Mastering Key JavaScript Concepts: A Developer's Comprehensive Guide
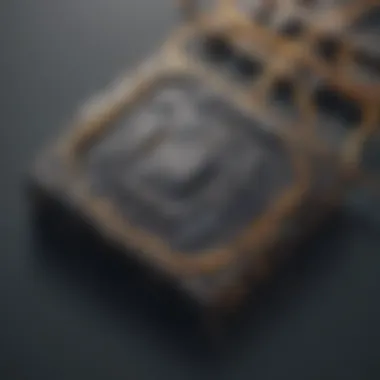
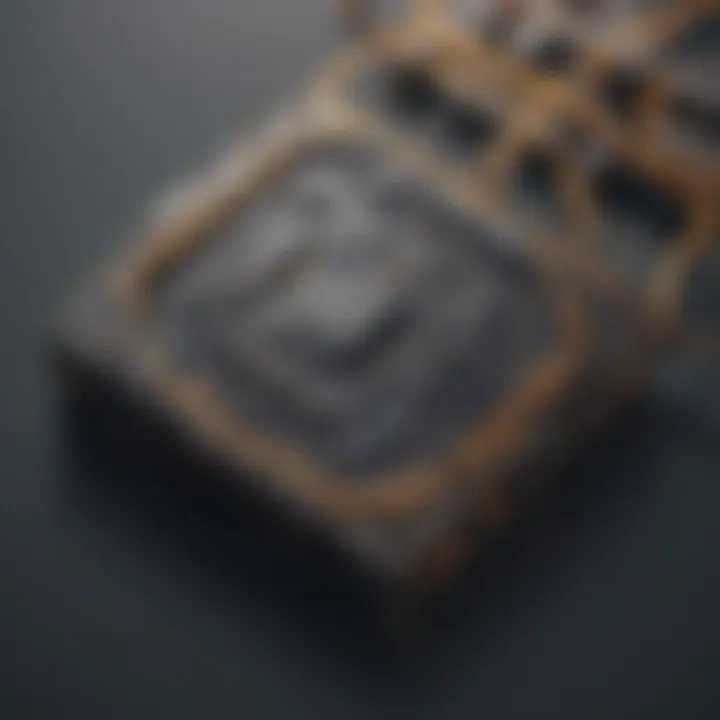
Overview of JavaScript Concepts
JavaScript is a versatile and powerful programming language that is essential for software developers aiming to build robust web applications. Understanding key JavaScript concepts is crucial for enhancing efficiency and optimizing code performance. From variable declaration to object-oriented programming principles, mastering these concepts is foundational in JavaScript development.
Fundamentals of JavaScript
Exploring the fundamentals of JavaScript involves grasping basic language constructs such as variables, data types, and operators. This knowledge forms the building blocks for more advanced concepts like functions, loops, and conditions. By learning how to manipulate data and control program flow effectively, developers can write concise and functional code.
Advanced Techniques in JavaScript
Advancing beyond the basics, software developers can delve into more sophisticated JavaScript techniques. This includes concepts like closures, prototypes, and asynchronous programming. By understanding these advanced topics, developers can optimize performance, manage state efficiently, and create responsive web applications.
Best Practices in JavaScript Development
Implementing best practices in JavaScript development is essential for producing high-quality code. Adhering to standards, utilizing modular code structures, and conducting thorough testing are crucial steps in ensuring code reliability and maintainability. By following industry best practices, developers can streamline development processes and minimize errors.
Common Pitfalls to Avoid
Despite its versatility, JavaScript development poses certain pitfalls that developers should be wary of. Common issues such as memory leaks, callback hell, and improper variable scoping can lead to inefficient code and performance bottlenecks. By identifying and avoiding these common pitfalls, developers can write cleaner, more efficient code.
Real-World Applications of JavaScript
JavaScript's versatility extends to a wide range of real-world applications, from interactive user interfaces to server-side development. Case studies showcasing successful implementations of JavaScript in e-commerce platforms, social media networks, and online games highlight its adaptability and efficiency. By examining these examples, developers can gain insights into best practices and optimization techniques.
Future Trends in JavaScript Development
As technology evolves, JavaScript continues to adapt and innovate. Emerging trends such as progressive web apps, serverless architecture, and artificial intelligence integration are shaping the future of JavaScript development. By staying informed about these trends and incorporating them into their workflow, developers can stay ahead of the curve and build cutting-edge web applications.
Practical Guides for JavaScript Development
To aid developers in mastering JavaScript concepts, practical guides and tutorials offer step-by-step instructions for applying key principles. These hands-on tutorials cater to beginners and advanced users alike, providing valuable tips and tricks for effective JavaScript utilization. By following these guides, developers can enhance their skills and stay abreast of the latest JavaScript developments.
Introduction to JavaScript
JavaScript serves as a fundamental pillar in modern web development. Understanding the nuances of JavaScript is paramount for software developers to craft interactive and dynamic web applications. This section will dissect the core principles of JavaScript, ranging from its inception to its current pivotal role in web development frameworks and libraries. An in-depth exploration of JavaScript's functionality, versatility, and ubiquitous presence in the digital landscape will provide valuable insights for aspiring and seasoned developers.
What is JavaScript?
JavaScript, often abbreviated as JS, is a versatile scripting language commonly utilized for client-side web development. Its ability to enhance web pages by enabling interactive elements and dynamic features sets it apart from traditional static websites. JavaScript empowers developers to manipulate webpage content, respond to user actions, and interact with external services, fostering an engaging user experience.
Evolution of JavaScript
Since its introduction in the mid-1990s by Brendan Eich, JavaScript has undergone significant evolution, transforming from a simplistic scripting language to a robust programming language. The evolution of JavaScript has been marked by the introduction of ES6 features, such as arrow functions, template literals, and classes, enhancing the language's readability and scalability. Understanding JavaScript's evolution provides developers with a historical context essential for harnessing its full potential.
Importance of JavaScript in Web Development
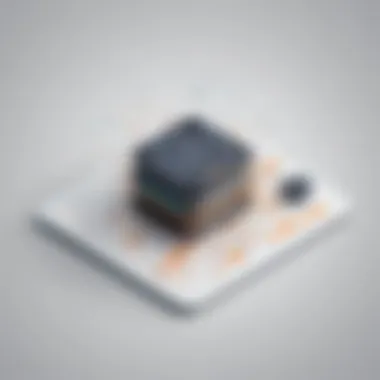
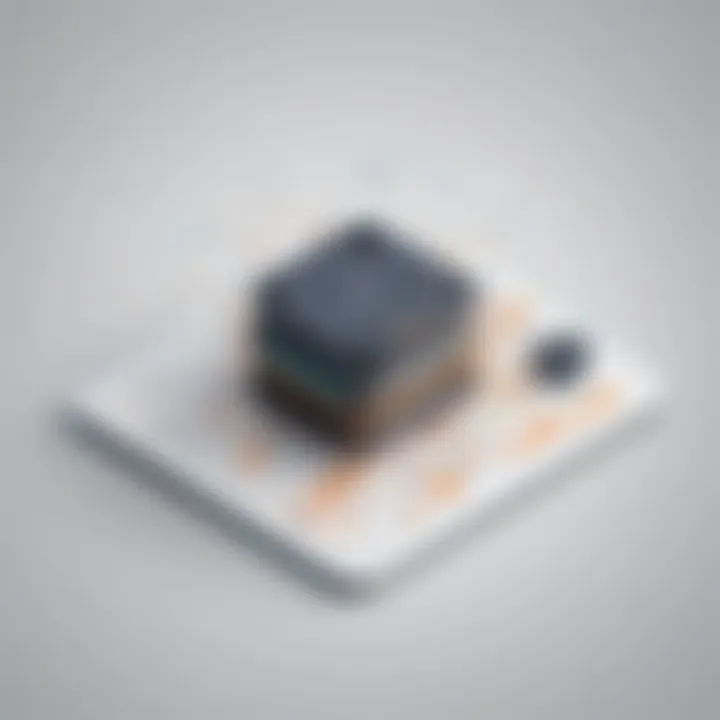
JavaScript stands as a cornerstone of web development, facilitating dynamic content creation, browser interactivity, and seamless user experiences. Its integration with HTML and CSS forms the bedrock of modern web applications, enabling responsive design and effortless data manipulation. Embracing JavaScript empowers developers to craft sophisticated web solutions, elevating user engagement and driving digital innovation.
Understanding JavaScript Fundamentals
Understanding JavaScript Fundamentals is crucial in the context of mastering key JavaScript concepts. This section serves as the bedrock for developers to grasp the core principles that govern the language. It delves into the fundamental building blocks that form the basis of JavaScript programming. By exploring Variables, Data Types, Operators, and Control Structures, developers gain a solid foundation for more advanced concepts.
Variables and Data Types
Primitive Data Types
Primtive Data Types in JavaScript play a vital role in defining the basic data units within the language. These data types include numbers, strings, booleans, null, and undefined. They are immutable, meaning their values cannot be changed once they are created. Despite their simplicity, primitive data types are essential for storing and manipulating basic values efficiently. Being lightweight and straightforward, they are ideal choices for fundamental programming tasks.
Complex Data Types
In contrast to primitive data types, complex data types in JavaScript are more sophisticated and can hold multiple values. Examples of complex data types are arrays and objects. These types allow developers to store and manage collections of data and complex structures. Although they are more advanced than primitive data types, complex types provide greater flexibility and functionality in handling complex data scenarios. However, they may require more memory and processing power compared to primitive types.
Operators and Expressions
Arithmetic Operators
Arithmetic operators in JavaScript are used for performing mathematical calculations such as addition, subtraction, multiplication, and division. These operators follow the standard rules of arithmetic, providing developers with the tools to manipulate numerical data effectively. They are highly useful in scenarios requiring quantitative computations and data manipulation tasks.
Comparison Operators
Comparison operators enable developers to compare two values and determine their relationship, such as equality, inequality, or relative size. These operators return boolean values based on the comparison results. They are essential for implementing conditional logic and decision-making processes in JavaScript programs, aiding in controlling the flow of program execution.
Logical Operators
Logical operators in JavaScript are used to combine and manipulate boolean values. These operators include logical AND, logical OR, and logical NOT. They are pivotal in evaluating multiple conditions and determining the resulting boolean value. Logical operators are fundamental for implementing complex logical operations and branching logic structures in JavaScript applications.
Control Structures
Conditional Statements
Conditional statements in JavaScript, such as ifelse and switchcase, allow developers to execute code based on specified conditions. These statements enable selective execution of code blocks, enhancing the flexibility and adaptability of JavaScript programs. By incorporating conditional statements, developers can create dynamic and responsive application behavior, tailoring the program's flow according to varying circumstances.
Loops
Loops play a crucial role in repetitive task execution within JavaScript programs. Examples of loops include for loop, while loop, and dowhile loop. They allow developers to iterate over data structures, perform batch operations, and automate tasks. Loops contribute to enhancing program efficiency and productivity by reducing manual repetition and enabling streamlined data processing.
Functions
Functions in JavaScript encapsulate reusable blocks of code that perform specific tasks. They enable developers to modularize code, promote code reusability, and improve code organization. Functions can take parameters, return values, and be invoked multiple times throughout a program. By leveraging functions, developers can achieve code abstraction, enhance maintainability, and streamline the development process.
Working with Objects and Functions
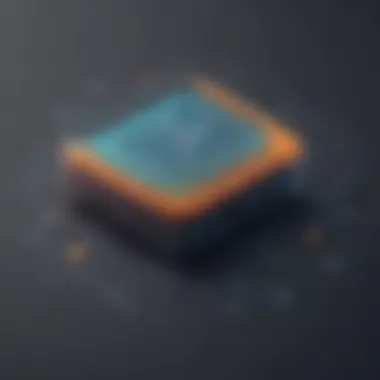
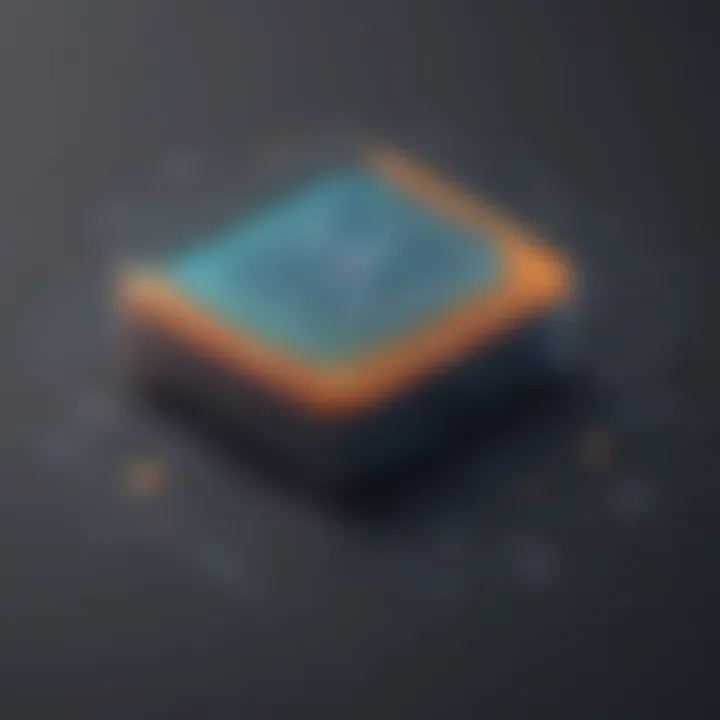
Working with Objects and Functions is a crucial aspect of this article, focusing on the foundational components that empower developers to create robust web applications. Understanding the principles behind object manipulation and function implementation is vital for mastering JavaScript. By exploring how objects and functions interact in code, developers can leverage the core concepts of JavaScript to their advantage. This section delves deep into the intricacies of object-oriented programming and function usage, providing a comprehensive guide for software developers striving to improve their coding skills.
Object-Oriented Programming in JavaScript
Creating Objects
Creating objects is a fundamental concept in JavaScript, allowing developers to encapsulate data and behavior within a single entity. The key characteristic of creating objects lies in their ability to represent real-world entities in code, enhancing the code's organization and readability. In this article, creating objects serves as a foundational building block for implementing object-oriented programming paradigms, fostering code reusability and modularity. Despite its advantages in structuring complex applications, creating objects may lead to potential issues related to memory management and performance optimization.
Prototypes
Prototypes play a significant role in JavaScript's object-oriented model by enabling object inheritance and behavior delegation. The distinctive feature of prototypes lies in their dynamic nature, allowing objects to inherit properties and methods from other objects. In this article, prototypes offer a powerful mechanism for sharing functionality among objects and defining object relationships efficiently. While prototypes promote code efficiency and flexibility, they require a clear understanding of prototype chain dynamics to avoid unintended consequences during code execution.
Inheritance
Inheritance is a fundamental concept in object-oriented programming that allows objects to acquire properties and behaviors from parent objects. The key characteristic of inheritance is its ability to establish hierarchical relationships between objects, facilitating code reuse and extension. In this article, inheritance serves as a cornerstone for building scalable and maintainable codebases, promoting the reuse of existing code and reducing development time. Despite its advantages in promoting code reusability, inheritance may lead to tight coupling between objects, making code maintenance challenging.
Functions in JavaScript
Function Declaration vs. Expression
The distinction between function declaration and expression is essential in JavaScript, impacting how functions are defined and hoisted within the execution context. Function declaration offers the advantage of hoisting, allowing functions to be called before they are defined in the code. In this article, understanding the nuances between function declaration and expression is crucial for implementing clean and efficient code structures. While function declaration simplifies function definitions, it may lead to potential naming conflicts and scope-related issues.
Closures
Closures play a vital role in JavaScript by capturing and preserving the lexical scope of a function, enabling functions to retain access to their enclosing scope's variables. The key characteristic of closures is their ability to maintain state across multiple function calls, promoting data privacy and encapsulation. In this article, closures offer a powerful mechanism for creating modular and reusable code components, enhancing code flexibility and security. While closures enhance code maintainability and flexibility, they may result in memory leaks if not managed properly.
Arrow Functions
Arrow functions are a concise way to define functions in JavaScript, offering a more compact syntax compared to traditional function expressions. The key characteristic of arrow functions lies in their lexical this binding, simplifying the handling of function context within callbacks and event listeners. In this article, arrow functions provide an elegant solution for writing concise and readable code segments, improving code readability and maintainability. While arrow functions streamline function definition processes, they have limitations concerning their binding behavior and may not be suitable for all use cases.
Advanced JavaScript Concepts
In this pivotal section of the guide on mastering JavaScript concepts, the focus shifts towards advanced topics that play a crucial role in enhancing a developer's expertise. Getting to grips with advanced JavaScript concepts is essential for software developers looking to elevate their coding capabilities and build more robust applications. By delving into advanced concepts, developers can explore intricate aspects of JavaScript that enable them to solve complex problems more efficiently. Understanding advanced JavaScript concepts provides developers with a strategic edge in creating cutting-edge solutions that surpass conventional coding practices.
Asynchronous JavaScript
Callbacks
Callbacks in JavaScript are a fundamental aspect of handling asynchronous operations, allowing developers to execute functions once certain tasks are completed. The key characteristic of callbacks lies in their ability to manage tasks that may take varying amounts of time to finish, ensuring that code execution proceeds without delays. Callbacks are a popular choice in JavaScript development due to their asynchronous nature, enabling developers to write non-blocking code and improve the efficiency of their applications. While callbacks offer flexibility and speed in executing tasks, they can lead to callback hell and make code harder to maintain in complex scenarios.
Promises
Promises in JavaScript offer a more structured approach to managing asynchronous operations, providing a cleaner alternative to callback functions. The key characteristic of promises is their ability to represent a value that may be available in the future, allowing developers to handle success or failure outcomes asynchronously. Promises are a beneficial choice for this article as they simplify asynchronous coding and make it easier to chain multiple asynchronous operations. The unique feature of promises lies in their capability to handle errors more gracefully than traditional callbacks, enhancing code readability and maintainability.
AsyncAwait
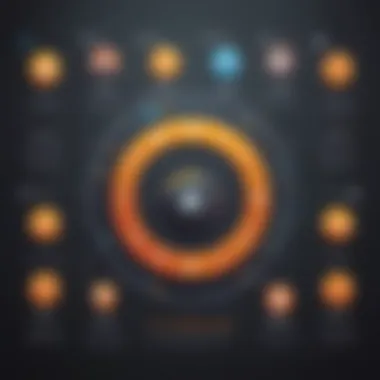
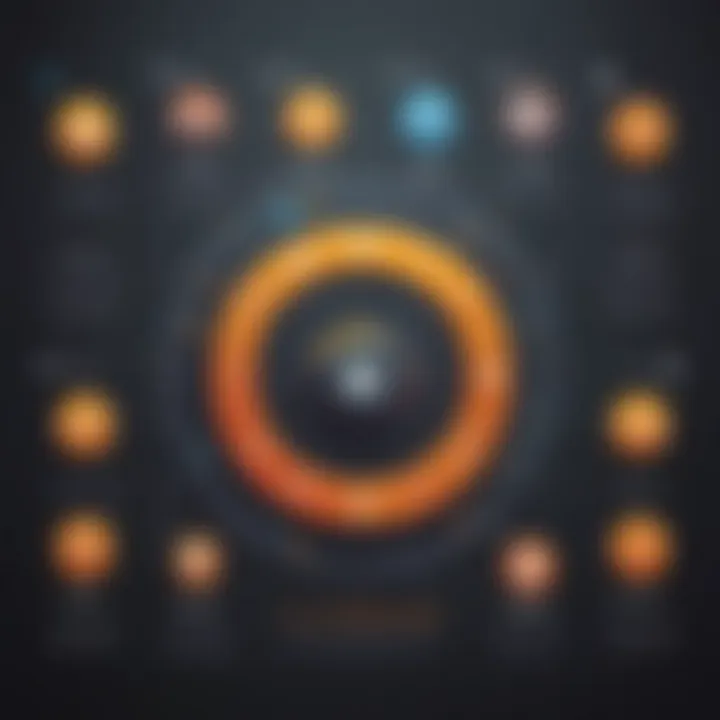
AsyncAwait is a recent addition to JavaScript that simplifies working with asynchronous functions by using asynchronous code in a synchronous manner. The key characteristic of asyncawait is that it allows developers to write asynchronous code that resembles synchronous code, enhancing readability and reducing complexity. Asyncawait is a popular choice in modern JavaScript development for its ability to streamline asynchronous operations and make code easier to understand. The unique feature of asyncawait lies in its ability to simplify error handling in asynchronous code, making it easier to write and maintain complex asynchronous functions.
Error Handling and Debugging
TryCatch
Integrating trycatch blocks in JavaScript allows developers to handle errors and exceptions gracefully, ensuring that code execution continues even in the presence of unexpected issues. The key characteristic of trycatch is its ability to intercept and manage errors within a specific block of code, preventing them from crashing the entire application. Trycatch is a beneficial choice for this article as it empowers developers to proactively address potential errors and enhance the overall robustness of their applications. The unique feature of trycatch lies in its capacity to provide a structured approach to error handling, enabling developers to catch and handle specific types of errors effectively.
Error Objects
Error objects in JavaScript play a crucial role in providing detailed information about runtime errors, aiding developers in identifying and resolving issues in their code. The key characteristic of error objects is their ability to encapsulate information about an error, including its type and message, for diagnostic purposes. Error objects are a popular choice in JavaScript development for their informative nature, helping developers pinpoint the root cause of errors and streamline the debugging process. The unique feature of error objects lies in their standardized format and the wealth of data they offer, facilitating efficient error tracking and resolution.
Debugging Strategies
Effective debugging strategies are essential for software developers to identify and rectify errors in their code efficiently. The key characteristic of debugging strategies is their systematic approach to troubleshooting, enabling developers to isolate and fix issues methodically. Debugging strategies are a beneficial choice for this article as they equip developers with a toolkit of techniques for uncovering and addressing bugs, ensuring the smooth functioning of their applications. The unique feature of debugging strategies lies in their adaptability to diverse scenarios, allowing developers to employ a range of methods such as console logging, breakpoints, and testing frameworks based on the nature of the problem.
Modules and Packaging
CommonJS vs. ES6 Modules
Exploring the differences between CommonJS and ES6 modules is vital for understanding how JavaScript manages module dependencies and organizes code. The key characteristic of CommonJS and ES6 modules lies in their respective approaches to defining and importing modules, offering flexibility and modularity in structuring code bases. CommonJS vs. ES6 modules is a significant choice for this article as it sheds light on the evolving standards of JavaScript module systems and their impact on code reusability and maintainability. The unique feature of CommonJS and ES6 modules lies in their compatibility with different environments and the sophistication they bring to module management in JavaScript development.
Module Bundlers
Module bundlers like Webpack and Rollup are instrumental in optimizing JavaScript code by bundling modules together for efficient delivery to users' browsers. The key characteristic of module bundlers is their ability to combine separate modules into a single file, reducing network requests and improving application performance. Module bundlers are a popular choice in modern web development for their role in simplifying module management and enhancing code organization. The unique feature of module bundlers lies in their support for various integrations and plugins, allowing developers to customize the bundling process to suit their specific project requirements.
Package Managers
Package managers such as npm and yarn streamline the process of installing, managing, and sharing JavaScript packages, facilitating dependency management and project collaboration. The key characteristic of package managers is their central repository of packages, enabling developers to access a vast ecosystem of libraries and tools with ease. Package managers are a beneficial choice for this article as they enhance productivity by automating package installation and version control, simplifying project setup and maintenance. The unique feature of package managers lies in their support for semantic versioning and dependency resolution, ensuring that projects stay up-to-date and free from compatibility issues.
Optimizing JavaScript Performance
In the realm of web development, optimizing JavaScript performance stands as a crucial pillar. Its significance in this comprehensive guide cannot be overstated, as efficiency in code execution directly impacts user experience and website responsiveness. When delving into the nuts and bolts of optimizing JavaScript performance, several key elements warrant attention. From streamlined code execution to reduced loading times, focusing on performance optimization ensures that web applications function seamlessly regardless of user interactions or devices. By exploring the nuances of JavaScript performance optimization, developers can fine-tune their code to deliver superior and agile web experiences.
Code Optimization Techniques
Minification
Minification plays a pivotal role in optimizing JavaScript performance within the context of this article. The primary essence of minification revolves around compressing code by removing unnecessary characters such as white spaces and comments. This process results in leaner code that loads faster, thus enhancing overall website performance. By embracing minification, developers can significantly reduce file sizes, leading to expedited loading times and improved website responsiveness. One key characteristic of minification lies in its ability to enhance code readability and maintainability while ensuring optimal performance. The benefits of minification cannot be undermined, as it empowers developers to deliver high-performing web applications efficiently, making it an indispensable choice for this comprehensive guide.
Caching
Within the spectrum of optimizing JavaScript performance, caching plays a pivotal role in augmenting website speed and responsiveness. Caching involves storing previously accessed data temporarily, enabling swift access upon subsequent requests. One key characteristic of caching is its ability to minimize redundant requests, thereby accelerating data retrieval and enhancing overall website performance. Embracing caching mechanisms empowers developers to leverage stored data efficiently, resulting in faster load times and improved user experience. However, developers must tread carefully, as improper caching strategies may lead to outdated content delivery or compromised data integrity. By strategically implementing caching mechanisms, developers can harness its advantages while mitigating potential drawbacks within the realm of this article.
Lazy Loading
Lazy loading emerges as a prominent technique for optimizing JavaScript performance, particularly within the purview of this article. The essence of lazy loading lies in deferring the loading of non-essential resources until they are required, thereby reducing initial loading times and conserving bandwidth. A key characteristic of lazy loading is its ability to prioritize essential content, ensuring seamless user interactions and enhancing overall website performance. By adopting lazy loading strategies, developers can strike a balance between resource optimization and user experience, resulting in agile and high-performing web applications. Understanding the unique features of lazy loading and its impact on performance optimization is crucial in delivering efficient and responsive websites tailored for the modern digital landscape.
Browser Rendering and Execution
In the dynamic realm of web development, browser rendering and execution play pivotal roles in determining website performance and user experience. By unraveling the intricacies of browser rendering and execution, developers gain insights into optimizing JavaScript performance and delivering fluid user interactions. Within this article, a closer examination of critical rendering path, rendering optimization, and efficient DOM manipulation sheds light on strategies to enhance website responsiveness and efficiency.