Understanding Functions: Their Critical Role in Coding
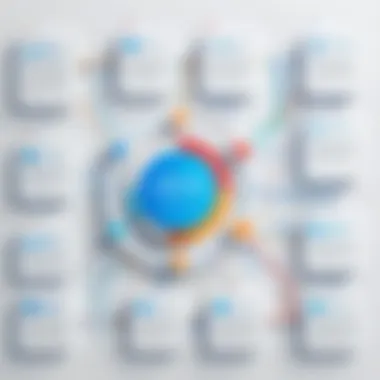
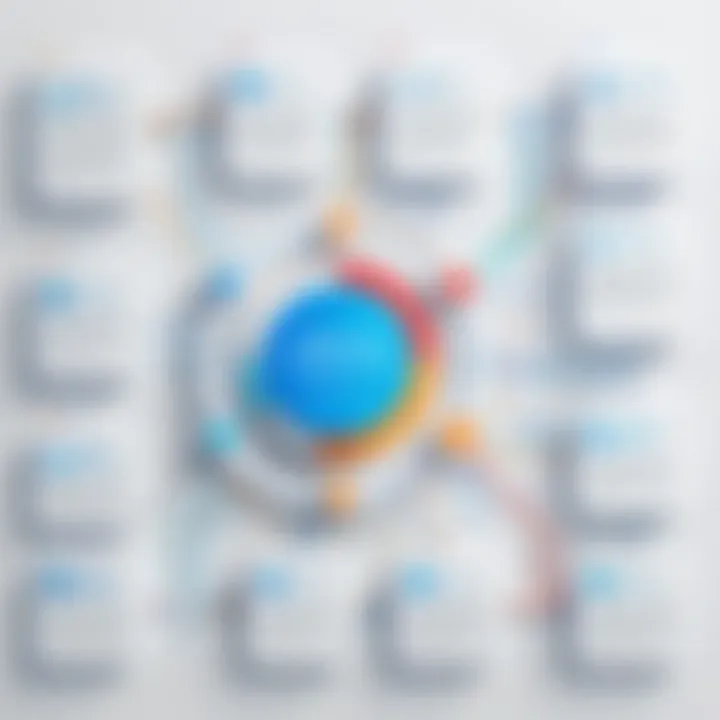
Intro
Functions are intrinsic to coding, serving not only as building blocks but also as the framework for effective software development. They encapsulate reusable code, allowing developers to break complex problems into manageable parts. This article examines the crucial role of functions, including their significance in modular design, code reusability, and project scalability.
By understanding the function's nature and purpose, developers gain essential insights into creating sophisticated applications and systems. Ranging across various programming languages, functions provide vital mechanisms for streamlining processes and enhancing code clarity. As we delve deeper into the role they play, it becomes evident why a comprehensive understanding of functions is pivotal for anyone engaged in software development.
Overview of Software Development
Software development is a systematic process that involves creating, designing, deploying, and supporting software applications. It calls for technical know-how, methodologies, and tools that enable developers to build functional products efficiently. Within this landscape, functions are fundamental to achieving a structured approach to coding.
Definition and Importance of Functions
Functions are self-contained blocks of code designed to perform a specific task. They help organize code, simplify debugging, and facilitate collaboration among developers. By utilizing functions, developers can reduce code redundancy and enhance readability, thereby making maintenance easier.
Key Features and Functionalities
- Encapsulation of Logic: Functions allow developers to bundle behavior, making code modular.
- Reusability: Functions can be reused in different parts of the program, saving time and effort.
- Improved Debugging: Isolating specific tasks in functions simplifies the process of testing and fixing bugs.
- Parameterization: Functions can accept inputs and return outputs, allowing for dynamic behavior.
Use Cases and Benefits
The advantages of using functions span numerous applications:
- Web Development: Functions manage UI interaction and data processing.
- Data Analysis: Functions streamline calculations and data manipulations.
- Machine Learning: Functions encapsulate algorithms for model training and evaluation.
In essence, the use of functions fosters efficiency, making them indispensable in the modern developer's toolkit.
Best Practices
To maximize the benefits of functions, adhering to industry best practices is crucial.
Industry Best Practices for Implementing Functions
- Keep Functions Focused: A function should accomplish one task only. This improves its maintainability.
- Proper Naming Conventions: Use meaningful names that convey the function’s purpose.
- Documentation: Commenting on code helps team members understand the function’s role and usage.
Tips for Maximizing Efficiency and Productivity
- Reuse existing functions where possible to reduce coding effort.
- Optimize performance by considering time and space complexity when designing functions.
Common Pitfalls to Avoid
- Overly Long Functions: Functions should be concise. Long functions can become difficult to manage.
- Poor Naming: Vague names can lead to confusion about a function's role, complicating code maintenance.
Case Studies
Real-world examples illustrate the power of functions in various environments.
Real-World Examples of Successful Implementation
- E-commerce Platforms: Companies like Shopify leverage functions to manage product inventory and process transactions efficiently.
- Machine Learning Workflows: Google’s TensorFlow utilizes functions to modularize its complex computational tasks.
Lessons Learned and Outcomes Achieved
- Enhanced Readability: Properly structured functions lead to cleaner, more understandable code.
- Scalability: Well-defined functions enable teams to scale projects and implement features more rapidly.
Latest Trends and Updates
The landscape of software development evolves continually.
Current Industry Trends and Forecasts
- The rise of serverless architecture emphasizes the importance of functions in building scalable applications.
- Tools like cloud functions and microservices are redefining how functions are structured in modern applications.
How-To Guides and Tutorials
For those looking to refine their skills or gain practical insight:
Step-by-Step Guides for Using Functions
- Identify the specific task you need to accomplish.
- Define the function’s parameters and return values clearly.
Practical Tips and Tricks for Effective Utilization
- Experiment with nested functions to handle complex problems in a structured way.
- Always test functions independently to ensure their correctness before integrating them into larger systems.
Understanding the role of functions in coding enhances development efficacy and promotes robust software design.
Preamble to Functions in Coding
Functions are a fundamental concept in programming, serving as a cornerstone for software development. They play a significant role in creating clean, modular code and enable developers to write reusable code blocks that enhance efficiency. This section aims to establish a solid understanding of functions, focusing on their definition and historical context to provide insights into their dramatic impact on coding practices.
Definition of Functions
A function is a self-contained block of code designed to perform a specific task. It can take input, called parameters, process it, and produce output known as return values. Functions promote code organization by encapsulating repetitive tasks within a single unit. This allows developers to avoid redundancy, which increases code clarity and reduces the likelihood of errors.
Functions generally consist of three primary components: a name, a set of parameters, and the function body that contains the code. For example, in Python, a simple function can be defined as follows:
This function, named , takes two parameters and returns their sum. By using functions, programmers can streamline their work, improve program readability, and facilitate debugging.
Historical Context
The concept of functions has its roots in mathematics but has evolved significantly since its inception in computer science. In the late 1950s and early 1960s, programming languages began incorporating function-like constructs to simplify coding processes. The development of procedural programming languages, such as Fortran and C, established the foundation for function definitions and usage, paving the way for modern programming paradigms.
Over time, the role of functions expanded beyond simple tasks. The introduction of object-oriented programming brought about the notion of methods—functions associated with objects. This marked a significant shift, allowing developers to write more intuitive and maintainable code. Today, functions are not just building blocks; they are integral components of various programming models, including functional programming, which treats computation as the evaluation of mathematical functions.
Types of Functions
Understanding the types of functions is key in grasping their overall role and importance in coding. Functions can be categorized based on their origin and usage, including built-in functions, user-defined functions, and anonymous functions. Each type serves distinct purposes and offers various benefits, enhancing the versatility of a programmer's toolkit. This section will explore these types in detail, showcasing how they fit within different programming contexts and their contribution to efficient coding practices.
Built-in Functions
Built-in functions are essential tools provided by programming languages that simplify common tasks. They are already defined and readily accessible, eliminating the need for programmers to write repetitive code blocks. Built-in functions cover a wide range of operations, from mathematical computations to string manipulation and data handling. For example, in Python, functions like get the length of a collection, and finds the maximum value in a list.
Here are some important benefits of built-in functions:
- Efficiency: Reduce the amount of code developers need to write and maintain.
- Reliability: These functions have been tested and optimized by the language designers.
- Consistency: Built-in functions provide a standard way to perform operations across projects, enhancing readability.
In summary, built-in functions play a critical role in programming, allowing for rapid development and less error-prone code.
User-defined Functions
User-defined functions, as the name suggests, are created by the programmer to perform specific tasks. This flexibility allows developers to encapsulate their unique logic and repeat it without rewriting code. By defining a function with parameters, users can customize the input and output according to their needs.
User-defined function benefits include:
- Modularity: Code can be divided into smaller, manageable pieces, making it easier to understand and maintain.
- Reusability: Once defined, these functions can be reused across various parts of the program or different projects, saving time and effort.
- Encapsulation: They help in hiding complexity from the main code, promoting cleaner and clearer code architecture.
Creating a user-defined function in Python might look like this:
The above function illustrates how a simple task can be encapsulated within a user-defined structure, thus streamlining the overall development process.
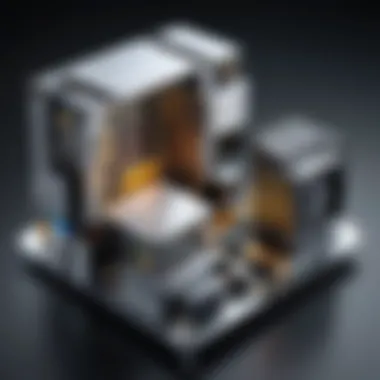
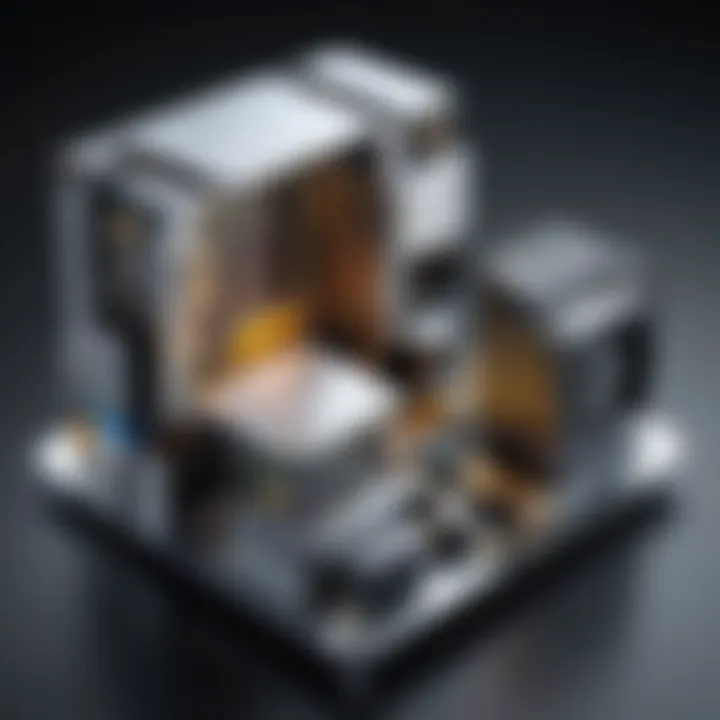
Anonymous Functions
Anonymous functions, also known as lambda functions in languages like Python or JavaScript, are functions without a name. They are often used for short-term tasks where defining a full function may appear excessive.
These functions can offer the following advantages:
- Concise syntax: They allow for less cluttered code in situations where a full function definition is unnecessary.
- Higher-order functions: They can be passed as arguments to other functions, providing flexibility in functional programming. For instance, passing an anonymous function to a sorting method can allow for custom sorting logic.
- Use in callback functions: They can simplify code when used as callbacks in asynchronous programming.
A common example in JavaScript might be:
Function Syntax Across Languages
The syntax of functions varies across programming languages, reflecting their unique philosophies and design principles. Understanding these differences is crucial because syntax influences how functions are defined, invoked, and utilized. Additionally, the clarity and structure of function syntax affect code readability and maintainability. Mastery of function syntax across multiple languages enhances a developer's versatility and problem-solving skills. This section discusses the syntactic particulars in three commonly used languages: Python, JavaScript, and Java.
Function Syntax in Python
Python functions are defined using the keyword followed by the function name and parentheses. Parameters are placed inside the parentheses, allowing for a flexible definition of inputs. The use of indentation is notable in Python, as it determines the block of code encapsulated within the function. Here's an example of a simple function definition in Python:
In this example, takes two parameters and and returns their sum. One important aspect of Python function syntax is its support for default parameters. This feature enables a function to be called with fewer arguments than defined, promoting greater versatility. For instance:
Here, if the argument for is omitted, it will default to , allowing to yield .
Function Syntax in JavaScript
JavaScript offers several ways to define functions, each with its nuances. The traditional approach uses the keyword, followed by the function name, parameters, and a code block enclosed in curly braces. Here’s an example:
In addition to the traditional function declaration, JavaScript has introduced arrow functions, which provide a more concise syntax. They eliminate the need for the keyword and use the operator. For example:
Arrow functions have the advantage of preserving the lexical context of , enhancing their usability in specific scenarios. Also, JavaScript supports default parameters similarly to Python, which enriches coding flexibility:
Function Syntax in Java
In Java, a function is typically referred to as a method, and it operates within the context of a class. The syntax requires specifying the return type before the method name. This is a critical distinction from Python and JavaScript. A basic method in Java looks like this:
Here, indicates access level, and defines the return type. One must declare the parameter types explicitly in Java, which enforces type safety but can add verbosity to the code.
Java also allows method overloading, which is the ability to create multiple methods with the same name but different parameter lists. For instance:
In this example, the method can accept different data types while maintaining the same name, illustrating the versatility of function definitions in Java.
Understanding function syntax across these languages provides valuable insight into their respective paradigms and functionalities. Each language showcases unique capabilities, influencing how developers structure their code to meet various application needs.
Parameters and Arguments
Understanding parameters and arguments is crucial for a deeper grasp of functions in coding. They serve as the means through which data is passed into functions. By using parameters and arguments effectively, developers can write more flexible and powerful code. They allow functions to operate on varying inputs, thus enhancing the code's reusability and modularity.
Understanding Parameters
Parameters act as placeholders within a function definition. They specify what type of information a function can accept. This specification allows a function to handle different types of input without having to redefine the function itself. Thus, when a function is called, arguments can be provided to fill these parameters. This setup creates a clear interface for the function, making it apparent what is required for the function to operate effectively.
Types of Arguments
Positional Arguments
Positional arguments are the most fundamental type of arguments in programming. Their placement in the function call is definitive, meaning that the order of arguments must match the order of parameters defined in the function. This characteristic makes positional arguments straightforward to use but can lead to confusion if the function accepts many parameters.
One key advantage of positional arguments is simplicity. They are easy to understand, especially for beginners. Furthermore, they allow for a clear declaration of the functional requirements. However, their rigidity can be a downside, particularly in instances where only some arguments need to be provided by the caller.
Keyword Arguments
Keyword arguments offer a flexible alternative to positional arguments. When using keyword arguments, a developer can specify which parameter an argument refers to by naming it directly in the function call. This feature greatly enhances code readability and maintainability.
The main benefit of keyword arguments is the ability to skip certain parameters or provide them in any order. This flexibility is particularly useful in functions with many parameters. However, keyword arguments may require more effort to implement in certain programming contexts, as they rely on the exact names of parameters defined in the function.
Default Arguments
Default arguments provide a way to assign a default value to a parameter if no argument is supplied by the caller. This feature contributes to the convenience of function calls, allowing certain parameters to be optional.
One significant aspect of default arguments is their efficiency. They help simplify function calls, particularly in cases where a logical default is appropriate. Yet, they can cause confusion if overused or if the default values are not well-documented. Developers must strike a balance between having useful defaults while still providing clear function usage guidelines.
"Parameters and arguments define how functions interact with inputs. Mastering this concept can greatly improve one’s coding skills and efficiency."
Return Values and Scope
Understanding return values and variable scope is fundamental to grasping how functions operate within programming. Functions can be thought of as tools that not only execute tasks but also communicate outcomes back to the calling code. This aspect makes return values critically important, while the concept of variable scope defines where variables can be accessed or modified in a program.
What are Return Values?
A return value is the output that a function produces after executing its code block. Every function is designed to perform a particular task, and its effectiveness is often measured by the correctness and usefulness of its return values. When a function completes its operation, it uses the keyword to send a value back to the point where it was called.
This mechanism allows for better modularity. Consider a function that calculates the sum of two numbers. When it returns the result, other parts of the program can utilize this data without repeating the calculation.
Here are some key points regarding return values:
- Data types: Functions can return a variety of data types including integers, strings, lists, or even complex objects, depending on the language used.
- Single return limit: Most languages allow only one return value per function. However, multiple values can be returned as packaged in an object or array.
- Void functions: Some functions do not return any value. These are often called void functions and are used when an operation doesn't require feedback.
Understanding Variable Scope
Variable scope refers to the accessibility and lifecycle of variables within a program. It defines where variables can be accessed within the code, which can affect how functions behave and how they interact with data.
Variables can have different scopes, including:
- Local scope: Variables declared within a function are only accessible inside that function. They cannot be reached outside, protecting the function's integrity and avoiding conflicts with other variables in the program.
- Global scope: Variables declared outside of any function can be accessed from anywhere in the program. This can create dependencies that make code harder to maintain.
The combination of return values and variable scope underscores the need for careful design in function development. When calling a function, understanding what it returns and how it influences the variables outside itself is crucial for ensuring reliable and efficient code.
Function Design Principles
Function design principles are fundamental to creating effective, efficient, and maintainable code in programming. These principles guide developers in writing functions that are easy to understand, reuse, and modify. By adhering to these principles, programmers can enhance the robustness of their applications and minimize the risk of introducing errors during development.
Benefits of Good Function Design
- Improved Code Readability: Functions that follow established design principles are typically easier to read and understand. This is crucial in collaborative environments where multiple developers work on the same codebase.
- Enhanced Reusability: Well-designed functions can be reused across different parts of an application or even in other projects. This reduces duplication of code and saves development time.
- Easier Maintenance: By keeping functions focused and organized, updates and bug fixes can be implemented with less effort. This is particularly important in long-term projects that may evolve significantly over time.
Single Responsibility Principle
The Single Responsibility Principle (SRP) states that a function should have one and only one reason to change. In other words, each function should perform a specific task or a closely related set of tasks. When a function adheres to SRP, it delineates its responsibilities clearly, which provides several advantages.
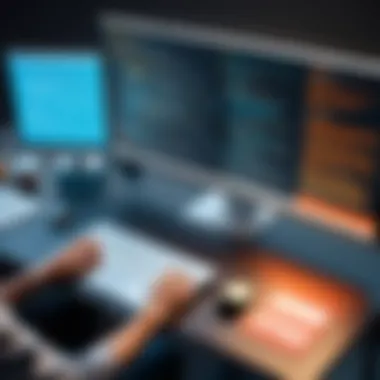
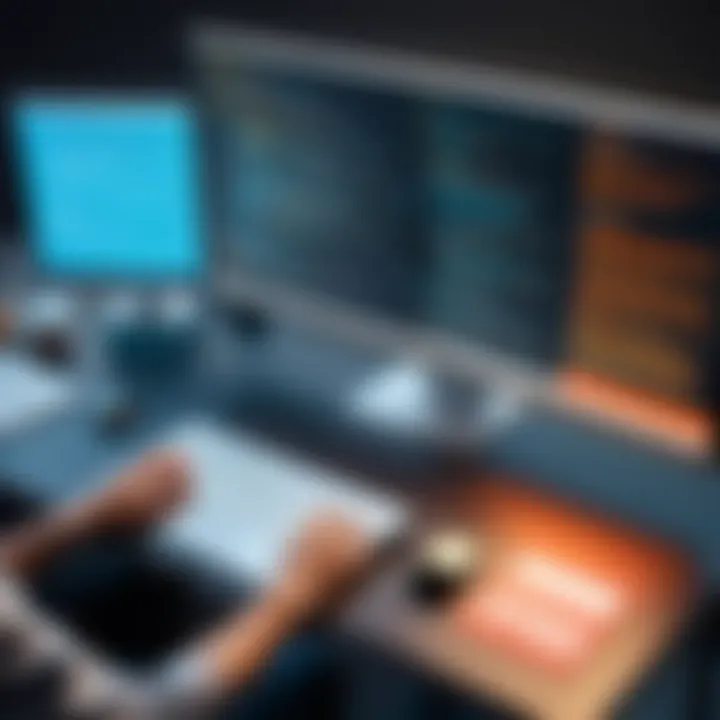
- Clarity: When a function does one thing, understanding its purpose becomes straightforward. For instance, consider a function designed solely for data validation. This clarity ensures that developers know what to expect and how to interact with that function.
- Testability: Functions that follow SRP are easier to test in isolation. This allows developers to create focused unit tests that validate the behavior of each function without the complications introduced by extraneous functionalities.
- Flexibility: Functions adhering to SRP can be modified or replaced without impacting other parts of the code. This promotes agility in development, particularly when adopting new technologies or refactoring existing code.
Code Maintainability
Code maintainability refers to how easily a programmer can make changes to a codebase over time. A strong focus on maintainability during function design ensures that code remains understandable and adaptable.
- Consistent Naming Conventions: Functions should have meaningful names that convey their purpose. For example, a function named is immediately clear about its function. Avoid using vague or overly generic names like as they hinder understandability.
- Documentation: Inline comments and documentation are vital for maintainability. They explain the logic and expected use of functions, making it easier for others (or the original developer at a later time) to understand existing code.
- Refactoring Opportunities: Regularly revisiting and refactoring functions can prevent technical debt. It is essential to identify parts of the code that can be simplified or improved over time, reinforcing the need for maintainability in function design.
"Good function design principles are not just best practices; they are essential strategies that affect the long-term success of software projects."
By prioritizing function design principles like SRP and maintainability, software developers ensure that their projects remain efficient and easy to update. This diligence ultimately leads to better quality code and smoother development processes.
Debugging and Testing Functions
Debugging and testing functions is a crucial aspect of software development. Without proper testing methods, even the most well-structured functions can produce unexpected results. Bugs can range from simple typos to logic errors that seriously affecting application performance. Therefore, developing a strategy for debugging and testing can significantly enhance code quality and minimize errors.
One key benefit of debugging is its role in improving the reliability of code. When functions are thoroughly tested, developers can identify issues before the code is deployed. This proactive approach not only saves time in the long run but also boosts confidence in the overall system performance.
Considerations in debugging and testing functions include understanding the nature of the test cases. Different functions may require different testing strategies. Some functions may be designed to handle a variety of input, necessitating robust test coverage across various scenarios. By devising effective test cases, developers can ensure that functions perform as intended under diverse conditions.
Common Debugging Techniques
There are several techniques developers can employ to debug functions effectively. Here are some of the most common methods:
- Print Statements: The simplest way to inspect function behavior is by placing print statements throughout the code. These can help track variable values at different execution points.
- Using Debuggers: Modern IDEs come equipped with debugging tools that allow developers to step through code line-by-line. This reveals the state of the application at each point of execution.
- Log Files: Writing detailed log messages can provide insight into application behavior. Logs can display error messages, providing additional context that may help identify issues.
- Assertions: Implementing assertions can aid in validating assumptions made in the code. Assertions can automatically halt execution if a condition fails, directing attention to potential errors immediately.
By using these techniques, developers can quickly find and fix issues, which is essential in maintaining high-quality code.
Unit Testing Functions
Unit testing is a fundamental practice in software development. It focuses on testing individual components or functions in isolation. This method is vital for ensuring that a function behaves correctly according to its specifications. Here are some key aspects of unit testing:
- Isolation: Unit tests evaluate functions without interference from external factors, allowing a clear assessment of logic.
- Automating Tests: Tools like JUnit for Java, PyTest for Python, or Jest for JavaScript enable developers to automate testing. This saves time and makes it easier to run tests after each code change.
- Regression Testing: After fixing bugs, unit tests can help ensure that the changes do not impact other functionalities. It serves as a safety net for future code modifications.
"Effective unit testing provides not only confidence to the developer but also creates a safety mechanism for continuing the development process with assurance."
In summary, debugging and testing functions play a pivotal role in software development. They enhance the quality and reliability of code, making applications more robust. Utilizing effective debugging techniques and employing unit tests can greatly increase developer efficiency, allowing for the successful management of complex codebases.
Advanced Function Concepts
Advanced function concepts play a critical role in elevating coding practices and enhancing software design. They encapsulate sophisticated ideas that can significantly impact both functionality and performance. Specifically, these concepts, which include higher-order functions and recursion, promote code clarity, reusability, and efficiency.
Higher-order Functions
Higher-order functions are those that can take other functions as parameters or return them as results. This concept transforms the way developers engage with functions, leading to more abstract and versatile code. In many programming languages, such as JavaScript and Python, higher-order functions are used extensively. They facilitate functional programming paradigms, enabling developers to create complex operations by combining simpler ones.
The primary benefits of higher-order functions include:
- Improved Code Reusability: They allow developers to abstract behavior, reducing redundancy and fostering a modular approach. This makes it easy to reuse functions throughout the codebase.
- Enhanced Readability: By promoting composition, higher-order functions can produce code that is easier to read and maintain. Easier understanding of what the code does leads to increased productivity.
- Encouragement of Declarative Programming: Developers can write code that focuses on what to execute rather than how to execute it. This shift in perspective leads to cleaner and more efficient code.
For example, consider a simple higher-order function in JavaScript:
This function takes an array and a callback function as parameters. It applies the callback to each element in the array, demonstrating how higher-order functions can abstract and simplify operations.
Recursion in Functions
Recursion is another fundamental concept that involves a function calling itself to solve problems. This approach is particularly powerful for tasks that can be broken down into smaller, simpler subproblems. Recursive functions are important in many fields, particularly in algorithms and problem-solving scenarios.
The act of breaking down problems can lead to elegant and concise solutions, especially for problems like tree traversal, sorting, and mathematical computations. Notably, recursion also aligns well with functional programming principles, ensuring a degree of immutability and simplicity in code.
Here are key considerations for using recursion:
- Base Case Requirement: Every recursive function must have an exit point, known as a base case, to avoid infinite loops. This aspect is crucial in maintaining stability during program execution.
- Stack Overflow Risk: Recursive calls consume stack space. A profound reliance on recursion can lead to stack overflow errors for deep recursions. Developers must ensure that their recursive solutions are efficient and, where needed, consider alternative approaches.
- Clarity and Elegance: While recursion can sometimes be hard to grasp, it often results in cleaner, more readable code compared to iterative solutions.
An example of a recursive function can be shown below in Python, which calculates the factorial of a number:
In this case, the base case is when is 0. Such recursive functions illustrate not just a method of coding but a way of thinking about solutions.
In summary, advanced function concepts like higher-order functions and recursion stand at the intersection of elegance and efficiency in programming. Mastering these concepts allows developers to navigate complex programming challenges with clarity and skill.
Functions in Object-Oriented Programming
Functions play a crucial role in object-oriented programming (OOP). They are often known as methods when they are associated with a class. They enable the encapsulation of behavior within objects, which is a cornerstone of OOP design. By organizing code into functions, you can create a more manageable and scalable structure, which significantly enhances code readability.
In OOP, methods are not just about performing tasks. They also reflect the internal state of objects and can manipulate that state. This interaction between functions and the object's data is what allows for a rich, cohesive programming model. Moreover, it promotes reusability. By using methods, developers can avoid code duplication, leading to fewer bugs and easier maintenance.
Methods vs Functions
Though often used interchangeably, there is a distinction between methods and functions. A method is a function that is defined within a class and operates on instances of that class. This is an important concept in OOP because it encapsulates functionality along with the data, leading to better design principles.
When you define a function within a class, it includes not only the operations the function performs but also its relationship with the data stored in the object. For example, if you have a class called , a method might be defined to start the car which intertwines functionality with the car's specific data.
Examples of Methods:
- - starts ignition and initiates functionality
- - halts the operation and resets conditions
- - modifies the speed property of the car object.
Function Overloading
Function overloading is the ability to define multiple functions with the same name but different parameter lists. This concept is particularly useful in OOP as it allows for methods to operate on different types of data or different numbers of arguments. Overloading can lead to more intuitive interfaces since the method name can convey common functionality.
For example, consider a class that handles mathematical operations. You might have an overloaded method that can handle different types of inputs:
In this case, the method is flexible and can be used with integers and doubles, as well as with different numbers of parameters. The benefit of function overloading is increased clarity and flexibility in the code.
Key Point: Function overloading helps simplify code, enabling methods to be more versatile without the need for unique names for each variant.
Ultimately, understanding functions in the context of object-oriented programming adds depth to a developer's skill set. It enables structuring code that is not only functional but also elegant and easy to maintain.
Functions and Performance
Functions play a pivotal role in determining the overall performance of a software application. They encapsulate reusable segments of code, which can significantly reduce redundancy and error rates. Each time a function is invoked, the time it takes to execute directly impacts the performance of the application. Therefore, understanding the implications of function design and implementation is crucial for any software developer.
Performance Considerations
When designing functions, several performance aspects need to be evaluated:
- Execution Time: This is the time taken from the start to the completion of the function. Optimizing execution time can lead to faster solutions and more efficient applications.
- Memory Usage: Functions can consume memory resources. It is essential to manage memory efficiently, as excessive usage can lead to slower performance and crash issues.
- Function Call Overhead: Each time a function is called, overhead is involved, such as setting up stack frames and handling parameters. Minimizing unnecessary function calls can enhance performance.
To maintain high performance levels, developers must apply these considerations in tandem when designing their functions.
Optimizing Functions
Optimizing functions involves various strategies that focus on improving both execution time and resource usage. Here are several techniques:
- Algorithm Optimization: The choice of an algorithm directly influences a function's performance. Selecting the most efficient algorithm for the task can significantly reduce execution time and complexity.
- Minimize Side Effects: Functions should avoid altering states outside their scope. This not only leads to unpredictable results but can also degrade performance. Keeping functions pure can reduce debugging time.
- Lazy Evaluation: Deferring the computation of a value until it is necessary can enhance performance. This reduces unnecessary calculations and conserves memory during execution.
- Inlining Functions: For small, frequently called functions, consider using inline functions. This can remove function call overhead, as the compiler directly replaces the function call with the actual code.
- Profiling Tools: Utilize tools that help identify bottlenecks in allocation and execution. Resources like gprof or Python's cProfile can provide valuable insights into performance issues.
By implementing these techniques, developers not only improve their application’s performance but also enhance the user experience.
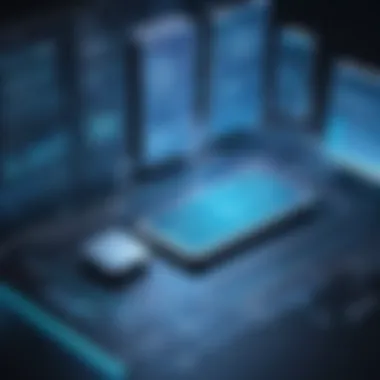
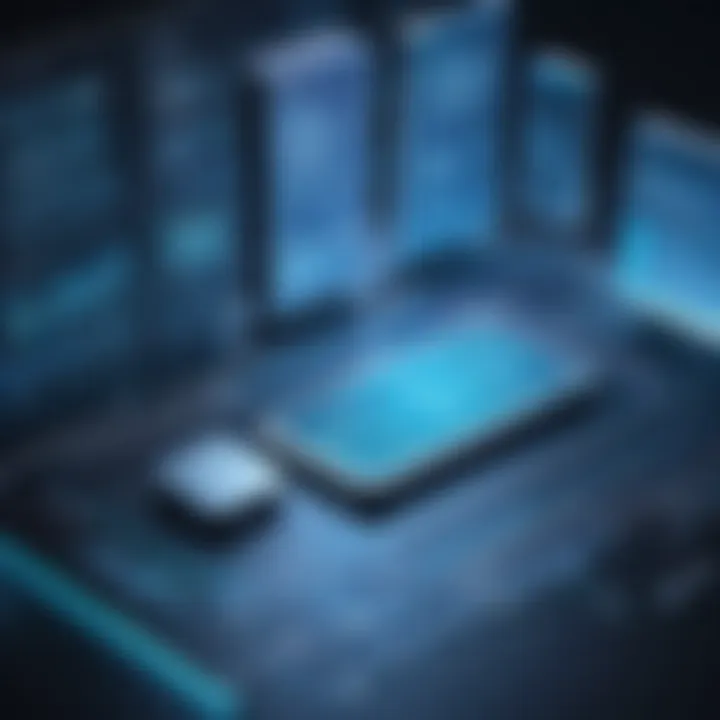
Optimizing functions is not just about speed; it's about resource management and efficiency.
In summary, functions are vital to performance considerations in coding. Careful design and optimization lead to efficient software that meets user expectations and performs consistently across various platforms.
Best Practices for Using Functions
Efficient function design is critical for any software project. Best practices for using functions enhance code readability, maintainability, and reusability. Following best practices not only reduces the likelihood of bugs but also improves the overall workflow for developers. When functions are designed properly, they become intuitive and effective tools in a programmer’s toolkit.
Naming Conventions
Naming conventions play a significant role in the clarity of code. A well-chosen function name should succinctly convey its purpose. This practice allows developers to understand functionality at a glance without needing to look at the implementation details.
Consider the following tips for effective naming:
- Be Descriptive: Use names that describe the behavior or action performed. For example, use instead of a vague term like .
- Use Consistent Style: Stick to a consistent naming style, such as camelCase or snake_case, depending on the programming language norms. This consistency helps create an intuitive flow in the codebase.
- Avoid Abbreviations: Using abbreviations can lead to confusion. Always prefer clarity over brevity.
- Use Action Words: Start function names with verbs. For example, clearly indicates that the function retrieves data.
Incorporating these practices can lead to a more understandable and maintainable code base.
Documenting Functions
Documentation is crucial in programming. Proper documentation of functions assists both the original author and any future maintainers of the code. Effective documentation provides information that is necessary to leverage the functions correctly, without requiring a deep dive into the source code.
Here are several aspects to consider when documenting functions:
- Parameter Descriptions: Clearly describe each parameter, including its type and purpose. This clarity can help avoid misuse of the function.
- Return Value: Always include information about what the function returns. This understanding is vital for anyone who calls the function as it dictates how outputs should be used.
- Use Comments: Inline comments can clarify complex code logic within the function body, helping future developers understand the thought process behind specific implementations.
- Examples of Usage: Providing code snippets showcasing how to use the function can be incredibly enlightening. This practice guides users in practical applications.
By implementing rigorous documentation standards, a codebase evolves into a valuable resource. This not only aids current team members but also future-proofs the project against new developers or other teams.
"Good documentation helps not only others but also your future self."
In summary, adhering to best practices when using functions is paramount in software development. Naming conventions and thorough documentation serve as foundational elements that promote understanding, enhance collaboration, and ultimately lead to more successful projects. With these best practices in mind, developers can ensure that their functions are not just effective, but also accessible to others in the coding community.
Real-world Applications of Functions
Functions play a crucial role in various real-world applications across numerous domains. Understanding how functions operate and their importance in coding helps developers create more efficient, scalable, and reusable software systems. In this section, we will focus on two primary areas where functions are applied: web development and data analysis.
Functions in Web Development
In web development, functions help streamline the development process and enhance the interactivity of applications. They encapsulate code segments, making it easier to manage and debug. Every time a user interacts with a web page, functions come into play to handle these events. For instance, a click event on a button can trigger a function that performs an action such as sending a request to a server or displaying a message on the screen. This promotes a tidy structure and a clear flow of logic in the codebase.
Benefits of Using Functions in Web Development:
- Code Organization: Functions allow developers to organize code more efficiently. This approach not only simplifies the task of locating code segments but also facilitates easier debugging and testing.
- Reusability: Often, similar actions in a web application require similar logic. By creating functions for these repetitive tasks, developers reduce code duplication and potential errors.
- Enhanced Readability: Functions denote a clear intention or action, enhancing the readability of the code. This clarity aids in future code maintenance and onboarding new developers to the project.
"Functions act as reusable building blocks in a web application, promoting cleaner and more maintainable code."
One example from web development is the use of functions in JavaScript frameworks like React or Vue.js. Here, functions serve as components that can maintain state, handle events, and render user interfaces based on data. Such modular design improves development speed and supports scalability.
Functions in Data Analysis
Data analysis is another field where functions are indispensable. Analysts typically deal with vast datasets, requiring efficient processing and manipulation. Functions enable analysts to encapsulate data operations, making it easier to apply the same transformations across different datasets without rewriting code.
Key Roles of Functions in Data Analysis:
- Data Cleaning: Functions assist in cleaning and transforming data by removing duplicates, filtering out unnecessary entries, and standardizing formats. This automation ensures that the datasets remain consistent and reliable for analysis.
- Statistical Operations: Functions are often utilized to perform statistical calculations. Whether it is computing averages, medians, or regressions, analysts can quickly apply these functions to derive insights from data.
- Visualization: Functions in libraries like Matplotlib or Seaborn in Python enable users to create visual representations of data efficiently. These visualizations help convey complex information in a more digestible format to stakeholders.
Functions empower data scientists to focus on their core tasks of interpretation and decision-making rather than getting bogged down by repetitive and manual coding processes. This efficiency ultimately leads to quicker insights, aiding organizations in making informed decisions.
In summary, functions are foundational in both web development and data analysis. They enhance code organization, promote reusability, and increase the efficiency of data processing. As programming continues to evolve, the significance of functions will only increase, making them an essential topic for anyone in the tech industry.
The Future of Functions in Programming
The landscape of programming continues to evolve at a rapid pace, and functions lie at the core of this transformation. They are not just tools for encapsulating code; they are evolving to meet the requirements of modern software development. As software systems grow in complexity, the efficient use of functions becomes pivotal for achieving scalability, maintainability, and adaptability. Evaluating the future of functions enables developers to leverage advancements effectively, ultimately enhancing productivity.
Emerging Trends
In the coming years, several trends will shape how functions are utilized in programming. Serverless Computing is gaining traction, where developers write functions to be executed in the cloud, eliminating the need to manage server infrastructure. Microservices Architecture also promotes the use of small, focused functions that communicate with one another, improving system organization and flexibility. Furthermore, the rise of Functional Programming emphasizes pure functions, immutability, and higher-order functions, representing a shift in how developers approach coding.
- Serverless Computing: Allows functions to run on-demand in a cloud environment, optimizing resource management and scaling.
- Microservices: Promotes small, independent functions that can be developed, deployed, and scaled separately.
- Functional Programming: Encourages writing functions that perform tasks without side effects, simplifying debugging and testing processes.
Each of these trends highlights the importance of functions in addressing contemporary challenges in software development.
Function-Based Paradigms
The future of programming embraces function-based paradigms as a way to facilitate more efficient and effective development practices. Emphasis is being placed on techniques that are centered around functions rather than classes or objects. This shift allows for cleaner, more intuitive code that reduces complexity.
- Declarative Programming: This paradigm focuses on what needs to be done rather than how it should be accomplished. Functions in this context describe computations succinctly.
- Reactive Programming: Functions in reactive programming respond to data changes or events, allowing for dynamic application behavior without manual intervention.
- Asynchronous Programming: Asynchronous functions enable non-blocking operations, enhancing performance in environments where latency is a concern.
By adopting function-based paradigms, developers can realize significant benefits, such as enhanced productivity, easier scalability, and an improved ability to manage the complexities of modern applications.
"The future of functions in programming is not just about efficiency; it’s about redefining how we solve problems through code."
As we look ahead, understanding these emerging trends and function-based paradigms is crucial for software developers, IT professionals, and tech enthusiasts. They will prepare us for seamless integration of functions into future software systems, ensuring that this vital construct continues to evolve alongside our needs.
Culmination
In summary, the conclusion of this article emphasizes the critical nature of functions within the realm of coding. Functions are not merely tools for coding; they serve as essential building blocks for any software development effort. When functions are implemented effectively, they enhance modularity, improve code readability, and contribute to the overall maintainability of the codebase. Moreover, the usage of functions promotes reusability, which can significantly minimize the time and effort developers expend in creating new software solutions.
Summary of Key Points
To encapsulate the major themes discussed, here are the core elements:
- Modularity: Functions allow developers to break down complex tasks into manageable pieces, facilitating easier troubleshooting and feature enhancement.
- Reusability: Functions can be called multiple times throughout the code, which reduces duplication and fosters a cleaner coding style.
- Scalability: As systems evolve, functions help maintain consistency, enabling seamless updates and adjustments.
Additionally, the precision in defining parameters and return values plays a significant role in function performance and usability. A well-defined function can interface clearly with other parts of a codebase, promoting both efficiency and efficiency.
Final Thoughts
The future of programming includes a continued emphasis on functions and their role in coding. As software systems become increasingly complex, there will be a greater reliance on function-based paradigms. Emerging trends point towards functional programming approaches gaining traction due to their robust handling of state and side effects. Consequently, understanding and mastering the use of functions will be paramount for any developer aiming to remain relevant in the tech landscape.
Further Reading and Resources
Understanding functions in coding is not a one-time task. It is an ongoing learning journey. Therefore, further reading and resources are crucial. These materials provide deeper insights and different perspectives on the application of functions across various real-world scenarios. Whether you are looking to solidify foundational concepts or explore advanced techniques, these resources can enhance your knowledge significantly.
Books on Programming Functions
Books dedicated to programming functions serve as excellent resources. They often cover the topic in depth, offering thorough explanations and practical examples.
Some notable titles include:
- "Clean Code: A Handbook of Agile Software Craftsmanship" by Robert C. Martin
- "JavaScript: The Good Parts" by Douglas Crockford
- "Python Cookbook" by David Beazley and Brian K. Jones"
- This book emphasizes the importance of writing clean and maintainable code, highlighting the role of functions in achieving those goals.
- It dives into the essential aspects of JavaScript functions and their significance in the language's structure.
- This resource provides practical recipes for using functions effectively in Python.
These books not only enhance theoretical knowledge but also give practical examples that can be applied in real development work. By studying such resources, software developers and other IT professionals can refine their coding practices.
Online Courses and Tutorials
Online courses and tutorials are another great way to learn about functions. They offer flexibility and accessibility, catering to diverse learning styles.
Some recommended platforms include:
- Coursera: Provides courses on programming functions across various languages tailored to different skill levels.
- edX: Offers a wide array of computer science courses, including extensive modules on functions in coding.
- Udemy: Features a multitude of practical tutorials focusing on specific programming languages and techniques involving functions.
These platforms often provide hands-on exercises, allowing learners to apply what they have studied in real-time scenarios. They create an interactive learning environment that is hard to replicate with just books alone.
Exploring further reading materials is essential for mastering functions in coding. It supports continuous growth and keeps one updated on evolving best practices.