Mastering GPU Programming: A Comprehensive Guide
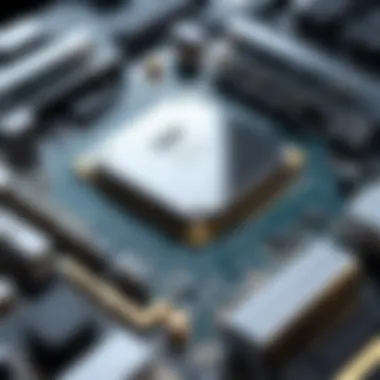
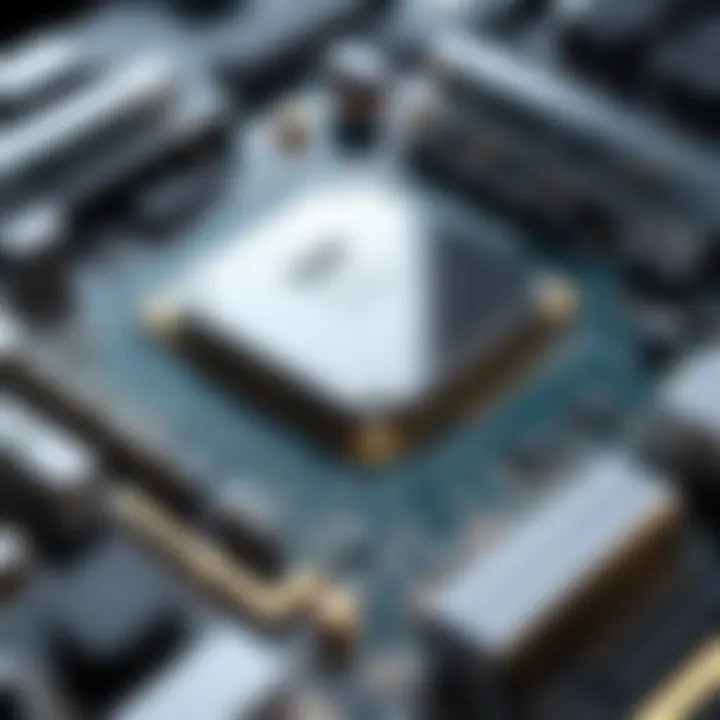
Intro
In recent years, the demand for faster processing speeds and more efficient computing has spurred the growth of Graphics Processing Units (GPUs) in various fields. This trend is not confined to gaming or graphics; rather, it has expanded into domains such as data analytics, machine learning, and cloud computing. This expansion highlights the versatility and power of GPUs, making them an essential component in modern computing. Understanding GPU programming is vital for professionals in technology, as it opens opportunities for enhancing applications and systems.
Overview of GPU Programming
Definition and Importance of GPU Programming
GPU programming refers to the practice of utilizing Graphics Processing Units to perform computations traditionally handled by CPUs. The significance lies in the ability of GPUs to execute many operations simultaneously, leveraging parallel processing. This capability is pivotal for tasks requiring extensive computations, making them essential in high-performance computing environments.
Key Features and Functionalities
The core features of GPU programming include:
- Parallelism: Multiple threads can run concurrently, significantly speeding up processing times.
- High throughput: Efficiently handles a large volume of calculations, ideal for data-intensive applications.
- Specialized APIs: Tools such as CUDA by NVIDIA and OpenCL allow developers to access GPU resources effectively.
Use Cases and Benefits
GPU programming is applied in several domains including:
- Machine Learning: Accelerating algorithms that require massive data processing.
- Data Analytics: Enhancing data visualization and processing speeds for big data.
- Computer Graphics: Producing realistic renderings in real-time.
- Scientific Computing: Enabling simulations and models that require rapid calculations.
The benefits of GPU programming include reduced computation times, improved resource utilization, and enhanced capabilities for handling complex tasks.
Best Practices
Industry Best Practices for Implementing GPU Programming
To get the most from GPU programming, several best practices should be followed:
- Test and benchmark different algorithms to discover which performs best on GPU.
- Optimize memory usage to avoid bottlenecks during data transfer between CPU and GPU.
- Use libraries and frameworks that are specifically designed for GPU computations.
Tips for Maximizing Efficiency and Productivity
Maximizing the effectiveness of GPU programming can be achieved through:
- Writing efficient kernels that minimize operations outside parallel regions.
- Profiling performance to spot and resolve slowdowns.
- Leveraging existing libraries for common tasks to save time and effort.
Common Pitfalls to Avoid
Developers should be cautious of the following pitfalls:
- Overloading the GPU with too many threads, which can reduce performance.
- Neglecting data transfer times, which can offset computational gains.
- Failing to properly understand GPU architecture, leading to inefficient coding.
Case Studies
Real-World Examples of Successful Implementation
In healthcare, researchers have utilized GPUs to process large volumes of medical imaging data. This use case allowed for quicker diagnosis and better patient outcomes.
Lessons Learned and Outcomes Achieved
The implementation of GPU programming in real-world applications demonstrates that an initial investment in learning and development can lead to significant performance improvements. Companies reported reductions in processing times by up to 90%.
Insights from Industry Experts
Experts emphasize the importance of continuous learning in GPU programming, noting the rapid evolution of technologies and practices in the field. Keeping updated on the latest advancements is crucial for maintaining a competitive edge.
Latest Trends and Updates
Upcoming Advancements in the Field
The GPU landscape is continually evolving, with trends focusing on machine learning and artificial intelligence, as GPUs become central to these technologies.
Current Industry Trends and Forecasts
Experts predict a growing reliance on GPUs for computational tasks, aligning with the increasing data-driven decision-making processes across industries.
Innovations and Breakthroughs
Breakthroughs in GPU architectures, such as NVIDIA’s Ampere architecture, provide improvements in performance and energy efficiency, setting the stage for future developments in GPU programming.
How-To Guides and Tutorials
Step-by-Step Guides for Using GPU Programming Tools
For newcomers, starting with CUDA could be beneficial. Begin by installing the necessary toolkit, learning the basics of kernel programming, and gradually working on small projects.
Hands-On Tutorials for Beginners and Advanced Users
Online platforms like GitHub and forums like Reddit often feature tutorials that cater to all skill levels. Engaging with community resources can offer valuable insights and practical knowledge.
Practical Tips and Tricks for Effective Utilization
Practicing with smaller datasets can help understand the behavior of algorithms in a GPU environment before scaling up.
Learning GPU programming is not just about speed and power, but also about thinking differently regarding problem-solving strategies.
Ultimately, mastering GPU programming equips developers and professionals with the skills necessary to tackle problems more efficiently, leveraging the extraordinary capabilities of modern GPUs in a variety of applications.
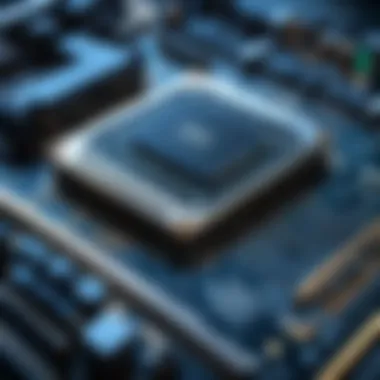
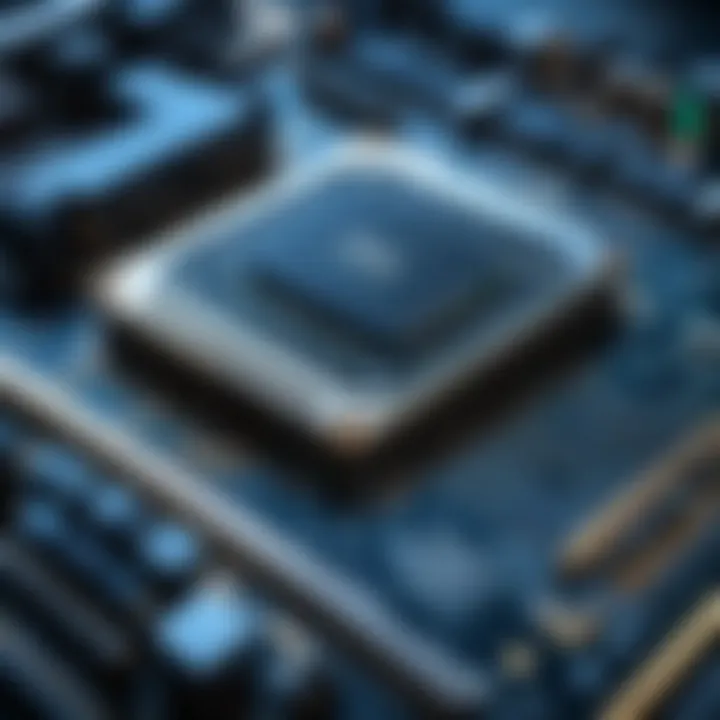
Preamble to GPU Programming
GPU programming has emerged as a critical avenue in the landscape of computing technologies. Understanding its significance is crucial for any professional delving into software development, data analysis, or scientific research. This section aims to unpack the foundations of GPU programming, emphasizing its importance, relevancy, and implications in modern computing.
Definition and Importance
Graphics Processing Units (GPUs) are specialized processors designed to handle parallel processing efficiently. They excel at performing a multitude of small calculations simultaneously, making them ideal for tasks that can leverage parallelism. The definition of GPU programming revolves around the practice of writing software that utilizes these devices to execute computations that would be inefficient or impossible on conventional Central Processing Units (CPUs).
The importance of GPU programming lies in its ability to accelerate various applications, particularly those that involve data-heavy operations. For instance, in fields such as machine learning, image processing, and complex simulations, GPUs can significantly reduce the time required to execute large datasets. By harnessing the power of GPUs, developers can unlock performance enhancements that were previously unattainable.
"The shift from traditional CPU-centric approaches to GPU-accelerated solutions represents a significant leap in computational capabilities."
Incorporating GPU programming into one's skill set not only enhances efficiency but also opens doors to innovative applications that are reshaping industries. As workloads become increasingly demanding, understanding GPU environments becomes essential for anyone aiming for productivity and innovation.
The Rise of GPU Computing
The rise of GPU computing corresponds with the mounting requirements of modern applications. Initially designed for rendering graphics in video games, GPUs have transitioned into versatile tools for a broad spectrum of tasks. This evolution is largely driven by the advancements in GPU architecture, enabling them to tackle more complex computation problems.
Over the last decade, the adoption of GPU programming has skyrocketed. Industries such as finance, healthcare, and autonomous driving are leveraging GPU processing power to gain competitive advantages. For example, GPU applications in machine learning have made it possible to train deep learning models in a fraction of the time it would take using CPU systems alone.
Furthermore, the development of APIs such as CUDA and frameworks like TensorFlow has simplified the process of GPU programming for developers. This accessibility facilitates an increasing number of professionals entering the realm of GPU programming, transforming it from a niche discipline into a fundamental component of computing strategy.
Overall, grasping the fundamentals of GPU programming is not just beneficial but increasingly necessary. As this guide progresses, the complexities of this field will be unfolded in successive sections, equipping readers with necessary knowledge to navigate and master GPU programming efficiently.
Understanding Graphics Processing Units
Understanding Graphics Processing Units (GPUs) is crucial in the realm of GPU programming. This section delves into their architecture and fundamental differences from Central Processing Units (CPUs). Grasping these concepts not only clarifies how GPUs work but also helps in leveraging their capabilities for various applications. From machine learning to scientific computing, the efficiency of GPU programming largely hinges on the understanding of GPU structure and processing methods.
Architecture of GPUs
The architecture of GPUs is a key factor contributing to their performance and functionality. A GPU consists of several core components that facilitate its operation and enhance its processing power.
Core Components
Core components of GPUs include the Graphics Processing Clusters (GPCs), Streaming Multiprocessors (SMs), memory interfaces, and the framebuffer. Each of these elements plays a distinct role in how graphics and computations are processed. The parallel processing ability of GPUs stems from the architecture that allows numerous operations to be performed concurrently.
This architecture is particularly beneficial for tasks involving large datasets, as it provides scalability and efficient processing.
A unique feature of these core components is the high number of individual cores available in a GPU compared to a CPU. While a CPU might have between 4 to 16 cores, GPUs can have hundreds or even thousands of smaller cores. This characteristic allows for exceptional performance in executing multiple threads simultaneously, thus making it an advantageous choice for parallel task execution in various fields such as data processing and gaming.
Threading and Parallelism
Threading and parallelism are integral to GPU functionality. The ability to execute several threads at once significantly accelerates processing tasks. Unlike traditional serial processing models found in CPUs, the threading model in GPUs supports massive parallelism. This means thousands of threads can operate independently, working on different data segments.
The key characteristic of threading in GPUs is the fine-grained parallelism that enables simultaneous execution. This parallelism is a beneficial aspect for computationally intensive tasks as it increases throughput and reduces latency.
A notable feature of the threading model is its efficient management of context switching between threads, which allows for smooth operation in complex algorithms requiring high computational power. This mechanism is advantageous because it minimizes the performance dip that can occur when switching between task contexts in a serial model.
Differences between CPUs and GPUs
Understanding the differences between CPUs and GPUs can provide essential insights for optimizing programming approaches. GPUs and CPUs have distinct processing models that make them suitable for different tasks.
Processing Model
The processing model of GPUs contrasts sharply with that of CPUs. CPUs are optimized for low-latency task execution and handle a few instruction threads but can switch between them very quickly. In contrast, GPUs excel in processing large volumes of data with relatively simpler tasks. This model prioritizes throughput over latency, resulting in enhanced performance for applications requiring heavy data manipulation like simulation, rendering, and more.
One unique aspect of GPU processing is its SIMD (Single Instruction, Multiple Data) architecture. This architecture allows identical operations to be carried out on multiple data points simultaneously, further boosting performance. However, due to this architecture, GPUs are not always the best fit for tasks requiring complex logic and branching.
Use Cases
Use cases for GPUs typically include machine learning, video rendering, and scientific simulations. The choice to utilize a GPU over a CPU often stems from the need to handle vast quantities of data efficiently, such as in deep learning applications.
The versatility in use cases is one of the primary reasons for the popularity of GPUs. However, it is essential to note that while GPUs excel in parallel processing, for certain tasks involving intricate decision trees, CPUs may perform better due to their adeptness with control-intensive programs.
Core Principles of Parallel Computing
Understanding the core principles of parallel computing is essential for anyone venturing into GPU programming. GPUs are designed to handle multiple operations simultaneously by utilizing a parallel processing approach. This method significantly enhances computational efficiency, particularly in tasks demanding high performance. The fundamental concepts in parallel computing include data parallelism and task parallelism, both of which serve distinct roles in optimizing workloads and maximizing resource use. An appreciation of these principles will empower developers to write more effective code, ultimately improving application performance.
Fundamentals of Parallelism
Data Parallelism
Data parallelism refers to the technique where the same operation is applied to multiple data elements simultaneously. This is a key characteristic of modern GPU architecture, which allows efficient processing of large datasets. For example, operations on image pixels, mathematical computations, or even machine learning tasks can benefit immensely from this approach.
One distinguishing feature of data parallelism is its ability to process data in parallel by breaking it into smaller chunks. This ensures that while one set of data is being processed, another can be queued up for the next cycle. The advantages of data parallelism include significantly reduced processing time and the capacity to handle immense datasets, making it a favored choice for tasks in fields like data science and AI.
However, data parallelism does come with its challenges. A notable disadvantage lies in its reliance on uniformity in the data. If the data varies significantly in complexity or size, it may lead to inefficiencies or underutilization of resources.
Task Parallelism
Task parallelism, on the other hand, emphasizes the execution of different tasks concurrently. Each task may involve distinct computations that can be independently executed. This approach allows for more flexible program designs and is particularly valuable in scenarios involving diverse operations that do not depend on one another.
A defining characteristic of task parallelism is its focus on multiple tasks running at the same time rather than the same task over different data. This can result in better resource utilization when different operations can exploit parallel execution. Developers often choose task parallelism for its versatility. It allows various parts of an application to be optimized individually, potentially leading to an overall increase in performance.
Nevertheless, task parallelism also has its pitfalls. Coordinating several independent tasks can lead to increased overhead, especially if there is a need for communication or synchronization between those tasks. This overhead can sometimes negate the performance benefits that parallelism aims to deliver.
Synchronization and Data Sharing
In implementing parallel computing models, synchronization and data sharing become critical components. As multiple threads or tasks operate simultaneously, establishing order and control over their interactions is essential. It helps in maintaining data integrity and preventing race conditions. Effective synchronization mechanisms, such as barriers and locks, are crucial in ensuring that tasks do not interfere with each other, thereby facilitating a smooth execution flow in GPU programming.
Programming Languages for GPU Development
Programming languages for GPU development play a crucial role in harnessing the power of Graphics Processing Units. They enable developers to write code that effectively utilizes parallel processing capabilities intrinsic to GPUs. The choice of language impacts performance, compatibility, and the ability to efficiently manipulate hardware features. Understanding the landscape of GPU programming languages is essential, as it allows developers to select the right tools that suit their project needs.
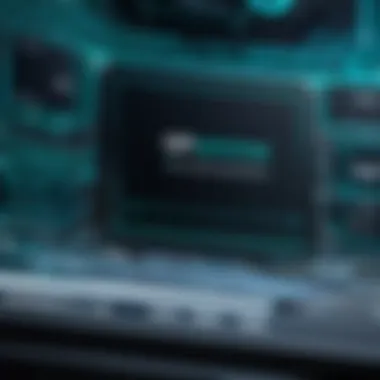
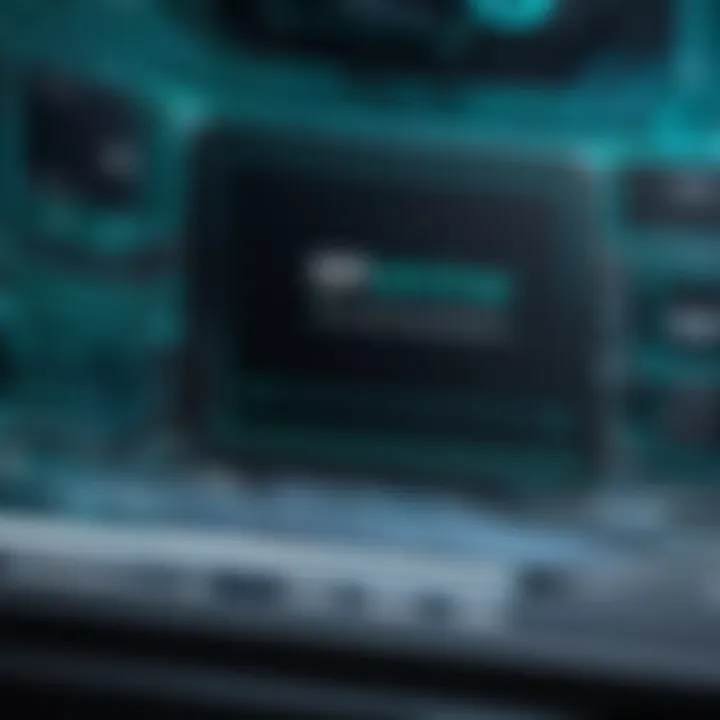
CUDA: The Industry Standard
CUDA, developed by NVIDIA, stands as the industry standard for GPU programming. Its significance lies in its ability to offer direct access to the GPU's virtual instruction set and parallel computational elements. This allows developers to leverage the GPU's architecture for intensive computing tasks effectively. Key benefits of CUDA include efficient memory usage and a vast ecosystem built around it, including libraries and community support.
CUDA enables performance optimization through features like Unified Memory, which simplifies memory management across CPU and GPU. The ease of use and extensive documentation make it a favorable choice for both beginners and experienced developers. By adopting CUDA, programmers can maximize the potential of NVIDIA GPUs for diverse applications ranging from machine learning to scientific simulations.
OpenCL: A Cross-Platform Option
OpenCL presents an alternative to CUDA, as it is not limited to NVIDIA hardware. It allows developers to write programs that execute across heterogeneous platforms, including CPUs, GPUs, and even FPGAs. This cross-platform capability is one of OpenCL's standout features, making it suitable for applications requiring compatibility across different hardware setups.
Another advantage is the flexibility that OpenCL provides in terms of language choice. Developers can integrate OpenCL with several programming languages, such as C or C++. Despite its broader applicability, OpenCL tends to have a steeper learning curve compared to CUDA. This makes it essential for users to have a good understanding of parallel programming concepts to effectively utilize OpenCL's capabilities.
Other Notable Languages
DirectCompute
DirectCompute is a part of Microsoft’s DirectX API. It allows developers to harness the computational power of GPUs for tasks beyond graphics rendering, especially in game development. A key characteristic of DirectCompute is its ability to provide a familiar environment for those already working with DirectX.
This compatibility feature makes it a beneficial choice for developers focused on gaming applications. DirectCompute enables various functionalities like GPGPU (General-Purpose computing on Graphics Processing Units), allowing for real-time data processing. However, its limitations include a dependency on Windows platforms, which may restrict cross-platform deployment.
Vulkan
Vulkan stands out due to its low-level access, giving developers more control over how they manage GPU resources. This feature enhances performance and enables real-time graphics rendering. Its key characteristic is the explicit control over memory and synchronization which can lead to more optimized applications.
Vulkan's versatility is another strength. It can be used for both graphics and compute workloads, making it popular in industries such as gaming and scientific simulations. However, the steep learning curve might present challenges for newcomers to GPU programming. Proper knowledge of the underlying hardware is necessary to fully leverage Vulkan's capabilities.
In summary, understanding programming languages for GPU development is essential for leveraging the massive computational power of GPUs effectively. Each language, from CUDA to Vulkan, has its unique strengths and considerations, influencing the choice of programming based on project requirements.
Frameworks and Tools for GPU Programming
Frameworks and tools for GPU programming play a crucial role in harnessing the computational power of Graphics Processing Units. They simplify the development process, providing essential libraries and APIs that abstract the complexities of GPU architecture. These frameworks allow developers to focus on algorithm design rather than low-level hardware details. This topic is particularly relevant as the demand for intensive computations, such as those in machine learning and scientific research, continues to grow.
Popular GPU Frameworks
TensorFlow
TensorFlow stands out as a robust framework specifically designed for deep learning applications. Its flexibility and strong community support contribute to its popularity in academia and industry alike. One of TensorFlow's key characteristics is its ability to perform efficient computations using data flow graphs, allowing for complex mathematical operations to be expressed as a series of nodes and connections.
A unique feature of TensorFlow is its eager execution mode, which allows for immediate evaluation of operations and makes it easier for developers to debug their models. However, while TensorFlow is powerful, it also comes with a steeper learning curve for newcomers. Additionally, managing large-scale models can become complicated if not handled properly.
PyTorch
PyTorch is another prominent framework that has gained traction in both research and production. Its primary focus is on enabling dynamic computation graphs, meaning that the structure of the network can change with each iteration. This feature greatly enhances its usability and speeds up experimentation, making it an attractive choice for researchers.
The key characteristic of PyTorch is its simplicity and ease of use, especially regarding debugging and real-time computations. Its seamless integration with Python allows developers to utilize standard Python libraries directly. However, PyTorch may lack some of the advanced features found in TensorFlow for large-scale deployment, which could limit its use in industrial applications.
Development Environments
IDE Selection for GPU Programming
The selection of an Integrated Development Environment (IDE) is crucial for efficient GPU programming. A well-chosen IDE can enhance productivity by providing features such as syntax highlighting, code completion, and debugging capabilities tailored for GPU languages. Popular choices include Visual Studio, JetBrains CLion, and Eclipse.
What sets an effective IDE for GPU programming apart is its support for GPU-specific plugins and efficient handling of large code libraries. This can significantly speed up the development workflow. However, choosing the wrong IDE may result in performance issues or lead to a cumbersome user experience, thus it is wise to assess various options based on individual project needs.
Debugging Tools
Debugging tools are essential for identifying and resolving errors in GPU programs. Tools like NVIDIA Nsight and AMD CodeXL provide comprehensive debugging environments that help visualize GPU processes, memory usage, and kernel execution in real time.
The key characteristic of these debugging tools is their ability to provide insights into parallel execution, making it easier to pinpoint performance bottlenecks. A unique merit is their user-friendly interfaces which cater to both novice and expert developers. Conversely, reliance on proprietary tools may limit portability across different hardware ecosystems.
Effective use of the right frameworks, IDEs, and debugging tools can transition a developer's approach from tedious and error-prone to streamlined and productive.
Best Practices in GPU Programming
In the realm of GPU programming, understanding and implementing best practices is crucial. These practices not only enhance performance but also ensure more efficient use of resources. Developers, scientists, and engineers rely on GPUs for tasks that require high processing power. Therefore, optimizing how we program GPUs becomes paramount. The importance of this section lies in its potential to equip programmers with the skills to produce high-performing applications.
Optimizing Performance
Memory Management
Memory management is a pivotal component of GPU programming. It dictates how data is allocated, accessed, and manipulated in GPU memory. Efficient memory management facilitates faster data retrieval which contributes directly to program performance. This is essential in high-performance computing scenarios where every millisecond counts. A key characteristic of effective memory management is minimizing data transfers between the central processing unit (CPU) and the GPU. Reducing these transfers can significantly enhance the overall execution speed of applications.
Some unique aspects of memory management include:
- Shared Memory Utilization: GPUs often have a form of fast local memory. Optimizing its use can greatly decrease access times.
- Coalesced Access Patterns: Organizing memory access in a way that multiple threads access contiguous memory locations increases throughput.
However, developers must navigate potential challenges such as memory leaks and buffer overflows, which can degrade performance significantly.
Efficient Kernel Design
Efficient kernel design is another essential best practice in GPU programming. This involves structuring the computational routine that runs on the GPU to utilize its parallel architecture effectively. Kernels should be designed in a way that maximizes thread occupancy, leading to better utilization of the GPU’s compute resources. A crucial feature of efficient kernel design is the ability to balance workload among threads to avoid situations where some threads sit idle while others are overburdened.
Key elements of efficient kernel design include:
- Minimizing Divergence: Designing kernels to ensure that all threads of a warp execute the same instruction improves efficiency.
- Grid and Block Optimization: Properly structuring grids and blocks to fit the computational task can significantly enhance performance.
On the downside, achieving this requires a deep understanding of both hardware and software aspects, which can be challenging for new developers.
Avoiding Common Pitfalls
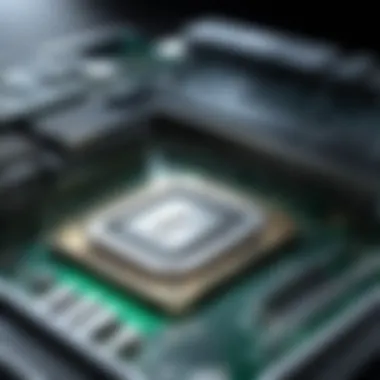
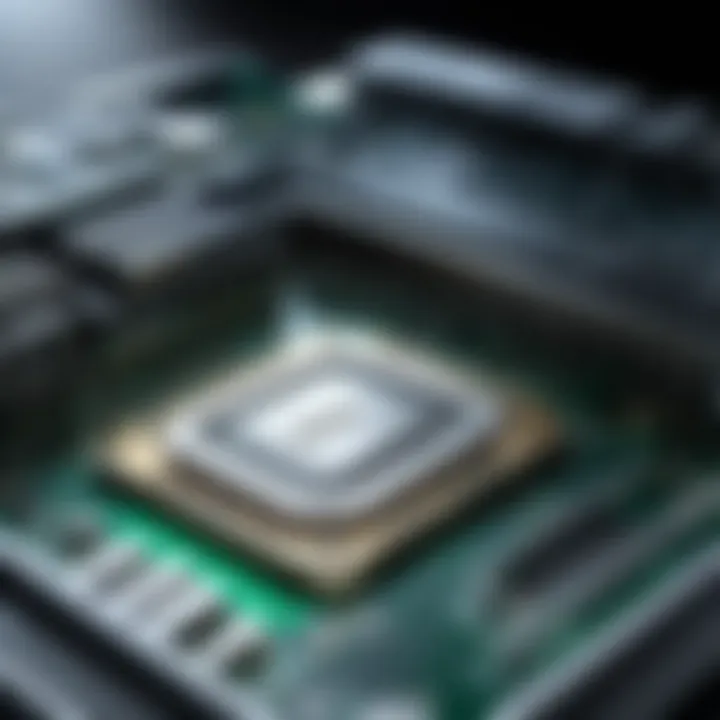
Success in GPU programming also requires awareness of common pitfalls that can hinder performance. These might include inadequate thread management, inefficient algorithm choices, and overlooking data dependencies. Ensuring clear structures and methodologies can assist in mitigating these issues. Here are several potential pitfalls to consider:
- Overutilization of Global Memory: Accessing GPU global memory frequently can cause bottlenecks.
- Neglecting Resource Limits: Not accounting for maximum thread counts can lead to unsuccessful kernel launches.
By knowing these pitfalls and strategically addressing them, programmers can significantly improve their development process and product efficiency.
Applications of GPU Programming
GPU programming is a pivotal area within the realm of computing, uniquely positioned to address the demands of increasingly complex applications. These applications harness the power of parallel processing to achieve high performance and efficiency. The significance of exploring GPU programming applications lies in understanding how to effectively leverage this technology across various fields such as machine learning, scientific computing, and computer vision. Each of these domains presents unique challenges and opportunities, underscoring the versatility and critical importance of GPUs in modern computing.
Machine Learning and AI
Machine learning and artificial intelligence (AI) are at the forefront of technological advancement today. GPUs provide the necessary computational power for training complex models. The parallel structure of GPUs allows them to execute numerous operations simultaneously, which significantly speeds up model training and enables experimentation with larger datasets. For instance, convolutional neural networks (CNNs), commonly used for image recognition, benefit immensely from GPU acceleration as they involve extensive matrix multiplications.
Commonly used frameworks such as TensorFlow and PyTorch leverage GPUs for enhanced performance. Developers can optimize their code to ensure efficient use of GPU resources, which leads to reduced training times and improved model accuracy. The integration of GPUs in machine learning pipelines not only increases throughput but also facilitates real-time processing in applications like natural language processing and recommendation systems.
Scientific Computing
The nature of scientific computing often requires extensive numerical simulations and analyses over large datasets. Traditional CPU-based processing can become a bottleneck in such scenarios. Here, GPUs shine due to their high parallel processing capabilities. Applications in fields like physics, chemistry, and bioinformatics utilize GPU computing to perform simulations that would otherwise be infeasible.
Using tools like OpenCL or CUDA, scientists can harness the massive parallel architecture of GPUs to perform computations quicker and more efficiently. A practical example is climate modeling, where researchers can run complex simulations of atmospheric conditions in a fraction of the time compared to CPU-based methods. These advancements enhance insights and foster innovations that address global challenges.
Computer Vision
Computer vision has evolved dramatically, powered significantly by GPU programming. GPUs handle the intensive processing required for image and video analysis, enabling real-time performance in applications such as facial recognition, object detection, and augmented reality. For example, deep learning models used for image classification necessitate robust computing power to analyze and learn from vast datasets of images.
In the context of computer vision, frameworks that utilize GPU support, including OpenCV and TensorFlow, allow developers to implement models that run efficiently. Additionally, the ability to process images in real-time creates new opportunities in various industries, including healthcare, automotive, and entertainment. This application of GPUs not only drives innovation but also enhances productivity in fields where visual information is paramount.
Challenges in GPU Programming
Understanding the challenges in GPU programming is crucial for developers and professionals looking to leverage GPU capabilities efficiently. Despite the vast potential GPUs offer in terms of speed and performance, there are inherent obstacles that can affect the success of a project. Addressing these challenges not only enhances the overall effectiveness of programming but also ensures that resources are utilized effectively. In this section, we will focus on scalability issues and integration with existing systems, both of which are significant factors influencing GPU programming today.
Scalability Issues
Scalability is a key concern in GPU programming. As applications grow in complexity, ensuring that they can effectively utilize multiple GPUs or scale up to meet increasing workloads becomes critical.
- Resource Management: Scaling applications often requires sophisticated resource management. This involves distributing tasks efficiently across multiple GPU units while avoiding bottlenecks.
- Performance Degradation: It is not uncommon for performance to degrade despite adding more GPUs. Effective load balancing and minimizing communication overhead are essential components to watch during scaling.
- Programming Model Limitations: Some programming models may not inherently support scalability. Developers must choose frameworks and tools that allow for easy scaling, like NVIDIA’s CUDA or OpenCL.
In many cases, addressing scalability can involve a combination of algorithm optimization and system architecture considerations. Developing a keen understanding of how algorithms behave on a larger scale is fundamental.
"Scalability is not just about adding more hardware; it is about making software that can effectively use that hardware."
Integration with Existing Systems
Incorporating GPU programming into existing architectures poses unique challenges. Many legacy systems were not designed to take full advantage of GPUs, leading to several potential barriers:
- Compatibility Issues: Older systems may have hardware or software that is incompatible with modern GPU programming languages and frameworks. This can necessitate significant updates or even complete overhauls.
- Data Transfer Limits: The speed of data transfer between CPUs and GPUs may introduce delays. Efficient data management and minimizing data movement are essential to ensure that the performance benefits of GPUs can be realized.
- Skill Gap: Often, team members might lack experience with GPU programming, leading to a steep learning curve. Companies may need to invest in training or hiring specialists to bridge this gap effectively.
Managing these integration issues is essential for a smooth transition to leveraging GPU capabilities. A strategic approach that encompasses thorough testing and incremental implementation can significantly lessen integration difficulties.
Future of GPU Programming
The landscape of GPU programming is shifting rapidly. As industries continue to demand increased computational power, understanding the future of this technology becomes crucial. GPU programming stands at the forefront of not only graphics rendering but also in fields such as artificial intelligence, data analysis, and scientific computing. The increasing complexity of tasks, coupled with the need for efficiency, will drive advancements in GPU technologies. In this article, we will discuss emerging trends and innovations in GPU programming, as well as the pivotal role GPUs will play in cloud computing.
Trends and Innovations
The pace of innovation in GPU programming is relentless. One major trend is the move towards heterogeneous computing. This term refers to the combination of different types of processors, such as CPUs and GPUs, to enhance performance. As applications become more complex, their computation might benefit from utilizing both GPU and CPU in tandem.
Another noteworthy trend is the advancement of machine learning frameworks designed specifically for GPUs. Frameworks like TensorFlow and PyTorch have made significant strides in optimizing performance on GPU architectures, enabling faster model training and inference. These innovations are essential in fields like deep learning where large datasets and complex models require substantial computational resources.
Moreover, real-time ray tracing has emerged as a cutting-edge technique in graphics rendering. This method, which mimics the physical behavior of light, has become more accessible with improved GPU architectures. As gaming and visualization software continue to demand higher fidelity graphics, GPUs capable of real-time ray tracing will become a standard in the industry.
"The future will see GPUs not merely as graphics chipsets but as pivotal components in a diverse set of computational tasks, illustrating their versatility beyond traditional applications."
Lastly, the integration of AI into GPU programming tools is another significant innovation. AI can help optimize resource allocation and manage workloads, enhancing the efficiency of GPU usage in various applications.
The Role of GPUs in Cloud Computing
With the rise of cloud computing, GPUs have found a substantial role in this domain. They support intensive processing tasks that are crucial for large-scale cloud applications. Companies like NVIDIA and Google have developed cloud computing services that utilize GPU clusters, allowing organizations to run demanding workloads without investing heavily in hardware.
The scalability of cloud services will also benefit from GPU adoption. As more businesses migrate to the cloud, the need for scalable solutions becomes apparent. GPUs provide the required computational power that can be distributed across multiple servers, ensuring that applications run efficiently.
Furthermore, the availability of GPU-optimized cloud services facilitates cost-effective solutions for startups and smaller companies. Organizations can leverage high-performance computing power without the burden of maintaining physical infrastructure, thus lowering the entry barrier for innovation.
The End
In the realm of technology, GPU programming emerges as a pivotal discipline that shapes modern computational tasks. This article has meticulously examined the various facets of GPU programming—highlighting its significance, the various programming languages, frameworks, and best practices that enhance efficiency.
Understanding GPU programming is vital for anyone looking to harness the power of parallel computing. GPUs offer remarkable capabilities in processing large volumes of data simultaneously. This is essential in fields like machine learning, scientific computing, and more.
Adopting robust programming methodologies significantly boosts optimization and productivity. The right practices lead to better performance and resource utilization. Being mindful of the common pitfalls ensures one can navigate challenges more effectively.
"Knowledge of GPU programming equips developers with the tools to tackle complex problems in innovative ways."
Overall, the insights gained reinforce the importance of continual learning and adaptation in this fast-evolving landscape. As more industries recognize the potential of GPUs, understanding their workings and capabilities becomes increasingly essential.
Summary of Key Takeaways
- Core Understanding: Grasping the architecture and differences between CPUs and GPUs is fundamental.
- Essential Tools: Familiarity with languages like CUDA and OpenCL is crucial for effective development.
- Best Practices Matter: Optimizing memory management and efficient kernel design can vastly improve performance.
- Applications are Vast: From AI to scientific research, the applications of GPU programming are extensive and impactful.
- Facing Challenges: Recognizing scalability and integration issues can save time and resources in project execution.
Next Steps in Learning GPU Programming
To advance in GPU programming, consider the following steps:
- Deepen Language Knowledge: Explore advanced features in CUDA or OpenCL to enhance coding skills.
- Hands-on Projects: Engage in real projects or open-source contributions to solidify your understanding.
- Join Communities: Participate in forums like Reddit or Facebook groups dedicated to GPU programming.
- Stay Updated: Follow innovations and trends in the field to remain relevant.
- Experiment with Frameworks: Explore frameworks like TensorFlow or PyTorch to understand their GPU capabilities better.
These steps will pave the way for in-depth knowledge and practical skills in GPU programming, ensuring you remain at the forefront of technological advancements.