Mastering Lists in Python: A Complete Guide
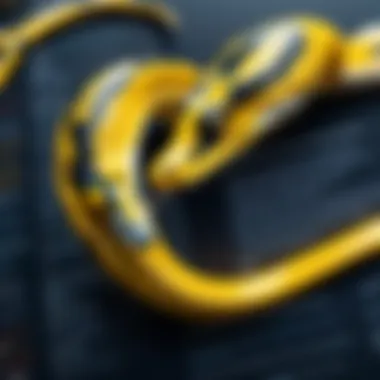
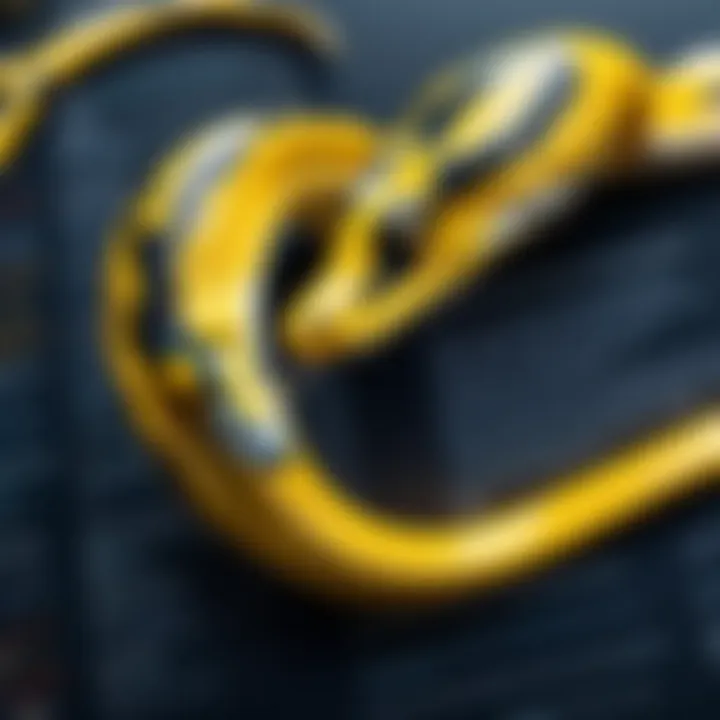
Intro
Python lists serve as one of the most versatile data structures available in programming. Lists are ordered collections of various data types, including strings, integers, and even other lists. This flexibility makes Python lists a vital aspect of building efficient and organized programs. Understanding how lists function can significantly impact a programmer’s ability to craft cleaner and more effective code.
Definition and Importance of Lists
A list in Python is defined by its ability to hold an ordered sequence of items. The items can be of heterogeneous types, meaning lists can contain integers alongside strings or even other lists. This characteristic allows for considerable freedom in data organization and manipulation.
Lists are important because they enable developers to store, access, and modify data efficiently. In many applications, data is frequently received or generated in bulk. Using lists helps manage this data systematically, thus streamlining programming logic and enhancing readability.
Key Features and Functionalities
Python lists come with several features that enhance their functionality:
- Mutability: Unlike some other data structures, lists can be modified after their creation. You can add, remove, or change items as needed.
- Dynamic Sizing: There's no need to declare a fixed size for a list; you can add as many elements as you require.
- Variety of Methods: Python provides built-in methods such as , , , and to manipulate lists easily.
These features make lists particularly adept for a wide range of tasks.
Use Cases and Benefits
Lists are practical tools in software development, with applications in various domains:
- Data Storage: Lists can hold user inputs, fetched data, or results from computations.
- Data Processing: Lists facilitate iterative processes, such as filtering or transforming data.
- Simulation: They can represent collections of entities in simulations or games.
The benefits stem from these capabilities, making projects more manageable and adaptable.
"Well-structured lists can simplify complex processes and improve overall code quality."
Best Practices
When working with lists, adhering to industry best practices can enhance efficiency:
- Clear Initialization: Initialize lists with a distinct purpose in mind to avoid ambiguity later.
- Limit Nesting: Keep nesting to a minimum to maintain readability.
- Choose Methods Wisely: Use list comprehension for concise data transformations.
Avoid common pitfalls such as underestimating mutability, which can lead to unintended side effects.
Finale
Understanding Python lists gives programmers a solid foundation for efficient coding practices. As a crucial component of Python's data handling capabilities, mastering lists opens a gateway to more complex programming challenges.
Prelims to Lists in Python
Lists in Python form a cornerstone of programming in this versatile language. A fundamental understanding of lists is crucial for anyone looking to develop their skills in Python, whether they are beginners or experienced programmers. Lists offer a simple yet powerful way to store and operate on collections of data. Their flexibility allows for a wide variety of applications, from basic data handling to complex algorithm implementations.
As we delve into this subject, it is important to highlight several specific elements. First, lists are mutable, which means you can change their contents without altering their identity. This is a significant benefit in programming, as it allows for dynamic data manipulation. Additionally, lists can hold items of different data types, including other lists, making them incredibly versatile. Understanding these capabilities prepares you for more advanced programming tasks and data structures later in your journey.
Moreover, identifying the various applications of lists can broaden your insight into effective coding strategies. Lists are not merely a way to collect items; they can be efficiently used in algorithms to sort data, perform calculations, or even handle user input.
In summary, mastering the concept of lists will significantly enhance your programming toolkit, allowing you to manipulate data structures in a way that elevates your coding practice to a higher level.
Definition and Importance of Lists
Lists are defined as ordered collections of items that can be changed, meaning you can add, remove, or modify items at any point. They are one of the built-in data structures in Python, making them fundamental. The order of items in a list is preserved, allowing for predictable access and manipulation. Lists are critical because they provide a basic method to store multiple data points under a single variable name.
Their importance cannot be overstated. As data grows in complexity and volume, lists allow for organized storage and retrieval. They are often the first data structure one learns in Python, setting the stage for deeper concepts, like nested lists and list comprehensions.
Applications of Lists in Programming
Lists have diverse applications, making them integral to various programming tasks. Here are some prominent use cases:
- Data Storage and Representation: Lists can act like arrays, storing multiple values for easy access, which is essential for handling collections of items.
- Iteration in Loops: Lists allow for easy iteration through items in loops, making it simple to apply operations on each element.
- Dynamic Sizing: Unlike fixed size arrays in other programming languages, Python lists can dynamically grow or shrink, adapting to the needs of your application.
- Integration with Functions: Lists are commonly used as arguments for functions, promoting reusable code and modular programming.
- Algorithm Implementation: Many algorithms, including sorting and searching, make extensive use of lists due to their ordered nature and flexibility.
By familiarizing yourself with the applications of lists, it becomes easier to implement practical solutions in your coding endeavors and solve real-world problems effectively. Through understanding lists, we unlock essential programming techniques that contribute to building robust applications.
Key Takeaway: Understanding the definition and applications of lists underpins more advanced Python programming techniques, making them indispensable in a developer's toolkit.
Creating Lists in Python
Creating lists in Python is fundamental to using the language effectively. Lists are versatile and essential data structures that allow programmers to store and manage collections of items. Understanding how to create lists opens up numerous possibilities for data manipulation and representation in programming tasks. It forms a foundational skill for every programmer, enabling efficient organization and retrieval of information.
Using List Literals
List literals provide a straightforward way to create lists. They allow developers to initialize a list with elements directly within square brackets. This method is intuitive and quick, especially when known values are available at the time of list creation.
For example:
In this example, contains integers, a string, and a boolean value. List literals support various data types, which is a core feature of lists. Moreover, the ability to nest lists within other lists is also possible, reflecting the capability of Python to handle more complex data structures.
Benefits of Using List Literals
- Simplicity: Using list literals is often the most effortless method of creating a list. It requires minimal syntax and is easy to read.
- Immediate Initialization: List literals allow for the immediate assignment of values, which can contribute to clearer and more maintainable code.
- Flexibility: You can mix different data types within a list, enhancing the ability to construct data collections suitable for a variety of scenarios.
Constructing Lists with the list() Function
Another method to create lists is by using the function. This approach is beneficial when converting other data structures, such as strings or tuples, into a list. The function takes an iterable as an argument and returns a new list object.
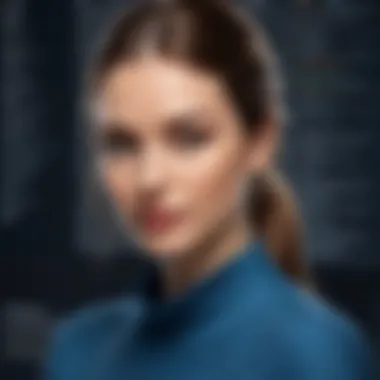
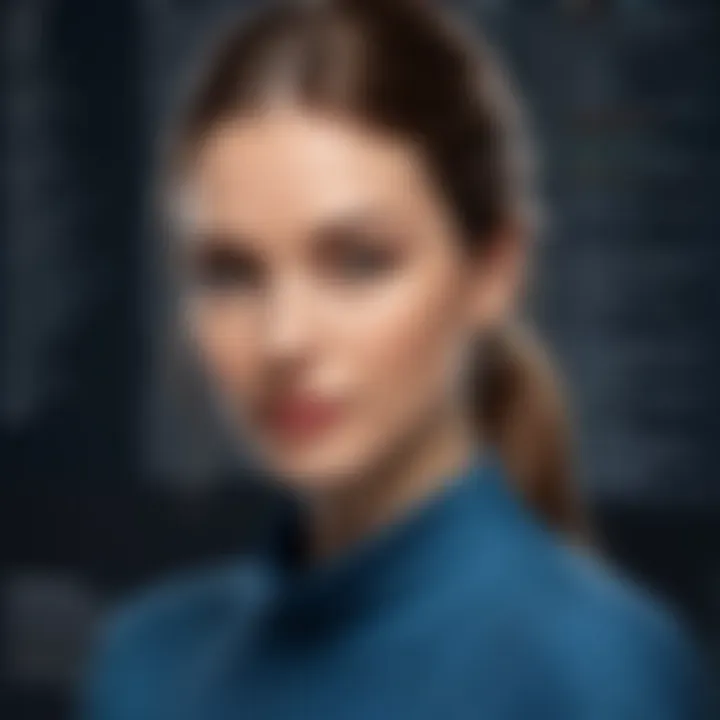
For instance:
In the first case, becomes , while will equal . This method is particularly advantageous when you need to manipulate or transform existing data structures into list format for further processing.
Considerations When Using list() Function
- Type Conversion: Be aware that will only work with iterable data types. Using it with non-iterable types will result in an error.
- Clarity: Sometimes, using can enhance code clarity when the data type is not immediately obvious, making the intent of list creation explicit.
By mastering these techniques for creating lists in Python, developers lay the groundwork for more complex operations and data management tasks in their programming endeavors.
Basic Operations on Lists
Understanding basic operations on lists is crucial for any programmer working with Python. It allows for effective data manipulation and enables developers to create robust applications. Mastering these operations not only enhances a programmer's ability to manage data but also aids in optimizing performance.
Accessing Elements
Accessing elements from a list is fundamental. In Python, lists are zero-indexed, which means the first element is at index 0, the second at index 1, and so on. For instance, if you have a list named , you can access the first fruit like this:
Through this operation, programmers can effectively retrieve data stored in lists, which is essential for further manipulation. One should also be mindful of attempts to access indices outside the list's range, which leads to an . This aspect becomes particularly relevant in applications dealing with dynamic data where the list length may vary.
Modifying List Elements
Modifying elements in a list is often necessary as data changes. Python provides straightforward methods for updates. You can change an item at a specific index easily:
This modification capability allows for numerous applications, such as correcting data entries or updating values based on user input. It's important to consider the implications of changing data, especially if other references point to the same list. This can affect overall structure and lead to unintended side effects in your code.
Removing Elements from Lists
Removing elements is another essential operation when working with lists. Python offers several built-in methods to delete items. The simplest approach is using the method, which eliminates the first occurrence of a specified element:
Alternatively, the method removes the last item by default but can take an index as an argument. This is useful for stacks or queues where the last or first item needs to be managed frequently. For example:
Removing or altering data in lists should be done cautiously to prevent losing important information. Backup copies of lists or using conditions before modifications can prevent data loss.
Advanced List Operations
Advanced list operations are crucial to fully leveraging the power of lists in Python. These operations enable developers to manipulate and access list data more effectively. They also enhance the efficiency and readability of the code, which is essential for maintaining high-quality software development practices. Understanding these operations is vital for anyone looking to improve their proficiency in Python and optimize their programming strategies.
Slicing Lists
Slicing lists allows programmers to extract specific portions of a list. This operation is performed using a simple syntax: . The index indicates where the slice begins, the index is exclusive, and controls the increment between each index. When you omit any parameters, Python defaults to the start and end of the list, as well as a step size of one.
Example of slicing:
Moreover, negative indexing allows for slicing from the end of the list. This adds flexibility when working with larger datasets or when only the last few elements are of interest.
Sorting and Reversing Lists
Sorting and reversing lists are fundamental operations that enhance data presentation and usability. The method sorts the list in place, while the function returns a new sorted list, leaving the original unchanged. You can specify the parameter to sort based on a specific criterion.
Example of sorting:
Reversing a list can be done using the method. This method simply changes the order of elements in the list without altering the values.
Example of reversing:
List Comprehensions
List comprehensions offer a concise way to create lists by iterating through iterable objects. This method combines the creation of new lists with loops and conditional statements in a single line, significantly reducing the amount of code needed. This feature not only saves time but also enhances the clarity and efficiency of the code.
An example of list comprehension:
Furthermore, list comprehensions can include conditionals to filter items during the creation of the list. Such flexibility makes it a powerful tool for data processing and manipulation.
In summary, mastering advanced list operations—slicing, sorting, reversing, and comprehensions—can significantly enhance the way programmers interact with data in Python. These operations not only simplify code but also improve performance and scalability, making them invaluable for successful programming.
Methods Associated with Lists
In programming, methods are essential tools that facilitate operations on data structures. Understanding methods associated with lists provides developers with efficient techniques to manipulate and manage collections of data. Lists in Python come equipped with a range of built-in methods that enable seamless addition, removal, and alteration of elements. Each method serves a distinct purpose and can significantly impact the performance of applications. Coping with data effectively requires knowledge of these methods, enhancing overall programming skills and improving software design.
Common List Methods
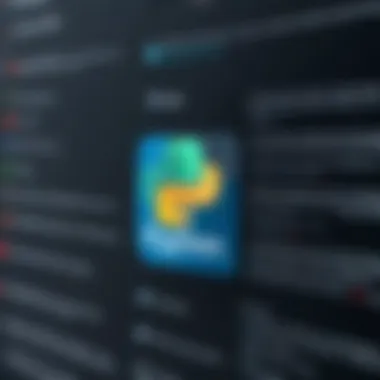
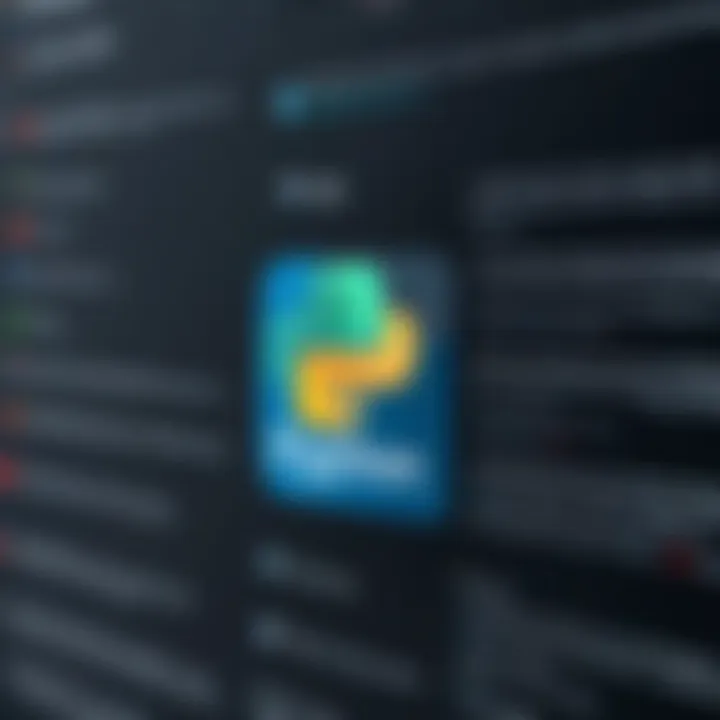
The following are some of the most frequently utilized list methods in Python:
append()
The method adds a single element to the end of a list. This method modifies the list directly, which means it does not return a new list. Its primary characteristic is simplicity, making it a beneficial choice for many developers. For example, appending an item is straightforward when building lists dynamically.
A unique feature of is its efficiency with larger lists. It operates in O(1) time complexity, allowing fast addition. However, users should note that can only add one element at a time. If multiple additions are required, one may find this method less efficient compared to others, like .
extend()
The method is used to add multiple elements to the end of a list. Unlike , can take an iterable, such as a list or a tuple, allowing for the convenient merging of collections. This characteristic is a significant advantage in situations where bulk data insertion is needed.
One downside of is that it directly modifies the original list. This property can lead to unintended consequences if the original list needs to be preserved. Overall, when working with multiple items, is an effective choice for maintaining code clarity.
insert()
The method introduces an element at a specified index within the list. By specifying the position, developers can manage the order of elements effectively. This method's flexibility makes it a notable feature, especially in scenarios that require ordered data structures.
One notable disadvantage is its O(n) time complexity due to the need to shift elements when inserting. Thus, while it’s powerful, might not be the fastest method for frequent insertions.
remove()
The method eliminates the first occurrence of a specified value from the list. Its primary function focuses on ease of use, enabling developers to delete items without the need for index knowledge. This characteristic is particularly valuable when dealing with data where the item's position is unknown.
However, if the value does not exist in the list, a is raised. This potential for error necessitates careful management and sometimes additional error handling when using it.
pop()
The method serves a dual purpose: it removes the item at a specified index and returns that item. If no index is specified, it defaults to the last item in the list. This method appeals to developers as it both modifies the list and retrieves a value simultaneously, aiding in scenarios where both actions are necessary.
However, similar to , it can raise an if the list is empty. Caution is thus needed to ensure that the list contains items before invoking this method.
index()
The method returns the first index of a specified value in the list. Its utility lies in quickly locating items within larger datasets. Thus, it is essential for operations that involve searching.
Yet, like some previous methods, if the value is not found, it raises a . This limitation can be a drawback in circumstances where a non-existent value might be queried, necessitating additional checks before usage.
count()
The method counts the total occurrences of a specified value within the list. It provides developers with crucial insights into data distribution, particularly essential for statistics and data analytics.
Its main limitation is its runtime complexity of O(n) since it needs to iterate through the entire list. In datasets with substantial sizes, this may not be optimal, but for smaller lists, it remains a highly effective tool.
List Methods for Searching and Counting
These methods are key for manipulating data efficiently. Knowledge about searching and counting helps inform decision-making in programming. Developers can leverage these operations to optimize performance through effective list handling.
Nested Lists
Nested lists represent a crucial concept within Python that enhances the flexibility and efficiency of data organization in programming. This structure allows developers to create lists that include other lists as elements. The ability to nest lists is particularly useful when working with complex data models, where related data is grouped together in a hierarchical manner. For example, a nested list can represent a matrix or a grid, where each element is itself a list. This capability enables data scientists, software developers, and IT professionals to manage data with multiple dimensions, promoting better organization and manipulation of information.
Understanding Multidimensional Lists
Multidimensional lists, often referred to as nested lists, facilitate the representation of data in multiple dimensions. They enable users to create structures that can represent two or more levels of data. For instance, a 2D list can effectively model a grid or a table. Below is an example of a simple 2D list:
In this example, contains three lists, each representing a row of the grid. Accessing elements in multidimensional lists is done using multiple indices, like so:
This statement retrieves the element from the second row and third column. Understanding the structure of multidimensional lists is vital for programmers who need to perform advanced data manipulation or matrix operations.
Accessing and Modifying Nested Lists
Accessing elements within nested lists requires a specific approach. Each element can be accessed using its coordinate, which is defined by the outer and inner index. When modifying elements, similar logic applies. For example, to change the value at a specific location in the previously defined , one would write:
After this operation, the first row of the matrix would now contain the values [1, 10, 3]. Adjusting multilevel lists requires careful attention to ensure the correct indices are targeted.
It is also beneficial to understand how to iterate through nested lists for various tasks such as printing values or processing them. Here’s an example of iterating through a 2D list using a nested loop:
This loop traverses each row and prints its elements.
In summary, nested lists offer superior data management capabilities, essential for complex programming tasks. Understanding how to access and modify these structures plays a crucial role in effective data manipulation.
Performance Considerations
Understanding the performance considerations of lists in Python is crucial for software developers and data scientists. Lists are a widely used data structure, but their efficiency can be impactful when working with large datasets or complex algorithms. When discussing performance, we mainly refer to two aspects: time complexity and space complexity.
Time Complexity of List Operations
The time complexity of list operations in Python varies depending on the specific action performed. Here are common operations and their associated complexities:
- Accessing an element: This is done in constant time, O(1). You can retrieve any element in a list directly using its index.
- Appending an element: Also a constant time operation, O(1). When you add an element to the end of a list, Python efficiently reallocates memory when necessary.
- Inserting an element: This has a time complexity of O(n). If you insert an element in the middle of a list, Python has to shift all subsequent elements.
- Removing an element: Similar to insertion, removing an element can also take O(n) time due to the need to shift remaining items.
- Sorting a list: The time complexity of sorting using the built-in method is O(n log n).
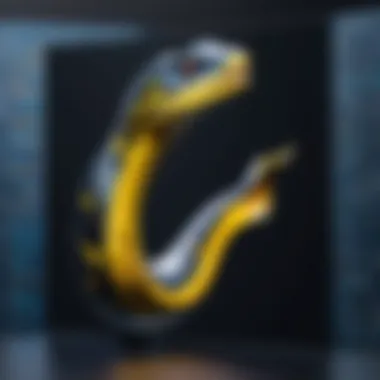
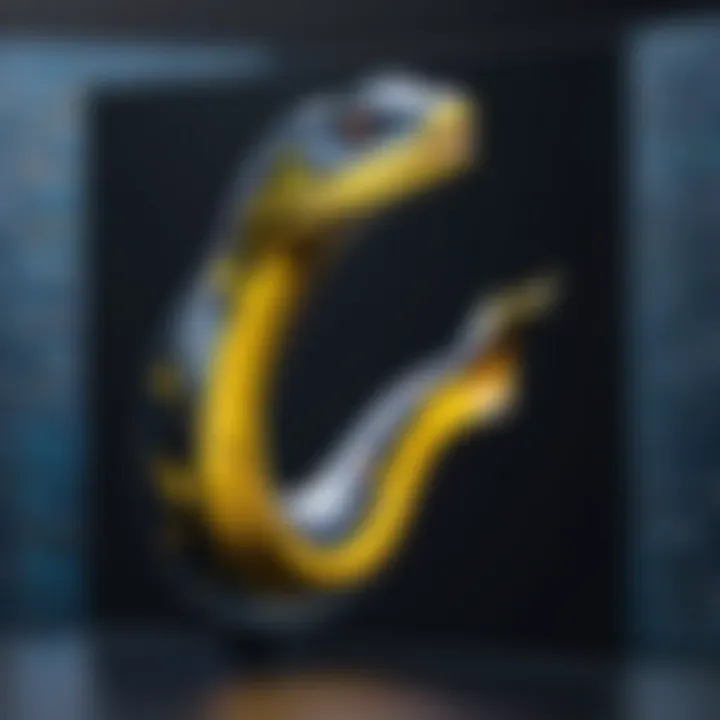
Understanding these values is vital for designing efficient algorithms, especially when lists are used in operations that repeat or scale up.
Space Complexity of Lists
Space complexity refers to the amount of memory a list occupies in relation to the number of elements in it. Lists in Python are dynamic arrays, meaning they can grow as needed. Some important points regarding space complexity are:
- Initial Allocation: When you create a list, Python allocates a certain amount of memory. As elements are added, Python may need to allocate more memory to accommodate additional items.
- Amortized Space Complexity: The average space complexity of a list remains O(n) where n is the number of elements in the list. This is due to the dynamic resizing of the array when new elements are added.
- Wasted Space: It is worth noting that additional memory might be used for internal management of space, meaning that the actual memory used can sometimes exceed the amount allocated for the elements alone.
Memroy managemnt affects the use of lists, particularly when working with large datasets. Be aware of performance when designing your systems.
In summary, considering both the time and space complexity allows better insight into how to effectively use lists in Python, assisting in making informed decisions about data structure choices in programming.
Comparing Lists with Other Data Structures
Comparing lists with other data structures is crucial to understanding their unique characteristics and optimal use cases in programming. Lists in Python serve a versatile role—they are ordered, changeable collections, which makes them a preferred choice for many scenarios. However, knowing when to use a list as opposed to structures like tuples, sets, or dictionaries can significantly impact a program's efficiency and performance.
Lists vs. Tuples
Lists and tuples are both used to store collections of items in Python, yet they differ in some key aspects. The most significant difference is in mutability. Lists are mutable, meaning you can change their content after they have been created. On the other hand, tuples are immutable. Once a tuple is defined, you cannot alter its elements.
- Use Cases: Tuples are often used for fixed collections of items, such as coordinates or database records, where the integrity of the data must not change. In contrast, lists come in handy when data is dynamic and needs to be modified.
- Performance: Due to their immutability, tuples can offer better performance than lists when dealing with large datasets. The memory usage of tuples is generally lower since they do not require extra memory for storing the methods associated with mutable objects.
Lists vs. Sets
Sets are another collection type in Python, and they differ from lists in terms of ordering and uniqueness. Sets are unordered collections of unique elements, which makes them ideal for membership testing and eliminating duplicates.
- Duplicates: Lists allow duplicate values, while sets do not. If maintaining unique entries is a priority, sets provide a better choice.
- Performance Considerations: Operations such as membership testing (e.g., checking if an item exists) are faster with sets than with lists. This is an important consideration when performance is a concern in applications that process large amounts of data.
Lists vs. Dictionaries
Dictionaries are key-value pairs and serve a different purpose compared to lists. While lists are ordered collections solely based on index, dictionaries provide a mapping of keys to values.
- Key-based Access: Lists use index-based access, making them ideal for cases where the order of items is important. Conversely, dictionaries enable faster lookups by key, which is beneficial in contexts where rapid access to items is needed.
- Complexity: Lists are simpler, whereas dictionaries can manage more complex structures. If there is a need to associate values with keys, dictionaries become essential.
Understanding these distinctions allows programmers to choose the appropriate structure based on the specific requirements of their projects. Each data structure has its strengths and weaknesses, and the choice often depends on factors like performance, mutability, and whether ordering is a concern.
"Choosing the right data structure is a fundamental skill in programming, influencing both efficiency and clarity of code."
Selecting between lists, tuples, sets, and dictionaries ensures not only the effectiveness of the code but also a clearer understanding of the data's role within the application. By grasping how these structures compare, programmers can make more informed choices that align with their design goals.
Practical Examples and Use Cases
In the realm of programming, theoretical knowledge must be complemented with practical application. Understanding how lists operate in real-world scenarios is essential for any developer. Practical examples not only illustrate the versatility of lists but also showcase best practices in various programming contexts. By examining concrete use cases, one can grasp the intrinsic value of lists and how they simplify data handling and processing tasks.
Using Lists for Data Storage
Data storage is one of the primary applications of lists. In Python, lists offer a dynamic way to store collections of items without needing to declare a fixed size. This flexibility makes lists ideal for scenarios where the amount of data is unknown or can change over time. For instance, if you are building an application that collects user responses, a list can store these entries efficiently as they arrive.
Consider a practical example:
Here, dynamically grows to accommodate each new entry. Using lists for data storage provides not only ease of implementation but also powerful manipulation capabilities, such as filtering, sorting, and accessing data by index.
Lists in Algorithms
Lists also play a significant role in algorithm development. Many algorithms rely on lists for efficient data manipulation. Sorting algorithms, searching algorithms, and even more complex algorithmic strategies like quicksort and mergesort utilize lists as their fundamental data structure.
For example, consider a simple sorting operation:
This snippet demonstrates how easy it is to manipulate lists directly in Python. The method modifies the list in place, showcasing the efficiency of lists in algorithm design.
By using lists in algorithms, developers can efficiently manage collections of elements, allowing for systematic processing and analysis of data. Lists serve as a foundation for more advanced data structures and contribute to the foundational logic of programming projects.
"Lists are not just a feature of Python; they are a fundamental construct that shapes how data is managed in software applications."
In summary, the importance of practical examples and use cases of lists in Python cannot be overstated. By integrating lists into data storage solutions and algorithm design, programmers can enhance their coding practices and ensure their solutions are both efficient and effective.
Common Errors and Debugging Lists
When working with lists in Python, errors can occur that hinder the functionality of a program. Understanding common errors and debugging techniques is crucial for programmers of all levels. By recognizing these challenges, developers can effectively troubleshoot issues, ensuring smoother code execution and better performance. Debugging is not just about fixing errors; it is an opportunity to improve one’s coding skills and enhance overall knowledge.
List-related errors typically arise from incorrect index usage, improper list modifications, or unintended data types. Identifying these errors promptly minimizes time spent on debugging and allows for faster development cycles. Additionally, debugging tools provide visual insights into list states, helping in pinpointing issues quickly.
Identifying and Resolving List Index Errors
List index errors are one of the most common mistakes made by programmers. These errors occur when accessing an element outside the defined range of indices for a list. In Python, lists are zero-indexed. This means that the first element of a list is accessed using index 0, the second with index 1, and so forth. If a programmer attempts to access a negative index that is not valid or exceeds the list's bounds, Python will raise an .
To resolve these errors, it is important to:
- Check the Length of the List: Always verify the list size before accessing an element. Using allows you to confirm the number of elements.
- Use Exception Handling: Implementing blocks can catch and handle it gracefully, providing a better user experience.
- Debugging Statements: Print statements can illuminate the current state of the list and the indices being accessed, aiding in the identification of mistakes.
For example, the following code snippet demonstrates how to handle an :
Using Debugging Tools for Lists
Utilizing debugging tools can significantly enhance the ability to find and fix errors related to lists. These tools offer powerful features that can provide more context about the internal state of a program. Here are some recommendations:
- Python Debugger (pdb): This built-in module enables stepping through code execution. With commands such as to move to the next line or to print variable values, it is effective for understanding list changes during runtime.
- Integrated Development Environments (IDEs): Many IDEs provide in-built debugging capabilities. For example, PyCharm offers visual representations of lists and allows you to view and modify them during execution.
- Online Debugging Tools: Websites like repl.it offer online coding environments with debugging features. This is useful for quick testing and debugging without the need to set up a local environment.
By leveraging these tools, one can gain insights and rectify problems related to lists more efficiently.
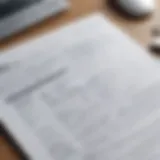
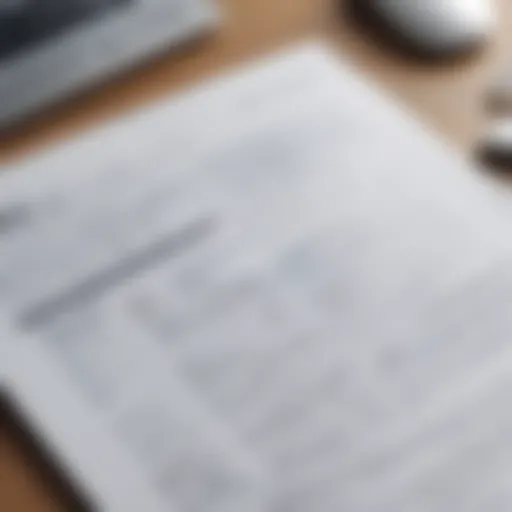