Comprehensive Guide for Beginners: Flask Fundamentals and Practical Examples
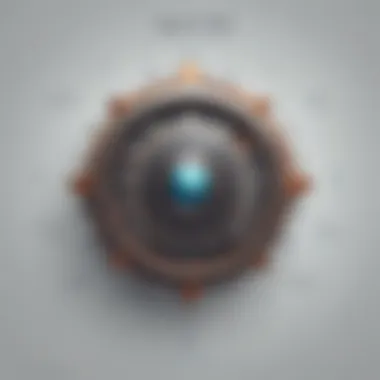
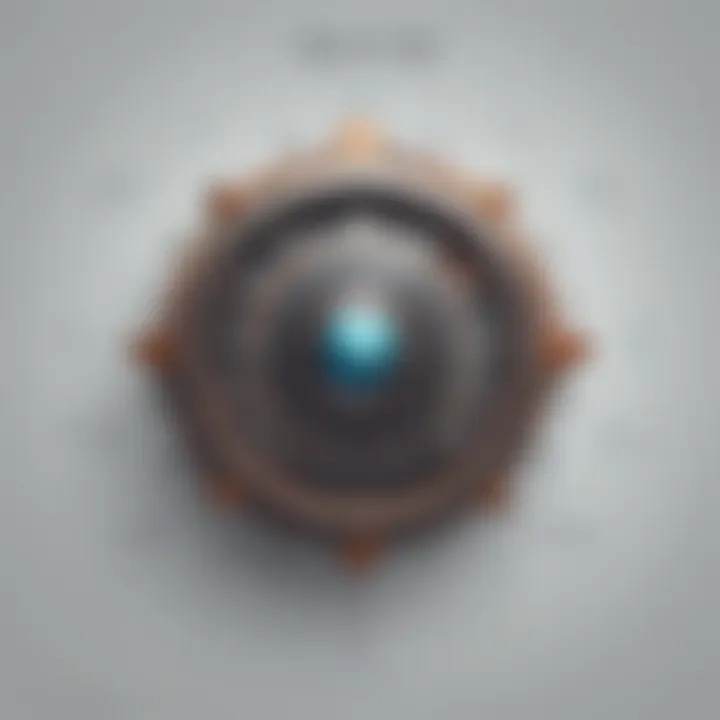
Flask, a widely used Python web framework, is an excellent starting point for beginners venturing into web development. In this comprehensive guide, we will delve into the fundamentals, installation process, basic concepts, and practical examples that are essential for embarking on your Flask development journey.
Overview of Flask
Flask is a lightweight and versatile web framework written in Python. Its simplicity and extensibility make it a popular choice for developing web applications. With Flask, developers have the flexibility to build scalable and secure web solutions efficiently.
Key Features and Functionalities of Flask
- Minimalistic design
- Built-in development server
- Support for unit testing
- Jinja2 templating
Use Cases and Benefits of Using Flask Flask is ideal for building prototypes, web applications, RESTful APIs, and more. Its lightweight nature and extensive documentation enhance developer productivity and allow seamless integration with other technologies.
Best Practices for Flask Development
When working with Flask, it is essential to follow industry best practices to ensure code quality and application scalability.
Industry Best Practices
- Structuring Flask applications using blueprints
- Implementing security measures such as encryption and authentication
Tips for Maximizing Efficiency
- Utilizing Flask extensions for enhanced functionality
- Writing clean and maintainable code to promote readability
Common Pitfalls to Avoid
- Overcomplicating application architecture
- Failing to handle exceptions and errors effectively
How-To Guides and Tutorials
In this section, we will provide step-by-step guides and tutorials to help beginners navigate their Flask development journey effectively.
Practical Tips and Tricks
- Setting up a virtual environment for Flask projects
- Creating basic routes and views in Flask
- Implementing CRUD operations with Flask and SQLAlchemy
Hands-On Tutorials for Beginners
- Building a simple web application using Flask
- Integrating Flask with a database for data storage
Overall, this detailed guide aims to equip beginners with the knowledge and practical skills needed to kickstart their Flask development experience. By following the information presented here, aspiring developers can gain a solid foundation in Flask and begin their journey towards creating robust web applications.
Introduction to Flask
Flask, a Python web framework, serves as the gateway for beginners into the world of web development. Understanding Flask is crucial for anyone venturing into web application creation using Python. The significance of mastering Flask lies in its simplicity, flexibility, and scalability. By grasping the basics of Flask, beginners can establish a strong foundation for creating dynamic web applications. Dive into Flask to elevate your programming skills and enhance your knowledge in web development.
What is Flask?
Overview of Flask
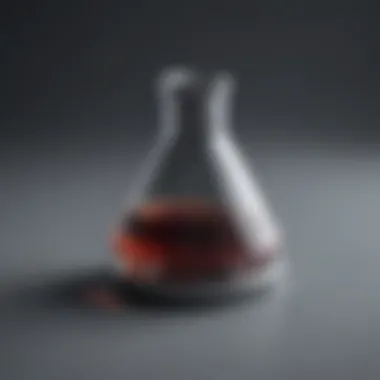
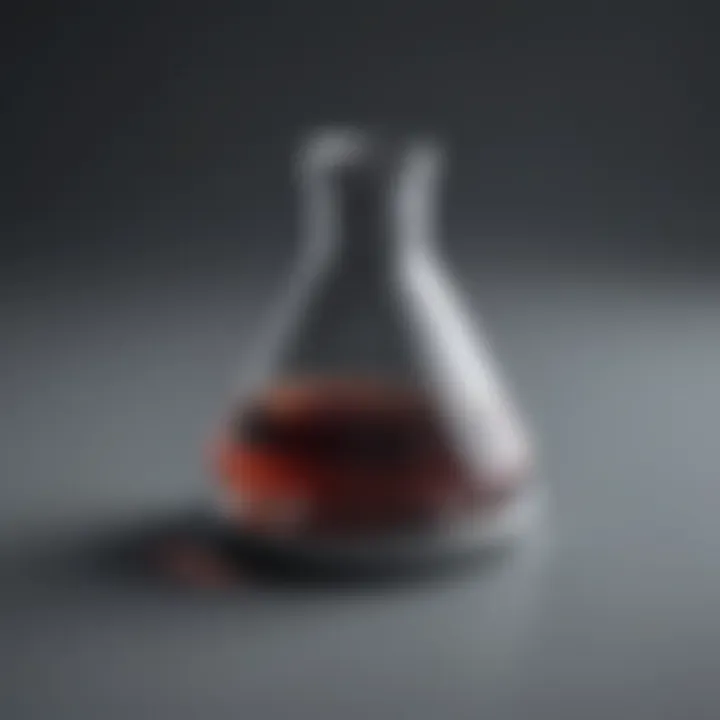
Flask stands out for its minimalistic design and ease of use. Unlike other bulky frameworks, Flask provides a lightweight and efficient solution for web development projects. Its simplicity allows beginners to grasp the fundamental concepts of web frameworks without being overwhelmed. The concise structure of Flask aids in rapid development and prototyping, making it an ideal choice for projects of all sizes.
Features and Benefits
The key features of Flask include its built-in development server, integrated support for unit testing, and Jinja2 templating engine. These features contribute to Flask's popularity among developers seeking a straightforward yet robust web framework. The benefits of Flask encompass its extensive documentation, active community support, and seamless integration with other libraries. Leveraging Flask's features allows developers to focus on coding logic rather than framework complexities, resulting in enhanced productivity and streamlined development processes.
Why Choose Flask?
Lightweight and Modular
Flask's lightweight nature and modular design enable developers to build customized solutions tailored to their specific requirements. The modular structure of Flask permits the integration of only necessary components, reducing bloat and enhancing performance. Its lightweight architecture ensures speedy execution of web applications, making Flask a preferred choice for projects where efficiency is paramount.
Flexible and Easy to Use
One of Flask's standout features is its flexibility, which grants developers the freedom to implement solutions in a manner that best suits their project needs. The simplicity of Flask's syntax makes it accessible to developers of varying skill levels, allowing beginners to quickly adapt to its conventions. Flask's ease of use extends to its intuitive API, extensive documentation, and straightforward configuration options, ensuring a hassle-free development experience.
Understanding Web Frameworks
Role of Web Frameworks
Web frameworks serve as the foundation for web application development, offering a structured approach to building and organizing code. The primary role of web frameworks like Flask is to provide essential tools and libraries for handling web-related tasks efficiently. By utilizing a web framework, developers can streamline development processes, adhere to best practices, and ensure scalability and maintainability in their projects.
Comparison with Other Frameworks
When comparing Flask with other web frameworks, its minimalist approach and modular design set it apart. While frameworks like Django prioritize convention over configuration, Flask empowers developers with more flexibility in implementing solutions. Flask's lightweight nature allows for quick project setup and minimal boilerplate code, making it a favorable choice for developers looking to create customized web applications with increased control over the development process.
Setting Up Flask
Setting up Flask is a crucial step in our comprehensive guide for beginners. This section focuses on the initial setup process, which forms the foundation for all Flask projects. By properly setting up Flask, developers ensure a smooth and efficient development experience. Ensuring the correct installation and configuration of Flask allows for seamless project creation and execution to follow. The intricacies of setting up Flask may seem daunting at first, but they are essential for an optimal development environment.
Installing Flask
Using pip
Installing Flask using pip is a common and efficient method for adding this web framework to your Python environment. Pip, Python's package installer, simplifies the installation process by automatically managing dependencies and ensuring a smooth setup. Utilizing pip for Flask installation streamlines the process and guarantees that the latest version is readily available. This approach saves time and effort for developers, promoting a more straightforward and standardized Flask setup.
Virtual environments
Virtual environments play a vital role in isolating project dependencies and preventing conflicts within different projects. By creating a virtual environment for your Flask project, you can maintain project-specific dependencies without interfering with the system-wide Python setup. This approach not only enhances project organization but also enables seamless collaboration and deployment. Embracing virtual environments in Flask development enhances project stability and reliability.
Project Structure
Organizing your Flask project
Organizing your Flask project effectively is key to maintaining a structured and manageable codebase. By structuring your project logically, you can improve code readability, scalability, and maintainability. A well-organized Flask project ensures that different components are appropriately separated and easily navigable. This approach simplifies debugging, testing, and overall project maintenance. Implementing a robust project structure from the start establishes a solid foundation for future project growth.
Creating essential files
Creating essential files, such as configuration files, initialization scripts, and module files, is a fundamental aspect of Flask project setup. These files are the building blocks of your Flask application and define its behavior, settings, and functionality. By creating these files thoughtfully and strategically, you can streamline development workflows and enhance code consistency. Well-defined essential files are critical for laying the groundwork for a successful Flask project.
Dependencies and Extensions
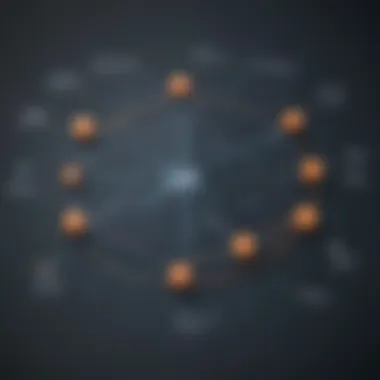
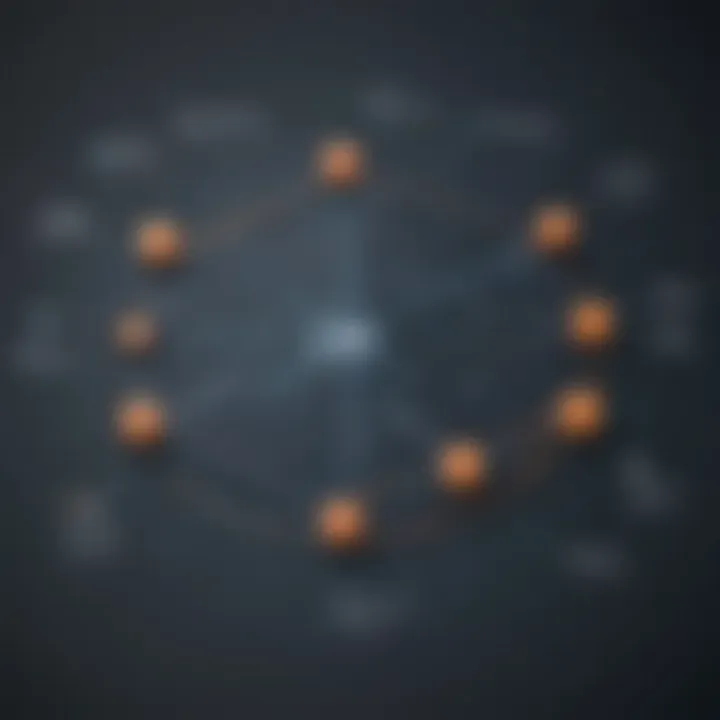
Exploring Flask extensions
Flask extensions offer additional functionalities and features that extend the core capabilities of the Flask framework. By exploring various Flask extensions, developers can enhance their projects with ready-made solutions for common requirements. These extensions cover diverse areas such as database integration, authentication, and form handling, saving developers time and effort. Leveraging Flask extensions empowers developers to build robust and feature-rich applications more efficiently.
Managing project dependencies
Effective management of project dependencies is essential for ensuring project stability and consistency. By managing dependencies carefully, developers can track and control the libraries and packages used in their Flask projects. This meticulous approach minimizes compatibility issues, security vulnerabilities, and version conflicts. Implementing a reliable dependency management strategy optimizes project performance and facilitates seamless collaboration throughout the development lifecycle.
Basic Concepts in Flask
In the realm of Flask, understanding the basic concepts serves as the foundation upon which developers construct their web applications. These fundamental principles delve into the core functionalities of Flask, elucidating the mechanisms behind routing, views, and user interactions. Grasping these concepts is pivotal for beginners as it sets the stage for more advanced development processes. By comprehending the essence of routing, views, templates, and forms in Flask, developers can streamline their coding endeavors and create efficient web solutions.
Routing and Views
Mapping URLs to Functions
In Flask's domain, mapping URLs to functions holds a paramount position in directing incoming web requests to their respective handler functions. This vital task ensures that each URL endpoint triggers the appropriate Python function, thereby enabling seamless navigation throughout the web application. The meticulous allocation of URLs to functions enhances the application's user experience by providing clear pathways for resource access. The versatility of Flask in defining these mappings empowers developers to design intricate routing systems tailored to their project's specific needs.
Handling HTTP Requests
Handling HTTP requests within a Flask application involves the intricate management of client-server interactions, ensuring the seamless exchange of data between the user interface and the server. By adeptly managing HTTP requests, developers can control how their application responds to user inputs, processes form submissions, and integrates dynamic content. Flask's adept handling of HTTP requests streamlines the development process, enabling developers to create responsive and interactive web applications efficiently. Though the process can be complex, Flask simplifies it by providing intuitive tools and methods, making it a favored choice for developers seeking efficiency in web development endeavors.
Templates and Static Files
Creating Dynamic Web Pages
The functionality of Flask extends to creating dynamically generated web pages that adapt to user inputs and database queries in real-time. By employing dynamic templates, developers can craft flexible and interactive web interfaces that cater to diverse user interactions. This dynamic capability not only enhances the user experience but also simplifies the maintenance of web content, allowing for effortless updates and modifications. Flask's adeptness in creating dynamic web pages empowers developers to build engaging and responsive websites that resonate with modern user expectations.
Serving Static Content
The process of serving static content in Flask involves delivering CSS, Java Script, and image files to enhance the visual and interactive elements of a web application. By efficiently serving static files, developers can optimize the loading speed of their web pages, leading to a smoother browsing experience for users. Flask's seamless integration of static content management simplifies the deployment process, enabling developers to focus on enhancing the aesthetic and functional aspects of their web applications. This strategic handling of static content sets Flask apart as a web framework that prioritizes optimal performance and user engagement.
Forms and User Input
Building Interactive Forms
Interactive forms play a crucial role in engaging users and collecting essential data for web applications. In Flask, developers can create dynamic forms that validate user inputs, handle submissions, and adhere to specific data requirements. By employing interactive forms, developers can enhance the interactivity of their web applications, enabling users to provide feedback, input information, and engage with the platform efficiently. Flask's robust form handling capabilities simplify the development process, empowering developers to create seamless user interfaces that facilitate smooth data exchange.
Processing Form Data
Processing form data in Flask involves validating and manipulating user inputs to extract meaningful information for database interactions or application workflows. By meticulously processing form data, developers can ensure the accuracy and security of data transmission within their web applications. Flask's robust data processing tools equip developers with the resources necessary to handle user inputs effectively, mitigating potential errors and optimizing the user experience. The precision and efficiency of form data processing in Flask contribute to the framework's appeal among developers seeking reliable solutions for data management within web applications.
Working with Data in Flask
In the realm of Flask development, working with data plays a pivotal role in ensuring the functionality and effectiveness of your web applications. Understanding how to integrate and manipulate data within Flask is crucial for creating dynamic and interactive user experiences. By delving into the nuances of data handling, developers gain the ability to store, retrieve, and process information efficiently, enriching the overall user interaction. Working with data in Flask empowers developers to craft sophisticated applications that cater to diverse user needs and preferences, setting the foundation for robust and scalable web solutions.
Database Integration
Connecting to Databases
Connecting to databases stands as a fundamental aspect of data management within Flask. This process enables applications to establish a connection with a database system, facilitating seamless data retrieval and storage operations. By configuring Flask to interact with databases, developers can leverage the inherent advantages of structured data storage, ensuring data consistency and reliability. The act of connecting to databases in Flask empowers developers to harness the full potential of database management systems, allowing for efficient query execution and manipulation of data elements. This integration facilitates the seamless flow of information between the application and the database, laying the groundwork for building data-driven web applications.
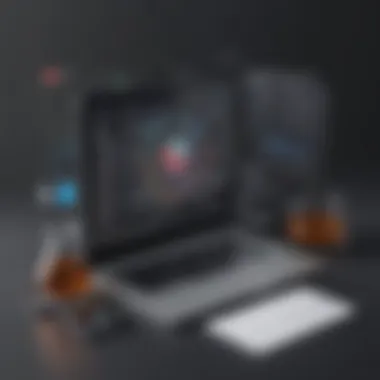
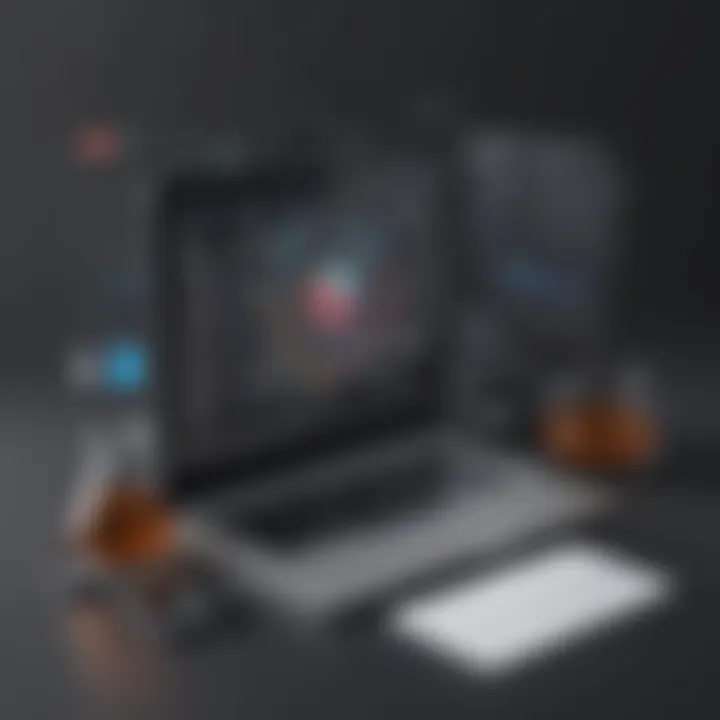
ORM Usage
Utilizing ORM (Object-Relational Mapping) in Flask streamlines the interaction between application objects and database entries, simplifying the data manipulation process. ORM usage abstracts the database operations into Pythonic objects, providing a more intuitive approach to handling data persistence. By employing ORM in Flask development, developers can focus on the application's logic and functionality without needing to delve deeply into database-specific intricacies. This abstraction layer enhances code readability and maintainability, fostering a structured approach to data management within Flask applications. Embracing ORM usage in Flask empowers developers to efficiently map Python objects to database entities, reducing the complexity of data handling and enhancing the overall development workflow.
Model-View-Controller () Pattern
Understanding Architecture
The Model-View-Controller (MVC) architectural pattern is a cornerstone of software design in Flask. Understanding the MVC architecture allows developers to separate the application logic into three interconnected components - models, views, and controllers. This segregation of concerns promotes code modularity and scalability, enhancing the maintainability and reusability of Flask applications. By grasping the essence of MVC architecture, developers gain the flexibility to modify individual components without impacting the entire application structure. This architecture fosters a systematic approach to application development, facilitating clear division of responsibilities and promoting efficient collaboration among development teams.
Separation of Concerns
The principle of Separation of Concerns in Flask emphasizes the importance of isolating distinct functionalities within the application components. By adhering to this principle, developers can segregate the application logic based on specific responsibilities, ensuring a coherent and organized codebase. Separation of concerns enhances code readability, simplifies debugging processes, and promotes code reusability across different parts of the application. Implementing a clear separation of concerns in Flask applications allows developers to focus on individual components without compromising the overall application structure, leading to enhanced maintainability and scalability.
Authentication and Authorization
User Authentication Processes
User authentication processes in Flask are paramount for securing web applications and validating user identities. Implementing robust authentication mechanisms ensures that only authorized users can access restricted resources, maintaining the confidentiality and integrity of the application data. By configuring user authentication processes in Flask, developers can authenticate users based on various credentials and establish secure user sessions. This layer of security enhances the user experience by safeguarding sensitive information and mitigating unauthorized access, bolstering the overall trustworthiness of the web application.
Role-based Access Control
Role-based access control in Flask defines and enforces user permissions based on predefined roles and access levels. By assigning specific roles to users, developers can regulate access to different parts of the application, restricting unauthorized actions and safeguarding critical functionalities. Role-based access control offers a granular level of access management, allowing developers to tailor user permissions according to specific role requirements. Employing role-based access control in Flask applications enhances security measures, fortifying the application against potential security threats and ensuring the confidentiality of sensitive data.
Advanced Topics in Flask
In the realm of Flask development, delving into Advanced Topics is a pivotal step towards mastering this Python web framework. This section aims to equip beginners with an in-depth understanding of sophisticated Flask functionalities that enhance the efficiency and scalability of web applications. Exploring Advanced Topics in Flask opens doors to implementing intricate features, optimizing performance, and adhering to industry best practices. By embracing these advanced concepts, developers can elevate their Flask projects, making them more robust, reliable, and competitive in the digital landscape.
RESTful APIs
Building API Endpoints
A cornerstone of modern web development, Building API Endpoints is crucial in enabling communication between different software applications. In this context, Flask's capability to create robust API endpoints facilitates seamless data exchange and interaction. Developers can define specific routes that handle various HTTP requests, allowing for structured data transmission and integration across diverse platforms. The flexibility and scalability of Flask's API endpoint creation empower developers to design responsive and dynamic web applications that cater to evolving user needs with ease.
Implementing CRUD Operations
Implementing CRUD (Create, Read, Update, Delete) operations in Flask is a fundamental aspect of database management and application functionality. CRUD operations form the basis of data manipulation within web applications, enabling users to interact with stored information efficiently. By incorporating CRUD operations in Flask development, developers can achieve comprehensive data management, ensuring data integrity and consistency. The implementation of CRUD operations simplifies the handling of user inputs, database queries, and data modifications, streamlining the development process and enhancing the overall user experience.
Error Handling and Logging
Managing Errors Gracefully
Effective error handling is paramount in Flask development to maintain application stability and user satisfaction. By addressing errors proactively and with precision, developers can prevent application crashes, identify root causes of issues, and provide meaningful feedback to users. Flask's approach to managing errors gracefully involves implementing robust exception handling mechanisms, logging error details, and presenting informative error messages. This ensures smoother user experiences, quicker issue resolution, and improved overall application reliability.
Logging Best Practices
Logging best practices in Flask are essential for monitoring application behavior, tracking system events, and diagnosing potential issues. By following established logging practices, developers can capture valuable insights into application performance, user interactions, and system activities. Flask's logging functionality enables developers to record critical events, errors, and warnings, facilitating efficient troubleshooting and proactive maintenance. Implementing logging best practices enhances overall application transparency, aids in debugging processes, and supports long-term application scalability.
Testing Flask Applications
Writing Test Cases
Writing test cases is a fundamental aspect of Flask application development, ensuring code quality, functionality validation, and system reliability. Test cases define expected behaviors, edge cases, and scenarios to validate the correct implementation of features and functionalities. By writing comprehensive test cases in Flask, developers can enhance code robustness, detect potential bugs early in the development cycle, and streamline the debugging process. Test cases serve as a safety net for application modifications, safeguarding against regressions and ensuring consistent performance.
Unit and Integration Testing
Unit and integration testing play a critical role in verifying the individual components and integrated modules of Flask applications. Unit tests focus on isolating and testing specific functions, methods, or classes, validating their behavior in isolation. Integration tests, on the other hand, assess the interactions and interoperability of multiple components within the application ecosystem. By integrating unit and integration testing practices in Flask development, developers can achieve comprehensive test coverage, identify integration issues early, and ensure the seamless operation of complex application structures.