Mastering Regex for Python: A Comprehensive Guide to Enhancing String Manipulation
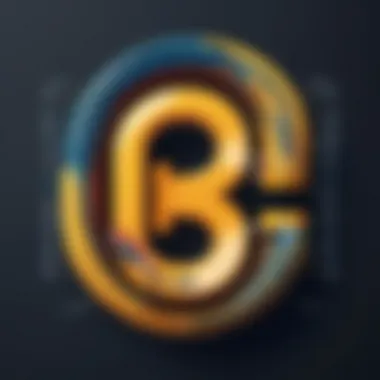
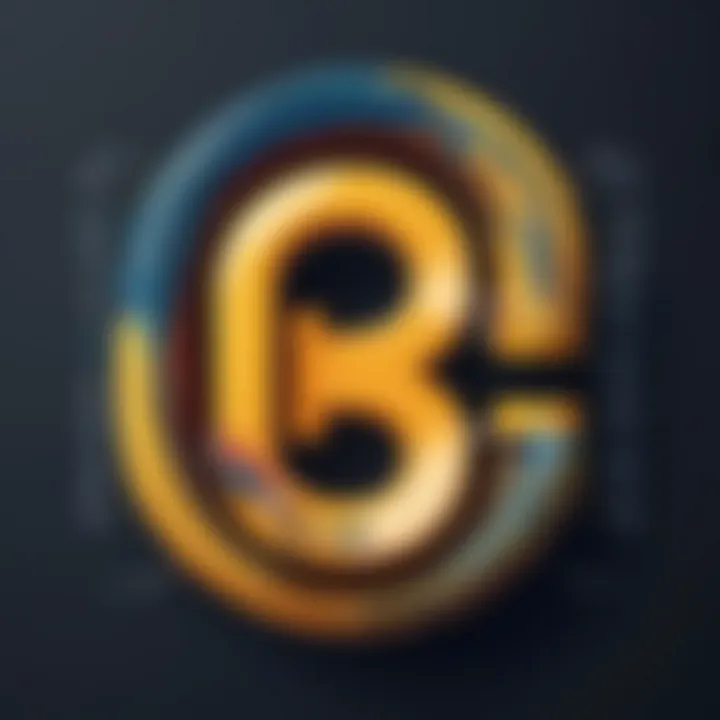
Overview of regular expressions in Python
Regular expressions, commonly known as regex, play a crucial role in string manipulation and pattern matching within Python programming. Understanding the nuances of regex is paramount for efficient text processing and validation, making it a formidable tool for developers and data scientists.
- Definition and importance of regular expressions
Regular expressions are a sequence of characters that form a search pattern, allowing developers to match specific patterns within strings. This functionality is invaluable for tasks such as data extraction, text validation, and search operations.
- Key features and functionalities of regex
Python's regex module offers a robust set of features, including pattern matching, substitution, and metacharacters for constructing complex search patterns. These functionalities enable programmers to perform intricate string manipulations with ease and precision.
- Use cases and benefits of regex
Regex finds a wide array of applications in tasks such as data parsing, input validation, and text mining. Its flexibility and power make it a versatile tool for various industries, aiding in tasks that require sophisticated string processing.
Best Practices for Mastering Regex in Python
Achieving proficiency in regex involves adhering to best practices that ensure efficient implementation and optimal performance. By following industry-recommended guidelines, developers can harness the full potential of regex for their projects.
Best practices for implementing regex
Effective implementation of regex involves writing clear and concise patterns, utilizing anchors for precise matching, and testing expressions thoroughly to avoid errors. Embracing readability and modularity in regex patterns enhances code maintenance and scalability.
Tips for maximizing efficiency and productivity
To maximize productivity with regex, developers should leverage precompiled patterns for performance gains, optimize pattern design for speed, and explore online resources and libraries for regex optimization techniques. Embracing efficient coding practices enhances the usability and performance of regex operations.
Case Studies on Successful Regex Implementation
Real-world examples demonstrate the practical applications and benefits of mastering regex in Python. These case studies provide insights into how industry experts leverage regex to solve complex problems and achieve remarkable outcomes.
Lessons learned and outcomes achieved
By studying real-world implementations, developers gain valuable insights into effective regex usage, error handling strategies, and performance optimizations. These case studies showcase successful approaches to regex application and highlight key takeaways for aspiring regex practitioners.
Latest Trends and Updates in Regex for Python
Staying informed about the latest advancements in regex technology is crucial for developers seeking to optimize their regex workflows and stay ahead of industry trends. By exploring upcoming developments and innovations in regex, professionals can enhance their skill set and adapt to evolving programming requirements.
How-To Guides and Tutorials for Mastering Regex
Step-by-step guides and tutorials offer practical insights for beginners and advanced users looking to enhance their regex proficiency. By following detailed instructions and hands-on examples, readers can deepen their understanding of regex concepts and refine their skills for effective regex utilization.
Intro to Regular Expressions in Python
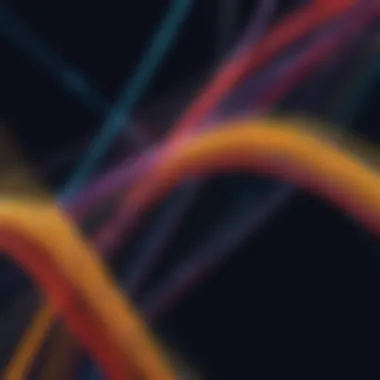
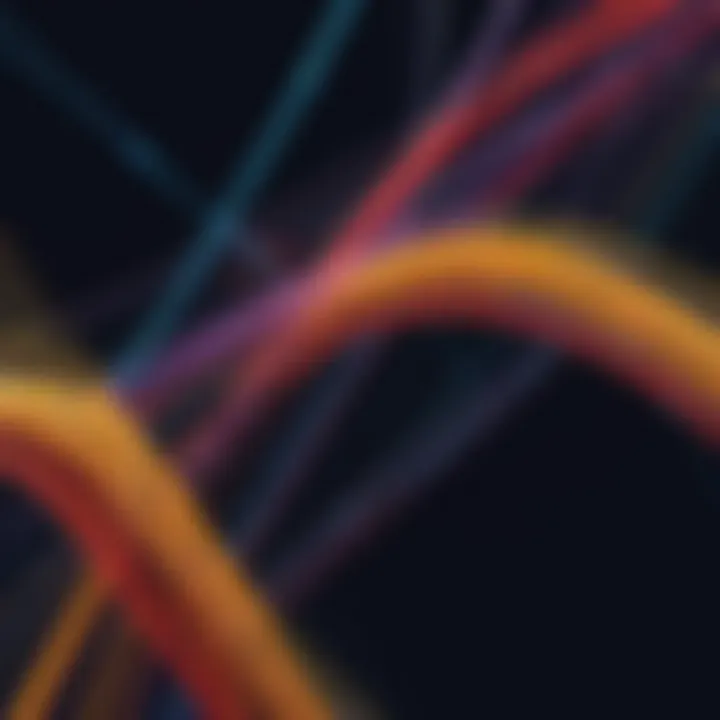
Regular expressions in Python are foundational tools for string manipulation and pattern matching. Understanding how to leverage regex in Python is crucial for developers, data scientists, and tech enthusiasts. By mastering regex, individuals can efficiently process and analyze text data, extract specific information, and validate input formats. This section will delve deep into the various aspects of regex in Python, from fundamental concepts to advanced techniques, providing a robust foundation for readers to enhance their Python programming skills.
Understanding the Basics of Regex
Definition and Purpose of Regular Expressions
Regular expressions, commonly known as regex, serve as a specialized language for defining search patterns within text. In this context, understanding the definition and purpose of regular expressions is key to comprehending their role in Python programming. The beauty of regular expressions lies in their ability to express complex search patterns concisely, aiding in tasks such as data validation, manipulation, and extraction. Embracing regex allows developers to create scalable solutions for text processing efficiently.
Syntax and Patterns in Regex
Syntax in regular expressions refers to the specific rules and components used to define search patterns. Patterns, on the other hand, represent the sequences of characters that the regex engine attempts to match in the input text. Mastering the syntax and patterns in regex is fundamental to constructing accurate and effective search queries. By grasping the nuances of regex syntax and various pattern elements like anchors, quantifiers, and character classes, programmers can craft precise search criteria tailored to their requirements.
Common Metacharacters in Regex
Metacharacters in regular expressions are symbols with special meanings that enable advanced searching capabilities. Understanding common metacharacters like '.', '^', '$', and '+' empowers users to perform sophisticated pattern matching operations. Each metacharacter serves a unique function, allowing developers to manipulate text data dynamically. Leveraging metacharacters efficiently enhances the flexibility and accuracy of regex searches, making it a valuable asset in Python programming.
Working with Regex in Python
Built-in 're' Module in Python
The 're' module in Python provides essential functionalities for working with regular expressions. By leveraging the built-in 're' module, developers gain access to a rich set of tools to parse and manipulate text data effectively. This module serves as the cornerstone for utilizing regex in Python, offering functions for pattern matching, substitution, and more. Integrating the 're' module into Python workflows simplifies regex operations and streamlines text processing tasks.
Compiling and Using Regex Patterns
Compiling regex patterns in Python enhances performance and reusability across different text processing tasks. By compiling regex patterns into objects, developers can avoid redundant pattern parsing operations, optimizing runtime efficiency. The ability to reuse compiled regex objects reduces computational overhead, making regex operations faster and more resource-efficient. Incorporating compiled regex patterns in Python code fosters a modular and scalable approach to regex implementation.
Flags and Functions for Regex Operations
Flags and functions play a pivotal role in fine-tuning regex operations in Python. Flags modify the behavior of regex patterns, influencing aspects like case sensitivity, multiline matching, and pattern handling. By applying flags strategically, developers can customize regex behavior to suit specific requirements effectively. Additionally, leveraging functions like 'search()', 'match()', and 'sub()' enables precise control over regex operations, empowering users to extract, replace, and manipulate text data with ease.
Fundamental Regex Operations in Python
Fundamental Regex Operations in Python play a pivotal role in this comprehensive guide on Regex mastery in Python. Understanding fundamental operations is crucial for laying a strong foundation in utilizing regular expressions effectively for string manipulation and pattern matching tasks. By delving into Pattern Matching and Search Functions, readers can grasp the essential techniques for navigating and manipulating text data efficiently within Python programs. This segment sheds light on essential concepts such as using Search() and Match() functions, exploring anchors and quantifiers in pattern matching, as well as understanding the significance of grouping and capturing within Regex patterns.
Pattern Matching and Search Functions
Using Search() and Match() Functions
The implementation of Search() and Match() functions is fundamental in Regex operations for Python, enabling users to search for specific patterns within text strings efficiently. These functions provide a robust mechanism for locating patterns at the beginning (Match()) or anywhere in the text (Search()). Their flexibility in pattern identification makes them valuable tools for extracting targeted information, contributing significantly to the overall effectiveness of Regex applications. Despite their power, careful consideration is required to optimize performance and accuracy while utilizing these functions within Python programs.
Anchors and Quantifiers in Pattern Matching
Anchors and quantifiers play a vital role in determining the boundaries and repetitions of patterns during matching operations. Anchors specify where in the text the pattern should start or end, enhancing precision and control over matching criteria. Quantifiers, on the other hand, define the number of occurrences or range of repetitions for specific characters or groups, allowing for flexible pattern recognition. The strategic use of anchors and quantifiers optimizes the accuracy and efficiency of pattern matching in Regex, contributing to the successful manipulation of text data.
Grouping and Capturing in Regex
The concepts of grouping and capturing in Regex empower users to structure complex patterns and extract specific information from text data. Grouping allows for creating logical units within patterns, enabling easier manipulation and identification of components during matching processes. Capturing, on the other hand, facilitates the retrieval of targeted elements from the matched text, enhancing the precision and versatility of Regex operations. Mastering grouping and capturing techniques is indispensable for proficient text manipulation and data extraction in Python, elevating the effectiveness of Regex applications.
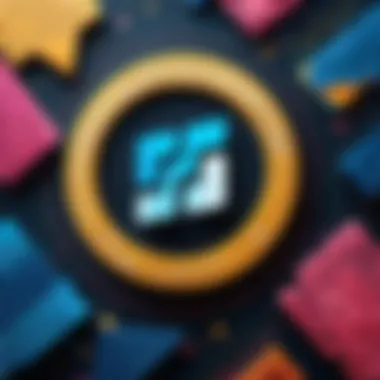
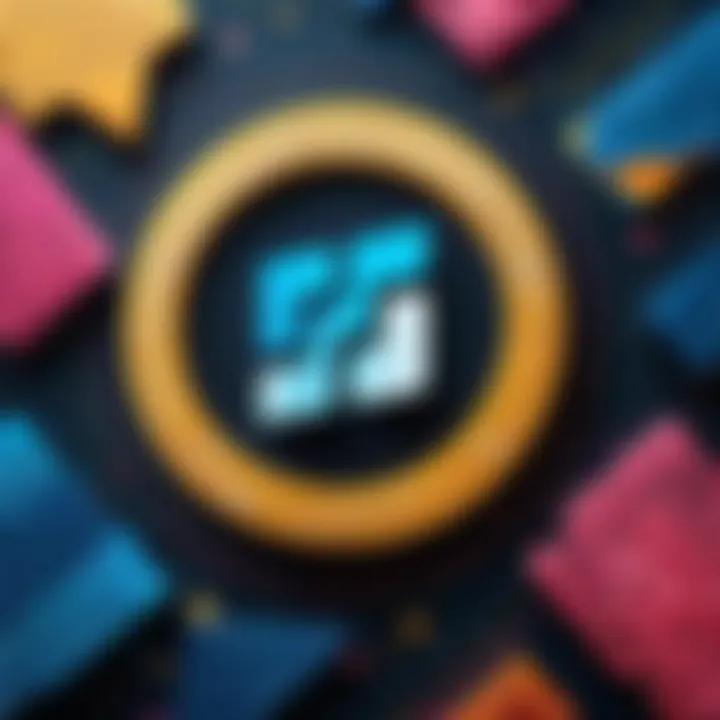
Handling Matches and Substitutions
In the realm of fundamental Regex operations, effectively handling matches and substitutions is essential for seamless text manipulation and data transformation. Accessing Match Objects and Attributes grants users the ability to retrieve detailed information about a match, facilitating further processing or analysis based on extracted data. The sub() method for substituting text offers a convenient way to replace matched patterns with specified content, streamlining text manipulation tasks. Leveraging Regex for data cleaning and transformation enhances data preprocessing workflows by automating repetitive tasks and ensuring data consistency across varied sources.
Accessing Match Objects and Attributes
Accessing match objects and attributes provides in-depth insights into matched patterns, enabling users to extract specific details or metadata for further analysis. By understanding and utilizing match attributes, users can gain precise information about matched patterns, facilitating informed decision-making and subsequent actions based on the extracted data. While accessing match objects offers a wealth of information, careful consideration is necessary to ensure accurate retrieval and interpretation of match attributes within Regex operations.
Substituting Text with sub() Method
The sub() method in Regex serves as a powerful tool for substituting matched patterns with alternative text or contents within Python programs. Its versatility in text replacement allows users to customize text transformations based on specific patterns, enhancing the adaptability and efficiency of data processing workflows. As a key feature in Regex operations, the sub() method streamlines the process of text manipulation and data cleansing, offering a flexible mechanism for addressing diverse substitution requirements in text analysis and transformation tasks.
Applying Regex for Data Cleaning and Transformation
Applying Regex for data cleaning and transformation unveils opportunities for refining textual data and standardizing formats across datasets. By employing Regex patterns for targeted data extraction and modification, users can automate data cleaning processes and ensure consistency in data structures. The systematic application of Regex for data transformation optimizes data preprocessing tasks, enabling efficient handling of large datasets and streamlining data integration processes for improved analytical outcomes.
Advanced Techniques for Regex Optimization
In this elaborate discourse on mastering regex for Python, delving into the segment of 'Advanced Techniques for Regex Optimization' is paramount. It encapsulates the essence of refining regex applications to attain peak efficiency and precision in string manipulation and pattern recognition within Python. Focusing on intricacies like Greedy vs. Non-Greedy Matching, Compile Flags for Improved Performance, and Anchoring and Lookahead Assertions, this section aims to unravel the nuanced dynamics crucial for optimizing regex functionality.
Optimizing Regex Performance
Greedy vs. Non-Greedy Matching:
When navigating the realm of regex optimization, a pivotal consideration surfaces in the form of the Greedy vs. Non-Greedy Matching methodology. This distinctive facet influences the overall efficacy of regex operations by dictating the prioritization of expansive matches (Greedy) versus conservative matches (Non-Greedy). Understanding the ramifications of this dichotomy is imperative, as it directly impacts the accuracy and computational intensity of regex implementations, thus holding significance within the context of this article.
Compile Flags for Improved Performance:
Another crucial facet elucidated within this domain pertains to Compile Flags for Enhanced Performance. These flags serve as directives that fine-tune the behavior of regex patterns, augmenting the speed and accuracy of pattern matching operations. By shedding light on the specialized characteristics of compile flags and their role in streamlining regex processes, this section underscores their substantial relevance in fortifying regex performance within Python.
Anchoring and Lookahead Assertions:
Furthermore, an exploration of Anchoring and Lookahead Assertions unveils additional layers of sophistication in regex optimization strategies. Anchoring techniques facilitate the restriction of search horizons within a text, enhancing the precision of pattern matching, while lookahead assertions enable the anticipation of specific patterns ahead of the current matching point. The distinctive attributes of these mechanisms contribute significantly to the meticulous fine-tuning of regex operations, amplifying the efficiency and accuracy of regex utilization as expounded in this article.
Using Compiled Regex Objects
Benefits of Compiling Regex Patterns:
Discussing the Benefits of Compiling Regex Patterns within the realm of regex optimization sheds light on the compelling advantages offered by this practice. By compiling regex patterns, developers can expedite pattern matching operations, reduce computational overhead, and enhance the reusability of compiled patterns. This in-depth analysis elucidates the pivotal role played by compiled regex objects in augmenting the overall performance and efficiency of regex workflows within Python.
Caching and Reusing Compiled Patterns:
Exemplifying the concept of Caching and Reusing Compiled Patterns underscores a strategic approach towards optimizing regex functionality. By caching compiled patterns, developers can leverage precompiled regex objects, mitigating redundant compilation processes and accelerating pattern matching tasks. This section accentuates the pragmatic benefits associated with caching and reusing compiled patterns, emphasizing their instrumental role in elevating the efficiency and responsiveness of regex implementations within Python.
Enhancing Efficiency in Regex Matching:
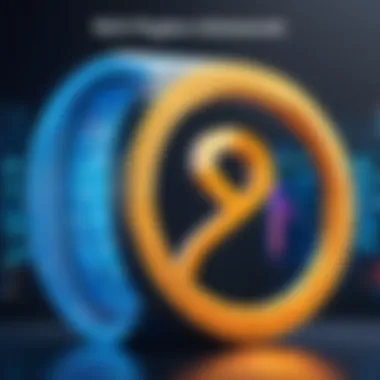
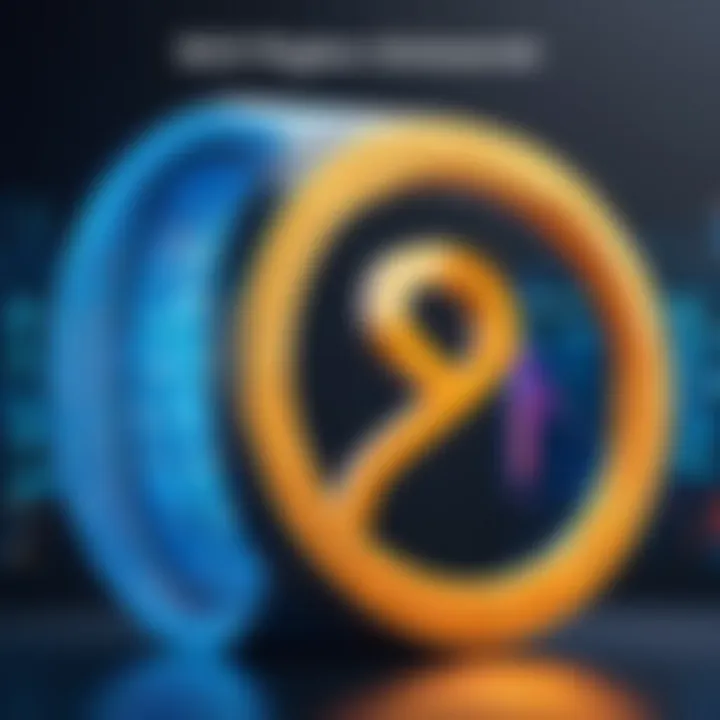
Lastly, the discourse on Enhancing Efficiency in Regex Matching encapsulates a holistic approach towards maximizing the performance of regex operations. By incorporating efficiency-driven practices such as optimized pattern structuring, memory management, and algorithmic optimizations, developers can bolster the speed and accuracy of regex matching processes. This detailed examination underscores the nuanced strategies aimed at optimizing regex efficiency, culminating in a comprehensive narrative that accentuates the indispensability of efficiency-enhancing techniques within Python regex applications.
Regex Integration with Python Applications
Regex integration with Python applications is a pivotal aspect discussed in our extensive guide on mastering regex for Python. Understanding how to effectively integrate regex in Python applications opens up a world of possibilities for software developers, IT professionals, and data scientists. By seamlessly incorporating regex into Python programs, individuals can streamline data processing, extraction, and validation procedures with precision and efficiency.
Regex in File Handling and Parsing
Extracting Data from Text Files
Peeling back the layers of extracting data from text files reveals a fundamental process crucial for leveraging regex in Python applications. This specific operation enables users to sift through textual data efficiently, extracting pertinent information while discarding extraneous content. The ability to pinpoint and extract data from textual sources with precision is a cornerstone for tasks requiring data parsing and analysis within the Python programming environment.
Validating Input Formats
Validating input formats represents a critical component in data validation procedures within Python applications. By employing regex to validate input formats, developers can ensure that user-provided data adheres to specified formats and standards. This meticulous validation process is essential for maintaining data integrity, accuracy, and consistency within software systems, contributing significantly to overall system reliability.
Automating Data Extraction Processes
Automation of data extraction processes through regex implementation delivers unparalleled efficiency gains for Python applications. By automating data extraction tasks, developers can reduce manual intervention, enhance processing speed, and minimize human error in handling voluminous data sets. The seamless integration of automated data extraction mechanisms powered by regex empowers Python applications to handle data-intensive operations with ease and precision.
Web Scraping and Data Extraction
Scraping HTML Content with Regex
Delving into the realm of scraping HTML content with regex unveils a sophisticated approach to extracting valuable information from web sources. This intricate process involves parsing HTML structures using regex patterns to extract specific data elements embedded within web pages. The ability to navigate and extract targeted information from HTML content using regex techniques fortifies data extraction capabilities within Python applications.
Extracting Information from Websites
Extraction of information from websites using regex functionalities serves as a strategic method for harvesting relevant data across online platforms. This strategic extraction process enables users to collect data from diverse web sources, aggregating valuable insights for various analytical purposes. Leveraging regex for information extraction from websites empowers Python applications with the ability to access and utilize web data effectively.
Customizing Regex for Web Data Mining
Tailoring regex patterns for web data mining purposes amplifies the data processing capabilities of Python applications. By customizing regex patterns to meet specific requirements of web data mining tasks, users can extract, filter, and manipulate data extracted from web sources with precision. The adaptability of regex customization for web data mining fuels enhanced data analysis and insights generation within Python programming landscapes.
Best Practices and Tips for Regex Mastery
In the realm of regex mastery within Python, adherence to best practices and expert tips is paramount. This section serves as a beacon of knowledge, shedding light on the intricate nuances that pave the way for regex proficiency. Delving deep into the core of regex operations, we unravel the foundational principles that underpin the crafting of efficient and effective regex patterns. Here, we not only underscore the significance of best practices but also elucidate on their practical application in real-world scenarios. Mastery of regex in Python demands a keen eye for detail, a meticulous approach, and a strategic mindset to navigate the labyrinth of string manipulation and pattern matching. By embracing the best practices outlined in this section, readers can elevate their regex skills to new heights, empowering themselves to tackle even the most complex of textual challenges with finesse and precision.