Mastering C# Programming: A Step-by-Step Guide
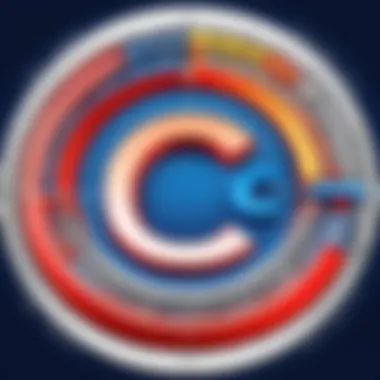
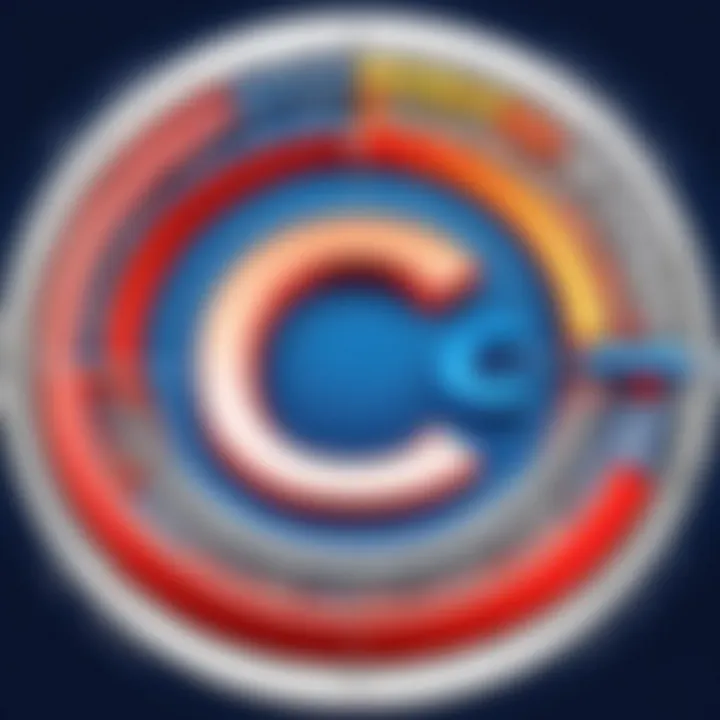
Intro
Creating programs in C# offers a spectrum of possibilities, making it one of the most versatile languages in today's software landscape. Understanding its foundational principles is essential not only for newcomers but also for seasoned developers who want to refine their approach. This guide aims to bridge the gap between abstract concepts and tangible skills, providing a roadmap for effective C programming.
Many people wonder how C# connects with broader themes like software development in general, cloud computing, and even data analytics. Grasping these intersections not only enhances understanding but also reveals how C can be most effectively applied.
Let us dive into key sections that will illuminate the relevance and power of C# programming, guiding you step by step from foundational skills to advanced applications.
Prologue to
Programming
C# programming is more than just a language; it's a gateway into the expansive world of modern software development. It blurs the lines between simplicity and power, providing developers â whether novices or seasoned professionals â an opportunity to create everything from simple applications to complex enterprise solutions. With its robust features, C is a linchpin in the tech ecosystem. Itâs especially revered for its object-oriented approaches, which help in crafting reusable and maintainable code.
Overview of
Language
C# (pronounced C-sharp) was designed with developer productivity in mind. It offers a syntax that is clean and organized. One key feature is type safety, which helps reduce errors during development. This ensures that the data types used in coding are checked at compile time, reducing the chances of unexpected behaviors at runtime.
Another significant aspect of C# is its versatility. Developers can build desktop applications, cloud-based solutions, and even mobile apps. With the advent of .NET Core, C has gained cross-platform capabilities as well, allowing programs to run on Windows, Linux, and macOS without significant changes to the codebase.
- Interoperability: C# seamlessly integrates with various technologies. For instance, a C program can interact with Java libraries through interoperability services.
- Memory Management: The garbage collector takes care of memory allocation and deallocation, freeing developers from the tedious task of managing memory. This automatic handle on memory not only reduces bugs but also enhances performance.
- Rich Library Support: The .NET library is vast, offering anything from simple data manipulation to complex algorithms right out of the box, which accelerates the development process.
"C# is not just a programming language; itâs an ecosystem that fosters creativity and innovation."
Historical Context and Evolution
C# first emerged in the early 2000s, developed by Microsoft as part of its .NET initiative. The language was aimed at addressing the evolving landscape of programming views, simplifying tasks while providing robust features. The evolution of C has been closely tied to the advancements within the .NET framework itself.
In the beginning, C# found its identity as a strong competitor against Java and other languages. Its simplicity, combined with speed and efficiency, made it a choice for many developers transitioning into Windows application development. Over the years, continuous enhancements have introduced features like LINQ, async/await, and improved support for functional programming.
The most significant shift came with the launch of .NET Core in 2016, marking C#'s transition into an open-source arena. This broadened its user base and led to a surge of community involvement in its development. As of now, C# stands strong with annual updates and growing support from a dedicated community, making it a relevant choice for emerging technology trends.
In summary, understanding the foundations and evolution of C# helps appreciate the language's capabilities. By equipping oneself with this knowledge, developers are well-prepared to utilize C effectively in a variety of applications.
Setting Up Your Development Environment
Setting up your development environment is like laying a solid foundation for a house; without it, your program might crumble before it even begins. In C# programming, the right tools are critical for maximizing efficiency and productivity. These tools not only streamline your workflow but also enhance your coding experience. By thoughtfully selecting your integrated development environment (IDE) and correctly installing the necessary frameworks, you set the stage for a smoother coding journey ahead.
Having the appropriate environment allows you to avoid unnecessary hiccups during coding and testing phases and can be the difference between a simplified project or a tangled mess. A well-configured setup can boost your motivation and keep you engaged as you delve further into C# development.
Choosing the Right IDE
Visual Studio
Visual Studio stands out as one of the most robust IDEs available for C#. Itâs a powerful tool that boasts a plethora of features designed to cater to both novice programmers and seasoned pros alike. One might say it is the Swiss Army knife of C# development due to its versatility and extensive functionalities. The key characteristic of Visual Studio is its integrated debugging tools, which allows developers to troubleshoot and optimize their code seamlessly. This environment offers built-in support for unit testing, making it a favorite choice for many who desire to ensure their code is not just functional but reliable.
However, while Visual Studioâs feature set is impressive, it can demand significant system resources. This means that on a lower-end machine, it could potentially run slower, which is a disadvantage for those who are working with limited hardware.
Visual Studio Code
Visual Studio Code (VS Code) has garnered a massive following due to its lightweight design and flexibility. It's a free code editor that supports various programming languages, including C#, thanks to its extensive library of extensions. One of its standout traits is the live server feature, enabling real-time updatesâan attribute that can significantly enhance the development process.
Since it is less resource-intensive than Visual Studio, VS Code is a preferred choice for developers working on less powerful machines or those who appreciate a minimalist setup. Despite its advantages, VS Code might need some initial effort in terms of configuration, as it often requires setting up extensions for a best-in-class C# experience.
JetBrains Rider
JetBrains Rider is an IDE that blends powerful functionality with a more agile environment. Known for its intelligent code completion, Rider dramatically enhances productivity. Its refactoring capabilities are top-notch, allowing for quick changes with minimal hassle.
Rider's performance when working with .NET frameworks is commendable, often making it a favored option among developers who dive deeply into enterprise-level software. The downside? Itâs a paid tool. While it comes with a rich set of features, some developers might hesitate to invest without first understanding if it fits their specific needs.
Installing the .NET Framework
After choosing your IDE, the next pressing issue is installing the .NET Framework. This framework is the backbone of your C# applications. To utilize the full capabilities of C#, you need to ensure the .NET Framework is installed properly. Here are a few points to consider:
- Compatibility: Ensure that your IDE supports the version of .NET you plan to work with. Version mismatches can lead to unforeseen issues down the line.
- Updates: Regularly check for updates on the .NET Framework to leverage new features and critical security patches.
- Installation Guide: Follow the official installation guidelines to avoid common pitfalls. An incorrect setup can lead to configuration headaches later!
In summary, setting up your development environment is an essential first step in your C# programming journey. By meticulously selecting the right tools, you create a conducive atmosphere for productive coding that can only pave the way for success in your programming endeavors.
"Programming isn't about what you know; it's about what you can figure out."
Basic Syntax and Structure of
Programs
Understanding the basic syntax and structure of C# programs is vital for anyone looking to develop effective software applications. Syntax refers to the rules that dictate how code must be written to be understood by the compiler, while the structure deals with how the code is organized. Getting a grip on these elements ensures your code is not only functional but also readable and maintainable. In the end, clarity in syntax and structure lays the blanket for better programming practices and easier debugging.
Understanding Variables and Data Types
Variables and data types are foundational concepts in C#. They serve as building blocks for creating more complex structures and logic within your programs. Variables can be thought of as containers for storing data, and different types specify how that data can be used.
Primitive Types
Primitive types in C# include integers, booleans, characters, and floating-point numbers. Each type represents a specific kind of data and is essential for performance and memory management. For instance, an integer uses less memory than a string, making it a popular choice for numerical calculations. Whatâs unique about primitive types is their straightforward declaration and use, which makes them incredibly approachable for beginners.
Key Characteristic: They are the simplest and most directly usable types in C#. Their straightforwardness often translates into faster performance in computations.
Advantages: Using primitive types minimizes memory usage and maximizes speed in execution, which is a significant consideration when performance matters. However, they lack the complexity needed to handle more intricate data structures, such as collections.
Reference Types
On the flip side, C# also offers reference types like strings, arrays, and classes. Reference types store references to the actual data rather than the data itself. This can be beneficial when you need to manipulate complex data structures or when you want to include operations that can alter the data.
Key Characteristic: Unlike primitive types, reference types can hold more complex data and support methods that can be used to manipulate the contained data.
Advantages: They allow for richer data representations and are especially useful in object-oriented programming, where encapsulation is key. However, because they function through references, memory management can become trickier, and issues like null references might arise.
Control Flow Statements
Control flow statements in C# manage the direction in which the program executes statements, allowing for conditional execution and loops. They are critical for managing the flow of the program logic, ensuring that the application behaves as intended.
if Statements
If statements present a way to execute code based on certain conditions. A vital component of control flow, they enable the creation of branches in logic, directing the program to different paths depending on whether certain conditions are met.
Key Characteristic: The ability to check conditions dynamically means that your program can adapt to various inputs and scenarios.
Advantages: They simplify decision-making processes in code but can lead to complexity if overused or nested deeply.
Switch Cases
Switch cases provide an alternative to if statements. This structure can make your code cleaner and more readable when dealing with multiple conditions that depend on the same variable. You could think of it as a more organized way to express decision-making.
Key Characteristic: They allow for multiple discrete cases to be evaluated rather than using multiple if statements, so they can make the code more compact and understandable.
Advantages: Utilizing switch cases can lead to streamlined code, but they are limited to single variable evaluations, which might restrict flexibility in more complex decision-making scenarios.
Loops
Loops are powerful constructs that allow the repetition of a block of code until a specified condition is met. This becomes crucial when dealing with repetitive tasks or when processing collections of data.
Key Characteristic: Loops such as , , and offer different mechanisms for iteration, each useful in different scenarios.
Advantages: They enable automation of repetitive tasks, but poor use of loops can lead to infinite executions or performance issues. Proper management is essential to avoid pitfalls in your code.
By comprehending C#âs basic syntax and structure, a developer lays down essential groundwork to more complex programming concepts.
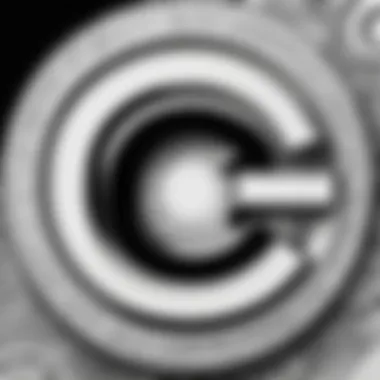
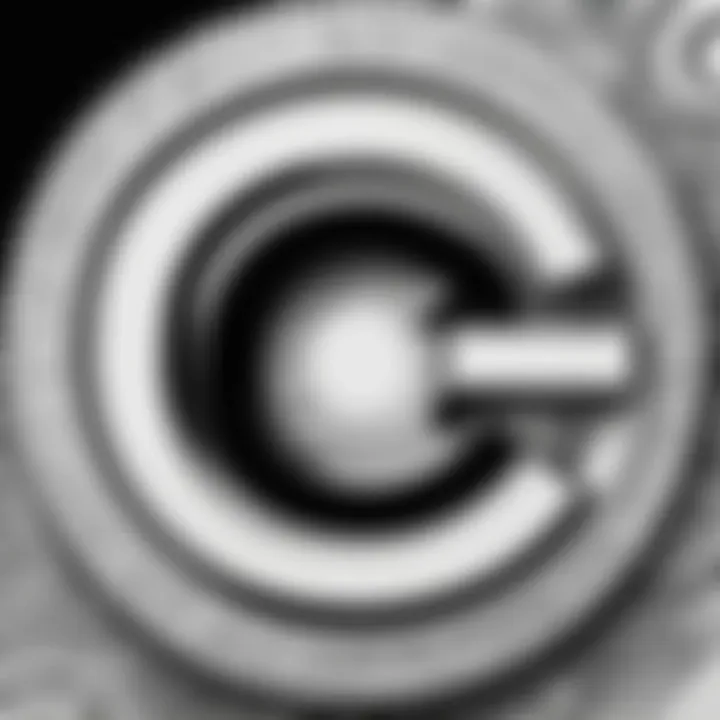
In summary, mastering these fundamental aspects of C# will not only enhance your coding proficiency but also pave the way for future learning and applications, allowing you to navigate more sophisticated programming challenges with confidence.
Writing Your First
Program
Getting your feet wet with C# programming is one of the most thrilling parts of learning this language. Itâs like stepping onto the dive board for the first time, looking out at the water, and feeling that mix of excitement and trepidation. In this section, weâll go through the steps to not just write your first program but also set a solid foundation for your coding journey. Being able to write and execute a program ignites a spark of understanding about how computer programs really work, and from here, the sky is the limit.
Creating a Simple Application
The first hurdle to conquer is crafting a simple application. While it may seem straightforward, this task is crucial in understanding the basic components of a C# program. Letâs kick things off with a little code snippet:
In this sample code, we have declared a class named , and within it, a method called . This method is the entry point for any C# programâbasically where the magic begins. The statement outputs text to the console, which is incredibly important since it allows you to see the results of your programâs execution.
Before you run off to test it, letâs digest some elements that make this such a great start:
- Namespaces: The directive means we can access classes in the System namespace, like .
- Class Structure: Everything in C# is encapsulated within classes. Itâs a fundamental concept of object-oriented programming.
- Method Execution: To execute code, it must be in a method; here, itâs wrapped up in .
This simple program not only helps you see immediate results, but sets the stage for more complex applications. Youâre not just typing away in a vacuum; every line of code begins to paint a clearer picture of your applicationâs workings.
Compiling and Running the Program
After crafting your first application, the next step is compiling and running it. This steps acts as a bridge between your written code and the actual execution of that code. When you compile a C# program, you convert your source code into an intermediate language, which the .NET runtime can interpret.
Hereâs how you typically go about this process:
- Open your IDE: If youâve chosen Visual Studio, launch it and open your created file.
- Build the Project: Hit or navigate through the menu to build the project. This process checks for any errors and compiles your code.
- Run the Program: After a successful build, you can start the program by pressing or clicking the green play button.
- View Results: The console will pop up, and you should see âHello, World!â printed on the screen.
This clear cycle of writing, compiling, and executing isnât just about making code work; itâs about fostering an intuitive grasp of how programming flows. đŻ
Key Takeaway: Writing and executing your first program serves as a critical stepping stone in programming. It builds confidence and encourages continuous learning. With this trajectory, you not only understand the foundational skills but also are ready to dive deeper into more intricate programming concepts as you progress.
So there you have it, a gateway into the world of C#. Take each piece of knowledge youâve uncovered and turn it into a stepping stone for bigger projects ahead. This is just the tip of the iceberg!
Object-Oriented Programming in
Object-Oriented Programming (OOP) has become a cornerstone of C# programming, allowing developers to create modular, reusable, and maintainable code. At its core, OOP encourages structuring software around the real-world problems it seeks to solve. This paradigm offers several benefits that can greatly enhance the developer's efficiency and the application's performance. By leveraging concepts such as classes and objects, inheritance, polymorphism, encapsulation, and abstraction, C programmers can build robust applications that can adapt to changing requirements with minimal frustration.
Understanding OOP allows developers to break down complex systems into manageable parts. It encourages a higher level of abstraction, transforming intricate relationships and behaviors into well-defined objects. As such, each object can manage its own state and behavior, which is prudent for avoiding conflict in larger codebases.
Furthermore, OOP promotes code reuse. Once a class has been defined, it can serve as a template for creating multiple objects with similar behaviors without reinventing the wheel. This not only saves time but also assures consistency across the codebase. These features make it easier to maintain and update the code since changes made to a class are reflected in all instances of that class.
"Object-oriented programming is an extremely bad idea which has survived because the programmers who dislike it are secretly doing it anyway."
â Lars Bak
Classes and Objects
In the realm of C#, classes and objects are the building blocks of OOP. A class serves as a blueprint or template from which objects are created. It encapsulates data for the object and methods to manipulate that data. For example:
In this example, the class has two properties: and , along with a method called . From this class, various objects can be instantiated, each with unique values for and . This instance-level encapsulation is both simple and effective, and it exemplifies the power of OOP.
Inheritance and Polymorphism
Inheritance is a fundamental aspect of OOP that allows a class to inherit characteristics and behaviors from another class. This hierarchy lets developers build upon existing classes without having to recreate code. For example, if you have a general class called , you can create a class that inherits from , gaining its properties and methods, while also adding specific features for cars.
Next, polymorphism allows for methods to do different things based on the object that invokes them. This means that the same operation can behave differently depending on the object's actual derived types. By leveraging polymorphism, we can achieve more flexible and dynamic code.
Encapsulation and Abstraction
Encapsulation refers to the practice of restricting access to the internal state of an object. This means that the data contained within an object cannot be modified directly; instead, it must be accessed and changed through methods defined within the class. This practice helps to prevent unintended interference and misuse of data, ensuring that it remains consistent and reliable.
Abstraction, in contrast, involves hiding complex realities while exposing only the necessary parts of an object. The idea is to simplify interactions with complex systems. In C#, interfaces and abstract classes serve as the primary tools for abstraction, allowing developers to define methods that derived classes must implement, without detailing how those methods must function.
In summary, understanding the principles of OOP in C# is crucial for any developer. It not only establishes a framework for organizing and managing code but also enhances collaboration and code maintainability. The principles of classes and objects, coupled with the concepts of inheritance, polymorphism, encapsulation, and abstraction, empower developers to build flexible software architectures that stand the test of time.
Advanced
Features
Exploring advanced features of C# is not just an academic exercise; it's an essential part of becoming a proficient developer. These features enable developers to write more efficient, maintainable, and powerful applications. Understanding these concepts enhances oneâs ability to tackle complex problems, thus providing more value in their work.
Beyond basic syntax, advanced C# features like delegates, events, and Language Integrated Query (LINQ) play a critical role in modern software development, allowing for better handling of asynchronous operations, seamless event management, and easy data manipulation. Utilizing these features can streamline workflows, reduce code redundancy, and boost application performance.
Delegates and Events
Delegates in C# can be thought of as pointers to functions. They provide a way to encapsulate method references, allowing methods to be passed as parameters. This flexibility is crucial when designing event-driven programs. Here's a quick breakdown of their significance:
- Decoupling: Using delegates helps separate the logic in your program. This way, classes can communicate without tightly coupling one another.
- Event Handling: Delegates are the foundation for events in C#. An event can be subscribed to by multiple methods, revealing a publish-subscribe model, which is quite handy in many applications.
For example, consider an event when a button is clicked in a user interface. Rather than hardcoding the response, you can simply define a delegate type and let other methods subscribe to that event:
This approach allows you to have a clean separation of concerns within your code, making it easier to maintain and extend.
LINQ (Language Integrated Query)
LINQ is a powerful querying language integrated directly within C#. It allows developers to perform queries directly on collections like arrays or lists using a SQL-like syntax. Here's why LINQ is important:
- Code Clarity: LINQ dramatically enhances the readability of the code. Rather than using loops and conditions, you can articulate your intentions more clearly. For instance, filtering data becomes succinct:
- Data Manipulation: LINQ enables seamless data handling, whether you're interacting with a database or working with in-memory arrays.
- Consistency: Using LINQ promotes a consistent querying experience across different data sources, be it XML, SQL databases, or even in-memory collections.
In summary, mastering advanced C# features such as delegates, events, and LINQ not only elevates a programmerâs skill set but also leads to building more responsive, maintainable, and robust applications. Embracing these tools opens up a world of possibilities for efficiency and creativity in code.
Working with Databases
Working with databases is a cornerstone in the world of software development. It plays an essential role in how applications manage and utilize data. As programs grow in complexity, so do their needs for efficient data storage and management. This section will delve into the art of connecting to databases and executing SQL queries in C#. Understanding these concepts not only strengthens your coding skills but also enhances your ability to create robust applications that leverage stored data effectively.
Connecting to a Database
When you want your C# application to interact with a database, establishing a connection is the first critical step. Several options exist to accomplish this depending on the type of database you are dealing with. Popular relational databases, like Microsoft SQL Server or MySQL, use a common standard called ADO.NET. This allows for a straightforward way to connect to the database.
To create a connection, you typically need a connection string containing details like server address, database name, user credentials, and other parameters to access the database. For instance:
After defining your connection string, the next step is to utilize it with the class. This step ensures that your program can communicate with the database. For example:
This code snippets showcase how to safely open a database connection, where the statement ensures that the connection is properly closed after its use. Knowing how to manage connections effectively helps in maintaining application performance.
Executing SQL Queries
Once you have your connection in place, the real magic happens when you start executing SQL queries. This aspect is crucial for data manipulation, whether you're reading, inserting, updating, or deleting records in your database. C# provides great support for executing these queries through ADO.NET.
Executing a SELECT statement can be easily done using the SqlCommand class. Hereâs a simple example to fetch data:
This snippet demonstrates how to retrieve data and read through it with a SqlDataReader. Each record fetched can be accessed column by column, which opens up numerous possibilities for application functionality.
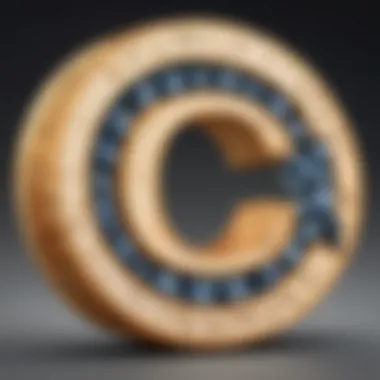
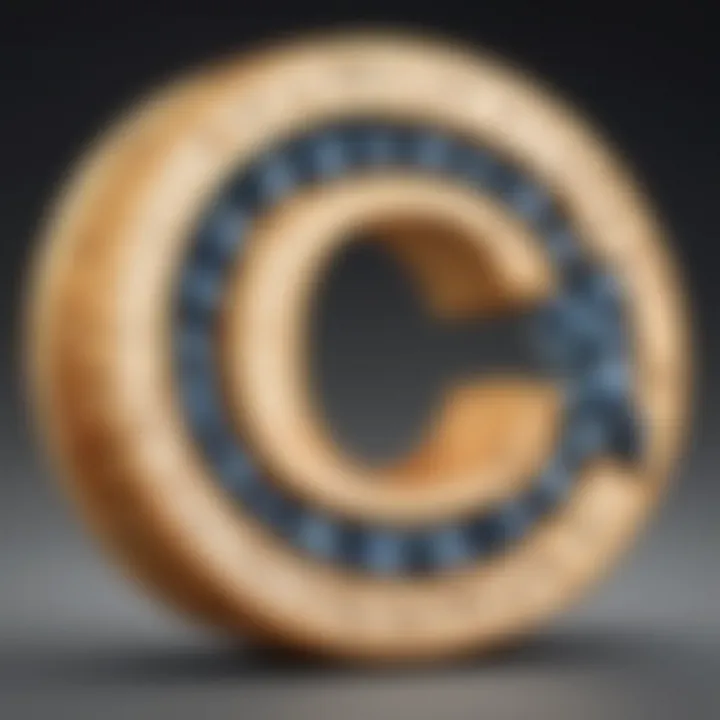
Moreover, executing SQL queries isnât limited to just retrieving data. You can also use for commands that alter data, like so:
This capability to manipulate data dynamically based on application logic can vastly improve the interactivity of applications you're creating.
"Efficient database manipulation is the backbone of application scalability. Mastering it can set you apart in the software development arena."
In summary, connecting to a database and executing SQL queries are fundamental skills for C# programmers. By grasping these practices, developers can create proficient applications that manage and utilize data effectively. Mastery of this topic not only enhances your understanding but also opens numerous doors to implementing data-driven solutions in your projects.
Testing and Debugging
Applications
Testing and debugging are pillars of software development, particularly when working with C#. These processes not only ensure that applications run smoothly, but they also enhance the code's reliability and maintainability. One can't just dive in and expect every line of code to work perfectly on the first try. Bugs, like pesky mosquitoes in summer, seem to pop up unexpectedly, and thatâs where testing and debugging come into play.
Importance of Testing
Testing serves as a safety net. It helps identify potential issues before they reach the end-user. If you write a small piece of code without some form of testing, you might end up spending hours trying to figure out what went wrongâonly to realize you missed a semicolon or had a variable name mixed up. How frustrating is that?
A well-thought-out testing strategy allows developers to pinpoint errors early in the development cycle, which reduces time spent on debugging later. The benefits of testing are numerous:
- Improved code quality: Thorough tests lead to cleaner, more understandable code.
- Easier maintenance: Well-tested code is easier to modify without introducing new bugs.
- Enhanced user satisfaction: Fewer bugs mean happier users.
Importance of Debugging
Debugging, on the other hand, digs a bit deeper into understanding why a program behaves in a certain manner. When any applications throw their tantrums, debugging steps in to investigate. The act of debugging involves analyzing code, stepping through it line by line, and considering edge cases to ensure every nook and cranny has been considered. This makes it fundamental for developers:
- Detecting logical flaws: Bugs can lurk in subtler cases than missing semicolons. Debugging helps weed out those sneaky errors.
- Understanding program flow: It helps you follow the path your code takes, giving deeper insights into logic and structure.
- Quick problem resolution: The quicker you understand a problem, the faster the fix can be applied.
By embracing both testing and debugging, developers can assure that their C# applications work under the best possible conditions, enhancing performance while minimizing user-facing issues.
Unit Testing with NUnit
Unit testing is one of the most effective ways to validate your code. NUnit is a popular testing framework specifically designed for C#. It allows developers to write tests for small "units" of code in isolation, ensuring each part returns the expected results. When writing unit tests with NUnit, you have the flexibility to create tests that are easy to read and maintain.
To get started with NUnit, hereâs a simple example:
In the above code snippet, you can see how clearly the tests are structured. They are composed of three main parts: Arrange, Act, and Assert. The beauty of this framework lies in the simplicity and clarity it provides, making it an excellent choice for both new and seasoned developers.
Debugging Techniques
So you've written your code, run tests, and boom! An unexpected error occurs. What now? Debugging techniques allow you to systematically address these hiccups. Here are some essential debugging techniques every developer should be familiar with:
- Breakpoints: Setting breakpoints in your code allows you to halt execution and inspect variable states at specific points. This gives you a better grasp of what is going on as the program executes.
- Step-through debugging: This allows you to run your application's code line-by-line, helping to locate the precise line where things go awry.
- Logging: Itâs a good practice to add logging throughout your application. Being able to write logs can greatly assist in figuring out what happens before and after a bug occurs.
- Try/Catch Statements: Not everything goes as planned, and catching exceptions can help prevent application crashes and provide insights into where a failure may be occurring.
Debugging may not be the most glamorous part of coding, but itâs undeniably essential. A seamless experience and efficient code rely on your ability to identify and resolve issues swiftly.
The neatest code can crawl along if not properly tested and debugged. Taking time for these processes isn't just a good idea â it's vital in the realm of software development.
By implementing these testing and debugging techniques and tools, you are not only safeguarding your application but also crafting a better experience for the end user. Itâs a win-win!
Developing User Interfaces
Creating user interfaces is an essential part of software development, particularly in today's world where user experience can make or break an application. The ability to design and develop interfaces that are not only functional but also visually appealing is crucial. A well-thought-out UI allows for smoother interactions, minimizes user errors, and provides valuable feedback. When diving into C#, developers have robust tools at their disposal to bring their ideas to life, whether they're crafting a simple app or a more complex system.
Easy navigation and clear layouts can significantly improve user satisfaction. Design choices such as colors, button placements, and typography all contribute to a user-friendly experience. Developers must consider accessibility, so all users, including those with disabilities, can effectively use the software.
Understanding Windows Forms
Windows Forms is part of the .NET Framework, and it serves as a great starting point for users getting into UI design with C#. It caters primarily to desktop applications. Hereâs why it matters:
- Rapid Development: Windows Forms provides a straightforward way to build desktop applications, allowing developers to drag and drop components, which speeds up the design process.
- Event-Driven Model: This model allows the user to influence the application's flow based on actions like clicks or key presses, providing a dynamic interaction experience.
- Rich Controls: It comes with a variety of pre-built controls like buttons, text boxes, and labels which can be easily customized.
Using Windows Forms isn't just about functionality; it often involves balancing aesthetics with performance. For instance, heavy graphics might improve the visual aspect but could slow down the application. For those starting with C#, Windows Forms is a fitting territory to explore, but eventually, all programmers will find their way to more modern frameworks.
Intro to WPF
Windows Presentation Foundation, commonly referred to as WPF, presents a distinctive way of creating UIs in C#. Unlike Windows Forms, WPF harnesses the power of XAML (eXtensible Application Markup Language), coupling a rich design experience with advanced features.
- 3D Graphics and Animation: While Windows Forms primarily uses 2D design, WPF allows for complex graphics, from simple shapes to intricate animations. This opens up a new dimension of creativity.
- Data Binding: Developers can bind UI elements directly to data sources, providing a responsive interface that updates in real-time as data changes. This means less manual updating and enhanced overall performance.
- Styles and Templates: WPF encourages the use of styles and templates to maintain consistency across the applicationâs visual elements. This promotes a cleaner codebase and easier maintenance.
"In crafting user interfaces, ease of use defines success. The more intuitive the design, the greater the engagement."
Both Windows Forms and WPF come with their intricacies and considerations, but mastering them can significantly enhance a developer's toolkit. Diving deep into user interface design isn't just about aesthetics. Both function and form are crucial in aligning with a user's expectations and needs.
Exploring .NET Core and ASP.NET
The realm of C# programming finds significant value in understanding .NET Core and ASP.NET. These frameworks not only streamline development processes but also offer developers the flexibility to create various applications ranging from desktop software to complex web solutions. By exploring these technologies, programmers can harness their capabilities to meet modern development demands effectively.
Differences between .NET Framework and .NET Core
When it comes to choosing between .NET Framework and .NET Core, it's crucial to understand the distinctions based on various aspects. .NET Framework has been around for many years, primarily focused on Windows applications. It's deeply integrated with the Windows operating system, making it a go-to for traditional desktop applications. However, it is not cross-platform, limiting its usability across different systems.
On the other hand, .NET Core stands out with its cross-platform capabilities. This means developers can run their applications on Windows, macOS, and Linux. Some key differences include:
- Operating System Compatibility:
- Performance:
- Deployment:
- .NET Framework: Restricted to Windows.
- .NET Core: Multi-platform, supporting Windows, macOS, and Linux.
- .NET Framework: Generally slower due to its monolithic structure.
- .NET Core: Optimized for performance with a modular architecture.
- .NET Framework: Requires full framework installation on the target machine.
- .NET Core: Self-contained deployments available, simplifying deployment and versioning.
"Choosing .NET Core aids in building high-performance, scalable applications for diverse environments."
Understanding these differences is pivotal for developers looking to leverage the strengths of each framework for their projects.
Building Web Applications with ASP.NET
ASP.NET is a framework that revolutionizes web application development. It empowers developers to create robust and dynamic websites, web services, and applications. This technology offers a plethora of features that enhance development efficiency and improve the user experience.
One of the standout features of ASP.NET is its rich set of tools and libraries that facilitate rapid development. Here are some highlights:
- MVC (Model-View-Controller) Architecture:
- Web API:
- Razor Pages:
- Encourages a clean separation of concerns, allowing for easier maintenance and scalability.
- Enables creating RESTful services, making it easier to build service-oriented architectures and integrate with other applications.
- Simplifies page-focused scenarios, allowing developers to write less code and focus on the logic of the page rather than the framework.
Additionally, with the support of modern development practices such as Dependency Injection, ASP.NET fosters a more modular approach to web application development.
Overall, diving into ASP.NET equips developers with the tools needed to tackle todayâs web challenges while enhancing their skill set in the fast-evolving tech landscape.
Best Practices in
Development
In the realm of C# programming, adhering to best practices is like having a well-tended garden. It ensures that your code not only flourishes but also is easier to maintain and adapt over time. With a solid grasp of these principles, developers can significantly improve the reliability and clarity of their applications, thus fostering a healthier coding environment.
Best practices in C# development pave the way for increased efficiency, reduced errors, and clearer communication within the team. Itâs about setting a standard that everyone follows, providing a unified approach to writing and maintaining code. Thereâs something to be said about following a roadmap laid out by experienced developers; it leads to fewer headaches down the line and avoids the infamous rabbit hole of debugging.
Several elements stand out when discussing these best practices:
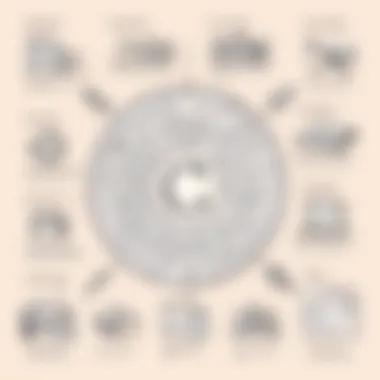
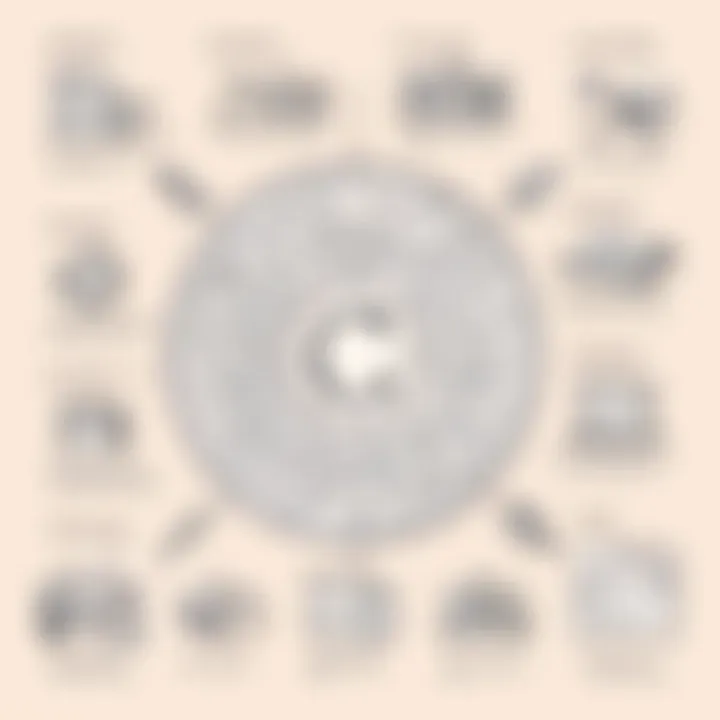
- Code Readability: Writing code that others can read easily isnât just a nice-to-have; itâs essential. Clear, descriptive naming conventions make it easy to decipher the purpose of a variable or function at a glance.
- Consistency: Adopting a uniform style across your codebase helps in reducing the cognitive load on developers. Whether itâs naming conventions or code structure, staying consistent breeds familiarity.
- Commenting Wisely: Comments serve as a guide but should not be overused. A well-named function should be clear enough that the comment "this function adds two numbers" is redundant. Use comments sparingly and for complex logic that needs more explanation.
- Embrace Version Control: A version control system like Git isnât just a tool for code history; itâs an indispensable part of the development process, facilitating collaboration and tracking changes effectively.
In summary, embracing best practices in C# isnât merely about following rules; itâs about fostering an environment conducive to learning, sharing, and improving. Developers who prioritize these elements are positioning themselves for success, both individually and as part of a team.
Code Readability and Maintenance
Code readability is often the cornerstone of effective programming. When writing a C# program, itâs crucial to create code that is intuitive for others (and for yourself in the future). The aim is to write code that communicates its design and intent clearly.
A few key points regarding code readability include:
- Descriptive Naming: Rather than using vague names like or , use clear names, such as or . Itâs like naming a child; you wouldnât want to give them a name that confuses others, right?
- Indention and Spacing: Proper formatting makes it easier to analyze blocks of code. Just like how breathing room in a room creates comfort, accurate indentation and spacing provide clarity.
- Refactoring: Donât hesitate to refine your code. If you find a chunk of code that seems messy or overly complex, itâs better to refactor it than to leave it as is. Good code is like a well-organized closet.
Maintaining readable code simplifies future adjustments. If someone (or you) needs to revisit it later for changes, they should be able to grasp the logic and flow without digging through layers of confusion.
Version Control with Git
Version control, especially with a system like Git, is absolutely essential for any serious development endeavor. This is like having a time machine for your codeâallowing you to navigate through the history of your project.
Here are a few benefits and considerations linked to version control:
- Change Tracking: Git allows developers to keep track of modifications made to the code over time, pinpointing when and why changes occurred. Itâs invaluable for understanding how your code has evolved.
- Collaboration: Multiple developers can work on different features simultaneously without code clashes, preserving sanity in the development process. Working together on a project can easily become a thorny mess without clear version control.
- Branching: Git empowers developers to work on several features simultaneously through branchesâfrom refining a function to launching a new feature. It's a bit like having separate paths in a wood; you can explore one without losing sight of the others.
Remember, maintaining readable code and using version control isnât just about good habits; itâs about laying a foundation for your future projects.
Performance Optimization in
When it comes to developing applications in C#, performance optimization is not just an afterthought; it's a necessity. As software systems grow, the need for speed and efficiency becomes paramount. Not only does it enhance the user experience, but it also reduces operating costs and resource consumption. Therefore, understanding performance optimization is crucial for developers, especially as they strive to create scalable, efficient, and high-performing programs.
Key Benefits of Performance Optimization:
- Improved Response Times: Applications that run faster keep users engaged and satisfied.
- Resource Efficiency: Optimized programs require less memory and CPU usage, extending the life of hardware.
- Scalability: Well-optimized code can handle increased loads without crashing or slowing down.
- Financial Savings: Reducing resource consumption can lead to lower costs in cloud services or other infrastructure.
Considerations in Performance Optimization:
- Trade-offs: Sometimes, optimizing one area can compromise another. Balancing speed with maintainability is a fine line.
- Profiling Tools: Using instruments to identify areas that need improvement is essential for effective optimization.
- Iterative Process: Optimization should happen iteratively, with consistent testing and assessment to gauge performance improvements.
Understanding these elements lays a solid foundation for delving into the specifics of performance optimization techniques and practices in C#.
Deploying
Applications
In the modern software landscape, getting your application from concept to user is just as crucial as the programming itself. Deploying C# applications is a pivotal aspect of the software development lifecycle. The deployment process not only ensures that your application is accessible to users but also impacts performance, scalability, and user satisfaction. Thus, mastering this process can give developers a significant edge in their projects.
When we talk about deployment, itâs not merely about flipping a switch and making your program live. There are critical elements and considerations that need attention. Here are some key elements to keep in mind:
- Environment Configuration: Understanding various environments (development, testing, production) and how your application behaves in these settings is vital. You might configure things differently in a test environment than you would in production.
- User Requirements: Knowing your audience dictates how you deploy. Think about where your users obtain your software, how they access updates, and the platforms they use.
- Performance Monitoring: After deploying, monitoring your application's performance is essential. It allows you to catch issues early and understand user behavior.
In essence, well-planned deployment leads to smoother rollouts, fewer bugs, and a better overall user experience.
Understanding Deployment Processes
To dive deeper, the deployment processes of a C# application involve several steps that can greatly influence its success. These steps include:
- Building the Application: This first step involves compiling your C# code into an executable format. Tools like MSBuild can help streamline this process, ensuring that your application is optimized for performance.
- Testing: Before any deployment, rigorous testing must be conducted to ensure that everything runs as expected. Unit tests and integration tests can be performed, detecting errors beforehand.
- Packaging: Once your application is tested and ready, it needs to be packaged appropriately. This might involve creating installers or zip files that can be easily distributed.
- Deployment: Here comes the moment of truth. Whether youâre deploying to a cloud server, on-premises infrastructure, or distributing to clients, each has its best practices you should follow. Typically, this step involves uploading your application files to the target server or passing the executable files to users.
- Post-Deployment Verification: After deployment, itâs critical to confirm that the application is working as intended. This involves checking all functionalities, performance, and error logs to ensure everything goes smoothly.
Each of these steps plays a crucial role in the overall deployment process, helping minimize risks and maintaining application integrity.
Continuous Integration and Delivery
The concepts of Continuous Integration (CI) and Continuous Delivery (CD) are game-changers in modern software development. CI/CD practices help streamline and automate the deployment pipeline, reducing manual effort and increasing consistency.
- Continuous Integration involves the practice of frequently integrating code into a shared repository. Every change is automatically built and tested, spotting issues early. This means that as a developer, you can focus on coding while the system checks for bugs and integration issues.
- Continuous Delivery takes it a step further by ensuring that your application can be deployed to production at any time. Automating the deployment process means that developers can release updates more frequently and reliably. It can significantly speed up the market entry of new features and fixes.
Implementing CI/CD in your deployment strategy can lead to increased efficiency, better quality of software, and, ultimately, a happier user base. As you integrate these practices into your workflow, youâll likely find that not only does project management become easier, but also the collaboration among team members improves drastically.
In summary, deploying C# applications is not just a technical step; it is an art that blends strategic planning with technical skills. Understanding deployment processes and adopting CI/CD practices can transform your development cycle, leading to more reliable and robust applications.
Resources for Further Learning
In the realm of C# programming, possessing foundational knowledge is merely the starting line. Considering the constantly evolving landscape of technology, ongoing education becomes imperative. Thatâs where additional resources come into play, allowing developers to stay sharp and informed. These resources can supercharge your learning and ensure youâre well-equipped to tackle any programming challenge. Whether you are a novice fumbling through your first lines of code or a seasoned developer honing your craft, having access to reliable resources can propel your skills to new heights.
One glaring benefit of exploring further learning resources is the ability to gain insights from varied perspectives. Books, online courses, forums, and community engagement expose you to a wealth of knowledge that is often not found in traditional educational settings. You may find tutorials that dissect complex principles or let you see what others are doing, sharing relevant experience that you hadnât considered. With the fast-changing technology environment, having multiple touchpoints can streamline your understanding, making it easier to grasp concepts that may initially seem complex.
Additionally, you should always keep in mind the importance of staying connected with your peers. There's immense value in engaging with fellow developers who share your appetite for knowledge. Their experiences can help shape your learning path and provide you insight into best practices, industry trends, and valuable tips that youâll find nowhere in textbooks.
Thereâs no denying that your growth as a C# programmer will flourish when you actively seek knowledge outside of your usual routines. Below, we shall explore some practical resources curated for your journey in mastering C programming.
Books and Online Courses
When it comes to books and online courses, the sheer volume of options can be daunting. A thorough examination of the best educational materials will pay off manifold. For books, consider titles such as:
- C# In Depth by Jon Skeet: This book digs deep into advanced C topics, providing a nuanced understanding of the language.
- Pro C# 9 with .NET 5 by Andrew Troelsen and Philip Japikse: A comprehensive guide for developers looking to explore core principles and practical implementations.
- The Pragmatic Programmer by Andrew Hunt and David Thomas: Though it covers programming in a broader context, it provides essential principles applicable to C# as well.
Aside from books, online learning platforms like Coursera and edX partner with universities to offer fantastic courses tailored to C#. A notable mention is the C# Programming for Unity Game Development course on Coursera, which blends C with game development, emphasizing real-world applications.
Furthermore, resources like Pluralsight and LinkedIn Learning provide an outstanding variety of video tutorials that cater to different skill levels. These courses often include exercises and assessments that can offer hands-on experience in a structured format.
Communities and Forums
Participating in communities and forums can provide you with a vibrant ecosystem of knowledge exchange. Websites such as Reddit are great platforms where you can join various subreddits, find threads, and connect with fellow C# developers.
- r/csharp: A subreddit specifically targeting C# questions, news, and resources.
- r/learnprogramming: While broader, this subreddit is excellent for beginners seeking guidance and tips.
In addition, Stack Overflow is a pivotal resource when youâre stuck solving a problem. Often, someone before you has encountered the same issue and posted their solution. Not only does this save time, but it also enriches your understanding by learning how others approach troubleshooting and problem-solving.
Lastly, joining local meetups or online groups can provide opportunities to network with professionals who might offer mentorship or collaboration in C# projects. Engaging in such communities fosters a sense of belonging, enhancing your educational journey by embedding you in an environment that thrives on collective knowledge.
"Surround yourself with people who are on the same path of learning, for they will empower you to reach your destination."
Ending
In the grand tapestry of C# programming, the conclusion serves as a vital stitch, weaving all the intricate threads of knowledge into a cohesive whole. It provides a space for the reader to step back and reflect on everything that has been discussed throughout the article. This moment of pause is essential not only to digest the information but also to contemplate its application in real-world scenarios.
A key element in this section is the recap of key concepts, which reinforces the learning journey undertaken. Itâs like taking a leisurely stroll through a gallery of ideas, revisiting the paintings that caught your eye, and understanding how each brushstrokeâbe it syntax, object-oriented principles, or the nuances of database handlingâcontributes to the overall masterpiece of programming in C#.
Furthermore, this is also a place to highlight the benefits of keeping abreast with C# advancements. The tech landscape shifts at a remarkable pace, and understanding where card is stacked today provides a critical roadmap for future learning, paving the way for adapting to the waves of change in the industry.
Considerations about this conclusion stretch beyond mere closure. It beckons the audience to not only implement what they have learned but also to stay curious and engaged with the C# community.
"The art of programming is not just writing code, it's about solving problems and constantly learning."
This resonates with the ethos of programming. Alongside technical skills, the life-long learning aspect is paramount in staying relevant and innovative.
In summation, the conclusion is more than a mere wrap-up; it's a call to action, urging developers and tech enthusiasts alike to carry forth the knowledge acquired and apply it in their respective quest for programming excellence. It stands as a reminder that the journey does not end here.
Recap of Key Concepts
As we step through the door of the recap, letâs quickly revisit the significant pillars established in the narrative:
- C# Language Fundamentals: Understanding the basic syntax, structure, and data types that form the backbone of programming in C#.
- Object-Oriented Principles: Gleaning insights into classes, objects, inheritance, and polymorphism which are essential for creating modular and reusable code.
- Handling Databases: The nuts and bolts of connecting, querying, and managing data effectively through ADO.NET and Entity Framework.
- Testing and Debugging: Learning how to ensure code quality and reliability through robust unit testing and effective debugging techniques.
- User Interface Development: The introduction to creating engaging applications using Windows Forms and WPF, ensuring user interaction remains a top priority.
- Performance Measures: Diving into optimization techniques, examining bottlenecks, and embracing asynchronous programming for efficient resource management.
Referring back to these concepts solidifies understanding, encourages coding practice, and highlights important aspects necessary for successful C# development.
Future Trends in
Development
C# programming is like a dynamic riverâever-flowing and continuously evolving to meet the needs of modern technology. Several trends are emerging that promise to reshape the landscape:
- Increased Adoption of .NET Core: More developers are leaning towards .NET Core for building cross-platform applications. This trend is bolstered by its flexibility and versatility, creating a smoother development experience across different environments.
- Asynchronous Programming: The need for handling numerous tasks simultaneously is only growing. Developers are leaning more towards asynchronous programming techniques, particularly with the advent of cloud computing and real-time applications.
- AI and Machine Learning Capabilities: C# is starting to incorporate more AI-driven functionalities, making it possible for developers to create intelligent applications capable of analyzing data patterns and predicting outcomes effectively.
- Rise of Blazor: The emergence of Blazor has reinvigorated web development in C#. Allowing developers to write client-side web applications with C# instead of JavaScript is a game changer, simplifying the tech stack.
- DevOps and Continuous Integration: With companies increasingly adopting DevOps practices, C# developers are engaging with tools that promote automated testing, deployment, and streamlined workflows.
Staying ahead of these trends is crucial for anyone serious about a career in C#. Keeping an eye on changes and emerging technologies will provide developers the edge they need to thrive in the fast-paced world of software development.