Mastering Java Code: A Comprehensive Guide to Enhance Your Coding Skills
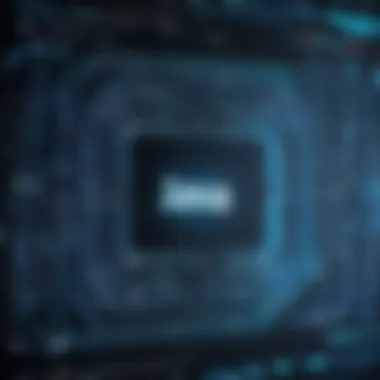
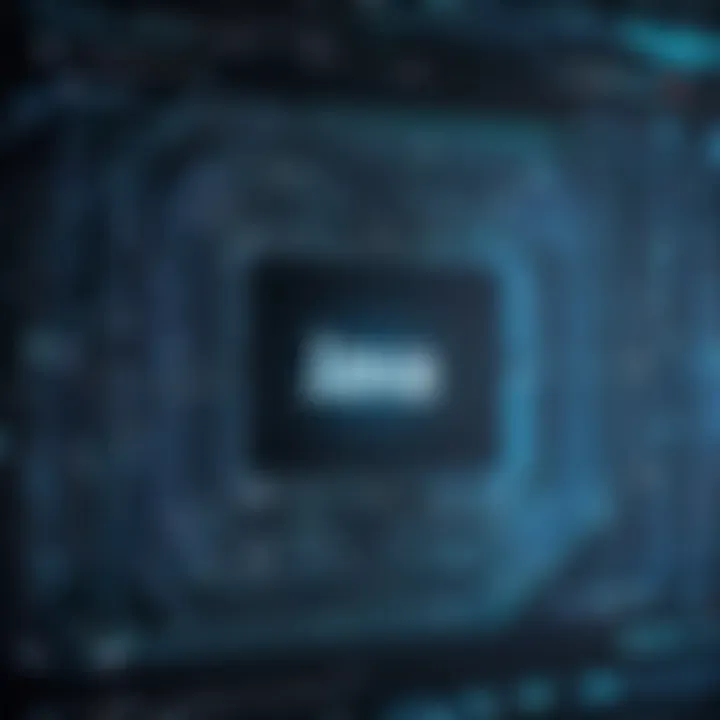
Overview of Java Programming Language
Java, a versatile and robust programming language, serves as the backbone for countless applications across diverse industries. Its clean syntax and object-oriented approach make it a popular choice for developers aiming to build scalable and efficient software solutions. Understanding the key principles of Java is essential for any aspiring programmer looking to elevate their coding skills and develop practical applications.
Best Practices for Java Code Mastery
When delving into the realm of Java programming, adhering to industry best practices is paramount for ensuring code quality and maintainability. By following coding standards, documenting code effectively, and writing modular and reusable code snippets, developers can streamline the development process and minimize errors. Embracing version control systems like Git, employing test-driven development practices, and conducting code reviews are additional measures that can contribute to enhancing productivity and code efficiency.
Case Studies in Java Development
Examining real-world case studies of successful Java implementations provides valuable insights into effective coding practices and project outcomes. From developing efficient algorithms for data processing to building robust backend systems for large-scale applications, Java remains a cornerstone in the software development landscape. Learning from the experiences of industry experts and analyzing the challenges they overcame can offer inspiration and guidance for developers embarking on complex Java projects.
Latest Trends and Advances in Java Programming
As the technology landscape evolves, staying abreast of the latest trends and innovations in Java programming is essential for remaining competitive in the industry. From advancements in Java frameworks to emerging paradigms like reactive programming, developers need to adapt to changing methodologies and embrace new tools to stay ahead of the curve. Keeping an eye on industry forecasts, attending tech conferences, and actively engaging with the developer community are ways to stay informed about the latest developments in Java programming.
How-To Guides and Tutorials for Java Coders
For beginners venturing into Java programming or seasoned developers seeking to expand their skill set, comprehensive how-to guides and tutorials offer practical insights and hands-on learning opportunities. Step-by-step tutorials on setting up development environments, creating Java applications, and troubleshooting common issues can aid in mastering Java coding concepts. Additionally, exploring advanced topics such as multithreading, Java collections, and design patterns can deepen one's understanding of Java programming principles.
Introduction to Java Code
Java, a stalwart in the realm of programming languages, serves as the bedrock for countless applications due to its versatility and robust nature. Understanding the Basics of Java lays a solid foundation for any aspiring developer embarking on a journey through the intricacies of code. Variables and Data Types form the building blocks of Java, offering a structured approach to handling information. Operators and Expressions bring life to code, enabling complex computations with ease. Control Flow Statements dictate the logical flow of a program, enhancing its efficiency and readability.
Understanding the Basics of Java
Variables and Data Types
Variables and Data Types are pivotal in Java as they dictate how information is stored and manipulated. By defining variables meticulously, developers can ensure data integrity and efficient memory usage. Choosing the appropriate data type is crucial for optimizing code performance and avoiding unnecessary overhead. While the versatility of variables allows for dynamic data handling, improper usage may lead to inconsistencies and errors.
Operators and Expressions
Operators and Expressions in Java facilitate mathematical and logical operations within a program. From arithmetic operators to bitwise operators, Java offers a comprehensive set of tools for data manipulation. Expressions, composed of operands and operators, simplify complex computations by breaking them down into manageable entities. Understanding operator precedence and associativity is paramount to achieving desired results efficiently.
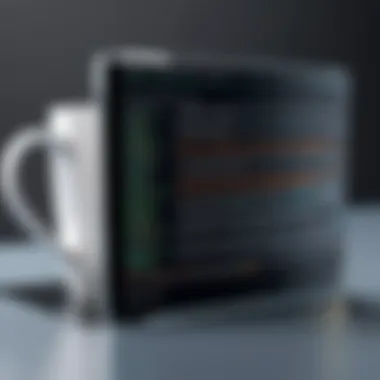
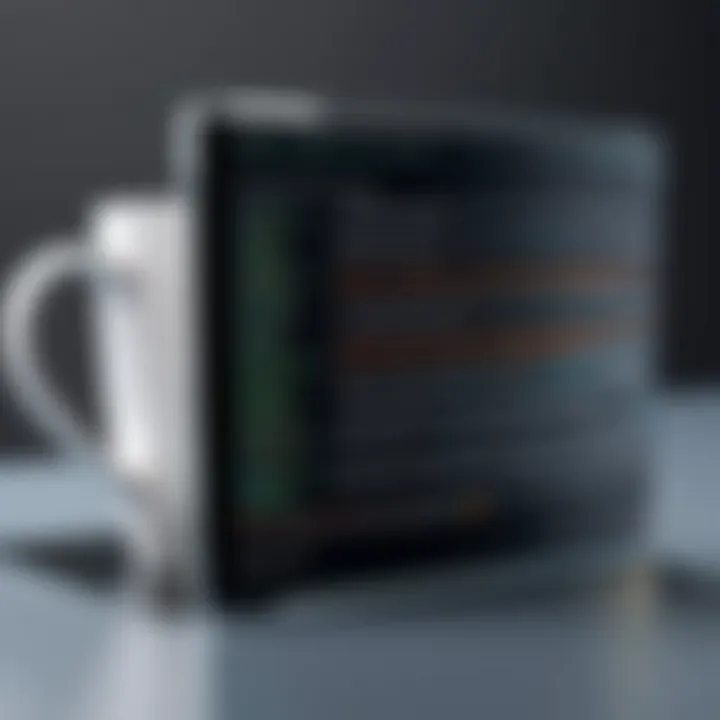
Control Flow Statements
Control Flow Statements govern the execution sequence of a program, enabling developers to implement conditions and loops. By using conditional statements like 'if' and 'switch,' programmers can control the flow based on specific criteria. Iterative statements such as 'for' and 'while' loop through code repetitively, streamlining processes and enhancing code reusability. Mastering Control Flow Statements is essential for creating well-structured and logical programs.
Setting Up Java Development Environment
Installing JDK
Installing the Java Development Kit (JDK) is the initial step towards setting up a Java environment. The JDK provides essential tools like the Java compiler and runtime environment, necessary for developing and executing Java applications. Configuring the JDK involves specifying paths and environment variables to ensure seamless integration into the development ecosystem. Understanding JDK installation is fundamental to kickstarting any Java development project.
Configuring IDEs
Integrated Development Environments (IDEs) offer a comprehensive platform for Java development, combining tools for coding, compilation, and debugging. Configuring IDEs involves customizing settings, installing plugins, and choosing coding styles according to individual preferences. IDEs enhance developer productivity by providing features like auto-completion, syntax highlighting, and debugging utilities. Selecting the right IDE and optimizing its configuration is crucial for a streamlined development experience.
Writing Your First Java Program
Creating Classes and Methods
Creating Classes and Methods forms the cornerstone of Java programming, allowing developers to structure code logically. Classes encapsulate data and behavior, promoting code reusability and maintainability. Methods contain executable code segments, enabling modular design and efficient problem-solving. Developing well-defined classes and methods is essential for constructing robust and scalable Java applications.
Compiling and Running Java Code
Compiling and Running Java Code bridges the gap between source code and executable programs. The Java compiler translates human-readable code into bytecode, understandable by the Java Virtual Machine. Running compiled code executes the program, producing desired outputs based on input and logic. Understanding the compilation process and runtime behavior is crucial for identifying errors and optimizing code performance.
Intermediate Java Concepts
Exploring the realm of Intermediate Java Concepts in this insightful piece is crucial for any individual delving into the art of Java programming. These concepts serve as the bridge between basic syntax and advanced techniques, providing a foundational understanding of core Java principles. Emphasizing Intermediate Java Concepts is paramount to comprehending the intricacies of the language, allowing programmers to elevate their skills and develop robust applications with efficiency and precision.
Working with Arrays and Collections
Array Operations:
Delving into the realm of Array Operations sheds light on the manipulation and handling of arrays, a fundamental data structure in Java programming. The significance of Array Operations lies in its ability to efficiently store and manage a collection of elements, facilitating quick access and retrieval within the codebase. Its deterministic nature and constant-time complexity make it a preferred choice for scenarios requiring sequential data storage and retrieval. However, the fixed size of arrays can pose a limitation, challenging developers in scenarios requiring dynamic allocation and resizing.
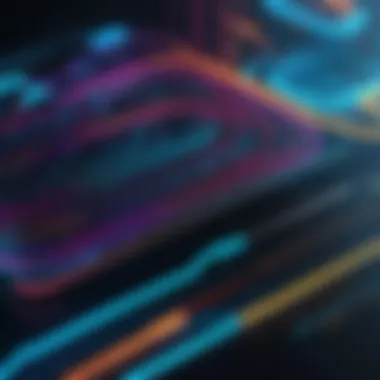
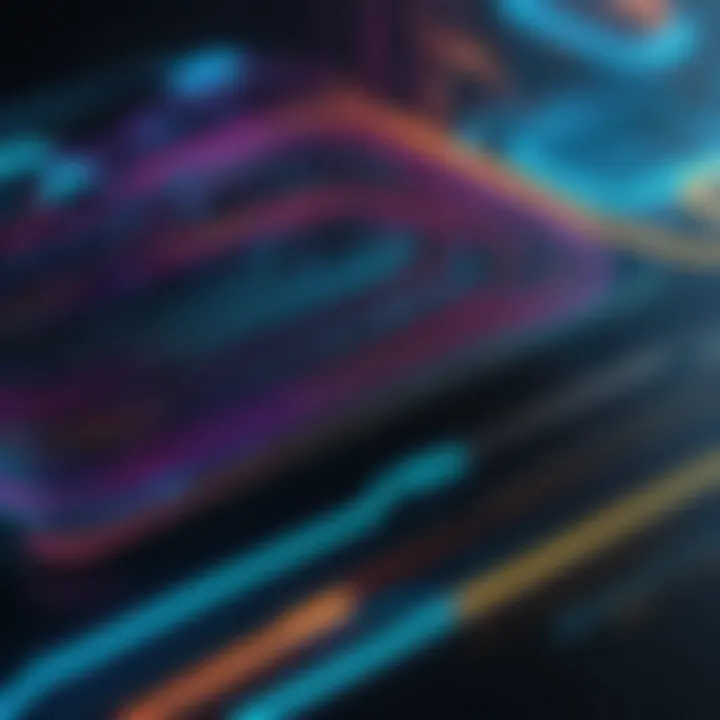
List and Map Interfaces:
Unpacking the essence of List and Map Interfaces unveils the power of versatile data structures in Java programming. List Interfaces offer a dynamic collection that can expand or shrink based on requirements, providing flexibility in managing ordered elements. Conversely, Map Interfaces facilitate key-value pair storage, enabling efficient data organization and retrieval through unique identifiers. The key characteristic of List and Map Interfaces lies in their adaptability to diverse application scenarios, supporting a wide range of data manipulation operations effectively. Despite their efficiency, improper utilization of these interfaces can lead to increased memory consumption and potential performance bottlenecks.
Stream API:
Navigating through the complexities of the Stream API illuminates the realm of functional programming in Java, enabling streamlined data processing operations on collections. The core characteristic of the Stream API lies in its ability to leverage functional interfaces and lambda expressions for concise and expressive data transformations. Its efficiency in handling large datasets and facilitating parallel processing makes it a valuable asset in modern Java development. However, the learning curve associated with mastering functional programming paradigms might pose a challenge for developers unaccustomed to this paradigm shift. Careful consideration and strategic implementation are essential to harnessing the full potential of the Stream API in enhancing code readability and efficiency.
Exception Handling and Debugging
Try-Catch Blocks:
Immersion into the realm of Try-Catch Blocks unveils a critical aspect of Java programming focused on error handling and recovery. Try-Catch Blocks play a pivotal role in intercepting and managing exceptions, ensuring the graceful handling of unforeseen runtime errors. The key characteristic of Try-Catch Blocks lies in their structured approach to exception handling, allowing developers to segregate error-prone code segments and implement tailored error recovery strategies. By encapsulating potential exceptions within try blocks and defining specific catch blocks for error resolution, developers can fortify their codebase against runtime failures. Despite their efficiency, excessive nesting of Try-Catch Blocks can lead to code complexity and reduced readability, emphasizing the importance of concise and strategic exception handling practices.
Throw and Throws Keywords:
Unraveling the significance of the Throw and Throws Keywords sheds light on the mechanisms for propagating and declaring exceptions in Java programming. The Throw keyword empowers developers to explicitly throw custom exceptions, signaling exceptional scenarios within the code execution flow. On the other hand, the Throws keyword facilitates the declaration of potential exceptions that a method may propagate to its caller, enabling transparent exception handling across method invocations. The unique feature of these keywords lies in their ability to delineate and manage exceptional circumstances effectively, enhancing code robustness and fault tolerance. However, improper or excessive usage of these keywords can lead to code verbosity and convoluted exception hierarchies, necessitating a balanced approach in exception declaration and propagation.
Debugging Techniques:
Embarking on the realm of Debugging Techniques unravels a crucial aspect of software development aimed at identifying and rectifying code anomalies. Debugging Techniques encompass a spectrum of methodologies and tools designed to pinpoint and resolve coding errors, ensuring the reliability and functionality of Java applications. The paramount characteristic of Debugging Techniques lies in their capacity to facilitate error diagnosis through step-by-step code execution, variable inspection, and runtime analysis. By leveraging debugging tools integrated within Java IDEs, developers can streamline the debugging process and expedite the resolution of code discrepancies. However, over-reliance on debugging tools without a comprehensive understanding of code logic can impede the debugging process and prolong issue resolution, underscoring the importance of proficient debugging skills and strategic debugging approaches.
Object-Oriented Programming in Java
Inheritance and Polymorphism:
Delving into the realm of Inheritance and Polymorphism elucidates the core tenets of object-oriented programming in Java, fostering code reusability and extensibility. Inheritance empowers classes to inherit attributes and methods from parent classes, establishing hierarchical relationships and promoting code modularization. Polymorphism enables entities to exhibit multiple forms based on the context of invocation, enhancing code flexibility and adaptability. The key characteristic of Inheritance and Polymorphism lies in their role in promoting code scalability and facilitating the implementation of complex behaviors through a structured inheritance hierarchy. However, excessive class hierarchy levels and deep inheritance chains can introduce code maintenance challenges and hinder code readability, necessitating a judicious approach in leveraging these object-oriented concepts.
Abstraction and Encapsulation:
Delving into the essence of Abstraction and Encapsulation unveils fundamental principles shaping Java code design and organization. Abstraction focuses on extracting essential characteristics and behaviors of entities, facilitating code simplification and modularity. Encapsulation encapsulates data and behavior within classes, promoting data hiding and information security. The key characteristic of Abstraction and Encapsulation lies in their ability to enhance code comprehensibility, maintainability, and security by abstracting complex implementations and encapsulating data access. Nonetheless, excessive abstraction levels and convoluted class structures can introduce confusion and hinder code readability, underscoring the importance of maintaining a balance between abstraction and practicality in Java programming.
Interfaces and Abstract Classes:
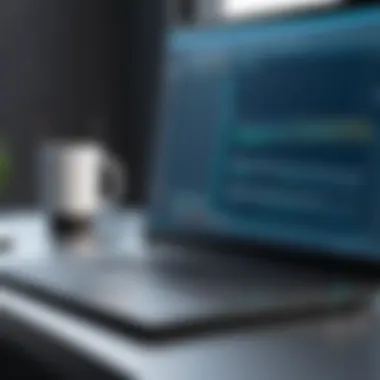
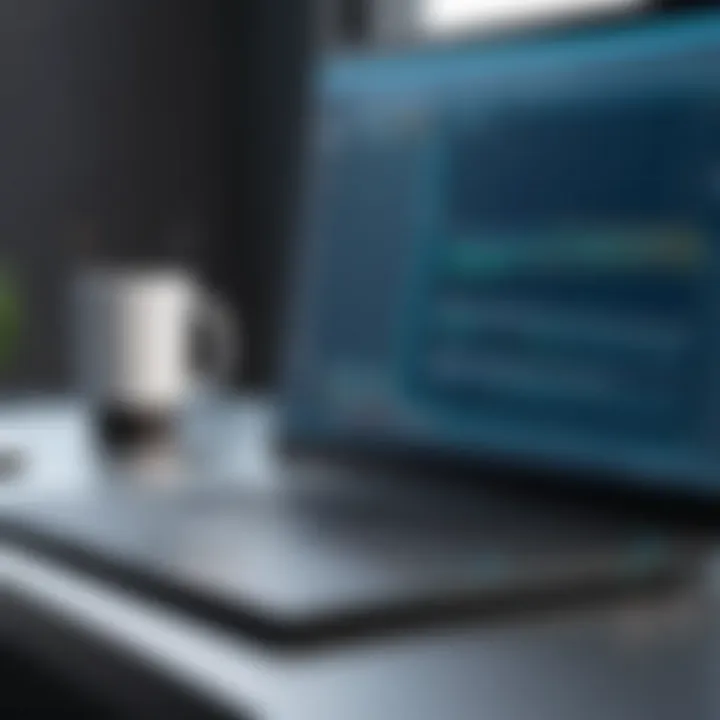
Navigating through the realm of Interfaces and Abstract Classes unveils a sophisticated paradigm in Java programming directed at defining contracts and common behaviors. Interfaces establish blueprints for classes to implement specific methods, promoting code consistency and standardization. Abstract Classes offer partial implementations to concrete classes, enabling shared functionalities and defining class hierarchies. The unique feature of Interfaces and Abstract Classes lies in their capacity to foster code extensibility and polymorphic behavior, facilitating code reuse and flexibility. However, improper interface or abstract class design can lead to code bloat and violated contract constraints, emphasizing the need for meticulous interface and abstract class formulation to ensure code coherence and adherence to programming best practices.
Advanced Java Techniques
The Advanced Java Techniques segment in this expansive guide plays a pivotal role in enhancing the understanding of intricate Java programming concepts. It encapsulates a myriad of advanced topics that are essential for honing coding skills and delving deeper into Java's capabilities. By focusing on Advanced Java Techniques, developers can elevate their coding expertise to a whole new level, embracing sophisticated programming paradigms and boosting overall efficiency.
Multithreading and Concurrency
Thread Class
Within the domain of Multithreading and Concurrency, the Thread Class holds significant importance in facilitating parallel execution within Java programs. This class enables developers to create and manage multiple threads, allowing for simultaneous task performance and enhanced program efficiency. The Thread Class's key characteristic lies in its ability to execute independent paths of code concurrently, making it a popular choice for optimizing performance in multithreaded applications. Despite its advantages in enabling efficient utilization of system resources, the Thread Class also comes with inherent complexities, such as potential thread synchronization issues that may lead to race conditions.
Synchronization
Synchronization, another crucial aspect of Multithreading and Concurrency, ensures thread safety and data consistency in Java applications. By synchronizing critical sections of code, developers can prevent conflicts between threads and maintain the integrity of shared resources. The primary feature of Synchronization is its capability to establish mutual exclusion, preventing concurrent access to critical sections of code. This feature is highly beneficial for maintaining data integrity and avoiding potential concurrency-related errors. However, excessive or improper use of synchronization mechanisms can lead to performance bottlenecks and hinder overall application speed.
Executor Framework
The Executor Framework serves as a cornerstone in Java's concurrency model, providing a higher-level abstraction for managing thread execution. By decoupling task submission from execution, this framework enhances the scalability and robustness of concurrent applications. One of its key characteristics is the ability to utilize thread pools, allowing for efficient reuse of threads and improved task execution management. The Executor Framework is a popular choice in this guide due to its versatility in handling asynchronous tasks, simplifying concurrent programming complexities, and enhancing application performance. However, developers must be mindful of potential thread starvation issues and resource constraints when utilizing this framework.
Java Database Connectivity (JDBC)
Mastering Java Code
Mastering Java Code is a pivotal section in this comprehensive guide, aiming to delve into sophisticated Java programming concepts crucial for advancing one's coding expertise. Understanding and implementing design patterns and architectural principles are fundamental in achieving mastery in Java coding. By exploring topics such as the Singleton Pattern, Factory Pattern, and MVC Architecture, developers can enhance their coding efficiency and maintainable codebase.
Design Patterns and Architectural Principles
Singleton Pattern
The Singleton Pattern plays a vital role in software design, ensuring that a class has only one instance and providing a global point of access. This pattern contributes significantly to this article by promoting efficient memory usage and simplifying resource management. The key characteristic of the Singleton Pattern lies in its ability to restrict instantiation to a single object, making it a popular choice for scenarios where a single point of control or coordination is required. However, it's essential to consider that while the Singleton Pattern offers benefits in terms of centralized instance access, it may lead to tight coupling and potential thread-safety concerns.
Factory Pattern
The Factory Pattern is a creational design pattern that emphasizes the centralized creation of objects without exposing the instantiation logic. In the context of this article, the Factory Pattern offers a scalable and flexible solution for creating objects based on specified parameters. Its key characteristic lies in decoupling object creation from the client, promoting code reusability and ease of maintenance. The unique feature of the Factory Pattern is its ability to encapsulate the object creation process, offering advantages such as enhanced code organization and simplified dependency management. However, it's important to note that the Factory Pattern may introduce additional complexity when managing a large number of product types.
Architecture
The Model-View-Controller (MVC) Architecture is a software design pattern that divides an application into three interconnected components - the model, view, and controller. MVC Architecture contributes significantly to this article by promoting the separation of concerns, enhancing code maintainability, and facilitating parallel development. The key characteristic of MVC Architecture is its clear differentiation of responsibilities among the model, view, and controller components, enabling efficient code organization and reusability. The unique feature of MVC Architecture lies in its support for multiple views based on the same data, offering advantages such as improved code flexibility and modular design. However, it's essential to consider the potential complexity introduced by the interconnection of multiple components in large-scale applications.