A Comprehensive Guide to Virtualenv for Python Development
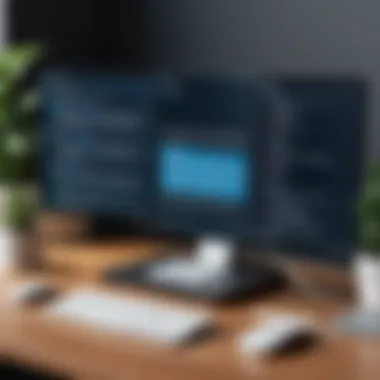
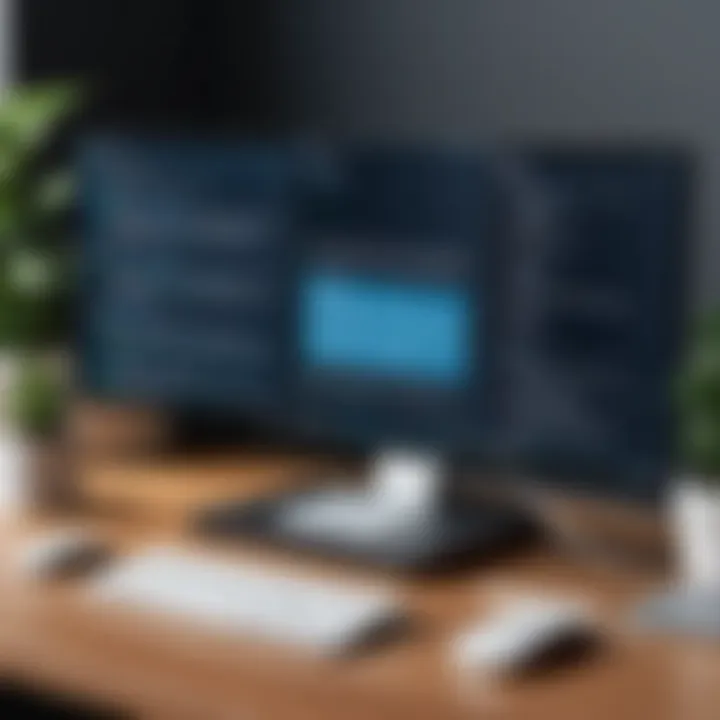
Intro
Virtualenv plays a pivotal role in Python development by allowing developers to create isolated environments for their projects. This isolation is crucial for maintaining cleanliness and organization within projects that may require different dependencies. As software evolves, so do the tools that facilitate development, and virtualenv stands out for its simplicity and effectiveness. Its utility goes beyond just managing dependencies; it offers a framework for efficient project management in an increasingly complex software landscape. This section will explore how virtualenv fits into the larger picture of software development, emphasizing its significance.
Overview of Virtualenv
Virtualenv is a tool that creates lightweight, self-contained Python environments. Each virtual environment has its own installation directories, which means libraries can be installed in an isolated setting. This avoids conflicts with other projects that may require different package versions.
Key Features and Functionalities:
- Environment Isolation: Projects can operate with distinct dependencies without interference.
- Easy Activation/Deactivation: Switching environments is straightforward, allowing for quick transitions across projects.
- Compatibility: Works with various package managers, enhancing its versatility in different development scenarios.
Use Cases and Benefits:
- Dependency Management: It ensures that the specific versions of packages needed for a project are the ones that get used.
- Testing: Developers can test code in same conditions it will run in production, which limits surprises during deployment.
- Team Collaboration: When multiple developers are working on a project, virtualenv aids in maintaining consistent environments across different machines.
Best Practices
Implementing virtualenv must come with an understanding of best practices to maximize its benefits. Having clear guidelines can prevent complications down the line.
Industry Best Practices:
- Create a new virtual environment for each project.
- Use a file to prevent the virtual environment directory from being pushed to version control.
Maximizing Efficiency and Productivity:
- Use a requirements file to track dependencies. This facilitates easy recreation of the environment.
- Regularly review and update packages to maintain security and stability.
Common Pitfalls to Avoid:
- Neglecting to activate the virtual environment can lead to issues with package versions.
- Forgetting to document dependencies can cause confusion for other team members.
How-To Guides and Tutorials
For those new to virtualenv, here’s a step-by-step guide on how to set it up and use it effectively.
- Install virtualenv using :
- Create a new virtual environment:
- Activate the environment:
- Install packages as needed:
- Deactivate when done:
- On Windows:
- On macOS/Linux:
Using these steps, developers can maintain clean project environments and enhance their workflow significantly.
"Isolated environments are essential in avoiding dependency hell."
Culmination
Virtualenv serves as a fundamental tool for Python developers aiming for organized, manageable project environments. By adhering to best practices and following simple setup procedures, developers can ensure their projects remain efficient and free from unnecessary complications. The ability to tailor environments for specific needs defines a crucial aspect of modern software development, ensuring projects are reliable and reproducible.
Foreword to Virtualenv
Virtualenv is a critical component in the Python development landscape. As software projects scale, especially in collaborative environments, managing dependencies becomes vital. In this light, Virtualenv stands as a tool that allows developers to create isolated Python environments, shielding projects from conflicting dependencies and ensuring that each project's specific needs are met without interference from others.
The ability to manage different versions of libraries and packages can drastically reduce the time spent troubleshooting issues arising from dependency clashes. This section introduces the foundations and principles of virtual environments, essential for a seamless software development experience.
Understanding Virtual Environments
A virtual environment is essentially a self-contained directory that houses a specific Python installation along with its libraries. When a developer creates a virtual environment using Virtualenv, they can install packages independently of the system-wide Python installation.
Here are some key aspects of virtual environments:
- Isolation: Each environment maintains its dependencies, preventing conflicts.
- Reproducibility: Developers can recreate environments easily, ensuring that all contributors to a project are using the same dependencies.
- Experimentation: It allows developers to test new packages or versions without affecting other projects.
Importance of Isolation in Python Development
Why is isolation so crucial in Python development? The answer lies in the diverse ecosystem of Python libraries. Each library version can introduce breaking changes or deprecations. Without isolation, a change in one project's dependencies can unexpectedly impact another project.
The benefits of maintaining isolation in development include:
- Version Control: Having the ability to specify exact versions of libraries ensures that projects remain stable.
- Clear Dependency Management: Developers can use files to document the specific dependencies needed.
- Minimized Conflicts: Running multiple projects requiring different library versions can be done without hassle.
"Using Virtualenv is not just a matter of convenience; it is a fundamental practice for ensuring the integrity and reliability of Python projects."
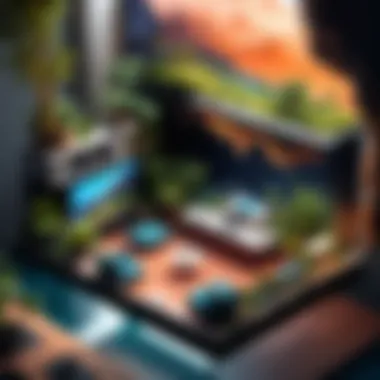
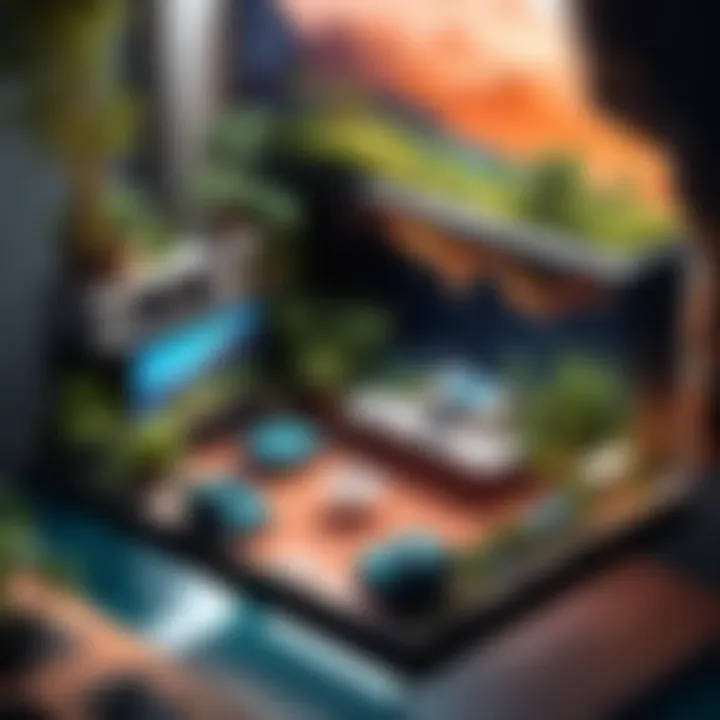
In summary, the introduction of Virtualenv sets the stage for more effective and structured Python development. Understanding the underlying concepts of virtual environments reaffirms the importance of isolation in a world where dependencies can vary widely and unpredictably.
Installation of Virtualenv
The installation of Virtualenv is a critical step in setting up a robust Python development environment. This process allows developers to create isolated environments for different projects, avoiding conflicts between dependencies and ensuring that each project’s requirements are met independently. Proper installation is fundamental for utilizing the extensive features of Virtualenv, which in turn enhances the ease of managing projects, especially in diverse and collaborative development settings.
Prerequisites for Installation
Before you can install Virtualenv, certain prerequisites must be met. First, you should have Python installed on your system, preferably Python 3.x, as most new projects benefit from the latest features and security enhancements. To verify if Python is installed, you can run the following command in the terminal:
If this command returns the version number of Python, you are set. However, if you encounter an error, you will need to download and install Python before proceeding.
Another requirement is having pip, Python’s package installer, which typically comes pre-installed with Python 3.x. You can check if pip is available by running:
If pip is missing, you can easily install it by following the instructions on the official pip website. Once these prerequisites are confirmed, you are ready to install Virtualenv.
Using pip for Installation
The installation of Virtualenv can be accomplished swiftly using pip, the package manager for Python. Open your terminal or command prompt and execute the following command:
This command will fetch the latest version of Virtualenv from the Python Package Index (PyPI) and install it on your system. It’s worth noting that running this command may require administrative privileges, depending on your operating system settings.
After the installation is finished, you will see a confirmation message indicating that Virtualenv has been successfully installed. In case of any errors, reviewing the output can provide insights into potential issues, such as permissions or conflicting packages.
Verifying the Installation
Once the installation is complete, it is crucial to verify that Virtualenv is functioning correctly. You can do this by checking the version of Virtualenv installed. Run the command below in your terminal:
If everything is in place, the terminal should display the version number of Virtualenv installed. If this process is successful, it validates that the tool is ready for use. If an error appears, it may suggest issues related to the installation process, possibly pointing to a misconfiguration or lack of permissions.
Important: Always ensure you are using a virtual environment whenever working on a project to maintain dependency isolation, which ultimately leads to a clean and maintainable codebase.
Creating a Virtual Environment
Creating a virtual environment is a fundamental practice in Python development. It enables developers to isolate project dependencies, thus avoiding potential conflicts between package versions required by different projects. This isolation not only streamlines development but also enhances reproducibility. Each environment is self-contained; this means that, regardless of the global Python installation, a developer can maintain consistent versions of libraries for a specific project. Thus, creating a virtual environment is pivotal when multiple projects involve diverse dependencies.
Basic Command for Environment Creation
To create a virtual environment, the simplest command is as follows:
In this command, is the name of the environment being created. Once this command is executed, a new directory will appear under the name specified. This directory contains subdirectories for the Python interpreter and site-packages, enabling this environment to function independently from the global Python setup. Additionally, using concise names for environments, which can indicate the project or purpose, is a good practice to promote organization.
Specifying Python Versions
When creating a virtual environment, developers often need a specific version of Python. This is particularly vital when a project is dependent on features or functionalities that exist only in certain releases. To specify a Python version, the command changes slightly:
Here, directs virtualenv to create an environment using the Python 3.8 interpreter. It is essential to ensure the specified Python version is installed on your system, otherwise the command will fail. This feature of virtualenv makes it a versatile tool for managing diverse project needs.
Using a Requirements File
Utilizing a requirements file is a robust method for maintaining dependencies within a virtual environment. This file typically named , can list all the packages to be installed in the environment. The format is straightforward; for example, it might contain:
Once the requirements file has been created, installing the packages can easily be done with the following command:
This ensures that anyone else working on the project can replicate the same environment by simply running the command above after activating their virtual environment. Using a requirements file not only simplifies the installation process but also aids in maintaining consistent dependencies, which is crucial for efficient project management.
A virtual environment is essential for stability in Python projects, aiding in better management of dependencies and minimizing the risk of conflicts.
Activating and Deactivating Virtual Environments
Activating and deactivating virtual environments is a fundamental aspect of managing project dependencies effectively in Python development. When you activate a virtual environment, you tailor the system to utilize the installed packages exclusively within that environment. This isolation helps prevent conflicts between different projects' dependencies, ensuring that changes in one environment do not inadvertently affect another. Proper understanding of this process enables developers to work seamlessly across multiple projects without the chaos of dependency mismatches.
When deactivating, the process reverses the situation, returning to the system's default Python environment. This provides a clear separation between your work and other configurations on your system, promoting a cleaner workflow.
Activation Commands for Different OS
Activating a virtual environment varies slightly across different operating systems. Below are the commands that developers can use based on their platform:
Windows
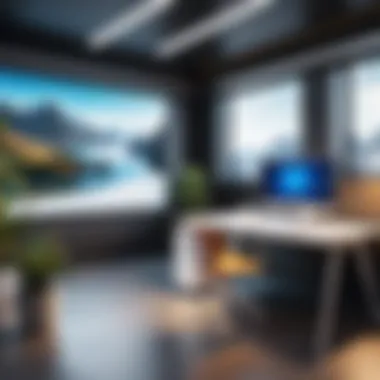
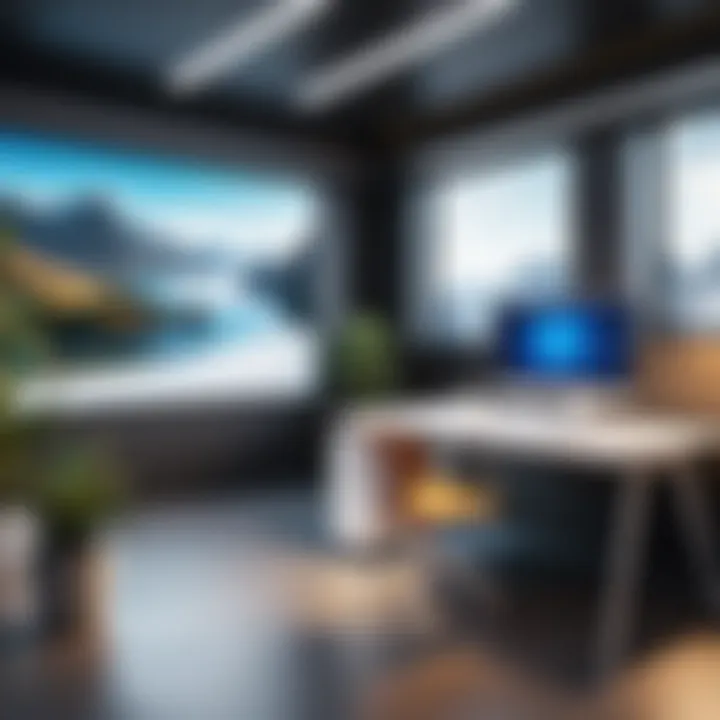
To activate a virtual environment on Windows, navigate to your project directory and run the following command:
macOS/Linux
For macOS or Linux users, the following command is used:
Note: Replace with the name of your virtual environment directory if it differs.
Once activated, you will notice the name of your virtual environment appears in the command prompt, indicating that the environment is now active. You can confirm that the environment is active by running the command or , depending on your OS, to check the path of the Python interpreter in use.
Deactivation Process
Deactivating the virtual environment is straightforward. Regardless of the operating system, the same command is utilized:
Once deactivated, the command prompt will return to its normal state. At this point, Python commands will default back to the system-wide Python installation.
Understanding how to activate and deactivate virtual environments ensures a smoother, more structured development process. It is a skill that enhances productivity, allowing developers to manage multiple projects effectively without cross-contamination of libraries and dependencies.
Managing Dependencies within Virtualenv
Managing dependencies within a virtual environment is essential for effective Python development. This process allows developers to maintain project-specific packages without interfering with system-wide installations. The use of virtualenv ensures that each project has its own set of dependencies, which can significantly reduce conflicts and compatibility issues. When multiple projects require different versions of the same library, a well-managed virtual environment becomes critical.
This section will cover three key aspects: installing packages, updating and uninstalling them, and utilizing a requirements file for efficient dependency management.
Installing Packages in the Environment
Installing packages within a virtual environment is straightforward. Once you have created and activated the environment, you can use to manage your dependencies. The command to install a package is simple:
Replace with the actual name of the package you wish to install. Notably, this will only add the package to your current virtual environment, isolating your project's dependencies from others. It is advisable to check for the latest version of the package before installation. You can do that using the command.
Updating and Uninstalling Packages
As project needs evolve, you may find it necessary to update or remove certain dependencies. To update a package, the command is:
This ensures you are using the latest version, which might contain important bug fixes or new features. On the other hand, if you need to uninstall a package, you can easily do so with:
By handling updates and removals in this manner, you maintain control over your environment and its dependencies.
Using requirements.txt for Dependency Management
A very convenient way to manage dependencies is using a file. This file lists all the packages your project depends on, including their versions. To create this file, use the command:
This will capture the current state of your environment. For new installations or when sharing your project, a simple command can replicate the environment:
This ensures that anyone working on the project can set up their environment accurately. It fosters consistency across different development environments, which is critical for collaboration in software development.
Using a requirements file enhances reproducibility and reduces integration problems, a common headache with software development.
In summary, effective management of dependencies within virtualenv is pivotal for streamlined projects. By mastering installation, updating, and proper use of requirement files, developers can create stable, isolated environments that cater to the unique needs of their projects.
Best Practices for Using Virtualenv
Using Virtualenv effectively requires some attention to detail, especially when it comes to best practices. These practices ensure that your development environments are organized, maintainable, and predictable. Here are several core elements that guide the optimal usage of Virtualenv.
Naming Conventions for Environments
Naming conventions play a crucial role in managing multiple virtual environments. Adopting a clear naming strategy helps in quickly identifying the purpose or the specific project related to an environment. This can prevent confusion and errors over time. Some useful techniques include:
- Project-Specific Names: Use the project's name as part of the environment name. For example, if you are working on a web application called "MyWebApp", you might name the environment .
- Including Python Version: If you are managing multiple versions of Python, include the version number in the name. For instance, can indicate the use of Python 3.8.
By following these naming conventions, you can facilitate easier navigation through environments later on.
Environment Location Strategies
Where you store your virtual environments significantly impacts both accessibility and organization. Making informed decisions on their locations can greatly enhance your workflow. Consider the following strategies:
- Local Project Directory: Placing the virtual environment inside your project folder can be beneficial. This way, your dependencies are contained and specified clearly within that project. For example, the environment can be located at .
- Global Environment Directory: Alternatively, you might opt to keep your environments in a centralized directory for easy management, especially in shared setups. Create a directory specifically for environments, such as , and place all your environments there for easier reference.
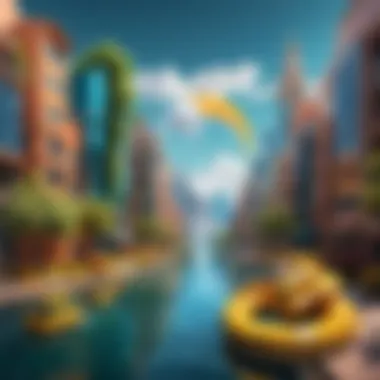
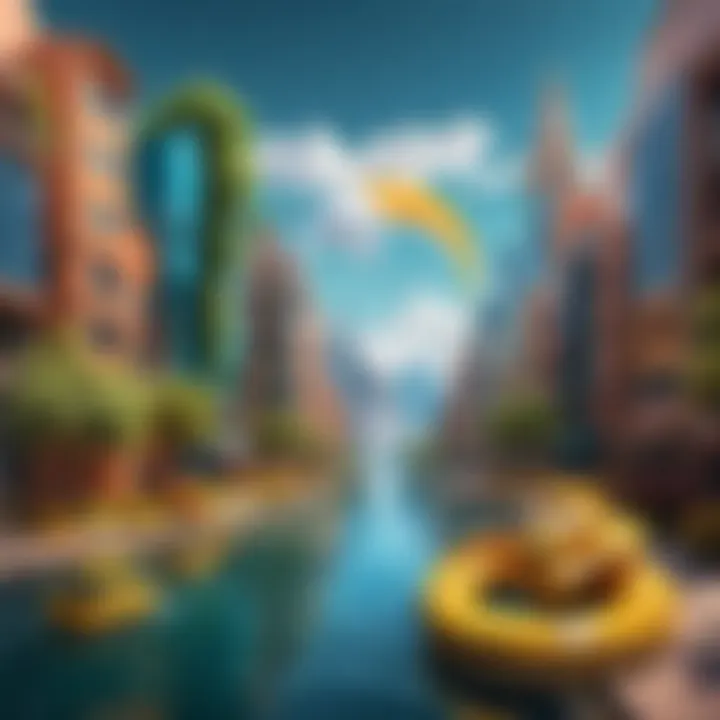
Selecting a location strategy that favors either locality or centralization will depend on personal preference and team dynamics.
Documentation and Maintenance Tips
Documentation is an often-overlooked aspect of using Virtualenv, but it is vital for long-term maintainability. Proper documentation not only assists you but also any team members who may work on the project later. Here are some tips:
- Document Environment Creation: Make sure to provide clear instructions on how to recreate the virtual environment. This includes necessary dependencies and how to activate it.
- Use a File: For maintaining dependency lists, the file is a standard practice. Document all packages using the command .
- Regular Updates: Periodically revisit your environments and upgrade dependencies when necessary. Keeping your packages up to date improves security and compatibility.
Regular documentation, naming conventions, and careful placement of your environments lead to smoother transitions between projects and give clarity to your workflow.
By following these best practices, you can enhance not only your productivity but also ensure your Python projects are organized, manageable, and reproducible.
Common Issues and Troubleshooting
Understanding common issues and troubleshooting techniques is essential for anyone using virtualenv. Virtual environments can save significant time and frustration, but they can also introduce complications. Developers are often tasked with isolating project dependencies, and the tools that assist with this are not always foolproof. By knowing how to address common issues, users can navigate difficulties more smoothly, ensuring a more efficient development process. Here, we will explore several critical areas: package conflicts, environment not found errors, and activation issues.
Package Conflicts and Resolution
Package conflicts happen when multiple projects require different versions of the same library. This is a frequent issue in software development. For example, Project A may depend on Flask version 1.0, while Project B requires Flask version 2.0. When both projects are in the same environment, the conflicting requirements lead to broken functionality.
To resolve package conflicts, developers can utilize the tool within their virtualenv. Here are steps to effectively manage these conflicts:
- Check Installed Packages: Use the command to view all packages and their versions in the current environment.
- Understand Dependencies: Familiarize yourself with what each package requires. Tools like can provide a visual representation of dependencies.
- Upgrade or Pin Versions: Upgrade to a newer package version or pin versions in your file. This can prevent future conflicts.
Resolution may not always be straightforward, but careful management of package versions can mitigate many issues.
Environment Not Found Errors
"Environment not found" errors often occur when trying to activate an environment that does not exist. This can be due to several reasons, such as changes in directory structure or misspelling the environment's name. They can be particularly troublesome as developers try to activate their environments.
To resolve this:
- First, confirm that the virtual environment indeed exists in the specified path. Use the command on Unix systems or on Windows to list directories.
- Check for any typos in the path or the environment name. It’s easy to make a mistake, e.g., missing a character or mixing up upper and lower case.
- If the environment is missing, recreate it using the necessary commands. For instance, you might run if needed.
Troubleshooting Activation Issues
Activation issues arise when the commands meant to enable a virtual environment fail to work. Each operating system has different commands for activation, which can lead to confusion. Missing paths or wrong command syntax can lead to activation failures.
To troubleshoot activation issues:
- Verify the Command: Use the correct command based on your operating system. For Windows, this might be , while for Unix-like systems, it should be .
- Confirm Correct Paths: Ensure that you're executing the command from the correct directory. The shell must be pointed to the environment's bin or Scripts directory.
- Permissions: Check if you have the required permissions to activate scripts on your system. Sometimes, the execution policy may prevent scripts from running.
By keeping these elements in mind, developers can efficiently navigate common problems that arise while using virtualenv. Addressing these issues not only saves time but also leads to smoother workflows and better use of the tools at hand.
Virtualenv vs. Other Environment Managers
When discussing Python development, it's essential to understand how Virtualenv compares with other environment managers. Each tool addresses the need for project isolation, but they approach it differently.
Virtualenv has been a long-standing choice, revered for its simplicity and effectiveness. As developers venture into the Python ecosystem, they encounter alternatives like venv and Poetry. Recognizing the advantages and constraints of these options is vital for efficient project management.
Comparison with venv
The first comparison is with venv, a module that comes packaged with Python 3. This might lead one to believe that venv is the superior option due to its accessibility. However, there are specific distinctions that are crucial to consider.
- Default Behavior: venv creates an isolated environment much like Virtualenv. However, it lacks some of the extra features found in Virtualenv, such as the ability to use different versions of Python at the same time, making it less versatile in certain projects.
- Speed and Dependencies: In many scenarios, venv is faster and uses fewer resources because it’s built into Python. Virtualenv can sometimes struggle with more complex dependencies due to additional overhead from its framework.
In essence, both tools serve the same primary function, but the choice between them can often depend on the specific requirements of the project, such as the need for advanced features or ease of use.
Using Poetry as an Alternative
Poetry is another environment manager that has gained popularity among developers. It not only manages environments but also handles dependencies comprehensively. With Poetry, users can specify their dependencies in a file, streamlining the management process.
- Dependency Resolution: Poetry excels in resolving dependencies, ensuring that the right versions are installed to avoid conflicts. This can save significant time compared to manually tracking dependencies in a Virtualenv.
- Unified Command: Unlike Virtualenv, which requires separate commands for environment creation and package management, Poetry provides a more unified approach. This can be helpful for beginners who find managing multiple commands cumbersome.
While Poetry offers stronger dependency management features, it can also introduce additional complexity that may not be necessary for simpler projects.
Advantages and Disadvantages
It is beneficial to consider the advantages and disadvantages of using Virtualenv relative to its competitors.
Advantages of Virtualenv:
- Flexibility: Works well with different Python versions and supports various configurations.
- Lightweight: Generally has a smaller footprint, making it suitable for quick setups.
Disadvantages of Virtualenv:
- Manual Dependency Management: Developers often have to manually ensure compatibility of packages.
- Lack of Integrated Features: Unlike Poetry, it does not come with the same level of built-in convenience for dependency management.
Epilogue
In the realm of Python development, understanding virtualenv is essential for building robust applications. This conclusion encapsulates crucial takeaways and emphasizes the significance of utilizing virtual environments effectively.
Recap of Key Points
- Isolated Environments: Virtualenv enables developers to create isolated spaces for projects. This allows different projects to maintain separate dependencies, avoiding conflicts that can arise from varying package versions.
- Installation Process: The straightforward installation process using pip ensures that even beginners can set up their environments efficiently.
- Managing Dependencies: Proper management of dependencies is vital in software development. Virtualenv supports this through features like requirements files.
- Troubleshooting: Addressing common issues is critical for maintaining productivity. This guide has outlined strategies to handle package conflicts and activation problems.
- Comparative Analysis: Understanding how virtualenv compares to alternatives like venv and Poetry helps developers make informed decisions based on their project needs and preferences.
The Role of Virtualenv in Modern Development
In today’s fast-paced development landscape, virtualenv plays a critical role. The capacity to create isolated development environments complements best practices in software engineering. It not only enhances productivity but also facilitates collaboration among teams.
As projects become more complex, the need for a systematic approach to managing dependencies is paramount. Virtualenv equips developers with the tools to ensure that their applications work consistently across various setups. Moreover, it promotes best practices in environment management, leading to better code quality and reliability.
To summarize, leveraging virtualenv effectively allows developers to navigate the complexities of modern software development with ease, making it a fundamental skill for Python practitioners.