Unlocking the Secrets of JavaScript Programming Mastery: A Comprehensive Guide
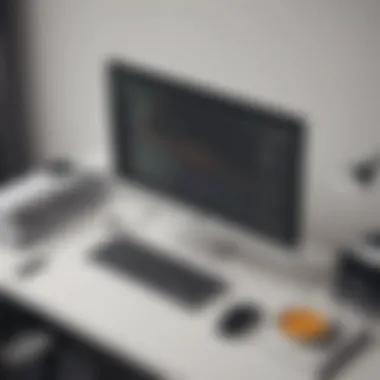
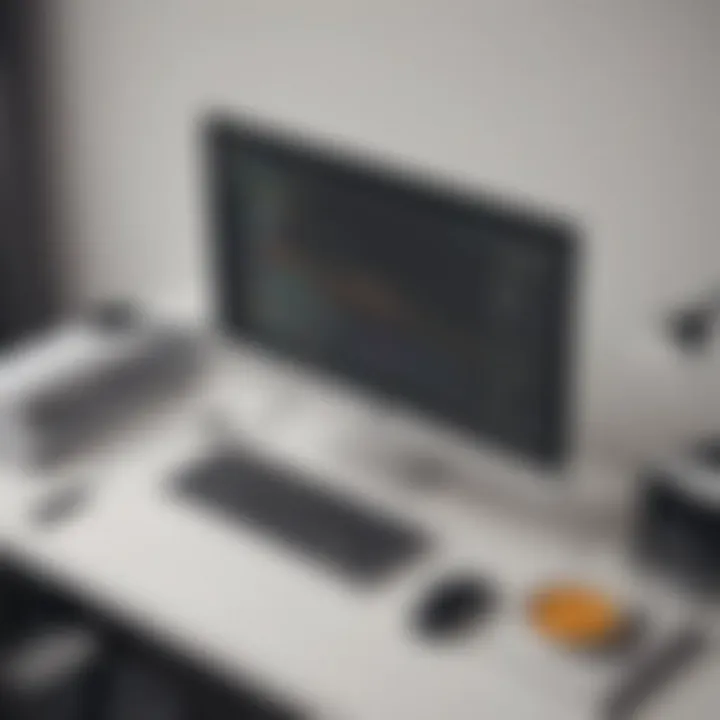
Overview of JavaScript Programming
JavaScript is a versatile and widely used programming language in web development. Its significance lies in its ability to create interactive and dynamic web pages, making it essential for both frontend and backend development. The language allows developers to add functionality, validate forms, create cookies, and much more, enhancing the overall user experience. JavaScript's key features include event-driven programming, asynchronous communication, and cross-platform compatibility, making it a fundamental tool for modern web development.
Best Practices
When delving into mastering JavaScript, there are several industry best practices to follow. It is crucial to maintain clean and organized code, adhere to coding conventions such as naming conventions, and utilize design patterns to enhance code reusability. Maximizing efficiency and productivity involves optimizing performance through techniques like code minification, reducing HTTP requests, and utilizing caching mechanisms. Furthermore, avoiding common pitfalls like callback hell, memory leaks, and overly complex code structures is essential to ensure maintainable and scalable JavaScript applications.
Case Studies
Examining real-world examples of successful JavaScript implementation provides invaluable insights into best practices. Case studies showcase how prominent websites leverage JavaScript to enhance user interactivity and overall functionality. By dissecting these implementations, developers can learn from the experiences of industry experts and understand the challenges faced and solutions implemented to achieve desired outcomes. Insights gathered from case studies offer practical knowledge that can be applied to personal projects, improving the quality and efficiency of JavaScript development.
Latest Trends and Updates
JavaScript continues to evolve rapidly, with upcoming advancements shaping the future of web development. Current trends focus on progressive web applications, serverless architecture, and the integration of machine learning with JavaScript. Industry forecasts predict a rise in JavaScript frameworks and libraries, emphasizing the importance of staying updated with the latest tools and technologies. Innovations such as WebAssembly and GraphQL are revolutionizing the JavaScript landscape, offering new possibilities for developers to enhance performance and scalability in their projects.
How-To Guides and Tutorials
For beginners and advanced users looking to master JavaScript, comprehensive how-to guides and tutorials are essential. Step-by-step instructions on setting up development environments, building interactive web applications, and utilizing frameworks like React or Angular provide practical insights into JavaScript programming. Hands-on tutorials offer a hands-on approach to learning, enabling developers to apply theoretical knowledge to real-world scenarios effectively. Practical tips and tricks, such as debugging techniques, performance optimization, and code refactoring, help elevate the coding skills of JavaScript enthusiasts at all levels of expertise.
Introduction
In the realm of JavaScript programming, mastering the basics is paramount. Understanding the foundational principles sets the stage for advanced coding prowess. This section serves as the stepping stone towards a comprehensive grasp of JavaScript, laying the groundwork for intricate programming techniques and concepts to follow.
Understanding JavaScript Basics
Variables and Data Types
Variables and data types constitute the building blocks of any programming language, and JavaScript is no exception. Variables allow for dynamic storage of data, while data types define the kind of data that can be stored. The versatility of variables and the flexibility of data types empower developers to manipulate information efficiently, enabling robust code structures and logical operations. However, aspiring JavaScript programmers must tread carefully to prevent common pitfalls such as type coercion and variable scoping issues.
Functions and Scope
Functions in JavaScript play a pivotal role in structuring code and encapsulating logic. With the ability to define and invoke functions, developers can create reusable blocks of code, enhancing code organization and maintainability. Scope, on the other hand, dictates the accessibility of variables within functions and blocks of code. Understanding function scope is essential for preventing variable conflicts and ensuring code behaves as intended. Exploring functions and scope sets the stage for mastering complex programming patterns and methodologies.
Control Flow
Control flow mechanisms in JavaScript dictate the order in which code is executed based on conditional statements and looping constructs. Efficient control flow enhances code efficiency and logic structuring, enabling developers to handle varying scenarios and make informed decisions within their applications. Delving into control flow equips programmers with the tools to create dynamic and responsive code structures, fostering a deeper understanding of logical operations and algorithmic thinking.
Setting Up Your Development Environment
Choosing an IDE or Text Editor
The choice of an Integrated Development Environment (IDE) or text editor significantly influences a developer's workflow and productivity. Selecting a suitable IDE tailored to JavaScript development streamlines coding tasks and offers essential features such as syntax highlighting, code completion, and debugging tools. Conversely, opting for a minimalist text editor provides simplicity and agility for quick coding sessions. Understanding the dynamics of different IDEs and text editors empowers developers to customize their environments for optimal coding efficiency and comfort.
Installing Node.js and npm
Node.js and npm (Node Package Manager) form the backbone of modern JavaScript development, enabling server-side scripting and package management capabilities. Installing Node.js grants access to a vast ecosystem of JavaScript libraries and tools, fostering a modular and efficient development process. Leveraging npm simplifies dependency management, facilitating seamless integration of external packages and modules into projects. Mastering the installation and utilization of Node.js and npm elevates developers' ability to build scalable and robust JavaScript applications.
Utilizing Version Control Systems
Version control systems such as Git revolutionize collaborative coding practices by enabling team members to track changes, review code history, and resolve conflicts effectively. Integrating version control ensures code integrity, facilitates seamless collaboration, and mitigates the risk of data loss. Understanding the fundamentals of version control systems equips developers with essential skills for managing code bases, streamlining workflows, and upholding best practices in software development.
Exploring Essential JavaScript Concepts
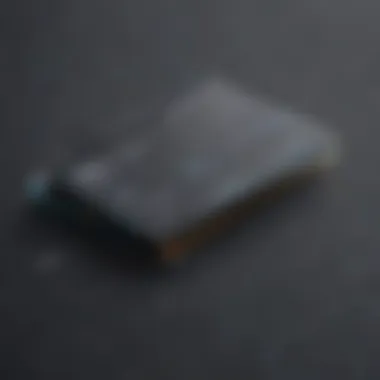
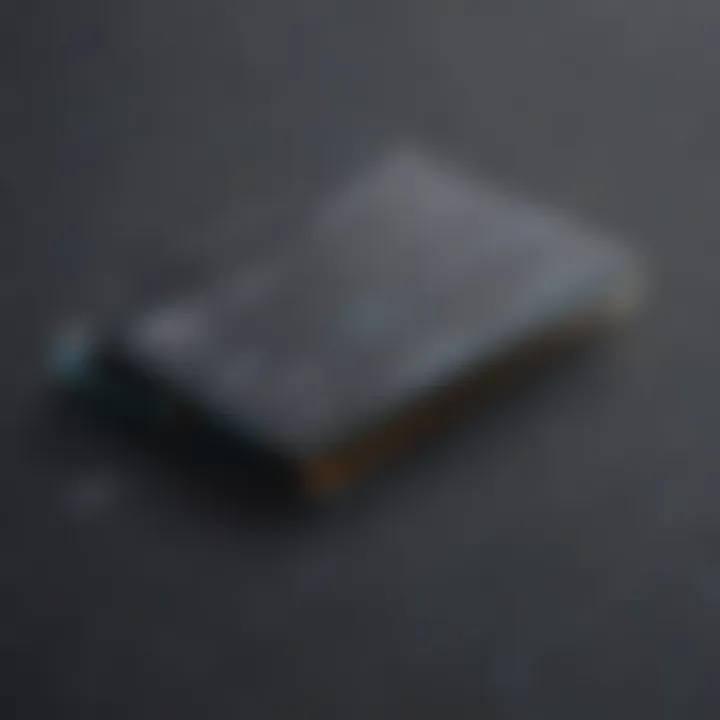
Arrays and Objects
Arrays and objects serve as fundamental data structures in JavaScript, allowing developers to store and manipulate collections of data with ease. Arrays facilitate the storage of multiple elements in a single variable, enabling efficient data processing through iteration and manipulation. Objects, on the other hand, define custom data structures with key-value pairs, fostering the creation of complex and versatile data models. Harnessing the power of arrays and objects empowers developers to design scalable and dynamic applications, bolstering data management and processing capabilities.
Loops and Iteration
Loops are essential constructs in JavaScript for repeating tasks and iterating over data collections. Understanding loop mechanisms enhances code efficiency and readability, enabling developers to automate repetitive tasks and streamline data processing operations. Iteration offers a systematic approach to data traversal and manipulation, optimizing code performance and fostering algorithmic problem-solving skills. Mastering loops and iteration techniques expands developers' ability to handle iterative tasks and manage data flow seamlessly within their applications.
Error Handling
Error handling is a critical aspect of JavaScript programming, ensuring the graceful handling of exceptions and unforeseen scenarios within applications. Implementing effective error handling mechanisms improves code reliability and user experience, preventing crashes and unexpected behavior. Strategies like try-catch blocks and error logging enable developers to identify and resolve issues proactively, enhancing application stability and robustness. Prioritizing error handling techniques equips developers with the agility to troubleshoot and mitigate potential software failures, promoting quality assurance and user satisfaction.
Advanced JavaScript Programming
In the realm of JavaScript programming, the focus shifts towards more intricate topics under Advanced JavaScript Programming. This segment serves as a pivotal point in the comprehensive guide, where developers embark on a journey to harness the full potential of JavaScript. Advanced JavaScript Programming extends beyond basic syntax and functionalities, delving into sophisticated concepts that push the boundaries of conventional coding practices. By exploring advanced techniques, developers can elevate their programming skills and tackle complex challenges with finesse.
Functional Programming in JavaScript
Higher-order Functions
Higher-order Functions play a critical role in functional programming paradigms within JavaScript. These functions can accept other functions as arguments or return them, providing a higher level of abstraction and flexibility in coding. The key characteristic of Higher-order Functions lies in their ability to enhance code readability and reusability by encapsulating behavior into functions. Their versatility makes them a popular choice in this article, contributing to the overall goal of mastering JavaScript by promoting modular and concise code structures. The unique feature of Higher-order Functions lies in their capacity to enable the implementation of more complex algorithms and functional patterns, albeit requiring a solid understanding to leverage their advantages effectively.
Lambda Functions
Lambda Functions, also known as anonymous functions, offer a concise way to define functions in JavaScript. Their key characteristic lies in their ability to shorten code snippets by omitting the function's name and reducing unnecessary verbosity. Within the context of this article, Lambda Functions are a beneficial choice as they promote cleaner code aesthetics and streamline the development process. The unique feature of Lambda Functions is their support for functional programming principles, enabling developers to write more declarative and succinct code. Despite their advantages in promoting code efficiency, Lambda Functions may pose challenges for beginners due to their abstract nature, requiring a solid grasp of functional programming concepts to utilize effectively.
Recursion
Recursion embodies a powerful concept in JavaScript programming, allowing functions to call themselves within their execution. The key characteristic of Recursion lies in its ability to solve complex problems by breaking them down into smaller, more manageable subproblems. Within this article, Recursion plays a crucial role in advancing the understanding of algorithmic design and problem-solving strategies. The unique feature of Recursion is its elegance in tackling repetitive tasks and data structures, offering a sophisticated approach to coding challenges. While Recursion provides an elegant solution to certain problems, it comes with the caveat of consuming more memory and potentially leading to stack overflow errors if not implemented judiciously.
Asynchronous JavaScript
Callbacks and Promises
In the realm of asynchronous programming, Callbacks and Promises stand out as essential constructs for managing non-blocking operations in JavaScript. Callbacks serve as functions that execute once an asynchronous operation completes, while Promises offer a more structured approach to handling asynchronous tasks. Their key characteristic lies in enabling developers to write asynchronous code that is easier to read and maintain, aligning with the overarching goal of this article. The unique feature of Callbacks and Promises lies in their ability to mitigate callback hell and streamline the execution flow of asynchronous operations. While they empower developers with efficient asynchronous programming techniques, Callbacks and Promises can introduce complexities in handling errors and ensuring proper error propagation.
AsyncAwait Syntax
The AsyncAwait Syntax revolutionizes asynchronous programming in JavaScript by simplifying the syntax for writing asynchronous code that behaves synchronously. Its key characteristic lies in combining Promises with a more sequential approach, enhancing code readability and maintenance. Within the context of this article, AsyncAwait Syntax emerges as a popular choice for simplifying complex asynchronous operations and improving code comprehension. The unique feature of AsyncAwait Syntax is its intuitive nature, making it easier for developers to manage asynchronous tasks without succumbing to callback hell. Despite its advantages in enhancing code readability, AsyncAwait Syntax may require a solid grasp of Promises and error handling to leverage its benefits optimally.
Handling AJAX Requests
Handling AJAX Requests is crucial for interacting with web servers asynchronously, allowing data exchange without refreshing the entire web page. The key characteristic of AJAX Requests lies in their ability to enhance user interactions by fetching data dynamically, aligning with the principles of modern web development emphasized in this article. The unique feature of Handling AJAX Requests is their role in optimizing user experience by retrieving data in the background without disrupting the main page flow. While AJAX Requests empower developers to create dynamic and interactive web applications, they introduce considerations around security vulnerabilities and user privacy, necessitating thorough data validation and error handling mechanisms.
Design Patterns and Best Practices
Singleton Pattern
The Singleton Pattern serves as a creational design pattern that restricts the instantiation of a class to a single instance, emphasizing global access and centralized control. Its key characteristic lies in ensuring that only one instance of a class exists throughout the application's lifecycle, promoting memory efficiency and resource management. Within this article, the Singleton Pattern emerges as a valuable choice for maintaining consistent states and sharing common resources across different parts of the codebase. The unique feature of the Singleton Pattern lies in its ability to provide a global point of access to a single resource, enhancing code modularity and scalability. While the Singleton Pattern offers advantages in promoting data consistency and resource sharing, it may introduce challenges related to testing and extensibility, requiring careful consideration in its implementation.
Module Pattern
The Module Pattern represents a structural design pattern in JavaScript that enables encapsulation and organization of code into discrete modules, fostering reusability and maintainability. Its key characteristic lies in facilitating modular development practices by defining private and public interfaces within modules, promoting code encapsulation. Within the scope of this article, the Module Pattern emerges as a popular choice for structuring JavaScript codebases and managing dependencies effectively. The unique feature of the Module Pattern is its ability to prevent global namespace pollution and support the creation of encapsulated components with clear interfaces. Despite its advantages in promoting code organization and reusability, the Module Pattern may involve complexities in module interdependency and require additional tooling for efficient module loading and bundling.
Error-First Callbacks
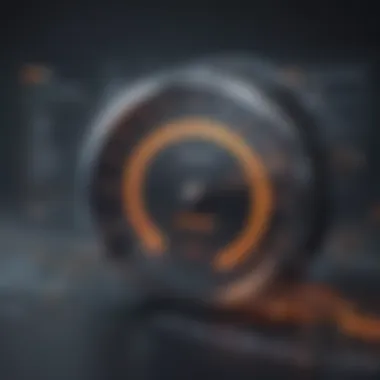
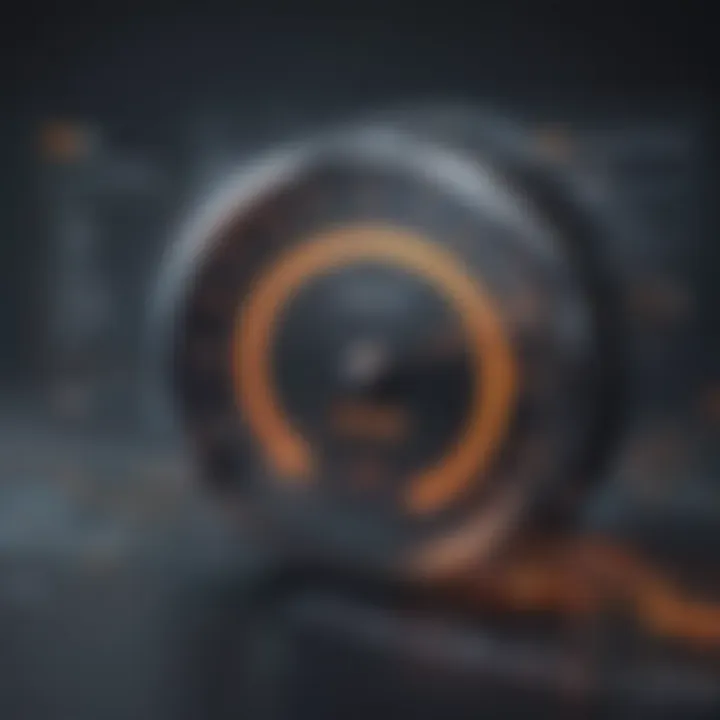
Error-First Callbacks represent a coding convention in JavaScript where callback functions receive the error as the first parameter, enabling robust error handling in asynchronous operations. Their key characteristic lies in promoting error-first best practices, where error propagation and handling take precedence over regular execution flow. Within this article, Error-First Callbacks play a critical role in implementing reliable error-handling mechanisms and promoting code resilience. The unique feature of Error-First Callbacks is their emphasis on handling errors proactively, reducing unexpected failures and enhancing the robustness of asynchronous operations. While Error-First Callbacks offer advantages in improving code reliability, they may introduce verbosity in callback signatures and necessitate meticulous error checking and propagation strategies for efficient error management.
Optimizing JavaScript Performance
In the expansive realm of JavaScript programming, optimizing performance stands as a critical facet warranting thorough exploration. Acknowledging the significance of optimizing JavaScript is akin to enhancing the execution efficiency and overall functionality of your codebase. Operating within the digital landscape demands sharp attention to detail and proficiency in optimizing JavaScript to ensure seamless performance across various platforms and devices. By delving into the intricacies of performance optimization, developers can mitigate bottlenecks, streamline processes, and improve user experience.
Code Optimization Techniques
Minification and Compression
Unpacking the concept of minification and compression unveils a pivotal practice in the realm of JavaScript programming. Minification entails the process of removing unnecessary whitespace, comments, and restructuring code to enhance its efficiency without altering functionality. On the other hand, compression focuses on reducing file sizes by employing techniques like gzip compression, thereby boosting loading speeds and resource utilization. Integrating minification and compression techniques into JavaScript workflows can substantially improve website performance, reduce bandwidth consumption, and elevate overall optimization levels.
Caching Strategies
The strategic utilization of caching emerges as a cornerstone in augmenting JavaScript performance metrics. By implementing caching strategies, developers can store frequently accessed data or computed values temporarily, circumventing redundant operations and accelerating response times. Employing browser caching, CDNs, and server-side caching mechanisms empowers developers to heighten website responsiveness, diminish server load, and fortify scalability aspects. Embracing caching strategies not only expedites data retrieval processes but also instills resilience against surges in traffic and resource-intensive operations.
Reducing DOM Manipulation
Addressing the pivotal role of reducing DOM manipulation in optimizing JavaScript performance underscores a key aspect of proficient web development practices. Excessive manipulation of the Document Object Model (DOM) can significantly impair rendering speeds and execution efficiency. By minimizing DOM manipulation through batch operations, utilizing virtual DOM structures, or optimizing event delegation, developers can avert performance bottlenecks, enhance webpage responsiveness, and ensure smoother user interactions. Prioritizing minimal DOM manipulations contributes to agile, high-performing web applications and fosters a seamless user experience.
Browser Rendering and Rendering
Embarking on an exploration of browser rendering and rendering mechanisms unravels the essential dynamics underpinning JavaScript optimization endeavors. Understanding the critical rendering path in web browsers illuminates the sequence of steps involved in processing and displaying content, elucidating opportunities for streamlining rendering processes and enhancing performance benchmarks. Harnessing insights into optimizing CSS and JavaScript delivery refines resource loading strategies, refactoring code structures, and economizing network requests to expedite rendering speeds and fortify website responsiveness. Implementing lazy loading techniques further refines rendering mechanisms, prioritizing the efficient loading of critical content and deferring non-essential resources until requisite, thereby bolstering performance metrics and user satisfaction levels.
Testing and Debugging JavaScript Applications
Testing and debugging JavaScript applications are crucial aspects of the programming process. By ensuring the code performs as expected and identifying any issues or errors, developers can deliver high-quality software. Effective testing can catch bugs early in the development cycle, saving time and resources. Debugging, on the other hand, helps in pinpointing and rectifying errors to ensure smooth functionality. Both testing and debugging promote code reliability and overall application performance.
Unit Testing with Jest and Mocha
Unit testing with Jest and Mocha is a fundamental practice in JavaScript development. Writing test suites allows developers to systematically test individual components of their code, ensuring each part functions correctly. Jest and Mocha are popular testing frameworks known for their simplicity and efficiency. Writing test suites provides a structured approach to testing, helping developers validate the behavior of their code under various scenarios. While Jest is favored for its ease of use and speed, Mocha offers flexibility and customization options to suit different testing needs.
- Writing Test Suites
Writing Test Suites
Writing test suites involves creating a set of test cases to verify the expected behavior of functions and modules within an application. This process ensures that each unit of code works as intended and helps in detecting any deviations from the desired functionality. Test suites enhance code quality by validating its performance under diverse conditions, leading to more robust and reliable applications. The structured nature of test suites aids in continuous testing and maintenance of the codebase, contributing to its overall stability.
- Mocking Dependencies
Mocking Dependencies
Mocking dependencies is a crucial practice in unit testing, allowing developers to isolate and test individual components independently. By simulating external dependencies or functions, developers can mimic real-world scenarios and evaluate the behavior of the code in isolation. Mocking dependencies facilitates faster and more focused testing, enabling developers to identify and resolve issues efficiently. While it streamlines the testing process, it is essential to ensure that the mocked dependencies accurately represent the actual behavior to obtain reliable test results.
- Running Tests
Running Tests
Running tests is the final step in the unit testing process, where developers execute the test suites to validate the code's functionality. By running tests regularly, developers can catch errors early, facilitating timely bug fixes and code improvements. Running tests not only confirms the correctness of the code but also instills confidence in its reliability and performance. Through test automation and continuous integration, developers can streamline the testing process and maintain code quality throughout the development lifecycle.
Debugging Tools and Techniques
Debugging tools and techniques are indispensable for identifying and resolving errors in JavaScript applications. Utilizing browser DevTools, logging, and remote debugging capabilities, developers can diagnose issues and troubleshoot code effectively. These tools provide insights into the application's behavior, allowing developers to trace and rectify errors efficiently.
- Using Browser DevTools
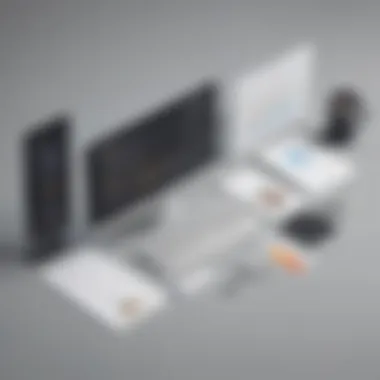
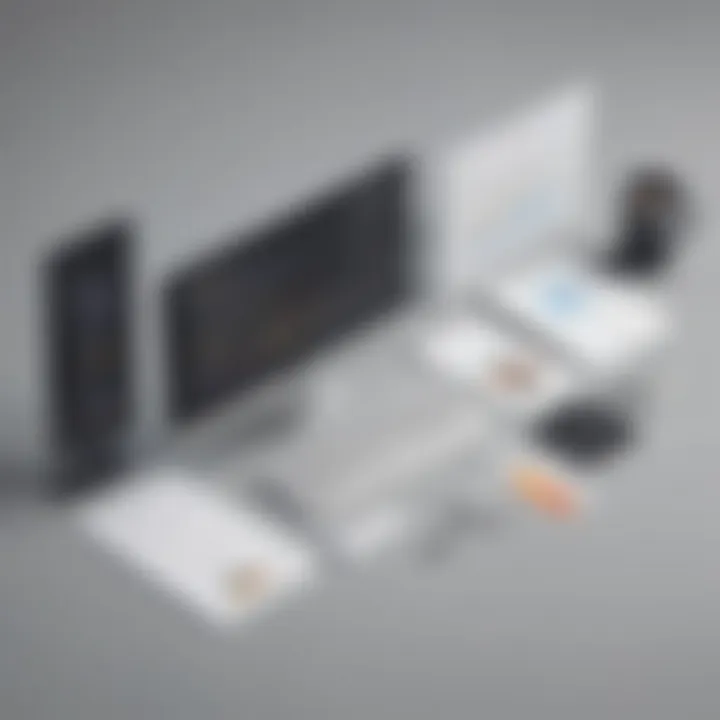
Using Browser DevTools
Browser DevTools offer a comprehensive set of debugging features, enabling developers to inspect elements, monitor network activity, and debug JavaScript code in real-time. By leveraging the power of DevTools, developers can analyze runtime behavior, identify performance bottlenecks, and troubleshoot issues with ease. The interactive nature of DevTools facilitates efficient debugging, empowering developers to enhance the quality and performance of their applications.
- Logging and Console Statements
Logging and Console Statements
Logging and console statements are valuable debugging aids that help developers track the execution flow and output of their code. By strategically placing logging statements in the codebase, developers can capture relevant information, errors, and variables at different stages of execution. Console statements provide immediate feedback during code execution, aiding in the identification of errors and unexpected behaviors. Leveraging logging and console statements enhances the debugging process, enabling developers to pinpoint issues and implement necessary fixes promptly.
- Remote Debugging
Remote Debugging
Remote debugging extends the debugging capabilities to remote environments, allowing developers to inspect and troubleshoot applications running on different devices or browsers. By connecting a development environment to a remote target, developers can analyze and debug code without direct access to the device. Remote debugging facilitates the identification of platform-specific issues, enabling developers to ensure cross-compatibility and optimal performance across various settings. This technique streamlines the debugging process, empowering developers to address issues remotely and enhance the overall quality of their applications.
Building Interactive Web Applications with JavaScript
In the realm of JavaScript programming, the segment regarding Building Interactive Web Applications plays a pivotal role. This portion delves into the practical application of JavaScript in developing dynamic and interactive web interfaces. By comprehensively exploring DOM Manipulation, Event Handling, Creating Single Page Applications, and Integrating Third-Party APIs, developers gain a holistic understanding of architecting engaging web experiences. Building Interactive Web Applications with JavaScript not only enhances user engagement but also facilitates the creation of responsive, feature-rich applications that cater to modern web development standards.
DOM Manipulation and Event Handling
Querying and Modifying DOM Elements:
At the core of web development, DOM manipulation forms the backbone of interactivity in web applications. When discussing Querying and Modifying DOM Elements, it refers to the ability to access and alter elements within the Document Object Model dynamically. This aspect significantly influences the user experience by enabling developers to manipulate content, structure, and styling on-the-fly. The feature of efficient element selection and modification in Querying and Modifying DOM Elements accelerates development processes, allowing for seamless user interaction and real-time updates within web applications.
Binding Event Listeners:
Event Handling with Binding Event Listeners is key to responsive web development. It involves associating specific actions or behaviors with user interactions, such as clicks, keypresses, or mouse movements. By binding event listeners to DOM elements, developers can trigger functions or operations in response to user actions, enhancing the interactivity and dynamism of web interfaces. The unique characteristic of event binding lies in ensuring synchronous and asynchronous handling of user inputs, enabling robust and interactive web application functionalities.
Handling User Interactions:
Another fundamental aspect of Building Interactive Web Applications is effectively managing user interactions. Handling User Interactions revolves around interpreting user actions within the web application and providing appropriate responses or feedback. This mechanism is crucial in creating user-friendly interfaces that respond intuitively to user behaviors, leading to enhanced engagement and usability. The advantage of meticulous user interaction handling lies in fostering seamless and intuitive user experiences, promoting a positive perception of the web application among users.
Creating Single Page Applications (SPAs)
Implementing Routing:
Within the paradigm of web development, the implementation of routing in Single Page Applications plays a critical role. Routing facilitates the navigation and display of different content sections within a SPA without the need to reload the entire page. By implementing routing mechanisms, developers can create smooth and fluid user experiences, akin to traditional multi-page websites. The unique feature of routing enables SPAs to deliver content dynamically, improving performance and responsiveness while maintaining a seamless user journey.
State Management:
Effective State Management is essential in SPA development to maintain application state across different components and interactions. It involves centralizing and managing the state of variables, data, and user input within the application. The key characteristic of state management lies in ensuring data integrity and consistency throughout the SPA, enabling dynamic content updates and seamless user interactions. By leveraging state management, developers can optimize workflow efficiency and enhance the scalability of SPAs, resulting in robust and user-centric applications.
Data Binding:
Data Binding is a crucial mechanism in SPA development that synchronizes the application state with the user interface. By binding data models to UI elements, developers establish a live link that updates the view automatically when the model changes. This feature streamlines data manipulation and visualization within the application, providing a responsive and immersive user experience. The unique advantage of data binding lies in its ability to facilitate rapid development, maintain data consistency, and enhance the overall functionality of SPAs, enriching user interactions and data presentation.
Integrating Third-Party APIs
Authentication with OAuth:
When integrating third-party APIs, Authentication with OAuth stands out as a vital security measure within web applications. OAuth enables secure and authorized access to external services by delegating authentication responsibilities to a trusted third party. This key characteristic ensures robust user authentication mechanisms, safeguarding user data and privacy in API interactions. OAuth's unique feature of token-based authentication enhances security measures, reduces vulnerability to unauthorized access, and promotes seamless integration of external services in web applications.
Working with RESTful APIs:
The collaboration with RESTful APIs empowers web applications to communicate and exchange data with external servers seamlessly. Working with RESTful APIs involves implementing standard methods for data retrieval, manipulation, or deletion via HTTP protocols. The key characteristic of RESTful APIs lies in their stateless and uniform interface design, simplifying data transactions and promoting interoperability. Leveraging RESTful APIs streamlines data exchange processes, accelerates development workflows, and enhances the functionality and connectivity of web applications.
Data Visualization:
Data Visualization is a critical aspect of integrating third-party APIs to represent complex data in a comprehensible format. Visualization techniques such as charts, graphs, and interactive diagrams enhance data interpretation, analysis, and decision-making within web applications. Its key characteristic lies in transforming raw data into visual representations that convey insights and trends effectively to users. Data Visualization's unique feature of enhancing data-driven decision-making promotes engagement, clarity, and usability in web applications, enriching user interactions and driving informed choices.