Mastering the Art of Debugging in Android Development
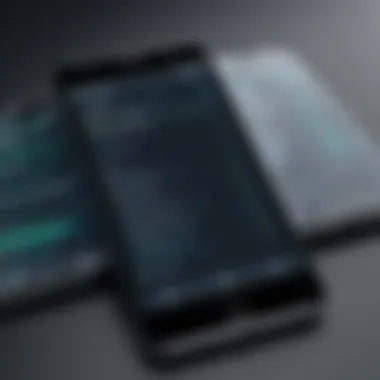
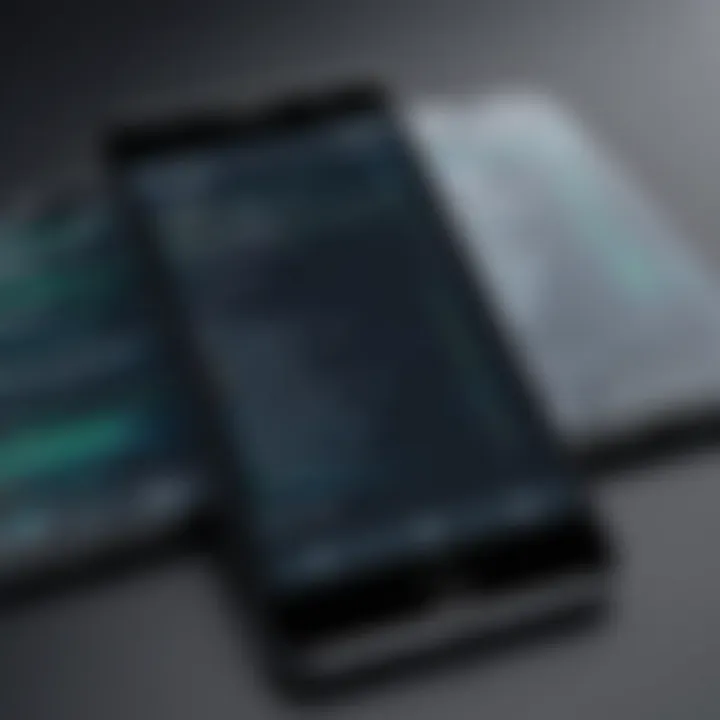
Intro
In the ever-evolving landscape of Android development, debugging stands as a crucial pillar that supports the construction of effective and reliable applications. Without doubt, a high-quality application can be marred by bugs and glitches, which often leave users frustrated. Consequently, grasping the nuances of debugging becomes indispensable for every developer aiming to thrive in this competitive arena.
Debugging isn't just about fixing issues; it's an art that involves systematically analyzing and resolving problems within your code. It’s akin to finding a needle in a haystack, where every line of code holds the potential for both errors and breakthroughs. As you navigate this process, having a well-defined approach, combined with the right tools, helps illuminate the path to successful app development.
By delving into the various debugging methodologies and tools, one can develop a more comprehensive understanding of how to tackle issues that may surface during the development cycle. Armed with this knowledge, developers will not only enhance their technical skills but also foster a more robust relationship with their applications.
In this article, we will traverse the landscape of debugging in Android development, stressing its significance, tools available for this endeavor, and the best practices that can lead to fewer headaches down the road.
Prolusion to Debugging in Android
When it comes to Android app development, the art of debugging is indispensable. It’s not merely about fixing bugs; it’s about understanding and navigating the intricate maze of code. Efficient debugging can be the difference between a smooth user experience and a jarring one. It ensures that applications run as intended and provides feedback for improvements.
Debugging plays a crucial role in the software development lifecycle. It allows developers to identify and address issues before they reach the user. This proactive approach saves both time and resources, while also enhancing code quality. Think of debugging as a crucial health check for your application—much like a doctor diagnosing a patient to prevent further complications.
The Importance of Debugging
Debugging is vital for several reasons. First, it safeguards the performance of an application. A properly debugged application is less likely to crash or behave unexpectedly, which can lead to user frustration and a tarnished reputation for your app.
Second, debugging helps in improving the overall user experience. End users expect apps to perform seamlessly; addressing bugs can enhance usability, engagement, and satisfaction. In a fiercely competitive market, an app that runs well can stand head and shoulders above its rivals.
Lastly, debugging fosters learning and skill enhancement among developers. Each encounter with a bug serves as a lesson, contributing to the developer's growth. The more you debug, the sharper your problem-solving skills become.
Common Bugs in Android Development
Android development comes with its fair share of challenges, and common bugs can often derail even the best-laid plans. Here’s a look at typically encountered issues:
- Null Pointer Exceptions: This occurs when an application tries to access an object or variable that has not been initialized. It’s one of the most frequent causes of crashes in Android applications.
- UI Thread Blocking: Long-running operations conducted on the UI thread can lead to unresponsive interfaces. It’s crucial to ensure that time-consuming tasks run in the background.
- Memory Leaks: These occur when an application holds onto memory that it no longer needs. Over time, this can lead to slow performance or even crashes, as the app consumes excessive memory.
- Incorrect Permissions: With a plethora of features requiring user permissions, failing to handle these correctly can result in malfunctioning apps.
- Incompatibility with Different Devices: Android runs on various hardware configurations. An app working seamlessly on one device may crash on another due to differences in specifications.
In summary, understanding the importance of debugging, along with recognizing common bugs, sets a strong foundation for developers. Addressing these issues early on minimizes the risk of larger problems later, ultimately leading to a more robust and user-friendly application.
Basics of the Android Debug Bridge
Debugging in Android development often hinges on the efficiency and effectiveness of the tools employed. One such indispensable tool is the Android Debug Bridge, commonly referred to as ADB. This powerful command-line utility acts as a bridge between developers' machines and Android devices. Understanding how ADB operates is critical to streamlining the debugging process and optimizing workflows.
What is ADB?
At its core, ADB is a versatile command-line tool that allows developers to communicate with Android devices. It's like the Swiss Army knife of Android development, offering a wide range of functionalities that include file transfers, installation of apps, and running shell commands. Think of it as a middleman that facilitates the connection between your development environment and the actual hardware or emulators you’re working with.
Here are some key functions of ADB:
- Device management: ADB can list connected devices that are available, making it easy to keep track of what you’re working on.
- Log output retrieval: Developers can view and analyze logs in real-time, which is an essential part of identifying issues.
- Installation and uninstallation of apps: ADB allows you to install apps directly from your computer without needing to navigate through your device’s UI.
- Shell access: It grants low-level access to your Android device’s shell, enabling detailed commands that assist in debugging overall performance.
In essence, ADB is fundamental to Android development; it simplifies many tasks that developers face daily, making it indispensable for streamlined debugging and testing.
Configuring ADB for Your Environment
Setting up ADB correctly is crucial for optimal performance. It involves ensuring that your computer can communicate with Android devices, which might seem daunting, but it’s straightforward.
Begin by following these steps to configure ADB:
- Download Android SDK Platform Tools: Make sure you have the latest version, which can be found on the official Android Developer website.
- Enable USB Debugging on Your Device: Go to your device’s settings, navigate to 'About phone,' tap 'Build number' seven times to unlock developer options. Then, enable USB debugging.
- Connect Your Device: Use a USB cable to connect your device to your computer. In most cases, the device should be recognized automatically. You can verify this by running the command .
- Set Environment Variables (for Windows users): Add the path to ADB in your system environment variables to run ADB commands from any command prompt window.
Proper configuration not only enhances the functionality of ADB but also saves time and effort in the debugging process.
“Efficient debugging is not just about fixing issues; it's about leveraging tools like ADB to improve overall productivity.”
When developers invest time in understanding and configuring ADB meticulously, they create a robust foundation for tackling any challenges that arise in the Android development cycle.
Debugging Tools Available in Android Studio
In the realm of Android development, the tools you choose can make all the difference in debugging efficiency. Without a solid understanding of available debugging tools, developers may find themselves tangled in a web of errors, inefficiencies, and missed deadlines. Android Studio is packed with several robust debugging tools that streamline the process, allowing developers to catch issues before they ever reach the end user. The heart of effective debugging is understanding how to wield these tools effectively.
The Logcat Tool Explained
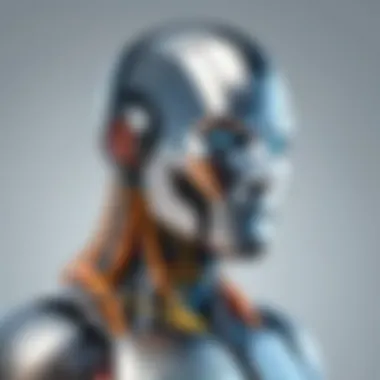
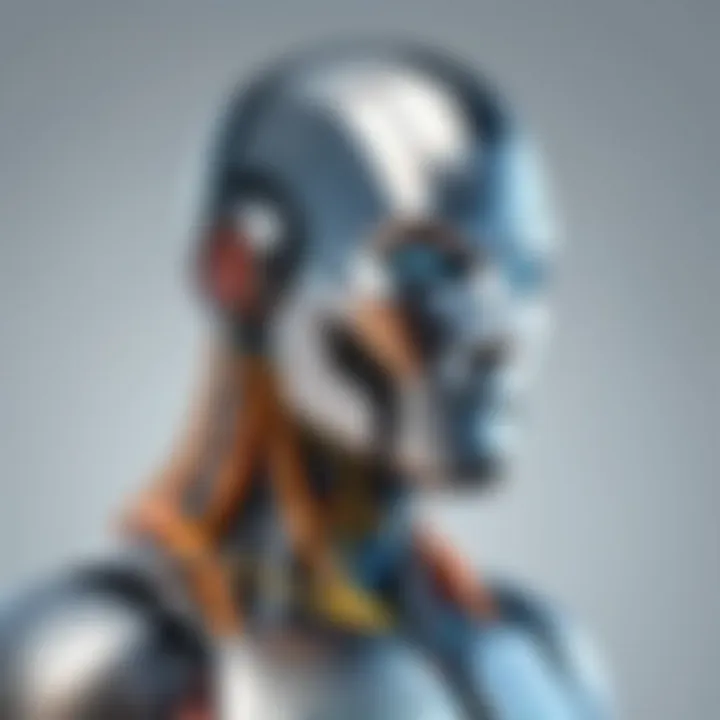
Logcat is arguably one of the most crucial debugging tools integrated into Android Studio. It serves as a log output for the various events occurring within the Android operating system and your application. It is where you can observe application logs, system messages, and errors that occur on the device. By filtering log messages, developers can target specific issues in their codebase swiftly.
Using Logcat effectively means knowing how to interpret the output it provides. Each log entry contains categories like "debug," "info," "warn," and "error." Understanding these categories can help you adjust your development approach as you troubleshoot.
For instance, you can search logs with keywords related to the issues you are experiencing. This allows you to pin down bugs without sifting through an avalanche of information. Here’s a simple example of how a log message may appear:
In this message, alerts you to a significant error, while the stack trace provides details about where in your code the issue lies. This makes Logcat a lifeline for developers grappling with elusive bugs.
Using the Android Emulator
The Android Emulator is more than just a virtual device; it’s a vital asset in your debugging toolkit. It allows developers to approximate the experience of various Android devices without needing the physical hardware. This can be particularly handy for testing how your application behaves under different conditions, such as varying screen sizes, orientations, or even network states.
The emulator’s ability to mimic real-world scenarios enables intensive testing. For instance, if your app needs to work offline and gracefully handle data syncing when a connection re-establishes, you can simulate loss of internet connection rather easily. This functionality not only aids in debugging but can also enhance the overall user experience by ensuring your app is resilient in adverse conditions.
Moreover, with the emulator, you can take advantage of its logging capabilities right alongside Logcat, allowing for a more integrated debugging experience. This seamless interchange empowers developers to identify issues rapidly while ensuring the application’s interface is responsive and intuitive.
The Profiler Tool for Performance Analysis
When it comes to optimizing your application’s performance, the Profiler tool in Android Studio becomes invaluable. Whether you're tracking CPU usage, memory consumption, or network traffic, the Profiler provides a comprehensive overview of your app's performance characteristics. This is particularly useful for spotting inefficiencies that can lead to lag or crashes, ensuring your app runs smoothly under typical user conditions.
The tool breaks down resource consumption in real time, with various graphs and metrics that highlight performance hotspots. For example, if your application is consuming an abnormally high amount of memory, you can quickly identify which methods or processes are responsible. This empowers developers to make targeted optimizations as opposed to guessing what might be causing the slowdown.
Moreover, using the UI to analyze how different components perform allows for a rich understanding of your app's architecture. You can isolate slow-performing activities, track memory allocation, and more—ultimately performing a deeper dive into performance bottlenecks.
The Profiler tool is essential for ensuring that your application runs lean and mean, enhancing user satisfaction and retention in an increasingly competitive app marketplace.
By becoming adept at utilizing these debugging tools within Android Studio, developers can not only increase their productivity but also ensure that they deliver robust applications to end users. The importance of these tools cannot be overstated, as they lay the groundwork for a more efficient debugging process and the refined end product.
Setting Up Breakpoints
In the world of Android development, breaking down the lines of code with precision can often be the difference between a simple bug fix and hours of painstaking searches. That's where setting up breakpoints comes into play. These little markers allow developers to pause the execution of code at specific lines, permitting them to inspect the state of the application at that moment. The beauty of breakpoints lies in their ability to illuminate the flow of your program, revealing insights that can be missed when scanning lines of code in a debugger or console.
How to Place Breakpoints
Setting a breakpoint in Android Studio is quite straightforward—think of it as placing a pin in a map where you want to stop and take a closer look. Here’s a quick rundown on how to place breakpoints effectively:
- Open the Android Studio and navigate to your source code.
- Find the line where you suspect an issue might be lurking.
- Click in the gutter area (the left margin where line numbers are shown) next to the line of code you want to inspect. A blue dot will appear, signifying that a breakpoint has been established here. 🛑
- You can also right-click on the line and select "Toggle Breakpoint" from the context menu.
- To run your code with breakpoints, simply start debugging by clicking the bug icon instead of the normal run icon.
Once the application hits your breakpoint during execution, the debugger will stop, allowing you to see the variables and state of your application at that exact moment; you can then step through your code line by line to understand its behavior better.
Conditional Breakpoints
Now, not all breakpoints need to be simple pausing points. Conditional breakpoints are like putting additional filters on your debug session. These allow you to specify conditions that must be met for the breakpoint to activate. This can greatly reduce the number of times your code stops, especially in loops or high-frequency methods.
To set up a conditional breakpoint in Android Studio:
- Right-click on the existing breakpoint dot in the gutter.
- Choose "Breakpoint Properties" from the menu.
- Check the box labeled "Condition".
- Enter your condition in the provided text field. This is typically a logical statement like or .
Once set, the breakpoint will only trigger if the condition specified evaluates to . This functionality can save a ton of time and focus, allowing you to bypass sections of code that are irrelevant to your current debugging session.
Key Takeaway: Breakpoints, particularly conditional breakpoints, can significantly streamline the debugging process by allowing you to target specific code execution points, helping navigate through the complex maze of your application's logic with ease.
Analyzing Errors and Exceptions
Understanding the intricacies of errors and exceptions is vital for developers working in Android. It serves not just as a tool for troubleshooting but also as a foundational element in improving application durability and user experience. By effectively analyzing these issues, developers not only rectify the current problems but also instill a habit of proactive problem-solving which is invaluable in the long run.
Analyzing errors and exceptions allows developers to trace failures back to their source, which is essential in preventing future occurrences. This process helps in identifying patterns that may indicate design flaws or misunderstandings in the API being used. With the Android ecosystem's complexity, getting a firm grip on how to interpret and react to these issues can save substantial time and effort.
Understanding Stack Traces
When an application crashes, the first line of attack is often the stack trace. This allows developers to pinpoint where the error occurred. A stack trace provides a snapshot of the active stack frames at a certain point during the execution of the program. It essentially tells you, "Here’s where you went wrong, and here’s how we got there."
In typical practice, stack traces can be a bit overwhelming. They serve up lines of code and method calls, which can appear cryptic at first glance. However, familiarizing oneself with its structure is crucial.
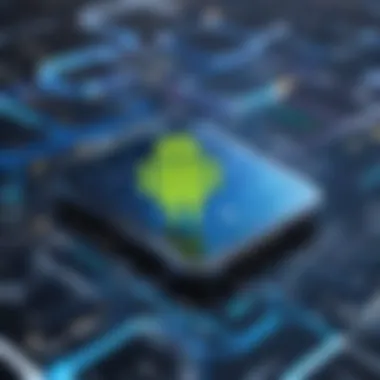
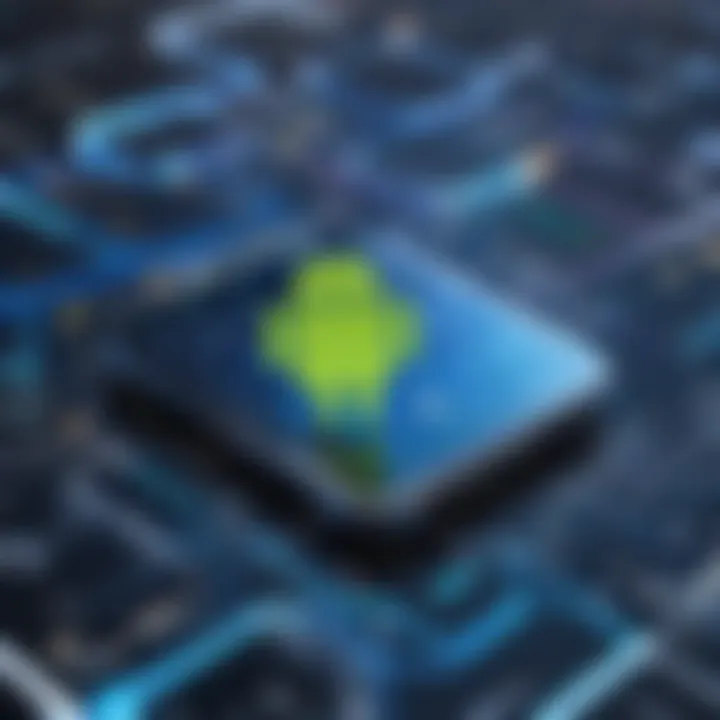
- Starting Point: The first line of the stack trace usually indicates the exception type—this is your seed of insight.
- Method Calls: Subsequent lines will show the path the application took before hitting the wall, essentially depicting the journey through various methods.
- File and Line Numbers: Each entry specifies the file name and the line number, directing you precisely to the culprit in your code.
Understanding this structure can shift one from an inexperienced coder to a more seasoned one over time.
"A problem well stated is a problem half solved."
- Charles Kettering
Common Exception Types
Android encompasses various exception types that developers should be mindful of. Each indicates a different kind of issue that can creep up during development. Some frequently faced exception types include:
- NullPointerException: Stemming from attempts to access methods or properties of an object that is not instantiated, this exception often feels like a ghost at the feast.
- ArrayIndexOutOfBoundsException: This occurs when an invalid index is accessed in an array. Basically, it’s trying to climb a tree but landing flat on the ground instead.
- ClassCastException: Happens when casting an object to a class it can't belong to. It’s like trying to fit a square peg in a round hole.
Addressing these exceptions typically involves careful examination of the code around the error. Ensure you’re checking for nulls where necessary, validating inputs, and keeping your indexes in check.
By recognizing and comprehending these common pitfalls, developers can sharpen their debugging skills, making their Android applications more robust and reliable.
Employing Unit Testing in Debugging
In the realm of Android development, unit testing holds a prominent place in the debugging arsenal, acting as a first line of defense against bugs sneaking into production. It’s not just an optional step; it transforms the debugging process from a chaotic hunt into a systematic approach. By offering a way to validate individual parts of the code, developers can catch issues earlier, leading to a cleaner integration down the road.
The core of unit testing is creating small, isolated tests that provide feedback on specific functionalities. This proactive stance can save developers a heap of time by identifying problems early on rather than dealing with the aftermath of a faulty application. Furthermore, it helps in building confidence while refactoring code by ensuring that existing functionality remains intact.
Benefits of Unit Testing
- Early Detection of Bugs: One of the most significant advantages is the ability to find and fix bugs sooner in the development cycle. This early detection minimizes the risk of introducing bugs during later stages of development.
- Simplified Debugging Process: When a unit test fails, the scope of the problem is usually limited to a small part of the code. This focused approach makes it easier to identify and fix issues compared to hunting through the entire application.
- Improved Code Quality: Writing tests encourages developers to write cleaner, more modular code. When code is easier to test, it often results in higher quality, more maintainable software.
- Facilitates Refactoring: With a robust suite of unit tests, developers can make changes to the codebase more confidently, knowing that tests will catch any breaking changes.
- Documentation of Expected Behavior: Unit tests serve as a form of documentation for the code, outlining what the code is supposed to do. This is particularly beneficial for onboarding new developers onto a team.
Best Practices for Writing Unit Tests
- Keep Tests Isolated: Each test should focus on a single unit of functionality. Isolation helps to ensure that tests do not interfere with each other, providing clearer feedback when something goes wrong.
- Descriptive Test Names: Naming tests in a descriptive and explicit manner provides context and makes it easier to identify what each test is verifying. For instance, a test called makes it much clearer than simply naming it .
- Test Coverage: Aim for high test coverage but focus on critical paths that are most likely to become problematic. Not every line of code needs a test; prioritize parts of the application that users interact with the most.
- Regularly Update Tests: As the application evolves, ensure that tests are updated accordingly. Outdated tests can lead to false positives or negatives, undermining confidence in the testing suite.
- Embrace Automation: Leverage continuous integration (CI) tools to automate the running of unit tests. This integration ensures that tests are run frequently, identifying potential issues as they arise.
"Unit testing is like having a safety net; it catches you before you hit the ground."
Through methodical unit testing practices, Android developers can not only make their debugging process leaner but also foster a culture of quality and reliability in their projects. As the scope and complexity of applications expand, the importance of integrating unit testing into the development workflow cannot be understated. This approach lays the groundwork for more sustainable and maintainable application development.
Advanced Debugging Techniques
When it comes to crafting a seamless Android application, the debugging process is not just a chore; it's an art. Advanced debugging techniques play a crucial role in sharpening this craft, allowing developers to track down elusive bugs that may otherwise slip through the cracks. In an era dominated by increasingly complex applications, deploying the right debugging strategies can be the edge needed to deliver a quality user experience while also minimizing production delays.
The significance of advanced debugging techniques extends beyond fixing immediate issues; they help in establishing a more robust development cycle. Understanding how to implement these methods not only fosters efficiency but also instills confidence in your debugging practices. Such techniques can save hours, sometimes days, of frustration rooted in unresponsive interfaces or erroneous outputs.
Remote Debugging Strategies
In today’s interconnected world, working remotely is the norm for many developers. Remote debugging emerges as a lifeline in such scenarios, allowing for real-time problem-solving without being bound to a specific physical location. This method typically employs tools such as Android Studio, which can connect to a device via Wi-Fi or USB, enabling developers to troubleshoot issues on actual hardware without needing to be physically present.
Utilizing remote debugging can be particularly beneficial when dealing with physical devices that exhibit bugs not replicable on an emulator. For example, performance issues related to specific device configurations often arise; remote debugging allows investigation directly on that device. With this setup, developers can:
- Place breakpoints and inspect variables in real time.
- Analyze log outputs to fine-tune performance and usability.
- Test app functionalities characteristic of specific OS versions or manufacturer modifications.
Considering security is paramount when setting up remote debugging. Employ secure connections and ensure that only authorized personnel have access. It's simple to overlook these details, but failing to do so can lead to serious vulnerabilities in deployed applications.
Debugging in Production Environments
Debugging within production environments is often a tightrope walk. Mistakes can lead to significant downtime or worse, a degraded user experience. Thus, a strategic approach becomes indispensable. First off, leveraging logs and exception reporting tools can facilitate near-instant feedback on a live application. Tools like Firebase Crashlytics or Sentry allow developers to gather insights on app crashes and exceptions in real time.
When debugging in these environments, consider adopting a robust method for monitoring app health alongside any performance metrics. This systematic analysis ensures that any issues can be swiftly addressed, reinforcing that the app continues running smoothly.
"Involving your end users in bug reporting can be a game changer. Their insights can lead to quicker resolution of issues that developers might overlook."
It's wise to minimize the direct debugging of live code. Any changes should be tested in a staging environment before deployment to production. Implementing feature flags can help in rolling out updates gradually, allowing for easier rollback in case problems arise. This strategy not only reduces risk but also improves the overall user experience, as features can be enhanced without bugs getting between users and app functionalities.
To sum it up, embracing advanced debugging techniques serves as a pathway to richer performance and more reliable applications. Whether through remote debugging or confidently handling issues in production, these strategies ensure developers create robust software that meets user needs.
Performance Tuning via Debugging
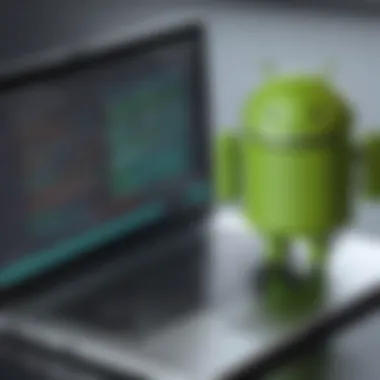
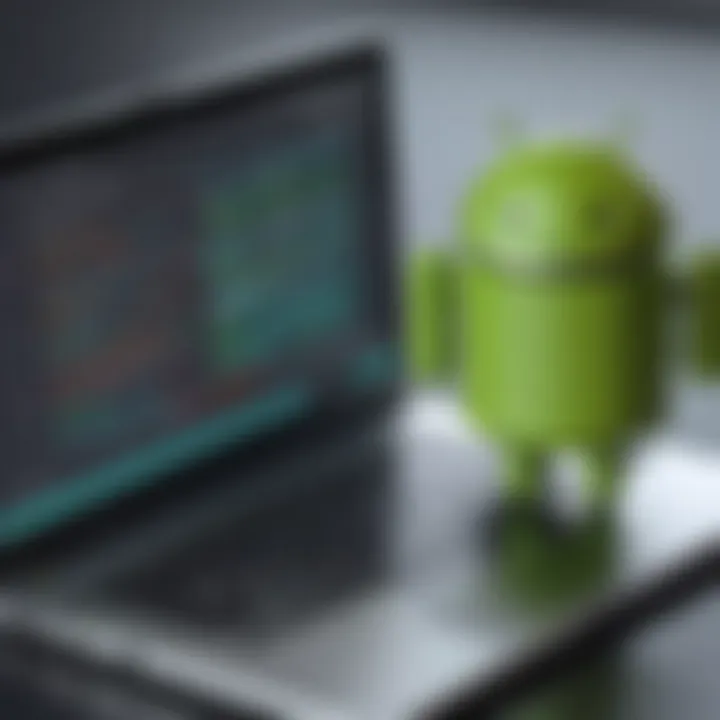
Performance tuning in Android development can make or break an application. When an app lags or crashes, user satisfaction plummets, resulting in uninstalls and negative reviews. By effectively tuning performance through debugging, developers can ensure a smoother user experience, enhance the app's responsiveness, and maintain stability.
Incorporating performance tuning into debugging offers several benefits. It leads to reduced load times, efficient resource utilization, and improved battery life for mobile devices. A keen eye on performance metrics helps developers identify bottlenecks, such as excessive memory usage or slow processing times. Addressing these issues not only polishes the app but also contributes positively to the platform’s overall ecosystem.
Using Profiling Tools for Optimization
Profiling tools stand as essential allies in the quest for optimization. Tools like Android Profiler provide real-time tracking of app performance across CPU, memory, network, and energy consumption. Engaging with these tools can surface insights that are neither apparent via traditional debugging nor observable in a casual code review.
For instance, the CPU Profiler can allow you to monitor thread activity and pinpoint which functions or processes are hogging resources. Notably, this granularity can enable timed segment analysis—showing exactly when specific functions are invoked and how the app behaves in response. Learning to interpret these profiling graphs can be a game changer, revealing underlying issues that would otherwise be baffling.
Some common steps you can take when using profiling tools include:
- Identify Hotspots: Determine where the application spends the most time.
- Measure Response Times: Analyze metrics for various functionalities to ensure they meet expected performance criteria.
- Track Resource Usage: Maintain an eagle eye on memory and CPU usage patterns.
Memory Leak Detection
Memory leaks are the silent killers of mobile applications. If an app is not releasing memory properly, it can lead to situations where the application becomes sluggish and unresponsive over time. Finding and fixing memory leaks is crucial—especially in more extensive applications with numerous components lingering in memory longer than necessary.
Using tools like LeakCanary, developers can seamlessly monitor their applications for memory leaks and receive actionable feedback. LeakCanary provides real-time notifications whenever it detects a memory leak, showcasing the object references still retained after being no longer needed. This feature can be invaluable, especially when combined with the Android Studio Profiler.
To ensure memory leak detection is part of your debugging routine, consider the following:
- Routine Checks: Regularly run diagnostic checks for memory leaks during development, not just during testing phases.
- Static Analysis: Deploy tools that automatically scan code for common memory management pitfalls before running the app.
- Garbage Collection Visibility: Understand when and how garbage collection occurs to appreciate the lifecycle of objects in memory effectively.
"A stitch in time saves nine; identifying memory leaks early can save hours of debugging later on."
By making these practices a habit, developers can substantially elevate their application's performance along with user satisfaction.
Common Pitfalls in Debugging
Understanding the common pitfalls in debugging is crucial for developers who want to write maintainable and functional applications. It’s easy to get lost in the intricacies of technology. The intent here is not just about highlighting these pitfalls, but also about nurturing an understanding that can lead to better practices in everyday development. With many developers focusing tirelessly on finding and fixing bugs, sometimes they overlook certain key elements that can complicate the process.
Over-Reliance on Tools
While tools like Android Studio, Logcat, and various testing frameworks can make debugging much easier, an over-reliance on these tools can lead to sloppiness. The dependence on automated processes might create a false sense of security, leading developers to believe that tools will catch every mistake. However, this view is misleading. Tools are just that—tools. They require human intuition and experience to interpret results correctly.
One example can be seen in analyzing stack traces. Novice developers may take them at face value, assuming they fully indicate where a bug resides. Advanced developers understand that stack traces might just point towards the symptom of a deeper issue rather than the original source of the problem. Here's an important thought:
"Tools can't think for you; they can only assist."
For instance, if a developer relies solely on unit tests provided by a framework, they may overlook scenarios that were not explicitly coded for testing. This can lead to significant bugs appearing later, during user interactions. To avoid this pitfall, it's essential to balance tool use with thorough understanding and manual checking processes.
Neglecting Documentation
Documentation often takes a backseat in the development process, but neglecting it can create a quagmire down the road. Many times, when issues arise, developers forget about the importance of documentation—both of the code itself and related knowledge. This neglect can lead to confusion and wasted time when trying to decipher how pieces of code interact or when onboarding new team members.
For example, if a function performs an unusual operation without clear comments or documentation, when bugs arise from that function, it takes far longer to debug, as the developer has to remember how it works rather than refer to documented explanations. Moreover, documentation doesn’t just have to be in text; flowcharts and diagrams can often communicate ideas far better than lines of code.
Here are some thoughts to keep in mind:
- Proper documentation can save hours of debugging time.
- It fosters teamwork, making it easier for peers to understand your work.
- Good documentation practices help in maintaining code over time.
In summary, knowing the traps that come with debugging is half the battle. Avoiding these pitfalls will not only make you a better developer but also ensure that your projects run smoothly in the long term.
Finale
As we close the chapter on debugging in Android development, it’s essential to reflect on the multitude of aspects that have been discussed. Debugging isn’t merely a chore to check off your development to-do list; it’s a fundamental skill that can significantly enhance your code's quality and functionality. By making debugging a crucial part of the development workflow, developers can pinpoint issues swiftly and efficiently, improving both productivity and the end-user experience.
Recap of Key Concepts
Underlining the significance of the key takeaways, here are several points that stand out:
- The Role of ADB: Understanding the Android Debug Bridge can transform how developers interact with devices and emulators, allowing for streamlined debugging processes.
- Utilizing Android Studio Tools: The built-in tools in Android Studio, including Logcat and the Profiler, are indispensable for diagnosing performance issues and runtime exceptions.
- Setting Breakpoints: Strategically placing breakpoints enables developers to inspect code execution closely, facilitating much clearer error identification.
- Errors and Exceptions: Familiarity with common stack traces and exceptions can help in debugging across different project types.
- Unit Testing Best Practices: Integrating unit testing into your development cycle can forewarn of potential issues, alleviating some of the debugging burdens.
- Avoiding Pitfalls: Over-reliance on tools or neglecting documentation can create further problems down the road.
This recap highlights that successful debugging requires a blend of technical acumen, tool utilization, and a systematic approach to potential pitfalls.
Future of Debugging in Android Development
Peering into the future, the landscape of debugging is likely to evolve in tandem with advancements in technology. Here are some trends and considerations that might shape the debugging processes:
- AI-Powered Debugging Tools: With the rise of artificial intelligence, expect tools that can automatically diagnose and suggest fixes for common bugs, potentially relieving developers from mundane tasks.
- Increased Emphasis on Automated Testing: The integration of more robust automated testing frameworks could lead to earlier detection of bugs, reducing the need for extensive debugging sessions.
- Cross-Platform Development: As cross-platform frameworks gain traction, understanding how debugging translates across different environments might become crucial.
- Greater Collaboration Tools: With teams increasingly working remotely, collaborative debugging tools that facilitate shared insights and solutions may see wider adoption.
In summary, debugging is a continuously evolving process that will adapt alongside changing technologies and methodologies. Staying updated on these trends will not only keep developers sharp but also equip them to tackle emerging challenges head-on.
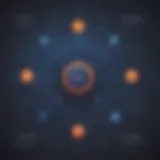
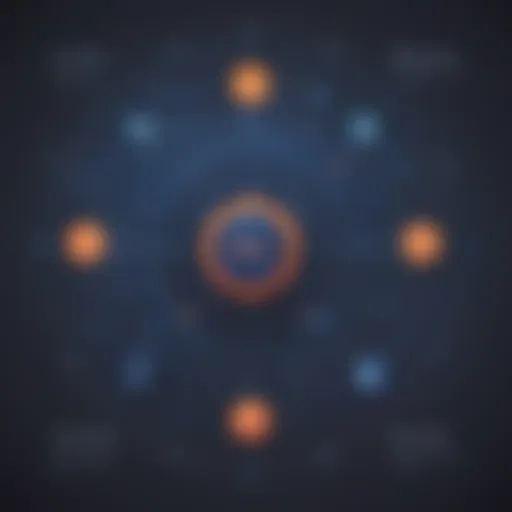