Master Golang Through Project Development: A Comprehensive Guide
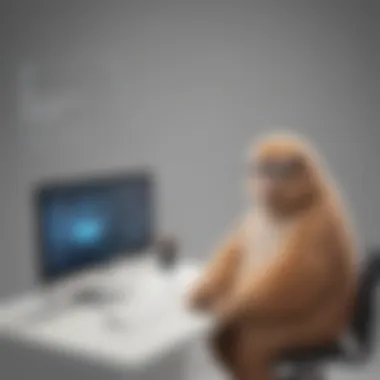
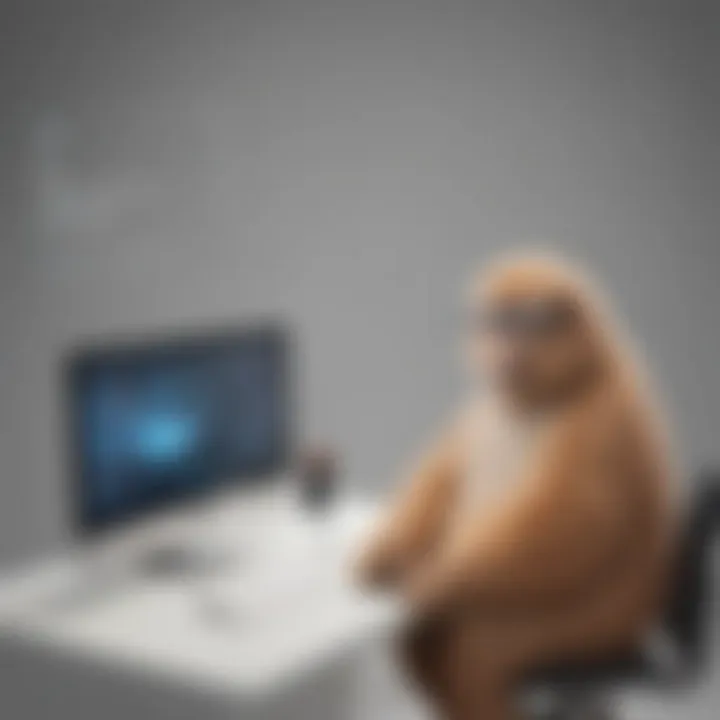
Overview of Go Programming Language
The Go programming language, also known as Golang, is a modern and efficient language developed by Google. It is renowned for its simplicity, concurrency support, and speed, making it an ideal choice for various software development projects. Golang boasts a robust standard library and a growing community, positioning it as a versatile language for both beginners and experienced developers.
- Definition and Importance: Go, designed for ease of use and readability, emphasizes efficiency and clear syntax. Its native support for concurrent programming sets it apart in the programming landscape.
- Key Features and Functionalities: Golang offers features like type safety, garbage collection, and built-in testing, enhancing development speed and code reliability. Its goroutines enable effortless concurrent execution, optimizing performance.
- Use Cases and Benefits: Industries spanning web development, networking, cloud services, and more leverage Go for building scalable, reliable, and high-performance applications. Its efficient compilation process and seamless integration capabilities make it a preferred choice for cloud-native deployments.
Introduction to Golang
In this article, we delve into the fundamental aspect of understanding the Golang programming language. Mastering Golang basics is the cornerstone for any developer looking to enhance their coding skills. By familiarizing oneself with Variables and Data Types, Control Structures, and Functions and Methods within Golang, developers can lay a solid foundation to excel in project-based learning endeavors. Understanding these core concepts is crucial as they enable developers to write efficient code, manipulate data effectively, and create robust applications in Golang.
Understanding Golang Basics
Variables and Data Types
Variables and Data Types in Golang play a pivotal role in defining how data is stored and manipulated in a program. Golang offers a strong type system that ensures code reliability and safety by enforcing strict typing rules. The static nature of variables assists in catching errors during compile time, offering developers a robust mechanism to identify potential issues early on. While this strictness may seem restrictive to some, it ultimately leads to more stable and predictable code, making it a preferred choice for building scalable applications in Golang.
Control Structures
Control Structures in Golang govern the flow of execution within a program, allowing developers to make decisions and iterate over data efficiently. The simplicity and effectiveness of Golang's control structures contribute to writing clean and understandable code. By leveraging constructs like loops and conditional statements, developers can implement logic seamlessly, enhancing the clarity and logic flow of their codebase. This conciseness and readability make control structures a valuable asset for developers working on complex projects in Golang.
Functions and Methods
Functions and Methods are vital components in Golang that encapsulate logic and enable code reusability. Golang's support for both functional and object-oriented paradigms empowers developers to create modular and maintainable code. By defining clear interfaces and separating concerns, functions and methods enhance code organization and foster collaboration among team members. This structured approach to programming elevates the reliability and scalability of applications, making Golang a compelling choice for project development.
Setting Up Development Environment
Installing Go Tools
The process of installing Go Tools is essential for initializing the Golang development environment. By downloading and setting up the necessary tools such as the Go compiler and package manager, developers can kickstart their Golang projects seamlessly. The Go Tools streamline the development process by providing essential functionalities like code compilation, dependency management, and tool integration, enabling developers to focus on writing efficient code.
Configuring Workspace
Configuring the workspace in Golang involves organizing project directories and setting environment variables for optimal development. Structuring the workspace according to best practices ensures project scalability and maintainability. By segregating code files, managing dependencies, and defining project paths, developers can enhance code readability and streamline collaboration within a team. A well-configured workspace paves the way for efficient project development in Golang.

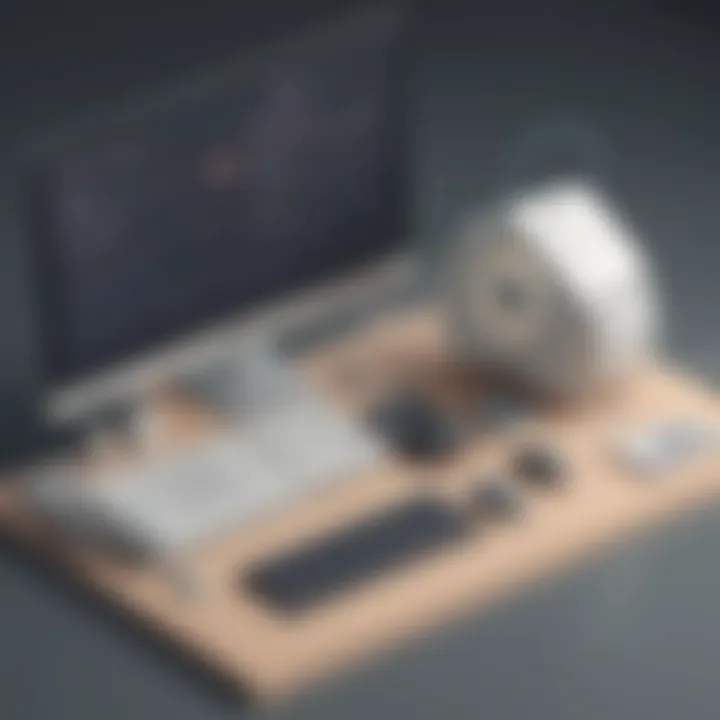
Project-Based Learning Approach
Benefits of Project-Based Learning
Embracing a project-based learning approach in Golang offers numerous benefits for developers seeking hands-on experience. By working on real-world projects, developers can apply theoretical knowledge in practical scenarios, reinforcing learning and skill acquisition. Project-based learning fosters creativity, problem-solving skills, and collaboration, nurturing a holistic development experience for developers. This approach empowers developers to explore diverse concepts and technologies, gaining insights that extend beyond traditional learning methods.
Selecting a Suitable Project
Selecting a suitable project is a crucial decision that influences the learning trajectory in Golang. Developers should choose projects that align with their interests, goals, and skill level to ensure a meaningful learning experience. By selecting projects that challenge and inspire growth, developers can expand their knowledge and expertise in Golang effectively. The process of project selection requires careful consideration of scope, complexity, and feasibility, enabling developers to embark on projects that resonate with their learning objectives.
Building Your First Project
In this section, we delve into the significance of embarking on the journey of building your very first project in Golang. Developing a project from the ground up serves as a practical application of the theoretical knowledge acquired in previous sections. It allows hands-on experience in coding, problem-solving, and applying Golang concepts in a real-world scenario. Building a project provides a holistic view of the language's functionality, enabling developers to understand the intricate details and nuances of Golang thoroughly. Through project development, one can grasp the logic flow, data manipulation, and the structuring of code in a systematic manner. The benefits of creating a first project in Golang include solidifying understanding, honing coding skills, fostering creativity in problem-solving, and laying a strong foundation for subsequent projects.
Project Ideation and Planning
Defining Project Scope
Discussing the importance of defining the project scope is paramount in ensuring the success of the endeavor. By setting clear boundaries and objectives, developers can streamline their focus, prioritize tasks effectively, and avoid scope creep. The defined project scope acts as a roadmap, outlining the project's goals, deliverables, functionalities, and constraints. It helps in managing stakeholders' expectations and aligning the development process with the project objectives. The unique feature of defining project scope lies in its ability to provide a structured approach to project development, reducing ambiguity and enhancing project clarity and direction.
Creating a Roadmap
Creating a roadmap is instrumental in guiding the project from ideation to completion. A well-defined roadmap breaks down the project into manageable tasks, establishes timelines, and allocates resources efficiently. It assists in tracking progress, identifying potential roadblocks, and making informed decisions throughout the development cycle. The key characteristic of a roadmap is its ability to offer a strategic overview of the project, ensuring that all aspects are taken into account and progress is measured against predefined milestones. While a roadmap provides a structured path for project execution, flexibility must also be maintained to adapt to evolving project requirements.
Implementing Core Features
Handling HTTP Requests
When it comes to Golang project development, handling HTTP requests plays a vital role in creating web-based applications. Managing HTTP requests involves routing incoming requests to the appropriate handlers, processing data, and crafting responses. The key characteristic of handling HTTP requests is its asynchronous nature, allowing developers to handle multiple requests concurrently without blocking other operations. This feature enhances the performance and responsiveness of web applications developed in Golang. While handling HTTP requests provides flexibility and efficiency in developing web services, proper error handling and security measures must be implemented to ensure the robustness and reliability of the application.
Data Manipulation
Data manipulation forms the core of many software applications, and in Golang, efficient data manipulation is essential for optimal application performance. Golang offers powerful built-in data manipulation functions and libraries that enable developers to process, transform, and analyze data seamlessly. The key characteristic of data manipulation in Golang is its simplicity and efficiency, allowing developers to manipulate complex data structures with ease. Utilizing Golang's data manipulation capabilities enhances the scalability and maintainability of projects, streamlining data processing tasks and enhancing overall application performance.
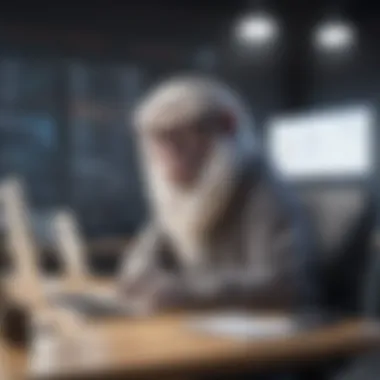
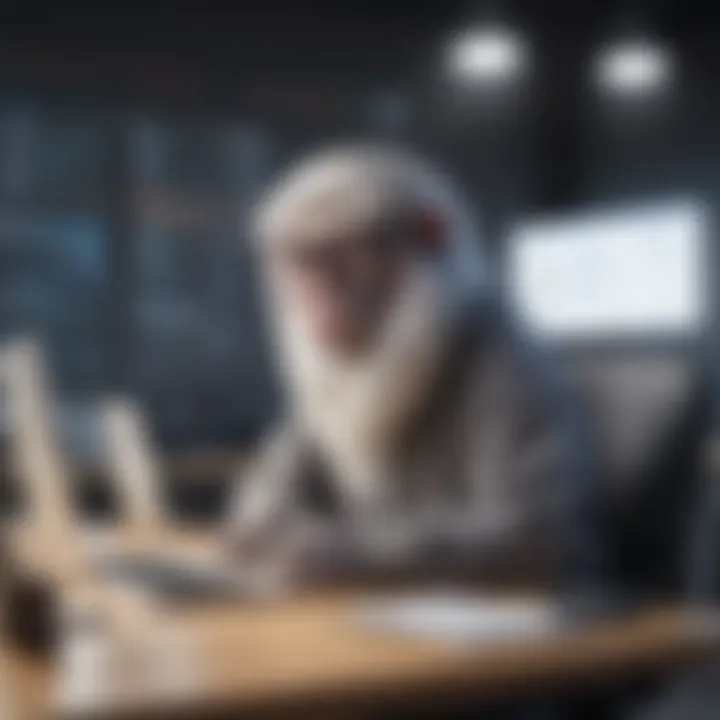
Testing and Debugging
Unit Testing
Unit testing is a critical aspect of Golang project development, ensuring that individual units of code function as expected. Unit tests verify the correctness of specific functions, methods, or modules, helping developers detect and rectify errors early in the development cycle. The key characteristic of unit testing lies in its ability to isolate and test individual components in isolation, ensuring that each unit operates as intended. Unit testing enhances code reliability, accelerates bug detection, and simplifies the debugging process, promoting overall code quality and maintainability.
Debugging Techniques
Effective debugging techniques are essential for identifying and resolving issues in Golang projects. Debugging involves systematically inspecting code, tracing execution paths, and diagnosing errors to pinpoint and rectify issues. The key characteristic of debugging techniques is their role in uncovering the root cause of anomalies, whether related to logic errors, runtime issues, or unexpected behaviors. By employing various debugging tools and strategies, developers can streamline the troubleshooting process, expedite issue resolution, and ensure the stability and robustness of the application.
Advanced Concepts and Enhancements
In this section, we will delve into the crucial elements of advanced concepts and enhancements within the realm of Golang project development. Understanding these concepts is paramount to elevating one's proficiency in Golang programming. By focusing on advanced concepts and enhancements, developers can optimize their code, enhance performance, and integrate external functionalities seamlessly. These topics are essential for those looking to take their Golang skills to the next level.
Concurrency and Parallelism
Goroutines
Goroutines play a vital role in achieving concurrency in Golang. They enable developers to concurrently execute functions or methods, allowing for asynchronous operations within a program. The key characteristic of Goroutines lies in their lightweight nature, making them a preferred choice for concurrent programming in Golang projects. This lightweight design ensures efficient resource utilization and scalability, contributing to improved performance in multi-threaded applications. Additionally, Goroutines introduce a unique feature known as channels, which facilitates communication and data sharing between concurrent Goroutines, enhancing synchronization and coordination in parallel tasks.
Channels
Channels serve as the communication backbone for concurrent Goroutines in Golang. They facilitate safe data exchange and synchronization between concurrent processes, promoting a structured approach to parallelism. The key characteristic of channels is their ability to ensure explicit communication and coordination, enabling developers to avoid common concurrency issues such as race conditions and deadlocks. Channels are a popular choice for managing inter-process communication in Golang due to their simplicity and robust error-handling mechanisms. However, developers should be cautious of overusing channels, as excessive synchronization can lead to performance bottlenecks in highly parallelized applications.
Optimizing Performance
Profiling
Profiling plays a pivotal role in optimizing the performance of Golang applications. It involves analyzing the execution time and resource utilization of different functions or sections of code to identify bottlenecks and inefficiencies. The key characteristic of profiling is its ability to provide detailed insights into runtime behavior, allowing developers to pinpoint performance issues and prioritize optimization efforts effectively. By leveraging profiling tools available in Golang, developers can streamline their code, improve resource allocation, and enhance overall application performance.
Benchmarking
Benchmarking is essential for gauging the performance and efficiency of code snippets or algorithms in Golang projects. It involves running standardized tests to measure the execution time and memory consumption of specific operations, helping developers make informed decisions on code optimization. The key characteristic of benchmarking is its quantitative analysis approach, enabling developers to compare different implementations and identify the most efficient solutions. By incorporating benchmarking practices into their development workflow, Golang programmers can fine-tune their code for optimum performance and scalability.
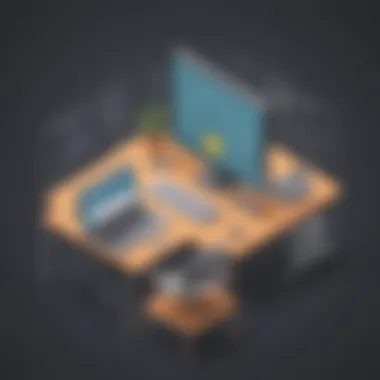
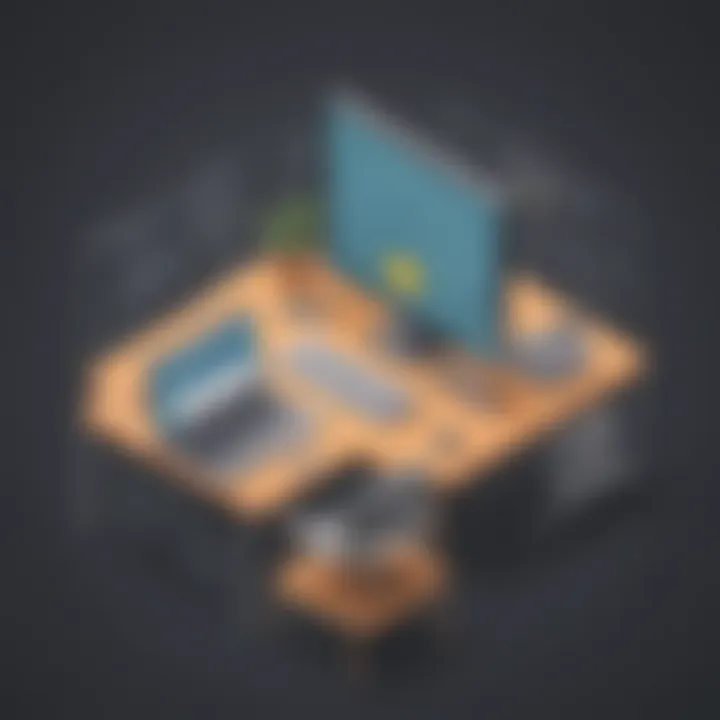
Integrating Third-Party Packages
Using External Libraries
Integrating external libraries is a common practice in Golang projects to leverage existing functionalities and accelerate development processes. External libraries offer pre-built modules and utilities that expand the capabilities of Golang applications, saving developers time and effort in coding from scratch. The key characteristic of using external libraries is the enrichment of project functionality without reinventing the wheel, promoting code reusability and modularity. However, developers need to exercise caution when selecting external libraries to ensure compatibility, security, and maintenance support throughout the project lifecycle.
Dependency Management
Effective dependency management is crucial for maintaining a stable and scalable codebase in Golang projects. It involves handling external dependencies, version control, and package dependencies to ensure seamless integration and consistency within the project. The key characteristic of dependency management is its role in simplifying project maintenance, updates, and collaboration between team members. By adopting efficient dependency management practices, developers can streamline their workflow, resolve dependency conflicts, and enhance project reliability and scalability.
Finalizing and Showcasing Your Project
Code Refactoring and Documentation
Improving Code Quality
Improving code quality is a fundamental aspect of software development that plays a crucial role in the success of any project. By focusing on enhancing the structure, readability, and efficiency of your code, you can ensure that it is easier to maintain, debug, and scale. Emphasizing code quality not only benefits the current project but also lays a solid foundation for future endeavors. One key characteristic of improving code quality is adhering to coding best practices and design patterns, which promote consistency and robustness. This approach is particularly beneficial in the context of the article, as it underscores the importance of producing high-quality code that aligns with industry standards.
Writing Documentation
Documenting your code is essential for ensuring that others can understand your project and collaborate effectively. Writing clear and comprehensive documentation provides insights into the project's architecture, functionality, and usage, making it easier for new developers to onboard. The key characteristic of writing documentation lies in articulating complex technical concepts in a simple and accessible manner, enabling smoother knowledge transfer and collaboration. In this article, highlighting the significance of documentation emphasizes the value of communication in software development, underscoring how well-written documentation can streamline the development process and foster teamwork.
Deploying the Project
Deployment Strategies
Choosing the right deployment strategy is crucial for ensuring that your Golang project reaches its target audience effectively. Whether you opt for continuous deployment, blue-green deployment, or canary releases, each strategy has its unique advantages and considerations. The key characteristic of deployment strategies is enabling seamless updates and rollbacks, minimizing downtime and disruption for end-users. In this article, discussing deployment strategies sheds light on the importance of planning and executing deployments efficiently, emphasizing the significance of a robust deployment pipeline.
Hosting Options
Selecting the appropriate hosting option for your Golang project is vital for its performance, scalability, and security. Whether you choose cloud hosting, dedicated servers, or serverless architecture, each option has distinct features and cost implications. The key characteristic of hosting options is providing a reliable infrastructure that can support your project's requirements while offering scalability and flexibility. By evaluating different hosting options, you can make an informed decision that aligns with your project's needs and objectives, underscoring the importance of selecting the right hosting solution in this article.
Sharing and Gathering Feedback
Utilizing Version Control
Utilizing version control systems like Git is indispensable for keeping track of changes, collaborating with team members, and maintaining code consistency. By leveraging version control, you can manage different project versions, track modifications, and resolve conflicts efficiently. The key characteristic of utilizing version control is ensuring code integrity and facilitating collaboration among developers, promoting transparency and accountability. In the context of this article, exploring version control underscores the significance of implementing industry-standard practices that enhance workflow efficiency and project scalability.
Seeking Peer Review
Seeking peer review is a valuable practice that can uncover blind spots, identify potential improvements, and validate the effectiveness of your code. By soliciting feedback from peers or mentors, you can gain fresh perspectives, learn new techniques, and refine your coding skills. The key characteristic of seeking peer review is fostering a culture of continuous learning and iteration, embracing feedback as a catalyst for growth and enhancement. In this article, emphasizing peer review highlights the collaborative nature of software development, illustrating how collective insights can elevate the quality and performance of your projects, and encourages the audience to engage in constructive critique and refinement.