Mastering the Art of Interface Classes in Java: A Comprehensive Guide
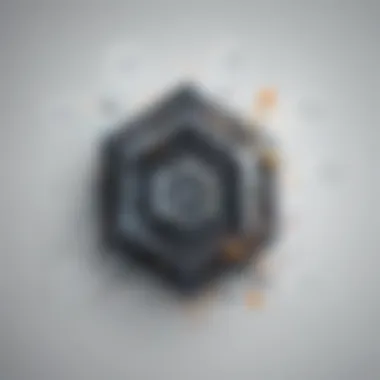
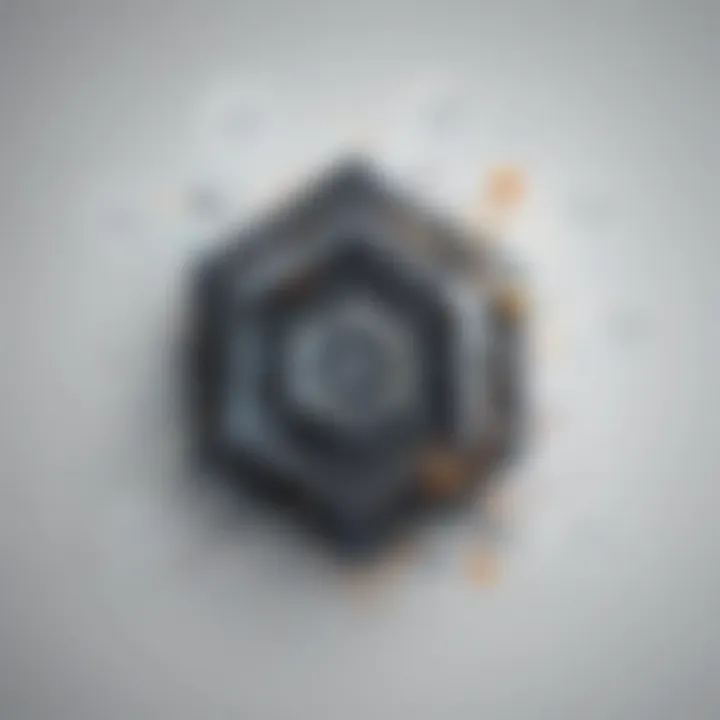
Overview of Programming Concepts
In the realm of Java programming, understanding interface classes is imperative for developers seeking to enhance the efficiency and maintainability of their codebase. Interface classes play a pivotal role in object-oriented programming, enabling the establishment of contracts that classes must adhere to. By delving into the nuances of interface classes, developers can unlock opportunities to create more modular and reusable code structures.
Definition and Importance of Interface Classes
Interface classes in Java serve as blueprints for implementing methods within classes that adhere to these blueprints. They define what methods a class must contain without specifying how they should be implemented. This abstraction allows for the separation of concerns and promotes loosely coupled designs, contributing to the overall robustness of the codebase.
Key Features and Functionalities of Interface Classes
One of the key features of interface classes is that a Java class can implement multiple interfaces, enabling it to inherit functionality from various sources. This promotes flexibility and adaptability in coding practices, facilitating the incorporation of diverse behaviors into classes while maintaining a cohesive architecture.
Use Cases and Benefits of Implementing Interface Classes
The implementation of interface classes empowers developers to enhance code reusability and maintainability by enforcing a standard structure across different classes. By leveraging interfaces, developers can decouple components, promote code scalability, and facilitate easier testing and debugging processes, ultimately leading to more robust and maintainable software solutions.
Best Practices for Leveraging Interface Classes
When utilizing interface classes in Java programming, adhering to industry best practices is essential for maximizing the efficiency and productivity of software development projects. By employing consistent naming conventions, focusing on defining clear and concise interfaces, and avoiding unnecessary complexity, developers can streamline their coding workflows and enhance code readability and maintainability.
Tips for Maximizing Efficiency and Productivity
To maximize the effectiveness of interface classes, developers should strive to keep interfaces cohesive and focused on a single responsibility. By adhering to the principles of interface segregation, where interfaces are tailored to specific client requirements, developers can avoid bloated interfaces and ensure that classes only depend on the methods they actually use.
Common Pitfalls to Avoid
In the realm of interface classes, common pitfalls include overusing marker interfaces, creating overly complex interface hierarchies, and violating the principles of object-oriented design. By steering clear of these pitfalls and adhering to best practices, developers can harness the full potential of interface classes to create robust and maintainable codebases.
Industry Insights and Lessons Learned
Real-world examples of successful implementation of interface classes can offer valuable insights into the practical applications of this programming concept. By examining case studies where interface classes have been instrumental in achieving software development goals, developers can glean lessons learned, understand the outcomes achieved, and gain inspiration from industry experts on leveraging interface classes effectively.
Latest Trends and Updates in Interface Class Development
As the field of Java programming continues to evolve, staying abreast of the latest trends and updates in interface class development is crucial for maintaining a competitive edge. By exploring upcoming advancements in the field, current industry trends, and forecasts for interface class innovation, developers can adapt their coding practices to align with emerging best practices and technological breakthroughs.
Conclusion
Introduction to Interface Class in Java
The realm of Java programming encapsulates a crucial entity known as interface classes. These classes stand as pillars of object-oriented programming, offering a fundamental foundation for constructing flexible and modular code structures. Delving into interface classes in Java unlocks a realm of possibilities for developers seeking to streamline their codebase efficiently. This segment sheds light on the core principles and functionalities associated with introduction to interface class in Java.
Understanding Interfaces in Java
Defining an Interface
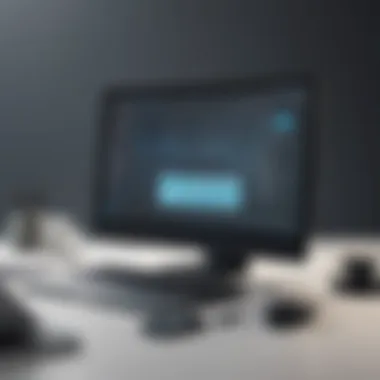
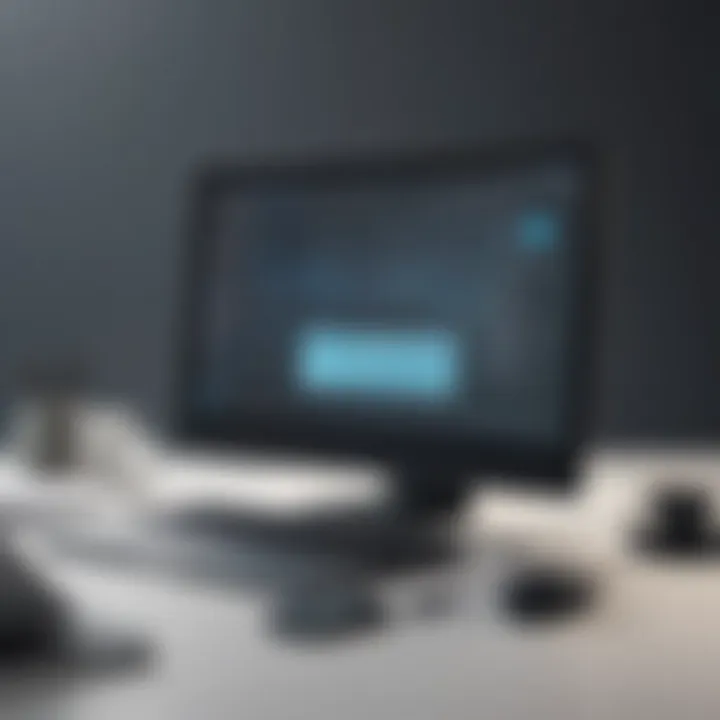
Venturing into the terrain of defining an interface in Java unveils a structured blueprint for classes. Defining an interface provides a contractual agreement outlining the methods a class must implement, fostering a consistent programming model. This pivotal aspect of interface definition enhances code organization and promotes adherence to predefined behaviors, cementing its standing as a prevalent choice for Java developers. The unique trait of defining an interface lies in its ability to enforce method implementation without specifying method logic, cultivating a clear separation of concerns within Java projects.
Implementing Interfaces
The implementation of interfaces in Java orchestrates a harmonious synergy between classes and contracts, culminating in a cohesive software architecture. Implementing interfaces empowers classes to fulfill their obligations outlined in interface definitions, promoting code interoperability and reusability. This facet of Java programming shines due to its capacity to define a uniform structure that multiple classes can adhere to, fostering a cohesive codebase. Synchronizing classes through implementing interfaces paves the way for scalable and modular software design, accentuating its role as a preferred approach in Java development.
Extending Interfaces
Extending interfaces in Java navigates the trajectory of inheritance within the realm of programming paradigms. By extending interfaces, developers can refine and augment existing interface definitions to encapsulate additional functionalities. This practice amplifies code extensibility and adaptability, allowing for seamless integration of new features while preserving the original contract. Extending interfaces in Java imparts a layer of flexibility and scalability to software systems, empowering developers to enrich codebases iteratively. The prowess of extending interfaces in Java lies in its ability to propagate interface enhancements across the codebase, fostering a cohesive and evolutionary programming environment.
Benefits of Using Interface Classes
Code Reusability
The facet of code reusability embedded in interface classes orchestrates an orchestra of efficiency within Java projects. Leveraging interface classes for code reusability cultivates a modular programming paradigm where common functionalities can be shared across disparate classes. This practice streamlines code maintenance and promotes consistency, bolstering the scalability and reliability of Java applications. The hallmark of code reusability in interface classes lies in its capacity to curate a library of standardized behaviors that can be seamlessly integrated into diverse classes, enhancing the robustness of software solutions.
Multiple Inheritance in Java
Multiple inheritance in Java, facilitated through interface classes, navigates the complex terrain of class relationships with finesse. By allowing classes to inherit characteristics from multiple interfaces, Java enables developers to craft sophisticated and interconnected class hierarchies. This feature empowers developers to amalgamate disparate functionalities into a single class, fostering code modularity and extensibility. The unique offering of multiple inheritance in Java lies in its capability to construct intricate class structures without descending into the pitfalls of diamond problem, showcasing Java's prowess in object-oriented design.
Maintainability and Flexibility
The marriage of maintainability and flexibility within interface classes encapsulates the essence of robust software engineering practices. Interface classes imbue Java projects with a layer of maintainability by establishing clear boundaries and contracts for method implementation. This structured approach streamlines code maintenance efforts, enabling developers to swiftly adapt to evolving requirements without compromising code integrity. Furthermore, the flexibility conferred by interface classes allows for seamless integration of new features and modifications, safeguarding the longevity and adaptability of Java applications. The intrinsic value of maintainability and flexibility in interface classes underscores their indispensable role in architecting resilient and agile software solutions.
Key Differences Between Interface and Abstract Class
Method Implementation
The dimension of method implementation delineates a key distinction between interface and abstract classes, unveiling contrasting approaches to method definition. In interfaces, methods are defined but not implemented, necessitating concrete classes to furnish method logic. This architectural choice promotes code modularization and flexibility, enabling classes to implement multiple interfaces concurrently. Abstract classes, on the other hand, permit method implementation, providing skeletal structures for subclasses to extend. This divergence in method implementation underscores the nuanced design considerations that developers must weigh when choosing between interface and abstract classes, shaping the architecture and behavior of Java projects.
Multiple Inheritance
The realm of multiple inheritance navigates divergent paths in interface and abstract classes, illuminating the intricacies of class hierarchy design. Interfaces facilitate multiple inheritance by enabling classes to inherit characteristics from multiple interfaces, unveiling a robust mechanism for fostering code reusability and modularity. This polyphonic approach empowers developers to craft intricate class relationships while avoiding the perils of diamond problem, bolstering code extensibility and versatility. In contrast, abstract classes permit single inheritance, constraining class hierarchies to a linear progression of superclass and subclass relationships. These contrasting paradigms of inheritance in Java underscore the imperative of thoughtful class design, harmonizing code structure with project requirements.
Access Modifiers
The sphere of access modifiers in Java delineates the accessibility boundaries of members within interface and abstract classes, dictating the visibility and encapsulation of class elements. Interfaces inherently enforce public access modifiers on methods by default, promoting method visibility across classes and packages. This design principle aligns with the ethos of interface classes, fostering code interoperability and reusability. Abstract classes, conversely, offer a spectrum of access modifiers, granting developers the flexibility to tailor member accessibility based on design requirements. This divergence in access modifier philosophy accentuates the varying design philosophies of interface and abstract classes, shaping the encapsulation and communication patterns within Java projects.
Implementing Interface Classes
Implementing interface classes in Java is a crucial concept to grasp, as it forms the backbone of object-oriented programming in the language. By incorporating interface classes, developers can achieve enhanced code reusability, maintainability, and flexibility in their codebase. It allows for the defining and implementation of standard interfaces across multiple classes, enabling a structured and modular approach to software development. Understanding how to effectively implement interface classes can significantly streamline the development process and contribute to writing more efficient and scalable Java code.
Declaring and Defining Interfaces
Syntax of Interface Declaration:
When discussing the syntax of interface declaration, we delve into the precise structure required to define an interface in Java. The syntax typically involves using the 'interface' keyword followed by the interface name, along with method signatures that outline the behavior expected from classes implementing the interface. This syntax plays a critical role in establishing a contract that classes must adhere to, ensuring consistency and cohesion in the codebase. The beauty of interface declaration lies in its ability to enforce a set of methods that must be implemented by classes, promoting a standardized approach to programming.
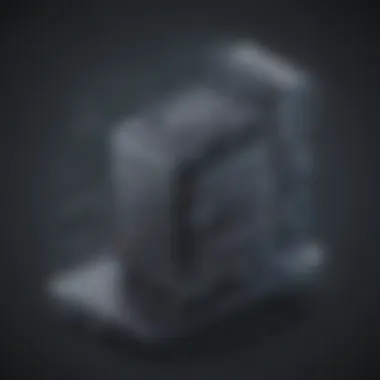
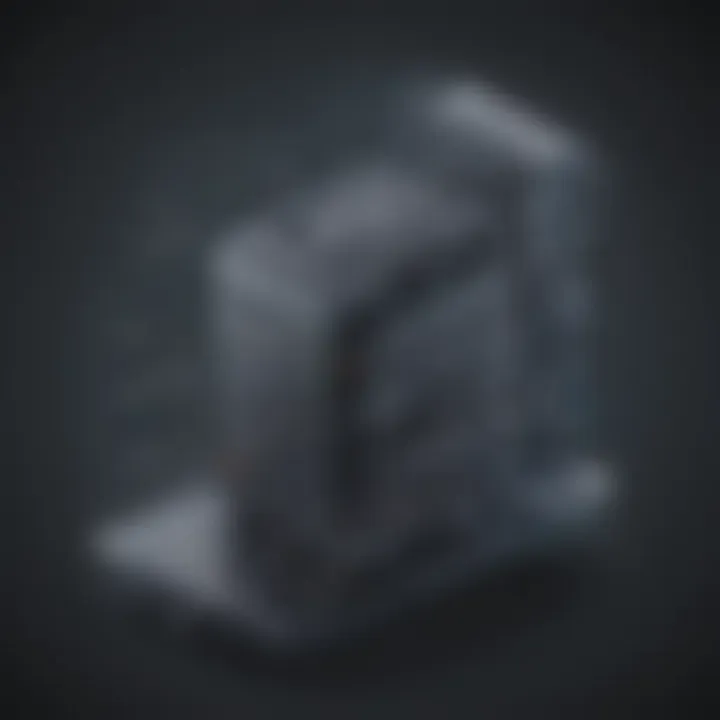
Defining Methods in Interfaces:
Defining methods in interfaces involves specifying the behavior that implementing classes must adhere to. By including method signatures without method bodies, interfaces act as blueprints for classes to implement their functionality. This approach fosters a clear separation of concerns, allowing for different classes to provide their unique implementation while conforming to a common interface structure. The practice of defining methods in interfaces promotes code reusability by enabling multiple classes to implement the same interface with custom functionalities as needed.
Constants in Interfaces:
Constants in interfaces serve as immutable variables that can be accessed using the interface name. By declaring constants within interfaces, developers can maintain a centralized repository of key values that are integral to the interface's functioning. This practice enhances the readability and maintainability of the code by encapsulating relevant constants within the interface declaration itself. Constants in interfaces eliminate the need for defining these values in multiple classes, promoting a more streamlined and organized codebase.
Using Interfaces in Java Classes
Implementing an Interface:
Abstract: Implementing an interface involves a class agreeing to the contract specified by an interface by providing concrete implementations for the interface's methods. This enables the class to exhibit behavior defined by the interface, promoting code consistency and structure. By implementing interfaces, classes can achieve polymorphism, enabling objects to be treated interchangeably based on the common interface they implement.
Overriding Interface Methods:
When a class implements an interface containing methods, it must override these methods with specific implementations. This process allows classes to tailor the behavior of interface methods to suit their unique requirements. By overriding interface methods, developers can customize the functionality of inherited methods, providing flexibility and customization within the codebase.
Inheriting Multiple Interfaces:
Java supports inheriting multiple interfaces, allowing a class to inherit behavior from multiple sources. By inheriting multiple interfaces, a class gains access to the methods and constants defined in each interface, enabling a diverse set of functionalities. This flexibility empowers developers to compose classes with a mix of behaviors from different interfaces, promoting code adaptability and extensibility.
Interface Default Methods and Static Methods
Default Method Implementation:
Default method implementation in interfaces allows developers to provide a default behavior for methods. This enables interfaces to evolve without breaking implementing classes that might not have implemented a newly introduced method. By incorporating default methods, interfaces can add new functionalities without requiring changes in existing classes, enhancing code maintainability and backward compatibility.
Static Methods in Interfaces:
Static methods in interfaces enable the definition of utility methods that are associated with the interface itself rather than with implementing classes. These methods can be called directly on the interface without the need for an instance, providing convenient and encapsulated functionalities. Incorporating static methods in interfaces streamlines code organization and promotes the encapsulation of shared utility methods within the interface definition.
Inheritance and Default Methods:
The interplay between inheritance and default methods in interfaces allows for a hierarchical composition of behaviors. Classes can inherit methods from interfaces while also providing custom implementations for specific methods. This combination promotes code reusability and flexibility, as classes can leverage default methods from interfaces while tailoring certain behaviors to meet individual requirements. By understanding the dynamics of inheritance and default methods, developers can design robust and adaptable class hierarchies that encapsulate shared functionalities.
Best Practices for Interface Class Usage
In the domain of Java programming, adhering to best practices for interface classes is paramount for crafting robust and scalable applications. By following these practices, developers can ensure code consistency, maintainability, and ease of collaboration within projects. Among the specific elements emphasized in this context include naming conventions and design guidelines, which play a crucial role in shaping the structure of software components. Well-defined interfaces with clear names contribute to better code readability and facilitate seamless integration with other modules, enhancing the overall quality of the codebase.
Naming Conventions and Design Guidelines
Descriptive Interface Names
When it comes to naming interfaces in Java, the principle of using descriptive and intuitive names is highly valued. Descriptive interface names provide a clear indication of the functionality and purpose of the interface, making it easier for developers to understand its role in the system. By choosing meaningful names that reflect the operations or services the interface encapsulates, developers can improve code comprehensibility and reduce the cognitive load required to work with the codebase. This approach promotes consistency and coherence in software design, aligning with industry best practices that prioritize clarity and semantic accuracy in naming conventions.
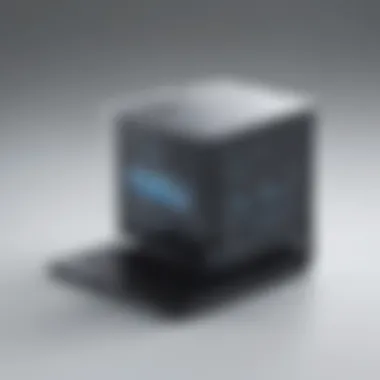
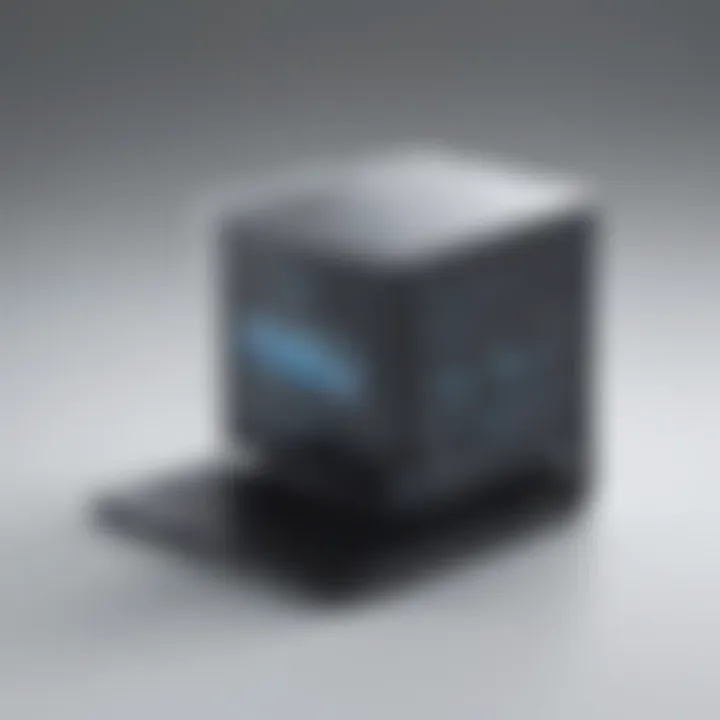
Single Responsibility Principle
The Single Responsibility Principle, a core tenet of object-oriented design, stipulates that each software component should have a single reason to change. When applied to interface classes, this principle advocates for interfaces that focus on a specific set of related functionalities, thereby promoting modular, maintainable code. By adhering to the Single Responsibility Principle in interface design, developers can isolate different responsibilities into separate interfaces, leading to loosely coupled components that are easier to understand, modify, and extend. This practice fosters code reuse and facilitates the evolution of software systems by mitigating the ripple effects of changes across the codebase.
Cohesive Method Signatures
Cohesive method signatures in interface classes are essential for ensuring logical grouping of methods that relate to a common purpose. By designing interfaces with cohesive method signatures, developers streamline the usability and understandability of the code, enabling efficient communication of intent between different parts of the system. Cohesive method signatures enhance code maintainability by reducing ambiguity and redundancy, promoting a clear separation of concerns within the software architecture. This practice not only improves code quality but also contributes to better testability, documentation, and overall system comprehensibility, aligning with industry standards for software development excellence.
Advanced Concepts and Considerations
In the realm of Java programming, advanced concepts and considerations play a pivotal role in shaping the landscape of software development. These components serve as the bedrock for innovation and efficiency within Java applications. Understanding these advanced concepts is crucial for developers to harness the full potential of the language and design robust, scalable solutions. By delving deeper into these concepts, developers can elevate their coding practices and create cutting-edge software that meets the dynamic demands of the industry.
Functional Interfaces and Lambda Expressions
Defining Functional Interfaces
Functional interfaces serve as a cornerstone in Java development, offering a streamlined approach to defining interfaces with a single abstract method. This simplification enhances code readability and conciseness, allowing developers to focus on the core functionality of their programs. The key advantage of defining functional interfaces lies in their flexibility and reusability, as they enable the implementation of specific behaviors without the need for extensive code repetition. However, it is essential to note that while functional interfaces reduce boilerplate code, they may constrain the design flexibility in certain scenarios, necessitating a careful balance between simplicity and extensibility.
Using Lambda Expressions with Interfaces
Lambda expressions represent a significant milestone in Javaβs evolution, revolutionizing the way developers write concise and expressive code with functional interfaces. By seamlessly integrating lambda expressions with interfaces, developers can leverage the power of functional programming paradigms and streamline their coding workflow. The primary benefit of using lambda expressions lies in their ability to encapsulate behavior as data, enabling a more expressive and succinct coding style. Despite the advantages they offer, lambda expressions may introduce complexities in debugging and readability, requiring developers to strike a balance between brevity and clarity in their code.
Functional Interface Annotations
Functional interface annotations provide additional meta-information to functional interfaces, enhancing their documentation and aiding developers in understanding the intended purpose of these interfaces. By annotating functional interfaces, developers can communicate critical information about the interface's usage, inheritance relationships, and potential constraints. The key feature of functional interface annotations is their ability to offer descriptive cues to both developers and automated tools, promoting better code comprehension and maintainability. However, the overuse of annotations can lead to code clutter and diminish code readability, highlighting the importance of judicious annotation usage in Java programming.
Marker Interfaces and Serialization
Role of Marker Interfaces in Java
Marker interfaces serve as passive indicators in Java, signaling a specific trait or behavior associated with classes that implement them. These interfaces play a pivotal role in triggering certain functionalities or indicating the readiness of a class for specialized operations. The primary benefit of marker interfaces lies in their ability to provide a simple and effective mechanism for categorizing classes based on shared characteristics, facilitating streamlined functionality integration. However, the reliance on marker interfaces may lead to a rigid class hierarchy and hinder code modularity, necessitating a balance between flexibility and adherence to Java's marker interface conventions.
Implementing Serializable Interface
The Serializable interface in Java enables objects to be serialized into byte streams, allowing for seamless object persistence and communication across network boundaries. By implementing the Serializable interface, developers can serialize objects and transmit them between different Java instances, facilitating efficient data exchange and storage. The key characteristic of the Serializable interface is its role in defining a class as serializable, marking it eligible for the serialization process. While serialization simplifies complex data transfer tasks, it introduces serialization overhead and potential security vulnerabilities, emphasizing the need for secure serialization practices in Java development.
Custom Serialization Handling
Custom serialization handling empowers developers to customize the serialization and deserialization process according to specific requirements, offering fine-grained control over object persistence. By defining custom serialization mechanisms, developers can address unique serialization challenges and optimize the performance of serialization operations. The unique feature of custom serialization handling lies in its ability to tailor the serialization process to suit diverse data structures and optimization goals, enhancing the efficiency and robustness of serialization tasks. However, custom serialization implementations may introduce complexity and maintenance overhead, necessitating careful consideration of trade-offs between customization and simplicity in serialization design.
Interface Evolution and Versioning
Adding New Methods to Interfaces
The evolution of interfaces in Java involves the addition of new methods to existing interfaces, enabling backward-compatible changes and enhanced functionality without breaking existing implementations. By augmenting interfaces with new methods, developers can extend the capabilities of interfaces and accommodate evolving requirements while preserving compatibility with older codebases. The key characteristic of adding new methods to interfaces is its ability to promote interface extensibility and facilitate gradual migration to newer interface versions. However, introducing new methods to interfaces may pose challenges in maintaining backward compatibility and managing interface dependencies, underscoring the importance of thoughtful interface evolution strategies in Java development.
Interface Compatibility and Backward Compatibility
Interface compatibility and backward compatibility are essential considerations in Java programming, ensuring seamless integration of new interfaces with existing codebases and preserving system stability across version upgrades. By adhering to compatibility standards, developers can avoid breaking changes and maintain the integrity of interface interactions within complex software ecosystems. The key characteristic of interface compatibility is its role in facilitating code interoperability and minimizing disruptions during system updates. However, ensuring backward compatibility may constrain interface design choices and limit the scope of interface enhancements, necessitating a delicate balance between innovation and backward compatibility in interface evolution.
API Design Considerations
API design considerations encompass a broad array of principles and best practices that guide the development of application programming interfaces in Java. These considerations address various aspects of API design, including interface clarity, consistency, and flexibility, to ensure optimal usability and developer experience. The key feature of API design considerations is their focus on creating intuitive and well-documented interfaces that promote code reuse and interoperability. While adhering to API design considerations enhances software maintainability and extensibility, overlooking these principles may lead to API fragility and usability issues, highlighting the significance of conscientious API design in Java applications.