Harnessing JavaScript for Advanced Form Functionality
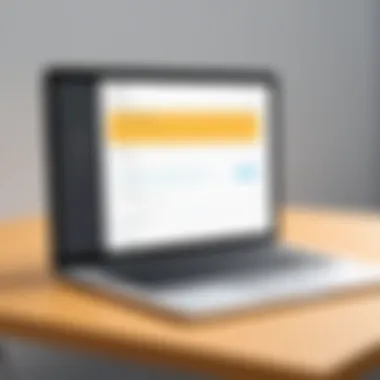
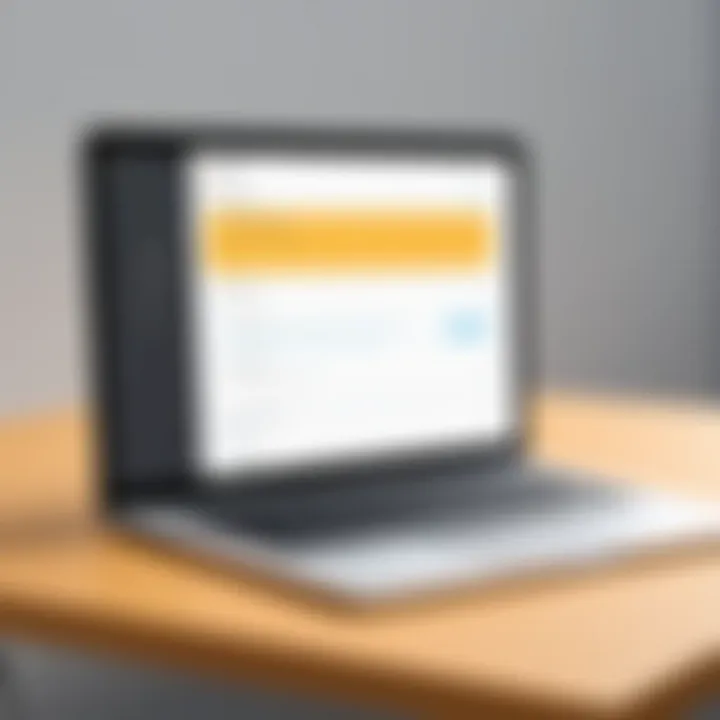
Intro
In the realm of web development, JavaScript plays an essential role, particularly in managing forms. Forms are often the primary means by which users interact with web applications. The user experience relies heavily on how efficient and responsive forms are. This article uncovers various techniques and methods to leverage JavaScript for much-improved form handling, validation, and submission.
This discussion will provide in-depth insights into the role of JavaScript for enhancing form functionality, addressing common challenges, and offering best practices for a successful implementation.
Overview of JavaScript in Web Forms
JavaScript is an interpreted programming language that enables developers to create dynamic and interactive applications. In web forms, its application is vast and multifaceted. Understanding the importance and core features of JavaScript within this context is essential.
Definition and Importance
JavaScript, developed in 1995, allows developers to elevate static HTML forms to dynamic interfaces. It is a client-side scripting language that runs on the user's browser, which provides quick interactions without making additional server requests. This direct execution is what makes it particularly important in modern web development.
Key Features and Functionalities
JavaScript offers several features that are particularly beneficial for form handling:
- Real-time Validation: Users receive instant feedback on their input, reducing errors upon submission.
- Asynchronous Communication: Using AJAX, forms can submit data in the background, enhancing user experience by keeping the page responsive.
- Dynamic User Interface Changes: JavaScript can manipulate HTML elements on the fly, thereby improving user interactions.
- Enhanced Security Measures: Through client-side validation, developers can reinforce security by preventing incorrect data entries before reaching the server.
Use Cases and Benefits
JavaScript's adaptability allows for various use cases, including:
- Login Forms: Where real-time validation for passwords is crucial.
- Registration Forms: Which can require extensive validation depending on user input.
- Feedback Forms: That benefit from dynamic updates based on user choices.
The benefits of using JavaScript in forms are significant. They result in:
- Improved user satisfaction and engagement due to faster interfaces.
- Reduction in server load by minimizing invalid submissions.
- Enhanced data collection through more accurate inputs.
Best Practices
To fully harness the power of JavaScript in forms, adhering to certain best practices is paramount.
Industry Best Practices for Implementation
Implementing JavaScript effectively involves:
- Utilizing Libraries: Frameworks such as jQuery simplify DOM manipulation and AJAX requests.
- Progressive Enhancement: Ensuring that forms are functional even with JavaScript disabled.
- Accessibility Considerations: Making forms accessible to all users, including those with disabilities.
Tips for Maximizing Efficiency
- Keep Code Organized: Maintain clear and concise code for ease of understanding and debugging.
- Modularize Functions: Create small, reusable functions rather than large blocks of code to boost maintainability.
- Throttle or Debounce Events: This technique helps in optimizing I/O operations and improving performance during user input.
Common Pitfalls to Avoid
- Neglecting Server-side Validation: Client-side validation is useful, but server-side checks are necessary for security.
- Too Much JavaScript: Overcomplicating forms with excessive JavaScript can lead to confusion for users.
- Ignoring Cross-Browser Compatibility: Test forms across different browsers to ensure consistent behavior.
Ending
By incorporating the outlined strategies and principles, developers can effectively utilize JavaScript to transform web forms into powerful tools for user interaction. Optimizing form handling and validation processes not only enhances user experience but also encourages more secure and efficient data collection.
Prolusion to JavaScript Forms
JavaScript is an essential tool in modern web development. Understanding how to utilize JavaScript effectively in forms is crucial. Forms serve as the primary interface for user interaction with web applications. They collect data, perform validations, and trigger submissions. As such, JavaScript enhances their functionality and improves user experience significantly.
In this section, we will explore the historical context of JavaScript in forms and how its evolution reflects the changing landscape of web development. We will also discuss the importance of forms, outlining why they are vital components of any web application.
Historical Context and Evolution
When JavaScript was first introduced in 1995, web forms mainly served basic functions. They were primarily used to collect user input and required manual submission via a page refresh. Over time, as web applications became more interactive, JavaScript evolved. It began to offer client-side scripting capabilities, allowing developers to enhance forms with dynamic behaviors.
In the early 2000s, technologies like AJAX emerged. This allowed forms to send data to the server without refreshing the whole page. The user experience improved, as instant feedback became possible. Gradually, JavaScript libraries like jQuery simplified form manipulation, enabling developers to create more responsive and user-friendly forms with less effort. This evolution has made forms an essential aspect of user interaction on the web today.
Importance of Forms in Web Development
Forms play a crucial role in web applications. They facilitate essential tasks such as:
- Data Collection: Forms enable users to submit feedback, register accounts, or provide necessary information.
- User Interaction: They are a primary means through which users communicate with the application.
- Validation: JavaScript enhances forms by allowing real-time validation, ensuring that user input meets the required standards before submission.
- Integration: Forms often integrate with back-end services, enabling functionalities like bookings or purchases.
Understanding the Basics of Form Elements
Understanding the basics of form elements is essential for building intuitive and user-friendly web applications. Forms serve as a critical interface for user input, allowing for data collection, user feedback, and various interactive functionalities. Grasping the different form elements and their intended uses paves the way for more efficient development and enhanced user interactions. It also helps in making informed choices regarding user experience and accessibility.
Input Types and Their Uses
HTML5 introduced several input types that allow developers to collect data in formats aligned with user expectations. Each type offers unique characteristics tailored to specific data. Here are several common input types and their particular uses:
- Text: The most basic type, suitable for single-line text entries. It’s versatile but should be used with caution regarding validation.
- Email: Automatically checks the format of email addresses. This provides users with instant feedback, enhancing data accuracy.
- URL: Ensures that input adheres to web link formats. Useful for requiring a valid web address.
- Number: Designed specifically for numerical data, enabling easy increment and decrements.
- Range: Allows users to select a value from a specified range. This is effective for ratings or quantity selections.
- Date and Time: Designed for selecting dates and times, these inputs often provide a calendar or time picker interface.
Utilizing the right input type is crucial for maximizing the data quality and overall user experience.
Using appropriate input types reduces the chance of errors and streamlines the data collection process. In addition, this approach can contribute positively to the website’s accessibility. For instance, screen readers can better interpret form fields when the correct input types are used, making your application more usable for all.
Choosing Appropriate Form Controls
Selecting suitable form controls is equally important in achieving functionality that aligns with user needs. Using the correct form controls not only enhances usability but also simplifies the interaction flow within your application. Here’s a breakdown of the key considerations:
- Buttons: Use buttons for clear submission actions. Consider options like submit, button, and reset based on the intended operation.
- Checkboxes: Ideal for options that allow multiple selections. Users can see all available choices and make selections accordingly.
- Radio buttons: Useful for mutually exclusive options, ensuring that users can select only one option in a category.
- Select boxes: Providing a dropdown list can save space and present a clean interface, especially when dealing with numerous choices.
When deciding on form controls, consider the goal of the form and the data you wish to collect. For instance, if you want user feedback on a product, you might choose a combination of checkboxes and radio buttons to allow diverse, yet targeted choices. Overall, thoughtful selection of form controls greatly enhances user experience and ensures that responses are precise and actionable.
Client-Side Form Validation
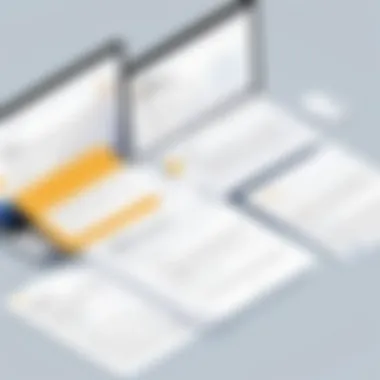
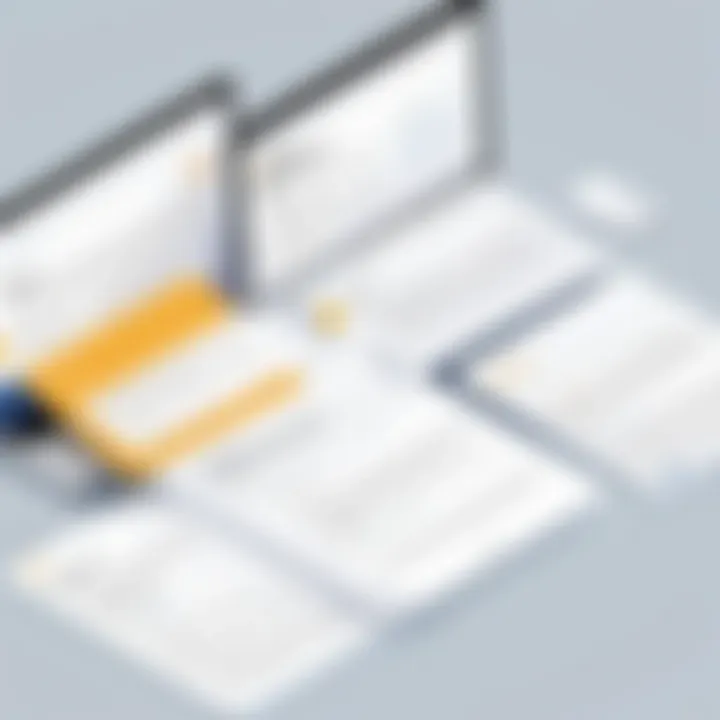
Client-side form validation has emerged as a critical aspect of web development. It provides developers with tools to verify user inputs before data submission. The importance of this practice is multi-faceted, impacting both functionality and overall user experience.
Importance of Validation and User Experience
Validation helps ensure that the data submitted through forms meets certain criteria. This not only reduces errors but significantly enhances user experience. When users receive immediate feedback on their inputs, they are less likely to encounter frustrations during submission.
By catching errors early, client-side validation saves time for users. For example, if a user forgets to enter an email address, real-time validation can alert them before they submit the form. This responsiveness leads to a smoother interaction with the form and ultimately fosters user trust and satisfaction.
Moreover, validation can handle different scenarios such as checking for valid email formats, creating strong passwords, or ensuring required fields are filled. Consequently, these mechanisms lead to cleaner data being sent to servers, which facilitates effective data processing.
Implementing Real-Time Validation with JavaScript
JavaScript provides robust options for implementing real-time validation in forms. This dynamic capability can enhance user interaction significantly. By utilizing event listeners, developers can validate inputs as users type.
Here is a simple example to demonstrate basic real-time validation for an email input:
This code snippet checks the validity of an email format during input. If the input does not match the regular expression, an error message is displayed instantly.
Managing Form Submissions
Managing form submissions is a crucial aspect of web development. It ensures that data entered by users is efficiently processed and handled. A well-structured form submission process can significantly improve user experience and increase the reliability of data collection. As JavaScript enables developers to enhance these processes, understanding its role in managing submissions becomes vital.
In a digital landscape where every interaction counts, it becomes essential to minimize user effort and maximize satisfaction. This section covers two primary methods of form submission: synchronous and asynchronous. Each method has its own merits, and the choice between them can affect how users interact with forms.
Synchronous vs. Asynchronous Submission
Synchronous submission is a traditional approach. When a user submits a form, the entire page refreshes to process the input data. This can sometimes lead to a less engaging user experience as it interrupts the flow. Users must wait for new data or feedback, which can be frustrating, especially on slow networks. However, it ensures that the server receives the complete set of data before any further interaction occurs.
On the other hand, asynchronous submission allows the page to stay static while the data is processed in the background. This is commonly done using JavaScript's AJAX capabilities. The advantage is clear: users receive instant feedback without interruption. For example, using or API, developers can send form data to the server and receive responses seamlessly. This method not only enhances the user experience but also allows for operations like dynamic validation or real-time updates.
Key Considerations
- User Experience: Asynchronous submission offers smoother interactions.
- Data Handling: Ensure proper validation before sending data.
- Network Dependencies: Network reliability can affect performance.
Handling AJAX Requests for Submissions
AJAX stands for Asynchronous JavaScript and XML. It is a powerful tool for submitting forms without page reloads. Handling AJAX requests involves several steps. Developers need to gather user input and send it to the server, managing responses to update the form dynamically.
To implement AJAX successfully, consider the following steps:
- Setup the Form: Use an event listener to trigger the AJAX call upon submission.
- Prevent Default Action: This stops the browser from its usual submission process.
- Create XMLHttpRequest or use the API to send data.
- Handle Server Response: Insert feedback into the form for user visibility.
Here’s a simple example using the API:
By implementing AJAX for form submissions, developers can enrich the user experience through responsive interactions. It also gives flexibility for additional enhancements, such as updating other elements based on user input in real-time.
"Utilizing AJAX enables real-time user experiences that engage users effectively and keep them informed instantaneously."
Enhancing User Interaction with JavaScript
Improving user interaction with JavaScript is vital in modern web development. Forms serve as a primary interface between users and applications. Effective enhancement of user interaction involves techniques that make forms intuitive and responsive. Dynamic form behaviors significantly reduce user frustration by allowing them to navigate more seamlessly through the input process. This also enhances overall user experience, increasing the likelihood of conversion. When looking at form design, consider ease of use, efficiency, and the ability to handle complex interactions with minimal input from users.
Dynamic Form Fields
Dynamic form fields allow the modification of the form structure based on user inputs or actions. This capability transforms static forms into interactive experiences. For instance, if a user selects a specific option from a dropdown menu, additional fields relevant to that selection can appear instantly. This not only reduces clutter by showing only necessary inputs but also guides users through complex forms without overwhelming them.
Implementing dynamic fields requires careful planning. Here are key points to consider:
- User Context: Understand the needs of your users to better anticipate what fields are necessary at any moment.
- Field Dependency: Leverage JavaScript’s ability to listen for events such as change or focus. This helps trigger the appearance or validation of other fields based on previous inputs.
- Smooth Transitions: Use CSS animations alongside JavaScript to make the appearance and disappearance of fields feel natural.
This approach not only helps in collecting precise data but also ensures users do not feel lost or confused. When done effectively, dynamic fields significantly improve the efficiency of form completion.
Progressive Enhancement Strategies
Progressive enhancement focuses on providing a baseline experience for all users while allowing enhancements for those with more capable browsers. This strategy ensures accessibility for users regardless of device or browser limitations. Utilizing JavaScript to add features to forms while ensuring they remain functional without it is essential.
Key elements to implement progressive enhancement in forms include:
- Basic HTML Forms: Always start with a functional HTML form. This provides the fundamental structure which is accessible to all users.
- JavaScript Enhancements: Gradually add scripts that enhance interaction, like validation or enabling/disabling fields.
- Graceful Degradation: If JavaScript fails or is disabled, the form should still allow users to interact and submit data.
When implementing these strategies, thorough testing is important. Ensuring that forms work smoothly across different platforms can prevent frustration and maintain user trust.
"Accessibility is not a feature, it is a fundamental requirement for a good user experience."
Security Considerations
In today's digital landscape, the security of web forms remains a paramount concern for developers. Forms are frequent targets for malicious activities. Effective security measures can prevent unauthorized access and data breaches. It is crucial to understand various vulnerabilities that might exist within forms and to implement best practices accordingly. With a well-structured approach, developers can enhance user trust and protect sensitive information.
Common Vulnerabilities in Form Handling
- Cross-Site Scripting (XSS): XSS occurs when an attacker injects harmful scripts into web pages viewed by users. This can happen if inputs are not properly sanitized. Proper validation of user input is necessary to mitigate this risk.
- SQL Injection: Through malicious input, attackers can manipulate databases and gain unauthorized access to sensitive data. This is often due to improper handling of user inputs in SQL queries. Using prepared statements can help prevent SQL injection attacks.
- Cross-Site Request Forgery (CSRF): This attack tricks a user into submitting a request that they did not intend to make. It can be prevented by using anti-CSRF tokens, ensuring that any actions taken by users are verified.
- Insecure Data Transmission: If sensitive data, such as passwords or payment information, is sent over unencrypted channels, it can be intercepted by attackers. Always implement HTTPS to protect data during transmission.
The presence of these vulnerabilities highlights the importance of security in form handling. By recognizing and addressing them, developers can build more resilient applications.
Implementing Security Best Practices
To ensure the efficiency and security of forms, developers should adopt the following best practices:
- Input Validation: Always validate inputs on both client and server sides. Ensure that all data conforms to expected formats before processing.
- Use HTTPS: Encrypt data in transit using HTTPS. This protects the information exchanged between users and the web application.
- Implement CSRF Tokens: Use anti-CSRF tokens for state-changing requests. This adds an extra layer of verification, reducing the risk of CSRF attacks.
- Sanitize Outputs: When displaying user inputs, ensure that the outputs are properly sanitized to prevent XSS attacks.
- Error Handling: Avoid disclosing sensitive information in error messages. Provide generic responses that do not reveal internal workings or data structures.
- Regular Security Audits: Conduct routine audits and testing to identify and rectify potential vulnerabilities. Automated tools can also aid in this process.
By integrating these practices, developers enhance the overall security posture of their applications. This proactive approach not only protects users but also maintains the integrity of the application.
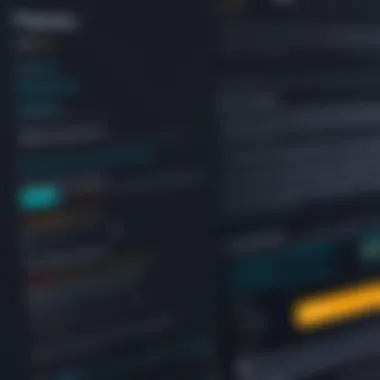
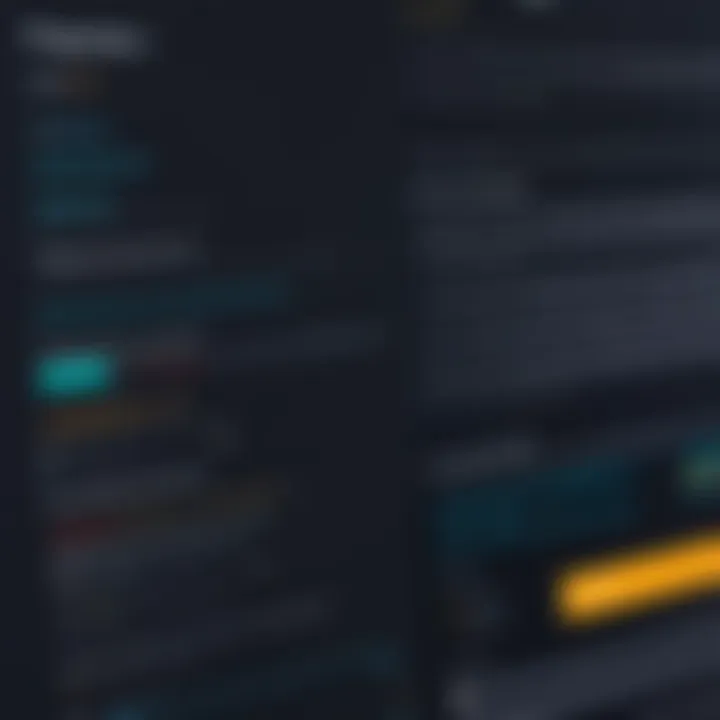
Debugging JavaScript in Forms
Debugging is an essential skill for any developer, especially when dealing with forms in JavaScript. Forms are often the interface for user interaction on a web application, and any malfunction can lead to a poor user experience. Effectively debugging JavaScript can save both time and frustration, ensuring forms work as intended. Addressing common errors and employing troubleshooting techniques can enhance the reliability and performance of web forms.
Skilled debugging not only resolves immediate issues but also enriches overall code quality. With each bug fixed, developers gain deeper insights into both their code and JavaScript itself, leading to fewer errors in the future. Importantly, debugging tools are invaluable for identifying and addressing these issues efficiently.
Common Errors and Troubleshooting Techniques
Debugging JavaScript in forms typically involves addressing common errors that developers encounter. Here are a few prevalent issues:
- Validation Failures: One of the most frequent problems arises when form validation fails. This can result from mismatched data types or incorrect input formats. Ensuring that the validation logic is accurate is crucial.
- Event Handling Misconfiguration: Misunderstanding how events work can cause significant troubles. For instance, not binding events correctly can prevent the JavaScript code from executing altogether.
- AJAX Errors: When working with asynchronous requests, errors can occur from the server side or network issues. Identifying the source of these errors is key to resolving them.
To address these issues, developers can apply specific troubleshooting techniques:
- Console Logging: Using statements helps trace the flow of data and identify where things go wrong. It allows examination of variable values in different stages of the process.
- Browser Developer Tools: These tools help inspect elements, monitor network requests, and debug the JavaScript code line by line. This functionality is crucial for pinpointing errors directly in the code.
- Validation of Inputs: Check input values before submission. This can help catch incorrect entries before they reach the server, thus improving user experience.
Utilizing Browser Developer Tools Effectively
Browser Developer Tools are a key asset for debugging JavaScript in forms. They provide a powerful suite of features that enable developers to troubleshoot issues in real time. Here are some effective usages:
- Inspect Element: This function allows observing the HTML structure and associated styles. By right-clicking on web elements, developers can access the source code, analyze attributes, and identify errors more easily.
- Console Panel: The console is a great space for executing JavaScript commands, observing console output, and checking for error messages. It can also run snippets of code to test certain functionalities without altering the original codebase.
- Network Panel: When forms utilize AJAX requests, the Network panel enables developers to monitor all outgoing and incoming requests. This view can highlight if requests are failing, allowing quick identification of the problem.
Using these tools strategically can substantially reduce the debugging time. Mastery of browser developer tools is not only beneficial for JavaScript developers but is also a fundamental skill in today’s web development landscape.
"A well-debugged application is not just about solving problems; it’s about understanding the underlying processes and improving them."
Overall, debugging is a vital component of web development, especially in forms with JavaScript. By recognizing common errors and leveraging developer tools effectively, developers can enhance the performance and reliability of their forms.
Integrating JavaScript Libraries and Frameworks
Integrating JavaScript libraries and frameworks is vital for developing robust web forms. These tools streamline coding, enhance functionality, and improve user experience. Libraries such as jQuery or React, provide pre-written code that simplifies complex tasks. This aspect of integration must be considered carefully, as choosing the right library can shape the performance and maintainability of your forms.
When you leverage libraries, you gain significant advantages. They often come with built-in methods for handling events, making AJAX requests, and managing DOM manipulation. This feature allows developers to focus on functionality rather than reinventing the wheel. Furthermore, libraries are typically well-documented, offering rich resources that can expedite the development cycle.
However, there are also considerations to account for. Each library comes with its own size and dependencies, which can slow down performance if not managed correctly. Keeping libraries updated is another factor to consider to avoid security vulnerabilities. The decision to integrate should weigh these aspects against the benefits achieved.
Leveraging Libraries for Enhanced Functionality
The ability to enhance form functionality through libraries cannot be overstated. By utilizing popular libraries, developers can implement advanced features with less effort. For instance, jQuery offers methods such as .validate() for form validation, streamlining this critical aspect of user input.
Moreover, form dynamic features improve user experiences. This includes auto-complete suggestions, pre-fill options, and real-time validation. Libraries enable these capabilities to be added quickly, often only requiring a few lines of code. As a result, users face fewer frustrations, ultimately leading to higher completion rates during submissions.
Additionally, libraries allow for better cross-browser compatibility. They abstract many nuances of browser behavior, ensuring that form functionalities remain consistent across different platforms. This quality is essential for maintaining a professional appearance and usability.
Popular Frameworks and Their Use Cases
Frameworks such as Angular, Vue.js, and React offer distinct advantages for building applications centered around forms. Each framework supports component-based architecture, allowing for reusable form components. This approach is highly effective in larger applications where forms can become complex.
- React: Known for its virtual DOM, React enhances performance and provides quick updates to the UI. Form validation and state management become simpler when using libraries like Formik or React Hook Form.
- Angular: This framework comes with integrated features for two-way data binding and reactive forms. These capabilities are ideal for applications where real-time data processing is required, such as chat applications or dynamic surveys.
- Vue.js: It balances complexity and simplicity, making it suitable for smaller projects or those new to full-fledged frameworks. Vue’s integration with existing applications is also seamless, which is beneficial for gradually enhancing an older codebase.
Optimizing Performance in Form Handling
The performance of forms is critical in web development. Forms are often the primary interface for user interaction on websites. When users experience delays during form submissions or validations, frustration rises quickly. Therefore, optimizing the performance of forms can significantly enhance user satisfaction and overall site efficiency. This section discusses specific strategies to minimize latency and establish best practices that help in efficient coding.
Minimizing Latency in Form Operations
Latency issues can occur due to various factors, including network delays, server response times, and client-side processing. Reducing latency not only leads to better user experience but also increases the likelihood of successful form submissions. Here are important strategies to consider:
- Asynchronous Processing: Utilizing AJAX techniques enables smooth, asynchronous submissions. This way, users can continue to interact with the page while the data is being processed on the server-side.
- Debouncing Input Events: By implementing debounce techniques, you can limit the number of requests sent to the server as users type. This reduces the load on both client and server. For instance, a delay of just 300 milliseconds can prevent excessive calls while still ensuring responsiveness.
- Optimized Payloads: Sending only necessary data reduces the size of requests. This can speed up the transmission of data, thus improving overall form responsiveness.
While latency might seem like a minor issue at first glance, its impact on user engagement cannot be understated. Users often abandon forms that seem sluggish or unresponsive, making it vital to focus on minimizing lag in form operations.
Best Practices for Efficient Code
Efficient code contributes significantly to both the maintainability and performance of web forms. Following certain best practices can lead to cleaner, faster, and more reliable JavaScript code. Here are some recommendations:
- Clean and Modular Code: Write modular functions to handle specific tasks. This makes debugging easier and promotes code reuse.
- Optimize Loops: When processing large datasets from forms, avoid nested loops where possible, as they can add significant overhead. Use methods like or as more performant approaches.
- Minimize DOM Manipulation: Accessing and manipulating the Document Object Model (DOM) is costly in terms of performance. Batch DOM updates to minimize reflows and repaints. For example, using can be beneficial.
- Use Event Delegation: Instead of attaching event listeners to each form element, attach a single listener to a parent element. This will optimize memory usage and improve performance when elements are added or removed dynamically.
Implementing these best practices can lead to significant optimizations, enabling faster code execution and a more enjoyable experience for users filling out forms.
Overall, optimizing performance in form handling should not be an afterthought. It requires a strategic approach from the outset to ensure users are met with efficiency, leading to successful engagements with your web applications.
Exploring Advanced Form Features
Advanced form features significantly enhance user experience and improve data handling in web applications. Utilizing JavaScript for these features allows developers to create more interactive, user-friendly forms that can adapt based on user input. This capability not only streamlines data collection but also enhances the overall aesthetic and functionality of web forms. Integrating advanced form features ultimately leads to higher conversion rates, making them an essential element in modern web development.
Custom Validations Using JavaScript
Custom validation is a crucial aspect of form development. It ensures that the data users input meets specific requirements before submission. The importance of using JavaScript for custom validations cannot be overstated. It allows developers to enforce their own validation rules dynamically, offering a tailored experience that standard HTML validation may not provide.
Benefits of Custom Validations:
- They provide instant feedback to users, facilitating smoother interactions.
- Developers can define complex rules that can handle specific business needs, such as matching passwords or validating email formats against proprietary criteria.
- Custom validations can reduce server load by ensuring only valid data is sent, thus minimizing the risk of backend errors.
To implement custom validation, developers can utilize the method along with event listeners to respond to user input changes. Here’s a simple example:
This code exemplifies how to provide instant feedback when the user inputs data. Such functionality is vital for enhancing user satisfaction and ensuring the reliability of submitted data.
Building Multi-Step Forms
Multi-step forms simplify the data entry process by breaking it into manageable sections. They guide users through a series of steps, which can help prevent overwhelming visitors with too many fields at once. This approach can dramatically improve completion rates for forms that require substantial input.
Key Considerations for Multi-Step Forms:
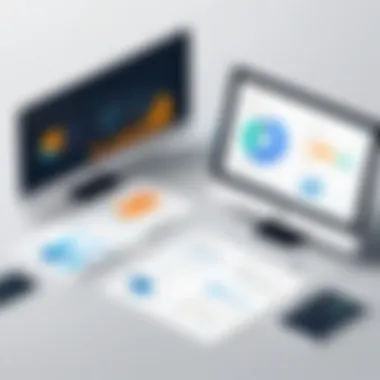
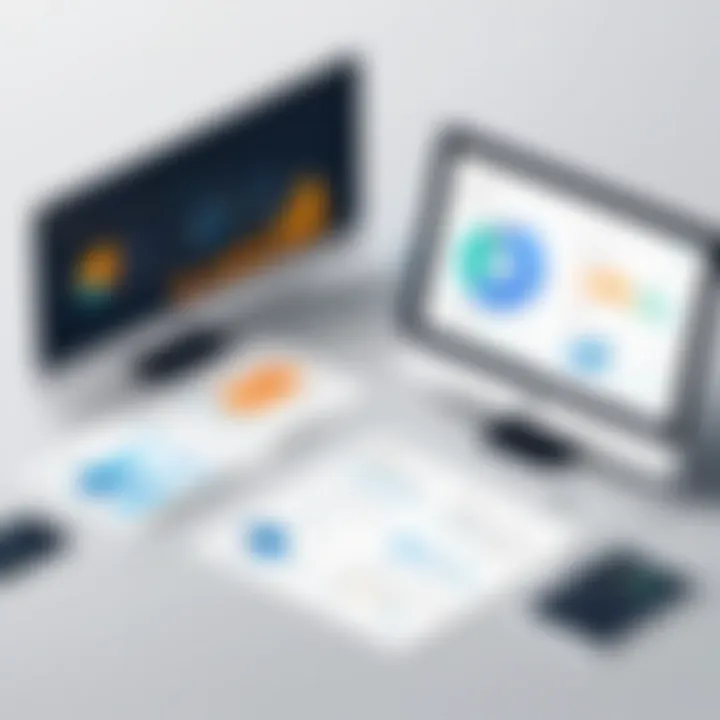
- Progress Indicators: They inform users how far they are in the process, enhancing user engagement and reducing drop-off rates. JavaScript can dynamically update these indicators as each step is completed.
- Validation and Data Persistence: It is vital to validate inputs at each step and maintain data between transitions. Developers should consider using the local storage or session storage API to temporarily hold data, ensuring users do not lose their inputs.
- Responsive Design: It is crucial for multi-step forms to be optimized for different devices. JavaScript enables adjustments to the visual layout or flow based on screen size, which is essential for mobile users.
For a multi-step form implementation, the following approach can be adopted:
This snippet demonstrates basic logic for transitioning between steps. The user experience is thus enhanced by providing a layered understanding of the form's requirements, guiding them more effectively through the process.
"Advanced features like custom validation and multi-step forms are essential for modern web applications. They not only enhance usability but also significantly improve data reliability."
In summary, exploring advanced form features through JavaScript contributes to enhanced functionality and user satisfaction. By implementing custom validations and multi-step forms, developers can create a more engaging and efficient data collection process.
Accessibility and Form Design
Accessibility in form design is crucial for creating web applications that can be utilized by all users, regardless of their abilities or disabilities. This topic focuses on the necessity of designing forms that comply with accessibility standards. When forms are accessible, they become easier to navigate and use for everyone, particularly people with visual, auditory, or motor disabilities. In addition, accessible forms can enhance the overall user experience and satisfaction, thereby increasing the likelihood of successful form submissions.
Legal considerations also play a role in the importance of accessibility in form design. Many jurisdictions have laws that require digital content to be accessible to individuals with disabilities. By prioritizing accessibility, developers not only comply with regulations but also demonstrate a commitment to inclusivity.
Principles of Accessible Forms
When creating accessible forms, several key principles should be followed:
- Use clear labels: Every form element should have a corresponding label that describes its function. This helps screen readers and users to understand the purpose of each field.
- Error identification: Users should receive guidance on how to correct errors in their input. Clear error messages can help improve users' confidence while submitting forms.
- Logical tab order: The tab order of the form should follow the sequence in which fields appear visually. This helps users navigate through forms efficiently using a keyboard.
- Visual contrast: Ensure sufficient contrast between text and background colors to facilitate reading.
- Flexible layouts: Design forms that can adapt to users' devices and screen sizes, providing a seamless experience on all platforms.
Implementing these principles results in forms that are user-friendly for everyone. They also minimize frustrations, leading to higher completion rates and overall satisfaction.
Using ARIA Roles with JavaScript
ARIA (Accessible Rich Internet Applications) roles enhance the accessibility of dynamic forms. They allow developers to provide additional context to web applications, making them easier to understand for users with disabilities. JavaScript can be employed to manage ARIA roles effectively, ensuring that dynamically created or modified content remains accessible.
For example, using can notify users when form validation errors occur. This alert informs them of the issue immediately without requiring them to search through the form for the problem. Additionally, attributes such as and can help indicate the status of form inputs.
Here's an example snippet that demonstrates how to use ARIA roles in a form:
By adhering to these best practices and utilizing ARIA roles effectively, developers can create forms that not only meet compliance requirements but also fulfill the usability needs of a broader audience. This is a fundamental step towards fostering an inclusive digital society.
Future Trends in JavaScript and Forms
The landscape of web development is constantly shifting, with JavaScript at its helm, particularly in the realm of forms. Understanding future trends in this area is crucial for developers who want to remain competitive and effective. As technology evolves, new methodologies and tools emerge, all of which can significantly enhance how forms function and interact with users. This section delves into emerging technologies impacting form design and functionality, along with predictions for future developments in this domain.
Emerging Technologies and Their Impact
Several cutting-edge technologies are shaping how JavaScript is utilized in forms. Notably, the rise of JavaScript frameworks such as React and Vue.js has revolutionized the way developers architect form components. These frameworks promote the use of reusable components, making it easier to create and manage complex form structures.
Another major technological evolution is the use of artificial intelligence in form validation and user interaction. AI can analyze user behavior in real-time, predicting potential errors during form completion. This capability can lead to more intuitive user experiences, reducing the friction often encountered in traditional form workflows.
Furthermore, cloud services have become more integrated into form handling. Developers can now leverage external APIs to validate data and manage submissions, enhancing performance and security. This trend allows forms to utilize robust backend services without the need for extensive local server management.
- Framework adoption ensures structure and consistency.
- AI technology offers predictive validation and error prevention.
- Cloud integration streamlines operations and enhances security.
Predictions for the Next Development Cycle
As we look ahead, several predictions can be made regarding the progression of JavaScript in forms. One significant forecast is the increase in the adoption of Progressive Web Apps (PWAs). PWAs combine the best of web and mobile apps, allowing forms to operate seamlessly across devices. Enhanced offline capabilities and faster load times are expected to elevate user engagement.
Security will also continue to be a top concern. Future iterations of JavaScript will likely incorporate more built-in security features for form submissions. This is essential given the rising number of data breaches and privacy concerns. Developers should prepare for stricter data handling protocols and better user consent management systems.
"The integration of AI and cloud technologies marks a pivotal shift in form management, promising smarter and more efficient solutions."
Moreover, the shift towards decentralized applications (dApps) might also influence how forms are handled. As blockchain technology gains traction, forms may evolve to integrate cryptocurrency transactions, necessitating new validation techniques and user interfaces.
In summary, monitoring these trends will better equip developers to optimize their form practices. Being proactive about adopting new technologies ensures that forms stay relevant and meet user expectations in a rapidly changing digital landscape.
Case Studies: Real-World Applications
In this section, the focus will be on case studies that illustrate the practical applications of JavaScript in forms. Case studies are invaluable in demonstrating real-world effectiveness and the varied uses of JavaScript concerning form handling. They provide insights into how different organizations have adopted these technologies to improve user engagement and overall functionality.
The significance of this discussion lies in the ability to learn from concrete examples. Through case studies, developers can see what strategies worked, what challenges appeared, and how various solutions can be tailored for specific needs.
Successful Implementations of JavaScript Forms
Many companies have recognized the potential of JavaScript in enhancing form interactions. Successful implementations showcase examples where JavaScript provides real-time validation, improves user experience via dynamic fields, and streamlines data submission.
- Lending Club: This peer-to-peer lending platform has integrated JavaScript to facilitate a smooth loan application process. The instant feedback on input errors minimizes user frustration and enhances completion rates.
- Airbnb: The highly popular lodging platform employs JavaScript to handle listings and booking forms. Their non-linear multi-step forms engage users without overwhelming them, improving overall submission rates while maintaining clarity and simplicity.
These implementations highlight how JavaScript serves as a critical tool in optimizing forms for user satisfaction. The adaptability and efficiency gained through these case studies can serve as a reference for developers aiming to implement similar strategies in their applications.
Lessons Learned from Industry Leaders
Reviewing these successful cases offers numerous lessons applicable to other developers and projects. Some of the key takeaways include:
- User-Centric Design: Focusing on the user experience is essential. Form structures should minimize confusion while facilitating easy navigation.
- Real-Time Feedback: Implementing real-time validation helps users correct mistakes on the spot. This approach vastly reduces frustration and increases the likelihood of form completion.
- Performance: Ensuring forms are responsive and load quickly. Slow loading times can lead to increased abandonment rates.
"Understanding the needs of users and adapting forms accordingly leads to greater satisfaction and use of applications."
Incorporating JavaScript effectively into forms can undoubtedly yield improved engagement metrics. Lessons from industry leaders can help shape approaches for developers, guiding them to create more efficient and user-friendly form interfaces.
Closure
Recap of Key Points
In this article, we have discussed several critical aspects pertaining to JavaScript's role in forms:
- Client-side Validation: Emphasized the necessity of validating user input before submission.
- Dynamic User Interactions: Showcased how JavaScript enables the enhancement of forms through dynamic fields that adapt in real-time to user actions.
- Asynchronous Data Handling: Highlighted the importance of AJAX in ensuring smooth form submissions without page reloads, promoting better user experience.
- Security Practices: Discussed various methods for safeguarding form data from common vulnerabilities such as cross-site scripting and SQL injection.
This recap crystallizes the core message that employing JavaScript in forms leads to improved functionality and user engagement.
Final Thoughts on JavaScript for Forms
As web technologies continue to evolve, the integration of JavaScript in form handling remains essential. The ability to create responsive, efficient forms directly affects user satisfaction, ultimately influencing website success. Developers must stay informed of best practices and emerging trends, adapting their strategies to meet user expectations and security standards. JavaScript is not merely a tool but a cornerstone in modern web development, particularly in the context of forms. Every form interaction, whether for data entry or authentication, greatly benefits from the enhancements JavaScript provides, making it an invaluable skill for professionals in the field.