Mastering Variables in CoffeeScript for Developers
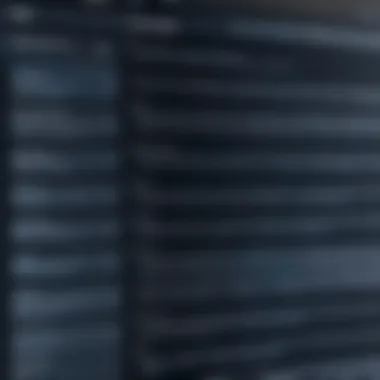
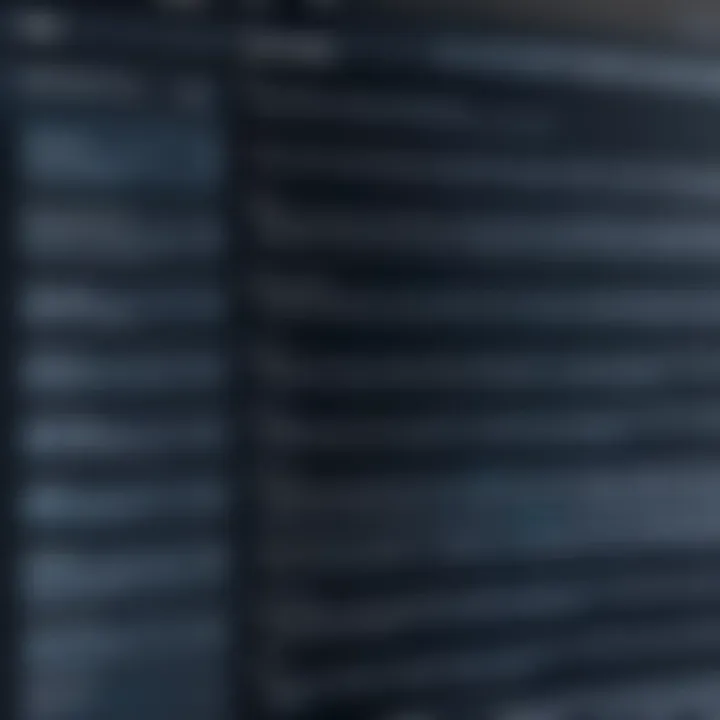
Intro
CoffeeScript is a programming language that simplifies JavaScript syntax, making it more concise and accessible for developers. The handling of variables in CoffeeScript is crucial to grasping the language's full potential. Understanding how to declare variables, their scope within code, and their types is fundamental for anyone looking to write more effective CoffeeScript. This article aims to provide a detailed examination of variables in CoffeeScript, offering insights into best practices and common pitfalls that developers may face.
Overview of CoffeeScript Variables
In essence, variables in CoffeeScript act as named storage for data. They enable developers to reference, modify, and manipulate data efficiently. The language's syntax makes variable declaration seamless and intuitive, which is a significant reason why many developers choose to adopt CoffeeScript.
Definition and Importance of Variables
Variables are vital in all programming languages. They are the groundwork for storing information, which can later be processed. In CoffeeScript, variables serve this function while also contributing to readability and maintainability of code. Well-managed variables can lead to less confusion and more robust applications.
Key Features of CoffeeScript Variables
- Simplicity: Variable declaration in CoffeeScript is straightforward. Unlike JavaScript, there is no need to specify , , or . Just use the variable name and assign a value.
- Scope Control: CoffeeScript allows easy management of variable scope. Understanding whether a variable is global or local can prevent many issues.
- Dynamic Typing: CoffeeScript handles types dynamically, allowing types of variables to change during runtime, which provides flexibility.
Use Cases and Benefits
The effective use of variables in CoffeeScript leads to better coding practices. For example, by using local variables, developers can avoid polluting the global scope, leading to fewer errors. Additionally, dynamic typing allows developers to build applications rapidly without being bogged down by rigid type restrictions.
Best Practices
To leverage the full potential of CoffeeScript variables, following industry best practices is essential.
Industry Best Practices
- Consistency: Always use clear and consistent naming conventions. It enhances code readability and makes future modifications easier.
- Limit Global Variables: Minimize the use of global variables. Instead, utilize local variables within functions to enhance encapsulation.
- Comments: Document important variable declarations with comments. This will help others (and your future self) understand the intent behind certain variable choices.
Tips for Maximizing Efficiency
- Use let for defining block-scoped variables if you're transitioning from CoffeeScript to ES6 features.
- Organize your variables logically in the code. Group related variables together, which aids in comprehension.
Common Pitfalls to Avoid
- Reassigning global variables unintentionally can lead to unexpected outcomes. It is crucial to be aware of which variables are in the global scope.
- Neglecting to initialize variables can cause reference errors. Always ensure a variable has a value before it is used.
"Utilizing variables correctly in CoffeeScript allows developers to write more efficient and manageable code."
Case Studies
Real-world examples can illuminate the impact that effective variable management has on development.
- Project X implemented CoffeeScript for a web application. The team adopted strong variable-naming conventions. As a result, they experienced a 30% reduction in development time.
- Company Y struggled with variable scope issues in their legacy JavaScript code. Transitioning to CoffeeScript with well-defined variable scopes helped them reduce bugs by 50%.
Latest Trends and Updates
The world of CoffeeScript is continuously evolving. Staying up-to-date with the latest trends is essential for any developer.
Upcoming Advancements
The upcoming CoffeeScript 2 update introduces several enhancements in variable declarations along with ES6 compatibility improvements. These changes aim to bridge gaps between CoffeeScript and modern JavaScript.
Innovations and Breakthroughs
Emphasis on compiler efficiency in new versions allows for quicker runtime performance. This leads to improved user experience in applications developed with the language.
How-To Guides and Tutorials
To fully utilize CoffeeScript variables, following step-by-step tutorials can be beneficial.
Step-by-Step Guide for Using Variables
- Declaring Variables: Simply state the variable name, followed by an assignment.
- Understanding Scope: Use functions to create local variables.
- Avoiding Common Mistakes: Ensure to declare all variables with proper initialization, avoiding reference errors during execution.
Practical Tips
For beginners, practice declaring variables in a simple project. This reinforces understanding and builds confidence in using CoffeeScript effectively.
Understanding Variables in CoffeeScript
Variables are central to programming. A solid grasp of how variables function is critical for any developer working in CoffeeScript. CoffeeScript enhances the JavaScript development experience with a more streamlined syntax. Understanding how variables work within this framework not only aids in writing elegant and efficient code but also minimizes potential errors.
Moreover, recognizing differences in variable management between CoffeeScript and its predecessor, JavaScript, can lead to better programming practices. It is essential to pay attention to how variables are declared, initialized, and utilized throughout an application. This knowledge empowers developers to leverage CoffeeScript's features effectively, fostering a smoother development process.
Definition of Variables
In programming, a variable serves as a symbolic name for a value. This value can change during the execution of a program. In CoffeeScript, like in other languages, variables hold data that can be manipulated through various operations. The definition is straightforward: a variable is created by defining a name, followed by an assignment of a value.
For instance, when you write , you are creating a variable named . The assignment operator () establishes a link between the name and the data stored in it. Variables can store different types of data, such as numbers, strings, and objects. This versatility is a fundamental aspect of programming.
Differences Between CoffeeScript and JavaScript Variables
When comparing CoffeeScript and JavaScript variables, several crucial differences stand out. First, CoffeeScript simplifies variable declarations. In traditional JavaScript, you would need to specify , , or before a variable name. In CoffeeScript, declaring a variable does not require such keywords. Instead, simply writing suffices.
Additionally, the handling of scope is more intuitive in CoffeeScript. Local variables are automatically scoped to the nearest enclosing function or block. This behavior reduces clutter and potential errors related to variable hoisting found in JavaScript. Consequently, developers may find it easier to manage variables effectively.
Variable Declaration
Variable declaration is a critical aspect of programming in CoffeeScript. Understanding how to properly declare variables not only enhances code clarity but also affects how the program functions. In CoffeeScript, the way variables are handled allows developers to write concise code while preserving readability. This section will focus on the elements involved in variable declaration, benefits, and various considerations that developers should keep in mind.
Using 'var', 'let', and 'const'
In CoffeeScript, the keywords 'var', 'let', and 'const' are essential for variable declaration. They define the variable’s scope and its mutability. While 'var' is used mostly for declaring variables that can be re-assigned, 'let' provides block-scoping. This means that a variable declared with 'let' within a block, such as an if statement or a loop, cannot be accessed outside that block. It enhances maintainability by limiting variable visibility.
On the other hand, 'const' is used for declaring constants. It ensures that once assigned, a variable cannot be re-assigned, which can prevent accidental changes to values that need to remain constant throughout the code.
Here is a simple example illustrating the use of these keywords:
In this example, 'counter' can be changed, 'increment' can update the counter, but 'maxCount' cannot be altered after its initial assignment. Choosing between these keywords can affect how your variables behave and how easily the code can be understood.
Implicit Variable Declaration
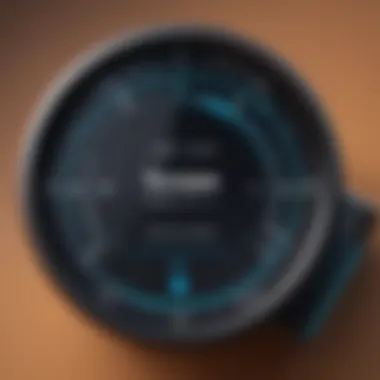
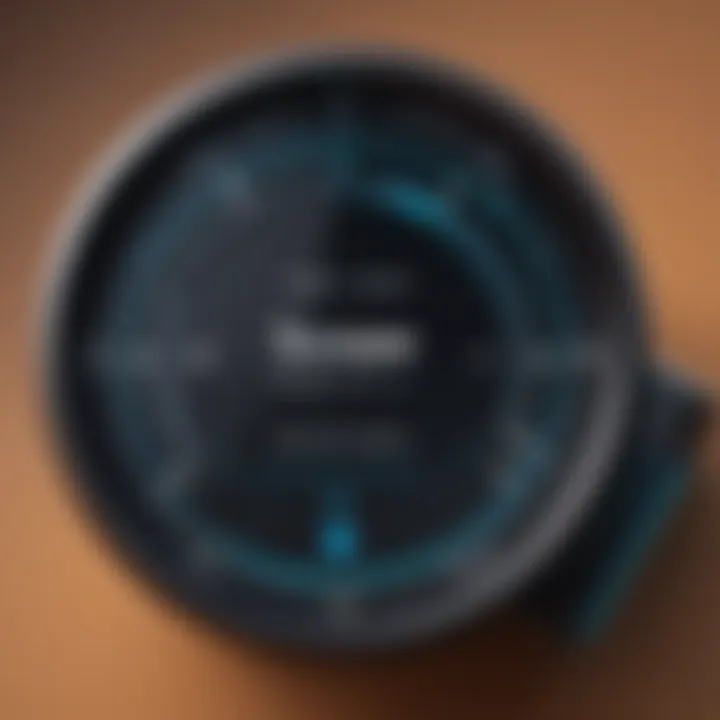
CoffeeScript simplifies variable declaration through implicit assignment. If a developer does not specify a keyword when defining a variable, CoffeeScript automatically treats it as a variable in the current scope. This is useful for quick prototyping or when clarity is maintained in the code. However, it is important to note that this may lead to unintended consequences, especially for developers who might come from languages that do not allow implicit declarations.
For example, the following code will automatically create a variable within the current scope:
While such shorthand can be beneficial, it can also result in ambiguity in larger codebases where variable scope might not be immediately clear. Developers must use this feature judiciously to maintain code readability.
Implicit variable declaration can be a double-edged sword: deeply useful but potentially dangerous if not understood properly.
Variable Types in CoffeeScript
Understanding variable types in CoffeeScript is essential for any developer wanting to maximize the language's potential. CoffeeScript introduces unique approaches to variable management, differing somewhat from the parent language, JavaScript. By categorizing variables into primitive and complex types, developers can handle data more effectively, aligning with the requirements of their applications. This section will explore both types, emphasizing their significance and practical applications.
Primitive Types
Primitive types in CoffeeScript are the simplest forms of data. They are immutable and include values such as numbers, strings, booleans, null, and undefined. Understanding these types is foundational as they often serve as building blocks for more complex data structures.
- Numbers: CoffeeScript treats numbers as either integers or floating-point values. This flexibility allows for seamless arithmetic operations without needing extra syntax. For example, is a simple declaration.
- Strings: Represented in single or double quotes, strings are vital for managing text within your code. For instance, is straightforward and effective.
- Booleans: These values only hold true or false. They are crucial in control structures and logical expressions. An example is .
- Null and Undefined: Null signifies the intentional absence of any value. Undefined occurs when a variable is declared but not initialized. This distinction holds importance for debugging or detecting errors in your code.
In this context, the understanding of primitive types aids in creating cleaner and more robust applications. Knowing when to use each type can greatly affect the efficiency of your code.
Complex Types
Complex types in CoffeeScript encompass a wider variety of data structures that hold collections of values or more intricate data than primitives. They are mutable, meaning their values can change after initialization. This aspect adds versatility to variable handling.
- Arrays: Arrays are ordered lists of values. CoffeeScript arrays can hold multiple types, which means you can create mixed-type collections easily. For example, showcases this flexibility.
- Functions: Function expressions can also be treated as first-class variables in CoffeeScript. This allows functions to be passed around as arguments, returned from other functions, or assigned to variables, enriching the language's expressiveness.
In summary, while primitive types serve as the foundation of data handling, complex types enable developers to build intricate systems that mirror real-world logic. Understanding how to use both types proficiently is key for any CoffeeScript developer, enhancing the capacity to create efficient, maintainable code.
Scope of Variables
In a programming language like CoffeeScript, the concept of variable scope is fundamental. It dictates where a variable can be accessed within the code. Understanding variable scope can help developers prevent errors and manage code efficiently. Variables can have different scopes, such as global, local, or function. Each scope dictates its visibility and lifecycle.
Global Scope
Global scope means that a variable can be accessed from any part of the code. In CoffeeScript, any variable declared outside of functions or blocks is considered global. This can be beneficial for variables that need to be accessed widely across files or modules. However, relying too heavily on global variables can lead to conflicts and unintended side effects, especially in larger applications.
When you use a global variable, be cautious. If multiple scripts modify the same global variable, it may lead to confusion and bugs that can be difficult to trace. To avoid collisions, it's advised to use well-defined naming conventions to clearly convey the purpose of each global variable.
Local Scope
Local scope defines the access of variables only within a particular function or block. These variables are created with 'var', 'let', or 'const' and cannot be accessed outside the function or block they reside in. This encapsulation is useful, as it prevents pollution of the global namespace and reduces the risk of variable conflicts.
Using local variables can lead to cleaner and more maintainable code. It also improves the performance as local variables are garbage collected more efficiently. Therefore, when declaring variables, consider whether they should be restricted to local scope to enhance your code organization and reduce potential errors.
Function Scope
Function scope is a specific subset of local scope, focusing on variables defined within a function. These variables exist only during the function execution and are erased once the function completes. If a variable is declared within a function, it cannot be accessed from outside that function. This characteristic is crucial when you want to encapsulate logic without exposing intermediate variables.
Understanding function scope is particularly important when dealing with closures in CoffeeScript, where an inner function maintains access to the variables of its outer function even after that function has completed execution. This can enhance flexibility but also requires caution, as overusing closures can result in memory issues.
Proper management of variable scopes can greatly enhance your coding experience, making your applications more robust and reducing the likelihood of errors.
Variable Initialization
Variable initialization is a core concept in CoffeeScript that dictates how variables are assigned initial values. Both in programming generally and in CoffeeScript specifically, the way a variable is initialized can influence its utility, behavior, and longevity within a program. Understanding this is essential for developers who want to ensure their code is efficient, clear, and error-free. An effectively initialized variable can prevent many common programming issues, making the understanding of this concept vital for anyone working in the language.
In CoffeeScript, variables can be initialized via two primary methods: default initialization and dynamic initialization. Each method has its particular benefits and applications, and knowing when to apply each is crucial for effective programming.
Default Initialization
Default initialization refers to assigning a variable a value at the moment of its creation. In CoffeeScript, this tends to be done by directly assigning a value when declaring a variable. For example:
This line creates a variable named and initializes it with a value of . The significance here is that developers can easily predict the state of after this line executes. It creates a clear starting point, which is beneficial for readability and debugging. This makes it easier to reason about what represents in further code, as its initial value is predetermined.
Default initialization also helps to avoid issues related to undefined variables. For instance, if a variable is used without an explicit initialization, it may lead to unintended behavior or runtime errors. This makes strong initialization practices important. Additionally, it allows developers to utilize constants within code, improving maintainability over long projects where variable assignment patterns may change over time.
Dynamic Initialization
Dynamic initialization, in contrast, involves assigning a variable's value based on external factors or computations that occur during runtime. This offers flexibility as values can be set based on user input, data retrieved from a database, or the results of complex operations. For example:
In this case, is initialized with a value that may vary according to different user input scenarios, making it vital for applications that must adapt to user data. While dynamic initialization provides versatility, it can also introduce complexity. Variables initialized dynamically can lead to unpredictable states if the external factors change frequently or unexpectedly, creating challenges in debugging and maintenance.
Effective variable initialization helps eliminate many common coding errors. Always consider the implications of how a variable obtains its value and the potential need for both clarity and flexibility in your code.
Best Practices for Variable Usage
The effectiveness of any programming language is largely influenced by how variables are managed. In CoffeeScript, adopting best practices for variable usage is essential to maintain clean, maintainable, and efficient code. Variables form the backbone of any program, enabling both flexibility and the potential for errors. Therefore, it is crucial to understand and implement practices that optimize their use. This section explores two important components of variable management: clear naming conventions and minimizing variable scope.
Clear Naming Conventions
Utilizing clear naming conventions is vital in ensuring code readability and maintainability. A well-named variable can instantly reveal its purpose and expected usage. This practice not only benefits the developer writing the code but also anyone who may work with that code in the future.
Here are some guidelines for clear naming:
- Descriptive Names: Choose names that reflect the content or purpose of the variable. For instance, is more informative than simply .
- Use Camel Case: In CoffeeScript, camel case (such as ) helps differentiate between words without spaces. This is a common practice that enhances readability.
- Avoid Abbreviations: While abbreviations might seem convenient, they often add confusion. It’s better to use full words to ensure clarity.
- Be Consistent: Maintain a consistent naming pattern throughout the codebase. This uniformity aids both current developers and any future collaborators in understanding the code.
"Clearer names help prevent misunderstandings and reduce the cognitive load on developers reading the code."
Minimizing Variable Scope
Minimizing variable scope is another key practice that enhances code quality. By limiting the accessibility of variables, developers can reduce the risk of unintended side effects and conflicts. When variables are too broadly scoped, they can unintentionally interfere with other parts of the code.
Here are some methods to minimize variable scope effectively:
- Declare Variables Locally: Always declare variables as locally as possible. For example, if a variable is used only within a specific function, declare it within that function rather than globally.
- Use Blocks: CoffeeScript encourages the use of blocks to wrap variables, which helps keep their scope limited. This is especially useful in loops and conditionals.
- Avoid Global Variables: Global variables are accessible throughout the code and can lead to naming conflicts and unexpected behavior. Try to limit their use to only those cases where it is absolutely necessary.
Common Pitfalls with Variables
Understanding the pitfalls associated with variables in CoffeeScript is crucial for developers aiming to write clean and efficient code. As with any programming language, mistakes in variable handling can lead to bugs that are often difficult to track down. Being mindful of these common mistakes can significantly improve code quality, maintainability, and efficiency. Therefore, it’s essential to address the pitfalls of variable hoisting and variable shadowing as they directly impact how you manage scope and state in your applications.
Variable Hoisting
Variable hoisting refers to the behavior where variable declarations are moved to the top of their containing scope during the compilation phase of JavaScript. In CoffeeScript, because it compiles to JavaScript, developers must be aware of this phenomenon, as it can lead to unexpected results.
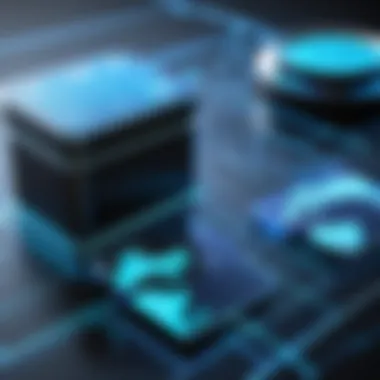
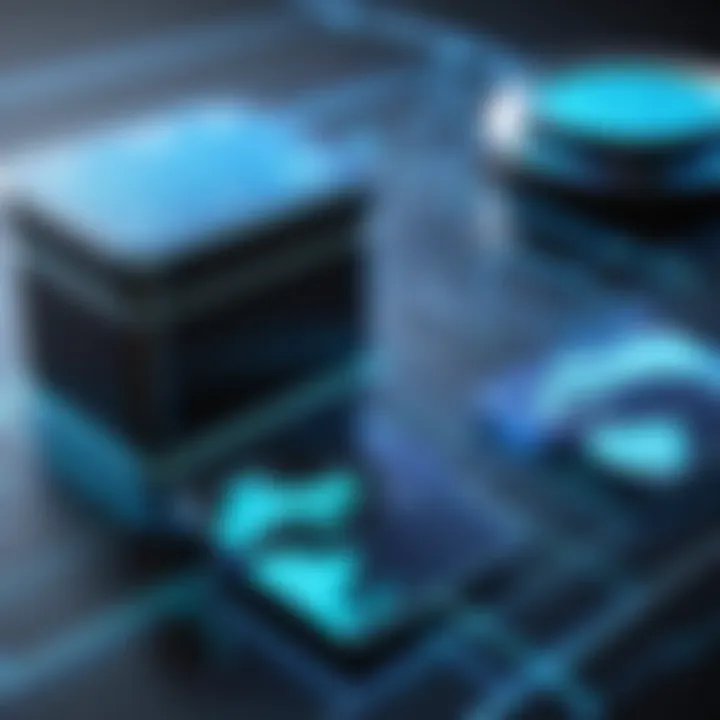
For example, consider the following code snippet:
In this case, "Hello, World!" will not be logged as expected. Instead, one would typically get an output. This happens because the declaration of is hoisted but not the assignment.
When writing CoffeeScript, a developer must always remember that while variables can technically be accessed before they are defined, their value at that point will be . This can cause bugs that are particularly troublesome during debugging. To mitigate such issues:
- Always declare variables at the top of their scope.
- Use clear logic to define variable lifecycles.
- Prefer using or over where possible, since they do not exhibit hoisting behavior in the same way.
Shadowing Variables
Shadowing occurs when a variable declared in a certain scope has the same name as another variable declared in an outer scope. This may lead to confusion, especially for new developers, as it can create ambiguous situations about which variable is being referred to at different points in the code.
For instance:
In this example, the within the if statement shadows the global . When the first is executed, it will output "I am inside," while the second will show "I am outside."
To avoid shadowing issues, consider the following practices:
- Use unique variable names that convey their intent or function clearly.
- Limit the use of similarly named variables within nested scopes.
- Regularly refactor code to clarify variable scope and reduce complexity.
CoffeeScript Compilation and Variables
In the context of CoffeeScript, understanding how variables are compiled into JavaScript is pivotal. CoffeeScript is designed to be a more human-readable alternative to JavaScript. However, behind the scenes, it is converted to JavaScript for execution. This compilation process can influence how variables are treated in your code, impacting their functionality and behavior in various scenarios. Knowing the nuances of this conversion is essential for developers aiming to write efficient CoffeeScript code that performs well when compiled.
How Variables are Compiled to JavaScript
When you declare variables in CoffeeScript, the compiler translates them into JavaScript equivalents. For instance, a CoffeeScript variable declared using the keyword becomes similar to how JavaScript handles variable scoping. Here is a simple example:
This line in CoffeeScript compiles to:
The translation retains the variable name and its assigned value but adheres to JavaScript syntax rules. This process ensures that CoffeeScript maintains compatibility with existing JavaScript environments while enabling a more concise and readable syntax for developers.
It is also worth noting that CoffeeScript introduces features that simplify variable declarations and improve readability, such as implicit variable declaration. This means that you can create variables without explicitly using , , or . However, these implicit declarations still get compiled into their JavaScript counterparts.
Impact of Compilation on Variable Behavior
The compilation from CoffeeScript to JavaScript can significantly affect variable behavior. First, the scope of the variables is crucial to grasp. Since CoffeeScript utilizes a different approach to scoping, developers need to be aware of how their variable declarations translate. For example, an implicitly declared variable in CoffeeScript may lead to unintended global variables, which can create confusion or bugs in larger codebases.
Moreover, due to optimizations during compilation, certain behavior may vary between CoffeeScript and JavaScript. Consider the following:
- Variable Hoisting: In JavaScript, variable declarations are hoisted. CoffeeScript attempts to reflect this in its design. Understanding how hoisting works can prevent potential runtime errors.
- Shadowing Variables: CoffeeScript allows for cleaner syntax, but it still retains JavaScript’s behavior regarding variable shadowing. Knowing how these variables interact post-compilation helps maintain code clarity.
The way CoffeeScript compiles to JavaScript can introduce subtle changes in how variables behave, making it essential for developers to understand these differences.
By examining the compilation of variables and their implications on behavior, developers can make informed decisions while coding in CoffeeScript, ensuring that they achieve the intended logic without unexpected outcomes.
Real-world Applications of Variables
The significance of understanding real-world applications of variables in CoffeeScript cannot be overstated. Variables serve as the backbone of programming logic, functioning as storage for data that influences the execution and flow of applications. This section will elucidate specific contexts where variables shine in the realm of frontend and backend development. Each context illustrates how CoffeeScript's unique handling of variables enhances productivity and code maintainability.
Variables in CoffeeScript for Frontend Development
In frontend development, variables are crucial for managing user interactions and dynamic content on the web. CoffeeScript simplifies the syntax compared to JavaScript, making it easier for developers to work with variables. This simplicity allows for faster prototyping and iteration.
Key applications include:
- DOM Manipulation: Variables store references to DOM elements, enabling seamless updates and interactivity. For example:
- Event Handling: Variables are used to manage state and behavior in response to user actions. For instance, form validation can utilize variables to check inputs before submission.
- Data Binding: In frameworks like Backbone.js or Angular.js, variables are linked to UI elements, creating a dynamic interface that reflects the current data state.
Frontend developers benefit from CoffeeScript’s variable usage as it encourages a cleaner codebase, making the application easier to maintain and extend over time.
Variables in CoffeeScript for Backend Development
For backend development, variables play a pivotal role in data management and server-side logic. CoffeeScript's succinct syntax means that backend developers can write less boilerplate code, enhancing focus on application logic.
Some common uses involve:
- Database Interactions: Variables hold relevant data fetched from databases, which can then be processed or transformed. For example:
- Asynchronous Programming: Variables control the flow of asynchronous operations, facilitating smooth execution in Node.js environments.
- APIs and Web Services: They are utilized to store and manipulate request parameters and responses, making data exchange straightforward and efficient.
Understanding how to utilize variables effectively in backend development ensures that applications can handle complex logic while remaining performant. It also supports scalable architecture, which is vital as applications grow.
"Mastering variables in CoffeeScript enhances your coding efficiency, making it an invaluable skill for both frontend and backend developers."
By leveraging CoffeeScript's handling of variables, developers can create effective, maintainable applications that adapt to changing requirements efficiently.
Debugging Variables in CoffeeScript
Debugging variables is a critical aspect of programming in CoffeeScript. Variables are at the heart of any program's functionality, serving as containers for data manipulation. Understanding how to debug these variables can lead to more efficient code and fewer errors.
Correct identification of variable issues can save significant time and effort in the development process, leading to better software performance. When developers encounter problems, knowing how to pinpoint issues in variable assignments or scope is essential.
In CoffeeScript, effective debugging techniques can help in understanding variable behavior much better than standard print statements might achieve. Using specialized tools can enhance the debugging process, allowing for more precise tracking of what variables contain at any given point in time and their interactions within the program's scope.
Identifying Variable Issues
Identifying variable issues involves recognizing when a variable does not hold the expected value or behaves incorrectly. Common signs include unexplained errors, unexpected output, or application crashes. This may stem from reasons like:
- Improper initialization or assignment
- Scope conflicts
- Unintentional overwriting of variable values
- Misuse of types, such as assigning a string to a variable that is expected to be a number
Developers should start by reviewing variable assignments and ensuring there are no conflicting identifiers. Variable hoisting can sometimes lead to confusion, especially for those transitioning from JavaScript. Therefore, a keen eye helps to catch misplaced variables or scope mishaps.
Tools for Debugging Variables
Various tools can assist in debugging to streamline the identification of variable issues. Here are some effective ones used in CoffeeScript:
- Browser Developer Tools: Nearly all modern browsers come equipped with developer tools that allow inspection of variable values at runtime, helping to track down issues interactively.
- Node.js Debugger: When running CoffeeScript on Node.js, it can be beneficial to use its built-in debugger. This experience is especially useful for navigating and testing backend code, offering command-line advantages.
- CoffeeScript Compiler: Using the CoffeeScript compiler's setting gives insight into how CoffeeScript translates to JavaScript. Understanding this compiled output can help clarify potential issues between the CoffeeScript and JavaScript-mediated execution.
- Logging: While not a formal tool, logging certain variables at key points in the code can provide context during execution and help expose errant behaviors.
In sum, paying attention to variable issues and using the appropriate debugging tools fosters a better environment for writers of CoffeeScript, resulting in cleaner, more reliable code.
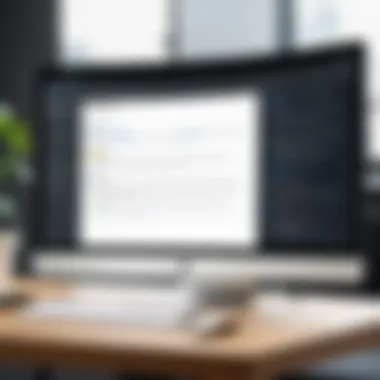
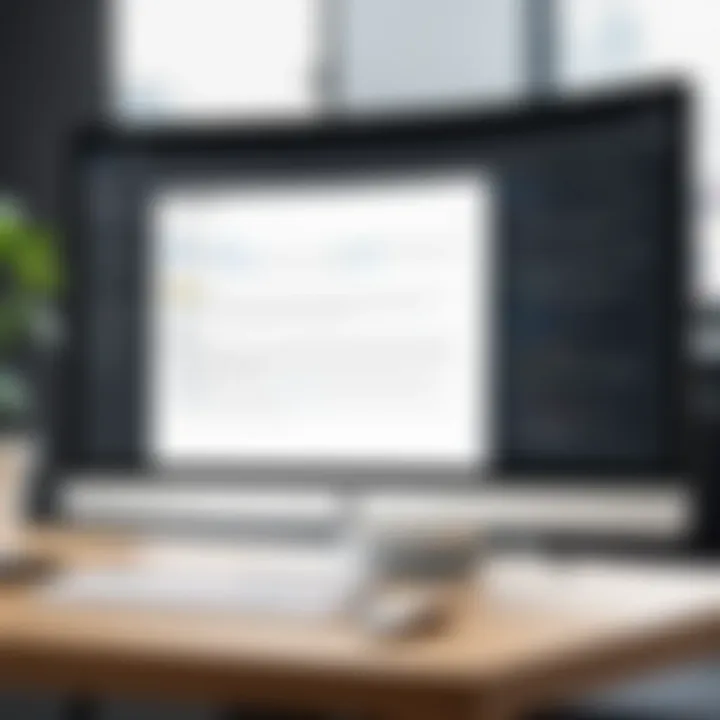
Advanced Variable Concepts
Understanding advanced variable concepts is essential for developers looking to maximize their efficiency within CoffeeScript. These concepts delve deeper into how variables can be utilized within more complex structures, such as classes and constants. Grasping these elements not only optimizes code but also enhances maintainability and readability.
Constants and Their Usage
Constants in CoffeeScript are a powerful tool that helps developers create variables whose values remain unchanged after being assigned. By using constants, developers can avoid accidental modifications which may lead to software bugs. In CoffeeScript, constants are declared using the keyword, signaling to the reader of the code that the value will not vary.
The use of constants can be particularly beneficial in various scenarios:
- Configuration settings: When certain values need to be consistent across the application (e.g., API URLs).
- Magic numbers or strings: To ensure clearer code, constants can replace hard-coded values.
- Improved readability: Constants give context to values, making the code more descriptive.
Here’s an example of a constant declaration in CoffeeScript:
Using constants effectively not only facilitates easier debugging but also safeguards crucial values throughout the development process.
Using Variables in Classes
Classes in CoffeeScript are syntactic sugar on top of JavaScript's prototype-based inheritance. Variables within classes serve distinct roles, distinguishing between instance variables and class variables. Understanding this distinction helps in structuring more robust software designs.
When using variables in classes, developers can define:
- Instance variables: Declared within the constructor, these variables are specific to each object created from the class. For example:
- Class variables: Defined with a symbol and shared among all instances. This approach supports scenarios where a property needs to be common across all object instances.
Employing variables in classes allows for encapsulated data. This structure aids in better organization of code and enhances its scalability by preventing unwanted access or modifications.
In summary, incorporating constants and structured variable use within classes forms a foundation for effective CoffeeScript coding practices. This understanding facilitates the construction of cleaner, more maintainable code, aligning with the evolving demands of software development.
Performance Considerations
Performance considerations are crucial for any programming language, and CoffeeScript is no exception. As a language that compiles to JavaScript, understanding how variables impact performance is essential for writing efficient code. This section emphasizes two main aspects: memory management of variables and the direct influence of variable usage on performance. By being aware of these factors, developers can optimize their CoffeeScript applications for better performance and resource utilization.
Memory Management of Variables
In CoffeeScript, memory management plays a vital role in maintaining the performance of applications. Memory is allocated for variables when they are declared. Understanding how and when these variables are created and destroyed is critical.
- Garbage Collection: CoffeeScript runs on the JavaScript engine, which has built-in garbage collection. This process automatically frees memory that is no longer in use. However, relying solely on garbage collection can lead to memory leaks if variables are not managed properly. Developers should avoid keeping unnecessary references to objects that should be released.
- Variable Scope: The scope of a variable determines its lifetime and accessibility. Variables declared within a function are eligible for garbage collection once the function execution is complete. In contrast, global variables persist for the life of the application and can lead to increased memory usage. It's important to minimize the use of global variables and keep the scope of variables as limited as possible.
- Primitive vs. Complex Types: Primitive types like numbers and strings use less memory compared to complex types like objects and arrays. Using primitive types when possible can lead to better memory performance. Developers should assess the needs of their application and choose variable types accordingly.
Impact of Variable Usage on Performance
The way variables are used impacts both the performance and the efficiency of a CoffeeScript application. Here are the factors that developers should consider:
- Variable Initialization: Proper initialization of variables affects performance. Uninitialized variables might lead to undefined behavior which can degrade performance during runtime. Initializing variables at the time of declaration helps ensure that they have a predictable and stable state.
- Scope Management: Using local variables instead of global variables reduces the chance of name conflicts and enhances performance. Global variables can slow down applications due to constant lookups, whereas local variables are generally faster as they reside in the stack memory of functions.
- Frequent Updates: Variables that change frequently can create overhead. If performance is critical, developers should control how often and how much variables are updated. This is especially important for inner loops in algorithms that require fast execution.
"Optimizing variable usage in CoffeeScript can lead to substantial performance improvements, significantly enhancing overall application efficiency."
- Profiling Tools: Using tools to profile performance can help identify bottlenecks related to variable usage. Tools specific to JavaScript, such as Chrome DevTools and Node.js profilers, can provide insights into memory consumption and variable lifetimes in CoffeeScript applications.
By understanding and managing these performance considerations, developers can optimize their CoffeeScript applications, improving both execution speed and resource management.
Comparison with Other Languages
In programming, comprehension of variables across various languages is essential. By examining how variables function in CoffeeScript compared to other languages, developers can grasp the unique features and potential pitfalls of CoffeeScript. This understanding enhances a developer's ability to write efficient code and leverage language-specific functionalities. It also fosters adaptability when shifting between languages. With each language having its peculiarities, developers often benefit from recognizing these differences, leading to better programming practices and reduced errors.
Variables in Python vs. CoffeeScript
Python is often celebrated for its readability and simplicity. Variable declaration in Python is straightforward; there is no need for explicit declarations using keywords like , , or . Instead, developers can assign a value to a variable directly. In CoffeeScript, while the banality of variable declaration has improved, it follows a more structured format.
Key Comparisons Between Python and CoffeeScript:
- Declaration: In Python, variables are implicitly declared upon assignment, while CoffeeScript benefits from traditional declaration keywords.
- Type Flexibility: Both languages support dynamic typing, but CoffeeScript's compilation to JavaScript can introduce different type behavior.
- Scope Management: Python employs function scope, whereas CoffeeScript emphasizes a more robust scope management system. This affects how variables behave in nested functions.
Here is a small code demonstration:
Both examples achieve the same goal in their respective languages, yet the way variables are handled reflects the languages' philosophies. Understanding these distinctions is valuable for developers transitioning between Python and CoffeeScript, as it reveals necessary adjustments and optimizations in code structure and logic.
Variables in Java vs. CoffeeScript
Java is a statically typed language, which differs significantly from CoffeeScript's dynamic nature. In Java, each variable needs a data type defined at declaration, ensuring type safety. This strictness can help catch errors early, making code less prone to certain types of bugs.
Comparative Insights:
- Type Declaration: Java requires explicit type declarations, while CoffeeScript does not, potentially leading to complications in larger projects for the latter.
- Syntax Differences: Java uses semicolons to end statements, while CoffeeScript encourages a cleaner syntax by omitting them, leading to succinct code.
- Memory Management: With its strict type system, Java can optimize memory using the strict scope of variables, whereas CoffeeScript relies on the JavaScript engine to handle memory management post-compilation.
For instance, in Java:
In CoffeeScript:
These examples exhibit the variance in type enforcement and syntax philosophy. Understanding these differences allows developers to approach variables with a clearer mindset, potentially improving their coding efficiency as they navigate between CoffeeScript and Java.
Understanding variable behavior across languages is critical for effective programming.
Future of Variables in CoffeeScript
The future of variables in CoffeeScript is pivotal for the language’s evolution. As the programming landscape shifts, the management and application of variables in CoffeeScript will adapt to new paradigms. This section delves into the significance of enhancing variable capabilities and the implications for software development. The way variables are processed and implemented can significantly impact code efficiency and readability, ensuring developers can write cleaner and more maintainable code.
Upcoming Features and Enhancements
Anticipated features for CoffeeScript variables highlight modernization. Developers are keen to see improvements, ensuring variables are more intuitive and flexible. Some of the features expected include:
- Enhanced destructuring capabilities, making it easier to manage complex data structures.
- Support for new data types reflective of evolving programming needs.
- Improvements in error handling regarding variable declarations and scope.
These updates promise to provide greater control. Handling variable types intelligently will encourage best practices, ultimately benefiting large-scale projects.
Adapting to Trends in Development
The rapid advancement of technology necessitates continual evolution in programming languages. Variables will likely adapt to trends in functional programming and the growing demand for reactive applications.
Some considerations for adapting include:
- Implementing features promoting immutability and ensuring state management.
- Emphasizing asynchronous programming, which may affect how variables are scoped and accessed.
- Aligning with emerging frameworks, potentially leading to innovative variable handling strategies.
Staying attuned to these trends is vital for developers. It can influence the direction CoffeeScript takes, impacting its relevance in a competitive field.