Mastering Go Programming: An In-Depth Guide
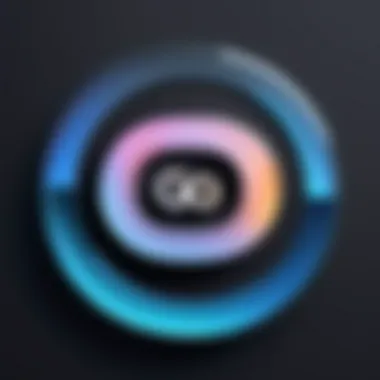
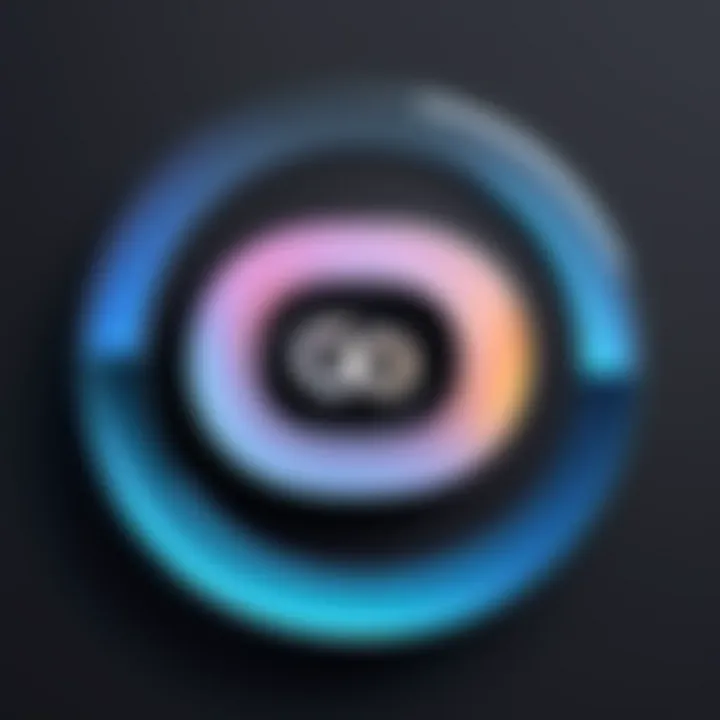
Intro
In the ever-evolving realm of technology, programming languages become akin to tools in a craftsman's shed, each serving a particular function. Go, or Golang as it's often called, has emerged as a bespoke tool suited for various applications, especially in a world increasingly leaning towards efficiency and performance. Its design philosophy is one of simplicity and clarity, making it an appealing choice for both seasoned developers and fresh-faced novices eager to dip their toes into the waters of programming.
Understanding Go is not merely about grasping syntax or coding conventions; it involves appreciating the principles underlying software development as a whole. As industries pivot towards cloud computing, data analytics, and machine learning, Go positions itself as robust yet nimble, demonstrating flexibility that can meet diverse programming requirements. Its concurrency model and performance efficiencies offer unique advantages, particularly for distributed systems and networked applications.
This guide endeavors to peel back the layers of Go, detailing its features, practical uses, and the best ways to learn it. We will explore Go's applicability within various technological domains and provide insights gleaned from industry standards and real-world applications. Each section is crafted with the intent of equipping readers with the knowledge and skills required to leverage Go effectively, setting a solid groundwork for further exploration in their programming journey. In doing this, we’ll unlock the path that can lead to mastering not just Go, but a broader understanding of the software development landscape.
Stay tuned as we embark on this comprehensive journey, diving into key elements that will enhance your understanding and application of Go in today's fast-paced tech environment.
Preface to Go
As the tech landscape continuously evolves, understanding programming languages that excel in performance, simplicity, and efficiency is vital for developers. Go, designed and developed at Google, has emerged as a powerhouse in the programming sphere since its inception in 2007. This section serves as a gateway to grasp the fundamentals of Go, shedding light on its evolution, unique characteristics, and why it has quickly become a go-to language for many.
In looking at Go, you’ll find not just another programming tool but rather a robust framework allowing seamless collaboration across various sections of software development. The language’s emphasis on readability and minimalism accelerates the development process, making it particularly attractive to novices and experienced coders alike. Understanding where this language comes from and what it offers is essential for anyone wishing to harness its power.
"The future belongs to those who believe in the beauty of their dreams." - Eleanor Roosevelt
This aphorism captures the essence of embarking on a journey with Go. Familiarity with its history and key characteristics sets the stage for a fruitful programming experience.
History and Evolution of Go
To appreciate Go fully, it’s beneficial to trace its history. The language was developed by Robert Griesemer, Rob Pike, and Ken Thompson, largely due to the frustrations they experienced in software development at Google. In trying to tackle the challenges posed by existing languages like C++, they sought to create a language that combines the efficiency of C with the simplicity of Python.
The first public version of Go was released in 2009, capturing the attention of the developer community. Its design includes garbage collection, concurrent programming features, and a strong emphasis on simplicity. Throughout the years, Go has seen numerous updates that have expanded its application, integrating deeper into cloud computing, web servers, and distributed systems. Today, it is pivotal in supporting large-scale infrastructure at various organizations, showcasing its resilience as a primary choice in backend development.
Key Characteristics of Go
Identifying what sets Go apart from its competitors is crucial. Its main characteristics include:
- Simplicity and Readability: Go’s syntax is straightforward, thereby reducing the cognitive load on developers. It promotes clean code that is easy to understand and maintain.
- Concurrency: One of Go’s flagship features is its built-in support for concurrent programming through goroutines and channels. This allows developers to execute multiple tasks simultaneously, efficiently handling operations such as web requests.
- Strong Standard Library: The wealth of built-in packages provides many tools to fulfill various needs without relying on external libraries. It streamlines development by simplifying everything from file handling to web server setups.
- Cross-platform Compatibility: Go enables developers to build applications that compile to native binaries for numerous operating systems, facilitating broader deployment options.
Understanding these elements is key to leveraging Go’s full potential for your development needs. As we dive deeper into the realm of Go programming, this foundational knowledge will serve as the bedrock for more complex concepts and practices.
Setting Up Your Development Environment
Setting up a development environment is often overlooked, yet it is crucial for working efficiently with programming languages like Go. Imagine trying to build a house without first laying a sturdy foundation. The same principle applies to software development. A proper environment not only helps in avoiding unnecessary headaches later on but also contributes significantly to your productivity.
When starting with Go, the emphasis should be on creating a space where you can comfortably stream together code, test it, and ultimately bring your applications to life. By understanding how to install Go properly and choosing the right Integrated Development Environment (IDE), you lay down the groundwork for a smoother programming experience.
Installing Go
The first step in establishing your Go programming environment is to properly install the Go programming language itself. The installation process is straightforward, but there's a systematic approach that can save time and effort in the long run.
- Download: Visit the official Go website to get the installation package appropriate for your operating system. Whether it’s Windows, macOS, or Linux, they provide a tailored installer that simplifies the whole ordeal.
- Installation: Run the installer and follow the prompts. Most of the time, it’s just clicking “Next” a few times. But hold on! Pay attention to the default installation path, as you may need it later.
- Setting Up Environment Variables: This is one of those details that could trip you up. After installation, you need to set the and add if it’s not automatically set. For instance, on Windows, you could go to System Properties > Advanced > Environment Variables. Ensure your Go bin path is added to your system's PATH variable. This setup not only allows you to run Go commands from the command line but also helps in organizing your projects.
- Verification: Finally, it’s prudent to confirm whether Go has been installed correctly. Open a terminal or command prompt and enter the command:If everything is in order, you will see the current Go version displayed. This small step helps in checking that there are no misconfigurations.
By following these steps, you will have Go up and running in no time, paving the way for the real adventure ahead – programming.
Choosing an Integrated Development Environment (IDE)
Choosing the right IDE can be as critical as the programming language itself. An IDE is not merely a text editor; it’s an ecosystem that can define how easily and efficiently you can write your code.
Go developers have a few popular choices: Visual Studio Code, GoLand, and Atom among others. Each IDE comes with its own set of features, but some key elements should guide your selection:
- Ease of Use: Opt for an IDE that feels intuitive. If it takes more than a few minutes to figure out how the interface works, it might not be the right tool for you.
- Support for Go Features: Look for IDEs that have extensions for Go. Features like code completion, debugging, and testing within the IDE can significantly increase your productivity.
- Community Support: An IDE backed by a strong community means you can find solutions quickly and share ideas with other developers. This can be a lifesaver when you hit a stumbling block.
Here are a few options worth considering:
- Visual Studio Code: This free and open-source editor is lightweight and has numerous extensions available for Go.
- GoLand: A paid option designed specifically for Go developers, offering advanced tools and an integrated experience.
- Atom: Another free text editor that is customizable and has community-contributed packages for Go.
Remember that you can change your IDE later on; the important thing is to begin with something that makes you feel comfortable.
"The right environment sets the stage for the right mindset."
Basic Syntax and Data Types
Understanding the basic syntax and data types in Go is akin to laying the groundwork of a sturdy building. If these foundational elements are clear, the structure that follows becomes more solid and easier to navigate. In programming, syntax rules are the language's grammar; they dictate how various symbols can combine to create meaningful expressions. Learning these elements is crucial as it enables developers to read and write code effectively. Moreover, familiarity with data types—like integers, strings, and arrays—allows developers to manipulate information accurately and perform operations on those data types seamlessly.
Understanding Go’s Syntax
Go adopts a clean and concise syntax that stands out significantly from many other programming languages. What makes it appealing is how straightforward it is, allowing one to focus more on the logic behind the programming rather than getting bogged down in complicated syntax rules. For instance, declaring a variable in Go can be done in a single line, like so:
This flexibility in declaration—using the keyword or even the shorthand —makes Go versatile yet robust. The syntax uses braces for defining code blocks, like in most C-based languages, which helps in organizing code and making it visually approachable:
In addition, Go emphasizes the significance of explicit declarations. For instance, when defining a function, you must specify the return type. This requirement ensures clarity and helps maintain strict data types throughout. Such design principles contribute not only to Go's readability but also elevate code quality, which effectively reduces errors when developing larger applications.
In traditional programming contexts, ambiguity can lead to unintended consequences; however, Go's syntax encourages clarity, a vital trait in collaborative environments. Thus, getting a handle on Go's syntax early on can save you from future headaches.
Exploring Data Types and Variables
Data types in Go are the various formats in which information can be stored and manipulated in your code. They come in different shapes and sizes, each serving specific purposes. Understanding these types is crucial for effective programming.
Go supports several fundamental data types such as:
- Integers (both signed and unsigned)
- Floats (for decimal numbers)
- Booleans (true or false)
- Strings (sequences of text)
- Arrays and Slices (collections of data)
For example, integers can be declared like this:
Or, you can use a slice to store a list of values, making it easier to manage dynamic data:
Variables act as placeholders for these data types, allowing you to store values and reference them throughout your program. Apart from using , the shorthand is very handy when you want to save some time, especially while writing functions or loops.
In Go, variables must be of a defined type, and this approach minimizes type-related errors, which can become bothersome in loosely typed languages. If you try to assign a string to an integer variable, Go will flag an error. This points to a larger benefit—Go's strong typing system encourages better code practices and leads to more maintainable applications.
Another key feature is that Go allows for type inference. When you initialize a variable with a value, Go intelligently infers its type. This can cut down on verbosity without sacrificing clarity, yet it still maintains that strong type enforcement.
Control Structures in Go
Control structures in Go are the bedrock of programming logic. They help you dictate the flow of execution in your code, transforming simple operations into intelligent sequences of actions based on varying conditions. If you want to craft applications that behave differently in response to input or events, understanding control structures is not just beneficial; it's imperative. In this part of the guide, we will explore how conditional statements and loops help shape the behavior of your programs, paving the way for dynamic efficiency in your coding journey.
Conditional Statements
Conditional statements in Go allow your code to make decisions. The primary player here is the statement, but it doesn't play solo—it's backed up by and . The beauty of these statements lies in their simplicity and power. When you run your code, these statements evaluate conditions and execute specific blocks contingent upon whether those conditions are true or false.
For example, consider a situation where you want to check if a user is eligible to vote:
In this code snippet, the checks the age against the voting age. If the age is 18 or more, it prints that the user is eligible. Otherwise, it tells them they can’t vote yet. This simple structure leads to clear, concise decision-making, which can then be built upon.
Moreover, the statement provides an efficient way of handling multiple cases without the clutter of many constructions. This can improve the readability of your code and make it easier to maintain.
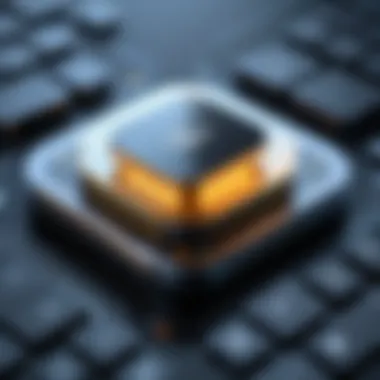
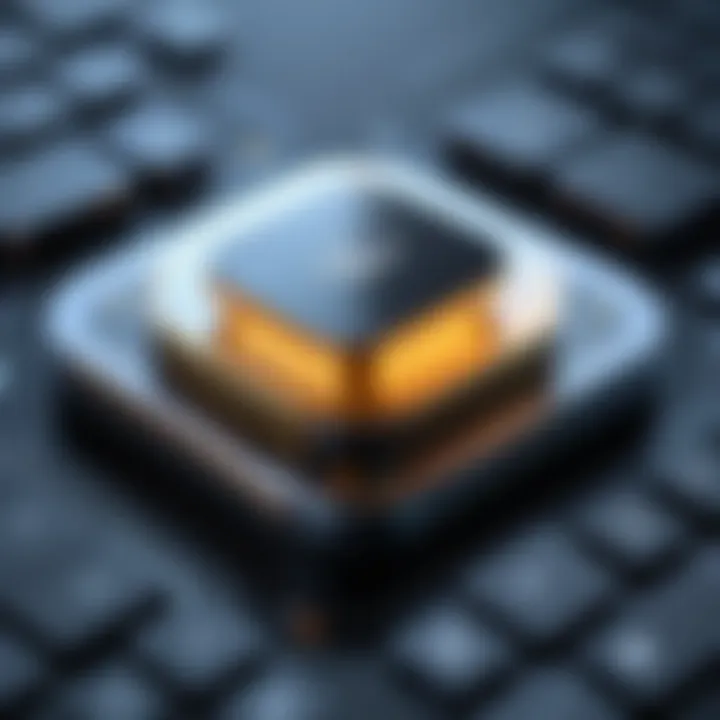
Loops and Iteration
Loops are the backbone of repetition in programming. In Go, the loop is your go-to tool for executing a block of code multiple times. The loop can take on several forms: a standard counter loop, a loop that continues until a condition is met, or even an infinite loop if you’re not careful.
A classic example of a counter loop might be iterating across an array:
Here, we use to go through each number in the slice. The loop prints the index and value, illustrating how you can succinctly work through collections of data.
Additionally, Go allows you to create a loop effect using the statement, leading to flexible control over iterations:
In this case, the loop runs until exceeds 5, demonstrating how you can base the continuation of your loop on varying conditions.
Understanding how to wield control structures effectively equips you with the tools to handle complex logic flows. The myriad possibilities you hold—with statements and loops—allow you to construct programs that can learn, adapt, and respond in real-time. The foundation laid by these fundamental constructs is not just a stepping stone; it’s the very essence of programming with Go.
Functions and Methods
Functions and methods are the bedrock of Go programming, shaping how developers craft reusable code blocks that contribute to clarity and efficiency. In the dance of programming, understanding these two concepts is akin to learning the steps before hitting the floor. This section aims to demystify functions and methods, showcasing the nuances that distinguish them while emphasizing their integral role in Go programming.
When discussing functions and methods, it’s essential to grasp their benefits. Functions serve as standalone blocks that perform specific tasks, while methods, being closely tied to types, introduce a degree of context that aids in encapsulation. This ability to group behaviors with data not only enhances code organization but also fosters modularity. Moreover, mastering functions and methods leads to cleaner, more maintainable code, which is cardinal for any project that aims for longevity in a dynamic tech world.
Defining Functions in Go
In Go, defining a function is a straightforward affair, yet the simplicity belies its power. A function in Go is defined using the keyword followed by a name, parameters, and a return type. The structure goes:
For instance, consider a function that adds two integers:
When you call , it returns , showcasing how functions can encapsulate logic and increase code reusability without cluttering the main codebase. One pertinent aspect to note is that Go supports multiple return values, allowing a function to return more than one result seamlessly. This feature is particularly handy when dealing with operations that need to return both data and an error, a common occurrence in Go.
It’s also wise to keep in mind some best practices. Aim to use clear, descriptive names for your functions; this clarity pays off when others (or even you in a few months) read your code. Avoid overly complex functions; instead, strike a balance by keeping functions focused on a single responsibility.
Understanding Method Receivers
Diving deeper, we find that methods in Go add another layer of sophistication. While similar to functions, methods are distinctly tied to types. A method is defined by associating it with a type through a receiver, enabling it to act on the data of that type. To define a method, you include the receiver in the method signature:
For example, you can define a method on a struct to calculate area:
In this case, operates on the instance it belongs to, making it evident how methods enhance functionality by utilizing data context. Moreover, Go allows methods to have pointer receivers, which can prevent unnecessary data copies, improving performance.
Understanding when to use functions versus methods revolves around design considerations. If general functionality is needed, functions suffice; however, if there's a need to manipulate or operate on an object’s state, methods should be your go-to.
"In programming, clarity often trumps cleverness; write to be understood, not to impress."
Structs and Interfaces
In the world of Go programming, understanding structs and interfaces is akin to grasping the essence of the language. Structs allow you to create complex data types that group together related fields, while interfaces facilitate a powerful way to define behavior without tying it to specific implementations. This duality is essential when developing robust and maintainable applications.
Working with Structs
Structs in Go serve as the building blocks for creating complex data structures. Think of a struct as a blueprint for a unique data type, much like a recipe that outlines the ingredients and steps needed to create a dish. A struct can contain multiple fields of varying types, which can be used to encapsulate related data.
Here’s a simple example of how a struct might be defined:
With the struct defined, you can now create instances of it:
This encapsulation not only organizes data but also simplifies its manipulation within your program. Additionally, structs can have methods associated with them, allowing for a clean and intuitive way to define behavior. For example:
By structuring your data like this, you're setting the stage for organized and maintainable code. However, one should consider:
- Field Visibility: Begin field names with uppercase letters to export them beyond the package. For instance, is exported while isn’t.
- Nested Structs: Go allows structs to contain other structs, enabling you to model complex relationships effectively.
Defining and Implementing Interfaces
Interfaces in Go offer a way to define a set of methods that a type must implement. They abstract behavior from concrete data types, promoting flexibility and allowing for interchangeable parts in your code. An interface can be regarded as a contract; a type that conforms to this contract must furnish implementations for the methods declared in the interface.
A practical example of an interface is:
Any type that includes a method with the proper signature fulfills this interface. This is useful for building applications that require pluggable components.
This kind of abstraction allows you to write functions that can accept any type that meets the interface's requirements, making your codebase more modular. Plus, Go's interfaces are implicitly satisfied. You don't have to declare that a type implements an interface. If it has the necessary methods, it does – it's that simple.
Now, there are a couple of considerations:
- Empty Interfaces: An empty interface can hold values of any type, making it a catch-all, but use it sparingly. It might lead to less type safety.
- Interface vs Struct: Use structs for data representation and interfaces for behavior specification. Keeping this distinction in mind will help you write clearer code.
The relationship between structs and interfaces is crucial for solid Go programming, marrying data with behavior and paving the way for scalable and maintainable software development.
"Structs and interfaces are like the two sides of a coin in Go programming; they complement each other to enable powerful abstractions and data modeling."
Understanding how to leverage both effectively can turn a good programmer into a great one.
Concurrency in Go
In contemporary software development, where responsiveness and efficiency reign supreme, concurrency emerges as a cornerstone of robust programming practices. Go, a language engineered by Google, leverages the concept of concurrency like no other. It provides a rich set of tools for developers to design applications that can execute multiple tasks simultaneously, a feature increasingly necessary in our multi-core, distributed computing world.
Concurrency is not about parallelism; it’s about structuring your code to manage multiple tasks effectively.
Understanding Goroutines
At the heart of Go's concurrency model lies the goroutine. A goroutine is a lightweight thread managed by the Go runtime. Unlike traditional threads, goroutines are easy to create and have a small overhead. You can think of them as executable functions that run independently, which makes Go handle tasks concurrently with minimal fuss.
Creating a goroutine is as simple as prefixing a function call with the keyword. Here’s a quick example:
In this snippet, runs as a goroutine, allowing the main function to continue executing. This simplicity does come with its nuances, however. Since goroutines operate outside the typical function call stack, it can introduce issues such as race conditions. A race condition occurs when two or more goroutines try to access the same resource simultaneously without proper synchronization. Developers therefore must be vigilant and often implement access control mechanisms, such as channels or mutexes.
Working with Channels

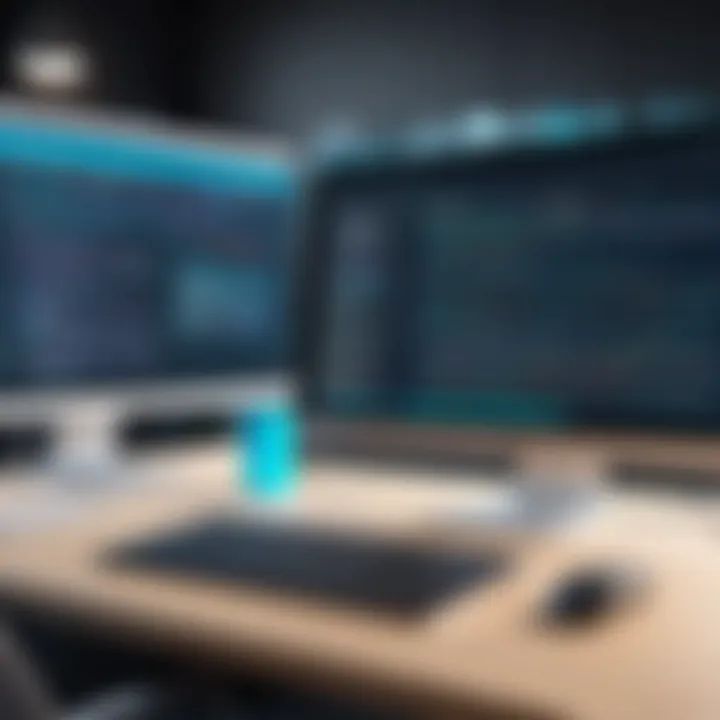
Channels are the lifeline for goroutines when it comes to communication and synchronization. Think of channels as conduits through which goroutines can send and receive messages, allowing for safe data exchange. The beauty of channels is that they help prevent race conditions, as they elegantly manage how and when data is shared between goroutines.
A simple illustration of a channel:
In the code above, a channel named is created, allowing one goroutine to send a message and the main function to receive it. This prevents data corruption often seen in concurrent systems.
It's crucial to note the benefits of buffered versus unbuffered channels. An unbuffered channel requires both sending and receiving goroutines to be ready at the same time, effectively synchronizing them. In contrast, buffered channels allow a specified number of messages to be stored until they are read, which can smoothen communication flow in certain situations and potentially lead to more efficient processing.
In summary, Go's concurrency model, driven primarily by goroutines and channels, offers developers a powerful toolkit to build highly responsive applications. Understanding how these components interact and recognizing the importance of synchronization are essential aspects of mastering Go's concurrency. Whether you’re building web servers or data processing pipelines, leveraging Go’s concurrency will often lead to more performant, scalable, and maintainable applications.
Error Handling
Error handling isn't just another checkbox in the programming checklist; it's a fundamental aspect that can significantly influence the robustness and reliability of applications. In the realm of Go programming, understanding how to manage errors effectively can be the difference between a flourishing application and one riddled with unexpected crashes. Hence, error handling is paramount in ensuring that your code behaves predictably even when things do not go as planned. Acknowledging this reality helps developers preempt failures rather than trying to fix them post-factum.
Go’s Approach to Error Management
In Go, errors are regarded as values. This design principle is quite distinct from some programming languages where exceptions are thrown. When a function encounters an issue, it returns an error type that indicates what went wrong. This approach encourages developers to handle potential failure points diligently.
For instance, consider the following code snippet:
In this example, the function attempts to open a file and returns an error if the file does not exist. The calling function checks for that error, embodying Go’s philosophy of explicit error handling. This transparency promotes better debugging and ultimately leads to more maintainable code.
Best Practices in Error Handling
Navigating the waters of error handling can be tricky, yet following certain best practices can smooth out the ride. Here are some key points to consider:
- Check for Errors Immediately: Always check function return values for errors right after the call. Delaying error checks can make it hard to trace where the failure is coming from.
- Wrap Errors: When propagating errors in Go, it's often beneficial to wrap an error with context before returning it. This can provide valuable debugging information:
- Log Meaningful Messages: Instead of simply logging the error, include relevant context. A well-structured log can save time and effort later on.
- Avoid Ignoring Errors: It’s easy to get into the habit of ignoring certain errors, especially when you think they might be insignificant. However, even small issues can cascade into larger, less manageable problems.
- Use Custom Error Types: For applications with complex error handling needs, consider defining custom error types that can encapsulate more context or status codes.
Ultimately, error handling in Go promotes a culture of diligence among developers. Error management isn’t simply about making the code work; it's about ensuring the code works safely and predictably, which is especially critical in larger, more complex systems. Following these practices can elevate not just the readability of your code but also its functionality in practical applications.
"In programming, the focus should not only be on writing the code but also on how that code behaves in face of errors."
Testing in Go
Testing is one of those often-overlooked aspects of programming that can make or break a project. In Go, testing is not just a good practice; it’s essential to building reliable software. Developers need to ensure that their code behaves as expected under a variety of scenarios. This not only increases confidence in the codebase but also significantly reduces the chances of surprising bugs showing up in production.
The Go programming language places a strong emphasis on testing, integrating it into the development process from the start. This means that writing tests isn’t an afterthought; it’s part and parcel of building an application. This section will delve into the nitty-gritty of writing tests and utilising Go's built-in testing package effectively.
Writing Tests in Go
When you sit down to write tests in Go, you’ll quickly discover the language makes it quite straightforward. Go uses a unique approach called "table-driven tests." This is where you define a slice of test cases and iterate through them to run your tests. This not only keeps your code clean and concise but also allows for easy maintenance. With this methodology, you can easily add new cases just by modifying a single slice.
Here’s a simple example of how you might structure a test:
In the snippet above, is a hypothetical function to sum two integers. Each test case is neatly organized into a struct, making it clear what input is being tested and what the expected outcome is. Keeping it simple like this avoids the pitfalls of cumbersome test logic.
Using Go’s Testing Package
Go’s native testing package offers a wealth of functionalities that can aid you in your quest for quality assurance. The package provides everything from simple assertions to benchmarks, making it an all-in-one solution for typical testing needs. By utilizing this package, developers can easily generate test reports and identify bottlenecks in performance.
A key feature of the testing package is its ability to run tests in parallel. This is especially useful for improving performance, particularly if you are running a large suite of tests. The method can be called within your test function to enable this. Simply add it at the start of your test function, and Go takes care of the rest, running tests concurrently.
Additionally, you can create custom test reports that can be quite handy when tracking regressions or performance issues. Going beyond standard output doesn’t require much effort, as you can direct the results of your calls to a file or another output stream if needed.
To wrap things up, testing in Go is not just about checking if your code works; it’s about ensuring it continues to operate correctly as it evolves. By writing structured tests and leveraging Go’s robust testing package, developers can significantly enhance their code quality, making testing an indispensable part of their programming toolkit.
"Reliable software is not written; it is tested."
With a solid grasp on how to write tests and use Go's testing tools, you're well on your way to becoming a proficient Go developer with a quality product.
Summary
Thus, with the right approach to testing in Go, you can maintain high standards of code integrity. It’s about preemptively catching issues, ensuring smooth operation, and ultimately delivering a product that you can be proud of.
Building Web Applications with Go
Building web applications with Go is not just about writing code; it's about crafting efficient, robust, and scalable solutions that can hold their own in today's competitive landscape. Go has been widely acknowledged for its performance and ease of use, making it an ideal choice for developers looking to create web services and applications. This section will explore various elements of building web applications in Go, including setting up a web server and understanding HTTP handlers, to illuminate why Go stands out in this domain.
Setting Up a Web Server
The first step towards constructing a web application using Go is setting up a web server. Unlike some programming languages that require extensive frameworks to get a server running, Go’s built-in package, , simplifies this process remarkably.
Here’s how you can start a basic web server in Go:
In the example above:
- The function maps the root URL (/) to a handler function that responds to requests with a simple welcome message.
- The function then listens on port 8080.
Now, you can your browser to access , and there it is—a Go web server in its simplest form. This straightforward approach saves developers from the hassle of complex configurations, allowing for rapid development.
Go’s built-in features help streamline server setup, making it accessible even for those just stepping into web programming.
Understanding HTTP Handlers
The heart of any web application lies in how it processes requests and sends responses — that’s where HTTP handlers come into play. In Go, handlers are simply functions that adhere to the interface. This interface requires implementing the method, allowing your function to handle HTTP requests.
When using , Go implicitly creates an HTTP handler for you. This is both flexible and powerful, enabling you to easily define custom behavior based on the incoming requests. For instance, you can differentiate actions based on the URL or the HTTP method used (GET, POST, PUT, etc.).
For a better grasp, consider this example:
In this case, the function only processes GET requests to the endpoint. It’s a simple yet effective way to manage the flow of data and operations in your application.
When designing web applications, understanding these handlers and their capabilities is crucial. They allow developers to build dynamic, user-focused experiences while ensuring clean code organization and efficient handling of HTTP requests.
Embracing Go for building web applications not only equips developers with speed and reliability but also fosters a strong foundation to explore more advanced features like middleware, authentication, and even routing — all in the spirit of simplicity that Go embodies.
Package Management
Managing packages is a pivotal aspect of programming, especially when it comes to Go. Understanding how package management works can be the difference between a messy project and a well-organized codebase. In the world of Go, package management involves how we handle code dependencies and modules. Without a solid grasp of this, both new and experienced developers might find themselves in a tangled web of complexities.
When working with Go, the concept of modules becomes important. Packages in Go allow developers to group related code together, promoting better organization and reusability. Each module can represent a single project or a library that can be shared across various applications. Effective package management streamlines the development process, allowing teams to easily incorporate third-party libraries while also simplifying updates and maintenance.
Here are some vital aspects to consider regarding package management in Go:
- Modularity: Go encourages developers to write modular code. This means your code can be easier to test, debug, and extend.
- Version Control: Each module comes with its own version. This means you can rely on particular features without worrying that an update may break your existing code.
- Simplicity: The Go toolchain includes built-in commands to manage packages, making it straightforward to add or remove dependencies.
"Understanding Go’s package management is not just a nice-to-have skill; it’s fundamental for collaboration and maintaining code efficiency in software development."
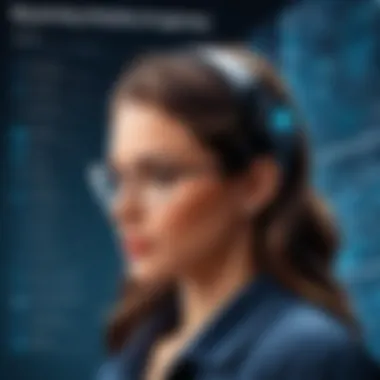
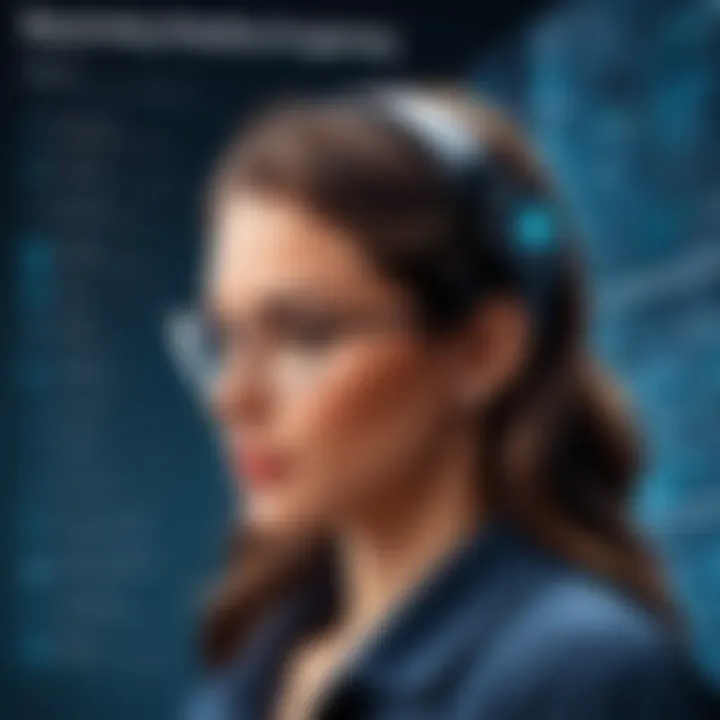
Using Go Modules
Go Modules introduced in Go 1.11, simplify package management significantly. Before Go Modules, developers often relied on GOPATH, a rather rigid system that could lead to complications when it comes to dependency management. With Go Modules, developers can handle their project dependencies outside of GOPATH, facilitating more flexible workflows.
To get started with Go Modules, a few basic steps need to be followed:
- Initialize a Module: You start by running . This command creates a go.mod file in your project directory, laying the groundwork for your module.
- Adding Dependencies: Use to fetch and add a dependency. Go will automatically update your go.mod file and create a go.sum file to ensure reproducibility.
- Upgrading Dependencies: If you need to fetch the latest version, simply run . This command updates the package within your module.
Additionally, Go Modules manage transitive dependencies effectively. This means if you depend on a package that requires another package, Go will handle that for you, keeping everything organized.
Managing Dependencies
Managing dependencies in Go can initially seem daunting, but once you grasp the underlying processes, it significantly boosts your development efficiency. Key practices in dependency management include understanding versioning, specifically semantic versioning (semver), and keeping your dependencies up to date.
Maintaining up-to-date dependencies reduces security vulnerabilities and compatibility issues. Tools and commands like enable you to see all your current dependencies, which is handy for auditing and ensuring you’re not carrying outdated packages.
Moreover, it is vital to regularly check for vulnerabilites in third-party packages. You can utilize resources like the Go Vulnerability Database to stay informed about security concerns.
Best Practices in Go Programming
In the realm of software development, adopting best practices is like having a compass in uncharted waters. Ensuring that your code not only functions, but is also readable, maintainable, and efficient, is vital. With Go’s clear syntax and powerful features, establishing these best practices becomes even more significant. Effective programming practices lead to fewer bugs, faster execution, and ultimately, a more satisfying programming experience. It’s essential for both seasoned developers and novices alike to understand these principles to navigate the Go landscape successfully.
Effective Code Organization
When it comes to effective code organization, structuring your Go projects is paramount. Go is renowned for its simplicity, but without proper organization, ongoing projects can quickly become a tangled mess. Here are a few crucial elements to keep in mind:
- Package Structure: Grouping related code into packages is a foundational practice in Go. This not only helps in maintaining clean code but also promotes reusability. Each package should ideally handle a specific functionality. For instance, if you were creating a web application, you might have separate packages for handling routes, database interactions, and business logic.
- Naming Conventions: Go follows a camelCase naming convention which is important for readability. Using meaningful names for variables and functions makes code understandable at a glance. Avoid too short or ambiguous identifiers; clarity should always take precedence.
- Documentation: Go’s standard practice encourages developers to document their code above the declarations. A well-documented codebase acts like a roadmap for future maintainers or even for your future self. It’s often said, “Good comments don’t ruin code,” and they play a significant role in effective code organization.
By keeping these practices in check, you can create an organized project structure that not only enhances your efficiency but also enhances team collaboration.
Performance Optimization Techniques
Performance is the name of the game in programming, and Go offers various avenues for performance optimization. Here are some techniques crucial for achieving peak performance in your applications:
- Profiling Your Code: Before attempting to optimize, it’s wise to understand where the bottlenecks are. Using Go's built-in profiling tools such as can help you identify slow-performing segments of your code. Once you know what needs improvement, you can focus your efforts efficiently.
- Efficient Data Structures: Choosing the right data structures is fundamental. Go provides built-in types such as slices, maps, and arrays, each with its use cases. Depending on your application, leveraging the most appropriate data structure can drastically improve performance.
- Concurrency and Goroutines: Go shines particularly when it comes to concurrency. Utilizing goroutines and channels effectively can lead to better resource utilization and diverse task execution without blocking the main thread. This capability is particularly beneficial in applications that handle multiple tasks simultaneously, such as web servers.
- Memory Management: Monitor memory usage closely. Go employs garbage collection, which simplifies memory management, but it’s crucial to be aware of how your code allocates and frees memory. Opting for stack allocation for temporary variables over heap allocation can often provide a performance boost.
Implementing these performance optimization techniques can yield significant dividends, enabling your applications to run smoothly and efficiently, enhancing user experience and possibly saving costs on resources.
Remember, even small tweaks often lead to great outcomes in performance. Pursuing efficiency is not just a technical necessity; it’s an art you’ll master with practice.
Exploring Go in the Real World
The relevance of Go programming in real-world applications cannot be overstated. As organizations increasingly rely on agile and efficient solutions, Go's attributes come forth as a game changer. With its simplicity and robust performance, developers are now able to tackle complex problems with ease. This section sheds light on how Go’s features are utilized across various industries, and how companies leverage them for better productivity and efficiency.
Use Cases in Industry
- Cloud Infrastructure:
Go is a predominant language in cloud-era technologies. Companies like Google and Dropbox have developed critical backend systems using Go, capitalizing on its concurrency features to manage large-scale server operations efficiently. - Web Development:
In the realm of web programming, frameworks built in Go, such as Gin and Echo, have automated many processes, streamlining the backend development for startups like Medium. The speed and reliability of Go enhance the load times and user experience on websites. - Data Processing:
Go’s ability to handle data streams effectively makes it a preferred choice for big data applications. For instance, companies involved in real-time analytics, like Uber, have adopted Go to gather and process vast amounts of data quickly. - Microservices Architecture:
Aside from being lightweight, Go supports building microservices seamlessly. Organizations such as Netflix and Soundcloud are exploiting Go’s capabilities to create microservices that scale effectively, leading to enhanced productivity and easier maintenance. - DevOps Tools:
Tools like Docker and Kubernetes, foundational blocks of modern DevOps practices, are developed in Go. This highlights Go’s suitability for building tools that ensure reliable and continuous software delivery.
Success Stories of Go Applications
Many success stories illustrate Go's prowess in modern software development. Among them:
- Google:
It’s no surprise that Google adopted Go for many of its internal projects due to its efficiency. Projects like Google Cloud and Google Earth benefit from Go’s capabilities, allowing these platforms to perform tasks that require heavy lifting without compromising on speed or reliability. - Uber:
The ride-sharing giant operates with billions of requests daily. To manage these requests, Uber chose Go, resulting in significantly improved performance metrics and overall user satisfaction. - Twitch:
The popular streaming platform moved parts of its backend from Python to Go, which led to decreased latency during peak usage hours. This shift resulted in an enhanced experience for millions of users. - Dropbox:
Originally built using Python, Dropbox migrated its server-side architecture to Go. This transition allowed for a scalable solution, improving performance while also reducing server costs and operational complexities.
"Adopting Go isn't just about leveraging a programming language; it’s about embarking on a journey toward building robust, maintainable, and high-performance applications."
In summary, as industries continue to evolve rapidly, the demand for performant and reliable programming languages becomes ever more pronounced. Go stands at the forefront of this revolution, demonstrating its utility through numerous use cases and success stories, making it a prime choice for developers aiming to solve 21st-century problems.
Resources for Learning Go
Learning Go can be a fascinating yet demanding journey. Therefore, having the right resources at your disposal is crucial. This section delves into various materials and platforms that can enhance your grasp of the language effectively. From books to online courses, each resource plays a specific role in providing insights, facilitating hands-on practice, and connecting with a community of like-minded individuals. A well-structured approach to learning through these mediums can streamline your experience, making it more productive and enjoyable.
Books and Online Courses
Books remain a staple in the quest for programming knowledge, and Go is no exception. Not just any books, though; look for those that clearly articulate concepts without drowning you in jargon. For beginners, "The Go Programming Language" by Alan A. A. Donovan and Brian W. Kernighan serves as an excellent starting point. It's concise yet comprehensive, offering a solid grounding in Go's syntax and features. On the other end of the spectrum, seasoned developers will find value in "Go in Action" by William Kennedy, which digs into advanced topics like concurrency and testing with real-world applications.
Online courses further democratize learning by offering flexibility. Platforms like Coursera and Udemy host various courses tailored for different skill levels. Check out "Programming with Google Go" on Coursera. It gives a structured approach combined with hands-on assignments that drive the learning home. The beauty of online courses lies in their visual and interactive content, which can sometimes make complex concepts easier to digest compared to traditional texts.
Each resource has elements to consider, such as your own learning style and the specific areas you want to focus on. It’s smart to try a mix of both books and courses to keep it fresh and engaging.
Community and Networking
The value of community in the learning process can’t be overstated. Engaging with others who share your passion for Go creates an environment conducive to growth. Developers often share experiences, workflows, and insights that simply can’t be found in textbooks.
Start by visiting Reddit communities like r/golang, where you'll find discussions, tutorials, and even job postings. This community aspect opens doors to networking, providing connections that can lead to collaborations on projects, job opportunities, or mentorship. Additionally, participating in local meetups or joining Go user groups is another way to step beyond the keyboard. Engaging with fellow programmers can bolster your skills and introduce diverse perspectives on problem-solving.
In summary, leveraging both books and online courses, complemented by a robust community, gives you a well-rounded approach to learning Go. You’ll find that bouncing ideas off others not only cements your understanding but also inspires innovative thinking.
Remember: Building a network is as important as mastering the technical skills. Collaboration and support can take your programming abilities to the next level.
Future of Go Programming
The realm of programming languages is vast and evolving at a breakneck pace, and Go, often referred to as Golang, is no exception. In this section, we look closely at the future of Go programming, marking its significance in today’s tech landscape and shedding light on what the forthcoming years may hold for this language. Since its inception, Go has been gaining traction for several reasons, and understanding its potential will help learners and seasoned developers alike align their projects with emerging trends.
Go's simplicity, efficiency, and concurrency model offer a refreshing take on traditional programming paradigms. With a growing community and robust ecosystem, the future looks promising. Professionals and organizations increasingly turn towards Go to build scalable and performant systems, which points to its staying power in the programming toolkit. Moreover, Go's alignment with cloud-native technologies, microservices, and its rich standard library enhances its appeal, especially as enterprises continue to migrate to cloud infrastructures.
Evolving Trends in Go Development
As we step deeper into a digital-first world, it's vital to recognize the trends steering Go's growth. Among these trends, the rise of cloud-native applications stands out prominently. The trend pushes the usage of microservices architecture, where Go shines due to its lightweight nature and efficient execution. More teams are employing Go in DevOps, spurred by its capability to handle tasks such as container orchestration efficiently.
Another significant trend is the integration of Go with AI and data science. As businesses seek to utilize machine learning to drive insights, Go's performance becomes a crucial factor in handling large datasets alongside visualization and real-time analytics needs. Libraries like Gorgonia serve as beacons for developers who wish to combine Go's strengths with machine learning applications.
Moreover, community support and contributions will continue to shape Go’s future. As more developers share their experiences and tools on forums like Reddit and GitHub, collaboration will foster faster language evolution.
Predictions for Go in the Tech Ecosystem
Looking further down the line, several predictions can be made about Go's role in the tech ecosystem. First and foremost, Go is likely to strengthen its position in cloud computing. As the industry embraces serverless architecture, Go's rapid startup times and efficiency in resource usage may make it the go-to language for serverless functions and microservices environments.
Additionally, the demand for asynchronous programming in web development is predicted to increase. Since Go’s concurrency model is built around Goroutines, we could see an upswing in its adoption for building web applications. The simplicity of handling concurrent tasks could potentially attract web developers looking for streamlined and highly performant backend solutions.
Furthermore, as IoT (Internet of Things) evolves, Go could emerge as a popular choice for developing applications that require robustness and scalability in interacting with multiple devices. Internet-connected devices require languages that manage workloads effectively, and Go’s capacity to handle high concurrency gives it an edge.
The future for Go programming appears to be on an upward trajectory, thanks to its focus on simplicity, performance, and the vibrancy of its community.
In summary, as programming paradigms shift and evolve, Go is poised to adapt and grow with them. Keeping an eye on these trends and predictions, developers can prepare and align their skills and projects, ensuring they remain at the forefront of technology.
Closure
Wrapping up this exploration of Go programming, it’s clear that this language is not just a tool, but a worthwhile investment for anyone in the tech sector. Its efficiency, simplicity, and strong community support make it invaluable, especially in the realms of concurrent programming and web applications. As technology continually evolves, adapting to new languages like Go is crucial for career growth.
Recap of Key Takeaways
Throughout our journey, we’ve highlighted several essential elements:
- Simplicity and Efficiency: Go’s design principles focus on simplicity, making it easier to learn and master for both beginners and seasoned developers.
- Concurrency Capabilities: Features like goroutines and channels open up a world of possibilities for handling multiple tasks simultaneously, making Go a go-to choice for high-performance applications.
- Rich Ecosystem: The availability of resources, including extensive libraries and strong community support, fosters an environment ripe for innovation and problem-solving.
- Real-world Applications: From web servers to cloud services, Go’s versatility is proven across various industries.
"Remember, the best way to learn is by doing. Write code, break things, and solve them. This is a key takeaway in mastering Go."
Next Steps in Your Go Programming Journey
As you conclude this guide, you might wonder, what’s next? Here are some steps to continue your Go programming adventure:
- Put Your Knowledge into Practice: Start small projects, experiment with Go modules, and gradually tackle more complex applications.
- Join the Community: Engage with Go communities on platforms such as Reddit or GitHub. Collaboration will provide insights and foster growth.
- Explore Advanced Topics: Delve into areas like Go’s type system, reflection, and building microservices to deepen your expertise.
- Contribute to Open Source: Get involved in existing Go projects. It’s a great way to learn collaborative coding and gain feedback from experienced developers.
By taking these steps, you'll not only enhance your skills but also position yourself as a valuable asset in the fast-paced world of tech.
Remember, the road to mastery takes time, persistence, and a great deal of passion. Embrace the journey.