Mastering MySQL Connections in Java
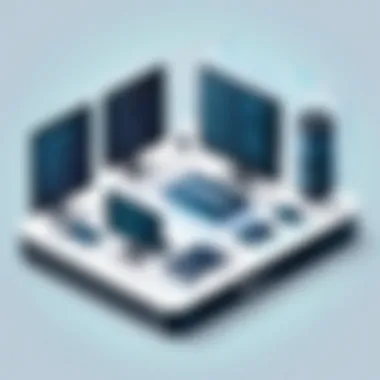
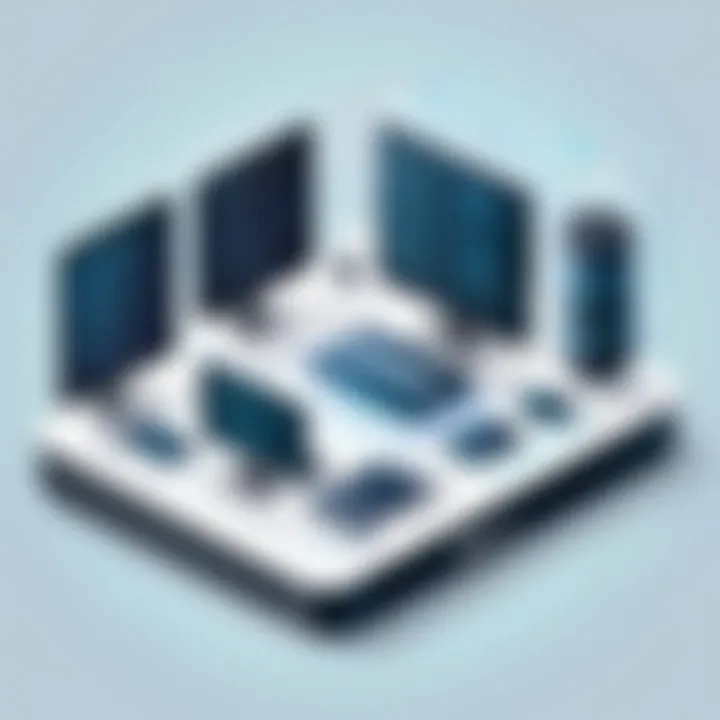
Intro
In the world of software development, the ability to manage and manipulate data effectively is paramount. A major piece of this puzzle lies in connecting to databases, and when it comes to Java applications, establishing a connection with MySQL databases is a task every developer will likely encounter. The intricacies of this task vary from handling basic queries to optimizing connections for better performance. In this guide, we will explore various aspects of MySQL connection in Java, from the foundational principles of JDBC to some advanced techniques such as connection pooling.
By understanding how to effectively establish and manage these connections, developers can build robust applications that interact seamlessly with databases.
Overview of MySQL and Java Connection
MySQL is a widely used relational database management system, known for its reliability and ease of use. When paired with Java, it opens up a world of possibilities for data handling. The relevance of MySQL in today’s tech ecosystem cannot be overstated; it stands as a backbone for countless applications ranging from small scale to enterprise-grade systems.
Definition and Importance of MySQL Connection in Java
In technical terms, a MySQL connection in Java refers to the link established between a Java application and a MySQL database. This connection allows Java applications to execute SQL statements, retrieve results, and even manipulate data residing in the database.
Connecting to a MySQL database serves several essential purposes:
- It enables data retrieval for dynamic content presentation.
- It allows for data updates, which is crucial for applications that require user input.
- It supports transaction management, ensuring that operations are completed successfully or rolled back without data corruption.
Key Features and Functionalities
The interplay of Java with MySQL provides numerous features that enhance application functionality:
- JDBC: Java Database Connectivity (JDBC) facilitates direct communication between Java applications and MySQL databases.
- Driver Support: MySQL provides various drivers for seamless integration, including JDBC driver which is paramount in connecting the two.
- Transaction Management: Java applications can execute multiple sequential SQL commands as a single logical unit through transactions, maintaining data integrity.
- Connection Pooling: This is crucial for performance, allowing multiple simultaneous connections without the overhead of establishing new connections for each request.
Best Practices
When working with MySQL connections in Java, adhering to industry best practices can greatly enhance efficiency and stability.
Industry Best Practices for Implementing MySQL Connections
- Use Connection Pooling: As mentioned, managing multiple database connections efficiently is essential. Connection pooling helps by reusing existing connections, which reduces latency and resource consumption.
- Close Connections Properly: Always ensure that connections are closed after use to prevent memory leaks. Utilizing a try-with-resources statement can simplify this process.
- Prepared Statements: Use prepared statements instead of regular statements to enhance performance and reduce vulnerability to SQL injection attacks.
Tips for Maximizing Efficiency and Productivity
- Regularly monitor connection performance to identify bottlenecks.
- Leverage caching mechanisms for frequently accessed data to minimize database hits.
Common Pitfalls to Avoid
- Avoid hardcoding database credentials in your code, to enhance security.
- Don’t ignore exception handling; ensure that your application can gracefully handle database connectivity issues.
Case Studies
Learning from real-world implementations provides invaluable insight. Below are examples of successful MySQL connections in Java applications:
Example 1: E-Commerce Platform
A popular e-commerce platform utilized MySQL connections to handle thousands of transactions. By implementing connection pooling and prepared statements, they reduced latency and ensured transactional integrity during peak loads. This led to a 30% increase in transaction efficiency.
Example 2: Banking Application
In a banking application, utilizing JDBC for MySQL connectivity allowed for smooth transaction processing. They prioritized robust error handling which resulted in minimal downtime during critical operations.
Latest Trends and Updates
Keeping up to date with the latest advancements can give developers an edge in their projects.
Current Industry Trends
- Microservices Architecture: More applications are shifting to microservices, necessitating efficient database connections to cater for multiple service instances.
- Increased Focus on Security: As breaches become more common, using features such as SSL connections in JDBC is now a standard practice.
Upcoming Advancements
With constant changes, developers should anticipate enhanced features in MySQL. For instance, improvements in performance tuning and automated management tools are on the horizon, promising even greater integration capabilities with Java applications.
How-To Guides and Tutorials
For developers looking to dive in:
Step-by-Step Guide for Establishing a Connection
- Add the MySQL JDBC Driver: Include the driver in your project dependencies.
- Load the Driver: Use to load the driver.
- Creating the Connection: Utilize
- Execute Queries: Use or for your database operations.
- Close the Connection: Make sure to close the connection after use.
Hands-On Tutorials
- Beginner tutorials might focus on simple CRUD operations using Java and MySQL.
- Advanced users can explore topics such as connection pooling management with HikariCP.
Practical tips include using configuration files for database credentials and managing dependencies with tools like Maven or Gradle.
Through understanding these concepts, both novices and seasoned developers have the tools necessary to effectively implement MySQL connections in Java, ensuring their applications run smoothly and efficiently.
Understanding MySQL and Java Integration
In today’s data-driven world, the collaboration between programming languages and database systems such as MySQL is not just important—it's essential. Integrating MySQL with Java allows for efficient data management and manipulation, helping developers build robust and scalable applications. Such integration plays a critical role in applications that require persistent data storage, like web-based platforms, mobile apps, and enterprise software.
One of the key aspects of this integration is the Java Database Connectivity (JDBC) API, which acts as the bridge between Java applications and MySQL databases. It enables developers to connect to databases, execute queries, and handle results seamlessly. The significance of understanding how MySQL and Java work together cannot be overstated, as it empowers developers with the knowledge to optimize performance and ensure data integrity.
Additionally, familiarity with MySQL’s features, such as transactions, indexing, and stored procedures, combined with Java's object-oriented capabilities, can lead to advanced system functionalities. This synergy not only enhances application performance but also allows for more sophisticated data handling techniques. Moreover, managing database connections properly can have a profound effect on the server's response time, resource usage, and scalability.
The following subsections will delve deeper into MySQL itself and unpack Java's pivotal role in effective database management.
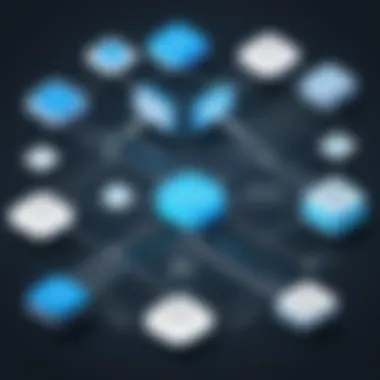
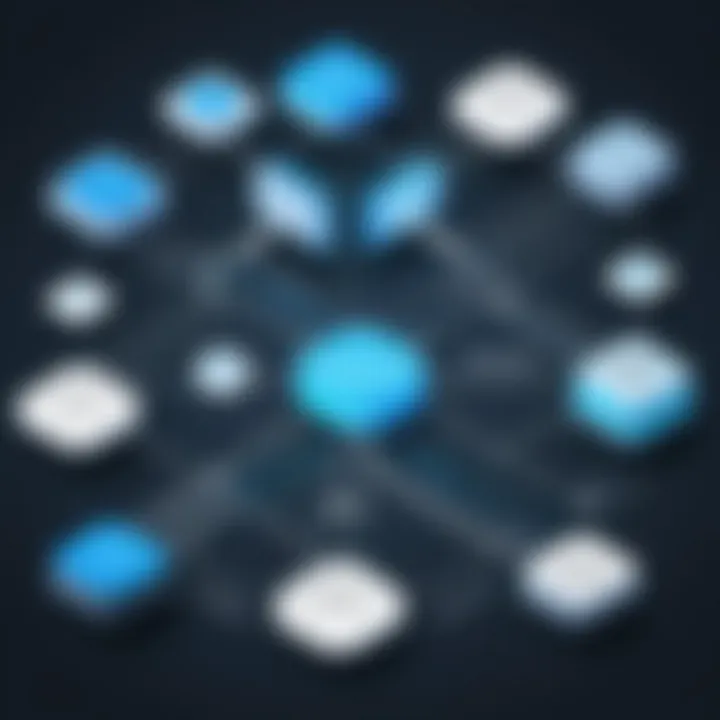
Overview of MySQL Database System
MySQL is a powerful open-source relational database management system (RDBMS), widely recognized for its speed, ease of use, and reliability. Its architecture is based on structured query language (SQL), making it a popular choice among developers who require a robust backend solution. MySQL's ability to handle vast amounts of data and concurrent user transactions makes it suitable for various high-demand applications.
Some notable features of MySQL include:
- Flexibility: MySQL supports various data types and structures, enabling developers to create tailored databases.
- High Performance: With features such as query caching and advanced indexing mechanisms, MySQL provides efficient query execution.
- Scalability: MySQL can be scaled vertically and horizontally, accommodating growing data and traffic demands.
- Secure Access: With layered security options, including user privileges and encryption, MySQL ensures that data remains protected from unauthorized access.
The extensive community support and documentation further facilitate easier troubleshooting and learning. For many developers, MySQL is the go-to database system due to its combination of these essential characteristics.
"MySQL stands as the backbone for countless applications, demonstrating the essence of power and flexibility in database management."
The Role of Java in Database Management
Java, being a versatile programming language, holds a significant place in the realm of database management. Its architecture, based on the principle of 'Write Once, Run Anywhere,' provides developers with an elegant solution for managing databases across different environments. One of the fundamental components of Java's database interactions is JDBC, which provides a standardized way for Java applications to interact with various databases, including MySQL.
Java's object-oriented nature allows for modular code that is easier to maintain and extend over time. The encapsulation of database logic within Java classes leads to better organization and can aid in minimizing errors. Furthermore, Java's rich library of frameworks—such as Hibernate and Spring—offers more sophisticated data handling capabilities, including ORM (Object-Relational Mapping) techniques that simplify database operations, making them more intuitive.
Key benefits of using Java for database management are:
- Consistency: Java's platform independence allows for consistent database handling across different systems.
- Ease of Integration: Java integrates effortlessly with MySQL via JDBC, providing a straightforward approach to execute SQL queries and manipulate results.
- Robust Error Handling: Java's built-in exception handling can help developers manage database-related errors efficiently.
- Community Support and Resources: A vast collection of libraries and frameworks aids developers in keeping pace with evolving database needs.
Learning how to effectively merge these two technologies paves the way for developing high-performance applications capable of addressing today's complex data requirements.
JDBC: The Foundation of Java Database Connectivity
JDBC stands as a cornerstone for empowering Java applications to harness the capabilities of relational databases like MySQL. Understanding JDBC is crucial for developers because it enables seamless communication with databases. By diving into JDBC, we explore not just a protocol, but an essential framework that bridges Java applications and database systems. Essentially, if you’re aiming to get your Java application to talk to MySQL, JDBC is your best friend.
What is JDBC?
Java Database Connectivity, or JDBC for short, is a Java-based API that facilitates the connection between Java applications and various databases. At its core, it provides a standard interface for querying and updating databases through SQL statements. This ability to execute SQL commands directly from Java makes data handling much more straightforward and efficient.
It essentially allows you to perform a range of operations such as:
- Connecting to a database
- Executing SQL queries
- Retrieving and processing data
- Managing database transactions
Without JDBC, interacting with databases would require different APIs based on the database vendor, complicating the development process. JDBC makes it easier for developers to write code that is more concise and adaptable, enhancing productivity.
JDBC Architecture and Drivers
Diving deeper into JDBC, its architecture is composed of two major components: the JDBC API and the JDBC Driver Manager. The API serves as the interface for Java applications, while the Driver Manager keeps track of different drivers, facilitating connection requests from applications.
Moreover, JDBC operates through various types of drivers:
- JDBC-ODBC Bridge Driver: This connects JDBC calls to ODBC calls, but it’s less favored due to performance issues.
- Native-API Driver: These drivers use the client-side libraries of the database, providing better performance compared to the bridge driver.
- Network Protocol Driver: A flexible driver that communicates with the database server through a network protocol.
- Thin Driver: This is a pure Java driver, meaning no native library is required. It’s lightweight and works directly with the database from any Java application.
Each type of driver suits different needs and requirements, making it essential to choose the right one depending on the project’s circumstances.
Selecting the Right JDBC Driver
Choosing the right JDBC driver can make or break your application's performance and reliability. Factors to consider include:
- Compatibility: Ensure the driver supports the version of the database you’re using. For instance, if you’re working with MySQL, you would typically select the MySQL Connector/J driver.
- Performance: Analyze how each driver copes with data loads. Native and Thin drivers usually provide better performance than the JDBC-ODBC bridge.
- Support and Documentation: Opt for drivers with reputable community support and robust documentation. It makes troubleshooting and development easier.
Here's a simple guideline on how to select a JDBC driver:
- Identify your project requirements.
- Evaluate the aforementioned factors.
- Test the driver in a development environment before full deployment.
In essence, JDBC is a vital element that connects Java with MySQL. Whether you’re crafting a simple application or a complex data-driven system, the role of JDBC cannot be overstated. Understanding its intricacies and selecting the right components considerably enhances your development journey.
In short, JDBC not only facilitates database interactions, but also acts as a bridge between Java applications and MySQL, ensuring that developers have the necessary tools to build efficient and effective data-driven applications.
Establishing a Connection to MySQL
Establishing a connection to MySQL is not just a step; it's a pivotal phase in the broader picture of database management in Java applications. Every interaction with the database relies on a successful connection. If you think about it, without this connection, the entire effort of data retrieval, updates, or deletions would simply come to a standstill. At its core, this connection allows you to execute SQL queries and retrieve results, making it the bridge between your application and the database.
There are specific elements intertwined with establishing a connection that developers must grasp, including the overall security of the connection, efficiency, and context of your application. For instance, a poor connection strategy can lead to performance bottlenecks. Conversely, a well-established connection can enable your application to perform efficiently, handling multiple queries without excessive delay.
"A smooth connection is like oil to a machine; it keeps everything running without a hitch."
A few considerations go hand in hand with this topic, especially regarding connection parameters. Understanding how to configure aspects such as timeout settings, authentication details, and server URLs can have a significant impact on your connection's reliability and security.
Setting Up MySQL Database
Setting up your MySQL database is the first step before diving into any code. You need a functioning database and a user account with the appropriate privileges. Here's a straightforward approach to get you started:
- Install MySQL: Follow the installation guide on the official MySQL documentation.
- Create a Database: Use the command line or a GUI tool like MySQL Workbench to create your own database. For instance, creates a new database.
- User Account: Make sure you have a user account that has permissions on your newly created database — perhaps a simple will do the trick.
These steps will ensure that the foundation is solid for later connecting via Java.
Connection String Format
The connection string acts as the roadmap for your Java application to find and access the MySQL database. The format is generally straightforward but requires precision: it needs to include the server address, database name, user credentials, and any options you want to specify.
A typical connection string for MySQL may look like this:
Here are the components explained:
- hostname: The server where your MySQL instance is running. Use if it's the same machine.
- port: By default, MySQL runs over port 3306. If you haven't changed it, just use this default.
- dbname: The name of the database you wish to connect to.
- username: Your MySQL username.
- password: The corresponding password for that user.
Code Example: Basic Connection
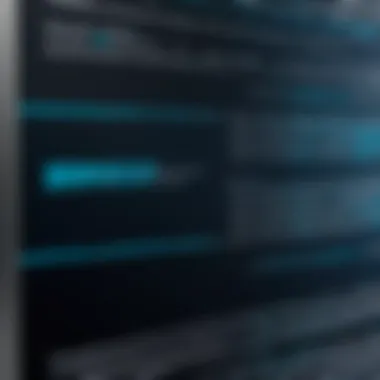
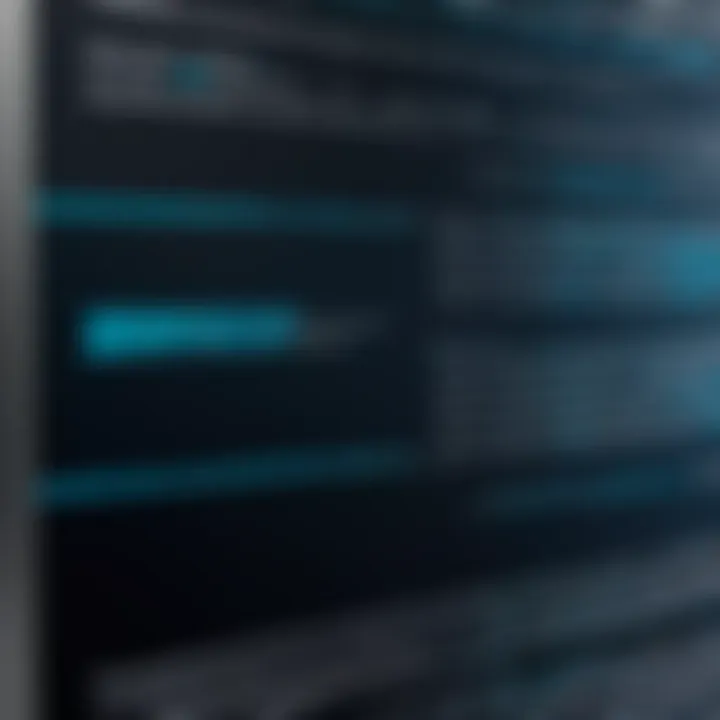
Now that you've set up your database and crafted a connection string, it's time to put it all together in code. Below is a simple example that embodies the fundamental connection process:
In this snippet, you can see how to use Java's to create a connection. Handling exceptions is crucial since any misstep in your connection parameters will throw an error. This example sets the stage for more intricate operations you will perform on the database.
Handling Exceptions and Errors
When it comes to database connectivity and management, handling exceptions and errors is crucial. Think of it as keeping a well-oiled machine running smoothly. In the case of integrating MySQL with Java, errors can arise from various sources, including network issues, incorrect JDBC configurations, and even data inconsistencies. Effective error handling not only enhances the stability of applications but also improves the overall user experience.
Without a proper strategy to manage exceptions, your application could face unexpected crashes or provide inaccurate results, leading to frustrated users and potential data loss. Cultivating a deep understanding of this domain prepares developers to troubleshoot issues efficiently and maintain a robust application.
Common JDBC Exceptions
In the encounter with JDBC, one must be wary of several common exceptions that can surface. Familiarizing oneself with these can save a lot of heartaches down the line. A few notable exceptions include:
- SQLSyntaxErrorException: This pops up when there's a syntax error in your SQL query. For example:
- SQLTimeoutException: This is raised when your query does not complete within a specified time frame.
- SQLException: This is the parent class of all SQL-related exceptions. Encountering this can hint at a range of problems, from connection issues to transactional errors.
These exceptions can provide pivotal clues when debugging connection problems. Keeping them in mind while coding can better prepare you for inevitable hiccups.
Best Practices for Error Handling
When facing errors, a systematic approach to handling them is essential. Here are some best practices that can help:
- Use Try-Catch Blocks: Wrapping your database code in try-catch blocks ensures that even if something goes wrong, the application doesn't crash unceremoniously.
- Log Errors: Implementing logging mechanisms allows developers to track problems better. Tools like Log4j or SLF4J can be particularly useful for logging, helping you to capture insights into the occurrence of exceptions.
- Show User-Friendly Messages: Instead of displaying confusing error messages to users, present them with friendly messages that guide them, perhaps suggesting some corrective actions.
- Roll Back Transactions: In cases where data integrity might be at risk, ensure that you're rolling back transactions upon encountering an error. This keeps the database in a consistent state.
- Validate Input: Syphon errors at the start by meticulously validating inputs before they hit the database. Doing this can prevent many SQL exceptions from cropping up in the first place.
Adhering to these best practices can be the difference between a stable application and a system filled with errors. Each time a developer writes error handling code, they are investing in the application's future reliability—no one wants to be digging through a mountain of errors in production.
Executing SQL Statements
Executing SQL statements is the cornerstone of any interaction with a MySQL database from Java. This section aims to explore the significance of SQL execution, spotlighting its various forms, the differences between prepared statements and regular statements, and providing a code sample to illustrate the concepts in a practical context.
One cannot underestimate the importance of executing SQL statements. Every database interaction—be it querying data, updating existing records, or affecting any change in the database—is fundamentally based on SQL commands. These operations happen through JDBC in Java. Without a proper grasp of executing SQL statements, one may encounter performance issues, security vulnerabilities, or even data integrity problems.
Types of SQL Statements
Understanding the different types of SQL statements is essential for effectively managing database interactions. In basic terms, SQL statements can be categorized into three main types:
- Data Query Language (DQL): This includes the SQL statement, which retrieves data from the database. Mastering DQL is critical as this is the primary method of fetching information.
- Data Manipulation Language (DML): This contains SQL statements like , , and , which allow the manipulation of data within the tables. DML is how you add, modify, or delete your records.
- Data Definition Language (DDL): It includes commands like , , and , which are vital for defining and modifying the structure of the database itself.
By knowing these types, developers can craft their SQL commands more efficiently, playing to the strengths of each category.
Prepared Statements vs. Statement
A crucial consideration in executing SQL statements is choosing between prepared statements and regular statements. Prepared statements offer multiple advantages:
- Precompiled Execution: They are precompiled by the database, which makes them faster for repeated execution, as the preparation time is only counted once.
- Security: Prepared statements help prevent SQL injection attacks as they separate SQL code from data values. This means user inputs are treated as data, not as code, a vital practice in safeguarding database interactions.
- Clarity: Prepared statements can improve code readability, particularly when executing complex queries with many parameters.
On the other hand, regular statements are simpler and may be more convenient for straightforward queries that do not need to be executed multiple times. However, they come with performance and security drawbacks that may disadvantage them in more sophisticated contexts.
Code Example: Executing Queries
To demonstrate executing SQL statements, let's consider a basic scenario where we want to retrieve a list of users from a database:
In this example, we set up our database connection and create a object to execute a simple query. This code fetches user accounts from the database and prints out their usernames. Notice the error handling included in the try-catch block. Understanding how to execute statements correctly while ensuring safety and performance is necessary for any developer working with Java and databases.
"Getting SQL right is crucial for a robust application. A single mistake can lead to poor performance and unresponsive systems."
Incorporating solid practices when executing SQL statements ensures that your application runs as smoothly as possible, safeguarding both data and user interactions.
Connection Pooling for Performance
When dealing with MySQL connections in Java, it's not just about establishing a link to the database. Performance plays a significant role, and this is where connection pooling comes into the picture. Think of it as a designated parking lot for your database connections. Instead of each application request scouting for an available parking space, pooling keeps a bunch of spots ready and waiting. This saves time and makes the entire system run smoother.
What is Connection Pooling?
At its core, connection pooling is a technique that allows multiple database connections to be reused without going through the overhead of creating a new connection every single time. When a program needs to access the database, it can pull an existing connection from the pool rather than establishing a new one. This greatly minimizes the latency involved in connecting to a database, which can otherwise be quite significant.
Pooling involves a set of connections that are opened when the application starts and held in reserve. When applications need these connections, they borrow them from the pool, use them for a brief period, and then return them to the pool when finished. This way, the resources are managed more effectively, leading to lower latency and increased throughput.
Benefits of Connection Pooling
Implementing connection pooling can bring several advantages:
- Efficiency: Reduces the overhead of repeatedly opening and closing connections. This is especially beneficial in applications with heavy traffic where connections are frequently needed.
- Resource Management: Limits the number of connections that can be opened at any one time, ensuring better control over database resources and avoiding clashes.
- Faster Response Times: By utilizing an existing connection, applications can respond more quickly to client requests, enhancing overall user experience.
- Load Balancing: Helps distribute load evenly across resources, reducing the likelihood of overwhelming any single database instance.
- Connection Timeout: Most pooling solutions come with built-in timeout settings that can help in managing stale connections automatically.
It's like having a corner cafe that keeps your favorite coffee hot and ready rather than needing to brew each cup from scratch.
Implementing Connection Pooling in Java
Setting up connection pooling in Java can be straightforward if done correctly. There are several libraries available, each with its own unique features. A popular choice is Apache Commons DBCP. Here's a step-by-step guide to get you started:
- Add Dependency: Ensure you have the necessary dependencies in your project. If you’re using Maven, you can include:
- Configuration: Set up the connection pool configuration within your application. Here’s a brief example:
- Using the Pool: Borrow a connection, use it, and return it to the pool when finished:
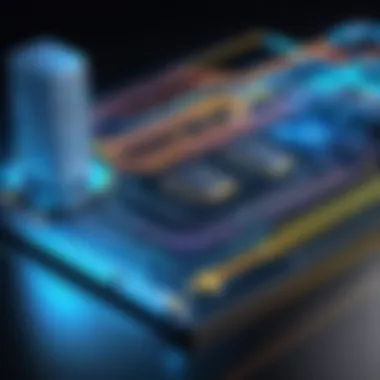
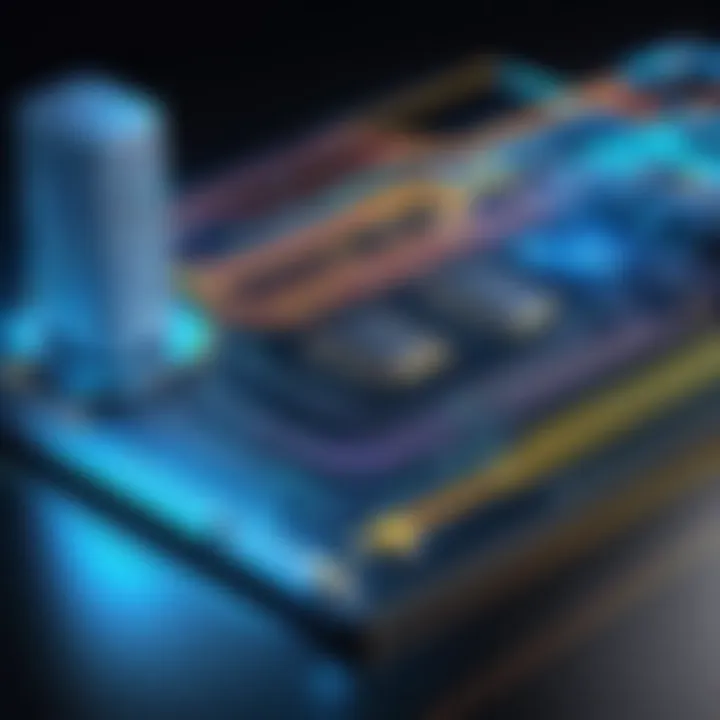
In the example above, replace the placeholders with your actual database details. It helps to be mindful of connection pool settings, such as the maximum size and initial connections, to tune performance.
By integrating connection pooling into your Java application, you’re not merely throwing a band-aid over potential issues. You’re deliberately enhancing efficiency and responsiveness, which is crucial for any robust data-driven application.
Connection pooling is about making the most of resources while ensuring the application remains responsive, even under high demand.
Security Considerations
Security is crucial when working with MySQL connections in Java. A compromised database can lead to data breaches, financial losses, and reputational damage. Therefore, understanding the ins and outs of database security is not just an option, it’s a necessity. In this section, we will explore how to secure your database connections and the measures to take against specific threats, notably SQL injection.
Securing Database Connections
When establishing a connection to a MySQL database from Java, ensuring the security of that connection should be a top priority. There are several strategies that can be employed to safeguard these connections:
- Use Secure Connections: Enabling SSL for database connections helps encrypt the data exchanged between the Java application and the MySQL server. This minimizes the risk of eavesdropping and man-in-the-middle attacks.
- Authentication Measures: Utilize strong authentication methods such as password hashing and multiple authentication factors. By not just relying on a username/password combination, you can significantly add to the security layer.
- Regular Updates: Keeping both MySQL and any libraries or frameworks up to date is crucial. Security patches that fix vulnerabilities are released periodically, and being on the latest version helps in standing guard against potential exploits.
- Configure Firewalls: Properly configure your network firewalls to restrict access to the database server. This limits exposure to potentially malicious traffic and unauthorized users.
- Permissions and Roles: Implement the principle of least privilege by assigning only necessary permissions to users. This minimizes the impact of compromised accounts.
Remember: "An ounce of prevention is worth a pound of cure." Implementing these security practices can save you from a wealth of troubles.
SQL Injection Prevention
SQL injection remains one of the most common and dangerous security issues for applications connecting to databases. It happens when an attacker can insert malicious SQL code through your application, often through input fields like forms or API requests. Here are several practices to mitigate this risk:
- Prepared Statements: This is perhaps the most effective method to prevent SQL injection. By using prepared statements in JDBC, you separate SQL logic from data, ensuring that any user input is treated solely as data, not executable code.
An example of a prepared statement in Java: - Input Validation: Always validate and sanitize user input. Reject any data that doesn’t conform to expected formats or ranges, which can act as a barrier against malicious inputs.
- Error Handling: Avoid displaying raw error messages to end users. Instead, log detailed errors for developers while showing user-friendly error messages to the end user. Raw messages might contain sensitive information that could help attackers.
- Database Permissions: Regularly review and adjust database permissions by only allowing necessary operations for each user role. Limiting capabilities can reduce the potential impact of a successful SQL injection.
By prioritizing security, you not only protect against unauthorized access and data breaches but also build trust with users. Security in database management might seem like a daunting task, but taking proactive steps can significantly lower risk and improve overall application integrity.
Closing the Connection
In the realm of software development, particularly when dealing with databases, understanding how to properly close connections is not just a best practice, but a fundamental aspect of responsible programming. Leaving database connections open after their use can lead to a myriad of issues, ranging from memory leaks to performance bottlenecks. The significance of this topic cannot be understated—effectively managing connections not only safeguards resources but also bolsters application performance and stability.
Importance of Closing Connections
When a connection to a MySQL database is established, the system allocates resources, which can include memory and handles. If these connections are not closed, they remain allocated even if they are no longer needed, which can lead to a situation commonly referred to as a "connection leak." This is dangerous as it can exhaust the pool of available connections, ultimately leading to degraded performance or even application crashes.
Moreover, unclosed connections can interfere with transaction integrity and data consistency, which is paramount when multiple users or processes interact with a database simultaneously. By closing connections after their use, you ensure that resources are returned promptly, allowing other operations to utilize them, thus enhancing overall application throughput.
To sum up, here are some key takeaways regarding the importance of closing database connections:
- Resource Management: Reduces the chances of memory leaks and keeps resource allocation in check.
- Performance Enhancement: Allows the application to scale efficiently by freeing up connections for other processes.
- Data Integrity and Security: Minimizes risks associated with data corruption and unauthorized access.
How to Properly Close Connections
Closing database connections in Java is straightforward, yet it requires careful implementation to avoid common pitfalls. The best approach is to always close connections in a block to ensure resources are released even if an exception occurs. Here’s a generic example to illustrate this:
In this code snippet, the connection is established and used within the block. Importantly, the block ensures that the connection is closed gracefully, even if an exception disrupts the flow.
Additionally, using a statement, introduced in Java 7, can simplify this process:
In this modern approach, the connection is automatically closed when the try block completes, eliminating the need for a separate close call. This not only reduces code verbosity but also reinforces good practices around resource management.
By following these guidelines, you can ensure that your application remains performant and stable. As the saying goes, "a stitch in time saves nine." In the context of database connections, a small effort to close connections can save much larger headaches down the line.
Testing and Debugging Database Connections
Ensuring a reliable connection between Java applications and MySQL databases is crucial for the smooth operation of any system. Without proper testing and debugging, applications can encounter unexpected failures, leading to downtimes or data inconsistencies. This section explores various methods and approaches that software developers can adopt to ensure their database connections are functioning as intended.
Strategies for Testing Connections
Testing database connections isn’t just about checking if everything is up and running. It’s about ensuring that the connection is stable, backed by sound performance metrics. Here are some strategies:
- Connection Health Checks: Regularly monitoring the state of your connections allows for early detection of any anomalies. You can implement a simple heart-beat mechanism that pings the database at set intervals to ensure it’s alive.
- Use Connection Pools: Leveraging a connection pool can also enhance testing practices. Pools manage multiple connections and can efficiently report their statuses. Testing connections from a pool helps identify leaky or dead connections swiftly.
- Unit Tests: Building unit tests that incorporate connection verification as part of the testing suite can be invaluable. Using frameworks like JUnit along with DBUnit can streamline the process of testing interactions with your database.
- Simulating Load: Conducting load testing can reveal how the application behaves under stress. Tools like Apache JMeter can simulate multiple connections and concurrent requests to help uncover performance bottlenecks.
Debugging Connection Issues
While establishing connections is one hurdle, the real challenge often lies in debugging when issues arise. Understanding how to troubleshoot effectively can save valuable time. Below are some techniques and considerations:
- Error Logging: Building comprehensive logging around your connection logic will assist in identifying problems. Capture exception messages and stack traces which can provide insights into why a connection failed. For example, checking if the port is blocked or if the credentials were incorrect.
- Reviewing Configuration: Often, the root cause of connection problems stems from misconfigured settings. Double-check the connection URL, user credentials, and JDBC driver specifics. Even a small typo can throw a wrench in the works.
- Network Diagnostics: Sometimes, the problem isn’t with your application but with network issues. Utilizing tools like or can help determine if there are networking issues that prevent successful connections to the database.
Remember, systematic approaches often yield better results than trial and error.
- Utilize Database Admin Tools: Tools such as MySQL Workbench can be used to validate credentials and connection strings outside of your application. They can help compare differences in behavior between a direct connection and that initiated from your code.
Future Trends in Java and Database Connections
The landscape of database connections in Java is ever-evolving. Understanding current and future trends is crucial for software developers and IT professionals aiming to maintain competitive advantages in their projects. Technologies change rapidly, and those who adapt effectively will flourish. Below, we delve into two specific trends: emerging technologies/frameworks and the evolution of database management.
Emerging Technologies and Frameworks
In recent years, we've seen a surge in technologies that facilitate interaction between Java applications and databases. Frameworks such as Spring Boot and Hibernate have gained traction, simplifying the development process while enhancing functionality. Here are some notable trends:
- Microservices Architecture: This pattern allows applications to be built as a collection of loosely coupled services. It's becoming the norm to use database per service, leading to improved performance and easier scalability.
- Reactive Programming: Increasingly popular with frameworks like Project Reactor and RxJava, reactive programming can handle asynchronous data streams effectively. This approach promotes a more efficient way of managing data, allowing developers to respond to changes in real-time.
- NoSQL Databases: The rise of NoSQL databases like MongoDB and Cassandra complements traditional relation databases. These databases offer flexible schemas and horizontal scaling, making them attractive for modern applications that require speed and adaptability.
"Keeping an eye on the emerging technologies can drastically improve the efficacy of a Java application’s interaction with databases."
These frameworks not only streamline development but also adapt to the changing needs of big data processing and microservices architectures, enhancing overall performance.
The Evolution of Database Management
Database management is undergoing a significant transformation driven by advances in technology and changing user needs. Key points to consider include:
- Cloud Databases: Platforms like Amazon RDS and Google Cloud SQL are shifting the focus from on-premise solutions to cloud-based alternatives. This not only increases accessibility but reduces the burden of maintenance for developers.
- Automated Database Management: With the growing use of AI and machine learning, automated systems can now optimize performance and manage updates with minimal human intervention. This trend reduces the risk of human error and frees developers to focus on innovation, rather than maintenance.
- Data Governance and Security: As more data breaches occur, the focus on security has intensified. Database solutions are now incorporating advanced security protocols and auditing features, necessary to protect sensitive information.