A Comprehensive Guide to the Go Programming Language
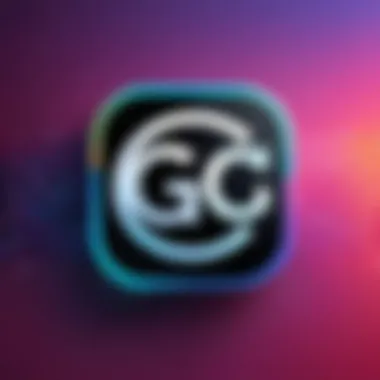
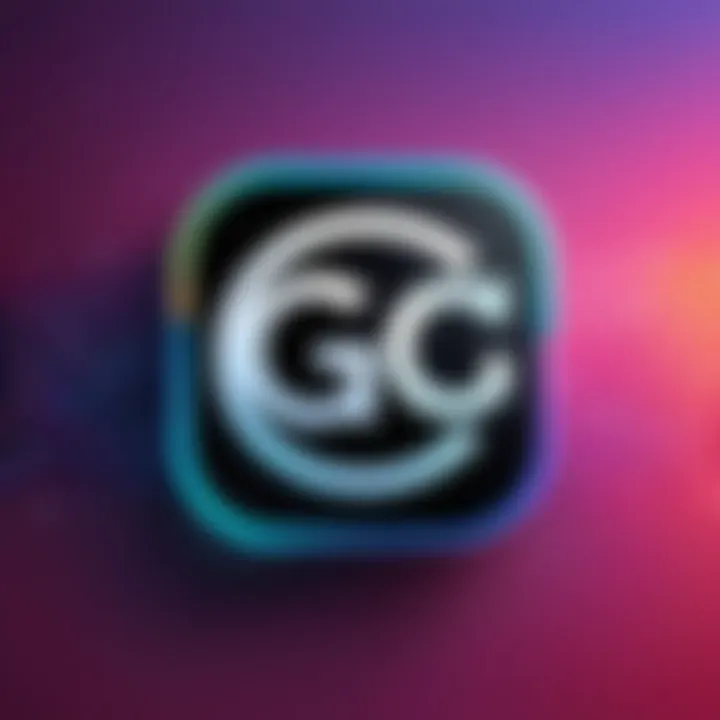
Intro
In recent years, the landscape of software development has changed quite a bit, and one name that rises above the noise is Go. Developed by Google, Go is not just another programming language; it’s a tool that blends simplicity with robust capabilities. For developers, this language brings forth a myriad of possibilities, whether in cloud computing, data processing, or machine learning applications.
Definition and Importance of Go
Go, often referred to as Golang due to its domain name, is a statically typed language known for its efficiency and speed. Its design prioritizes ease of use, allowing developers to write clear and maintainable code while capitalizing on powerful features. The importance of Go in today's software ecosystem cannot be overstated. It facilitates the creation of scalable and high-performance applications which are crucial in an era where data-driven decisions are made in a blink of an eye.
Key Features and Functionalities
Go boasts some hallmark features that distinguish it from other programming languages. These include:
- Goroutines: This is where it shines, allowing lightweight thread management. Developers can efficiently handle concurrent tasks without the overhead of traditional threading models.
- Garbage Collection: Automatic memory management reduces the likelihood of memory leaks, crucial for building stable applications.
- Strong Standard Library: With a solid set of libraries that cover common programming tasks, Go provides developers a powerful toolkit at their disposal.
- Cross-Compilation: Build applications for various platforms using Go's built-in tools, simplifying deployment.
These functionalities make Go an appealing choice not just for startups but also for large-scale enterprises looking to streamline their development processes.
Use Cases and Benefits
Go's versatility allows it to fit into many scenarios effectively:
- Web Development: Frameworks like Gin and Echo help in creating robust web applications swiftly.
- Cloud Services: Many cloud-native tools, including Kubernetes, are built on Go, showcasing its relevance in the cloud arena.
- Microservices: Its efficiency in managing concurrency makes it perfect for building microservices architecture.
The benefits range from better performance to ease of deployment, making it a favored language among many developers.
"Go’s simplicity makes it accessible for beginners, while its powerful features empower seasoned developers to build complex solutions efficiently."
Adopting Go can accelerate project timelines and enhance productivity, which is why it’s gaining traction in various fields of technology.
Prelude to Go
In the crowded landscape of programming languages, Go stands out for its efficiency and simplicity. This section aims to introduce readers to Go, emphasizing its significance in today’s software development scenarios. With its unique combination of performance akin to C, and the ease of use found in languages like Python, Go has carved a niche for itself, especially among developers working on networked and cloud applications.
What is Go?
Go, also known as Golang, is an open-source programming language that Google developed. It was created to handle challenges that arose from the need for scalable and maintainable applications. What sets Go apart is its emphasis on simplicity and clarity, which boosts developer productivity. The syntax is clean, akin to C, yet devoid of the clutter that often burdens modern languages. Here’s a simple illustration:
This snippet demonstrates basic syntax while also showcasing the minimal amount of code required to achieve functionality.
History and Evolution of Go
The history of Go is a tale of necessity and innovation. It was conceived in 2007 by Robert Griesemer, Rob Pike, and Ken Thompson, driven by the challenges they faced in programming with other languages. Go was officially announced in 2009, and since then, it has evolved significantly, gaining traction in a range of applications, from system programming to web services. Over the years, various updates have introduced features such as garbage collection, type safety, and built-in support for concurrent programming through goroutines—helping the language adapt to contemporary needs.
One could say that Go has grown up alongside the increasing complexity and demands of modern systems, making it a desirable choice for developers who have to juggle multiple tasks.
Core Principles of Go
At the heart of Go’s design are several core principles that influence its functionality and utility:
- Simplicity: Go strives to keep things easy to understand, making it accessible for newcomers while maintaining powerful capabilities for seasoned pros.
- Efficiency: It compiles quickly and produces statically linked binaries with minimal dependencies, which is a boon for deploying applications in various environments.
- Concurrency: Go ’s approach to concurrency is among its most significant features. By utilizing goroutines and channels, it allows developers to write efficient concurrent code effortlessly.
- Robust Standard Library: Go comes equipped with a rich standard library, enabling developers to accomplish a wide range of tasks—from web services to file manipulation—without needing third-party libraries.
- Strong Static Typing: This reduces the likelihood of many errors and enhances program reliability, which is essential for large-scale applications.
Go represents a balance between a simple syntax and powerful capabilities, making it a formidable tool in the hands of developers.
In summary, the Introduction to Go provides a crucial foundation for understanding this programming language's relevance and application. Especially for software developers, IT professionals, and data scientists, grasping Go’s fundamentals ushers them into a world of efficient programming practices and scalable applications.
Setting Up the Go Environment
Getting your Go environment up and running is a pivotal step in your journey as a Go developer. The setup not only lays the groundwork for coding but also influences your overall experience with the language. A well-configured environment leads to efficient coding, fewer headaches down the line, and a solid understanding of the Go ecosystem. It's essential to consider some key elements: the installation process, the choice of integrated development environments (IDEs), and the understanding of Go modules, all of which you will tackle in this section.
Installation Guide for Go
To kick things off, let’s talk about installing Go. The installation is straightforward, but it’s vital to follow the steps carefully to avoid confusion. Start by heading over to the official Go website at golang.org where you will find the downloads for various operating systems, including Windows, macOS, and Linux.
- Download the installer: Choose the appropriate binary package for your operating system.
- Run the installer: For Windows and macOS users, the installer will set up Go automatically. Linux users may need to extract files manually.
- Set environment variables: Make sure to add the Go binary directory to your . This is crucial for running commands from any terminal. On Unix-like systems, you might add something like this to your or :
- Verify installation: To confirm that Go is installed correctly, open your terminal and run:You should see the installed version of Go. If everything is set, you're ready to dive deeper.
Setting Up Your IDE
Choosing the right IDE can dramatically enhance your coding experience. While Go developers frequently use text editors like Visual Studio Code, GoLand, or Vim, the choice ultimately boils down to personal preference and project requirements. For those who prefer a more integrated experience, GoLand is a robust option, specifically designed for Go development. However, if you're entering this world on a budget, Visual Studio Code with the relevant Go extensions can fit the bill perfectly.
Here’s how you can set up Visual Studio Code for Go:
- Install Visual Studio Code: Download it from code.visualstudio.com.
- Add Go extension: Open VS Code, navigate to Extensions (Ctrl+Shift+X), search for "Go" by Google, and install it.
- Configure the environment: Once installed, the extension may prompt you to install additional tools. Follow through with this, as they enhance your Go experience.
- Customize settings: You can tweak various settings to suit your style. For example, enabling format on save helps keep your code tidy without additional steps.
Understanding Go Modules
Go Modules are a game-changer in the Go development landscape. They allow you to manage dependencies, versioning, and package structures effectively. This feature addresses some of the challenges a developer might encounter when handling different project requirements.
To get started with Go Modules:
- First, ensure you have at least Go 1.11 or later installed.
- Use the following command to create a new module:This will create a file in your project directory, where you can specify dependencies.
- You can then manage dependencies by using commands like to add new libraries, or to clean up unused ones.
By adopting Go Modules, you can easily manage the complexities of dependencies without the clutter that used to come with building Go applications. It embraces a more structured approach to development, saving you time and effort.
A well-setup Go environment unlocks the capabilities of the language, ensuring a smoother coding experience and greater productivity.
In summary, setting up the Go environment encompasses a few essential steps that form the foundation for your coding endeavors. The installation of Go, the choice of IDE, and understanding Go modules set you on the path to developing efficient and enjoyable applications.
Go Syntax and Basic Constructs
Understanding Go syntax and basic constructs forms the bedrock of coding in this language. As with any programming language, grasping these elements is essential to writing functional and maintainable code. For Go, simplicity is crux; its syntax aims to reduce complexity while facilitating higher efficiency.
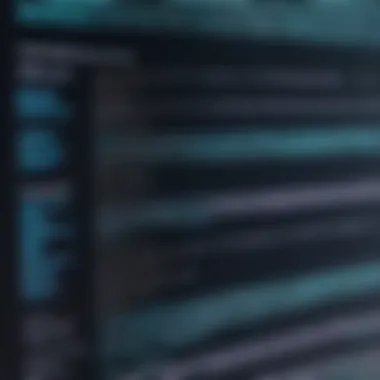
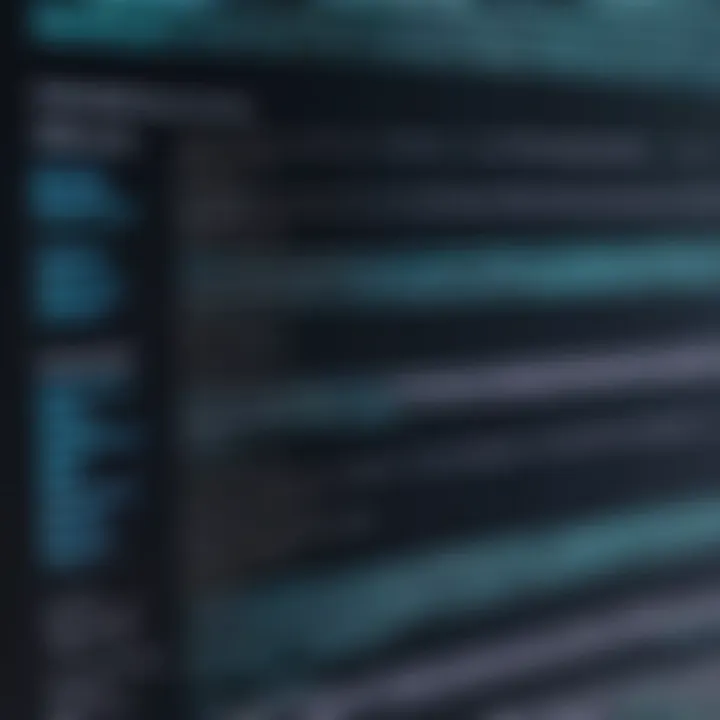
Variables and Data Types
When it comes to variables and data types in Go, it’s important to first recognize that everything starts with these fundamental building blocks. Variables in Go are declared using the keyword. For instance:
From the above example, the variable is of type . Go also supports shorthand notation for variable declarations that combines declaration and initialization:
This ease of variable declaration makes it intuitive for programmers to handle data without getting bogged down by unnecessary syntax. The language offers several data types - such as integers, floats, booleans, and strings - each suited to specific purposes. By striking a balance between simplicity and capability, Go enables developers to leverage types to their advantage without overwhelming them with choices.
It's advisable to understand data types thoroughly, as this affects memory usage, performance, and the program's overall functionality. Also, Go’s strong typing reduces the risk of type-related bugs, making code more robust and less error-prone.
Control Structures
Control structures are vital for directing the flow of a Go program. In essence, they dictate how different blocks of code execute based on certain conditions. Go provides common control structures like , , and . For example:
The statement allows for decision-making in code. In Go, loops are particularly interesting, as they are the only loop construct. This might seem limiting but, in practice, it simplifies how developers iterate over collections:
Control structures in Go bring clarity and help maintain simplicity in logic flows. As one navigates their programming journey, understanding these will be critical, especially for complex functionalities like error handling and concurrency.
Functions in Go
Functions serve as fundamental units of code reusability and modularity in Go. They allow developers to encapsulate specific operations into standalone units that can be called multiple times. Functions are defined using the keyword, followed by the function name and parameters:
The example creates a function that takes a parameter and returns a string. Functions can also return multiple values:
This feature of returning multiple values is quite handy, particularly when you need both quotient and remainder from a division operation. Functions allow for clean, organized code while promoting reusability and logical separation of concerns. With well-defined functions, your Go programs can become easy to read and maintain.
"In programming, one must first learn to think with your code, creating efficient and clean solutions through functions."
By mastering these syntax elements, you pave the way for a smoother coding experience in Go, making subsequent topics and applications more manageable and coherent.
Object Orientation in Go
Object orientation is a powerful programming paradigm that emphasizes the use of objects to represent data and methods. While Go isn’t a traditional object-oriented language like Java or C++, it certainly incorporates many core concepts allowing the creation of structured, modular, and reusable code. Understanding these concepts can greatly benefit developers in structuring their applications logically and effectively.
Understanding Structs
One of the bedrocks of object-oriented programming in Go is the . A struct is essentially a collection of fields, allowing developers to group related data together. The beauty of structs is their simplicity and practicality. For example, consider a scenario in which you want to manage a library system. You could define a struct like this:
In this case, the struct has three fields — Title, Author, and Year. This encapsulation of the book's attributes allows for clean and logical representation. Accessing and manipulating this data becomes manageable, as each book can be treated as a unique entity.
Additionally, structs can be embedded into other structs, which allows for composition. This means you can build more complex data structures while benefiting from the simple, declarative style Go employs. They serve as an excellent foundation for building types that share behavior and state.
Methods and Interfaces
Methods in Go are functions that are associated with a specific type. We can define methods on our previous struct, like so:
Here, the method generates a justifiable string representation of the book. It shows how you can add behavior to your data types, hence facilitating object-oriented design.
Next comes interfaces. An interface is a set of method signatures, and any type that implements those methods is said to satisfy the interface. For example:
Any type that has the method now satisfies the interface. This abstraction allows for flexible code design, enabling different types to be treated uniformly as long as they adhere to the required interface.
Embedding and Composition
One of the notable aspects of Go’s approach to object orientation is its composition over inheritance paradigm. Rather than relying on complex hierarchies, Go encourages embedding. By embedding structs within other structs, we create a clear, concise relationship without the overhead of traditional inheritance models.
In this example, Author is embedded into Book. It allows direct access to the fields through , encouraging code reuse and ensuring a flat structure. This approach promotes clarity and reduces the mess that can often accompany deep inheritance trees.
The use of structs, methods, interfaces, and composition in Go fosters a modern, yet pragmatic approach to object-oriented programming that accommodates simplicity and flexibility, vital traits that modern software demands.
In summary, while Go's object orientation may not follow the conventional mold set by other languages, it provides distinct, effective tools that streamline code organization and functionality. Understanding these concepts is vital, especially for software developers and IT professionals looking to harness the full power of Go.
Error Handling in Go
Error handling in Go is more than just a safety net; it is a fundamental part of the programming paradigm. Unlike many other languages that emphasize exceptions, Go approaches errors with a simplicity that resonates well with its overall design philosophy. This section aims to dissect the significance of error handling, how to understand errors in Go, and the various techniques available to handle them effectively.
Understanding this aspect of Go not only improves code quality but enhances overall reliability in applications, making it an essential topic for any developer.
Understanding Errors
In Go, errors are represented by the built-in type, which is an interface that allows developers to define error behaviors easily. This straightforward approach encourages programmers to handle errors explicitly rather than relying on an exception mechanism.
When a function encounters an error, it typically returns an error value along with the functional result. Here’s a simple code snippet to illustrate the point:
In this example, the function returns an error if a division by zero is attempted. This approach forces the caller to check for errors, promoting more mindful coding practices.
Essentially, understanding how to work with errors helps in recognizing different types of failures—which could be user error, system failures, or network issues—and in responding appropriately.
Error Handling Techniques
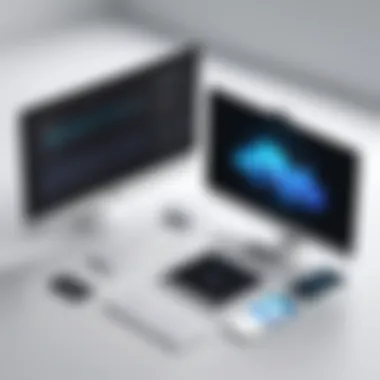
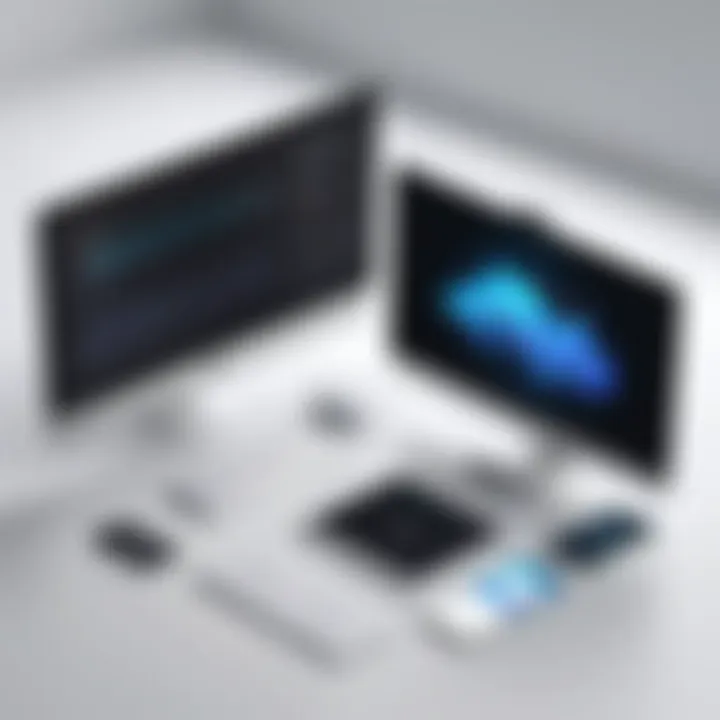
Handling errors in Go is executed through a variety of techniques that not only ensure errors are accounted for but can also provide informative logs and recovery paths when needed. Here are some useful techniques to consider:
- Check and Handle Errors Immediately: Always check errors right after a function call, as this helps in handling problems as they arise. Ignoring errors can lead to unexpected behavior down the line.
- Wrap Errors for Additional Context: If you're passing errors up the call chain, it’s a good practice to wrap them with additional context before returning. Go provides the to enable you to do this seamlessly.
- Use Custom Error Types: When your application deals with specific error conditions repeatedly, custom error types can help distinguish between different categories of errors, thus enriching your error handling strategy.
- Logging: Implement robust logging for error events. This not only aids in debugging but also provides insights during production issues.
- Panic and Recover: While typically discouraged in favor of explicit error checks, there are scenarios where panicking is suitable—particularly in the case of programming errors (like attempting to read from a nil pointer). This approach can be paired with and to manage unexpected situations gracefully.
"In Go, handling errors is not just about preventing crashes but about delivering an experience that anticipates and responds to user/app needs effectively."
By adopting these techniques, developers can significantly bolster their application's resilience. Each method contributes to building a robust software foundation that both respects and responds to failures.
Concurrency in Go
Concurrency is a cornerstone of modern software development, allowing programs to handle multiple tasks simultaneously. In Go, concurrency is not just a feature; it's woven into the fabric of the language. This ensures that developers can build efficient, scalable applications without wrestling with the complexities often associated with traditional threading models. By leveraging concurrency, Go applications can significantly improve performance and responsiveness, which makes it particularly beneficial in fields like web development, cloud services, and data processing.
Goroutines Explained
Goroutines are perhaps one of the most attractive features of Go, making concurrency easier to achieve than in many other programming languages. A goroutine is a lightweight thread managed by the Go runtime. They are simple to create, initiated with the keyword followed by a function call.
Here’s a quick example:
In this snippet, the function runs concurrently with the function. This ability to spawn thousands of goroutines without much overhead is what sets Go apart. Each goroutine uses only a fraction of the memory of a traditional thread.
Channels and Communication
Channels in Go serve as conduits for goroutines to communicate with each other. They provide a way to send and receive data safely between multiple goroutines, helping to avoid race conditions. By offering a built-in mechanism for synchronization, channels allow developers to structure their programs in a more predictable manner.
For example:
In this code, a channel is created, and the function sends a message through it. The main function waits to receive the message, ensuring that both goroutines work in harmony. Using channels efficiently prevents many of the pitfalls that come with concurrent programming, notably deadlocks and race conditions.
Synchronizing Goroutines
When working with multiple goroutines, it's crucial to manage their synchronization. Go provides various tools for this purpose. One of the simplest methods is to wait for goroutines to finish using the structure.
Here’s how that might look:
In this example, is instrumental in coordinating the completion of several goroutines. As each goroutine finishes its task, it calls . The function will only proceed after all tasks are complete, avoiding premature termination.
Goroutines and channels create a model of concurrency that is simple, yet powerful. Developers can express complex concurrent patterns clearly and concisely.
Understanding these concepts can give developers a substantial edge in the software development world, enhancing their ability to write robust, high-performance Go applications.
Testing and Debugging in Go
Testing and debugging form the backbone of sound software development, and Go helps developers with robust tools that streamline these processes. As Go enthusiasts will know, writing reliable code is no walk in the park. Bugs can be sneaky little gremlins, lurking in our applications, waiting to pounce when we least expect it. That is why implementing testing and debugging from the get-go is key to enhancing code quality and maintaining agility in software development.
The significance of testing in Go can’t be emphasized enough. Testing ensures that every part of your application behaves as expected, catching errors before they escalate into costly issues in production. Utilizing Go’s built-in testing package simplifies the creation and execution of tests, empowering developers to verify their code efficiently. Moreover, with continuous integration being the gold standard in the development environment, adding robust tests helps in stimulating a seamless workflow.
Writing Tests
Writing tests in Go is straightforward, thanks to its testing package. First off, you need to create a file that ends in . This tells Go that this file contains tests. Here’s a step-by-step approach:
- Import the testing package: Make sure to import the package to gain access to the testing functionality.
- Create test functions: Test functions must start with the word , followed by the name of the function you want to test, like so:
- Use helper functions: The parameter in your test function is an instance of . You can use its methods like or to log failure messages. Here’s a simple example:
- Run your tests: You can run your tests by executing the command in the terminal. This command will find all test files and execute the tests within them, making it easy to see what passes and what fails.
By integrating tests associated with your development process, you'll find that it becomes habit-forming. As you write more tests, coding becomes less daunting, and you’ll likely feel a sense of achievement watching the tests pass.
Using the Go Debugger
After you’ve put your testing strategies in place, a debugger is the next tool in your belt. Go comes with a built-in debugger called Delve, and it is incredibly powerful for diving deeper into the code execution flow. Debugging may seem like a chore, yet it’s a necessary evil to attain clarity in complicated situations.
Delve allows you to analyze the state of your variables, observe function calls, and follow the execution path step-by-step. Using the debugger might seem overwhelming at first, but the clarity it offers can be priceless. Here are some fundamental tasks you can accomplish with Delve:
- Set Breakpoints: Stops the execution at specified lines allowing you to examine the context at that moment.
- Inspect Variables: You can see the values of variables at any given point, helping you unravel how your application reached a certain state.
- Step Through Code: Walk through your code line by line to see the exact flow and understand where things may be going off the rails.
Here’s a quick snippet on how to start the debugger:
Subsequently, you can set breakpoints with a simple command like , where is the function you want to pause at.
"Effective debugging is not just a routine; it’s a crucial skill that saves time and frustration down the line."
Packages and Libraries
In Go, the concept of packages and libraries is paramount. They are the backbone of Go programming, enabling developers to write modular, reusable, and maintainable code. The structure of Go encourages a clean separation of code into packages, which can then be easily imported and utilized across different projects.
Using packages brings a multitude of benefits:
- Modularity: By breaking down code into distinct packages, developers can isolate functionalities, making the codebase easier to manage and understand.
- Reusability: Once a package is created, it can be reused across multiple projects. This prevents wasted effort and promotes efficient coding practices.
- Collaboration: Packages facilitate collaboration among developers. Teams can work on different packages independently, which reduces the chances of code conflicts.
However, there are important considerations to keep in mind when working with packages in Go. Namely, it is vital to adhere to naming conventions and structuring guidelines to prevent confusion and maintain consistency across codebases. Poorly designed packages can lead to ambiguity and increased complexity down the line.
"Good code is its own best documentation."
— Steve McConnell
Core Standard Libraries
Go's standard library is rich and diverse, featuring packages that cover a variety of essential functionalities. This includes support for input/output, string manipulation, and networking, among others. Utilizing these core libraries can significantly accelerate the development process, as it eliminates the need to reinvent the wheel.
Some noteworthy standard libraries include:
- net/http: This package is crucial for building web applications. It provides HTTP clients and servers, making network communications a breeze.
- fmt: Key for formatted I/O, it allows developers to print outputs in various formats, which is fundamental for debugging and user interaction.
- encoding/json: Parsing and serializing JSON has become a standard in many applications, and this package simplifies working with JSON data significantly.
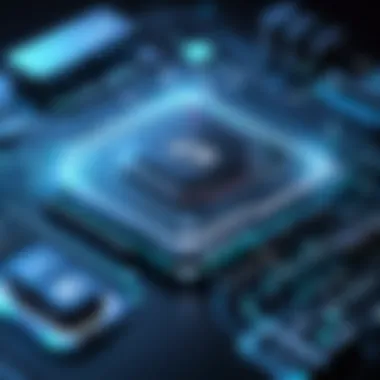
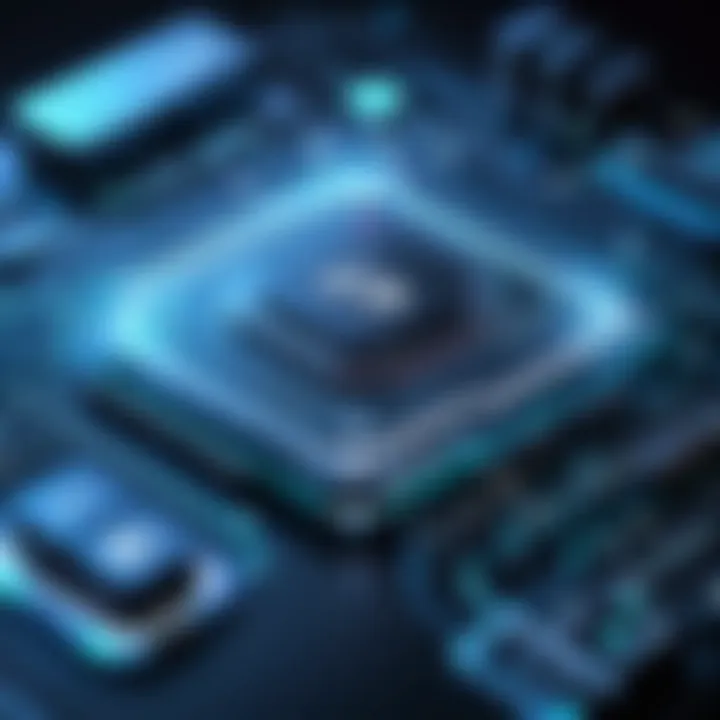
Familiarizing oneself with these libraries not only enhances productivity but also empowers developers to craft efficient, high-performance applications.
Creating Custom Packages
Building custom packages allows developers to tailor Go’s functionality to specific needs or preferences. This is particularly useful for niche functionalities that aren't covered by the core libraries. Creating a custom package can sound daunting, but it follows a straightforward process.
- Define Your Package: Decide on the purpose of your package and outline its functions.
- Structure Your Code: Follow Go's conventions—name your directory with the package name and ensure your files are named consistently.
- Write the Code: Focus on keeping your code clean, well-commented, and properly organized.
- Testing: Make sure to include tests for your package to verify functionality and performance. Go's testing framework makes this easy to implement.
- Documentation: Good documentation is essential. Use Go's documentation tools to create helpful descriptions for your package's functions and usage.
In summary, understanding and leveraging packages and libraries effectively is crucial for any Go developer. By harnessing the power of the core standard libraries and creating custom packages, programmers can greatly enhance their coding workflows and build robust applications.
Practical Applications of Go
Go has carved out a unique niche in the programming landscape, functioning powerfully in various real-world applications. The relevance of focusing on practical applications can't be overstated, especially for developers keen on understanding how to leverage Go's unique features. Learning about real-world use cases adds a layer of understanding to the language itself, which can help clarify theoretical concepts. From spinning up web services to constructing command-line tools, Go's characteristics enhance productivity and performance in diverse projects.
Building Web Services
One of the most prominent uses of Go is in building web services. This isn’t just another trend; it's a fundamental aspect of how software is developed today. The simplicity of creating concurrent requests, combined with Go’s efficient memory management, makes it ideal for high-performance web servers. With a built-in HTTP package, developers can create robust web applications without needing extensive external libraries.
- Performance: Go’s concurrency model, based on goroutines, ensures that web applications handle numerous simultaneous connections efficiently.
- Simplicity: The language's clean syntax and strong standard library minimize boilerplate code, helping programmers focus on features rather than fighting with frameworks.
"Go has a reputation for being fast, both in execution and in the development cycle itself."
To illustrate, imagine crafting a high-traffic RESTful API. Go’s built-in tools simplify routing and middleware management, while libraries like Gin and Echo provide even more functionality. This means quicker iterations and lesser time spent on debugging or configuring extra parts of the stack.
Developing Command-Line Tools
The second application worth discussing is developing command-line tools. For many developers, CLI tools are the bread and butter of their workflow. Go shines here because of its cross-compilation capabilities. You can compile your Go code into an executable for different operating systems with minimal fuss.
- Cross-Platform: With a single codebase, you can create binaries for Windows, Mac, and Linux, making deployment straightforward.
- Speed and Efficiency: Go compiles to machine code, resulting in fast binaries that execute swiftly, which is crucial when executing scripts or automation tasks within the dev process.
For example, many organizations have benefited from developing CLI utilities in Go that streamline their CI/CD pipelines. The straightforwardness in making a tool to handle tasks, such as deploying to servers or managing configurations, proves appealing. Notably, tools like Cobra and Survey in Go extend CLI functionalities, enabling rich user interactions and advanced command parsing features.
When wielding Go for practical applications, the key is to leverage its strengths—efficiency, simplicity, and robust performance. With increasing demands in software development, mastering Go becomes an invaluable asset for developers looking to enhance productivity and effectiveness in their day-to-day tasks.
Best Practices in Go Development
Best practices in Go development are not merely suggestions; they form the backbone of robust, maintainable, and efficient code. In a world where software is constantly evolving, adopting these practices ensures that developers can keep pace with changes while producing high-quality applications. By establishing strong coding habits, developers not only optimize their workflow but also improve collaboration with other developers.
Code Structuring and Organization
When it comes to coding in Go, organizing your code might seem trivial but it's vitally important. A well-structured codebase enhances readability, making it easier for other developers (or even your future self) to navigate the logic of the application. Here are a few specific elements to consider:
- Use of Packages: Go's package system is one of its standout features. Organizing related functions into packages not only keeps files tidy, but it also encapsulates functionality. For instance, if you're building a web application, you might have packages for handlers, models, and utilities. This clear separation allows for simpler testing and debugging.
- File Naming Conventions: Stick to clear and consistent naming conventions. For example, if you have a file related to user authentication, name it something like instead of just . This way, anyone glancing at the directory can understand the purpose of the file without diving in.
- Folder Structure: A typical Go project often follows a standard layout. This generally includes directories like , , and . Each directory serves its purpose, separating executable applications from libraries and internal packages that aren’t meant for public use. This architecture significantly aids in managing larger projects.
Overall, structuring and organizing code in Go isn't just a best practice; it's an efficient roadmap for ongoing development and scalability.
Documentation and Comments
As much as effective code speaks for itself, clear documentation and well-placed comments are the unsung heroes of software development. They bridge the gap between the developer and the code.
- GoDoc: An excellent tool that automates documentation in Go is GoDoc. It allows you to write documentation inline using simple comments, which are then transformed into HTML. This makes it easy for others to understand how to use your packages without sifting through the code.
- Commenting Practices: While commenting can help, overdo it, and your code might become even more cluttered. Ensure comments add value. For instance, rather than merely restating what the code does, explain why it does it. A good comment should clarify intent or detail complex logic that might trip up a reader later.
- README Files: Providing a comprehensive README file at the root of your project can be a lifesaver. Include sections like installation instructions, usage examples, and contribution guidelines. This helps users and contributors understand your project quickly and can significantly impact its adoption.
"An ounce of prevention is worth a pound of cure." - Benjamin Franklin. In Go development, the same applies to proper documentation and comments. Spending time upfront can save countless hours of confusion later on.
In summary, adhering to best practices in code structuring, organization, and documentation can set developers apart in the competitive tech landscape. By focusing on these elements, you help ensure your Go applications are not only functional but also maintainable in the long run.
Community and Resources
In the world of programming, having a solid community and rich resources can make all the difference. The Go programming language, often referred to simply as Go, has built a vibrant ecosystem over the years. This not only benefits beginners trying to find their footing but also experienced developers seeking to contribute or learn new tricks. Engaging with the community and leveraging available resources enhances one's programming journey significantly.
Contributing to Open Source
Open source is the lifeblood of innovation in the tech industry. When we speak about contributing to open-source projects in Go, we see a unique opportunity to connect with other developers. It's a two-way street; as you contribute, you also learn from others. Whether it's fixing bugs, adding features, or writing documentation, such contributions amplify one's skill set.
Moreover, many Go projects, like Kubernetes or Hugo, are open-source. Engaging with these projects not only polishes your coding practices but also exposes you to large-scale application architecture. If you are wondering where to start, consider browsing GitHub for Go repositories. Participating in these projects can prove beneficial to understanding coding patterns and project management styles.
Some steps to get involved with open source include:
- Find a Project: Look for projects that align with your interests. Many sites list Go projects seeking contributors.
- Read the Documentation: Familiarize yourself with the project structure and guidelines.
- Start Small: Begin with minor issues, then gradually tackle more complex tasks.
- Engage in Discussions: Participate in chats or forums related to the project to gain insight and make connections.
Getting involved in open-source is not just an individual benefit; it strengthens the community as a whole.
"The best way to find yourself is to lose yourself in the service of others."
— Mahatma Gandhi
This idea resonates strongly in the open-source community where you learn and grow while helping others.
Online Communities and Forums
In addition to open source, online communities play a huge role in the Go ecosystem. Platforms like Reddit or specialized forums allow developers to ask questions, share knowledge, and offer advice. These forums can be great for obtaining immediate help or discussing complex topics in depth.
The Go Forum, for instance, serves as a dedicated space for Go enthusiasts. You can engage in discussions ranging from basic syntax to advanced concurrency patterns. Subreddits like r/golang provide an informal atmosphere where both new and seasoned developers freely share resources, job postings, project ideas, and more.
Key benefits of participating in these online communities include:
- Knowledge Sharing: Engage in discussions that can clear up confusion or reinforce understanding.
- Networking: Connect with other professionals, potentially leading to collaboration or job opportunities.
- Resource Access: Find valuable resources like tutorials, articles, or tools shared by fellow members.
- Mentorship Opportunities: Seek out seasoned developers who can offer guidance or insight.
Community engagement is not a one-off thing; it's an integral part of a developer's life. Staying active in these spaces ensures continuous learning and growth as new trends emerge and technologies evolve.
Overall, the synergy created between contributing to open source and engaging in online communities fortifies the Go developer landscape. Leveraging both avenues opens doors to immense growth, knowledge sharing, and networking opportunities, transforming the way developers interact with technology and each other.
Ending and Future of Go
As we wrap up this extensive exploration of the Go programming language, it's crucial to reflect on why this topic is so pertinent in the current tech landscape. Go has carved out its niche, being recognized not just for its simplicity but also its powerful capabilities in building scalable and efficient applications. Developers today are in a fast-paced world where efficiency and performance can't be left out of the equation. Here, Go shines. Its concurrency model, coupled with an easy-to-grasp syntax, makes it a go-to language for various projects, from web services to cloud applications.
Summary of Key Takeaways
- Simplicity Meets Efficiency: One of the standout features of Go is its simplicity. The language is designed to be easy to learn yet robust enough to tackle complex solutions. This is a combination that benefits both newcomers and seasoned professionals alike.
- Strong Concurrency Features: Go's approach to concurrency, using goroutines and channels, allows developers to manage multiple tasks efficiently. This is particularly beneficial when dealing with I/O-bound applications.
- Rapid Development: With a clean standard library and a rich ecosystem of packages, developing software in Go can be remarkably fast. This allows teams to deliver projects quicker without compromising on quality.
- Growing Community and Resources: The Go community is vibrant and continuously growing. From forums on Reddit to discussions on Facebook, there's a wealth of resources available for developers looking to enhance their Go skills or seek help with particular problems.
- Robust Performance: While it may not be the fastest language out there, Go often strikes a balance between performance and development speed. This makes it suitable for a variety of applications where performance is essential, but not the only criterion.
The Role of Go in Modern Development
Go has emerged as a significant player in software development, especially considering the tech industry's shift toward microservices, cloud computing, and other contemporary architectural patterns. Companies like Google, Dropbox, and Netflix utilize Go for mission-critical applications. It's no wonder that many up-and-coming startups are adopting Go as part of their tech stack.
Additionally, the "Cloud Native Computing Foundation" recognizes Go as one of the primary languages for cloud-native applications, positioning it at the forefront of modern software development. Its ability to handle high concurrency and efficiency positions it perfectly for developing services that scale with user needs.