Mastering Error Handling for Efficient Software Development
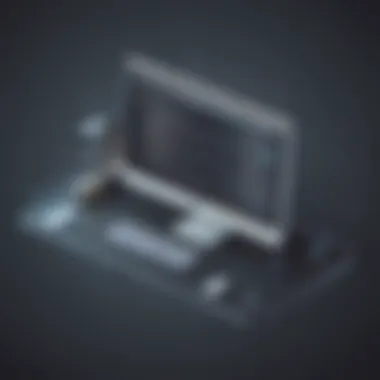
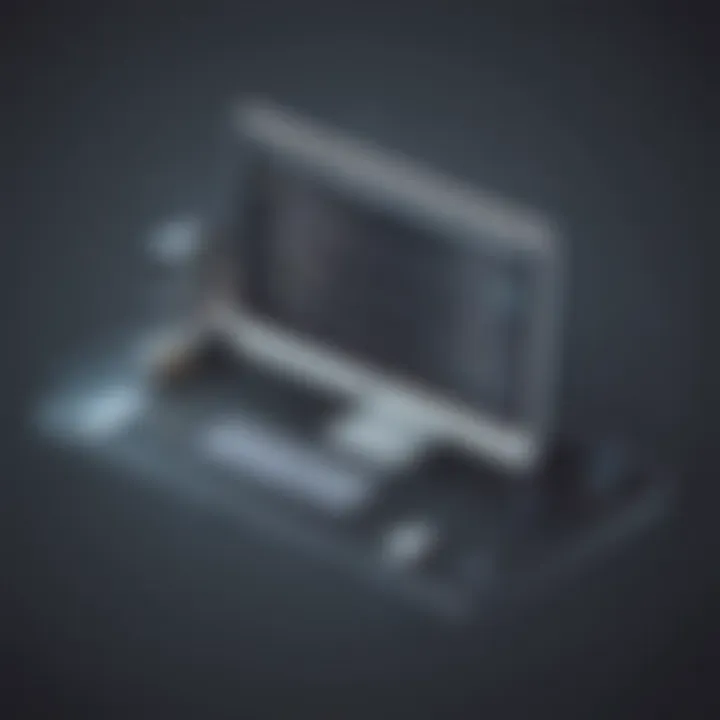
Overview of Error Handling in Software Development
Software development encompasses a complex landscape where errors are inevitable. The intricacies of error handling are fundamental to creating resilient and efficient software applications. Robust error management techniques are crucial for mitigating risks and ensuring the seamless operation of programs. Understanding the nuances of error handling is paramount for developers striving to craft high-quality software solutions.
Best Practices for Error Handling
Implementing industry best practices in error handling is essential for maintaining the integrity of software systems. By adhering to proven strategies and techniques, developers can enhance the reliability and maintainability of their codebase. Maximizing efficiency and productivity in error management involves establishing clear protocols, conducting thorough testing, and fostering a culture of continuous improvement. Avoiding common pitfalls, such as ignoring error logs or insufficient error alert systems, is key to achieving robust error handling.
Case Studies in Error Handling
Real-world examples of successful error handling implementations provide invaluable insights into best practices and lessons learned. By examining case studies of error resolution in diverse scenarios, developers can glean practical knowledge on addressing complex issues efficiently. Drawing from the experiences of industry experts who have navigated challenging error scenarios offers a unique perspective on effective problem-solving strategies.
Latest Trends and Updates in Error Handling
Staying abreast of the latest trends and advancements in error handling is crucial for developers seeking to optimize their software development process. Emerging technology solutions, such as AI-driven error detection tools and automated error resolution mechanisms, are revolutionizing the field of error handling. By leveraging cutting-edge innovations and keeping pace with industry trends, developers can enhance the efficacy of their error management practices.
How-To Guides for Effective Error Handling
Comprehensive how-to guides and tutorials empower developers to implement error handling techniques effectively. From beginner-friendly step-by-step instructions to advanced troubleshooting strategies, these resources offer practical insights for streamlining error detection and resolution processes. Practical tips and tricks for optimizing error logging, debugging, and error recovery mechanisms enable developers to navigate complexities in software development with confidence.
Introduction
In the realm of software development, mastering error handling stands as a pivotal juncture between functional code and catastrophic breakdowns. Expertise in error management transcends mere troubleshooting skills, delving into the core foundation of resilient and efficient software design. As developers navigate the complex landscape of coding, understanding the nuances of error handling becomes paramount to ensuring the reliability and robustness of their creations.
Understanding Error Handling
An Overview of Error Handling
An essential facet of software development lies in comprehending the meticulous art of error handling. The intrinsic nature of identifying and addressing errors lays the groundwork for stable and secure applications. Thorough knowledge of error handling mechanisms enables developers to preemptively detect and resolve issues, minimizing the occurrence of critical failures. Implementing effective error handling strategies fosters a culture of software integrity, bolstering user confidence and system dependability.
The Significance of Effective Error Management
Effective error management serves as the linchpin in the development process, ensuring that applications maintain operational resilience and user satisfaction. The pivot towards a proactive approach to error handling underscores the necessity of preemptive measures over reactive remedies. By prioritizing robust error management, developers can fortify their code against potential pitfalls, ultimately enhancing performance and stability.
Common Types of Errors
Syntax Errors
Syntax errors manifest as fundamental discrepancies in the code structure, impeding proper interpretation by the compiler or interpreter. These errors serve as foundational hurdles that, if left unaddressed, can lead to cascading malfunctions within the software. Understanding and rectifying syntax errors are fundamental to fostering code accuracy and coherence, encapsulating the first line of defense in error prevention.
Runtime Errors
Runtime errors occur during the execution phase of a program, signaling deviations from the intended behavior. Mitigating runtime errors demands a comprehensive grasp of the software's flow and logical pathways, enabling developers to pinpoint and resolve anomalies expeditiously. Addressing runtime errors is pivotal in optimizing software performance and ensuring seamless user experiences.
Logical Errors
Logical errors pose intricate puzzles within the software codebase, often concealed beneath layers of complex logic and conditional statements. Detecting and remedying logical errors necessitate meticulous scrutiny and systematic testing procedures, unraveling the intricacies of program flow and decision-making. The resolution of logical errors amplifies the software's reliability and functional accuracy, elevating the overall quality of the application.
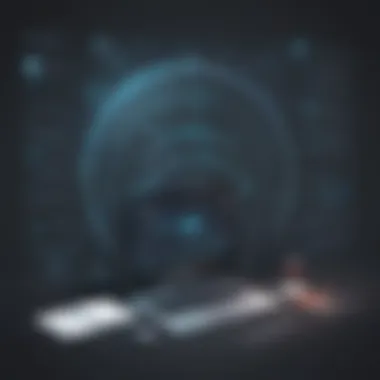
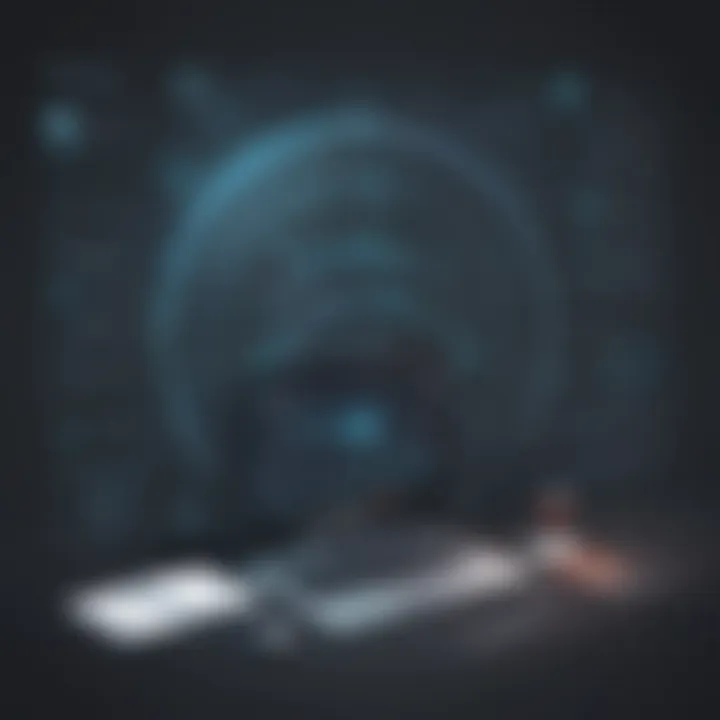
The Philosophy of Error Handling
Fail Fast vs. Fail Safe
The dichotomy between 'fail fast' and 'fail safe' encapsulates divergent philosophies in error handling, delineating the approach towards risk mitigation and system response. The 'fail fast' ideology advocates for immediate error exposure and abrupt termination, aiming to swiftly identify and rectify issues at the expense of system stability. Conversely, 'fail safe' principles prioritize error containment and graceful degradation, insulating the user experience from abrupt malfunctions by implementing robust fallback mechanisms. Understanding the nuances of these paradigms is instrumental in crafting error-tolerant software architectures.
Principles of Defensive Programming
Defensive programming principles epitomize a proactive stance towards software robustness, emphasizing preemptive error handling mechanisms and rigorous validation checks. By ingraining defensive coding practices, developers fortify their applications against unforeseen contingencies, erecting resilient barriers to prevent system vulnerabilities and malfunctions. The integration of defensive programming tenets augments code reliability and engenders a culture of meticulous error mitigation within the development lifecycle.
Benefits of Effective Error Handling
Enhanced Software Reliability
Elevating software reliability through effective error handling engenders a trust-based relationship between users and applications. By cultivating a reputation for operational consistency and reliability, software products instill confidence in their functionality and data integrity. Enhanced software reliability not only minimizes downtime and data loss but also fosters user loyalty and positive brand perception.
Improved User Experience
Augmenting user experience hinges fundamentally on the seamless functionality and error-free operation of software applications. Prioritizing error handling mechanisms that shield users from disruptive malfunctions heightens satisfaction and engagement. Improved user experience propels user retention rates, driving sustained user interaction and fostering organic growth through positive word-of-mouth referrals.
Faster Debugging and Troubleshooting
The expeditious resolution of errors is a cornerstone of software efficiency, instrumental in streamlining development cycles and minimizing operational disruptions. Fast-tracking debugging and troubleshooting processes accelerates problem resolution, enabling developers to allocate resources strategically and optimize productivity. Faster error resolution not only enhances code quality but also fortifies the overall development ecosystem, paving the way for continuous innovation and refinement.
Error Handling Strategies
In this section, we delve deep into the fundamentals of error handling strategies within the realm of software development. Having a robust error management system is crucial for maintaining stability and reliability in applications. By implementing effective error handling strategies, developers can mitigate risks and streamline the debugging process. Understanding the specific elements and benefits of error handling strategies becomes paramount in creating resilient software.
Reacting to Errors
Implementing Try-Catch Blocks
The implementation of try-catch blocks stands as a pivotal aspect of error handling strategies. These blocks allow developers to identify and manage exceptions efficiently, ensuring that the application can gracefully handle errors without crashing. Try-catch blocks offer a structured way to handle potential issues, promoting better code quality and fault tolerance. Despite the minor overhead they introduce, try-catch blocks are a popular choice for error handling due to their effectiveness in trapping and handling exceptions.
Utilizing Error Objects
Utilizing error objects provides a more nuanced approach to error handling within software development. Error objects encapsulate detailed information about an error, including its type and message, enabling developers to gain insights into the root cause of issues. By leveraging error objects, developers can create custom error messages, track error origins, and facilitate better error diagnostics. While error objects enhance error-handling capabilities, they may add complexity to the code and require careful implementation to avoid potential overhead.
Asynchronous Error Handling
Promises and Error Handling
The integration of promises in error handling represents a significant advancement in managing asynchronous operations. Promises offer a structured way to handle responses from asynchronous tasks, allowing developers to chain operations and handle errors effectively. By combining promises with error handling mechanisms, developers can ensure smooth error propagation and resolution in asynchronous workflows. Despite their advantages, promises may lead to callback hell if not handled properly, emphasizing the need for meticulous error handling practices.
Handling Errors in AsyncAwait Functions
Asyncawait functions streamline asynchronous programming by simplifying the syntax and enhancing readability. When it comes to error handling, asyncawait functions offer a more concise and intuitive way to manage asynchronous errors. By leveraging the try-catch syntax within async functions, developers can easily capture and process errors, promoting cleaner and more maintainable code. However, improper error handling within async functions can lead to uncaught exceptions and runtime issues, underscoring the importance of error management in async programming.
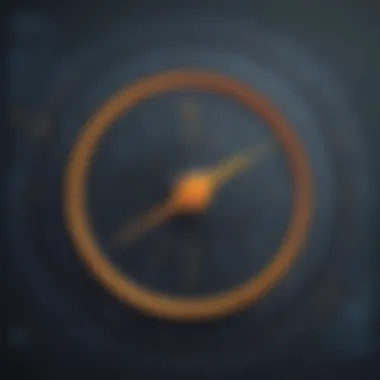
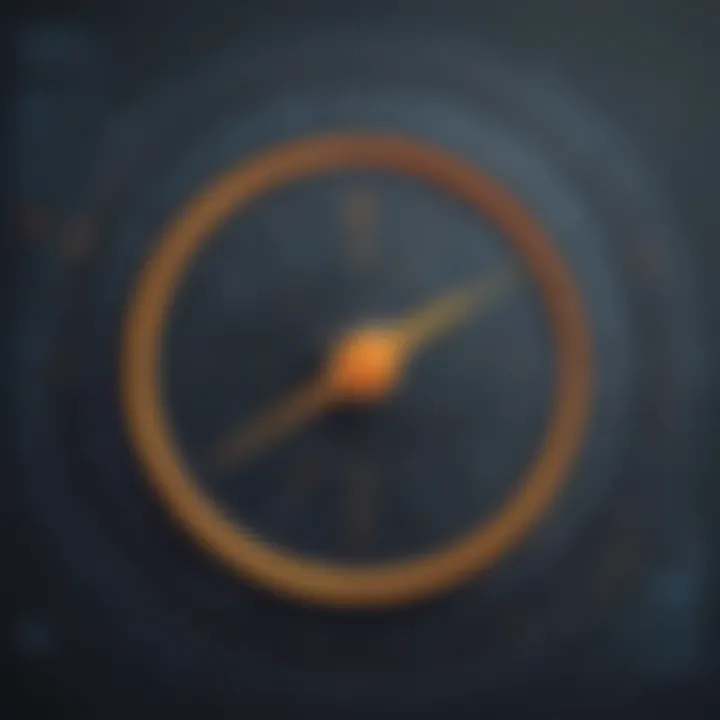
Logging and Monitoring
Logging Error Details
Logging error details plays a critical role in error monitoring and diagnostics within software applications. By recording pertinent information about errors, such as timestamp, error type, and stack trace, developers can gain valuable insights into application failures. Logging error details enables retrospective analysis, issue tracking, and performance optimization, enhancing the overall resilience of the software. While logging error details is essential for effective error management, overzealous logging can impact performance and pose data privacy concerns, necessitating a balanced approach to error logging.
Integrating Error Monitoring Tools
Integrating error monitoring tools elevates error detection and response capabilities in software development. Error monitoring tools provide real-time insights into application errors, enabling developers to proactively identify and address issues. By leveraging these tools, teams can set up alerts, track error trends, and prioritize critical issues, fostering a culture of continuous improvement. Despite their benefits, integrating error monitoring tools requires careful configuration and monitoring to avoid false positives and ensure accurate error reporting.
Section 3: Advanced Error Handling Techniques
In the realm of software development, mastering advanced error handling techniques is paramount for ensuring robust and reliable applications. Embracing advanced error handling techniques equips developers with the tools to effectively detect, manage, and recover from errors, thereby enhancing the overall resilience and performance of software systems. By delving into advanced error handling, developers can proactively anticipate and address potential issues, resulting in more robust and efficient software applications.
Error Recovery and Retry Mechanisms
Implementing Exponential Backoff
In the context of error handling, implementing exponential backoff is a crucial technique for mitigating failures and improving system stability. This approach involves gradually increasing the time between retries following each consecutive failure, allowing the system to recover and prevent overwhelming the resources. Implementing exponential backoff helps in alleviating congestion and reducing the likelihood of cascading failures, ultimately enhancing the overall fault tolerance of the system. While this strategy may introduce additional latency, its effectiveness in minimizing system overload and improving reliability makes it a valuable asset in error recovery scenarios.
Retrying Failed Operations
When faced with failed operations, retrying is a common strategy employed to ensure the successful completion of tasks. By automatically retrying operations that have failed due to transient issues, developers can enhance the chances of successful execution without requiring manual intervention. Retrying failed operations can improve the fault tolerance of systems by offering resilience against temporary failures, thereby reducing the impact of intermittent issues on the overall system performance. However, it is essential to implement retry logic carefully to prevent incessant retries that could potentially worsen the situation, striking a balance between recovery attempts and system stability.
Circuit Breaker Pattern
Principles of Circuit Breaker
The circuit breaker pattern is a vital component in error handling, enabling systems to limit the impact of failures and prevent cascading disasters. By integrating the principles of the circuit breaker pattern, developers can enhance system reliability by isolating faulty components and providing alternative pathways for processing requests. This proactive approach not only safeguards system integrity but also promotes graceful degradation, allowing the system to maintain partial functionality in the face of failures. The circuit breaker pattern serves as a pivotal mechanism for enhancing fault tolerance and minimizing the overall system downtime, making it an indispensable asset in modern software development.
Benefits of Circuit Breaker Implementation
Implementing the circuit breaker pattern offers a plethora of benefits for software systems, including improved reliability, enhanced fault tolerance, and efficient resource utilization. By introducing circuit breakers, developers can proactively monitor and manage system errors, preventing them from propagating throughout the system. The presence of circuit breakers not only safeguards critical services but also optimizes system performance by dynamically adjusting to varying conditions. Additionally, the implementation of the circuit breaker pattern promotes system stability and robustness, ensuring consistent service delivery even under challenging circumstances.
Graceful Degradation
Prioritizing Essential Functionality
In the context of error handling, prioritizing essential functionality is a strategic approach aimed at preserving core system capabilities in the event of failures. By identifying and prioritizing critical functions, developers can ensure that key services remain operational even during adverse conditions, thus maintaining overall system functionality. Prioritizing essential functionality allows systems to gracefully degrade by focusing on core services, while non-essential features may be temporarily suspended to allocate resources efficiently. This method of prioritization contributes to improved user experience and ensures that vital functions are available to users even under suboptimal conditions.
Designing for Partial Service Outages
Designing for partial service outages involves creating resilient systems that can adapt and operate effectively despite experiencing disruptions. By anticipating potential failures and planning for partial service outages, developers can implement strategies to minimize the impact on the end user experience. This proactive approach enables systems to maintain critical functionalities while gracefully degrading non-essential services, ensuring that users can continue to access key features even during service interruptions. Designing for partial service outages fosters system resilience and enhances overall user satisfaction by prioritizing essential functions and streamlining error recovery processes.
Error Handling in Microservices Architecture
Resilience Strategies for Microservices
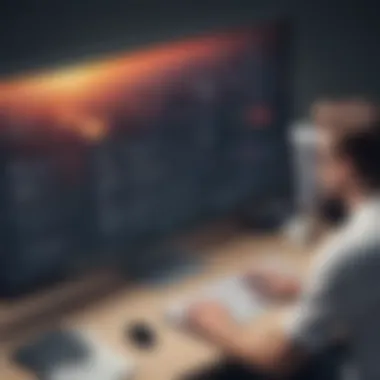
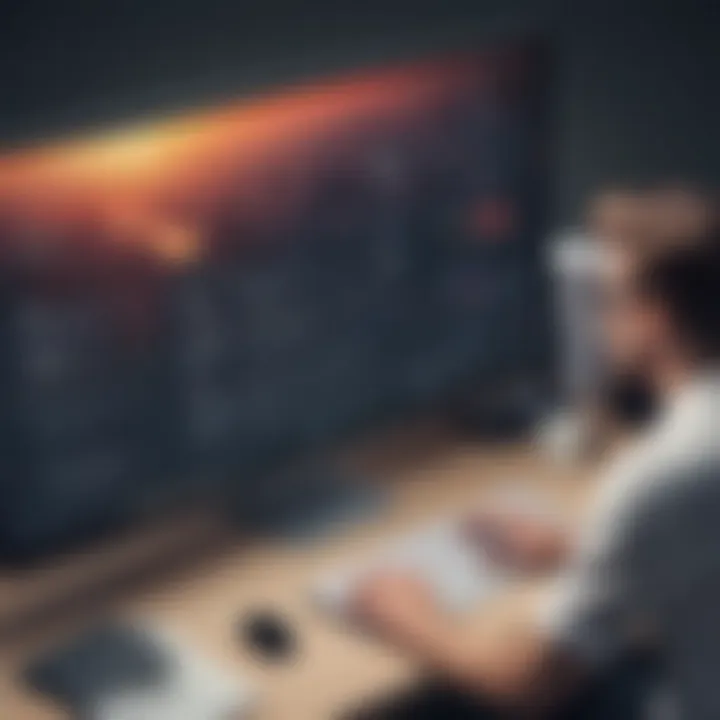
When dealing with errors in a microservices architecture, implementing resilience strategies is essential for maintaining system stability and performance. By adopting resilience strategies, developers can design microservices that can withstand failures and adapt to changing conditions without comprising overall functionality. Resilience strategies incorporate mechanisms such as fault isolation, graceful degradation, and auto-recovery to enhance the robustness of microservices systems. By prioritizing resilience, developers can ensure continuous service availability and seamless operation across distributed environments, mitigating the impact of errors on user experience.
Implementing Distributed Tracing for Error Identification
Implementing distributed tracing is a pivotal aspect of error handling in microservices architecture, enabling developers to monitor, analyze, and troubleshoot distributed systems efficiently. By implementing distributed tracing solutions, developers can track and trace the flow of requests across microservices, identifying error sources and performance bottlenecks accurately. This approach facilitates rapid error identification and resolution, improving system reliability and performance. Distributed tracing empowers developers to gain greater visibility into system behaviors and optimize error handling processes, ensuring seamless operation and enhanced user experience in microservices environments.
Best Practices and Tips
In the realm of software development, mastering the art of error handling requires a meticulous approach. Best practices and tips play a pivotal role in ensuring the smooth functioning of a software application. By adhering to these practices, developers can preemptively tackle errors and minimize their impact on the system. Emphasizing best practices not only enhances the efficiency of error handling but also promotes a culture of continuous improvement within the development team. The significance of implementing best practices and tips cannot be overstated; they serve as a blueprint for robust error management strategies, contributing to the overall stability and reliability of the software.
Documentation and Communication
Maintaining Detailed Error Logs
Maintaining detailed error logs is a cornerstone of effective error handling in software development. By meticulously recording error occurrences, developers gain valuable insights into the functioning of the application and can proactively identify and address recurring issues. Detailed error logs provide a comprehensive trail of events leading to an error, facilitating swift troubleshooting and resolution. The key characteristic of maintaining detailed error logs lies in its ability to act as a historical record, enabling developers to track the evolution of errors over time. This practice is a fundamental choice for this article as it promotes transparency and accountability in error management, empowering developers to make informed decisions based on data-driven analysis.
Establishing Clear Error Communication Channels
Establishing clear error communication channels is essential for fostering effective collaboration and swift problem resolution within a development team. Clear communication ensures that all team members are on the same page regarding error occurrences, facilitating seamless coordination and expedited error handling processes. The key characteristic of clear error communication channels lies in promoting transparency and clarity in conveying error-related information, minimizing misunderstandings and enhancing overall productivity. This practice is a popular choice for this article as it enhances organizational efficiency and promotes a culture of open communication, fostering a conducive environment for error resolution.
Continuous Improvement
Analyzing Common Error Patterns
Analyzing common error patterns is a crucial step towards refining error handling strategies and mitigating recurring issues in software development. By identifying patterns in error occurrences, developers can implement targeted solutions to address underlying root causes effectively. The key characteristic of analyzing common error patterns is its ability to drive proactive problem-solving by anticipating potential issues based on historical data. This practice is a beneficial choice for this article as it empowers developers to detect trends and patterns, facilitating preemptive measures to enhance error management practices.
Feedback Loops for Enhancing Error Handling
Implementing feedback loops for enhancing error handling fosters a culture of continuous learning and improvement within a development team. Feedback loops enable developers to gather input from users, stakeholders, and team members regarding error experiences, allowing for iterative refinement of error management strategies. The key characteristic of feedback loops lies in their role in promoting agility and adaptability in error resolution, enabling developers to iterate on solutions based on real-time feedback. This practice is a popular choice for this article as it encourages a feedback-driven approach to error handling, emphasizing the importance of user-centric design in software development.
Collaboration and Knowledge Sharing
Cross-Team Error Resolution Workflows
Enabling cross-team error resolution workflows is imperative for fostering communication and collaboration across different departments within an organization. By streamlining error resolution processes and workflows, companies can expedite the identification and mitigation of errors, minimizing system downtime and enhancing overall productivity. The key characteristic of cross-team error resolution workflows lies in their ability to break down silos and encourage interdisciplinary collaboration, leading to holistic error management practices. This practice is a beneficial choice for this article as it promotes synergy and knowledge sharing among diverse teams, fostering a culture of collective ownership and responsibility towards error resolution.
Conducting Error Handling Workshops
Conducting error handling workshops is an effective way to disseminate best practices and share knowledge regarding error management techniques within a development team. Workshops provide a structured platform for team members to learn from each other, exchange ideas, and collectively enhance their error handling skills. The key characteristic of error handling workshops is their role in promoting continuous learning and skill development, equipping developers with the necessary tools to tackle errors efficiently. This practice is a valuable choice for this article as it encourages interactive learning and peer-to-peer knowledge sharing, fostering a culture of collaboration and continuous improvement in error handling practices.
Conclusion
In the overarching realm of software development, the aspect of error handling stands as a beacon of critical importance. The Conclusion section of this article serves as the capstone, merging all preceding discussions into a cohesive understanding of the significance of mastering error handling. By encapsulating the key points elucidated throughout the narrative, developers are reminded of the pivotal role error management plays in creating robust and resilient software applications. Furthermore, it emphasizes the long-term benefits of investing time and effort into honing error handling skills, as they directly contribute to enhanced software reliability, improved user experience, and expedited debugging processes. Acknowledging the multifaceted nature of error handling is paramount in instilling a culture of continuous improvement within development practices, ensuring that mastery of this art is not just a goal but a necessity in the quest for software excellence.
Embracing Error Handling Excellence
The Path to Mastery
Delving into the intricacies of error handling, 'The Path to Mastery' underscores a pivotal aspect that defines the pursuit of excellence in error management. This specific facet emphasizes the journey that developers undertake in refining their error handling skills, nurturing an environment of mastery and adept problem-solving. The key characteristic of this path lies in its iterative nature, where each encountered error serves as a stepping stone towards greater proficiency. Adopting 'The Path to Mastery' not only fosters a proactive approach to error detection and resolution but also cultivates a mindset geared towards continuous learning and enhancement. Despite the challenges posed by complex error scenarios, embracing 'The Path to Mastery' equips developers with the resilience and adaptability required to navigate the intricate landscape of software development effectively.
Innovation Through Error Management
'Innovation Through Error Management' carves a distinctive niche within the domain of software development by spotlighting the transformative potential inherent in leveraging errors as avenues for innovation. This specific facet champions a paradigm shift in viewing errors not as setbacks but as springboards for creative problem-solving and novel solutions. The key characteristic of this approach lies in its ability to incite a culture of experimentation and creative thinking, wherein errors are reframed as opportunities for growth rather than obstacles to success. By embracing 'Innovation Through Error Management,' developers can harness the power of adaptive resilience, fostering a dynamic ecosystem that thrives on constructive failure and iterative improvement. Despite the inherent risks associated with innovation, this approach carries the promise of breakthroughs that redefine traditional paradigms and propel software development towards new frontiers of excellence.