In-Depth Exploration of Java Collections Framework
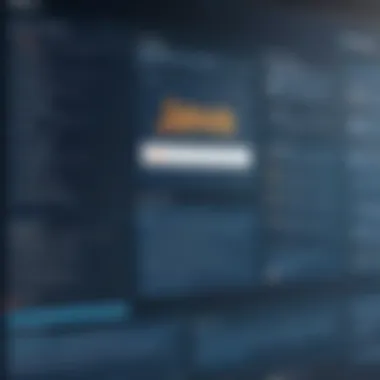
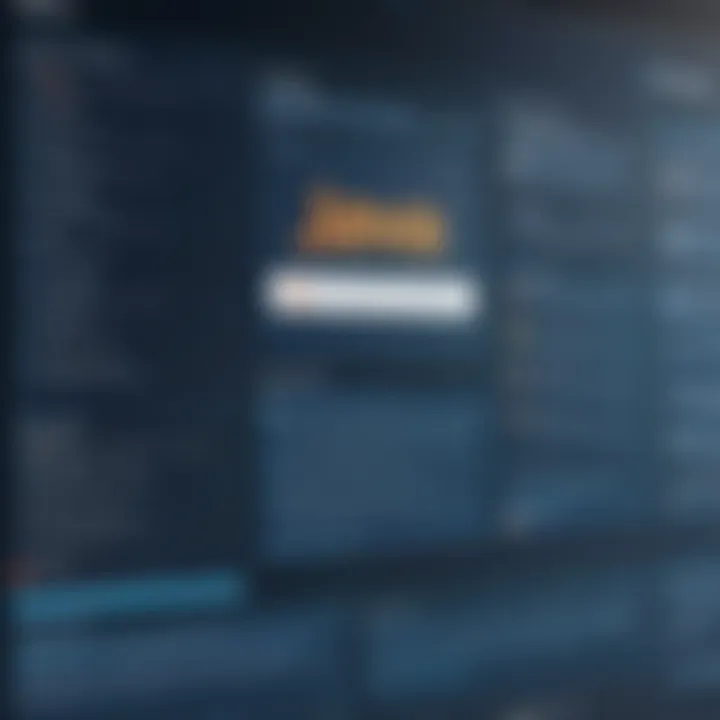
Intro
The realm of software development encompasses a multitude of tools and frameworks that drive efficiency and innovation. Among these, Java Collections stands out for its structured approach to managing groups of objects. Understanding its architecture is essential for developers looking to enhance their coding strategies. Java Collections is not just a simple framework; it acts as a foundation for creating robust applications that harness the power of data structure management.
Java Collections Framework (JCF) offers a rich set of interfaces, implementations, and algorithms. This allows developers to manipulate data effectively and optimally. This article delves into the various components of Java Collections, highlighting essential features, unique characteristics, and practical applications relevant to software development.
Overview of Java Collections
Java Collections provides a unified architecture for representing and manipulating collections, which are essentially groups of objects. The definitions within this framework include key features such as stability and performance. A solid grasp of collections is necessary for modern programming tasks. This section explores what Java Collections encompasses and what makes it a vital component in software development.
Definition and Importance of Java Collections
The Java Collections Framework serves as an essential toolkit for managing data structures. By offering several interfaces like List, Set, and Map, it allows developers to choose the appropriate collection type based on specific requirements. This adaptability is crucial for achieving the desired outcomes in applications, making it a necessary study area for any Java developer.
Key Features and Functionalities
Java Collections Framework boasts several formidable features:
- Interfaces and Implementations: A set of well-defined interfaces and their concrete implementations provide flexibility.
- Algorithms: Built-in methods for sorting and searching data enhance performance.
- Type Safety: Generics enhance type safety, minimizing runtime errors.
- Concurrency Support: Classes like ConcurrentHashMap facilitate multi-threaded programming.
Best Practices
To maximize the efficiency of Java Collections in application development, certain best practices should be observed:
- Choose the Right Collection Type: Assess your needs and pick the appropriate type to ensure optimal performance. For instance, utilize ArrayList for dynamic arrays and HashSet for unique collections.
- Avoid Unnecessary Wrappers: Keeping code clean and avoiding excessive wrapping may improve performance.
- Leverage Generics: Utilize generics to prevent ClassCastException and ensure type safety.
- Consider Immutability: Whenever possible, use immutable collections to avoid unintended changes.
Common Pitfalls to Avoid
Implementation of Java Collections can lead to inefficiencies if best practices are not followed. Common pitfalls include:
- Using the Wrong Collection: Selecting a collection type ill-suited for a task can hurt performance.
- Not Using Iterators Properly: Failing to use iterators correctly can lead to ConcurrentModificationException.
Case Studies
Real-world applications of Java Collections provide insight into their practical uses:
- E-commerce Platforms: Utilizing HashSet for product inventory helps ensure that each product is unique.
- Social Media Applications: Leveraging ArrayList to handle user comments allows for dynamic content management, enabling fast retrieval and display.
Lessons from these implementations underline the importance of selecting the right collection based on context and performance requirements.
Latest Trends and Updates
As technology evolves, so too does the Java Collections Framework. Current trends indicate:
- Integration with Functional Programming: Java 8 introduced streams to work with collections, allowing for more functional-style programming.
- Increased Focus on Concurrency: As multi-threading becomes a more common requirement, enhanced concurrent collections are gaining prominence.
Upcoming advancements in the field
Innovations may include improvements in performance metrics and enhanced APIs to support more complex data structures efficiently.
How-To Guides and Tutorials
To make the most of Java Collections, consider these steps:
- Start with the Basics: Begin by understanding key interfaces and implementations.
- Hands-On Practice: Engage in small projects using various collections to develop familiarity.
- Conduct Performance Analysis: Use tools to analyze the performance of different collection types in your specific use cases.
Practical tips, such as utilizing Java's documentation available on Wikipedia and community resources at Reddit, are invaluable for aspiring Java developers.
Intro to Java Collections
The Java Collections Framework (JCF) is a fundamental component of Java programming. Its importance cannot be overstated when discussing how Java handles groups of objects. There is a variety of collection types, each tailored for specific tasks and performance needs. Understanding these collections is essential for software developers, IT professionals, and data scientists seeking to optimize code and improve efficiency.
Definition and Purpose
Java Collections provide a structured means to manage and manipulate groups of objects. The framework defines a set of interfaces and classes that facilitate working with data structures, like lists, sets, and maps. The primary purposes include:
- Storage: Collections allow for the effective organization of large numbers of objects.
- Manipulation: They provide methods for searching, inserting, and deleting elements with efficiency.
- Iteration: Java Collections enable iterating over groups of elements conveniently and cleanly.
These unified tools make coding simpler and more intuitive. By leveraging the framework, developers avoid the intricacies of building such structures from scratch, thus focusing on their application logic.
Historical Context
The evolution of Java Collections reflects the advancing needs of developers and the growing complexity of applications. Initially, Java provided simple arrays and rudimentary data management tools. However, as application demands increased, the need for a more versatile solution emerged.
In 1998, with the release of Java 2 (JDK 1.2), the Java Collections Framework was introduced. This marked a significant turning point by offering robust data structures as part of the Java Development Kit (JDK). Since its release, the framework has undergone numerous enhancements, including the introduction of new collection types and interfaces, and improved performance.
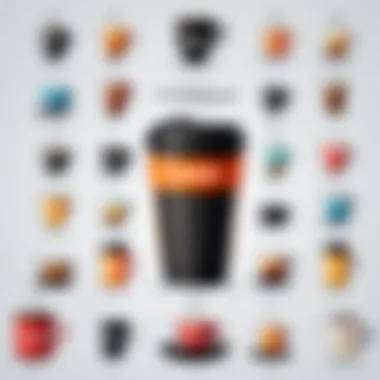
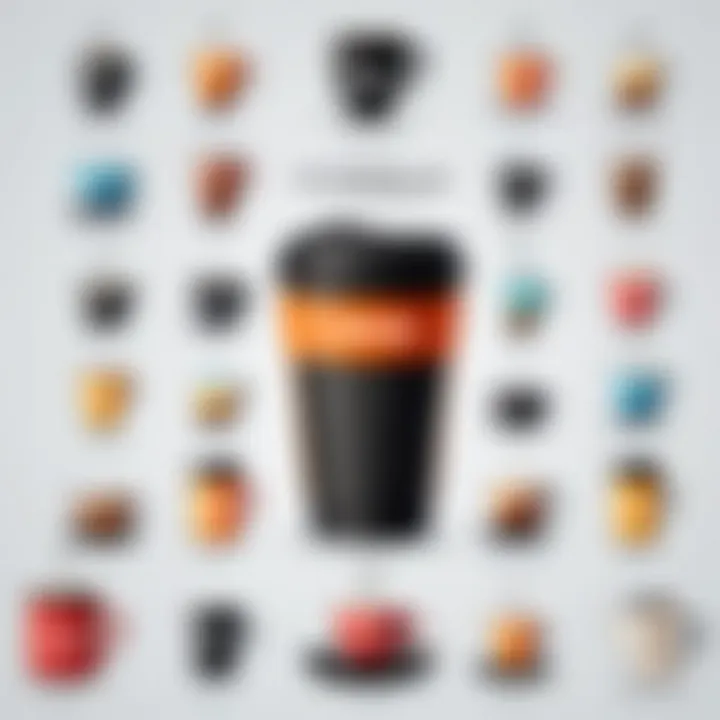
The historical development of JCF has shaped how Java is utilized today. Its thoughtful design has integrated better algorithms and allowed for concurrency support, which remains crucial in multi-threaded applications. Understanding this history gives developers insight into how best to utilize collections for their needs.
"The Java Collections Framework is like a library that contains all the necessary tools for efficient data manipulation."
This background helps in appreciating the role Java Collections play in modern software development.
Understanding the Java Collections Framework
The Java Collections Framework (JCF) plays a crucial role in managing groups of objects in Java programming. It provides a well-defined architecture that helps developers store and manipulate collections efficiently. The framework encompasses several interfaces, implementations, and algorithms, which enhances the overall effectiveness of data handling. Understanding JCF is fundamental as it lays the groundwork for optimal data structure management, leading to enhanced performance in applications.
Key Interfaces
Collection Interface
The Collection Interface is the root of the collection hierarchy in Java. It defines the core methods that all collections should implement. This interface contributes significantly to the overall structure of the framework by ensuring a unified way to access different types of collections. One key characteristic of the Collection Interface is its ability to allow polymorphism. This means that a developer can refer to any collection type using a common interface, enhancing flexibility in coding. The unique feature of this interface is its provision of basic operations like adding, removing, and iterating through elements. The advantages include ease of use and consistency when working within the framework. One potential disadvantage is that it does not provide any methods specific to order or randomness; these must be implemented in the child interfaces.
List Interface
The List Interface extends the Collection Interface and introduces a sequence of elements. It is crucial for scenarios where order matters, as it allows duplicates and permits positional access. A key characteristic of the List Interface is its support for indexed access to elements, which is essential for applications requiring manipulation of data sequences. The List Interface is favorable for applications where you need to maintain order and allow duplicate entries. A unique feature of this interface is the ability to insert, update, or remove elements at specific positions. Although Lists are powerful, they may consume more memory compared to other collection types, which could impact performance in large datasets.
Set Interface
The Set Interface, another extension of the Collection Interface, focuses on storing unique elements and preventing duplicates. This characteristic is valuable when dealing with a collection where each item must only appear once, such as a group of user IDs. The key aspect of the Set Interface is its fundamental property of uniqueness. This makes Sets a popular choice in applications that require fast membership tests. A unique feature is the efficient implementation of operations like union and intersection, which can be beneficial in complex data manipulation tasks. However, Sets do not maintain order, which may limit their utility depending on the scenario.
Map Interface
The Map Interface, while not a true collection, is essential for key-value pair storage. It provides a framework for associating unique keys with corresponding values. This interface is particularly useful for scenarios that require quick lookups based on a key, such as configurations or databases. One key characteristic of the Map Interface is that it allows for the storage of data in a way that is easily accessible and manageable. A unique feature of this interface is its ability to include null values and keys, providing flexibility not found in other collection types. On the downside, Maps can be complex and require more memory, especially when there are many key-value pairs.
Common Implementations
ArrayList
ArrayList is a widely used implementation of the List Interface. It offers dynamic arrays that can grow as needed, making it a popular choice for developers needing flexible data structures. A defining characteristic of ArrayList is its ability to allow random access to elements, which enhances lookup speed significantly. Its unique feature lies in its capacity for dynamic resizing, which means it can accommodate growing datasets without performance degradation. However, the trade-off for this flexibility is that adding or removing elements can be slow if it requires resizing the underlying array.
LinkedList
LinkedList is another implementation of the List Interface that uses nodes to store data. It allows easy insertions and deletions, which is beneficial for applications where data is frequently modified. A significant characteristic of LinkedList is its efficient handling of memory allocation. Unlike ArrayList, which requires resizing, LinkedList can grow or shrink as necessary without reallocating the entire structure. The unique feature is its ability to insert elements at both ends efficiently. Unfortunately, it does not support random access as well as ArrayList does, which can lead to slower access times for index-based retrievals.
HashSet
HashSet is a popular implementation of the Set Interface. It stores its elements in a hash table, allowing for efficient data retrieval and insertion. A key characteristic of HashSet is its performance, especially for operations like add, remove, and contains, which generally offer constant time complexity. Its unique feature is the ability to quickly determine if an item is already present. However, the lack of order may be a disadvantage if the sequence of elements is important in an application.
TreeSet
TreeSet, also an implementation of the Set Interface, stores its elements in a sorted tree structure. This guarantees that the elements are sorted in natural order or by a specified comparator. The key characteristic of TreeSet is its ability to maintain order among the elements, making it useful for scenarios requiring sorted collections. A unique feature is the ability to perform range searches. However, the TreeSet can be slower for common operations as it needs to maintain its sorted properties, which may impact performance in applications requiring frequent modifications.
Algorithms for Collection Manipulation
Algorithms for collection manipulation form a critical backbone of the Java Collections Framework. They provide predefined operations for sorting, searching, and modifying collections. These algorithms ensure that developers can focus on application logic rather than on the underlying data structure complexities. Using standardized algorithms also increases code portability and reduces errors, as developers can utilize well-tested solutions.
Types of Collections in Java
Understanding the various types of collections in Java is fundamental for any software developer or IT professional. Collections serve as the backbone for managing groups of objects. Each type of collection has its own unique characteristics and behaviors that cater to different programming needs. This section delves into List, Set, and Map collections, describing their structures, advantages, and specific use cases. By grasping these concepts, developers can make informed decisions, optimizing performance and resource management in their applications.
List Collections
Characteristics of List
List collections in Java maintain an ordered sequence of elements. This structure allows for the duplication of elements, which is one of its key characteristics. Lists facilitate efficient data retrieval by position, making them particularly useful when order matters. Additionally, the ability to insert and remove elements at specific indices further enhances their flexibility. This characteristic makes lists a popular choice in many applications.
One unique feature of List collections is their capability to store data in a dynamic manner. As elements are added or removed, the List can grow or shrink accordingly. This offers a significant advantage over traditional arrays, which have fixed sizes. However, it is also important to consider that operations such as insertion or removal can be costly in terms of performance, especially when handling a large number of items.
Use Cases for Lists
Lists are widely used in several applications due to their versatility and dynamic nature. Their sequential access makes them particularly suitable for scenarios like maintaining a playlist or storing user input data where duplicates may occur. Since the order of elements is preserved, developers rely on Lists when the sequence of data must be consistent.
In practical terms, you might find Lists employed in GUI applications, where user actions can be logged in a particular sequence, or in data processing tasks that require frequent updates and retrievals. However, users should be mindful of performance considerations, particularly with large data sets, as heavy modifications may lead to inefficiencies.
Set Collections
Unique Elements
The Set collection in Java is designed to store unique elements, effectively preventing duplicates. This fundamental characteristic not only ensures data integrity but also simplifies the management of collections. For instance, when you need a collection of unique items, such as user IDs or tags, Sets are the ideal choice.
Another notable feature of Sets is their ability to perform set operations like union, intersection, and difference efficiently. These operations can be crucial in applications that require comparisons, like database record processing. However, it is important to realize that, due to their unique nature, Sets do not guarantee the order of elements, which may limit their use in certain applications.
Use Cases for Sets
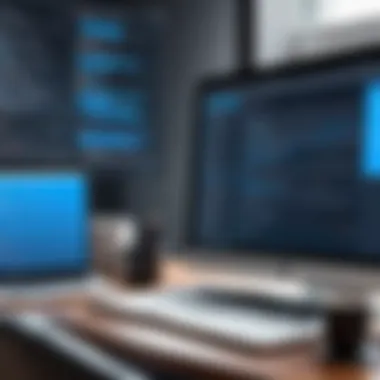
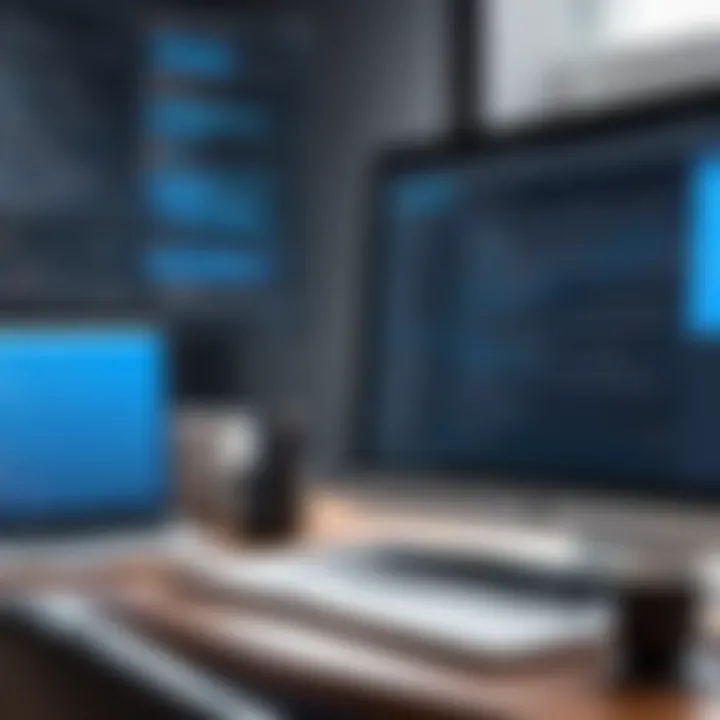
Sets are efficient in scenarios where uniqueness is paramount. For example, they are well-suited for scenarios like maintaining a list of unique users who have access to a system, or tracking unique product IDs in an inventory system.
Their performance benefits become particularly evident when performing operations that involve checking for the existence of items. For developers who need to manage large collections without duplicates, using Sets helps streamline code and improve execution times, although the lack of order can sometimes be a drawback.
Map Collections
Key-Value Pair Structure
Map collections in Java consist of key-value pairs, where each key is unique. This structure is highly beneficial, as it allows for efficient data retrieval using keys. The relationship between keys and values offers intuitive access mechanisms that aid in data organization.
One of the unique features of Maps is their ability to store and manage large sets of data while providing quick lookups. However, the insertion and data retrieval can sometimes lead to high memory usage depending on the implementation. Understanding the underlying Map types, such as HashMap or TreeMap, helps developers choose the most suitable one for their needs.
Use Cases for Maps
Maps are versatile across a vast range of applications. Typical use cases include implementing caches, maintaining user settings in applications, or even as a representation of data structures like graphs. The key-value pairing is especially useful when associating user profiles with their data.
In summary, Map collections serve as powerful tools for developers looking to efficiently match keys to values. Understanding the unique aspects of Map collections and their use cases can enhance data handling and retrieval operations in complex software systems.
Performance Considerations
Understanding the performance characteristics of Java Collections is vital for software developers and IT professionals. This section delves into two critical aspects: time complexity and space complexity. These elements directly impact the efficiency of algorithms and the use of memory, thus influencing the overall application performance. By grasping these concepts, developers can make informed choices about which data structures to employ, ensuring that applications run smoothly and efficiently under various conditions.
Time Complexity Analysis
Time complexity measures the time an algorithm takes to complete as a function of the length of the input. In the context of Java Collections, it is crucial to analyze how different operations (like adding, removing, or accessing elements) affect performance. Each collection type has unique properties influencing these time complexities.
For example,
- An ArrayList allows for quick random access with O(1) time complexity due to its underlying array structure. However, adding elements randomly can lead to O(n) time complexity if the array needs resizing.
- A LinkedList, on the other hand, has O(1) complexity for adding or removing elements when the reference is known, yet accessing an element requires O(n) due to the need to traverse the list.
- The HashSet can typically retrieve elements in O(1) time, assuming a good hash function, but in the case of collisions, it may degrade to O(n) in the worst-case scenario.
Understanding these intricacies fosters better choices when selecting collections based on their expected use case.
Space Complexity Insights
Space complexity considers the amount of memory an algorithm uses in relation to the size of the input data. This aspect is especially relevant in Java Collections, as different collection types consume variable amounts of memory.
- An ArrayList consumes O(n) space, where n is the number of elements stored. Its space usage is more efficient than a LinkedList, which allocates additional memory for node pointers, thus incurring overhead.
- The HashMap typically requires more space due to its hash table structure, where both keys and values need storage. As it grows, it may also need to allocate additional capacity and thus affect performance due to resizing.
In any data-intensive application, evaluating both time and space complexities becomes essential for performance optimization. By analyzing these factors, developers can enhance data handling, optimize resource allocations, and ultimately improve the efficiency of their applications.
"The performance considerations of Java Collections are not merely theoretical; they shape how applications react under load and how efficiently they use system resources."
In summary, understanding time and space complexities allows developers to tailor their use of Java Collections effectively, ensuring both performance and reliability within applications. By considering these metrics, they can avoid bottlenecks and manage resources wisely.
Best Practices for Using Java Collections
Effective utilization of Java Collections is crucial for software developers and IT professionals aiming to write efficient and maintainable code. Understanding best practices helps avoid common pitfalls and enhances data manipulation and management. This section explores practical strategies for leveraging the Java Collections Framework effectively.
Choosing the Right Collection Type
Selecting the appropriate collection type is vital. Different collection types serve distinct purposes, and understanding these differences allows developers to optimize their code.
- List: When needing to maintain an ordered collection that allows duplicates, choose or . For quick access and frequent updates, is often preferred, while is better for scenarios where insertions and deletions are frequent.
- Set: If uniqueness of elements is required, use or . While offers faster access and insertion, keeps elements sorted.
- Map: For key-value associations, provides average O(1) time complexity for access, while maintains insertion order.
Hence, understanding the characteristics and suitable use cases of each collection type is essential. Consider both time and space complexities when making your choice.
Avoiding Common Pitfalls
Certain behaviors can lead to inefficient code or bugs when using Java Collections. Recognizing these pitfalls helps developers write cleaner and more efficient code.
- Not Understanding Null Handling: Some collection types, like and , allow null elements while others may not behave predictably with nulls. Understanding how each collection handles nulls is important.
- Using Synchronized Collections: Many developers confuse thread safety with synchronization. Instead of trying to synchronize standard collections, consider using classes in the package, like .
- Excessive Iteration: Be cautious about how often you iterate through collections. Frequent iterations in large collections can significantly affect performance. Using iterators effectively can mitigate this issue.
Always preferring appropriate collection types and understanding their underlying mechanics forms the backbone of efficient Java programming.
By adhering to these best practices in choosing the right collection and avoiding common pitfalls, software developers can maximize the performance and reliability of their applications.
Concurrency and Java Collections
Understanding concurrency in Java Collections is crucial for writing efficient and robust applications. Concurrency allows multiple threads to execute simultaneously, which enhances performance. However, when dealing with collections that are accessed by multiple threads, issues such as data inconsistency and race conditions can arise. Thus, proper thread safety mechanisms are important. The goal here is to ensure that collections can be shared between threads without leading to unintended effects.
Understanding Thread Safety
Thread safety in Java Collections refers to the ability of a collection to function correctly when accessed from multiple threads. A collection that is not thread-safe can lead to problems, such as lost updates or corrupted data. To mitigate these risks, developers can utilize synchronized collections or those designed for concurrent access. Understanding the distinction here is vital for performance and stability in applications where threads interact with shared data.
Concurrent Collections Overview
Concurrent collections are specialized classes designed to handle concurrent access more effectively than standard collections. They reduce the necessity of explicit synchronization, which can lead to performance bottlenecks. Two notable concurrent collections in Java are the CopyOnWriteArrayList and ConcurrentHashMap.
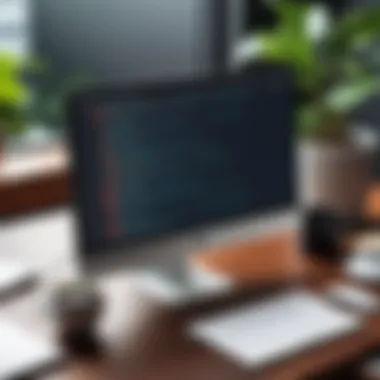
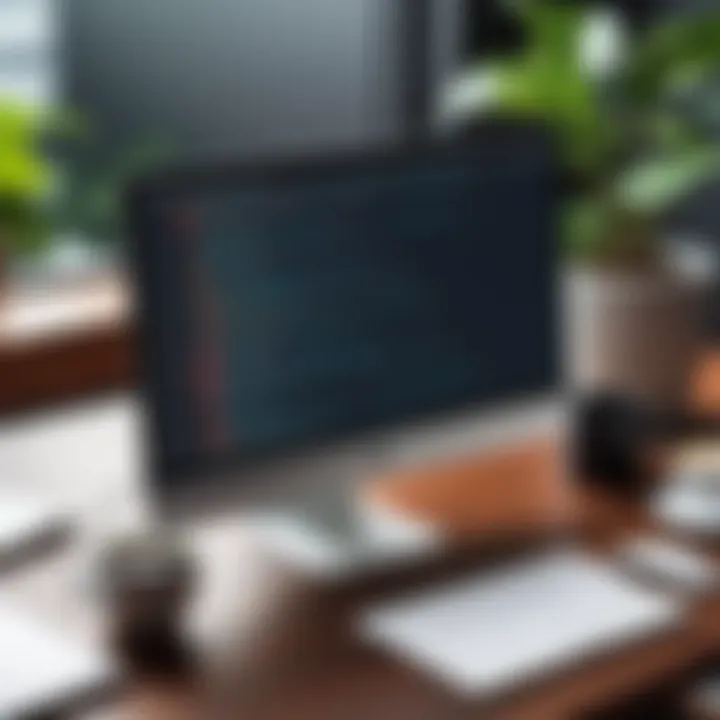
CopyOnWriteArrayList
The CopyOnWriteArrayList is a concurrent collection that has a unique approach. It creates a new copy of the underlying array whenever a write operation occurs, such as adding or removing elements. This characteristic of immutability during reads enhances performance under concurrent accesses, especially in scenarios with frequent reads and infrequent modifications. The primary benefit of CopyOnWriteArrayList is its simplicity in coding and its ability to offer thread-safe operations on lists.
However, while it is beneficial for scenarios with many readers and few writers, its unique feature also presents disadvantages. For example, it can lead to increased memory usage since new copies of the array are created for every write operation. Additionally, if write operations happen frequently, performance can degrade significantly due to the overhead of copying.
ConcurrentHashMap
ConcurrentHashMap is another significant concurrent collection that is widely used in Java. This map allows concurrent access without significant contention. It accomplishes this by dividing the map into segments and allowing multiple threads to read and write to different segments simultaneously. Its notable characteristic is its scalability, making it an excellent choice for high-throughput applications.
The unique feature of the ConcurrentHashMap lies in its design that permits concurrent retrieval and updates. As a result, the operations are generally faster than synchronized maps. However, users should note that even with the advantages, it does not guarantee total consistency across the map, especially during updates. Understanding how to use ConcurrentHashMap effectively is key to building high-performance and reliable applications in Java.
"Concurrency is about doing many things at once. When it comes to collections, choosing the right approach is essential for success."
In summary, concurrency significantly shapes how Java Collections are utilized within applications. By choosing the right concurrent collections, developers can ensure data integrity while optimizing performance for applications that require multi-threading. The CopyOnWriteArrayList and ConcurrentHashMap highlight the diverse strategies available for handling concurrency, providing essential tools for effective software development.
Real-World Applications
Understanding the real-world applications of Java Collections is crucial for software developers and IT professionals. The ability to effectively manage data structures can significantly impact the performance and scalability of applications. This section delves into two primary areas: data handling in software development and the role of collections in various frameworks and libraries. Each aspect reveals the versatility and importance of the Java Collections Framework (JCF).
Data Handling in Software Development
Java Collections provide a systematic way to handle data. Software development often requires developers to deal with dynamic and complex datasets, which can be efficiently manipulated using collections. For instance, when a developer needs to store customer information for an e-commerce application, a can be used to efficiently store and retrieve user data based on unique customer IDs.
In data handling, several key benefits of Java Collections standout:
- Efficiency: The varied data structures like , , and allow for optimizing data access and updates.
- Flexibility: Different data types can be handled through generics, promoting type safety without sacrificing performance.
- Ease of Use: High-level abstractions make it simpler for developers to implement algorithms for data manipulation without complex logic.
Consider a scenario where a software application requires filtering and sorting data frequently. Instead of implementing a complex algorithm, developers can leverage built-in methods provided by the collections framework. Using the class for sorting allows for cleaner code and reduced chances of error.
Use in Frameworks and Libraries
Java Collections play a foundational role in various frameworks and libraries, enhancing their capabilities. Many popular frameworks, like Spring and Hibernate, rely on these collections to manage data effectively.
Some important points include:
- Spring Framework: Utilizes collections in its dependency injection and management of beans. Lists and maps are commonly employed to configure application contexts.
- Hibernate: Makes use of collections to manage relationships in data models. For example, a might be used to represent the unique emails of users in a certain system, ensuring data integrity and preventing duplicates.
The integration of Java Collections into frameworks simplifies tasks like data storage, retrieval, and manipulation. Frameworks provide built-in support for collections, allowing developers to focus on higher-level application designs rather than low-level collection management.
Using the Java Collections Framework effectively enhances not just individual applications but also improves the overall architecture of software solutions.
Future Trends in Java Collections
The landscape of Java Collections is always evolving with technology advancements. Understanding future trends in Java Collections is crucial for software developers and IT professionals. These trends not only enhance efficiency in software development but also ensure that applications remain scalable and maintainable.
Evolution of Collections Framework
The Java Collections Framework has seen significant changes since its introduction. Initially, it primarily focused on performance and memory efficiency. Over the years, new collection types have emerged, and existing structures have evolved to include more versatile data handling capabilities. For instance, the introduction of the API in Java 8 allows developers to process and manipulate collections with functional programming techniques. This shift enhances readability and expressiveness of the code.
Moreover, the evolution of the framework incorporates better optimizations and additional features that enable easier integration with other libraries. As developers migrate to more modern architectural patterns, such as microservices, they may find new ways to leverage collections effectively within their applications. This means understanding changes in data structures will be vital.
Important aspects include:
- Greater emphasis on immutability, leading to safer data access patterns.
- The combination of collections with reactive programming and asynchronous data processing.
Impact of Functional Programming
Functional programming has begun to play a crucial role in how Java developers utilize collections. With features like lambda expressions and method references introduced in Java 8, developers can write more concise and intuitive code.
This programming paradigm encourages a different mindset when handling data collections. For instance, the use of streams allows operations like filtering, mapping, and collecting data to be performed in a more declarative manner. Below are some implications of functional programming on Java Collections:
- Higher-Order Functions: Collections can now be transformed using functions passed as parameters.
- Immutability and State Management: This encourages a safer approach to handling data while mitigating side effects.
As functional programming continues to gain traction, developers must adapt their approaches to collections. This adaptation not only enhances productivity but also improves code maintainability.
The ongoing integration of these modern principles into Java Collections fosters powerful tools that can transform how developers approach and solve complex problems.
By preparing for these trends and adapting accordingly, software professionals can stay ahead in a rapidly changing field.
The End
The conclusion of this article reinforces the critical nature of Java Collections within the software development landscape. A solid understanding of collections enhances developers' ability to manage data efficiently and improve code performance. The wealth of information provided leads to insights that can optimize everyday coding practices. Key topics include the architecture of the Java Collections Framework, the various collection types, and the role they play in real-world applications. In a field where efficiency and performance are paramount, recognizing the strengths and constraints of different collection implementations is vital.
Key Takeaways
- Java Collections Framework serves as the backbone for data manipulation in Java applications.
- Understanding different types, such as Lists, Sets, and Maps, allows for informed choices in coding.
- Performance considerations, including time and space complexity, optimize applications, ensuring faster execution and lower memory usage.
- Best practices and avoidance of common pitfalls lead to cleaner, more maintainable code.
Looking Forward
As we move into an era dominated by functional programming paradigms and increased demand for concurrent processing, the Java Collections Framework is likely to evolve. Future versions may integrate enhancements that focus on functional interfaces and better thread safety. Staying informed about these upcoming changes will equip developers with the tools necessary to adapt their coding strategies. New trends in data handling will emerge, and those well-versed in Java Collections will be positioned to tackle these challenges head-on, adapting to new development environments and techniques.