Mastering Concurrent Java Programming Techniques
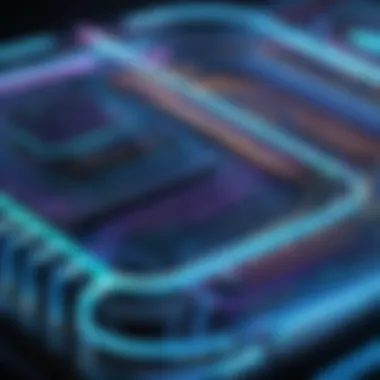
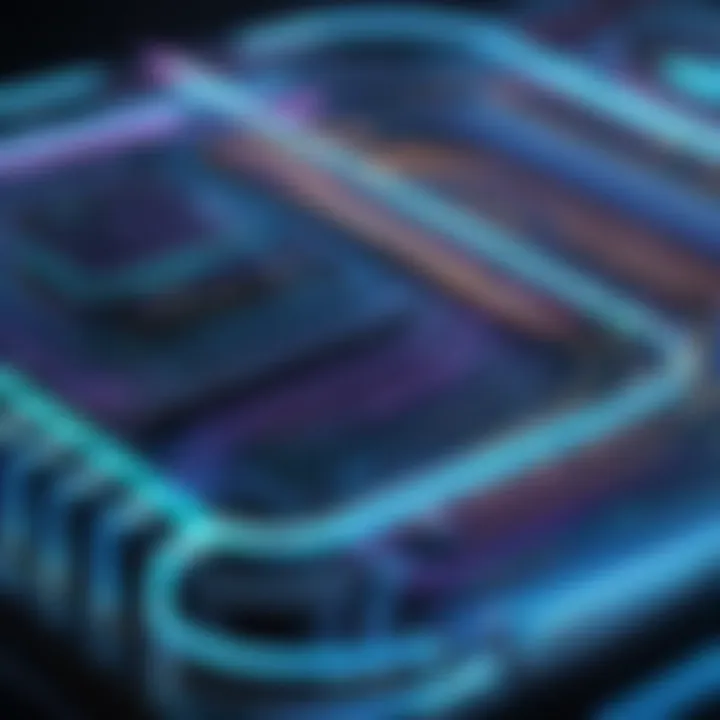
Intro
In the current landscape of software development, the demand for efficient and responsive applications continues to grow. Concurrent programming plays a vital role in this evolution, especially in programming languages like Java. This approach allows multiple threads to function simultaneously, greatly enhancing productivity and performance. In this article, we will explore the intricacies of concurrent Java programming and provide developers with the tools needed to master this essential skill.
Overview of Concurrent Programming in Java
Concurrent programming refers to the capability of a program to execute multiple tasks at the same time. In Java, this is primarily achieved using multithreading. The importance of concurrent programming cannot be overstated. By leveraging multiple threads, applications can handle more simultaneous operations, respond more quickly to user input, and maximize resource utilization.
Key features of Javaâs concurrent programming include:
- Thread Management: Java provides built-in support for managing threads through the class and the interface.
- Synchronization: To avoid data inconsistency when multiple threads interact, Java introduces synchronization, allowing controlled access to shared resources.
- Concurrency Utilities: Java implements various classes, such as those found in the package, to facilitate easier and safer concurrent programming.
Use Cases
Concurrent programming in Java is applicable in various scenarios, such as:
- Web servers handling multiple client requests
- Real-time systems where timely responses are critical
- Data processing applications that can perform multiple operations at once
The benefits of employing concurrent programming techniques can result in enhanced application performance, improved resource management, and a smoother user experience.
Best Practices
To ensure effective implementation of concurrent programming in Java, developers can follow several industry best practices:
- Use Thread Pools: Instead of creating new threads for each task, which is resource-intensive, utilize thread pools. The framework provides an efficient way to manage a pool of worker threads.
- Minimize Synchronization: Overusing synchronization can lead to bottlenecks. Aim to minimize the synchronized sections of your code.
- Utilize Concurrent Collections: Java offers collections designed for concurrent access, such as , which are preferable over traditional collections.
Tips
- Regularly profile your application for performance bottlenecks related to thread usage.
- Prefer immutability for shared data to reduce synchronization needs.
- Understand the different thread statesâNEW, RUNNABLE, BLOCKED, WAITING, TIMED_WAITING, and TERMINATEDâas this understanding aids in debugging concurrency issues.
Common Pitfalls
Some pitfalls to avoid include:
- Ignoring thread safety when accessing shared resources.
- Creating too many threads, which can lead to overhead and decreased performance.
- Underestimating the complexity of debugging concurrent applications.
"Concurrency is not just a technique but a fundamental shift in how we design our applications."
Case Studies
Prominent examples of concurrent programming implementations showcase the technique's effectiveness:
- Apache Tomcat: This widely-used web server utilizes concurrent programming to manage multiple connections effectively.
Lessons Learned: The use of thread pools enabled Apache Tomcat to handle thousands of concurrent connections, demonstrating optimal resource management.
Latest Trends and Updates
As the technology landscape evolves, trends in Java concurrency include:
- Reactive Programming: Frameworks like Project Reactor and RxJava promote the use of event-driven, non-blocking applications.
- CompletableFuture: This tool provides a way to write asynchronous, non-blocking code in a more readable format than traditional approaches.
Current Predictions: The rise of microservices architecture may further influence developments in concurrent programming techniques and their implementations.
How-To Guides and Tutorials
Developers aiming to enhance their Java concurrency skills can utilize the following resources:
- Step-by-Step Guide:
- Hands-on Tutorial: Follow specific examples that demonstrate the use of Javaâs for concurrent task execution.
- Set up your Java development environment with the necessary tools.
- Create a simple Java application utilizing threads to understand basic multithreading.
Practical Tips: Always start small. Understand the basic concepts of threads and synchronization before tackling more complex problems.
By following the guidance outlined in this article, developers can wield the power of concurrent programming in Java effectively. This skill, applied thoughtfully, can lead to significant improvements in application performance and resource efficiency.
Prolusion to Concurrent Programming
Concurrent programming is a fundamental concept in software development, particularly in the context of Java programming. This section aims to elaborate on the critical aspects of concurrency that will be discussed in the article, emphasizing its importance in today's tech landscape.
In the era of multi-core processors and resource-intensive applications, the ability to execute multiple threads of execution simultaneously is essential. Concurrent programming allows developers to optimize resource utilization and enhance application performance. By managing multiple operations at once, programs can achieve better responsiveness and efficiency. This is especially relevant in applications that demand real-time processing of data, such as web servers or financial systems.
Concurrency is not just a technical necessity; it also presents numerous benefits and considerations for developers. Mastering concurrent programming can lead to more robust applications, as it enables better handling of tasks and improved application scalability. However, with these advantages come challenges, such as potential race conditions, deadlocks, and complexities in debugging. Understanding these elements is key for developers working with multi-threaded applications in Java.
Definition of Concurrent Programming
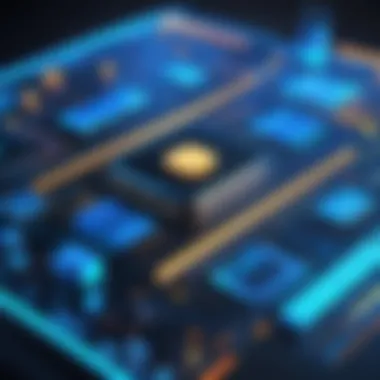
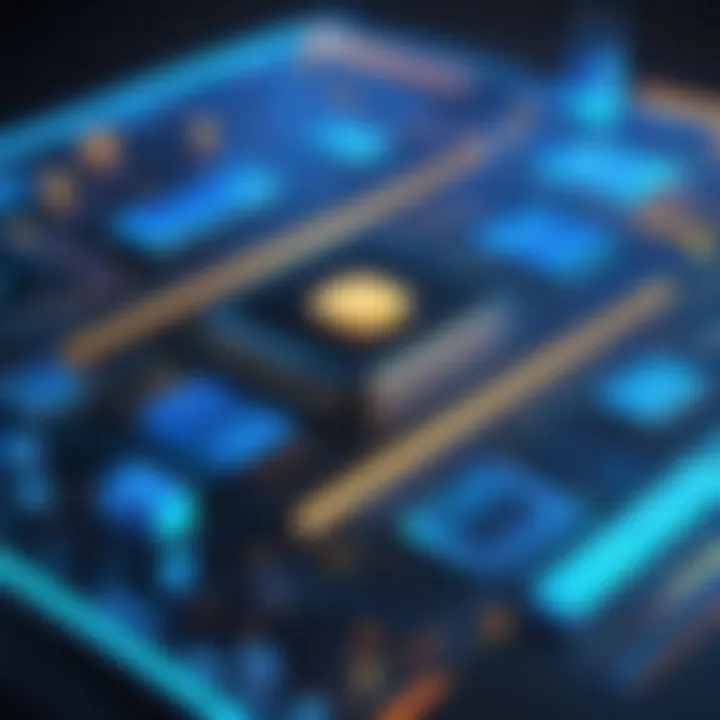
Concurrent programming refers to the ability of a program to execute multiple tasks simultaneously or in overlapping time periods. This does not necessarily mean that the tasks are running at the exact same instant (as with parallel programming). Instead, it emphasizes the management of multiple threads that can make progress independently, enhancing the overall efficiency of a program.
In Java, concurrency is built into the language through several constructs that allow developers to work with threads, execute tasks, and manage shared resources. The main goal of concurrent programming is to improve the performance of applications, particularly those that handle multiple users or require high levels of computation. Understanding its fundamental principles is vital for any software developer looking to create high-performance Java applications.
Importance of Concurrency in Software Development
The significance of concurrency in software development cannot be understated. As applications become more complex, the need for concurrency grows. Here are several reasons why concurrency is crucial:
- Performance Enhancement: Concurrent applications can accomplish more tasks in less time, especially when leveraging multi-core processors. This can lead to better throughput and response times.
- Resource Optimization: By executing tasks concurrently, developers can utilize system resources more effectively, minimizing idle CPU time.
- Improved Responsiveness: Applications can remain responsive to user input while performing background tasks. This is particularly beneficial in user-interface-heavy applications, ensuring a seamless user experience.
- Scalability: Concurrency allows applications to scale more easily by handling an increasing number of operations without a linear increase in resource consumption.
However, developers must also consider the complexities introduced by concurrency. Effective management of multiple threads requires careful design to prevent common issues such as race conditions and deadlocks. Understanding the risks and employing best practices can help mitigate these challenges, making concurrency an invaluable aspect of modern programming.
"Concurrency is no longer just an option; it is a necessity in software development today."
The exploration of concurrent programming in Java is a journey through its essential components. In the following sections, we will delve into the fundamentals of multithreading and the synchronization mechanisms that make concurrent Java programming both powerful and manageable.
Fundamentals of Multithreading in Java
Multithreading is a core concept in concurrent programming within Java. Understanding the fundamentals of multithreading is essential for developers aiming to build efficient applications that leverage the capabilities of modern multi-core processors. Effectively managing multiple threads enables applications to perform numerous tasks simultaneously, enhancing responsiveness and throughput.
Concepts of Threads
A thread, in simple terms, is a lightweight process. Each thread shares the same memory space as the main application, allowing quick communication and minimizing overhead. Threads can run concurrently, which means that multiple threads can execute parts of a program at the same time. This is advantageous in scenarios where tasks can be broken down into smaller, independent units of work.
It is also key to understand that, in Java, each application has a main thread, and any new threads are child threads of this main thread. This hierarchy allows for structured thread management. If any child thread encounters an issue, it can affect the main thread and consequently the whole application. Therefore, understanding thread management is crucial for reliability and performance.
Creating Threads
Creating threads in Java can be achieved in two primary ways: by extending the Thread class and implementing the Runnable interface. Each approach has its own merits, which makes them suitable for different situations.
Extending Thread Class
Extending the Thread class is perhaps the most straightforward method of creating a thread. By subclassing the Thread class, developers can define the task to be executed in the method. This approach offers a clear and direct way to encapsulate functionality.
One of the key characteristics of extending the Thread class is that it allows direct manipulation of the thread itself. This means developers can control the threadâs lifecycle by calling methods like , , or directly on their thread instances. However, this method has a limitations; since Java does not support multiple inheritance, extending the Thread class restricts the class from extending any other class.
- Benefits:
- Disadvantage:
- Simplicity in writing and understanding.
- Direct access to thread methods.
- Limited flexibility due to single inheritance.
Implementing Runnable Interface
The Runnable interface offers an alternative approach to threading. By implementing Runnable, developers can write code to define the task in the method, while still retaining the ability to extend other classes. This method is often preferred for larger applications where the class structure needs to be maintained.
A key characteristic of this approach is its ability to separate the task from the threadâs execution mechanics. This separation of concerns often leads to cleaner code and improved maintainability. Developers create an instance of a Thread and pass an instance of a class implementing Runnable. This not only provides flexibility but also allows multiple threads to share the same Runnable instance.
- Benefits:
- Disadvantage:
- Greater flexibility as it allows class inheritance.
- Separation of task definition and execution.
- Slightly more complex than extending the Thread class.
Thread Lifecycle
The lifecycle of a thread is fundamental to understanding its behavior during execution. Java threads can exist in several states:
- New: Thread is created but not yet started.
- Runnable: Thread is active and ready to run.
- Blocked: Thread is blocked waiting for a monitor lock.
- Waiting: Thread is waiting indefinitely for another thread to perform a particular action.
- Timed Waiting: Thread is waiting for another thread to perform an action for up to a specified waiting time.
- Terminated: Thread has completed execution.
Understanding these states and transitions can aid developers in debugging and optimizing concurrent applications. Managing transitions properly can mitigate issues such as resource contention and ensure efficiency across multi-threaded processes.
Proper management of threads is critical. Understanding the lifecycle ensures better control over application performance.
Synchronization Mechanisms in Java
In the realm of concurrent programming, synchronization mechanisms play a pivotal role in ensuring data integrity and consistency while allowing multiple threads to access shared resources. This section focuses on the significance of synchronization in Java, elucidating its essential components, benefits, and considerations that developers must keep in mind.
Why Synchronization is Necessary
Synchronization is crucial in multithreaded programming because it helps avoid the phenomenon known as race conditions. A race condition occurs when two or more threads attempt to modify shared data simultaneously. This situation can lead to unexpected or erroneous outcomes, undermining the reliability of applications.
When threads are not synchronized, the resulting inconsistencies can manifest in various ways:
- Data corruption, where the final value of a shared resource might not reflect the intended results of operations.
- Unpredictable behaviors that make debugging challenging, potentially causing software failures in production.
- Security vulnerabilities introduced by allowing concurrent access to sensitive data.
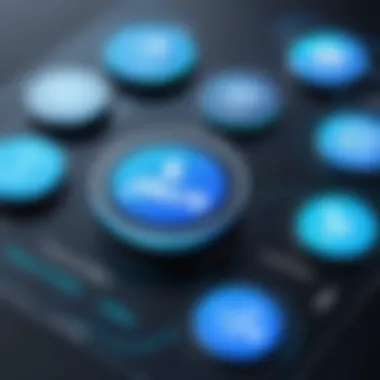
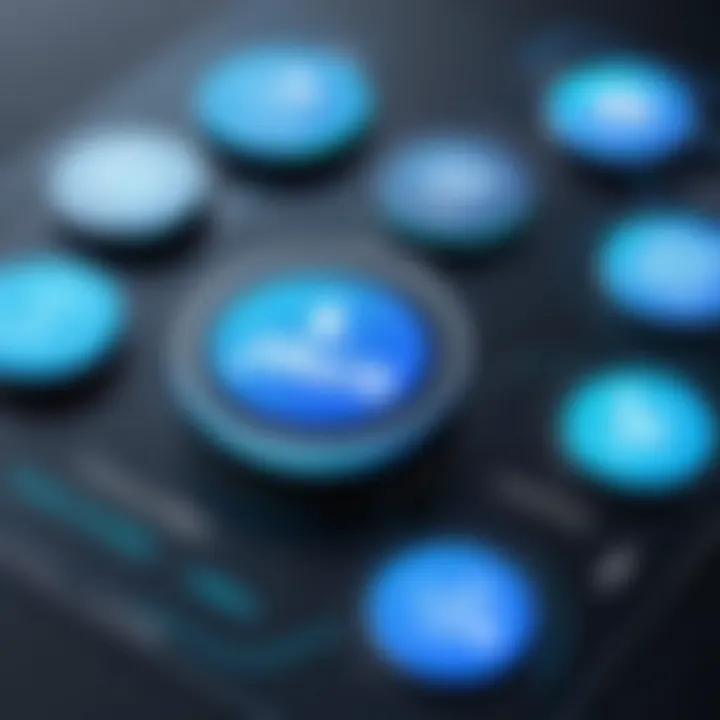
Hence, employing synchronization mechanisms is not just a good practice; it is essential for the development of robust concurrent Java applications.
Synchronized Methods and Blocks
Java provides built-in support for synchronization through the use of synchronized methods and synchronized blocks. Both approaches serve to control access to an object's resources, but they differ in their implementation and scope.
- Synchronized Methods: By declaring a method as synchronized, Java ensures that only one thread can execute this method on a particular object instance at a time. This is done by locking the object instance (or the class if the method is static). Here it is important to note that while it guarantees exclusive access, it can also lead to performance bottlenecks if overused.Example of synchronized method:
- Synchronized Blocks: In contrast to synchronized methods, synchronized blocks allow more granular control over synchronization. They can lock only a specific section of code, reducing the time that the lock is held and allowing greater concurrency. This is especially useful when you need to synchronize only part of a larger method.Example of synchronized block:
Using synchronized blocks can help enhance performance while still providing the necessary thread safety. However, careful consideration is needed to avoid deadlocks.
Locks and Reentrant Locks
Beyond synchronized methods and blocks, Java also offers a more advanced mechanism called locks, specifically through the package. Locks provide a higher level of control over synchronization than the traditional synchronized keyword.
- Locks: With explicit locks, developers can manage conditions under which threads may acquire or release the locks. This added flexibility includes trying to acquire a lock without blocking or interrupting the thread if the lock is not available. This is useful in environments that require high concurrency and performance.
- Reentrant Locks: One type of lock provided is the reentrant lock. A reentrant lock allows a thread to re-acquire a lock it already holds, preventing deadlocks related to recursive locking. This makes it particularly advantageous for applications that involve recursive function calls.Example of using ReentrantLock:
In summary, understanding synchronization mechanisms is vital for any developer dealing with concurrent Java programming. Using synchronized methods, synchronized blocks, and locks effectively can significantly contribute to the creation of reliable, efficient applications. As developers navigate these tools, they must remain vigilant about potential issues like deadlocks and performance bottlenecks, ensuring they use synchronization judiciously.
Concurrency Utilities in Java
Concurrency utilities are essential in Java programming. They provide tools that simplify the complexities of concurrent programming. As applications become more complex, the need for efficient concurrency management increases. In this context, concurrency utilities serve as enablers for developers to write better, more maintainable code.
The package is a significant contribution to this domain. It offers various classes and interfaces designed to facilitate concurrent programming. Utilizing these utilities can lead to improved application performance. Developers can manage multiple threads more effectively and handle synchronization issues with greater ease. This section delves into specific elements of these utilities, highlighting their benefits and considerations.
Prologue to java.util.concurrent Package
The package is a cornerstone of Java's concurrency model. It provides important classes for managing thread behavior and interaction. This package includes features like thread pools, synchronization mechanisms, and atomic variables. By using this package, developers can avoid common pitfalls associated with concurrency and improve their applications.
Java's concurrency utilities support scale and performance. For programmers, they allow for writing cleaner and more efficient code. Instead of managing threads directly, developers can leverage higher-level abstractions provided by the package. This can reduce debugging time and improve overall productivity.
Executor Framework
The Executor Framework redefines how tasks are executed in Java. It introduces a new level of abstraction for managing and controlling threads.
Executor Interface
The Executor Interface is a fundamental part of the package. Its role is simple yet powerful: it decouples task submission from the mechanics of how each task will be run. This means that the developer can focus on defining the task, while the execution details are managed by the framework.
A key characteristic of this interface is its flexibility. It allows different execution strategies, depending on the need of the application. This is beneficial for developers aiming to enhance performance and maintainability. Executor Interface is popular because it allows modern Java applications to handle thread management more systematically, avoiding pitfalls such as manually managing thread lifecycles.
But, it does come with some considerations. For instance, proper understanding is needed when choosing the appropriate type of executor, like or , to avoid deadlocks or resource overutilization.
Thread Pools
Thread Pools are a critical component of the Executor Framework. They offer a way to manage multiple threads more effectively by reusing them rather than creating new ones each time a task runs. Thread Pools manage a set of worker threads and queue tasks for execution. This characteristic is especially useful for applications that require high throughput, as it minimizes the overhead associated with thread creation.
The unique feature of Thread Pools is their ability to regulate the number of active threads. This can help in avoiding resource exhaustion when too many threads run simultaneously. They are a popular choice because they provide a more efficient use of system resources while keeping the application responsive.
Future and Callable Interfaces
Future and Callable Interfaces augment the concurrency capabilities further by providing mechanisms to retrieve results from asynchronous computations. The Callable Interface allows tasks to return results, unlike the normal Runnable interface, which cannot. Future serves as a handle for managing those tasks, enabling checking of task completion and retrieving results safely when available. This adds significant value in use cases where thread tasks produce output and application logic depends on that output without blocking the executing thread waiting for it.
Using Future and Callable Interfaces contributes to a more orderly and manageable concurrency model. As a result, developers can write robust applications that interact efficiently with their asynchronous responses.
Handling Concurrency Challenges
Concurrency challenges present significant obstacles for software developers working with multithreaded applications in Java. Understanding these challenges is essential for creating robust, efficient programs. Mismanagement of concurrent processes can lead to various issues, including deadlocks, starvation, and livelock, which in turn can compromise application performance and reliability.
Effective management of these challenges requires careful attention to thread interactions and resource sharing. Developers must consider the implications of how threads work concurrently and the potential conflicts that arise. Addressing concurrency issues not only enhances application stability but also improves overall performance, providing a smoother experience for end-users.
Deadlock
Deadlock occurs when two or more threads are unable to proceed because each is waiting for a resource held by another. This situation can halt application progress, causing it to freeze indefinitely. Knowing the causes and how to prevent deadlock is crucial for maintaining flow in concurrent Java programming.
Causes of Deadlock
The causes of deadlock typically arise from the way resources are allocated among threads. A key characteristic of deadlock is the circular wait condition, where two or more threads hold specific resources and wait for each other to release the locked resource. This makes it a concerning aspect of concurrency management.
Each thread locks a resource and waits for another resource that another thread holds. This creates a bottleneck, as both threads continuously wait for each other. Such circumstances can lead to severe delays or a complete halt in your application. Recognizing these causes allows developers to design systems that thwart this issue before it manifests.

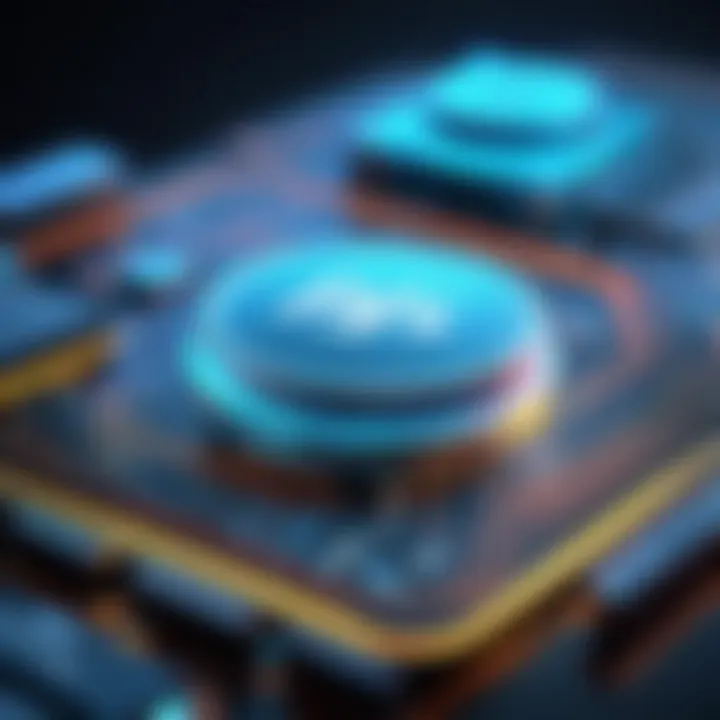
Preventing Deadlock
Preventing deadlock involves implementing strategies to ensure that it does not occur in the first place. A valuable method is the resource hierarchy principle, where resources are always requested in a consistent order. This approach aims to eliminate circular waiting by structuring resource acquisition. It is a popular choice for this article due to its straightforward implementation and effectiveness.
Another technique includes using timeouts when attempting to acquire locks. This allows a thread to back off if it cannot obtain a resource within a certain time period, reducing the likelihood of getting stuck. The unique feature of this method is its ability to maintain application responsiveness, as threads can recover and try again later.
Starvation and Livelock
Starvation happens when one or more threads are perpetually denied access to resources they need for execution, usually due to thread scheduling. On the other hand, livelock involves threads continuously changing states in response to each other's actions without making any progress. Both concepts highlight the importance of fair resource allocation among threads to ensure smooth operation in concurrent Java programming. Proper understanding of these issues can significantly enhance performance and stability in multithreded applications.
Best Practices for Concurrent Java Programming
In the realm of concurrent programming, adopting best practices is essential. These practices ensure that Java applications are not only effective but also stable and safe. As developers deal with multiple threads, issues such as race conditions, deadlocks, and latency come into play. Following proven strategies minimizes risks and enhances application performance. Below are key considerations and techniques for achieving this goal.
Designing Thread-Safe Classes
Creating thread-safe classes is pivotal in concurrent programming. A thread-safe class ensures that its methods can be called by multiple threads simultaneously without leading to inconsistent states. To achieve this, developers can use several approaches:
- Synchronized Methods: Implement synchronization in methods to prevent multiple threads from altering shared data simultaneously.
- Atomic Variables: Utilize classes such as from the package. They allow safe operations without traditional locking mechanisms.
- Immutability: Design classes whose instances are immutable. Immutable objects can be freely shared between threads without synchronization, as their state remains constant.
Using these approaches helps in reducing complexity and enhances reliability. A well-designed thread-safe class contributes to overall system performance by mitigating issues associated with concurrent access.
Minimizing Shared Resources
Reducing the number of shared resources in an application is a highly effective practice. Shared resources often become the bottleneck in concurrent systems, leading to increased contention and latency. Here are practical methods to minimize shared resources:
- Local Variables: Favor local variables whenever possible. Since they are stored on the stack, each thread has its own version and does not need synchronization.
- ThreadLocal Variables: Consider for variables that need thread-specific instances, avoiding shared states between threads.
- Design Patterns: Implement design patterns such as the Producer-Consumer or Publisher-subscriber that inherently reduce direct resource sharing.
Managing resource utilization effectively can lead to a significant enhancement in performance. This practice not only improves speed but also reduces the complexities associated with concurrency control.
Testing Concurrent Applications
Testing concurrent applications poses unique challenges. It is critical to ensure that the application behaves correctly under simultaneous thread access. Developers should employ specific strategies and tools to address these challenges:
- Unit Testing: Use frameworks like JUnit to write test cases that simulate concurrency. This helps to identify race conditions and deadlocks.
- Concurrency Testing Tools: Utilize tools like JMeter and ConTest for stress testing your application under load. This can reveal threading issues that may otherwise be missed.
- Code Review and Static Analysis: Engage in rigorous code reviews and leverage static analysis tools to identify potential concurrency problems before they become issues in production.
Proper testing techniques are paramount. They reduce the risk of issues arising in production, thus ensuring a stable user experience.
"The effectiveness of concurrency management directly impacts application performance and reliability."
In summary, integrating best practices in concurrent programming is essential for developing robust Java applications. Designing thread-safe classes, minimizing shared resources, and conducting thorough testing are foundational practices for success in this complex domain.
Emerging Trends in Java Concurrency
The landscape of Java concurrency is evolving. Developers must stay informed about the latest trends to optimize their applications. Emerging trends often focus on improving performance, enhancing code maintainability, and simplifying the development process. Adopting these new approaches can lead to more responsive applications and an overall boost in productivity for software development teams.
Reactive Programming
Reactive programming is a paradigm that deals with asynchronous data streams. It emphasizes the propagation of changes and offers a more dynamic approach to handling user interactions and event-driven programming. In Java, frameworks such as Project Reactor and RxJava facilitate the implementation of reactive programming. This model is particularly valuable in scenarios where applications need to handle a large number of concurrent users or processes efficiently.
Some benefits of reactive programming include:
- Responsiveness: Applications can react to events as they occur, leading to a more interactive user experience.
- Scalability: By decoupling data source and processing, reactive systems can scale better under load.
- Ease of composition: Operators provided in reactive libraries allow for elegant chaining of operations on data streams.
However, there are some considerations. Developers need to grasp the concepts of backpressure and how to handle it effectively to avoid overwhelming consumers.
Project Loom
Project Loom represents a significant shift in how Java manages concurrency. It introduces a new concurrency model that simplifies the development of high-throughput applications by enabling lightweight, user-mode threads. These threads, known as fibers, can replace traditional threads in many use cases while maintaining the performance benefits expected from the Java platform.
Key aspects of Project Loom include:
- Lightweight Fibers: Fibers allow developers to run millions of concurrent tasks without incurring the overhead associated with managing traditional Java threads.
- Continuation: Project Loom introduces the concept of continuations, making it easier to pause and resume computations without blocking resources.
- Simplified Programming Model: This project aims to integrate the fiber concept seamlessly into existing Java code, allowing developers to write more understandable asynchronous code.
As Project Loom continues to mature, it has the potential to change how Java applications are structured and developed. This can lead to a significant reduction in complexity and an increase in developer productivity.
Understanding emerging trends like reactive programming and Project Loom is essential for any developer looking to leverage concurrency effectively in Java.
End
The conclusion serves as a vital summarization of the key insights presented throughout the article on concurrent programming in Java. It allows readers to reflect on the significance of concurrency in modern software development and its integral role in enhancing application performance. Understanding concurrency enables developers to create robust applications that can handle multiple operations simultaneously, thus maximizing resource utilization and improving user experience.
Summary of Key Concepts
This article has covered several essential themes, primarily emphasizing the following points:
- Definition of Concurrent Programming: An overview of what concurrency entails and its relevance in programming.
- Multithreading Fundamentals: Exploration of threads, their lifecycle, and methods to create threads effectively, ensuring a comprehensive grasp of Javaâs multithreading capabilities.
- Synchronization Mechanisms: Importance of synchronization, including the distinction between synchronized methods and blocks, as well as advanced locking techniques.
- Concurrency Utilities: Introduction to the java.util.concurrent package and its features like the Executor Framework, which simplifies thread management.
- Handling Concurrency Challenges: Identification of common pitfalls such as deadlock and starvation, along with strategies to mitigate these issues.
- Best Practices: Recommendations for designing thread-safe applications, reducing resource contention, and effective testing methodologies.
- Emerging Trends: Insight into cutting-edge developments, including Project Loom and reactive programming, which are reshaping how concurrency is approached in Java.
The Future of Concurrency in Java
As software development continuously evolves, the future of concurrency in Java appears promising. Future advancements aim to simplify current concurrency models and make concurrent programming more accessible for developers. Project Loom, for example, proposes lightweight, user-mode threads, allowing applications to handle a significantly higher number of concurrent tasks without the complexities typically associated with traditional threading.
Also, the growing importance of reactive programming aligns with the need for systems that can adapt to changing resource availability and user demands dynamically. This paradigm shift is set to greatly enhance how developers approach concurrency, driving efficiency and performance.