanced Software Engineering
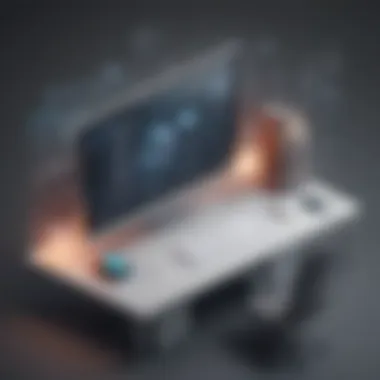
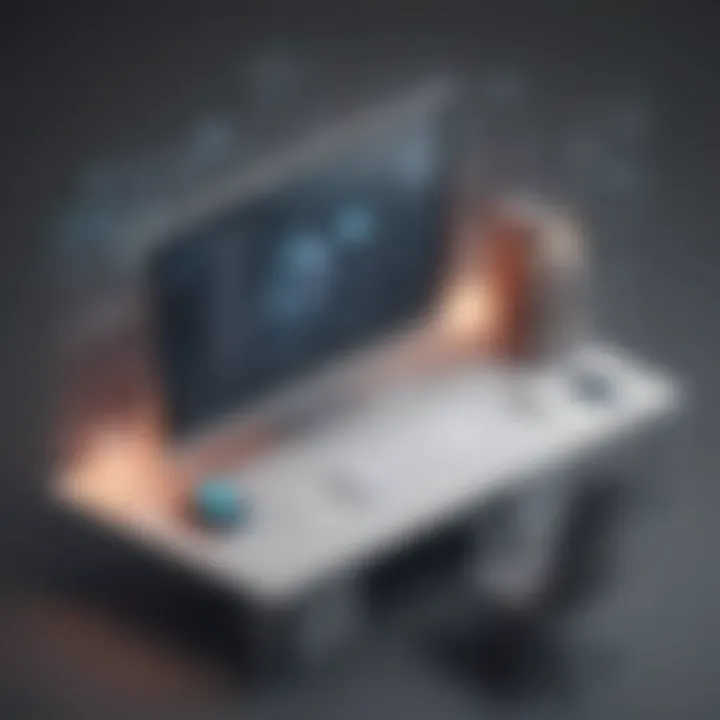
Overview of Node.js and JavaScript in Software Development
Node.js and JavaScript form a crucial symbiotic relationship in modern software development, offering developers a powerful duo of technologies to create robust applications. Node.js, built on the V8 JavaScript runtime engine, extends the functionality of JavaScript to the server-side, enabling efficient and scalable backend development. JavaScript, known for its versatility and ubiquity in web development, serves as the foundation for dynamic frontend interfaces. Understanding the intersection of Node.js and JavaScript is paramount for developers seeking to leverage their combined capabilities to build cutting-edge applications.
Best Practices
When working with Node.js and JavaScript, industry best practices recommend maintaining a modular code structure to promote reusability and maintainability. Leveraging npm packages and libraries optimizes development efficiency and enhances functionality. Continuous testing and integration practices ensure the reliability and stability of applications. To maximize productivity, developers should adhere to coding standards, utilize version control systems effectively, and prioritize documentation throughout the development process. Common pitfalls to avoid include neglecting security measures, not optimizing performance, and overlooking scalability requirements.
Case Studies
Real-world examples showcase the successful implementation of Node.js and JavaScript in various applications. For instance, companies like Netflix have utilized Node.js to enhance their streaming services, leveraging its non-blocking IO model for handling a high volume of traffic efficiently. Through the integration of JavaScript frameworks like React, Vue, or Angular, developers have achieved seamless user experiences across platforms. Industry experts emphasize the importance of optimizing performance, utilizing asynchronous programming techniques, and staying updated with the latest advancements in Node.js and JavaScript for competitive application development.
Latest Trends and Updates
The landscape of Node.js and JavaScript continues to evolve rapidly, with upcoming advancements focusing on enhancing performance, security, and developer experience. Current industry trends indicate a shift towards serverless architectures, microservices, and containerization for scalable and fault-tolerant applications. Innovations such as Deno, a secure runtime for JavaScript and TypeScript, and ES modules in Node.js signal a progressive direction in JavaScript ecosystem. Developers are adapting to emerging trends by exploring GraphQL for efficient data fetching, adopting progressive web app techniques for enhanced user experiences, and integrating WebAssembly for performance-critical operations.
How-To Guides and Tutorials
For beginners delving into Node.js and JavaScript development, step-by-step guides offer a comprehensive introduction to setting up the development environment, creating RESTful APIs, and deploying applications. Hands-on tutorials cover advanced topics such as using WebSocket communication, implementing authentication mechanisms, and optimizing application performance. Practical tips include debugging strategies, performance profiling techniques, and deployment best practices for ensuring a seamless development experience across the software development life cycle.
Introduction
In the realm of modern software development, the symbiotic relationship between Node.js and JavaScript plays a pivotal role in crafting innovative applications. This article embarks on a journey to unravel the intricacies of leveraging Node.js with JavaScript, shedding light on how these technologies intersect to build resilient and dynamic software solutions. By exploring the fusion of Node.js and JavaScript, a deeper understanding of their collaborative potential in driving development processes is unveiled. Let's delve into the heart of this synergy in software creation.
Understanding Node.js and JavaScript
Evolution of JavaScript
As we trace the evolutionary path of JavaScript, its transformation from a basic scripting language to a multifaceted programming language becomes evident. The growth of JavaScript from its humble origins to a versatile tool for web development underscores its significance in the software landscape. The agility of JavaScript in adapting to varying development needs and its widespread adoption in creating interactive web interfaces highlight its pivotal role in modern coding practices. Although met with challenges, such as browser compatibility issues, JavaScript's evolution has been marked by continuous enhancements, making it a compelling choice for developers seeking a dynamic and responsive development environment.
Role of Node.js in JavaScript Development
The role of Node.js in enhancing JavaScript development cannot be overstated, offering a robust, server-side platform for executing JavaScript code. Node.js empowers developers to leverage JavaScript beyond the confines of the browser, enabling server-side programming with unparalleled efficiency. Its event-driven architecture and non-blocking IO operations provide a performant environment for running JavaScript code, revolutionizing the way backend applications are constructed. Embracing Node.js in JavaScript development leads to a seamless synergy between frontend and backend components, streamlining the overall development process and enhancing code reusability.
Key Concepts
Delving into the key concepts underpinning Node.js and JavaScript integration unveils a plethora of fundamental principles that drive application development. From understanding event-driven programming to mastering asynchronous operations, developers are equipped with essential tools to navigate the intricacies of modern software architecture. Leveraging concepts such as callbacks and package management with npm, developers can optimize code efficiency and maintainability, fostering a culture of sustainable and scalable software solutions. The fusion of these key concepts with Node.js and JavaScript sets the stage for innovative development practices, laying the foundation for building cutting-edge applications that resonate with the demands of today's digital landscape.
Node.js Fundamentals
Node.js Fundamentals play a vital role in the context of this article, delving into the core components and functionalities that underpin the integration of Node.js with JavaScript. Understanding the event-driven architecture and package management with npm is crucial for developers to harness the full potential of these technologies. By mastering Node.js fundamentals, software developers can leverage its power to build efficient and scalable applications. Key elements include event-driven programming, asynchronous capabilities, and streamlined package management, all of which contribute to enhancing the overall software development process.
Event-Driven Architecture
Asynchronous Programming
Asynchronous programming is a cornerstone of event-driven architecture, allowing tasks to be executed in a non-blocking manner. This feature enhances the performance of applications by enabling multiple operations to run concurrently. The key characteristic of asynchronous programming lies in its ability to handle IO operations efficiently, making it a popular choice for scenarios requiring parallel processing. Its unique feature of callback functions facilitates seamless task execution without blocking the main thread. While advantageous for improving application responsiveness and efficiency, asynchronous programming may introduce complexities in code readability and sequential logic.
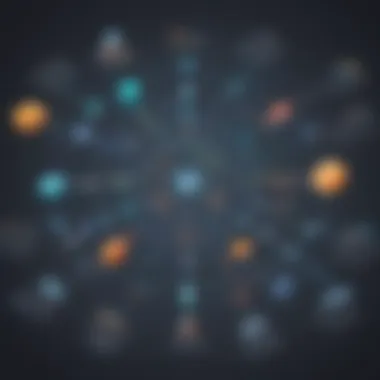
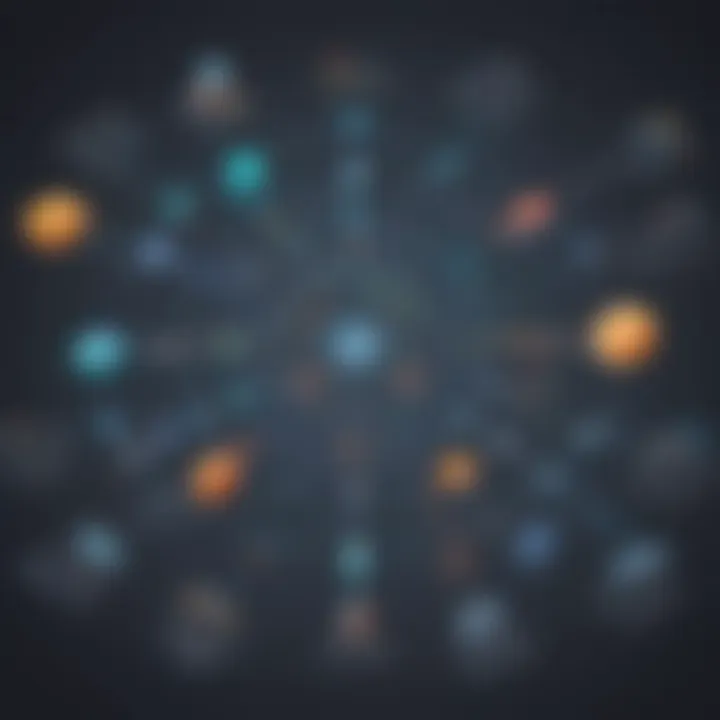
Callbacks
Callbacks are essential components of event-driven architecture, serving as mechanisms to handle asynchronous operations effectively. They enable functions to be invoked upon the completion of specific tasks, ensuring proper synchronization of events. The key characteristic of callbacks is their ability to manage the flow of asynchronous operations by providing structured ways to handle responses. This feature makes callbacks a popular choice in scenarios where precise sequencing of tasks is critical. Their unique feature lies in facilitating error handling and resource cleanup within asynchronous code blocks. However, callbacks can lead to callback hell, a situation where nested callback functions result in unreadable and convoluted code structures, posing challenges for developers.
Package Management with npm
Dependency Management
Dependency Management plays a crucial role in handling external libraries and modules required for software development projects. npm (Node Package Manager) simplifies the process of integrating third-party packages into Node.js applications. The key characteristic of dependency management is its capability to resolve dependencies and version conflicts automatically, ensuring seamless integration of external resources. This feature makes dependency management a preferred choice for managing project dependencies efficiently. npm's unique feature of package-lock.json file aids in locking dependency versions, preventing unexpected updates that may introduce compatibility issues. While advantageous for maintaining project stability and consistency, dependency management through npm may incur additional overhead, particularly in projects with numerous dependencies.
Package Installation
Package Installation is a fundamental aspect of the software development lifecycle, allowing developers to install and manage project dependencies effortlessly. npm offers a streamlined approach to installing packages, providing a wide range of libraries and tools accessible through a centralized repository. The key characteristic of package installation is its convenience and simplicity, enabling developers to add functionalities to their projects with minimal effort. This feature makes package installation a popular choice for rapidly incorporating new features or functionalities. npm's unique feature of package.json configuration file assists in defining project details and dependencies, facilitating straightforward project setup and maintenance. While advantageous for enhancing project productivity and extensibility, package installation may lead to dependency bloat if not managed efficiently, impacting project performance and scalability.
JavaScript in Node.js Development
Node.js, being built on the JavaScript runtime, shares a close relationship with JavaScript in modern software development. Understanding the nuances of JavaScript in the Node.js environment is crucial for developers aiming to harness the full potential of these technologies. In this segment, we delve into the significance and implications of utilizing JavaScript within Node.js, exploring key elements, benefits, and considerations that underpin their intersection.
ES6 Features
Arrow Functions
Arrow Functions, a feature introduced in ECMAScript 2015 (ES6), streamline function syntax in JavaScript, offering a concise and elegant way to define functions. Their implicit return and lexical scoping make them a preferred choice in Node.js development for enhanced code readability and efficiency. The key characteristic of Arrow Functions lies in their ability to preserve the context of 'this,' simplifying code structuring and promoting better code maintainability. Despite their advantages in terms of compactness and clarity, Arrow Functions might present challenges in some contexts due to their lack of binding 'this' behavior, necessitating a deep understanding of their usage to avoid potential pitfalls.
Promises
Promises in JavaScript serve as a pivotal tool for managing asynchronous operations, facilitating better handling of callback hell and enabling a more structured approach to asynchronous code execution. Promises offer a clean and intuitive mechanism for handling asynchronous tasks, promoting code reliability and readability in Node.js applications. Their key characteristic rests in providing a clear path for asynchronous task completion or failure, enhancing error handling and code robustness. While Promises streamline asynchronous programming, improper usage or chaining complexity can lead to callback chain issues and potential performance bottlenecks. Understanding the intricacies of Promises is essential for harnessing their full potential in Node.js development.
Working with JSON
JSON (JavaScript Object Notation) plays a vital role in data exchange between servers and web applications, serving as a lightweight and human-readable data format. Within Node.js development, working with JSON involves serialization and deserialization processes to convert JavaScript objects to JSON strings and vice versa. Serialization entails encoding JavaScript objects into JSON format, ensuring compatibility for data interchange. The key characteristic of Serialization lies in its simplicity and ease of use, making it a preferred choice for data serialization in Node.js projects. However, compatibility issues and potential data loss can arise during the serialization process, necessitating cautious handling of data transformations.
Deserialization
Deserialization, the reverse process of Serialization, involves converting JSON strings back into JavaScript objects for data consumption within the application. Deserialization enables the restoration of complex data structures from JSON format to their original JavaScript form, facilitating seamless data manipulation. The key characteristic of Deserialization lies in its ability to reconstruct JSON data into native JavaScript objects, simplifying data parsing and retrieval processes. Nevertheless, improper deserialization procedures can lead to security vulnerabilities such as code injection or object manipulation if input data is not sanitized and validated thoroughly. Striking a balance between efficiency and data integrity is crucial when working with Deserialization in Node.js applications.
Integration of Node.js and JavaScript
The Integration of Node.js and JavaScript section in this article plays a crucial role in unraveling the seamless amalgamation of these two technologies. By exploring this integration, we delve into the core of modern software development practices where Node.js and JavaScript synergize to empower developers to create robust applications. The significance lies in the ability to leverage the strengths of each technology to benefit software projects. Node.js provides a runtime environment for executing JavaScript code on the server-side, allowing for efficient and scalable application development. Integrating Node.js with JavaScript opens up possibilities for building highly dynamic and performant systems. To fully grasp the potential of this integration, developers must understand the specific elements, benefits, and considerations involved in blending Node.js and JavaScript seamlessly.
Optimizing Performance
Enhancing performance is a critical aspect in the realm of software development. This section focuses on optimizing performance within the context of the interconnected dynamics of Node.js and JavaScript. Optimizing performance ensures that applications run efficiently, delivering a seamless user experience. By implementing robust strategies for performance optimization, developers can fine-tune their applications to operate at peak efficiency, meeting user expectations and business requirements. It involves a deep dive into refining code, implementing efficient algorithms, and leveraging the strengths of both Node.js and JavaScript to achieve optimal performance levels.
Caching Strategies
In-Memory Caching
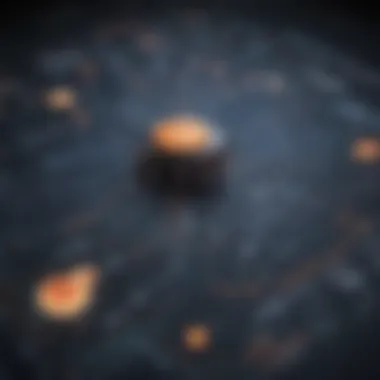
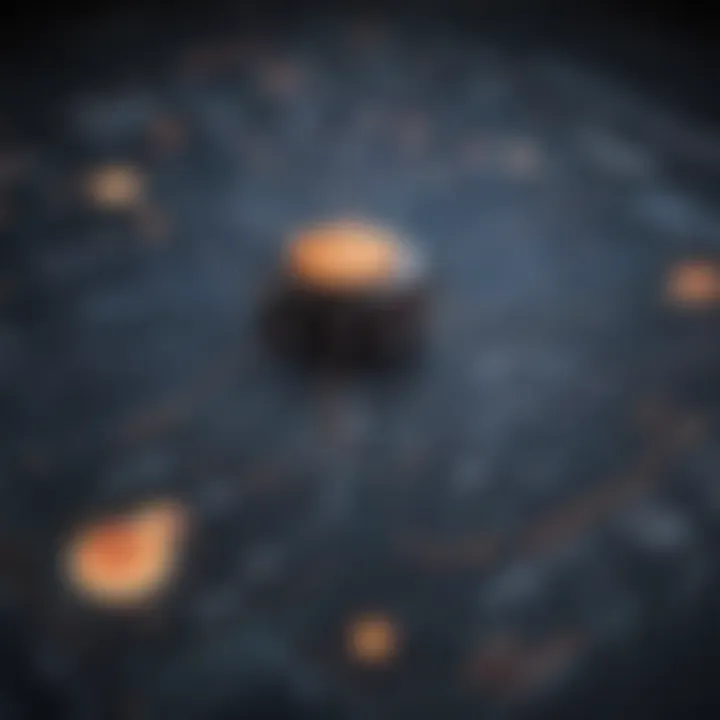
In-Memory Caching plays a pivotal role in optimizing performance by storing frequently accessed data in memory. This strategic approach reduces latency by providing rapid access to cached data, eliminating the need to retrieve information from disk or external sources repeatedly. The key characteristic of In-Memory Caching lies in its ability to enhance application speed and responsiveness. Its fast data retrieval mechanism makes it a preferred choice for high-performance applications that demand quick access to data. However, the main disadvantage of In-Memory Caching is the limitation of storage capacity, which may impact its suitability for handling large volumes of data.
Redis
Redis, a sophisticated caching solution, contributes significantly to optimizing performance by offering advanced data structures and cache functionalities. Its key characteristic lies in its support for versatile data types and complex operations, making it a versatile choice for caching needs. Redis excels in improving application performance by reducing database load through efficient caching mechanisms. The advantage of Redis lies in its scalability and persistence features, allowing seamless integration with various application architectures. However, the potential disadvantage of Redis is the complexity in setting up and maintaining Redis clusters for distributed caching, requiring careful configuration and monitoring for optimal performance.
Scalability Considerations
Load Balancing
Load Balancing is a crucial scalability consideration that involves efficiently distributing incoming network traffic across multiple servers. This ensures optimal resource utilization, prevents server overload, and enhances application reliability. The key characteristic of Load Balancing lies in its ability to increase application availability by maintaining consistent performance levels during varying traffic conditions. Load Balancers act as traffic managers, routing requests to the most suitable server, optimizing performance and response times. However, one potential disadvantage of Load Balancing is the added complexity in managing and configuring load balancer setups across multiple nodes.
Microservices Architecture
Microservices Architecture offers a scalable approach to application development by breaking down large monolithic applications into smaller, independently deployable services. The key characteristic of Microservices Architecture is its modular and decentralized nature, allowing teams to develop, deploy, and scale services independently. This approach enhances agility, scalability, and resilience in complex applications by isolating functionalities into manageable units. Microservices Architecture's advantage lies in its flexibility and resilience, enabling seamless updates and maintenance without disrupting the entire system. However, managing inter-service communication and ensuring data consistency across microservices may pose challenges in maintaining system integrity and performance.
Security Best Practices
Security Best Practices play a crucial role in software development, especially when delving into the intricate relationship between Node.js and JavaScript. Implementing robust security measures ensures the protection of sensitive data, mitigates potential vulnerabilities, and upholds the integrity of applications. When exploring the intersection of Node.js and JavaScript, adhering to Security Best Practices becomes paramount. Developers must consider elements such as encryption, authorization mechanisms, and secure coding practices to fortify their applications against cyber threats. By prioritizing Security Best Practices, developers can instill trust and confidence in their software, safeguarding it from malicious actors and ensuring a seamless user experience. Embracing a security-first mindset not only enhances the overall quality of the application but also demonstrates a commitment to safeguarding user privacy and data integrity.
Authentication Mechanisms
Authentication Mechanisms, including JWT Tokens and OAuth, form the cornerstone of secure application development.
JWT Tokens
JWT Tokens offer a stateless, secure method of authentication, contributing significantly to the overall security of applications. The key characteristic of JWT Tokens lies in their ability to encode user information into a compact, digitally signed token, allowing for secure transmission between parties. This approach eliminates the need for server-side storage of session data, streamlining authentication processes and reducing server load. The beauty of JWT Tokens lies in their versatility and efficiency, making them a popular choice for securing Node.js and JavaScript applications. However, developers must carefully handle token expiration and validation to prevent security loopholes and unauthorized access. Despite their benefits, improper implementation of JWT Tokens can lead to token-based vulnerabilities, underscoring the importance of thorough security measures during integration.
OAuth
OAuth stands as a robust authorization framework that enhances the security of applications by enabling secure, delegated access to resources on behalf of the user. The key characteristic of OAuth lies in its ability to facilitate secure authorization flows between multiple services, allowing users to grant limited access to their information without disclosing their credentials. This approach minimizes the exposure of sensitive data and reduces the risk of unauthorized access, bolstering the overall security posture of applications. OAuth's seamless integration with popular platforms and its support for various grant types make it a preferred choice for securing Node.js and JavaScript applications. However, developers must adhere to OAuth best practices, including secure token storage and revocation mechanisms, to maintain the confidentiality and integrity of user data. While OAuth offers a convenient and flexible authorization solution, rigorous security implementations are essential to prevent misuse and data breaches.
Input Validation
Input Validation forms a critical component of application security, playing a vital role in safeguarding against injection attacks and data manipulation.
Sanitization
Sanitization involves cleansing and validating user input to remove potentially harmful characters and maintain data integrity. The key characteristic of sanitization lies in its ability to filter out malicious content, preventing cross-site scripting (XSS) attacks and SQL injection vulnerabilities. This proactive approach ensures that only valid and safe input is processed by the application, reducing the risk of code injection and other security exploits. The meticulous implementation of sanitization routines enhances the overall robustness of Node.js and JavaScript applications, instilling confidence in the system's ability to handle user input securely. However, developers must strike a balance between strict sanitization protocols and user experience, ensuring that legitimate user input is not inadvertently filtered out. While sanitization serves as a fundamental defense mechanism, continuous monitoring and updates are necessary to adapt to evolving security threats and maintain the defenses against emerging vulnerabilities.
Validation Libraries
Validation Libraries offer a convenient and standardized approach to input validation, streamlining the validation process for developers. The key characteristic of validation libraries lies in their extensibility and flexibility, providing pre-built validation rules and error handling mechanisms for common data types. By integrating validation libraries into Node.js and JavaScript applications, developers can expedite the validation of user input, reduce code duplication, and enhance code readability. Additionally, validation libraries offer built-in protection against common security pitfalls, such as inconsistent data formats and injection attacks, promoting secure coding practices and data integrity. While validation libraries simplify the validation process, developers must stay updated on library versions and maintain adherence to best practices to leverage their full security potential. Incorporating validation libraries into the development workflow empowers developers to fortify their applications against common security threats, ensuring a robust defense mechanism against input-based vulnerabilities.
This detailed exploration of Security Best Practices, Authentication Mechanisms, and Input Validation provides valuable insights into the complexity of securing Node.js and JavaScript applications. By prioritizing security measures, implementing robust authentication mechanisms, and enforcing stringent validation protocols, developers can safeguard their applications against cyber threats and uphold the integrity of their software. Embracing a security-centric approach not only elevates the quality of applications but also instills trust in users, fostering a secure and reliable software ecosystem. The integration of these security principles into the development lifecycle ensures that Node.js and JavaScript applications stand resilient against evolving threats, reinforcing their position as key players in modern software development.
Testing and Debugging
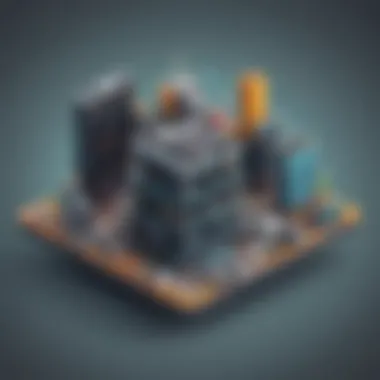
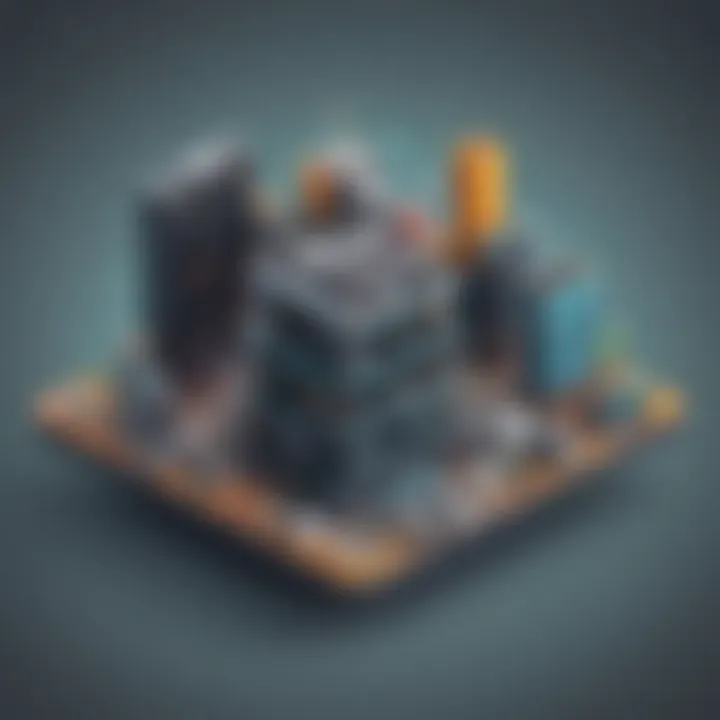
In the realm of software development, testing and debugging play a paramount role in ensuring the functionality, reliability, and performance of applications. In the context of the interplay between Node.js and JavaScript, the segment of testing and debugging holds great significance. Testing helps in identifying issues and ensuring that the code operates as intended, while debugging is essential for pinpointing and rectifying any bugs or errors that may arise during development.
Unit Testing with Jest
Test Suites
The concept of test suites within the domain of software testing is crucial as it allows developers to organize and execute a set of test cases to validate the behavior of specific modules or components. In the context of Node.js and JavaScript development, using Jest for unit testing provides a structured approach to create comprehensive test suites. Jest's ability to run tests asynchronously and set up mock environments facilitates thorough testing of individual units of code, contributing to the overall quality and robustness of the application.
Jest's seamless integration with Node.js environments and its user-friendly syntax make it a popular choice among developers for writing test suites. Its ability to generate detailed reports and simplify the process of running tests enhances the efficiency of testing procedures. However, one consideration to note is that Jest is primarily designed for unit testing and may not be suitable for all types of testing scenarios, requiring developers to supplement it with other testing tools when necessary.
Mocks and Spies
Mocks and spies are essential components in the realm of unit testing, allowing developers to simulate dependencies, control the behavior of functions, and track the execution of specific code sections. When applied in the context of Node.js and JavaScript development, mocks and spies enable developers to isolate components for testing, verify the interaction between different parts of the code, and streamline the testing process.
The distinctive feature of mocks lies in their ability to mimic external dependencies or functions, allowing developers to test components in isolation without relying on external resources. Spies, on the other hand, monitor the execution and behavior of specific functions, providing insights into how the code interacts within the application. While mocks and spies enhance the efficiency and accuracy of unit testing, developers should exercise caution to avoid over-reliance on these mechanisms, as excessive mocking may lead to an artificial test environment that does not reflect real-world scenarios accurately.
Deployment Strategies
Deployment strategies play a pivotal role in the realm of software development, orchestrating the smooth transition of applications from development environments into production. In this article focusing on the intersection of Node.js and JavaScript in software development, the deployment strategies section serves as a crucial cog in the development lifecycle. Emphasizing the importance of streamlining deployment processes, this segment sheds light on optimizing efficiency, minimizing errors, and improving overall software quality.
Containerization with Docker
Dockerizing Applications
Diving into Dockerizing Applications within the context of software deployment strategies within this article, the focus is on encapsulating applications into lightweight, portable containers. This encapsulation ensures consistency across different environments, making deployments more reliable and efficient. The key characteristic of Dockerizing Applications lies in its ability to isolate dependencies, facilitating easier deployment and scalability. This approach proves beneficial in maintaining development consistency and promoting collaboration among team members, underscoring its popularity in modern software development. The unique feature of Dockerizing Applications is its flexibility and compatibility, allowing developers to seamlessly deploy applications across various platforms. While Docker brings immense advantages in terms of efficiency and reliability, some challenges such as complex networking configurations may arise.
Orchestration
Exploring Orchestration in the context of deployment strategies elucidates the management and streamlining of multiple Docker containers, ensuring seamless coordination and scalability of applications. The key characteristic of Orchestration lies in automating the deployment, scaling, and management of containerized applications, simplifying intricate processes and enhancing operational efficiency. Its appeal in this article stems from its capability to optimize resource utilization, automate tasks, and improve overall system resilience. The unique feature of Orchestration is its ability to handle complex deployments effortlessly, streamlining the management of interconnected containerized components. While Orchestration offers significant benefits in terms of scalability and resource optimization, it may pose challenges in terms of initial setup complexity and orchestration overhead.
Continuous IntegrationContinuous Deployment ()
Automation Pipelines
Delving into Automation Pipelines within the scope of CICD in software deployment strategies sheds light on the automation of testing, integration, and deployment processes. Automation Pipelines streamline the delivery pipeline, ensuring rapid feedback loops and efficient deployment cycles. The key characteristic of Automation Pipelines is their ability to automate repetitive tasks, minimizing human intervention and accelerating deployment timelines. This aspect proves beneficial in achieving continuous delivery and integration, aligning with agile development practices. The unique feature of Automation Pipelines lies in their customizable nature, enabling teams to tailor workflows to suit specific project requirements. While Automation Pipelines offer advantages in terms of productivity and consistency, managing complex pipeline configurations may present challenges.
Deployment Environments
Analyzing Deployment Environments within the realm of CICD strategies underscores their role in creating isolated environments for testing and deploying applications. Deployment Environments ensure consistency between development, testing, and production stages, mitigating risks associated with variations in configuration. The key characteristic of Deployment Environments is the ability to replicate production conditions for testing purposes, enhancing the reliability and accuracy of deployments. This aspect proves beneficial in detecting bugs early in the development cycle and ensuring smooth transitions into production. The unique feature of Deployment Environments is their scalability and versatility, allowing for streamlined parallel testing and deployment of multiple application versions. While Deployment Environments offer advantages in terms of testing efficiency and risk mitigation, maintaining consistency across different environments may pose challenges.
Conclusion
In the realm of software development, the conclusion encapsulates the pivotal essence of harmonizing Node.js and JavaScript's prowess. It imbues the reader with a profound understanding of the symbiotic relationship between these technologies, emphasizing their combined potential to revolutionize modern application building. By carefully dissecting the intricate interplay between Node.js and JavaScript throughout this article, developers are equipped with the knowledge to leverage these tools optimally, unlocking a realm of possibilities for innovative software solutions. This section serves as the culminating point, tying together the threads of discussion and affirming the indispensable role of Node.js and JavaScript in contemporary development landscapes.
Embracing the Synergy of Node.js and JavaScript
Future Prospects
Exploring the future prospects in the context of Node.js and JavaScript unveils a horizon brimming with opportunities and advancements. The forward-looking nature of embracing these technologies not only caters to current needs but also anticipates and adapts to upcoming trends in software development. With a keen eye on scalability, performance optimization, and enhanced user experiences, future prospects hold the promise of driving innovation to unprecedented heights. Incorporating cutting-edge features and functionalities, this trajectory ensures that applications stay relevant and competitive amidst the ever-evolving technological landscape. While ushering in a new era of possibilities, future prospects demand a commitment to continuous learning and adaptation, positioning developers for sustained success.
Innovation in Development
Delving into innovation in development unravels a tapestry of creativity and boundary-pushing within the Node.js and JavaScript paradigm. This facet shines a light on the art of thinking beyond conventional boundaries, fostering inventive solutions and novel approaches to problem-solving. By embracing innovation, developers inject a dose of ingenuity into their projects, propelling them towards game-changing outcomes. The core characteristic of innovation lies in its disruptiveness, challenging the status quo and paving the way for transformative digital experiences. While embracing innovation spices up the development process, it also demands meticulous attention to detail and a willingness to explore uncharted territories. Through a blend of vision, experimentation, and relentless pursuit of excellence, innovation in development serves as a cornerstone for realizing the full potential of Node.js and JavaScript in software creation.