Mastering the Spring Framework for Java Development
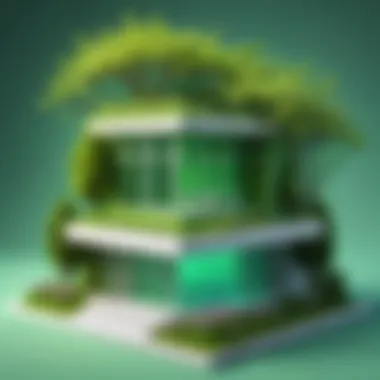
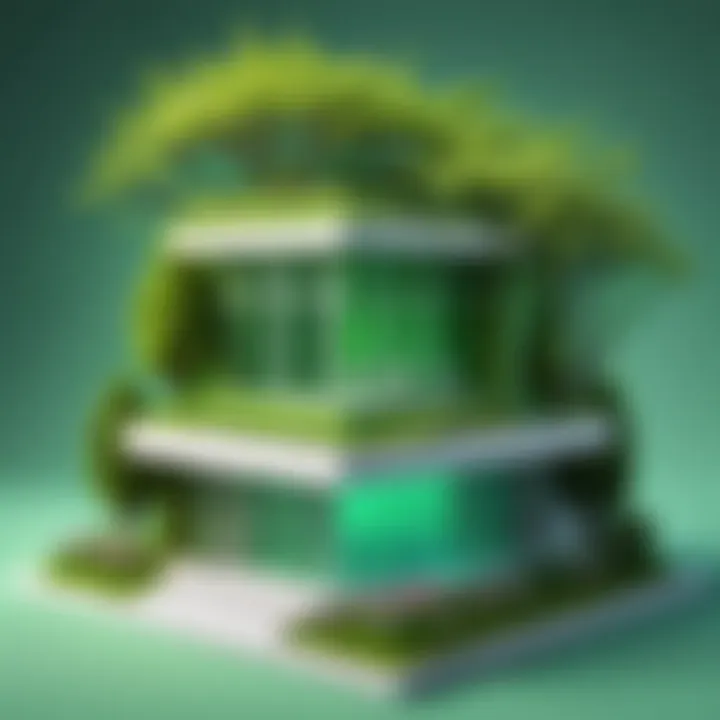
Intro
The Spring Framework represents a crucial component of Java programming. It provides a rich infrastructure to support various aspects of application development, particularly for enterprise-level projects. Understanding this framework is fundamental for modern software development. This tutorial will navigate through significant concepts such as dependency injection and aspect-oriented programming. We will unpack the utility of Spring Framework in practical scenarios and highlight best practices that developers can adopt.
Overview of Spring Framework
Definition and Importance of the Framework
Spring Framework is an open-source application framework that offers comprehensive infrastructure support for developing Java applications. It simplifies Java development and promotes good design practices. Its modular architecture allows developers to pick and choose the components they require, enhancing flexibility and reducing bloat. Its importance lies in its ability to build robust, maintainable, and scalable applications.
Key Features and Functionalities
Some of the essential features of the Spring Framework include:
- Dependency Injection: This allows for better separation of concerns and improves code testability. It helps manage object creation and dependencies effectively.
- Aspect-Oriented Programming: Spring enables developers to implement cross-cutting concerns such as logging and security efficiently.
- Data Access Integration: It offers support for various data access technologies, including JDBC, Hibernate, and JPA, simplifying database interactions.
- MVC Framework: A flexible model-view-controller framework helps in the development of web applications.
Use Cases and Benefits
The applications of the Spring Framework are robust. Common use cases include:
- Enterprise applications requiring transaction management.
- Microservices, due to its extensive support for RESTful application development.
- Rapid application development with simplified configuration using Spring Boot.
The benefits encompass lower development costs and time, due to pre-built components and frameworks that enhance productivity.
Best Practices
Industry Best Practices for Implementing Spring Framework
- Use Spring Boot: It accelerates application development by providing a range of ready-to-use features.
- Follow Layered Architecture: Organize the application into distinct layers like presentation, business logic, and data access.
- Utilize Spring Security: For securing applications, integrating this module is paramount.
Tips for Maximizing Efficiency and Productivity
- Leverage Spring's support for testing frameworks to ensure reliability.
- Use dependency injection wisely to manage dependencies cleanly.
Common Pitfalls to Avoid
- Overcomplicating configurations can hinder progress. Keep configuration manageable.
- Failing to implement best practices for exception handling leading to maintenance issues.
Case Studies
Real-World Examples of Successful Implementation
Many companies have successfully implemented Spring Framework solutions. For example, Netflix employs Spring Cloud for its microservices architecture, enhancing resilience and scalability.
Lessons Learned and Outcomes Achieved
Companies reported increased developer productivity and faster time to market. Reduced bugs and improved maintainability are other key outcomes.
Insights from Industry Experts
Experts emphasize the importance of employing the entire ecosystem Spring provides to streamline development processes.
Latest Trends and Updates
Upcoming Advancements in the Field
The Spring community is continually evolving. Recent improvements in Spring 5 underscore better support for reactive programming through WebFlux.
Current Industry Trends and Forecasts
Cloud-native development approaches are becoming more prevalent, leveraging Spring’s capabilities.
Innovations and Breakthroughs
Innovations in Spring’s programming model, like enhanced support for GraalVM for compiling Java applications, emphasize performance.
How-To Guides and Tutorials
Step-by-Step Guides for Using Spring Framework
To kickstart using the Spring Framework, one can set up a simple application using Spring Initializr. This tool helps bootstrap Spring applications quickly.
Hands-On Tutorials for Beginners and Advanced Users
Beginner tutorials provide insight into the basics of Spring projects, whereas advanced tutorials delve into specifics like security and integration with cloud services.
Practical Tips and Tricks for Effective Utilization
Regularly review the Spring documentation to keep up with best practices and new features as they become available.
The Spring Framework is not just a tool, but a powerful partner in creating robust Java applications, adapting seamlessly to technological advancements and developer needs.
Preface to Spring Framework
The Spring Framework is a crucial part of modern Java development. It provides a comprehensive programming and configuration model. This model is essential for building robust, scalable applications. Understanding the Spring Framework allows developers to take advantage of its many benefits. This includes improving productivity, enhancing performance, and reducing complexity.
Overview of the Framework
Spring Framework is designed to make Java applications easier to manage and develop. It offers a range of features that assist developers in creating high-quality applications. Key components include the core container, which offers dependency injection, and aspect-oriented programming (AOP) support. These features help developers focus on application logic rather than boilerplate code.
Moreover, Spring allows for a clean separation of concerns, which facilitates easier testing and maintenance. This makes it a favored choice among many organizations. With Spring, teams can integrate various technologies seamlessly. It also remains flexible and adaptable, allowing for evolution alongside changing project needs.
Incorporating Spring into your projects leads to improved modularity and testability of code. Through features like Spring Boot, developers can quickly set up necessary components and rapidly develop microservices. This flexibility makes Spring suitable for applications of any scale.
Historical Context and Evolution
The journey of Spring Framework began in 2003, initiated by Rod Johnson. Initially, it aimed to simplify Java Enterprise applications that were often cumbersome due to excessive configuration. The first version was welcomed for its lightweight container. It diverged from heavy solutions like Java EE and birthed new architecture principles.
Over the years, Spring Framework evolved significantly. New versions introduced various features catering to changing technological landscapes. For instance, the launch of Spring MVC facilitated building web applications efficiently. The introduction of Spring Boot in 2014 streamlined the development process by minimizing configuration needs further.
Today, Spring Framework continues to evolve, adapting to trends like cloud computing, microservices, and the integration of artificial intelligence. It remains at the forefront of Java — a testimony to its enduring relevance. The development community continues to embrace Spring, citing its effectiveness in real-world applications.
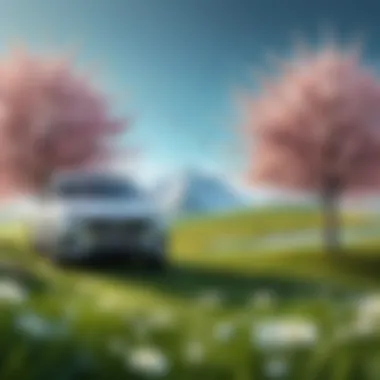
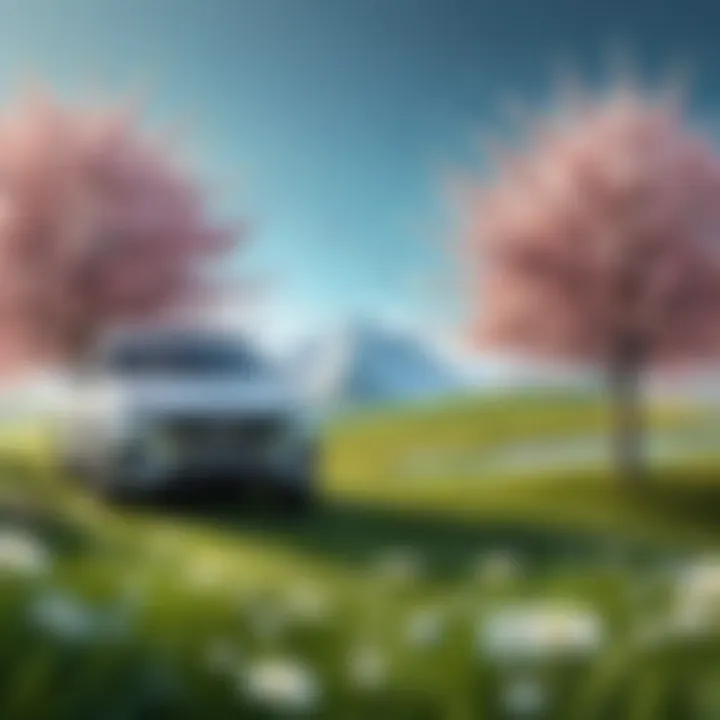
"Spring Framework represents the evolution of Java development, simplifying complexity and fostering innovation."
In this rapidly changing environment, staying updated with Spring’s advancements is crucial for developers aiming to build efficient and effective applications.
Core Concepts of Spring Framework
The core concepts of the Spring Framework underpin its powerful capabilities and flexible structure. Understanding these foundational elements can significantly enhance a developer's ability to create and manage software applications effectively. Among the notable core concepts, Inversion of Control (IoC) and Dependency Injection (DI) stand out, often serving as the bedrock for other features within the framework. These concepts help simplify the complex interactions within an application, promoting a modular architecture that can be easily maintained and scaled.
Inversion of Control
Inversion of Control is a key principle in software design that shifts the responsibility of object creation from the program itself to an external entity, such as the Spring container. This decoupling of components provides several advantages, which include increased flexibility and easier management of application dependencies.
When using IoC, the dependencies are injected into a class rather than the class actively creating them. As a result, a developer can change the behavior of one component without the need to alter the others that depend on it. This is particularly useful in large applications where managing dependencies manually can quickly become cumbersome. By embracing IoC, developers can create applications that are more resilient to changes and better suited for testing.
"Inversion of Control allows for greater separation of concerns, essential for building large and maintainable applications."
In practice, IoC can be achieved through methods such as constructor injection, where dependencies are provided via a class constructor, or setter injection, where dependencies are set through methods. Both of these techniques enable a developer to manage dependencies efficiently while promoting cleaner, more readable code.
Dependency Injection
Dependency Injection, while a specific form of Inversion of Control, deserves its own attention due to its profound impact on software development in Spring. Dependency Injection facilitates the automatic provision of an object's dependencies, which alleviates the burden of managing connections between different components.
With DI, a developer can declare what dependencies a class requires without worrying about how those dependencies are created or managed. The Spring container handles this complexity, allowing developers to focus on writing business logic. This also leads to improved testability since mock objects can easily replace real dependencies during unit testing.
In Spring, DI can be configured using XML or annotations, enabling the framework to manage the lifecycle of beans effectively. Constructor-based DI is often seen as a best practice since it makes dependencies explicit and ensures that a class cannot be instantiated without the necessary dependencies. Alternatively, setter-based DI offers flexibility, allowing dependencies to be modified after object creation, which can be useful in certain scenarios.
In summary, the core concepts of Inversion of Control and Dependency Injection form the foundation for an effective use of the Spring Framework. By understanding these principles, developers can make informed decisions in designing software that is modular, maintainable, and adaptable to change.
Fundamental Modules in Spring
Understanding the fundamental modules in Spring Framework is vital for effective application development. The Spring ecosystem is composed of several key modules, each designed to cater to specific functionalities. Together, these modules form a comprehensive structure that allows developers to build scalable and maintainable applications. They provide vital services such as configuration management, data access, and web layer functionalities. This section will explore the core modules that make up Spring, focusing on their importance, benefits, and practical implications.
Spring Core Container
The Spring Core Container is the foundation of the Spring Framework. It consists of essential components that manage the lifecycle of application objects, commonly referred to as beans. At its heart lies the BeanFactory interface, which provides the mechanism for managing dependencies through Inversion of Control (IoC).
Key Benefits:
- Loose Coupling: By using IoC, the Core Container promotes loose coupling between the components of an application. Developers can change or replace components without major disruptions.
- Configuration Flexibility: Beans can be defined in XML files or via Java annotations, allowing flexibility in configuration styles.
- Lifecycle Management: The framework automates the process of creating and destroying bean instances, reducing the boilerplate code developers must manage.
In practice, configuring the Core Container involves defining beans in an application context. For example:
Spring AOP
Aspect-oriented Programming (AOP) is another significant module of the Spring Framework, aimed at addressing cross-cutting concerns such as logging, security, and transaction management. AOP enhances modularity by allowing developers to separate these aspects from the core business logic.
Benefits of AOP:
- Code Reusability: AOP allows for reuse of aspects across different parts of an application, promoting cleaner code.
- Centralized Management: By managing cross-cutting concerns centrally, maintenance becomes easier and less error-prone.
- Flexibility: AOP adds behavior to existing code without modifying its structure.
For instance, a logging aspect can be defined as follows:
Spring Data Access/Integration
The Spring Data Access/Integration module provides comprehensive support for data access technologies. It includes support for JDBC, JPA, Hibernate, and other ORM tools. This module simplifies database interactions, reduces boilerplate code, and handles exception management effectively.
Advantages:
- Simplified Data Access: Spring offers a consistent approach for accessing data regardless of the underlying technology.
- Declarative Transaction Management: This feature enables easier transaction management through annotations or XML configuration.
- Support for Multiple Data Sources: Spring can work with multiple data sources, providing adaptability across different configurations.
This simplifies data handling significantly. A simple example using JdbcTemplate might look like this:
Spring
Spring MVC is a powerful module for building web applications. It follows the Model-View-Controller design pattern. This separation of concerns enables developers to manage the complexity of web applications effectively.
Core Features:
- Flexible URL Routing: Spring MVC allows for clear routing of HTTP requests to the appropriate controllers.
- Integration with View Technologies: It seamlessly integrates with various view technologies, including JSP, Thymeleaf, and FreeMarker.
- RESTful Support: Spring MVC provides comprehensive features for building RESTful web services.
Here’s a quick example of a simple controller:
Building Applications with Spring
Building applications using the Spring Framework is crucial for any Java developer looking to create robust, scalable, and maintainable applications. Spring provides a solid foundation that supports a wide range of programming paradigms, making it suitable for various types of applications, including web applications, microservices, and enterprise solutions. This section outlines the essential components and considerations for effectively building applications with Spring.
Spring's flexibility and modularity are among its most significant advantages. Developers can choose specific modules to fit their project needs without using the entire suite. This streamlining helps simplify development processes while addressing performance issues and resource management. Additionally, Spring promotes good coding practices by encouraging separation of concerns and clear architecture. These principles result in cleaner, more organized code that is easier to understand and maintain.
In this section, we will delve into three critical aspects of building applications with Spring: setting up your development environment, creating a simple Spring application, and configuring beans and contexts. Each of these topics offers practical steps that every developer should master to harness the full power of the Spring Framework.
Setting Up Your Development Environment
Setting up your development environment paves the way for a productive Spring programming experience. Begin with installing the Java Development Kit (JDK), as it is essential for compiling and running Java applications. It's recommended to use JDK 11 or higher for compatibility with the latest Spring features.
Next, you can choose an integrated development environment (IDE) such as IntelliJ IDEA, Eclipse, or Spring Tool Suite. These IDEs offer robust support for Spring, including project templates and built-in tools that enhance productivity.
Additionally, you will need to install Apache Maven or Gradle. Both are powerful build automation tools that facilitate dependency management. Each tool has its own advantages, so select one based on your project’s requirements and your personal preference.
"A well-configured development environment is the first step to postive experiences in application building."
Lastly, you should familiarize yourself with Spring's official documentation and online resources. Engaging with the community through forums such as Reddit can also be beneficial.
Creating a Simple Spring Application
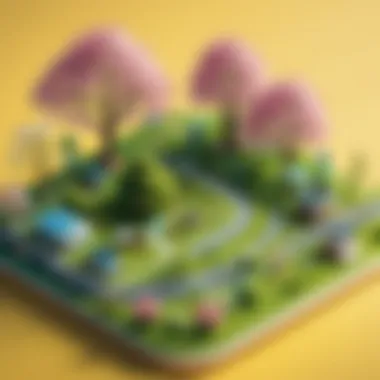
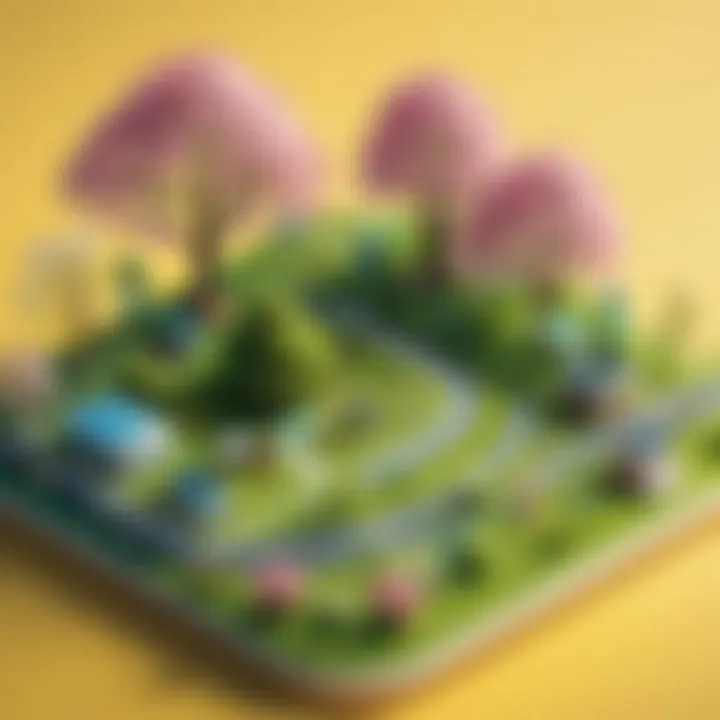
With your development environment established, the next step is to create a simple Spring application. This process begins with setting up a new project using your chosen build tool. For example, using Maven, you can create a file, which declares project dependencies.
Here is an example of what the might look like for a basic Spring application:
Following the setup, create your main application class, which serves as the entry point for your Spring application. This class should be annotated with to enable component scanning and configuration.
Configuring Beans and Contexts
Configuring beans and application contexts is fundamental when using Spring. Spring employs a container that manages the lifecycle of your beans, which are essentially objects created and maintained by the Spring IoC (Inversion of Control) container.
You can define beans either through XML configuration or using Java annotations. Using annotations has become the preferred approach due to its conciseness and readability. For example, a simple bean configuration can be achieved as follows:
Here, we define a configuration class and declare a bean of type . The Spring container will manage the lifecycle and dependency of this bean.
Advanced Features of Spring Framework
The advanced features of the Spring Framework are essential for developers who seek to build robust, scalable, and maintainable applications. Utilizing these features can streamline development processes, enhance security, and foster better integration with other modern technologies. This section covers critical components such as security mechanisms, Spring Boot as an abstraction layer for rapid development, and the utilization of microservices architecture within the Spring ecosystem.
Security in Spring
Security is a paramount concern in software development. The Spring Framework provides a comprehensive security module that ensures robust authentication and authorization. By integrating Spring Security, developers can manage user access levels effectively. This involvement spans several functions such as role-based access control, protection against common vulnerabilities like cross-site scripting, and integration with OAuth for third-party login capabilities.
One notable feature of Spring Security is its customizable authentication mechanisms. This allows developers to implement various methods to secure applications, thus providing flexibility regardless of application complexity.
Moreover, security is paramount not only during application development but also in maintenance cycles. With its declarative security model, Spring Security facilitates the easy auditing of security settings. It simplifies the task of ensuring compliance with security standards, protecting sensitive data from breaches, and this is a critical consideration in today’s digital environment.
"Security is not a product, but a process.” - Bruce Schneier
Spring Boot Overview
Spring Boot is an extension of the Spring Framework that simplifies the setup and development of new Spring applications. It provides features like convention over configuration and the ability to run applications standalone. This efficiency is particularly beneficial for developers who want to focus on writing business logic instead of dealing with boilerplate configurations.
Key benefits of using Spring Boot include:
- Auto Configuration: Automatically configures your application based on the dependencies you have added. This means less setup time and less manual configuration.
- Embedded Servers: Allows applications to run without the need for an external server. Therefore, application setup becomes more straightforward.
- Production-Ready Features: Spring Boot also includes production-level features such as health checks, metrics, and externalized configuration. These aspects validate the application's state and performance in real-time.
Thus, Spring Boot enhances developer productivity and reduces the time from ideation to deployment.
Microservices Architecture with Spring
Microservices architecture is a modern approach to software development that focuses on developing small, independent, and loosely coupled services. The Spring Framework provides strong support for building microservices, aiding in the development of more resilient and maintainable applications.
Spring Cloud is a suite of tools for managing microservices. It offers features like service discovery, configuration management, and circuit breakers out of the box. This support enables developers to build systems where individual services can scale independently or update without affecting the entire system.
Benefits of utilizing microservices with Spring include:
- Scalability: Individual services can be scaled independently according to demand, which leads to better resource utilization.
- Fault Isolation: If one service fails, it does not bring down the whole application. This makes apps more resilient.
- Technology Diversity: Each microservice can use different technologies based on its requirements. This aspect allows teams greater flexibility in choosing the correct stack for specific needs.
Integrating Spring with Other Technologies
Integrating Spring with other technologies is essential for building modern applications that leverage the strengths of various frameworks and tools. This integration allows developers to create a robust architecture that can efficiently handle different business needs. In today’s diverse development landscape, the ability to interact seamlessly with other technologies enhances application capability and performance. Factors such as scalability, maintainability, and ease of deployment come into play when considering integration options.
Spring and Hibernate
Hibernate is a powerful framework for object-relational mapping (ORM) in Java. It simplifies database interactions by allowing developers to work with Java objects instead of SQL queries. Integrating Spring with Hibernate provides numerous benefits, such as reduced boilerplate code and enhanced transaction management.
With this integration, developers can leverage Spring’s dependency injection to manage Hibernate sessions. This allows for more flexible data access management. The Spring framework also provides a set of templates, such as , which simplifies the use of Hibernate by managing boilerplate code related to opening and closing sessions.
Important considerations include:
- Configuration: Ensure that both Spring and Hibernate are properly configured in the application context file. It often involves setting up data source properties and Hibernate-specific configurations.
- Transaction Management: Use Spring’s transaction management features for handling transactions across different layers of the application effectively. This approach improves data consistency and reliability.
Spring with RESTful Services
RESTful services have become a standard for building APIs due to their lightweight nature and use of standard HTTP methods. Integrating Spring with RESTful services transforms Java web applications by enabling the development of REST APIs quickly. This integration can greatly enhance systems interoperability and allow for more straightforward communication between clients and servers.
By utilizing Spring MVC, developers can define REST controllers, which handle requests and responses for different endpoints. Annotations such as and simplify the process of managing API endpoints, making the code more maintainable and easier to read.
Key benefits of this integration include:
- Ease of Development: Spring’s annotations provide a clean and concise way to define endpoints, streamlining development.
- Content Negotiation: Spring allows for handling different media types easily, making it simple to respond with JSON or XML based on client requirements.
- Error Handling: Advanced error handling features in Spring MVC help maintain the API's robustness without cluttering business logic.
Spring and Cloud Technologies
In an era focused on cloud computing, integrating Spring with cloud technologies has become increasingly important. Using tools like Spring Cloud allows applications to leverage microservices architecture effectively. This integration permits developers to build systems that are easily scalable, resilient, and manageable across cloud platforms such as AWS, Azure, or Google Cloud.
Spring Cloud provides features like service discovery, circuit breakers, and centralized configuration, which enhance the microservices experience. For example, offers solutions like Zuul for API routing and Eureka for service registry.
Considerations for this integration include:
- Distributed Systems: It's vital to have a solid understanding of how to manage distributed systems since this often introduces complexities related to data consistency and reliability.
- Monitoring and Security: Implement strategies for monitoring microservices and ensuring secure communication between them. Solutions like can be integrated to manage authentication and authorization effectively.
Integrating Spring with various technologies enhances the flexibility and scalability of applications, allowing for more adaptable systems tailored to business needs.
Thus, grasping the integration of Spring with other vital technologies is foundational for developers. It ensures that they can create systems that not only meet current demands but also adapt to future challenges.
Testing in the Spring Framework
Testing is a crucial element of software development, particularly within the Spring Framework. It ensures that applications are robust, reliable, and maintainable. The importance of testing in Spring lies in its ability to verify the functionality of components, reduce bugs, and improve overall quality. By implementing a comprehensive testing strategy, developers can catch issues early, enhancing the scalability and performance of applications. Additionally, well-tested applications can significantly reduce unexpected behavior in production, which is essential for both user satisfaction and business outcomes.
Unit Testing with Spring
Unit testing focuses on validating the individual components or units of an application. In the context of the Spring Framework, unit tests are typically focused on beans or services. Spring provides numerous tools and utilities to facilitate this process. The main benefit of unit testing is that it allows developers to isolate components and ensure they behave as intended.
To conduct unit testing effectively, developers often use the JUnit framework in conjunction with Spring's testing support. This combination can easily mock dependencies using tools such as Mockito. Testing configurations can be loaded using the annotation, which allows a developer to test components in isolation.
An example of a simple unit test might look like this:
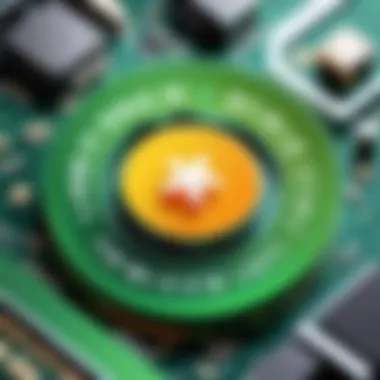
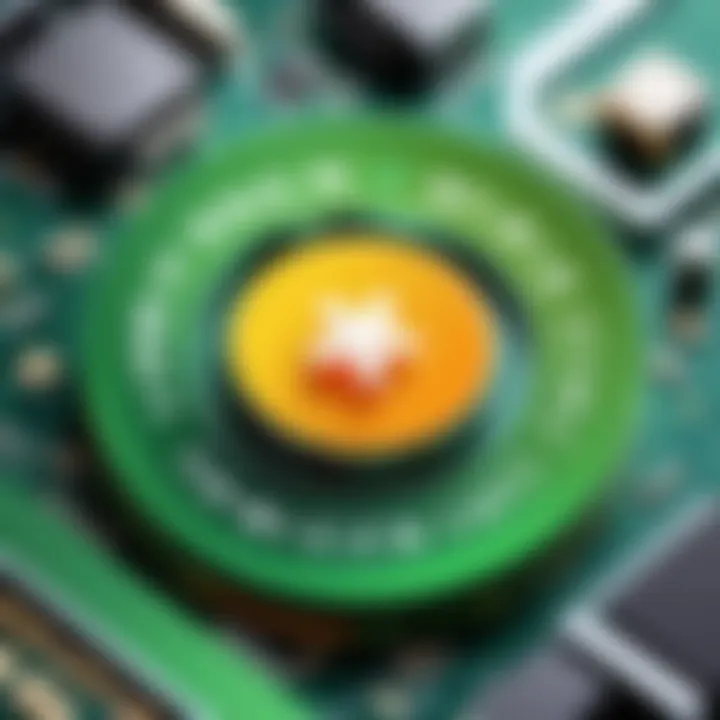
This is a straightforward approach to test a service method's output.
Integration Testing Strategies
Integration testing is a higher level of testing that focuses on the interactions between multiple components. In Spring, this means checking how different layers of the application work together. Integration tests verify that the system as a whole meets specific requirements. They are essential for identifying issues that may not become evident during unit testing, such as configuration or integration issues.
Spring provides various tools for integration testing, including the Spring TestContext Framework. This framework allows for loading full application contexts and integrating with external resources like databases or message brokers. A common approach to integration testing in Spring is to use the annotation, which sets up an application context for the tests to run in.
Consider the following strategies when implementing integration tests in a Spring application:
- Utilize the annotation to set properties specific to the test environment.
- Use for testing web applications, allowing request mocking and response testing without needing a running server.
- Employ to ensure that database changes during tests do not affect the actual data.
Integration testing is crucial, especially in scenarios where multiple components interact, such as with database transactions or web services.
"Integration testing helps ensure that different modules of an application function together as expected."
In summary, robust testing strategies in Spring Framework—both unit and integration—are vital for creating high-quality applications. By applying these methods, developers can ensure their software meets both functional and performance requirements.
Best Practices in Spring Framework Development
Employing best practices in the development of applications using the Spring Framework plays a crucial role in ensuring long-term maintainability, efficiency, and scalability. Following established guidelines helps developers avoid common pitfalls and allows teams to create robust applications that can adapt to changing requirements. It not only enhances the performance but also simplifies the debugging and testing processes, making deployment smoother.
When developers adopt best practices, they align with known patterns and structures that ease collaboration within teams. This becomes especially useful when onboarding new team members who can quickly grasp the organization of the codebase. Typical best practices encompass configuration management and performance optimization, both of which the Spring ecosystem supports.
Configuration Management Guidelines
Configuring Spring applications correctly is foundational to achieving desired outcomes. Effective configuration management can mitigate issues related to beans and application contexts. Here are some guidelines for managing configurations efficiently:
- Use Profiles: Leveraging Spring profiles helps define different configurations for various environments, such as development, testing, and production. This creates a separation of concerns and allows for environment-specific customizations.
- Externalize Configuration: Instead of hardcoding values, externalizing configuration using property files or YAML can streamline updates and make it easier to adapt the application to different contexts.
- Consider Application Context: Utilize the ApplicationContext wisely to load bean definitions from multiple sources. Using XML or Java-based configurations should complement each other when appropriate.
- Keep it Simple: Avoid excessive complexities in your configurations. Simple setups are easier to manage and understand. Aim to adhere to a convention-over-configuration approach whenever possible.
Additionally, modularize configuration files. Breaking configurations into smaller, manageable pieces enhances readability. Non-native configurations can cloud understanding, so always aim for clarity.
Performance Optimization Techniques
Optimizing application performance is essential for any successful project. The Spring Framework offers various built-in features that can help developers address performance-related issues:
- Lazy Initialization: Consider using lazy initialization for beans that do not need to be loaded at startup. This can significantly decrease startup time.
- Use @Cacheable Annotation: Caching frequently accessed data can improve performance. The use of the @Cacheable annotation allows Spring to cache method results.
- Profile Your Application: Regularly profiling the application will help identify bottlenecks. Tools like VisualVM or YourKit assist in monitoring resource usage and can highlight areas for improvement.
- Minimize Database Calls: Reduce the number of database queries by utilizing batch processing or fetching required data in fewer calls. This can greatly enhance application responsiveness.
- Consider Asynchronous Processing: Implement asynchronous processing when dealing with I/O bound operations. This will enhance throughput by allowing the application to continue processing other requests while waiting for I/O operations to complete.
Implementing these optimization techniques requires continuous monitoring and iteration. By maintaining a mindset of efficiency and improvement, developers can ensure that Spring applications perform optimally over their lifecycle.
In summary, prioritizing configuration management and performance optimization not only strengthens the application's infrastructure but also contributes to a more enjoyable development experience. This holistic approach benefits teams and leads to the creation of superior software.
Real-World Use Cases of Spring Framework
The Spring Framework has a wide array of practical applications, showcasing its versatility in real-world environments. Understanding these use cases provides insight into why many developers and organizations adopt this framework. Companies can build scalable applications, enhance efficiency, and leverage its features to solve specific problems such as managing complex system integrations and ensuring robust security.
By studying real-world implementations, developers can appreciate the tangible benefits of using Spring. It allows easy configurations for applications, supports various databases, and integrates well with numerous web technologies. Organizations can achieve better modularity and testability, which enhances overall productivity, allowing teams to deliver software faster and with higher quality.
Furthermore, insights from these use cases can guide developers in decision-making processes and implementation strategies for their projects.
Enterprise Applications
Enterprise applications demand reliability, scalability, and efficient data management. The Spring Framework meets these needs through its various modules tailored for enterprise-level solutions. For instance, the Spring MVC module allows the creation of web applications that follow best practices in design and development, ensuring a clean separation of concerns.
The Spring Core Container provides essential features like Dependency Injection, which is crucial for application maintainability and testability. With Spring's expansive environment, developers can integrate applications with various external systems, facilitating smooth communication between services.
Benefits of using Spring in enterprise applications include:
- Flexibility: Developers can choose components according to their needs without modifying the entire project.
- Integration: Spring supports seamless integration with databases, messaging services, and third-party APIs, a must for enterprise solutions.
- Security: Built-in security features allow developers to safeguard their applications effectively against threats.
"Spring Framework offers a comprehensive toolset for creating reliable enterprise applications, combining simplicity with powerful capabilities."
E-commerce Platforms
E-commerce platforms are particularly dynamic, requiring technology stacks that can adapt quickly to changing demands. Spring provides a robust solution for these platforms by enabling developers to create scalable and maintainable applications. The framework's ability to manage transactions effectively is vital for handling financial operations securely and reliably.
Moreover, Spring's support for RESTful services allows e-commerce platforms to deliver APIs that enhance interoperability between front-end and back-end systems. This is essential for creating a responsive user experience and integrating with various payment gateways and inventory systems.
Key considerations for e-commerce applications using Spring include:
- Resilience: Building applications that can handle high traffic and provide uninterrupted service.
- Security protocols: Implementing SSL, authentication, and authorization to protect user data and transactions.
- Performance: Optimization techniques to reduce load times and improve efficiency in processing user queries.
In both enterprise and e-commerce contexts, the Spring Framework proves itself as a critical resource for developers, offering tools and functionalities to create effective and reliable applications.
Upcoming Trends and Future Directions
Exploring the upcoming trends within the Spring Framework is essential for developers and IT professionals today. By understanding these trends, one can adapt to the rapidly changing landscape of software development and ensure that their skill set remains relevant. As technology evolves, so does the framework, offering new opportunities and challenges that must be navigated carefully.
Evolving Landscape of Spring Framework
The Spring Framework is constantly evolving to meet the demands of modern software development. One significant trend is the increased adoption of microservices architecture. This approach allows applications to be built as a collection of loosely coupled services. Each service can be developed, deployed, and scaled independently, leading to greater flexibility and resilience.
Additionally, modularization of the framework is gaining traction. Developers can utilize only the components they need, reducing the application's footprint. This modular approach not only optimizes performance but also encourages a more targeted use of resources. With the continuing rise of cloud-native applications, integration with cloud services has become pivotal. This includes support for platforms like Amazon Web Services and Google Cloud, which simplifies deployment and management of Spring applications.
"The ability to adapt to new technologies is crucial in the software development space. Spring Framework embraces change and encourages developers to do the same."
Integration of AI and Spring
As artificial intelligence becomes more integrated into applications, Spring Framework is also starting to adopt this trend. By facilitating the inclusion of machine learning models, developers can harness AI's power to enhance their applications. This integration offers the potential for more intelligent and responsive software solutions, elevating user experience.
Moreover, Spring's support for reactive programming aligns well with AI implementations, ensuring lower latency and better performance. As AI technologies evolve, Spring will likely provide enhanced libraries and tools to support developers in creating AI-driven applications.
In summary, keeping abreast of trends such as microservices, modularization, and AI integration within the Spring Framework is vital. These elements not only influence how applications are developed but also shape the competencies needed in the development workforce. Understanding and leveraging these trends ensures that developers remain at the forefront of technology.
Finale
The conclusion serves as a vital part of this article on the Spring Framework, summarizing the significant concepts and applications discussed throughout. By reflecting on what has been covered, it solidifies the reader’s understanding and emphasizes the relevance of the Spring Framework in contemporary software development.
Final Thoughts on Spring Framework
Spring Framework stands out for its powerful features and flexibility. It enables developers to produce scalable and maintainable applications efficiently. With its comprehensive ecosystem, Spring facilitates not just simple applications but also complex, enterprise-level solutions.
Key Points to Remember:
- Emphasis on Core Concepts: Understanding dependency injection and aspect-oriented programming is fundamental for harnessing the full potential of Spring.
- Real-World Applications: The framework is extensively utilized in various industries, from e-commerce platforms to enterprise applications, proving its versatility.
- Best Practices: Following best practices can optimize the development process, ensuring that applications remain robust over time.
The importance of this conclusion is to encourage both new and seasoned developers to continuously explore the numerous resources available, such as community forums and documentation. The path of mastering the Spring Framework is continuous, with evolving trends and future directions revealing exciting opportunities. This article serves as a robust foundation for anyone keen on leveraging the Spring Framework for exceptional software development.