Mastering Flask with Python 2: Installation to Integration
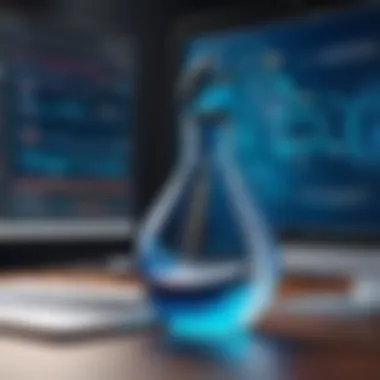
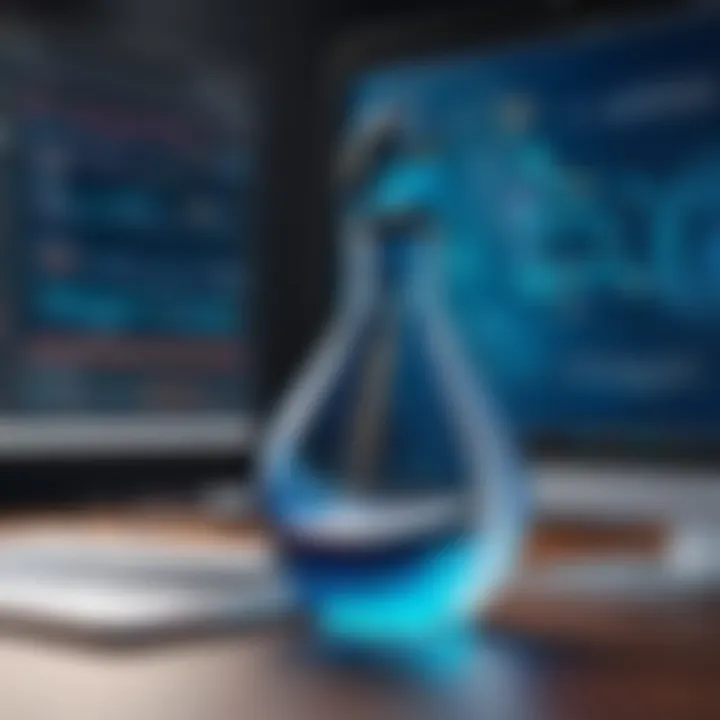
Intro
Flask, a lightweight web framework for Python, emerges as a compelling choice for developers looking to build web applications quickly and efficiently. In a world where application responsiveness and scalability are paramount, understanding how to utilize Flask within the confines of Python 2 becomes increasingly relevant. This guide aims to provide a thorough exploration of Flask's integration with Python 2, delving into installation, configuration, routing, template rendering, form handling, and integration with extensions. Developers and tech enthusiasts will find practical insights, ease of implementation, and maintenance pathways that will enrich their development experience.
Overview of Flask and Python
Definition and Importance of Flask and Python
Flask is a microframework for Python, designed to allow easy extensions and minimal boilerplate code. Although Python 2 has been largely superseded by Python 3, many legacy systems still operate on it. This guide will highlight how Flask, when applied within a Python 2 environment, can still deliver value, particularly for maintaining older applications without a complete overhaul.
Key Features and Functionalities
Flask offers numerous features that amplify its appeal:
- Simplicity: Its design promotes simplicity, making it a great choice for smaller applications.
- Flexibility: Flask does not impose a rigid project structure, allowing developers to dictate their architecture.
- Extension Support: A large array of extensions is available to add functionality, ranging from database integration to authentication.
Use Cases and Benefits
Flask's flexibility caters to various use cases, especially for projects demanding rapid development cycles. The benefits include:
- Quick iteration on product features.
- Ease of integration with other components or services.
- Ability to scale applications from small to large.
Best Practices
Industry Best Practices
Following best practices can greatly improve your experience with Flask in Python 2:
- Maintain modular design: Break down your application into manageable, logical components.
- Regularly update your dependencies: Use tools like Pip to manage libraries and extensions effectively.
Tips for Maximizing Efficiency and Productivity
- Use Flask's debugging features during development to catch errors early.
- Employ Blueprints for structuring your application, which promotes organization.
Common Pitfalls to Avoid
When working with Flask and Python 2, be cautious of:
- Neglecting compatibility issues with libraries designed for Python 3.
- Overlooking security vulnerabilities in extensions or custom code.
How-To Guides and Tutorials
Step-by-Step Guides for Using Flask with Python
- Setup: Install Flask using pip. You can use the following command:
- Configuration: Set up your application configuration using an file, where you declare settings such as database URLs and secret keys.
Hands-On Tutorials for Beginners
For those new to Flask, a simple project can be to create a basic web page. You might start with:
This sets up a minimal web application responding to the root URL.
Practical Tips and Tricks for Effective Utilization
- Frequently check Flask documentation for the latest best practices and functionalities.
- Leverage community forums, such as Reddit, for support and advice from experienced developers.
Flask empowers developers with flexibility and simplicity, even when utilizing older versions of Python.
End
By following the insights and methodologies discussed, developers can harness the power of Flask, ensuring the continued success and relevance of their web applications.
Prolusion to Python and Flask
In the realm of programming, understanding older technologies can sometimes seem counterintuitive. However, Python 2 continues to have significant value in certain contexts. It is essential to recognize that Python 2 serves as the foundation for many legacy systems. For software developers and IT professionals, this context is crucial when integrating Flask, a popular web framework, into projects using Python 2.
Significance of Python in Modern Development
Although Python 2 has officially reached its end of life, it remains a tool frequently used in existing software projects. Many applications run on this version, requiring maintenance and updates. Noting that it contributes to codebases that many companies still rely on, understanding its nuances can be vital for developers.
The importance of Python 2 in modern development lies in:
- Maintenance of Legacy Systems: Many companies have significant investments that rely on Python 2. Learning the intricacies enables developers to support and upgrade these systems.
- Migration Challenges: Transitioning from Python 2 to Python 3 poses challenges. Knowledge of both versions creates a smoother migration pathway.
- Community Resources: Many resources are still available, bolstering best practices with Python 2 development.
Overview of Flask as a Web Framework
Flask is a micro web framework for Python that is lightweight and flexible. This minimalistic approach allows developers to add only what they need, but it still maintains the capability for larger applications. Flask's advantages include:
- Simplicity: Its easy-to-understand premises make it an attractive choice for web development, particularly for those familiar with Python.
- Extensibility: With Flask, developers can expand the framework's functionality through extensions, adapting it according to project requirements.
- Built-in Development Server: This feature allows immediate testing of applications in a controlled setting, facilitating rapid prototyping.
Flask's versatility makes it suitable not only for simple applications but also for more complex systems requiring scalable architecture.
Use Cases for Combining Python and Flask
Integrating Python 2 with Flask allows developers to harness the best of both worlds. The following are significant contexts where this combination shines:
- Legacy Application Enhancement: When building new features for existing applications originally coded in Python 2, Flask can provide a modern web interface without the complete overhaul.
- Rapid Prototyping: For projects needing quick iterations, combining Python 2 with Flask allows for fast deployment, making it useful in startup environments.
- Educational Purposes: Learning Flask in a Python 2 setting can help understand web development principles without the noise of complex features.
"Understanding the collaboration of legacy systems with modern frameworks ensures that developers can maintain relevance in ever-evolving technology landscapes."
In summary, the intersection of Python 2 and Flask offers unique advantages, particularly in maintaining existing applications while leveraging modern development practices.
Setting Up Your Environment
Setting up the environment for Python 2 and Flask is a critical step for developers looking to efficiently build web applications. A well-configured environment not only enhances productivity but also ensures that the application runs smoothly across different platforms and setups. It establishes a foundational structure that accommodates libraries, packages, and dependencies required for the development process. In addition, proper setup prevents common issues such as package conflicts, version disparities, and other potential technical hurdles that can arise during application development.
Prerequisites for Python Programming
Before diving into Python 2 and Flask, having a minimal set of prerequisites is essential. First and foremost, one must ensure that Python 2.7 is installed on their system. Unlike Python 3, Python 2 remains popular in legacy systems, and many existing applications are maintained on this version.
To install Python 2, you can visit the official Python website for the latest distribution compatible with your operating system.
Aside from the Python installation, it is advantageous to have a general understanding of programming concepts, especially in Python syntax and structures. Basic knowledge of web development principles, such as HTTP, RESTful APIs, and client-server architecture, will also be helpful. Another important prerequisite is choosing a suitable code editor or integrated development environment (IDE). Options such as PyCharm, Visual Studio Code, or even a simple text editor like Sublime Text can significantly improve the coding experience.
Installing Flask in a Python Environment
Installing Flask within a Python 2 environment is a straightforward process that involves the use of package managers like pip. To begin, ensure that pip is installed on the system, as it is the primary tool used for installing Python packages.
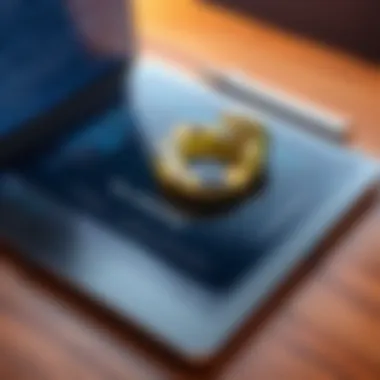
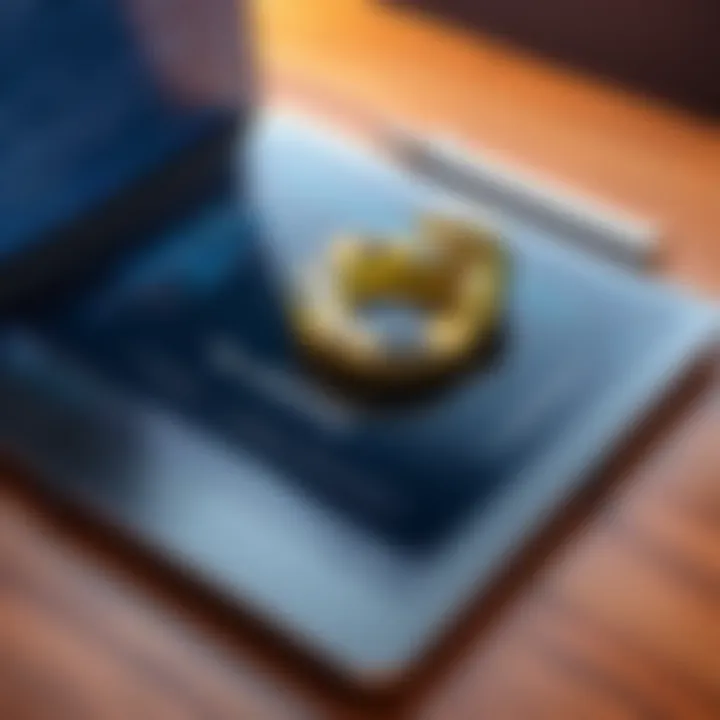
To install Flask, follow these steps:
- Open a command line interface (CLI) or terminal.
- Use the following command to install Flask:
- Validate the installation by executing:
This command should return the installed version of Flask if the installation was successful. If errors arise during installation, check if your version is up to date or look into potential issues related to your Python environment.
Configuring a Development Environment for Flask
Configuring your development environment is the next logical step after installing Flask. It involves tailoring your workspace to facilitate effective coding workflows and project management.
A recommended approach is to use virtual environments. Virtual environments allow you to create isolated spaces for each project, preventing library version conflicts. To set up a virtual environment, use the following steps:
- Install the package:
- Navigate to your project directory.
- Create a new virtual environment:
- Activate the virtual environment:
- On Windows:
- On macOS or Linux:
Once activated, your terminal will show the environment name, indicating that any package installations or configurations will apply only inside this environment. This setup is a best practice for maintaining a clean project structure.
By effectively setting up the environment, developers position themselves to leverage the full potential of Python 2 and Flask, thus paving the way for successful project development.
Creating Your First Flask Application
Creating your first Flask application is a foundational step in understanding how to leverage Flask with Python 2 effectively. Establishing a working application serves as the basis for future development. It introduces you to Flask's core concepts and allows you to grasp how the various components of the framework fit together. Moreover, getting the basics right at this stage will save you time and effort in your projects down the line.
Flask is known for its simplicity but also for its power. By creating an application, developers can immediately appreciate the benefits of a lightweight yet flexible framework. Understanding how the application is structured, how routes and endpoints function, and how request and response objects operate is essential for building efficient web applications.
This section will detail the essential elements involved in creating a basic Flask application, articulate the associated benefits, and consider important factors that may influence your approach.
Basic Application Structure
The fundamental structure of a Flask application is simple yet crucial. At its core, a Flask application consists of several key files and configurations. The basic setup typically includes:
- Application Module: This is where you define your Flask application. It typically begins with importing the Flask class and instantiating an application object.
- Routing: Each route corresponds to a specific function that handles a request to a particular endpoint. This is set up within the application module, defining how the application reacts to various web requests.
- Run the Application: Finally, you need to include logic to run your application. This is generally done at the end of the module to check if the script is executed directly.
The overall simplicity of this structure is key to understanding Flask. It allows developers to see how the framework enables a seamless flow from request to response.
Defining Routes and Endpoints
Defining routes and endpoints is where Flask truly shines. Each route corresponds to a function that handles requests sent by users. By clearly outlining the routing of your application, you create a powerful and intuitive interaction layer.
When defining a route, you use the decorator followed by a function that returns a response. Flask supports dynamic URLs, allowing you to capture variables within the route. For example:
In this example, the application dynamically returns a message based on the username captured in the URL. Efficiently designing your endpoint structure is critical for usability and maintainability. Good practices involve:
- Keeping URLs user-friendly and logical.
- Using HTTP methods appropriately (GET, POST, etc.).
- Clearly handling errors with proper status codes.
Understanding Request and Response Objects
Flask interacts with web requests and generates corresponding responses through the use of Request and Response objects. Understanding these components can significantly enhance how you handle data in your application.
The object contains data related to the client request, including parameters and form data. Accessing request data can be achieved easily:
Similarly, the object allows you to define what your application sends back to the user. It is possible to customize headers, cookies, and status codes:
By mastering the Request and Response objects, developers can build more interactive and responsive applications, ultimately leading to a better user experience.
Handling Templates in Flask
Templates play a crucial role in web development, especially when using Flask for creating web applications. In the Flask ecosystem, they serve as a bridge between backend logic and frontend UI. Proper handling of templates enhances the flexibility and maintainability of projects. This section explores various aspects of templates, focusing on their structure, dynamic content creation, and best practices for their effective use.
Intro to Jinja2 Templating Engine
Jinja2 is the default templating engine used by Flask. It allows developers to create dynamic web pages by embedding Python-like expressions directly in HTML. Jinja2 supports template inheritance, filters, and macros, providing a powerful toolkit for developers.
The core features of Jinja2 include:
- Control structures: Use loops and conditionals to dictate rendering logic based on data.
- Filters: Enhance variables with built-in functions for formatting and manipulation.
- Extensions: Extend the existing functionality to suit specific needs.
Utilizing Jinja2 effectively streamlines the development process, allowing for separation of concerns between application logic and presentation logic.
Creating Dynamic HTML Pages
In modern web applications, static HTML pages are often insufficient. They lack interactivity and personalization that users expect. Using Jinja2, developers can dynamically generate HTML content tailored to the end-user's requests.
Creating dynamic pages typically involves:
- Rendering templates: Using the function from Flask to link Python code with the Jinja2 template.
- Passing variables: Sending data from the backend to the template allows for personalized experiences.
- Conditions and loops: Implementing Jinja2 constructs to display different content based on user input or application state.
For example, to render a template with variables:
This snippet renders , using specified variables to customize the content.
Template Inheritance and Extensions
Template inheritance promotes code reusability and organization. It allows developers to create a base template which contains common elements such as headers, footers, and navigation bars. Child templates can inherit from this base, overriding specific blocks when necessary.
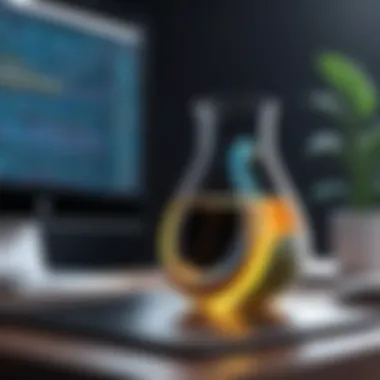
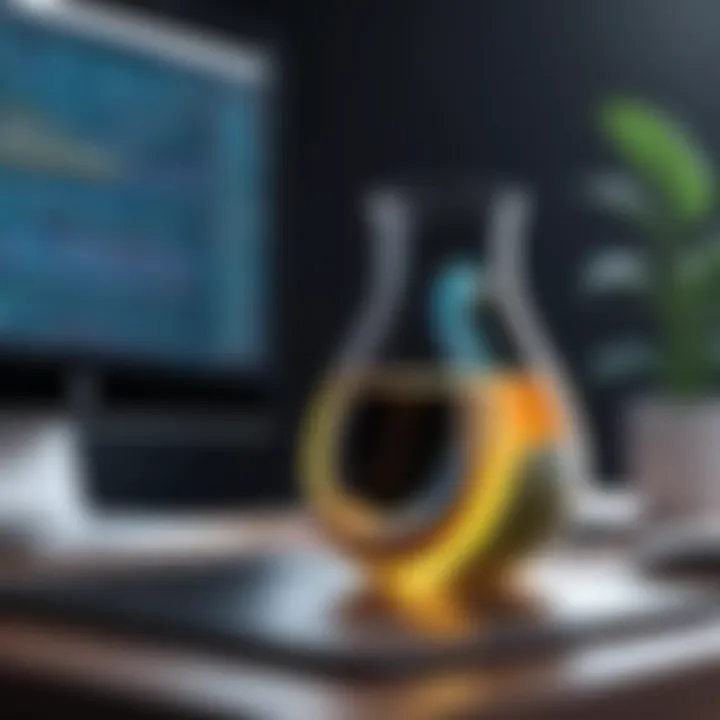
The benefits of using template inheritance include:
- Consistency: Share common layout across multiple pages.
- Maintainability: Changes made to the base template automatically reflect in all inheriting templates.
- Efficiency: Reduces code duplication, leading to cleaner and more manageable code.
Additionally, Jinja2 provides the possibility to create extensions. Custom extensions allow developers to introduce new template tags, filters, or preprocess data before rendering.
In summary, handling templates in Flask optimizes web development by facilitating dynamic content management and promoting clean architecture. The integration of Jinja2 not only simplifies the development process but also greatly enhances the user's experience on the web.
Working with Forms
In any web application, forms serve as a critical interface for user interaction. They collect input, provide a method for user authentication, and enable various interactions such as data submission, comments, or file uploads. Their importance cannot be overstated, especially in the context of Flask applications built on Python 2. Navigating forms effectively allows developers to harness user inputs while maintaining data integrity and application functionality.
Creating and Handling Forms in Flask
Creating forms in Flask involves using the Flask-WTF extension, which simplifies the process of generating and validating forms. This extension integrates nicely with Jinja2 templating, allowing the dynamic rendering of forms in HTML. By defining forms in a Pythonic way, it becomes easier to handle user inputs.
To create a form, start by importing the required modules. A basic form class may look like this:
This simple form captures a userās name. When integrating this form into a Flask route, you'll handle both rendering the form and processing submissions. Upon submission, Flask evaluates the input and determines its validity according to the specified validators. Efficient handling of forms can greatly enhance user experience and interface reliability.
Form Validation Techniques
Validation is essential in any form handling process. It ensures that the data submitted by the user meets specific criteria before processing. Flask-WTF offers built-in validators that reduce development time and improve reliability.
For instance, you can use validators to check for required fields, formats, or custom conditions. Here's a short list of common validation techniques:
- DataRequired: Confirms that a field is not submitted empty.
- Email: Validates that the submitted input follows the email format.
- Length: Ensures that input meets minimum and maximum length requirements.
An example of using validators in a form class:
This approach not only enhances the dataās quality but also improves the user interface by providing immediate feedback on errors. Therefore, robust validation techniques are crucial in protecting the application and enhancing usability.
Dealing with File Uploads
Handling file uploads requires careful consideration. Forms for file submissions require different file types and size validations. Flask-WTF provides an elegant way to manage file input through FileField.
The following is a fundamental outline for handling file uploads:
- Creating the File Upload Field: Use FileField in your form class.
- Setting File Size Limits: Define maximum file sizes to avoid performance issues.
- Process the Upload: Write logic in your Flask route to save the uploaded file.
An example file upload form:
When processing the uploaded file in your Flask view function, ensure to validate the file type. Commonly accepted file types should be defined based on your applicationās needs. By doing this, you maintain conformity to rules and minimize potential risks to your applicationās integrity.
"Form handling is not just about data retrieval; itās about preserving user trust through effective validation and data handling mechanisms."
This approach in your Flask application must be strategic, ensuring that forms are not only functional but also integral to the user experience.
Data Management and Databases
Data management is an essential aspect of any application development process. When working with Flask and Python 2, understanding how to effectively manage data is crucial for building robust and scalable applications. The integration of data management techniques allows developers to handle user information, application state, and other necessary datasets that are integral to a functioning web application.
The use of databases provides a structured way to organize, retrieve, and manipulate data. Utilizing databases in Flask projects ensures data integrity and simplifies the complexities involved in data operations. Well-structured data management leads to better performance, easier maintenance, and greater user satisfaction.
Connecting to Databases with SQLAlchemy
SQLAlchemy acts as an Object-Relational Mapping (ORM) tool, bridging the gap between relational databases and Python data models. In Flask, SQLAlchemy allows for seamless interaction with databases such as MySQL, PostgreSQL, or SQLite. By using SQLAlchemy, developers can focus more on writing Python code rather than dealing with SQL queries directly. This abstraction streamlines the integration of databases in application workflows.
To connect to a database using SQLAlchemy, follow these steps:
- Install SQLAlchemy: Ensure you have SQLAlchemy installed in your Python environment. You can use pip to install it:
- Set Up Your Database URI: Define the connection string in your Flask application configuration:
- Initialize SQLAlchemy: Create a SQLAlchemy instance and bind it to the application:
By following these steps, you can establish a connection to your chosen database and begin to utilize SQLAlchemy's features.
Managing Database Migrations
Database migrations are necessary for maintaining the integrity of your database schema over time. As an application evolves, the underlying database structure may need changes to support new features or correct errors. Flask-Migrate, a powerful extension for Flask, simplifies the migration process.
Hereās how to manage database migrations:
- Install Flask-Migrate: Add Flask-Migrate to your project using pip:
- Integrate with Your Application: Initialize the migration component in your Flask app:
- Create Migration Scripts: Generate migration files by running:
- Apply Migrations: Finally, update the database schema with:
These steps ensure that your database schema evolves along with your application, reducing the risk of errors as features change.
Implementing CRUD Operations
CRUD operationsāCreate, Read, Update, and Deleteāform the backbone of data management in applications. Implementing these operations using Flask and SQLAlchemy allows developers to perform essential data manipulation tasks efficiently and effectively.
To implement CRUD operations, consider the following functions:
- Create: Insert new records into the database. A simple example:
- Read: Retrieve existing records. For instance, fetching the first record:
- Update: Modify existing records based on certain criteria:
- Delete: Remove records from the database:
Through these CRUD operations, developers maintain data flow effectively, ensuring a robust application experience for users.
Optimizing Your Flask Application
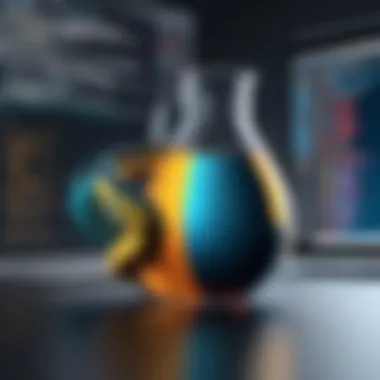
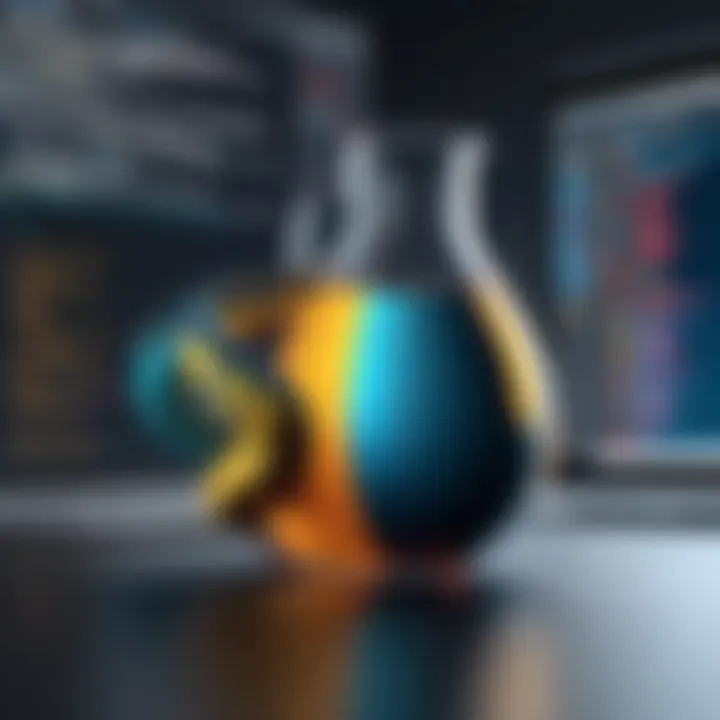
Optimizing your Flask application is essential for enhancing user experience and ensuring long-term sustainability. In the context of Python 2, specific considerations enable developers to maximize performance while navigating the constraints of an older programming environment. This topic not only addresses efficiency but also encompasses aspects such as scalability and security. Without optimization, applications risk becoming slow, unresponsive, and vulnerable to various threats. Thus, understanding the nuances of optimization can provide a significant advantage in applications that utilize Flask with Python 2.
Performance Considerations
Performance is a critical aspect for any web application. Flask provides a solid foundation, but certain practices can bolster its effectiveness. Here are the key performance considerations to keep in mind:
- Minimize Middleware: Each layer of middleware adds overhead. Use only what is necessary.
- Optimize Database Queries: Use SQLAlchemy effectively by minimizing the number of queries. Employing methods like eager loading helps prevent the N+1 query problem.
- Enable Caching: Implement Flask-Caching to store data temporarily. This reduces redundant database queries and speeds up response times.
- Static File Management: Configure your server to serve static files efficiently. Using a reverse proxy like Nginx can significantly improve loading times.
- Use Gunicorn: Running your Flask app behind Gunicorn allows for better handling of concurrent requests.
Debugging Techniques for Flask Apps
Debugging is a crucial part of the development process. Effective debugging techniques ensure that applications run smoothly and errors are quickly identified and resolved. Here are some strategies to enhance debugging in your Flask applications:
- Flask Debugger: Utilizing Flask's built-in debugger helps identify issues in a development environment. It provides detailed error reports and stack traces.
- Logging: Implement logging using Pythonās logging module. Logging errors can provide insights long after the problem has occurred.
- Use Postman or cURL: These tools are excellent for testing APIs and routes independently of the web interface. They allow developers to observe how the application responds to various inputs.
- Debugging Tools: Leverage tools such as PDB (Python Debugger) to step through the code and inspect variables during execution.
Security Best Practices
Security should never be an afterthought in application development. Implementing proper security measures from the onset can protect sensitive data and maintain user trust. Here are some best practices to ensure your Flask application remains secure:
- Input Validation: Always validate user inputs. Libraries like WTForms can help manage and validate form data properly.
- Use HTTPS: Encrypt data in transit with HTTPS. This prevents Man-in-the-Middle attacks and secures user information.
- Session Management: Set up secure sessions by using Flask-Session. Always store sensitive information securely.
- Regular Updates: Even when using Python 2, it is critical to apply security patches and updates to libraries and dependencies regularly.
Regular maintenance and proactive security strategies are vital for safeguarding your application from potential threats.
By paying attention to performance, debugging, and security, developers can greatly enhance their Flask applications. Each of these areas plays a significant role in ensuring that applications not only function correctly but also provide a safe and responsive experience for users.
Extending Flask Functionality
Extending the functionality of Flask is a key concern for any developer seeking to create a robust application. Flask, being a micro-framework, is designed to be lightweight and flexible. However, this also means it does not come with many features out of the box. This is where Flask extensions become essential. They allow for the addition of various functionalities without the need for heavy lifting or complicated configurations, giving developers the power to customize their applications according to specific needs.
Benefits of Using Flask Extensions:
- Modularity: Extensions enable developers to add specific features modularly, which helps maintain a clean codebase.
- Ease of Use: Integrating an extension is often straightforward, requiring minimal code changes. This allows developers to focus on building functionalities rather than worrying about underlying complexities.
- Community Support: Many popular extensions are widely used and supported by the community, providing access to a wealth of documentation and resources.
In this section, we will look closely at how to utilize Flask extensions effectively, explore API development using Flask-RESTful, and discuss strategies for implementing authentication and authorization in your applications.
Using Flask Extensions
Flask extensions are add-ons that enhance the framework's capabilities. There are numerous extensions available that cater to various requirements such as database integration, user authentication, form management, and more. For instance, the Flask-SQLAlchemy extension simplifies database operations and makes it easier to use SQLAlchemy with Flask applications.
To install a Flask extension, you usually use the following command:
Replace with the name of the desired extension.
It is important to note that while extensions add functionality, they also require developers to manage additional dependencies and compatibility issues. Ensuring that all installed extensions work well together is crucial for maintaining application stability.
API Development with Flask-RESTful
Flask-RESTful is a powerful extension that simplifies the process of building RESTful APIs with Flask. This extension allows developers to define API resources easily and provides built-in support for common tasks such as handling HTTP requests and formatting responses.
One key feature of Flask-RESTful is the class, which serves as a base for all API resources. Hereās a brief example of creating a simple API with Flask-RESTful:
This code snippet demonstrates how easily you can create an API endpoint using Flask-RESTful. The integration of such an extension vastly accelerates the development process, allowing for quick iterations and testing.
Authentication and Authorization Strategies
Implementing secure authentication and authorization mechanisms is crucial for any application. Flask provides various extensions, such as Flask-Login, which simplify user session management.
Using Flask-Login, developers can manage user sessions that ensure users are authenticated before accessing specific routes. This is vital for protecting sensitive data and operations.
Some key concepts to consider include:
- Session Management: Securely track user sessions and validate logged-in users.
- Role-Based Access Control: Define what actions specific roles can perform within the application, enhancing security measures.
- Token-Based Authentication: Use tokens instead of cookies for session management, suitable for modern API-based applications.
For example, the following code snippet showcases a simple implementation of user authentication:
In this example, developers can easily leverage Flask-Login to ensure that users are authenticated before accessing protected routes. By focusing on these security strategies, developers can protect their applications effectively.
Through the use of extensions, Flask not only elevates the development experience but also bridges the gap between simplicity and feature-rich applications. This balance is key when working with Python 2 and Flask.
Challenges and Considerations
When working with Python 2 and Flask, a thorough understanding of the underlying challenges is essential for developers. This section will explore critical components that every developer should consider, ranging from the inherent learning difficulties to the implications of using an outdated language.
Learning Curve for New Developers
For new developers, diving into Flask using Python 2 can introduce a steep learning curve. The dual challenge arises because Flask is a micro-framework that expects some prior knowledge of web development concepts. Beginners must familiarize themselves with Flask's unique stylistic features, along with the syntax of Python 2, which differs significantly from Python 3.
New developers often struggle with Flask's routing and templating. Grasping these essentials requires an understanding of how HTTP requests work, along with basic concepts of web servers and client-server architecture. The following factors contribute to the learning curve:
- Python 2 Limitations: Python 2 lacks some modern conveniences found in Python 3. The absence of built-in features means that developers might need to implement workarounds for functionalities readily available in Python 3.
- Documentation and Resources: While Flask has comprehensive documentation, much of the community support leans towards Python 3 versions. Thus, new learners may find it harder to find relevant examples and guidance.
Dealing with Python End of Life
Python 2 reached its end of life on January 1, 2020. This cessation means there will be no further updates or support, thereby directly affecting applications developed in Python 2. Organizations using Flask with Python 2 need to confront several considerations:
- Security Risks: Without ongoing updates, applications are more vulnerable to security threats. New vulnerabilities might arise, exposing critical weaknesses in systems.
- Compatibility Issues: External libraries and frameworks are increasingly dropping support for Python 2. Thus, utilizing packages not designed for Python 2 could lead to integration difficulties.
It is crucial for development teams to discuss strategies and decide if they should remain on Python 2 or migrate to Python 3.
Migration Strategies to Python
Migrating from Python 2 to Python 3 can seem daunting but it is often necessary. By employing some systematic strategies, developers can ensure a smoother transition. Here are some suggestions:
- Code Review and Refactoring: Assess existing code for compatibility with Python 3. Consider using tools like 2to3 that aid in converting Python 2 code to Python 3 code.
- Gradual Migration: Instead of a complete overhaul, implement a gradual migration strategy. This approach may include running both Python versionsānoting that Flask supports code split.
- Testing and Validation: After migration, conduct thorough testing. Use unit tests to ensure that the application behavior remains consistent across both versions.
Key Insight: Seeking community input is fundamental during this process. Engaging with developer forums or platforms such as Reddit can provide significant assistance during the migration.
End
The conclusion is a pivotal segment of this article, summing up the rich insights gathered throughout the discussion on integrating Python 2 with Flask. It encapsulates the importance of Python 2, not just as a legacy language but as a utility tool for certain developers. While acknowledging the gradual shift toward Python 3, itās essential to underline that Python 2 still has relevance in various legacy systems.
Summarizing Key Takeaways
In our exploration, some key takeaways emerge:
- Compatibility: Understanding the nuances between Python 2 and Python 3 is vital, especially for developers maintaining or upgrading older systems.
- Flaskās Versatility: Flask stands as a lightweight, flexible framework suitable for various projects, allowing for rapid development and deployment.
- Community and Resources: There remains a robust community and resource pool for Python 2 and Flask, making it easier for developers to seek help or improve their skills. This includes documentation and forums typically found on sites like Reddit and Wikipedia.
- Best Practices: Emphasizing code efficiency and the need to follow best practices when developing with Flask ensures maintainable applications.
Reminding developers that every project might have distinct requirements, thus necessitating careful choice of tools.
Future of Flask and Python Development
Looking forward, the landscape of Python developmentāespecially with Flaskāpromises to be dynamic. The ultimate transition from Python 2 to Python 3 reveals an important lesson; leveraging modern tools improves security and introduces new features. Future considerations include:
- Continuous Evolution: Frameworks like Flask are continually updated to accommodate modern architectures, such as microservices and serverless computing.
- Integration with New Technologies: Developers must stay aware of emerging technologies, particularly in data science and machine learning, that are increasingly becoming mainstream. This can offer richer features to applications built using Flask.
- Migration Pathways: As the years pass, gradual migration strategies from Python 2 to Python 3 could become less daunting due to improved tools and automated converters becoming standard practice.
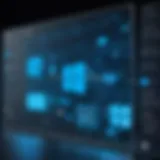
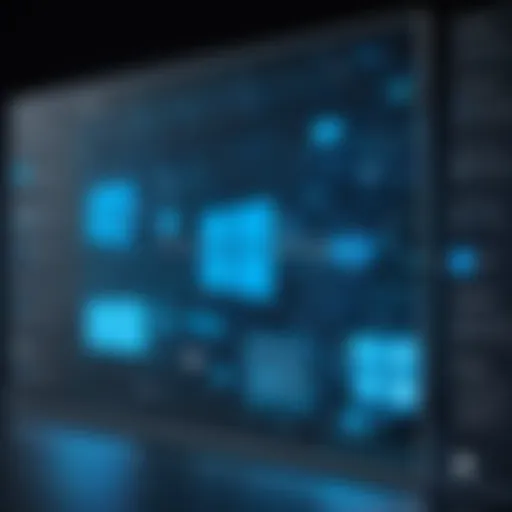