Mastering C++: Your Comprehensive Coding Guide
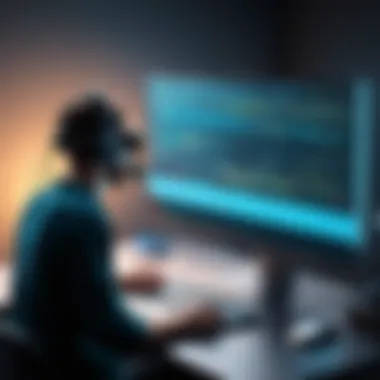
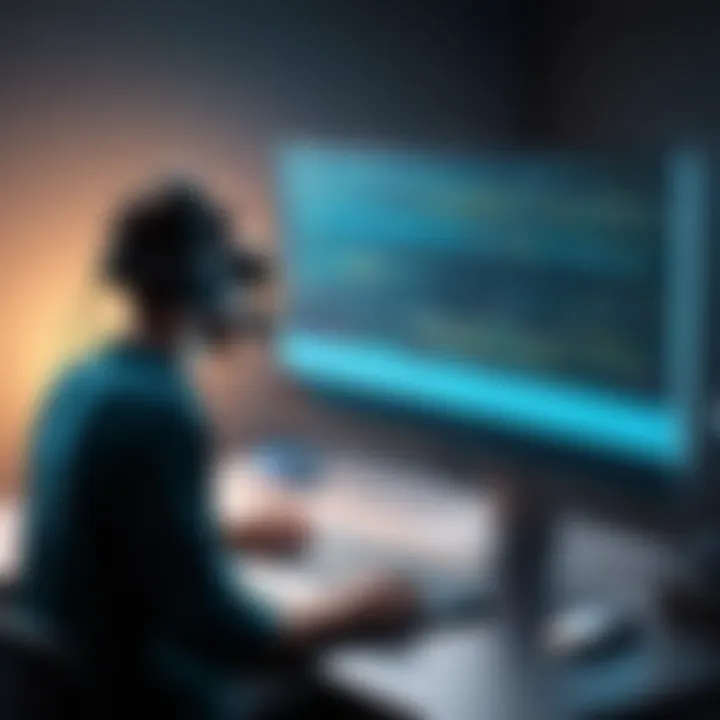
Intro
C++ emerges as a fundamental language deeply integrated into software development. As both a high-level and a low-level language, it provides the flexibility and control needed by various applications, from simple programs to complex systems. This guide seeks to uncover the complexities of C++, empowering programmers to fully embrace its extensive capabilities. It lays the groundwork for understanding key concepts and then advances towards sophisticated techniques, ensuring that people of different experience levels can benefit.
Overview of software development
Software development relies fundamentally on programming languages like C++. Programming plays a crucial role in creating applications that drive modern technology. C++ promotes efficient memory management, used in systems programming and high-performance applications. This language harmonizes object-oriented features with a multi-paradigm approach.
Definition and importance of ++
C++ is a powerful, versatile programming language designed for systems and application development. It holds importance due to its efficiency, speed, and value in programming environments.
Key features and functionalities
- Object-Oriented: Enables creating reusable code components.
- Standard Template Library: Provides pre-written algorithms and data structures.
- Compatibility with C: Allows integration with vast amounts of existing C code.
- Compile-time polymorphism: Enhances performance through templates.
Use cases and benefits
C++ functions well in various application domains such as:
- Operating systems (Windows, Linux)
- Game Development (Unreal Engine)
- High-Performance Computing (scientific simulations)
- Embedded Systems (automotive systems)
The versatility and control provided by C++ make it a go-to choice in environments where performance is critical.
Best Practices
To master C++, following industry best practices is key.
- Write manageable code: Break the code into smaller functions. It improves maintainability and helps debugging.
- Use smart pointers: This technique reduces memory leaks by managing the lifetime of dynamic objects efficiently.
- Consistent naming conventions: This small effort pays off in enhancing readability and collaboration in projects.
Industry best practices for implementing ++
- Minimize global variable usage.
- Favor composition over inheritance for flexibility.
- Leverage RAII principles for resource management.
Tips for maximizing efficiency and productivity
- Use profiling tools to understand bottlenecks in performance.
- Optimize compiler settings to fully leverage library features.
Common pitfalls to avoid
- Avoid premature optimization as it creates intricate code without clear benefits.
- Failing to handle exceptions leads to unstable software.
Case Studies
These examples highlight C++ in action.
Real-world examples of successful implementation
Google effectively uses C++ for its more extensive systems corresponding to performance. Similarly, Microsoft combines it with C# for critical infrastructure.
Lessons learned and outcomes achieved
Developers discovered that implementing smart pointers remarkably improved resource management, creating a dissociation from manual memory control hence decreasing errors.
Prolusion to ++ Programming
Understanding C++ programming is essential for any software developer today. This section discusses the fundamental aspects of the language, its development, key features, and its relevance in modern software projects. C++ is both a powerful and flexible choice, allowing programmers to craft efficient and high-performance applications.
History and Evolution of ++
C++ has a rich history, originating from the need to add object-oriented features to the programming language C. Developed by Bjarne Stroustrup in the late 1970s at Bell Labs, C++ was designed to provide enhancements to C, enabling better abstraction and encapsulation. The first edition of the C++ programming language was published in the early 1980s. Over time, different versions have emerged, like C++98, C++03, C++11, C++14, C++17, and most recently, C++20, each bringing enhancements and new features that improve the development experience.
C++'s evolution has kept pace with advancements in technology while adhering to core programming principles. This adaptability is a significant factor in its enduring popularity across using contexts, from systems programming to game development.
Key Features of ++
C++ offers a range of distinctive features that contribute to its robustness:
- Object-Oriented Programming (OOP): Supports concepts like inheritance, encapsulation, and polymorphism
- Performance: Provides low-level memory access, which enhances performance
- Standard Template Library (STL): Includes powerful tools for data structures and algorithms that aid efficiency
- Cross-Platform: Allows code to be run on various operating systems with minimal modifications
- Multi-paradigm: Supports various programming paradigms, including procedural and functional programming
These features make C++ an appealing choice for projects that prioritize performance and practicality without sacrificing the quality of code structure.
Importance of ++ in Modern Software Development
C++ plays a crucial role in software development today. Its ability to combine efficiency with high-level abstractions is a significant advantage for specialized areas, such as game development, embedded systems, and AI programming. Notable applications of C++ in modern development include:
- Game Engines: Tools like Unreal Engine leverage C++ for seamless performance
- Operating Systems: Generating highly efficient system-level applications where performance is paramount
- Hardware Programming: Working closely with hardware for resource-constrained environments
Setting Up Your Development Environment
Setting up your development environment is a critical step in mastering C++. A well-configured environment not only boosts productivity but also simplifies debugging and testing. When embarking on an endeavor to learn or improve skills in C++, the significance of choosing the right tools cannot be overstated. Everything begins from here—contrary to popular belief that coding is merely about writing lines of seemingly endless characters, a suitable development space cultivates organization and efficiency.
Choosing the Right IDE
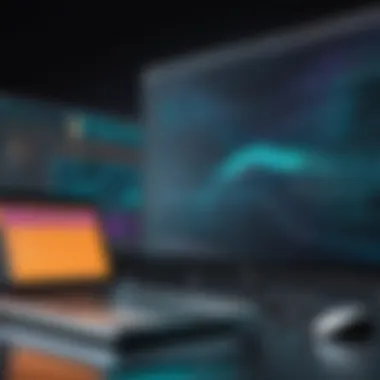
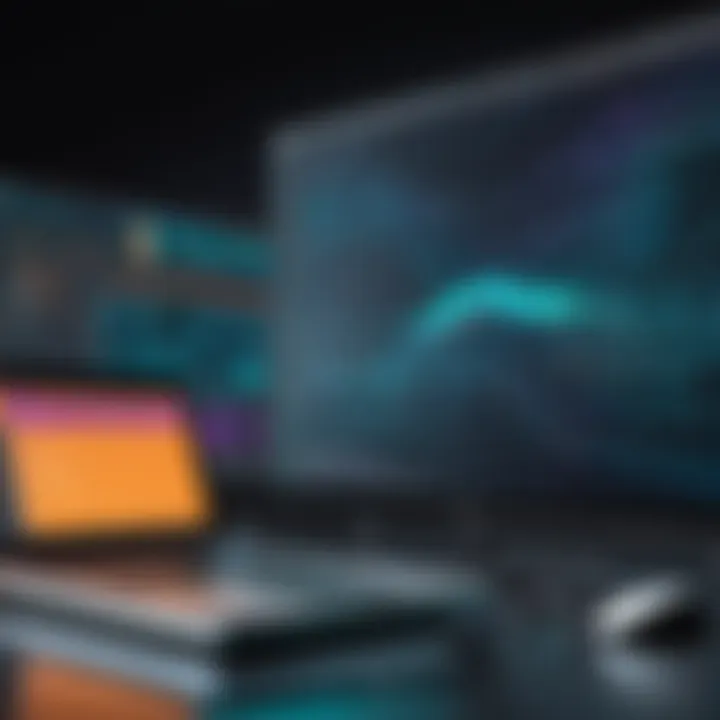
Selecting an Integrated Development Environment (IDE) aligns with serious code crafting. An IDE dictates your interaction with the code and provides essential features, like syntax highlighting, debugging tools, and code completion, that enhance the developmental workflow. Among the prevalent options is Microsoft Visual Studio. Its robust capabilities frequently attract both learners and experienced developers. Alternatively, Code::Blocks provides versatility and remains lightweight.
While as you explore IDE selection, consider what features matter most. For instance, project management, version control integration, and support for plugins can influence your project appearance.
Installing the ++ Compiler
Proper compiler installation is another cornerstone in development setup. The C++ compiler translates your code into executable programs. Without it, your code will remain dormant, stuck in the realm of text. Popular C++ compilers include GCC and Clang, both known for consistency across platforms.
The installation often involves downloading the setup file from the official site, following the on-screen instructions, and configuring environmental paths to make the compiler easily callable from command lines. It should be understood that a typical oversight involves missing these artifact setups, leading to frustrations and needless project delays.
Configuring Your Project Settings
After appropriately acquiring the IDE and compiler, the final task lies in configuring the project settings. This action profoundly affects how you manage your development and debugging procedures. Start by setting the project type—whether it's a console application or a graphical one. It helped concerted efforts and saved hitting directions late in the game. Default configurations might work at first, but customizing them gives clarity to intricate project codes.
Here are some practical points to consider during configuration:
- Defining include paths for header files
- Setting up libraries to be linked
- Proper discovery of build tools and versions along the workflow
Taking these steps ensures your first steps into longer journeys are stable, aligning C++'s capabilities with a harmonious environment that allows mastery over code, fostering a setting where creativity can flourish.
Remember: A foundational setup can make or break your programming experience. Invest the time upfront to configure your environment thoughtfully.
Fundamentals of ++ Programming
Understanding the fundamentals of C++ programming is essential for anyone looking to grasp this language effectively. This knowledge establishes the groundwork needed for advanced coding skills. C++ is versatile, so knowing its basics allows one to navigate its more complex areas smoothly. Learning these fundemental elements can increase productivity and decrease coding errors significantly. Ultimately, it empowers developers to create robust and efficient applications.
Basic Syntax and Structure
C++ possesses a distinct syntax that often draws from other popular programming languages like C and Java. Starting with basic syntax helps developers grasp how C++ operates. It's crucial for beginners to be aware of the grammar for coding:
- Keywords are reserved terms that have specific meanings.
- Operators perform operations on variables and values.
- Identifiers are names given to various elements in a code, such as functions, variables, and classes.
- Statements end with a semicolon and define the end of an instruction.
A simple example can illustrate these concepts:
In this code, we see how a basic program structure comes together. The familiar parts like header files, the main function, and statements highlight C++'s fundamental syntax.
Data Types and Variables
Choosing the right data types and understanding variables is fundamental in coding. Data types inform the program how to handle the stored values. In C++, there are several built-in data types:
- for integers.
- for characters.
- for floating-point numbers.
- for double-precision floating-point.
Variables act as containers for these data types. Declaring a variable involves specifying the data type followed by an identifier. For instance, using establishes an integer variable named age and assigns it a value.
Control Structures: Loops and Conditionals
Control structures allow developers to dictate the flow of a program. They help in executing particular blocks of code based on specified conditions or repeat actions. Key to this is understanding loops and conditionals.
Conditionals, such as if-else statements, enable decisions within a program:
Loops (like for and while) help automate repetitive tasks. A common example is a for loop that counts to ten:
Such structures are essential for creating dynamic and responsive applications, enhancing overall functionality.
Functions and Modular Programming
Functions are a cornerstone of effective programming in C++. They allow developers to encapsulate code functionality, making it reusable and manageable. A well-structured function provides clear inputs, outputs, and behavior. General benefits include:
- Enhancing code readability.
- Facilitating debugging and maintenance.
- Breaking down complex tasks into simpler components.
A basic function might look like this:
This demonstrates how to create a function for adding two numbers. Optimizing code through functions fosters an environment for systematic development.
Object-Oriented Programming in ++
Object-Oriented Programming, often abbreviated as OOP, stands as a cornerstone in modern software development. This programming paradigm allows for more manageable and scalable code. In C++, OOP provides a straightforward approach to organizing complex software structures. Instead of relying on procedural programming, where the focus is on functions and logic, OOP emphasizes objects, which encapsulate data and behavior. This shift enhances reusability and adaptability, which are crucial for evolving software.
Understanding Objects and Classes
At the core of OOP are objects and classes. A class serves as a blueprint for creating objects. Each object is an instance of a class that can hold data and perform functions. In C++, you can define a class as follows:
In the example above, a class contains properties such as and , and a method called . This structuring of code enables developers to define types that mirror real-world entities with both attributes and behavior. The encapsulation of data allows for safer and more predictable code behavior.
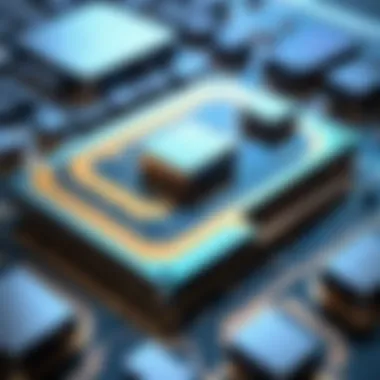
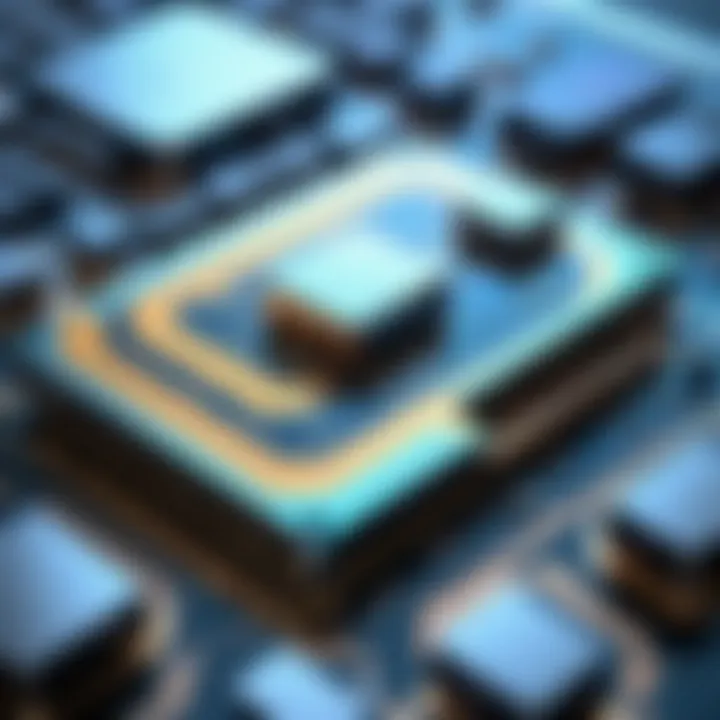
Encapsulation, Inheritance, and Polymorphism
OOP introduces three fundamental principles: encapsulation, inheritance, and polymorphism.
- Encapsulation refers to bundling the data with the methods. It restricts direct access to some of an object's components, which can help prevent unintended interference and misuse. For instance, utilizing and access specifiers controls the accessibility.
- Inheritance allows new classes to inherit properties and methods from existing ones. For example, if you have a class, a could inherit from it, gaining its characteristics and promoting code reusability.
- Polymorphism enables methods to do different things based on the object it is acting upon, even if they share the same name. This dynamic behavior is a powerful feature that allows for more flexible code development.
Implementing these principles can lead to productive coding habits.
Abstract Classes and Interfaces
In C++, abstract classes play a vital role in establishing interfaces that must be implemented by derived classes. An abstract class could contain pure virtual functions that child classes need to provide specific implementations for. This mechanism supports flexibility, ensuring different derived classes are compatible with expectations set by their base class.
For instance:
Deriving a class from this abstract class mandates that derived classes provide a definition for . Derived classes like and could implement a specific version of , catering precisely to their unique shape behavior while adhering to a common interface. This structure enforces a consistent architecture across your code, enhancing maintainability and understanding.
OOP in C++ shapes the way developers think about their software, fostering clear organization, flexibility, and improvements in the maintenance of code.
Advanced ++ Concepts
Exploreing advanced C++ concepts is vital for programmers who seek to enhance their coding capabilities. These concepts help developers to write efficient, robust, and maintainable code. Mastery of advanced topics not only boosts productivity but also allows for leveraging C++ to its full potential.
Templates and Generic Programming
Templates facilitate code reuse and lead to more maintainable programs. By allowing functions and classes to operate with generic types, templates promote flexibility without compromising type safety. This means developers can craft algorithms and data structures that work with any data type, removing redundancy in code.
The elegance of templates lies in reducing duplicated code, thereby enhancing maintainability.
Key benefits of templates include:
- Reusable Code: Write algorithms once and use them for any data type.
- Type Safety: Ensures errors are caught during compilation rather than at runtime.
- Performance: Inline expansion for templates improves runtime performance.
A basic example of a template function for swapping two values is as follows:
Exception Handling
Exception handling provides a structured way to respond to runtime errors. It allows programmers to manage errors gracefully without disrupting program flow. Using try, catch, and throw statements, developers can isolate error handling logic, resulting in cleaner and more understandable code. This is increasingly important in larger applications where unhandled exceptions may cause system crashes.
The performace enhancement in exception handling include:
- Error Recovery: Allows programs to recover from unexpected situations.
- Resource Management: Helps maintain resource allocation by ensuring cleanup.
- Clear Code Flow: Keeps error handling separate from business logic.
Here is an example in C++ demonstrating exception handling:
Memory Management: Pointers and References
Effective memory management is crucial in C++. Pointers and references provide additional control over memory allocation and resource management. Understanding when and how to use these features allows developers to optimize their applications for performance.
Points of consideration include:
- Direct Access: Pointers provide direct access to memory locations, vital for dynamic memory allocation.
- Ownership Management: Proper usage prevents memory leaks by ensuring memory is properly freed.
- Efficient Use of Resources: References provide safer alternatives by avoiding direct memory manipulation while still allowing resource management.
Here’s a small code snippet highlighting pointer usage to manage dynamic memory:
Understanding the Standard Template Library (STL)
The Standard Template Library is a powerful feature of C++ that provides a collection of data structures and algorithms. STL enhances development by offering ready-to-use components that are well-tested and efficient.
Key features of STL include:
- Containers: Structures like vectors, lists, and maps align with specific data operations.
- Algorithms: Searching and sorting functionalities are optimized and easy to implement.
- Iterators: Provide uniform access to various container types, promoting code consistency.
STL empowers developers to focus more on problem-solving and less on implementing frequently used data structures and algorithms. For anyone serious about C++, mastering STL is essential for writing efficient and scalable applications.
Best Practices for ++ Development
In the context of C++ programming, adhering to best practies is not just advisable; it's essential. By following established guidelines, developers can produce code that is easy to read, maintain, and scale. This foundation allows projects to evolve without introducing bugs, which is particularly crucial as systems grow in complexity.
Crafting software involves more than just getting it to work; it is about ensuring that it remains functional and understandable in the long run. Emphasizing best practices means promoting a vision of C++ coding that never sacrifices quality for expediency. Here are several critical components to consider.
Code Readability and Maintenance
Code readability greatly impacts how easily others can understand your work. Clear naming conventions for variables and functions are vital. Avoid abbreviations unless they are universally accepted. Descriptive names convey intent and help in grasping what specific portions of code are doing. Consistency in formatting is also significant. Align your braces, maintain uniform space, and structure each function with proper indentation.
This careful organization allows others—and your future self—to navigate your code without mental overhead.
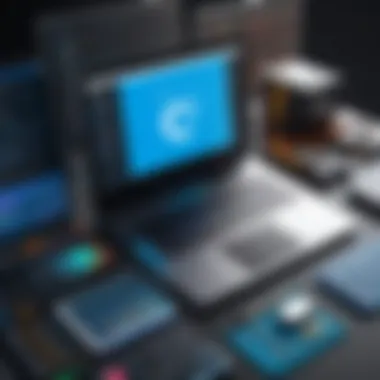
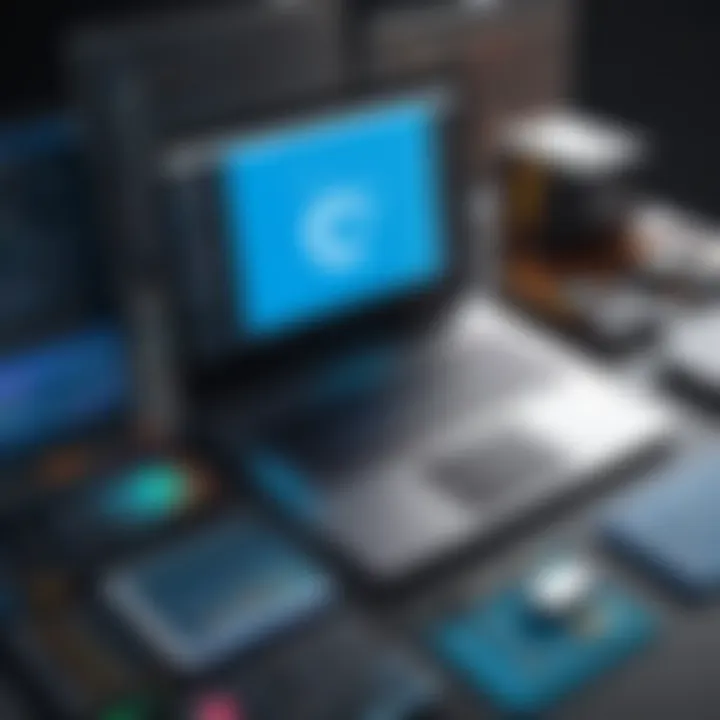
Here’s a list of tips for maintaining quality readability:
- Use clear and meaningful identifiers.
- Comment on non-obvious logic.
- Align templates cleanly to maintain visibility.
- Refactor code regularly if parts become unwieldy.
Pro Tip:
For better maintenance, create documentation as you code. This can include both instinctual understandings in comments and broader design documentation in external references to clarify the architecture. Balancing commentation and documentation is fundamental.
Effective Debugging Techniques
Debugging is an integral part of development. Using tools effectively can save considerable time. Resourceful developers tend to leverage Xcode, Visual Studio, or other environments. Before you start debugging, ensure that you have made:a few pre-checks. These should include reviewing your input, expected outcome, and firmness of your design's logic.
Here are a few practices to enhance your debugging efforts:
- Incorporate logging statements throughout your codebase. This provides a trail you can follow if you encounter issues.
- Familiarize yourself with the debugger tool of your IDE. Learn to set breakpoints and inspect running variables.
- Employ a methodical approach. Fix one issue at a time instead of trying to tackle multiple problems simultaneously.
Reminder:
Debugging is an iterative process. The same techniques and the methodical approach should be employed continuously to prevent bugs from proliferating in production code. It's also beneficial to know that hygiene in debugging practices contributes significantly toward resilient applications.
Leveraging Version Control Systems
Using a version control system is crucial in today’s codebases. Systems like Git allow developers to track changes efficiently, revert when necessary, and work collaboratively without clashes in code. These systems promote a disciplined approach to managing projects of any size.
Consider adopting strategies such as:
- Frequent commits: Smaller, more manageable changes allow for understanding project evolution.
- Meaningful commit messages: Good messages describe the changes and give insight into intention.
- Use of branches: Work on features or bug fixes away from the main production code to avoid adding instability prematurely.
More experienced developers understand how version control can evolve a project.
Effective use of version control can make a fundamental difference in collaborative endeavors whereby maintaining a history allows for traceability and accountability in code decisions.
Resources for Continued Learning
Continued learning is vital in the ever-evolving field of software development. Resources for continued learning in C++ offer structured pathways to deepen understanding and improve practical skills. While classroom settings provide foundational knowledge, the real challenge lies in applying this knowledge. Resources that blend theory and practical application promote competency and adaptability. Learning never stops when programming languages evolve. Learning from various formats, such as courses, books, and community engagement systems supplies fresh insights into existing knowledge.
Online Courses and Tutorials
Online courses and tutorials are fundamental for contemporary learning. They provide flexibility, allowing learners to study according to their availability. Platforms like Coursera, edX, and Udemy offer a range of C++ courses tailored for different skill levels. Favorable aspects of these courses include:
- Structured Learning: Many courses divide content into modules, making complex topics manageable.
- Interactive Elements: Quizzes and hands-on projects foster a deeper understanding, more than passive reading.
- Community Support: Some platforms have forums or discussion groups that enrich the learning experience, permitting learners to ask questions and get responses from peers or instructors.
Tutoring and peer-to-peer platforms like Tutor.com allow learners to get personalized help and clarify doubts. Utilizing these resources effectively can lead to considerable improvement in coding confidence and survival skills.
Books and Reference Material
Books are essential, as they provide a thorough exploration of C++ concepts. Several titles stand out and should be considered for any a C++ developer’s library: Effective C++, C++ Primer, and The C++ Programming Language. Here’s what to look for in books:
- Updated Content: Ensure the book is recent enough to cover modern practices and standards. Selecting older materials may hinder understanding of current coding st184dards.
- Hands-On Examples: Look for books with numerous examples illustrating theoretical concepts. This dissemination supports practical understanding.
- Comprehensive Index: A good reference book should include index sections to quickly locate topics when needed. This can save valuable time during coding projects.
Alongside books, printed resources such as compiler documentation become useful for in-depth knowledge.
Community Forums and Open Source Projects
Engagement within the programming community is an indispensable resource for growth. Forums such as Reddit's C++ Subreddit are business-soriented, with clarified boundaries for asking unique questions. They provide opportunities to shade different perspectives through shared experiences. Participating helps in:
- Problem-Solving: Describing challenges within community forums can lead to alternative solutions that books or tutorials may not cover.
- Networking: Building connections with others can promote collaboration and future opportunities.
- Open Source Contributions: Contributing to open-source projects offers real-world experience. Websites like GitHub allow developers to collaborate, refine their skills, and understand existing codebases.
Open source involvement rounds out the language learning experience by ensuring that understanding transcends excitement into cross-team collaboration skills, essential for progressing in development environments.
Effective learning is a journey where utilization of diverse resources prepares developers for the future, ensuring they remain agile to rapid technological trends and evolutions in programming.
End
The conclusion serves a pivotal role in summarizing the journey through mastering C++. It presents a chance to reinforce critical lessons gained in preceding sections. By recapping these foundational elements, readers strengthen their understanding and solidify their knowledge.
Moreover, the conclusion provides insight into the future of C++ development. This has significant implications for software developers, guiding them on what skills will be in demand. C++ plays a vital role in various sectors, from system programming to game development. Recognizing this potential ensures that developers align their learning with industry trends.
Recap of Key Concepts
In this article, several core concepts regarding C++ programming have been discussed:
- History and Evolution: C++ introduced many objects-oriented features which transformed programming approaches.
- Object-Oriented Principles: Encapsulation, inheritance, and polymorphism enrich code architecture in significant ways.
- Best Practices: Implementing effective debugging techniques and fostering code readability are critical to successful development processes.
Understanding these concepts not only supports efficient programming but also enhances collaboration among developers. Each aspect is intricately linked:
- The language's history shapes modern functionalities.
- Object-oriented programming enables clean systems.
- Best practices elevate team efforts in software projects.
As novices or experienced developers recall these points, they lead to a cohesive wrapper around the complexities of learning C++.
Future Trends in ++ Development
Examining future trends is essential for any programmer seeking to remain competitive. Notably, there are a few trajectories observed:
- Expansion of Libraries: New libraries like the GPU Computing System and enhanced multithreading capabilities will propel performant applications and programming experiences.
- Emphasis on System Efficiency: As systems become more complex, efficient coding becomes paramount. Developers must focus on optimization for faster performance.
- Development within Diverse Areas: Beyond legacy systems, C++ usage will continue to branch into industries such as AI, machine learning, and IoT.
Adopting these trends fosters growth not only individually but also for the broader software development community. C++ remains integral, and awareness of upcoming developments encourages continual education and personal refinement among developers. This ensures readiness to meet future challenges head-on.
"Understanding where C++ is headed allows developers to embrace the future while honing existing skills."