Unraveling the Significance of Interfaces in Java Programming
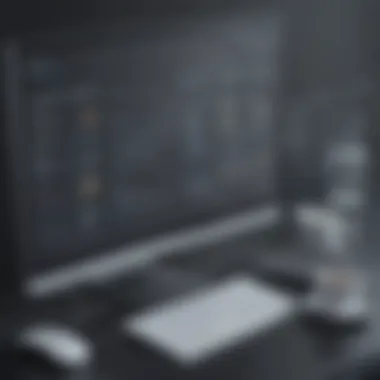
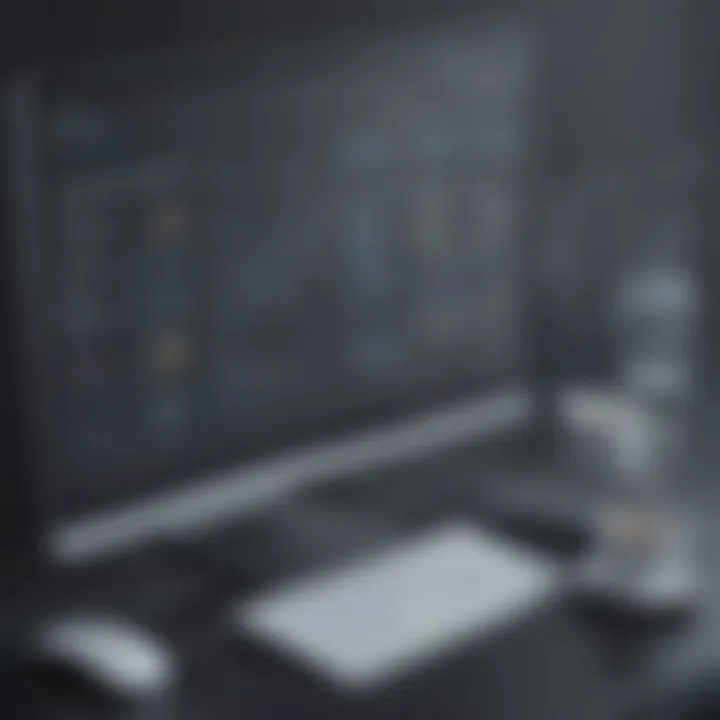
Overview of Java Interfaces
Java interfaces are a fundamental concept in Java programming, serving as a blueprint for classes to implement methods without the need for actual implementation details. Their significance lies in promoting code modularity and flexibility within the Java environment. By exploring Java interfaces, developers can streamline their code structure and enhance its adaptability.
Definition and Importance of Java Interfaces
Java interfaces act as a contract for classes to adhere to, defining a set of method signatures without specifying the implementation. This enforces a consistent structure across classes that implement the interface, facilitating interchangeable components and reducing code dependencies. The importance of interfaces in Java programming cannot be overstated, as they play a pivotal role in achieving code modularity and promoting code reusability.
Key Features and Functionalities
The key feature of Java interfaces is their ability to enable multiple inheritances, allowing classes to implement multiple interfaces. This flexibility grants developers the freedom to design intricate class relationships without being bound by the constraints of single inheritance. Additionally, interfaces support the concept of polymorphism, enabling objects to be treated uniformly based on the methods defined in the interface.
Use Cases and Benefits
Java interfaces find extensive utilization in scenarios where classes belonging to different inheritance hierarchies need to exhibit similar behaviors. By implementing interfaces, developers can ensure compatibility among disparate classes and facilitate a cohesive design structure. The benefits of using interfaces extend to enhancing code maintainability, enabling efficient debugging, and promoting scalable software development practices.
Best Practices for Implementing Java Interfaces
When incorporating Java interfaces into a project, adherence to best practices is crucial for optimizing code efficiency and promoting a robust architecture. Developers should focus on designing clear and concise interfaces, avoiding overly complex definitions that might hinder code readability. Additionally, it is recommended to use interfaces judiciously, ensuring they align with the overarching design principles of the application.
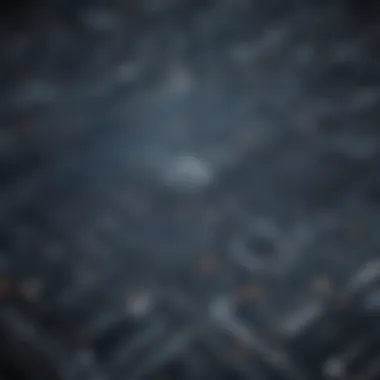
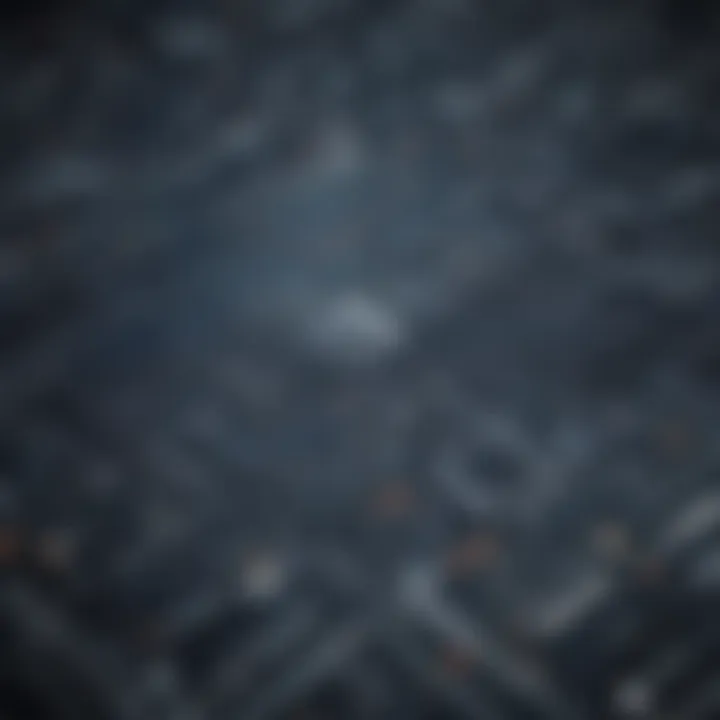
Tips for Maximizing Efficiency and Productivity
To maximize productivity when working with Java interfaces, developers should strive to adhere to naming conventions and establish a coherent interface hierarchy that aligns with the project's objectives. Consistent documentation of interfaces and their intended purpose can greatly enhance code comprehension and facilitate collaboration among team members.
Introduction to Interfaces in Java
In the world of Java programming, interfaces play a crucial role in enabling code modularity and flexibility. Understanding the concept of interfaces is fundamental for developers aiming to write clean and efficient code. Interfaces in Java serve as blueprints for classes, defining a set of methods that must be implemented by any class that claims to implement the interface. They facilitate the implementation of multiple inheritance and help achieve a high level of code reusability. The structuring imposed by interfaces enhances the scalability and maintainability of Java codebases.
Understanding Java Interfaces
The definition and purpose of interfaces
Within Java, interfaces serve as contracts that specify a set of methods that a class must implement. This enforces a level of consistency across various classes that implement the interface, promoting a standardized behavior within the codebase. The core benefit of interfaces lies in decoupling the definition of methods from their implementation, allowing for interchangeable components and promoting a modular design approach. Interfaces also enable polymorphism, enhancing the flexibility and adaptability of Java programs.
How interfaces differ from classes in Java
Interfaces differ from classes in Java primarily in their structure and functionality. While classes allow for both method implementations and state definitions, interfaces only contain method signatures without the method bodies. This clear distinction emphasizes the abstraction provided by interfaces, focusing solely on defining the structure of a class without delving into its implementation details. By enabling multiple interface implementation - unlike Java's limitation of single class inheritance - interfaces offer a more versatile approach to structuring code.
Declaring Interfaces
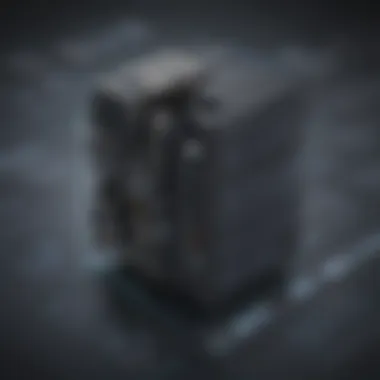
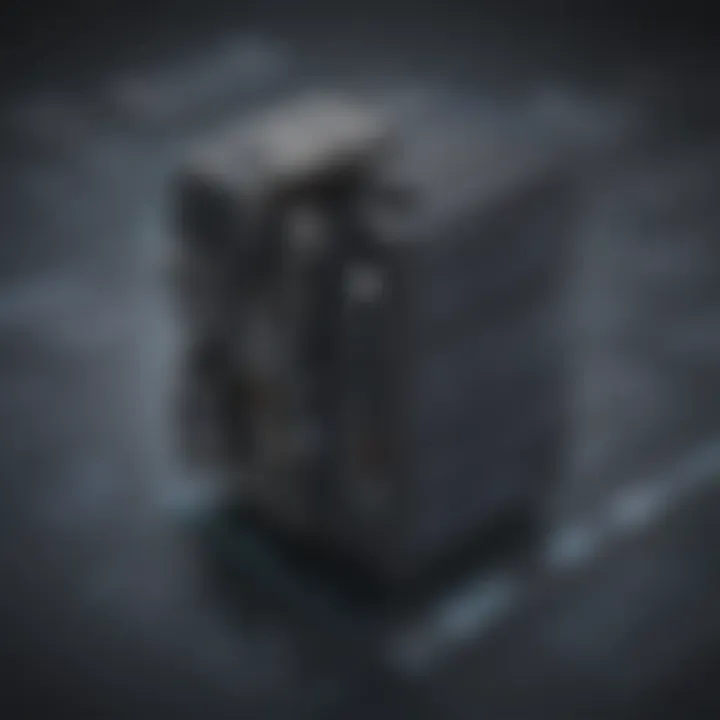
The syntax for interface declaration
In Java, declaring an interface involves using the 'interface' keyword followed by the interface's name. The syntax highlights the abstraction provided by interfaces, emphasizing the method signatures without specifying their implementations. This clear segregation of interface methods from class implementations simplifies the development process, promoting clarity and reusability. Adhering to a consistent naming convention for interfaces ensures code readability and maintainability, facilitating collaboration and code comprehension among multiple developers.
Rules and considerations for interface naming
Naming interfaces in Java follows similar conventions to class naming, primarily adhering to camelCase as the standard naming format. Choosing descriptive and meaningful names for interfaces enhances code understanding and promotes easier navigation within complex projects. Additionally, prefixing interfaces with 'I' or utilizing suffixes like 'able' or 'ible' can convey the purpose or behavior the interface defines. By establishing clear naming guidelines, developers can streamline the communication of interface functionalities across teams, fostering a cohesive and structured codebase.
Implementing Interfaces in Java
In the realm of Java programming, implementing interfaces holds significant importance. Understanding how classes interact with interfaces is crucial for developing flexible and modular code structures. By implementing interfaces, developers can achieve a higher level of abstraction, enabling easier scalability and code maintenance. This section delves into the key aspects of implementing interfaces in Java, shedding light on how it influences the overall architecture of Java applications. From defining interface methods to handling multiple interfaces within a class, the process of implementation plays a pivotal role in enhancing code functionality and extensibility.
Class Implementing an Interface
Implementing interface methods in a class
One integral aspect of Java programming is the implementation of interface methods within a class. By implementing interface methods, developers can enforce a contract specified by the interface, ensuring that the class provides concrete implementations for all defined methods. This practice promotes code consistency and allows for the interchangeability of classes based on shared behaviors defined in interfaces. Implementing interface methods in a class offers a structured approach to defining class functionalities, fostering code reusability and simplifying debugging processes. While this approach enhances the flexibility of Java applications, developers must carefully consider the design implications to maintain code coherence and adherence to interface contracts.
Handling multiple interfaces in a class
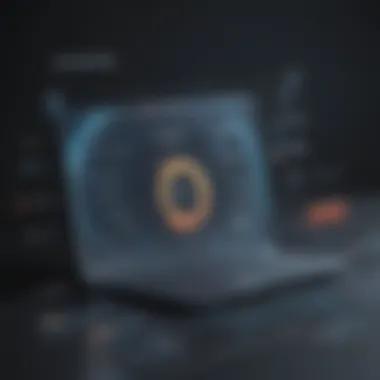
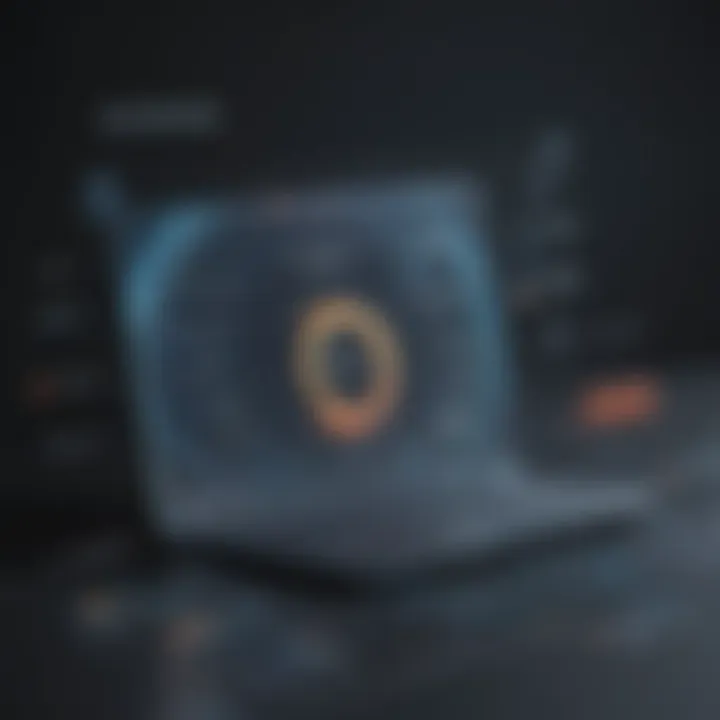
Another crucial consideration in Java programming is the effective management of multiple interfaces within a class. When a class implements multiple interfaces, it inherits behaviors and specifications from each interface, enabling the class to exhibit diverse functionalities. Handling multiple interfaces in a class requires careful attention to method resolution conflicts and interface hierarchies to prevent ambiguity and ensure the coherence of the class structure. By strategically incorporating multiple interfaces, developers can leverage existing code implementations, promote code modularity, and encapsulate related functionalities within the class. While this approach enhances code flexibility, developers must navigate interface dependencies judiciously to uphold code integrity and minimize complexities within the class hierarchy.
Extending Interfaces
Extending interfaces to define subtypes
Extending interfaces to define subtypes is a powerful mechanism in Java for refining interface functionalities and establishing specialized behaviors. By extending interfaces, developers can create hierarchical relationships between interfaces, allowing for the categorization and customization of interface functionalities. This approach facilitates the definition of specific behaviors for subtypes, promoting code extensibility and adaptability to varying programming requirements. Extending interfaces to define subtypes fosters a modular design approach, enabling developers to tailor interface implementations to specific use cases while maintaining code uniformity and consistency. However, careful consideration must be given to the level of abstraction and the coherence of subtype definitions to avoid excessive interface hierarchies and ensure the scalability of interface implementations.
Combining multiple interfaces using inheritance
Combining multiple interfaces using inheritance is a strategic practice in Java programming for deriving comprehensive interface specifications and integrating diverse functionalities. By inheriting multiple interfaces, classes can inherit behavior and method contracts from each interface, amalgamating distinct functionalities into a cohesive structure. This inheritance mechanism promotes code reuse and simplifies the integration of disparate modules, streamlining the development process and enhancing code maintainability. Through the strategic combination of multiple interfaces, developers can orchestrate complex interactions between classes and interfaces, fostering a robust and adaptable architecture. However, maintaining clarity in interface inheritance relationships and managing potential conflicts in method definitions are essential considerations to uphold code clarity and facilitate future modifications.
Interface Example Demonstrations
Real-world Interface Applications
Creating interfaces for various scenarios in Java involves defining abstract contracts that classes can implement. This practice enhances code modularity and helps in achieving a separation of concerns. By creating interfaces tailored to specific scenarios, developers can enforce consistency in methods across different classes, promoting scalability and adaptability in the codebase. This approach is particularly beneficial in large-scale projects where maintaining a structured architecture is crucial. However, one must carefully consider the granularity of interfaces to avoid overcomplicating the design.
Utilizing interfaces in practical programming streamlines the development process by promoting code reusability and polymorphism. Integrating interfaces allows for coding flexibility, as classes can implement multiple interfaces to inherit and override behavior as needed. This practice fosters extensibility and enables developers to write cleaner, more maintainable code. While leveraging interfaces in programming simplifies complex systems and encourages a modular design, it is essential to strike a balance between abstraction and concreteness to ensure the efficiency and clarity of the code.
Benefits of Interface Usage
Enhancing code reusability and maintainability through interfaces is a cornerstone of modern software design. By defining contracts that classes can adhere to, interfaces facilitate the reuse of code snippets across different parts of an application or among multiple projects. This not only saves time and effort in coding but also minimizes the risk of errors and inconsistencies. Moreover, interfaces promote a standardized approach to development, making it easier for teams to collaborate and maintain codebases efficiently.
Facilitating design patterns through interfaces empowers developers to implement robust and scalable architectures. Interfaces serve as blueprints for defining relationships between objects, enabling the application of popular design patterns like the Strategy or Observer pattern. By leveraging interfaces to establish common protocols and abstract behaviors, developers can create flexible and extensible systems that can evolve with changing requirements. This not only enhances the maintainability and readability of the code but also fosters a more modular and testable codebase, encouraging software sustainability and innovation.