Mastering Golang Development: The Definitive Guide for Coders
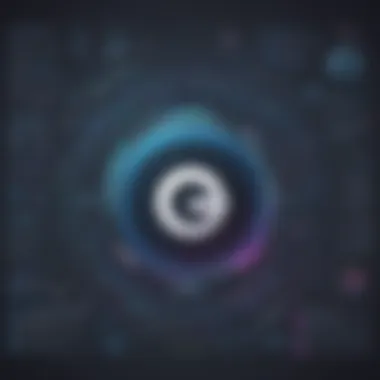
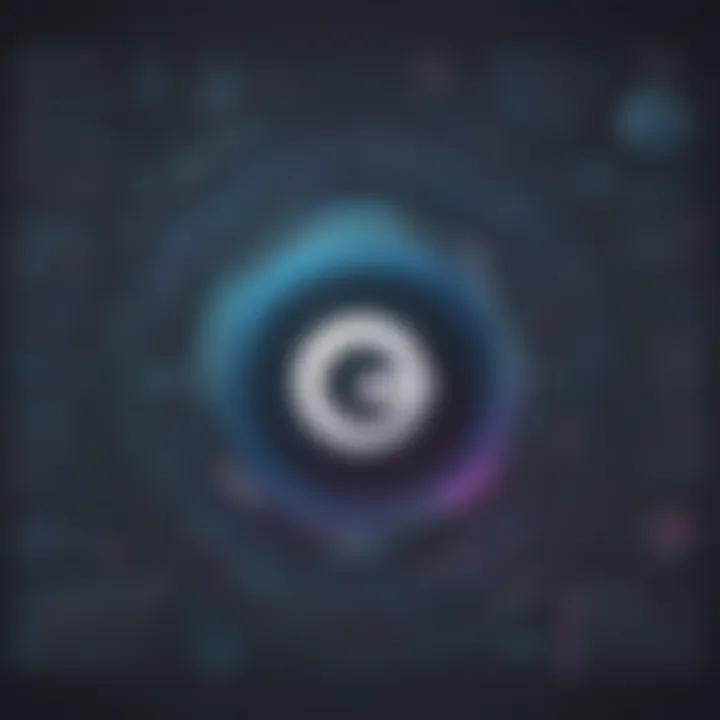
Overview of Golang Development
Golang, also known as Go, is a statically typed programming language developed by Google. It has gained popularity for its efficient compilation, concurrency support, and readability. The importance of Golang lies in its ability to handle large codebases with ease and its scalability, making it ideal for building robust and high-performance software applications. Key features of Golang include built-in support for concurrency through goroutines and channels, a simple and clean syntax, and a rich standard library. Developers often leverage these features to create fast and efficient software solutions. Golang is commonly used in web development, cloud services, network programming, and system tools. Its speed and efficiency make it suitable for building microservices and distributed systems, enhancing overall performance and scalability.
Best Practices for Golang Development
When working with Golang, it is essential to follow industry best practices to ensure code reliability and maintainability. Adopting solid testing strategies, utilizing effective error handling mechanisms, and adhering to Go's idiomatic style guidelines are crucial for developing high-quality Go programs. To maximize efficiency and productivity in Golang development, developers should leverage tools like gofmt for code formatting, profiling tools for performance optimization, and version control systems such as Git for collaborative coding. Common pitfalls to avoid in Golang development include excessive mutability, neglecting error checking, and inefficient resource management. By addressing these issues proactively, developers can enhance the quality of their Golang projects.
Case Studies in Golang Development
Several companies have successfully implemented Golang in their projects, showcasing its versatility and effectiveness. For example, Uber utilizes Golang for its microservices architecture, improving the performance and scalability of its platform. The lessons learned from such implementations emphasize the importance of leveraging Golang's strengths for specific use cases. Industry experts highlight the benefits of Golang in enhancing developer productivity, simplifying complex problems, and enabling seamless collaboration on large-scale projects. Their insights provide valuable perspectives on the practical applications of Golang in real-world scenarios.
Latest Trends and Updates in Golang
The Golang community continues to evolve, with ongoing advancements shaping the future of Golang development. Emerging trends include increased adoption of Golang in cloud-native environments, improvements in tooling for dependency management, and enhancements in the performance of the Golang runtime. Current industry forecasts project sustained growth in Golang usage, driven by its efficiency in building containerized applications, serverless functions, and distributed systems. Innovations such as improved compiler speed and enhanced module support further solidify Golang's position as a leading programming language.
How-To Guides and Tutorials for Golang Development
Navigating the intricacies of Golang development can be simplified with comprehensive how-to guides and tutorials. Beginners can benefit from step-by-step instructions on setting up a Golang environment, writing basic programs, and understanding core language features. Advanced users, on the other hand, can explore hands-on tutorials for building scalable web applications, implementing concurrency patterns, and optimizing performance in Golang. Practical tips and tricks for effective Golang utilization include utilizing interfaces for code extensibility, profiling application performance for bottlenecks, and integrating third-party packages for additional functionality. By following these guides, developers can enhance their Golang proficiency and unlock new possibilities in software development.
Introduction to Golang
In the realm of programming languages, Golang stands out as a robust and efficient option for developers. This section serves as the gateway into understanding the essence of Golang and its significance in modern software development landscapes. Delving into Golang's core principles and design objectives, developers can grasp why Golang has gained traction and popularity in the tech community. Exploring the foundational concepts of Golang sets the stage for a deep dive into its various applications and benefits.
What is Golang?
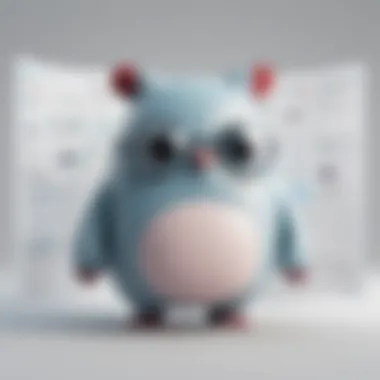
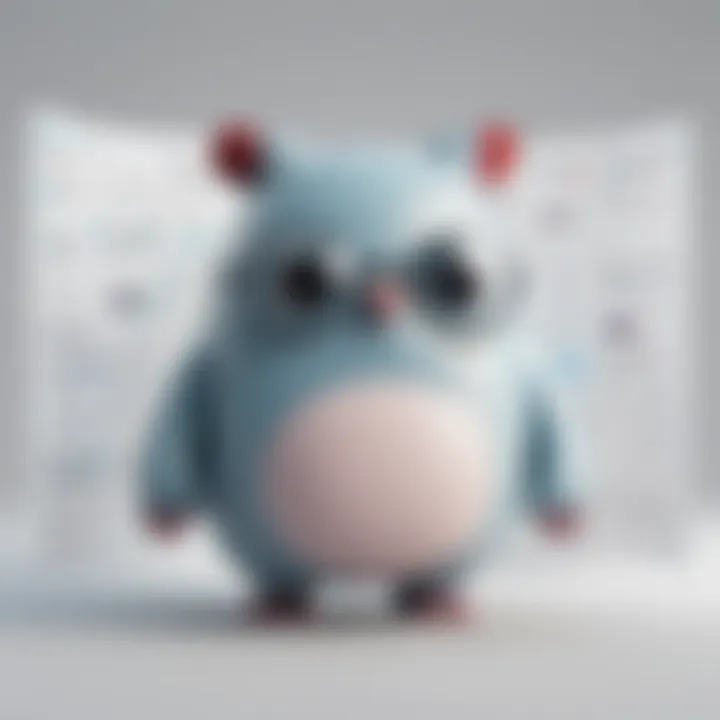
Golang, also known as Go, is an open-source programming language developed by Google. Launched in 2009, Golang was created with a focus on simplicity, efficiency, and concurrency. Its syntax is minimalistic yet powerful, making it easy to learn and use for developers across different skill levels. Golang is renowned for its fast compilation times and built-in support for concurrent programming, making it ideal for tasks requiring high-performance and scalability.
History of Golang
Understanding the origins of Golang provides insight into the driving forces behind its development. Golang was conceived by Google engineers Robert Griesemer, Rob Pike, and Ken Thompson as a response to the shortcomings they experienced with existing programming languages. Stemming from a need for a language that could address modern computing challenges with simplicity and efficiency, Golang was designed to eliminate unnecessary complexity and offer solutions for concurrent programming. Tracing the evolution of Golang from its inception to its current state helps developers appreciate the thoughtful design choices that underpin its functionality.
Advantages of Using Golang
Embracing Golang as a development tool brings forth a myriad of advantages for software projects. Its robust standard library, efficient compilation process, and built-in concurrency support streamline the development workflow and enhance productivity. Golang's static typing system catches errors at compile time, reducing bugs and improving code reliability. Moreover, its strong community support and growing ecosystem of packages and frameworks make it a versatile choice for diverse application domains. By harnessing these advantages, developers can unlock the full potential of Golang and leverage its features to build scalable and high-performance software solutions.
Getting Started with Golang
To truly grasp the essence of Golang development, it is imperative to embark on a journey of understanding the fundamentals. The 'Getting Started with Golang' section serves as the gateway to a world of limitless possibilities and efficient coding practices. By immersing oneself in this foundational knowledge, developers can lay a robust groundwork for their Golang projects. From setting up the development environment to writing the first Golang program and mastering basic syntax, this section acts as the stepping stone towards Golang proficiency.
Setting Up the Environment
Setting up the environment for Golang development plays a pivotal role in ensuring a smooth and seamless coding experience. Developers need to install the Golang compiler, set the appropriate environment variables, and configure their Integrated Development Environment (IDE) to support Golang coding. By meticulously configuring the environment, developers can streamline the coding process, leverage Golang's capabilities to the fullest, and eliminate any hindrances that may impede their progress.
Writing Your First Golang Program
Crafting the first Golang program marks a significant milestone in a developer's journey towards mastering the language. With a focus on simplicity and functionality, beginners can experiment with basic syntax, variable declarations, and control flow structures. By initiating their coding journey with a foundational program, developers can gain hands-on experience, understand the core principles of Golang, and lay a solid framework for building more complex applications in the future.
Understanding Basic Syntax
Understanding the basic syntax of Golang is essential for developers to effectively communicate their coding instructions to the compiler. From declaring variables to implementing functions and control structures, mastering the basic syntax enables developers to write concise, readable, and efficient code. By delving deep into the intricacies of Golang syntax, developers can unlock the language's full potential, enhance code maintainability, and embody best practices in their programming endeavors.
Intermediate Golang Concepts
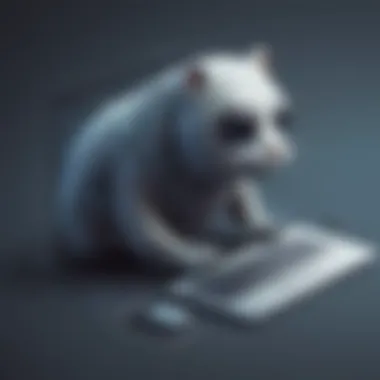
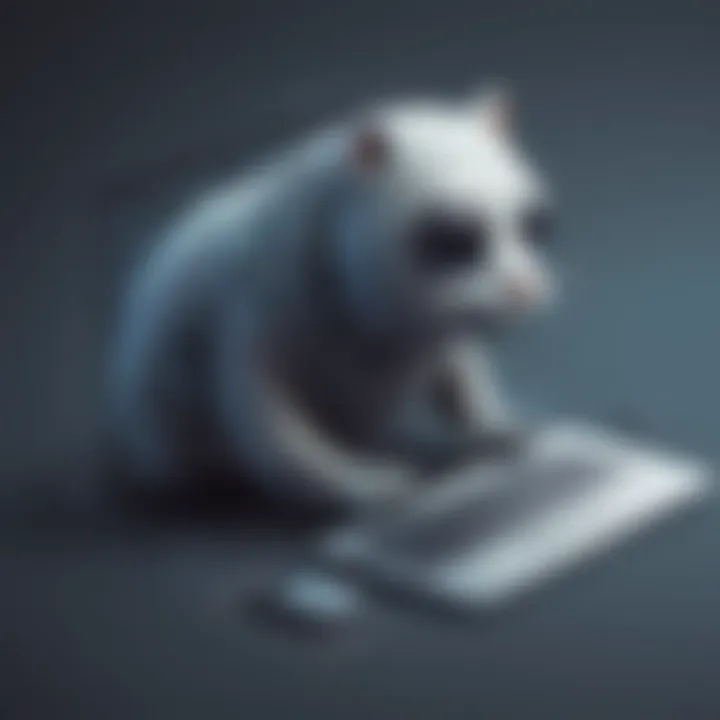
In the realm of Golang development, the subsection of Intermediate Golang Concepts holds a significant position in equipping developers with a deeper understanding of Golang's functionalities. This section bridges the gap between foundational knowledge and advanced techniques, paving the way for developers to enhance their coding expertise. By delving into Intermediate Golang Concepts, developers can explore more nuanced aspects of the language, enabling them to write more efficient and sophisticated code.
Working with Functions and Packages
Working with functions and packages in Golang is essential for structuring code in a modular and reusable manner. Functions act as building blocks of a Golang program, facilitating code organization and enhancing readability. By understanding how to work with functions effectively, developers can optimize code maintenance and promote code reusability across projects. Similarly, packages play a crucial role in Golang development by enabling code encapsulation and modularization. Leveraging packages allows developers to manage dependencies efficiently, leading to more structured and scalable codebases.
Error Handling in Golang
Error handling is a fundamental aspect of Golang development that ensures the reliability and robustness of software applications. Proper error handling mechanisms help developers identify and address potential issues within their code, enhancing the overall stability of Golang programs. By mastering error handling in Golang, developers can write more fault-tolerant code and provide better user experience through informative error messages. Understanding common error scenarios and implementing effective error handling strategies is paramount for ensuring the resilience of Golang applications.
Concurrency in Golang
Concurrency lies at the core of Golang's design philosophy, making it a powerful language for building scalable and efficient applications. Golang's concurrency model, based on goroutines and channels, offers developers a streamlined approach to handling parallel tasks and managing concurrent operations. By exploring concurrency in Golang, developers can leverage the language's built-in mechanisms to create responsive and high-performance applications. Understanding how to effectively implement and synchronize concurrent processes enables developers to maximize the utilization of system resources and optimize application performance.
Advanced Golang Techniques
In the domain of Golang development, mastering advanced techniques is paramount for developers aiming to optimize their coding efficiencies and elevate the performance of their applications. This section delves deep into the intricacies of advanced Golang Techniques, shedding light on the nuances that can make a significant difference in development projects. By understanding and implementing these techniques, developers can streamline their code, enhance scalability, and improve the overall robustness of their applications.
Optimizing Performance
Optimizing performance in Golang applications is a critical aspect that directly impacts user experience and operational efficiency. By meticulously fine-tuning code, leveraging efficient data structures, and implementing best practices, developers can significantly enhance the speed and responsiveness of their applications. This subsection explores various optimization strategies in Golang, ranging from algorithmic optimizations to memory management techniques, equipping developers with the knowledge needed to create high-performing applications.
Using Interfaces and Reflection
Interfaces and reflection are powerful features in Golang that enable developers to write flexible and extensible code. By leveraging interfaces, developers can define a set of method signatures that a type must implement, promoting code reusability and modularity. Reflection, on the other hand, allows for the examination of a type's structure at runtime, enabling dynamic type introspection and manipulation. This subsection dives into the nuances of using interfaces and reflection in Golang, illustrating how these features can simplify complex code logic and facilitate seamless integration with external systems.
Building Web Applications with Golang
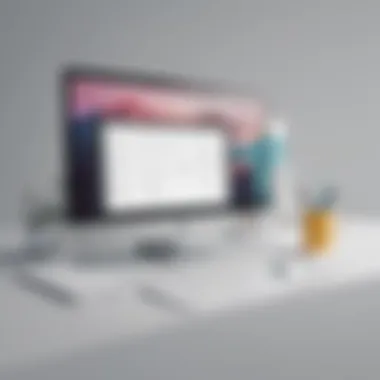
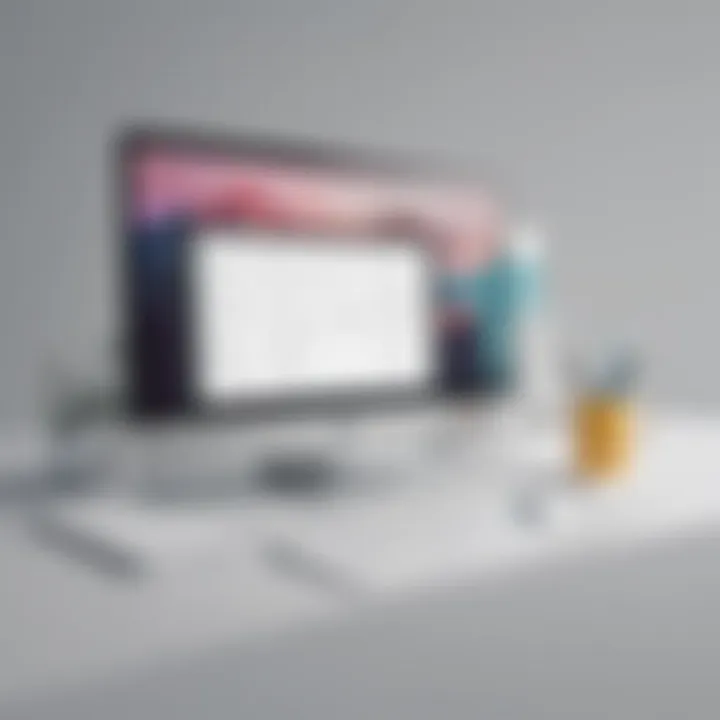
Building web applications with Golang showcases the language's versatility and efficiency in handling concurrent requests and processing HTTP operations. Golang's standard library offers robust packages for routing, serving HTTP requests, and implementing middleware, providing developers with a solid foundation for building scalable web applications. This subsection guides developers through the process of architecting and deploying web applications in Golang, highlighting best practices for creating performant and secure web solutions that meet modern development standards.
Best Practices and Tips
In the realm of Golang development, embracing best practices and tips is paramount for achieving optimal coding outcomes. This pivotal section delves deep into the fundamental significance of adhering to best practices and offers invaluable insights for developers keen on honing their craft. By meticulously following best practices, developers can streamline their code, enhance overall project efficiency, and foster a standardized approach to software development - thus facilitating seamless collaboration among team members. An emphasis on consistency, readability, and maintainability underscores the essence of incorporating best practices, ultimately elevating code quality and mitigating potential errors. Furthermore, integrating best practices ensures scalability and future-proofing of projects, empowering developers to navigate complex coding scenarios with finesse.
Code Optimization Strategies
Efficient code optimization strategies serve as the cornerstone of proficient Golang development, enabling developers to enhance performance, scalability, and resource utilization within their applications. By employing agile and strategic optimization techniques, developers can fine-tune their codebase, mitigate bottlenecks, and improve overall system efficiency. Leveraging tools such as profiling and benchmarking, developers can meticulously analyze code performance, identify areas for enhancement, and implement targeted optimization measures to boost execution speed and minimize resource consumption. Moreover, adopting modular and organized coding practices facilitates optimization efforts, allowing for seamless code refactoring and performance enhancements without compromising functionality. Incorporating optimization strategies proactively not only reinforces code quality but also reinforces developers' ability to deliver robust and high-performing applications.
Effective Debugging Techniques
Navigating the intricate landscape of Golang development demands a profound understanding of effective debugging techniques to swiftly identify, isolate, and rectify software bugs and anomalies. This section delves into the pivotal role of debugging in the software development lifecycle, emphasizing the importance of systematic debugging processes in ensuring code reliability and stability. By employing systematic debugging methodologies, developers can expedite troubleshooting procedures, pinpoint root causes of issues, and expedite bug resolution - thereby minimizing downtime and optimizing software performance. Leveraging tools such as integrated development environments (IDEs), debuggers, and logging utilities empowers developers to streamline the debugging workflow, facilitate error tracking, and enhance code maintainability. Cultivating a strategic approach to debugging not only enhances code quality but also instills a culture of efficiency and precision within the development environment.
Documentation and Testing
Effective documentation and rigorous testing protocols form the bedrock of robust Golang development, underscoring the significance of maintaining comprehensive documentation and executing thorough testing procedures throughout the software development lifecycle. Thorough documentation serves as a vital communication tool, enabling developers to convey critical information about the system architecture, design decisions, and codebase functionalities, fostering clarity and transparency within development teams. Embracing a systematic approach to documentation ensures project sustainability, facilitates knowledge transfer, and mitigates technical debt by encapsulating vital project insights and guidelines. In parallel, diligent testing practices, including unit testing, integration testing, and continuous integrationcontinuous deployment (CICD), are instrumental in validating code functionality, identifying defects, and ensuring software robustness. By incorporating robust documentation practices and rigorous testing frameworks, developers can fortify code reliability, accelerate development cycles, and deliver superior, bug-free applications that meet stringent quality standards.
Golang Ecosystem
The Golang Ecosystem is a critical aspect of this comprehensive guide for Golang development. It serves as the backbone that supports Golang developers in building robust and efficient software solutions. Within this ecosystem, developers can access a plethora of tools, libraries, and resources that enhance their productivity and streamline the development process. By delving into the Golang Ecosystem, developers gain insights into best practices, patterns, and architectures that can significantly impact the quality and performance of their code.
Popular Libraries and Frameworks
Popular Libraries and Frameworks play a pivotal role in shaping the Golang development landscape. These pre-built modules and frameworks offer ready-to-use functionalities that expedite development processes and eliminate the need to reinvent the wheel. By leveraging popular libraries such as Gin, Gorilla, and Viper, developers can enhance scalability, improve code maintainability, and accelerate project delivery. Frameworks like Echo and Beego provide architectural guidance and help in structuring Golang applications for better organization and scalability.
Community Support and Resources
Community Support and Resources constitute a valuable asset for Golang developers seeking collaboration, learning opportunities, and networking. The Golang community is vibrant and inclusive, offering forums, meetups, and online platforms where developers can share knowledge, seek help, and stay updated on the latest trends and practices in the Golang ecosystem. Leveraging community resources such as GitHub repositories, Slack channels, and online forums enables developers to tap into a wealth of collective expertise, gain insights from peers, and contribute to the growth of the Golang community.
Future Trends in Golang Development
Exploring Future Trends in Golang Development unveils exciting possibilities and innovations that promise to shape the future of Golang programming. Emerging trends such as cloud-native development, serverless computing, and microservices architecture are revolutionizing the way Golang applications are designed, developed, and deployed. By staying abreast of these trends and technologies, developers can future-proof their skills, stay competitive in the job market, and align their career paths with the evolving demands of the industry.