Explore Advanced JavaScript Coding Techniques for Software Development Mastery
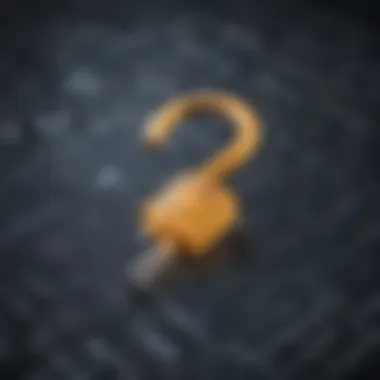
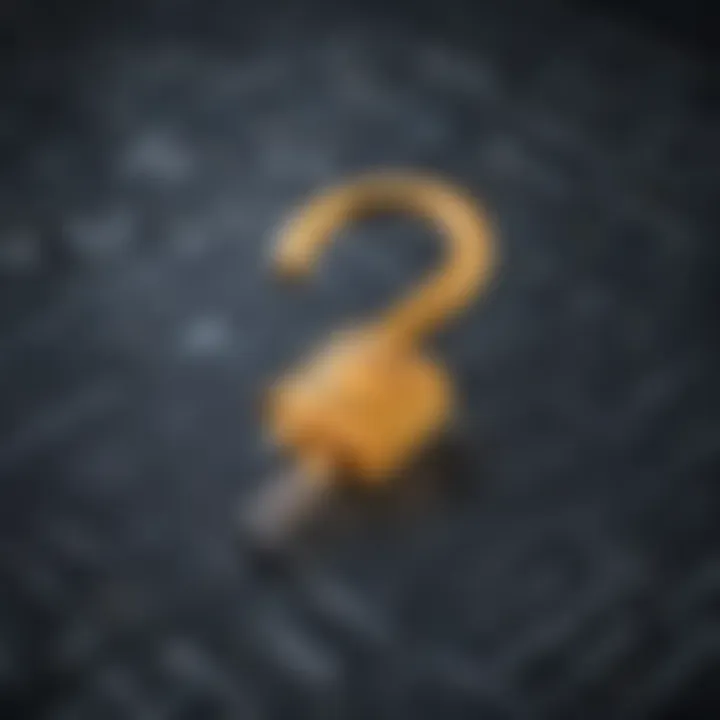
Overview of Advanced JavaScript Coding Techniques
JavaScript, a versatile programming language, plays a pivotal role in modern software development. With its widespread use in web development, JavaScript offers a multitude of functionalities for creating dynamic and interactive web applications. Understanding advanced coding techniques in JavaScript is essential for developers looking to elevate their programming skills and optimize their code efficiency.
Definition and Importance of Advanced JavaScript Coding Techniques
Advanced JavaScript coding techniques refer to the implementation of sophisticated methods and strategies to enhance the performance, scalability, and maintainability of JavaScript code. By delving into advanced concepts such as closures, prototypes, asynchronous programming, and functional programming, developers can write more efficient and robust code for complex applications.
Key Features and Functionalities
Key features of advanced JavaScript coding techniques include optimizing algorithms, leveraging design patterns, implementing error handling strategies, and using cutting-edge libraries and frameworks. These techniques empower developers to overcome challenges, streamline development processes, and create high-quality software solutions.
Use Cases and Benefits
Advanced JavaScript coding techniques find application in a wide range of scenarios, including web development, mobile app development, game development, and desktop application development. By incorporating these techniques into their workflow, developers can improve code maintainability, boost application performance, enhance user experience, and accelerate development cycles.
Best Practices for Mastering Advanced JavaScript Coding Techniques
Mastering advanced JavaScript coding techniques requires adopting industry best practices, maximizing efficiency and productivity, and avoiding common pitfalls that can hinder coding progress and performance.
Industry Best Practices
Industry best practices for mastering advanced JavaScript coding techniques encompass embracing coding standards, following modular and scalable design principles, regularly refactoring code, and conducting code reviews to ensure quality and consistency.
Tips for Maximizing Efficiency and Productivity
Tips for maximizing efficiency and productivity in advanced JavaScript coding include setting clear objectives, prioritizing tasks, optimizing algorithms, identifying and eliminating bottlenecks, leveraging automation tools, and staying updated on the latest trends and technologies.
Common Pitfalls to Avoid
Common pitfalls to avoid in mastering advanced JavaScript coding techniques include neglecting error handling, overcomplicating code logic, ignoring performance optimization, neglecting code documentation, and failing to conduct thorough testing and debugging.
Case Studies of Successful Implementation of Advanced JavaScript Coding Techniques
Real-world case studies provide valuable insights into the successful application of advanced JavaScript coding techniques, lessons learned, outcomes achieved, and expert perspectives on industry developments.
Examples of Successful Implementation
Real-world examples of successful implementation of advanced JavaScript coding techniques showcase how businesses and developers have leveraged these strategies to overcome challenges, achieve project milestones, and deliver innovative software solutions.
Lessons Learned and Outcomes Achieved
Lessons learned from case studies highlight the importance of adopting best practices, overcoming challenges, collaborating effectively with team members, and continuously improving coding skills to drive project success and achieve desired outcomes.
Insights from Industry Experts
Insights from industry experts shed light on emerging trends, best practices, and strategies for mastering advanced JavaScript coding techniques. By learning from experienced professionals, developers can gain valuable knowledge and guidance to enhance their coding proficiency and excel in their careers.
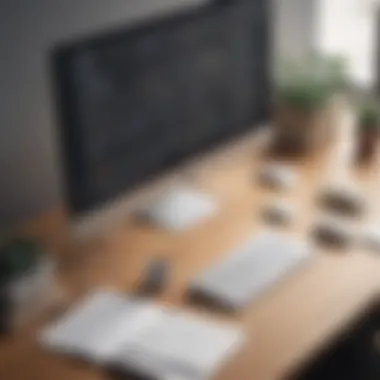
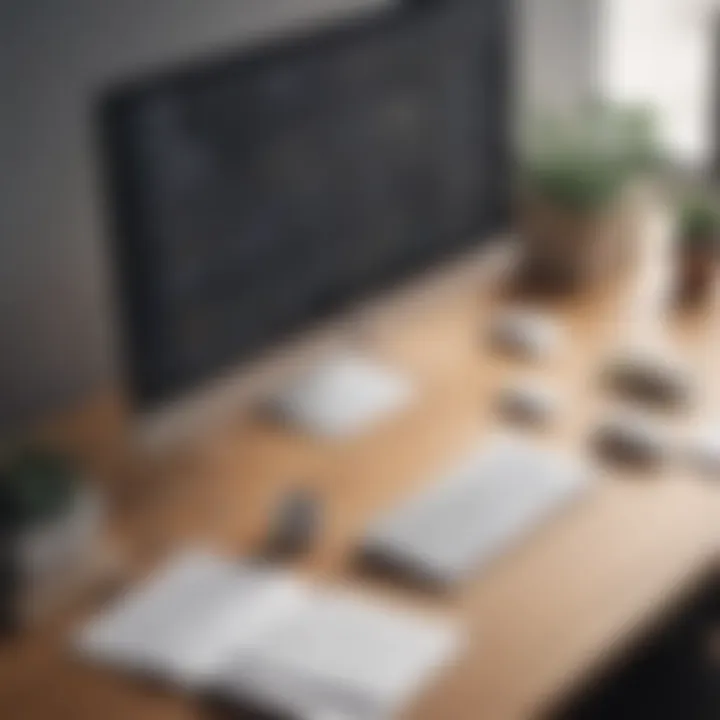
Latest Trends and Updates in Advanced JavaScript Coding Techniques
Staying abreast of the latest trends and updates in advanced JavaScript coding techniques is essential for developers seeking to optimize their coding practices, adapt to industry changes, and stay ahead of the curve.
Upcoming Advancements in the Field
Upcoming advancements in advanced JavaScript coding techniques include enhancements in performance optimization, the integration of machine learning algorithms, the evolution of web components, and the proliferation of cloud-native development approaches.
Current Industry Trends and Forecasts
Current industry trends and forecasts in advanced JavaScript coding techniques indicate a shift towards microservices architecture, the widespread adoption of serverless computing, the rise of progressive web applications (PWAs), and the growing emphasis on security and data privacy.
Innovations and Breakthroughs
Innovations and breakthroughs in advanced JavaScript coding techniques encompass the development of new libraries, frameworks, and tools that streamline development processes, enhance developer productivity, and enable the creation of cutting-edge software applications.
Introduction
This section sets the stage for the in-depth exploration of JavaScript's intricacies that await us. We will elucidate the significance of understanding JavaScript fundamentals and grasp the importance of mastering its coding techniques. From variable manipulation to handling functions and intricate data structures like arrays and objects, JavaScript forms the backbone of modern web applications. By delving into the basics and laying a solid foundation, developers can propel themselves towards crafting efficient and robust code.
Amidst the ever-evolving landscape of web technologies, a firm grip on JavaScript becomes indispensable for software development enthusiasts. The evolution of JavaScript has introduced advanced concepts like closures, prototypes, and asynchronous programming, enriching the developer's toolkit. This section paves the way for an immersive journey through the realms of JavaScript coding artistry, igniting a passion for unraveling its complexities. Buckle up as we embark on a detailed exploration of JavaScript coding techniques, aiming to empower developers with the knowledge and skills necessary to excel in the digital realm.
Understanding the Basics of JavaScript
In the realm of software development, understanding the fundamentals of JavaScript serves as the cornerstone for building robust and efficient applications. Mastering the basics of JavaScript is akin to laying a solid foundation before embarking on more advanced coding techniques. For both aspiring developers and seasoned professionals, grasping the core concepts of variables, data types, functions, scope, arrays, and objects is imperative. By comprehending these fundamental aspects, developers can write cleaner code, enhance application performance, and debug more effectively. Furthermore, a strong grasp of JavaScript basics facilitates seamless transition into exploring more complex programming paradigms and frameworks.
Variables and Data Types
Variables and data types play a pivotal role in JavaScript programming. Understanding how to declare variables, assign values, and manipulate data types is crucial for writing dynamic and flexible code. In JavaScript, variables act as containers to store different types of data, such as numbers, strings, booleans, objects, and arrays. By mastering variables and data types, developers can efficiently manage information within their code, perform calculations, and handle various operations. Moreover, a solid understanding of data types ensures data integrity and prevents errors during execution, contributing to the overall reliability and performance of the software.
Functions and Scope
In JavaScript, functions serve as reusable blocks of code that perform specific tasks when invoked. Understanding functions and their scope is essential for structuring code logically and promoting code reusability. Functions enable developers to encapsulate functionality, abstract complex operations, and improve code readability. Grasping the concept of scope in JavaScript is equally crucial, as it defines the visibility and accessibility of variables within different parts of the program. By mastering functions and scope, developers can create modular, efficient code that fosters maintainability and scalability.
Arrays and Objects
Arrays and objects are fundamental data structures in JavaScript that enable developers to store and manipulate collections of values. Arrays allow for the organization of similar data elements in a sequential order, facilitating easy access and manipulation through index-based operations. Objects, on the other hand, represent complex entities with properties and methods, offering a versatile way to model real-world entities in code. Understanding how to work with arrays and objects empowers developers to build dynamic data structures, implement algorithms effectively, and create interactive user interfaces. Mastery of arrays and objects is essential for leveraging the full capabilities of JavaScript and crafting efficient, maintainable code.
Understanding the Basics of JavaScript
In this section, the foundation of JavaScript is explored, serving as a crucial stepping stone for any developer. By delving into Variables and Data Types, developers can grasp the fundamental building blocks of coding in JavaScript. Understanding how Variables and Data Types work is essential for manipulating information effectively within the code. Functions and Scope are also paramount aspects covered in this section. By mastering Functions and Scope, developers can control the flow of their code and efficiently manage variables' visibility and accessibility. Arrays and Objects are another key focus, providing developers with powerful tools for organizing and storing data efficiently in their applications.
Advanced JavaScript Concepts
The Advanced JavaScript Concepts section delves into sophisticated programming techniques that elevate JavaScript proficiency to new heights. Expounding on the importance of Advanced JavaScript Concepts within the broader context of JavaScript development, this section equips developers with the essential knowledge needed to address complex coding challenges effectively. By exploring topics like Closures and Prototypes, developers can harness the power of encapsulation and inheritance in their code. Understanding Closures enables developers to manage variable scopes and create efficient, modular code structures. Prototypes, on the other hand, facilitate object-oriented programming in JavaScript, enabling the creation of reusable code components that enhance application scalability and maintainability.
Closures and Prototypes
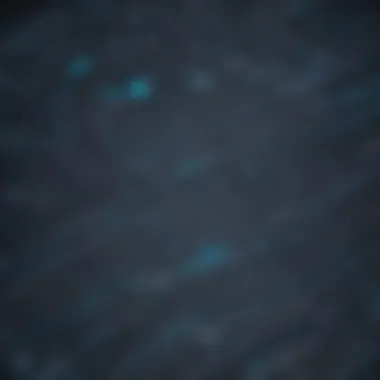
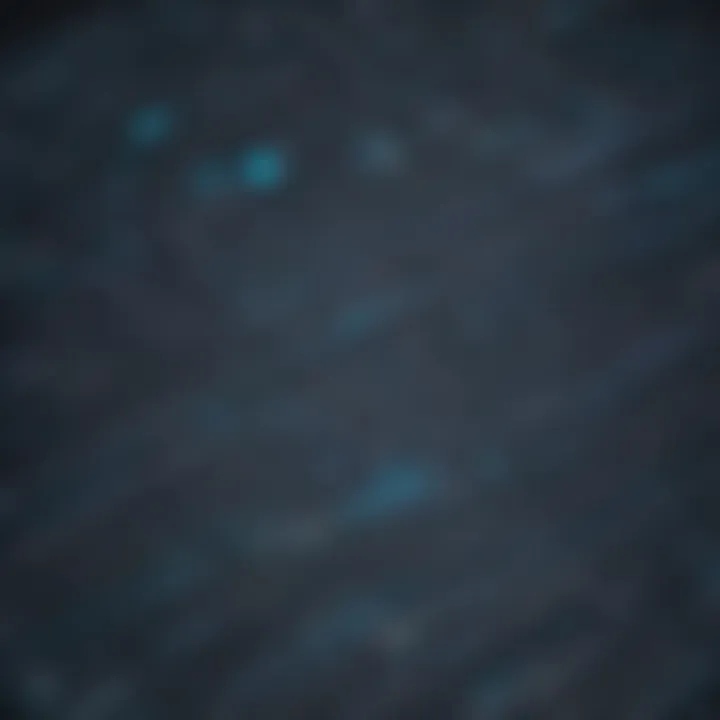
Closures and Prototypes are pivotal concepts in JavaScript programming, offering developers versatile tools to enhance code functionality. Closures allow developers to create private variables and functions, improving code security and encapsulation. By leveraging Prototypes, developers can establish inheritance relationships between objects, promoting code reusability and extensibility. Mastery of Closures and Prototypes empowers developers to craft robust, maintainable codebases that cater to evolving project requirements and facilitate seamless code management.
Asynchronous Programming
Asynchronous Programming revolutionizes JavaScript development by enabling non-blocking, concurrent execution of tasks. This subsection explores the significance of asynchronous programming in building responsive, high-performance applications. By adopting asynchronous methodologies, developers can enhance user experience by eliminating UI freezes and improving application responsiveness. Asynchronous programming allows developers to execute time-consuming operations efficiently without halting program execution, leading to enhanced application scalability and performance.
Error Handling
Error Handling plays a pivotal role in ensuring application robustness and stability. This segment delves into the importance of implementing effective error handling mechanisms to detect, manage, and rectify errors within JavaScript code. By proficiently managing exceptions and errors, developers can fortify their applications against unexpected failures, enhancing reliability and user satisfaction. Error Handling techniques like trycatch aid in identifying and resolving errors, promoting code resilience and facilitating smoother application operation.
Optimizing Code Performance
In the realm of JavaScript programming, optimizing code performance stands out as a critical component for efficient software development. The emphasis on optimizing code performance lies in enhancing the speed and responsiveness of applications, ultimately improving user experience. By delving into the intricacies of optimizing code performance, developers can pinpoint inefficiencies, streamline processes, and boost overall application performance. This section focuses on key elements such as identifying bottlenecks, implementing performance-enhancing techniques, and fine-tuning code to ensure optimal functionality.
Efficient DOM Manipulation
Efficiently manipulating the Document Object Model (DOM) is essential for creating dynamic and interactive web applications. DOM manipulation involves interacting with the structure of a webpage to update content, style elements, and respond to user actions. In JavaScript development, inefficient DOM manipulation can lead to sluggish performance and increased load times. By adopting best practices such as minimizing DOM traversal, batch processing updates, and leveraging modern DOM APIs, developers can significantly improve the speed and responsiveness of their applications.
Minification and Bundling
Minification and bundling are integral processes in optimizing code for production environments. Minification involves removing unnecessary characters and spaces from code to reduce file size, thereby enhancing page load speed. Bundling combines multiple files into a single file, reducing the number of server requests and optimizing resource loading. By incorporating minification and bundling techniques into the development workflow, developers can create leaner, more efficient codebases that result in faster load times and improved performance.
Code Profiling and Optimization Tools
Code profiling and optimization tools play a crucial role in identifying performance bottleneencies Lies inillness oforrirus and optimiziSeIngulaeaet y ilgraeace enema. These tools allow developers to analyze code execution, identify inefficient algorithms, and detect memory leaks or bottlenecks. By leveraging code profiling tools such as Chrome DevTools, Lighthouse, or WebPageTest, developers can gain insights into code performance, pinpoint areas for improvement, and implement optimizations for enhanced functionality. Incorporating these tools into the development process empowers developers to create high-performing applications that deliver exceptional user experiences.
Best Practices for JavaScript Development
In the realm of software development, adhering to best practices is paramount. This section of the article delves into the foundational principles that underpin efficient JavaScript development. By systematically organizing code and implementing modularization, developers can enhance scalability and maintainability. Adopting best practices ensures code robustness, which is critical for the seamless functioning of complex applications.
Modularization and Code Organization
Modularization plays a pivotal role in structuring code with a focus on reusability and clarity. By breaking down a codebase into cohesive modules, developers can isolate functionalities, thereby simplifying maintenance and enhancing collaboration. Code organization not only streamlines the development process but also fosters code readability and understandability. This section elucidates the significance of modularization in JavaScript projects and offers insights into best practices for effective code organization.
Version Control and Collaboration
Version control systems like Git have revolutionized collaborative software development. This subsection delves into the importance of version control in tracking changes, managing contributions, and resolving conflicts in JavaScript projects. Emphasizing the significance of a systematic version control workflow, this segment explores strategies for seamless collaboration among team members. From branching strategies to merging best practices, mastering version control is essential for ensuring project integrity and code synchronization.
Testing and Debugging Strategies
Testing and debugging are indispensable components of the software development lifecycle. This segment expounds on the various testing methodologies and debugging techniques tailored for JavaScript applications. From unit testing to integration testing, developers rely on a suite of testing strategies to validate code functionality and identify bugs. Effective debugging practices, including console debugging and breakpoint inspection, empower developers to troubleshoot and rectify errors efficiently. By following meticulous testing and debugging protocols, developers can deliver high-quality, bug-free JavaScript applications.
Frameworks and Libraries for JavaScript Development
In the realm of software development, utilizing frameworks and libraries plays a crucial role in enhancing efficiency and streamlining the coding process. When it comes to JavaScript development, the selection of suitable frameworks and libraries can significantly impact the overall productivity and success of projects. Frameworks like React, Angular, and Vue.js offer pre-built components, architecture patterns, and a robust ecosystem that developers can leverage to expedite development processes and design scalable applications.
React
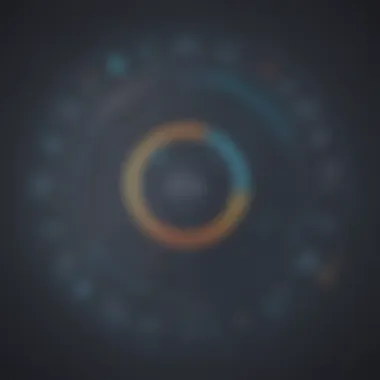
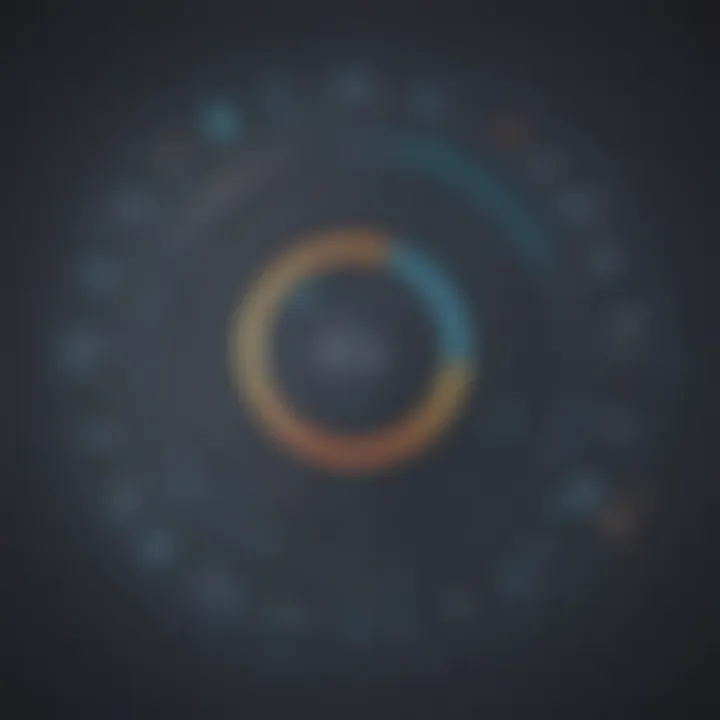
React, developed by Facebook, stands out as a highly popular JavaScript library used for building user interfaces. Its component-based architecture allows for the creation of interactive UIs with ease. React’s virtual DOM feature enables efficient rendering of components, leading to better performance. Developers benefit from the reusability of components, simplifying code maintenance and promoting code consistency. Moreover, React’s one-way data flow ensures predictable behavior and enables the creation of sophisticated web applications with optimal user experience.
Angular
Angular, a comprehensive framework maintained by Google, provides a full-fledged solution for front-end development. With its Model-View-Controller (MVC) architecture, Angular facilitates the structuring of applications and separation of concerns. TypeScript support in Angular enhances code quality and enables developers to catch errors at compile time. Angular offers features like two-way data binding, dependency injection, and powerful directives that expedite development and contribute to building dynamic single-page applications (SPAs) efficiently.
Vue.js
Vue.js, known for its progressive and approachable nature, strikes a balance between the robust features of React and Angular. Its simplicity and ease of integration with existing projects make it a favorite among developers. Vue’s reactive data binding ensures seamless data manipulation and updates without extra configuration. With Vue’s lightweight size, swift rendering capabilities, and support for server-side rendering, developers can create interactive interfaces and high-performing applications effortlessly. Vue.js fosters rapid prototyping and empowers developers to build scalable applications with minimal overhead.
Exploring JavaScript Ecosystem
In the realm of JavaScript development, exploring the JavaScript ecosystem is a vital undertaking that every proficient developer must engage with. The ecosystem encapsulates a vast array of libraries, tools, and resources that are pivotal in enhancing the efficiency and productivity of JavaScript coding projects. From package managers to build tools and beyond, navigating this ecosystem equips developers with the necessary arsenal to streamline their development process, optimize performance, and stay abreast of the latest advancements in the JavaScript landscape.
Package Managers
Package managers play a pivotal role in the JavaScript ecosystem by facilitating the seamless integration of third-party packages and dependencies into project workflows. These tools, such as npm and Yarn, automate the process of package installation, version management, and resolution of package conflicts. By efficiently managing dependencies, package managers not only enhance code modularity and reusability but also ensure that projects are built on solid foundations.
Build Tools
Build tools serve as the backbone of modern JavaScript development, empowering developers to automate repetitive tasks, optimize code, and streamline the build process. Tools like webpack, Parcel, and Rollup enable efficient bundling of assets, transpilation of code, and code splitting, ultimately improving the performance and scalability of JavaScript applications. By adopting the right build tools, developers can achieve faster build times, reduced bundle sizes, and enhanced code maintainability.
ES6 and Beyond
The evolution of JavaScript beyond ES6 introduces a plethora of new features, syntax enhancements, and functional programming paradigms that elevate the language to new heights. ES6, also known as ECMAScript 2015, brought significant advancements such as arrow functions, template literals, and destructuring, enhancing the readability and expressiveness of JavaScript code. Subsequent ES versions continue to push the boundaries of the language, introducing features like asyncawait, optional chaining, and nullish coalescing, which streamline asynchronous programming and error handling processes.
Security Considerations in JavaScript Development
In the realm of JavaScript coding, understanding security considerations holds paramount importance for developers. With the ever-evolving cyber threat landscape, developers must be vigilant in safeguarding their applications from vulnerabilities. Security considerations in JavaScript development encompass a range of crucial aspects that directly impact the safety and integrity of software systems. Whether it is protecting against malicious attacks or ensuring data privacy compliance, incorporating robust security measures is non-negotiable.
Cross-Site Scripting (XSS) Prevention
Cross-Site Scripting (XSS) is a prevalent security risk that can compromise the data integrity of web applications. By injecting malicious scripts into trusted websites, attackers can manipulate user interactions, steal sensitive information, and disrupt system functionality. Preventing XSS attacks requires implementing stringent input validation mechanisms, encoding user-generated content, and utilizing secure coding practices. Developers must prioritize sanitizing user inputs and validating data to thwart XSS vulnerabilities effectively.
Content Security Policy (CSP) Implementation
Content Security Policy (CSP) serves as a vital defense mechanism against various web security threats, including XSS attacks and data injection vulnerabilities. By defining and enforcing a robust CSP, developers can control the resources that a browser is allowed to load for a web page, mitigating the risks associated with arbitrary script execution. CSP implementation involves configuring policies to restrict the origins of content, preventing unauthorized scripts and resources from compromising the application's security. Embracing CSP best practices strengthens the overall resilience of web applications against malicious exploits.
Data Sanitization Techniques
Data sanitization plays a pivotal role in fortifying the security posture of JavaScript applications by cleansing input data and removing potentially harmful content. In a data-driven environment, ensuring the integrity and validity of user inputs is essential to mitigate the risks of injection attacks and data breaches. By employing robust data sanitization techniques such as input validation, output encoding, and parameterized queries, developers can sanitize data at various entry points to prevent vulnerabilities. Implementing data sanitization practices rigorously is integral to upholding the confidentiality and integrity of sensitive information within JavaScript applications.
Conclusion
In the dynamic realm of JavaScript coding techniques, the Conclusion section serves as the epilogue that ties together the intricate tapestry of skills and insights presented in this exhaustive guide. It encapsulates the significance of reflecting on the learnings acquired throughout the article. Understanding the relevance of Conclusion is paramount as it not only summarizes the key takeaways but also provides a pathway for developers to internalize and implement the knowledge gained.
Exploring the denouement in a holistic context unveils the pivotal role it plays in fortifying one's grasp of not just the technical aspects but also the strategic and logical reasoning intertwined with JavaScript development. By reiterating the core concepts elucidated in the preceding sections, the Conclusion segment acts as a beacon illuminating the way forward for developers to apply these principles in their coding endeavors.
Delving deeper, the Conclusion segment extends beyond mere summarization, offering a contemplative space for readers to ruminate on the broader implications of harnessing advanced JavaScript coding techniques. Emphasizing the immersive nature of mastering JavaScript, it underscores the iterative nature of learning and the perpetual evolution embedded within the coding landscape.
Furthermore, the Conclusion section bridges the chasm between theoretical knowledge and practical application, instilling a sense of purpose and direction in developers' quest for honing their coding acumen. By elucidating the relevance of synthesizing information, this conclusive segment empowers readers to transcend the confines of traditional coding paradigms and venture into uncharted territories of innovation and efficiency.
In essence, the Conclusion section serves as a beacon of guidance, steering developers towards a comprehensive understanding of not just JavaScript coding techniques but also the underlying principles that underpin software development. As readers navigate through this conclusive discourse, they are poised to emerge not just as adept coders but as visionaries sculpting the future trajectory of JavaScript innovation and excellence.