Unveiling the Intricacies of Python's For Loop Syntax
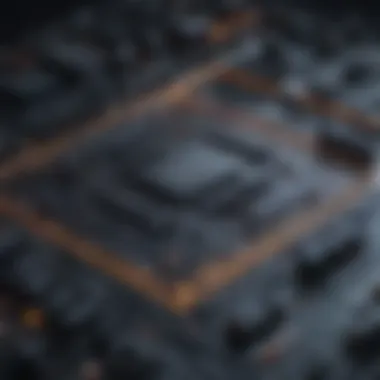
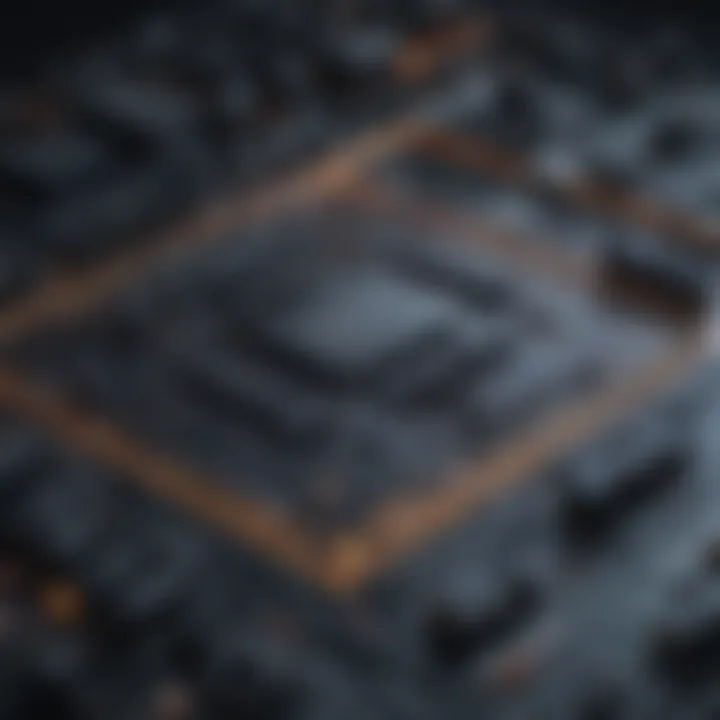
Overview of Python's For Loop Syntax
Python, a widely-used programming language known for its flexibility and readability, offers the for loop as a fundamental construct for iterating over sequences. The for loop in Python provides a concise and powerful way to iterate through elements in lists, tuples, strings, and other iterable data structures. Understanding the nuances of the for loop syntax is crucial for efficiently processing data and automating repetitive tasks in Python code. This section will delve into the intricacies of Python's for loop syntax, from its basic structure to advanced techniques, equipping readers with a robust comprehension of how to leverage this essential feature in Python programming.
Best Practices for Utilizing Python's For Loop
When working with Python's for loop, adhering to industry best practices is essential for writing clean, efficient, and maintainable code. To maximize productivity and avoid common pitfalls, developers should aim to follow certain guidelines. These include optimizing loop performance by minimizing unnecessary computations within the loop body, utilizing list comprehensions for succinct iteration, and employing meaningful variable names to enhance code readability. By incorporating these best practices, developers can streamline their programming workflow and write more robust Python code.
Real-World Examples and Case Studies
Exploring real-world examples can provide valuable insights into how the for loop is used effectively in practical scenarios. By examining successful implementations and outcomes achieved through the application of Python's for loop, readers can gain a deeper understanding of its versatility and utility in various contexts. Case studies showcasing different approaches to iteration, data processing, and algorithmic problem-solving will offer actionable takeaways and inspire innovative uses of the for loop in Python programming projects.
Emerging Trends and Future Developments
In the rapidly evolving landscape of Python development, staying informed about the latest trends and updates pertaining to the for loop syntax is crucial. By keeping abreast of upcoming advancements in iteration techniques, current industry trends, and forecasted innovations in Python programming, developers can evolve their skills and adopt cutting-edge practices. Embracing new methodologies and breakthroughs in the realm of Python's for loop syntax can pave the way for more efficient, scalable, and forward-thinking software development practices.
Practical How-To Guides and Tutorials
To assist developers of all proficiency levels in mastering Python's for loop syntax, comprehensive how-to guides and step-by-step tutorials are indispensable resources. From beginner-friendly tutorials that cover the basics of loop iteration to advanced techniques for seasoned Python developers, these practical guides offer hands-on instructions for leveraging the full potential of the for loop. By combining theoretical insights with practical tips and tricks, these tutorials empower readers to hone their Python programming skills and unlock new possibilities through the effective utilization of the for loop.
Introduction to For Loop
In the realm of programming, the for loop stands as a pivotal construct when it comes to iterating over sequences. Understanding the intricacies of this structure is crucial for any Python enthusiast looking to harness the language's full potential. From its basic syntax to more advanced techniques, navigating the for loop landscape opens up avenues of efficient and effective data processing within Python.
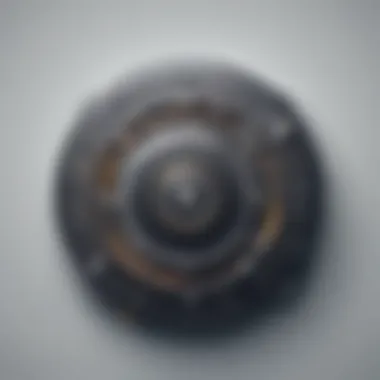
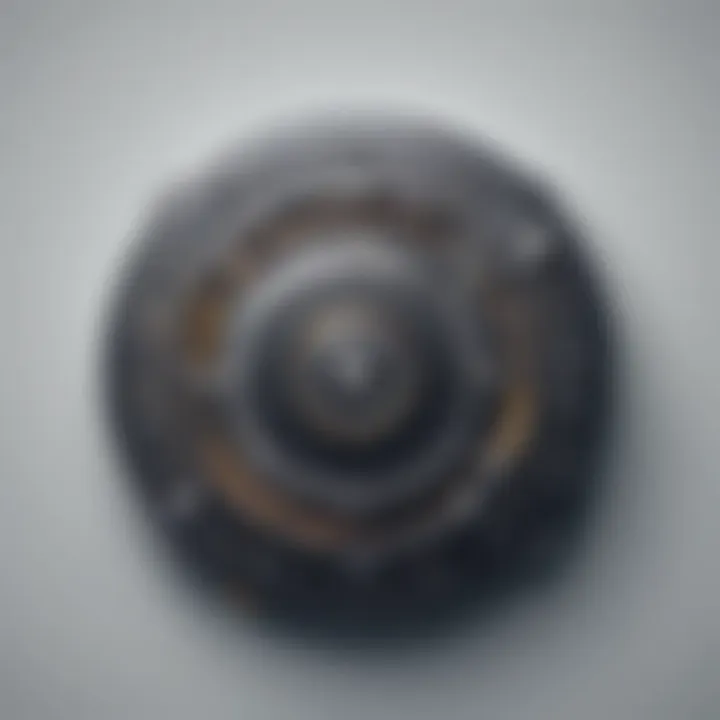
Understanding Iteration
Sequential Processing
Delving into sequential processing within the context of the for loop sheds light on its fundamental role in handling data in a step-by-step manner. Embracing sequential processing allows for a systematic approach to working with sequences, making it a cornerstone in ensuring ordered data manipulation. The linear progression of actions facilitated by sequential processing brings clarity and predictability to code execution, contributing to the overall reliability and maintainability of Python programs.
Repetitive Tasks
On the flip side, tackling repetitive tasks through the for loop brings forth the essence of automation and efficiency in programming workflows. By automating recurring actions, programmers can execute tasks with precision and consistency, reducing the chance of errors and enhancing productivity. The ability to loop through operations repetitively empowers developers to streamline processes, ultimately saving time and effort in handling repetitive tasks efficiently.
Basic Syntax
Initialization
Beginning with initialization in the for loop syntax unveils its role in setting the stage for iteration. Initialization marks the starting point of the loop, defining initial conditions and preparing the ground for subsequent iterations. By initializing variables or values at the onset, programmers establish a baseline for the loop's execution, ensuring a structured and controlled progression through the sequence. This proactive approach enhances code clarity and readability, fostering a smooth flow of iteration within the loop.
Condition
The condition segment within the for loop acts as a gatekeeper, determining the continuation or termination of the loop based on specified criteria. Setting conditions enables developers to control the loop's behavior dynamically, adding flexibility and adaptability to the iteration process. Conditionals provide a mechanism for incorporating logical checks within the loop, ensuring that iterations align with predetermined requirements, thereby optimizing the workflow and enhancing code accuracy.
Iteration
At the heart of the for loop lies the iteration phase, where the actual processing and manipulation of data occur. Iteration allows for the traversal of elements in a sequence, enabling access, modification, or analysis of each item iteratively. This iterative approach to data handling promotes a systematic and comprehensive exploration of sequences, empowering programmers to work with individual elements efficiently and apply necessary operations seamlessly.
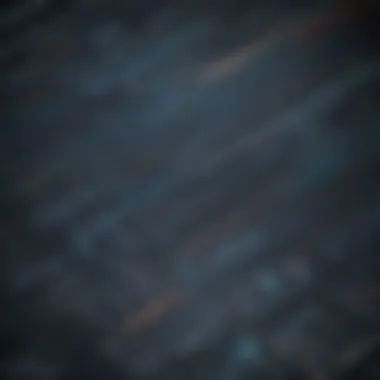
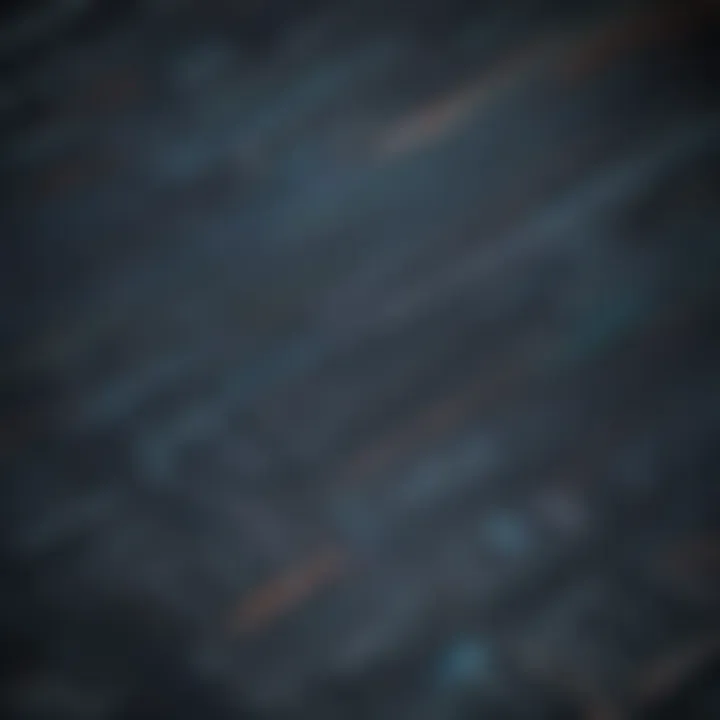
Iterating Through Lists and Tuples
In the realm of Python programming, iterating through lists and tuples stands as a fundamental aspect that encapsulates the essence of effective sequence manipulation. Understanding the nuances of iterating through these data structures not only enhances the efficiency of code but also allows for comprehensive data processing. Lists and tuples serve as vital components in Python, offering a plethora of benefits in storing and retrieving data efficiently. When delving into the intricate world of Python's for loop syntax, mastering the art of working with lists and tuples becomes imperative for any adept programmer.
Working with Lists
Accessing Elements
Accessing elements within a list holds substantial significance when navigating through extensive data sets. This specific operation allows programmers to retrieve individual elements based on their index position, facilitating targeted data extraction. The key characteristic of accessing elements lies in its capability to provide direct access to data points, streamlining the retrieval process effectively. This method emerges as a popular choice for its simplicity and directness, enabling programmers to access and manipulate data with ease. The unique feature of accessing elements underscores its efficiency in fetching specific data entries swiftly, making it a valuable asset in Python programming. While access comes with speed and precision, one must also be cautious of potential indexing errors that could arise, affecting the accuracy of data retrieval in complex list structures.
Modifying Elements
Modifying elements within a list presents a transformative capability essential for dynamic data manipulation. This operation contributes significantly to the overall data processing flow by enabling alterations to existing data points seamlessly. The key characteristic of modifying elements lies in its power to update individual elements or sections of a list, fostering data adaptability and flexibility. This feature's popularity stems from its ability to enhance data integrity by allowing real-time modifications without reconstructing the entire list structure. The unique feature of modifying elements lies in its versatility, empowering programmers to tailor data elements according to evolving requirements. While the advantages of modification are evident in its data-altering efficiency, caution must be exercised to maintain data coherence and consistency throughout the modification process.
Handling Tuples
In the domain of Python, handling tuples signifies a nuanced approach to managing immutable sequences efficiently. Iterating through immutable sequences such as tuples brings a distinct value to the overall data processing workflow. Tuples, characterized by their immutability, offer a stable and secure data storage mechanism for preserving critical information. When exploring the intricacies of iterating immutable sequences, the key characteristic of tuples shines through in their reliability and data integrity preservation. This unparalleled reliability makes tuples a popular choice for scenarios requiring data immutability and integrity assurance. The unique feature of iterating immutable sequences underscores the seamless and secure data traversal within tuple structures, providing a robust foundation for data manipulation. While the advantages of tuples in data integrity are undeniable, limitations may arise in situations requiring dynamic data modifications due to the inherent immutability of tuples.
Advanced Techniques
In the realm of Python programming, mastering advanced techniques associated with for loops is paramount to enhance efficiency and productivity. The Advanced Techniques section in this article serves as a crucial component, shedding light on nuanced methodologies that go beyond the basics. By delving into this realm, readers can elevate their coding prowess and tackle more complex problems with ease. Understanding and implementing these advanced techniques provide a competitive edge in the realm of Python programming, making them essential topics to explore.
Using Enumerate
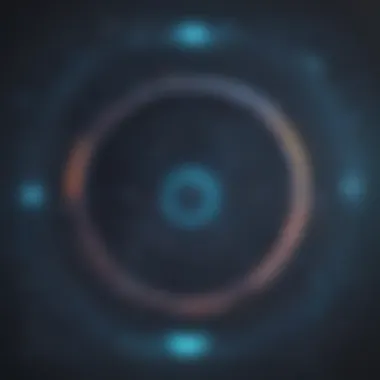
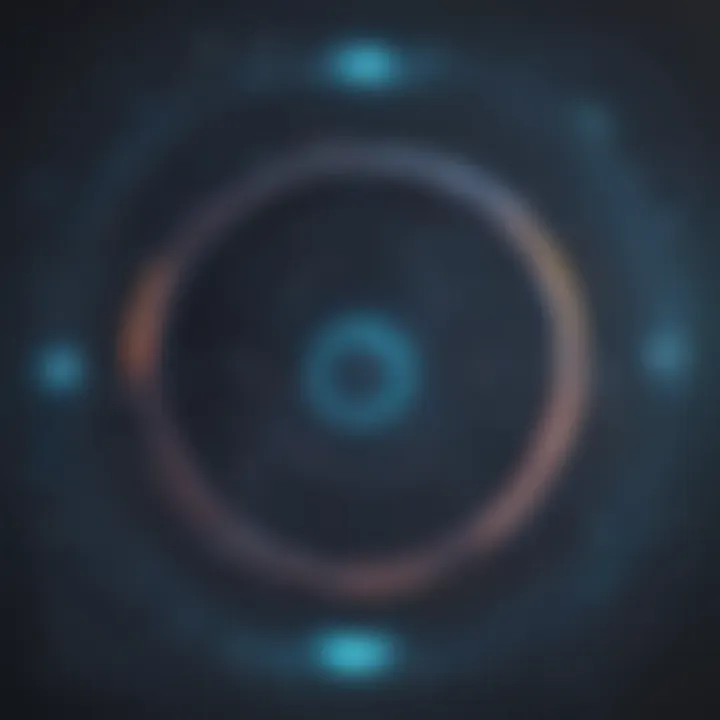
Accessing Index and Value Simultaneously
Unraveling the intricacies of accessing index and value simultaneously through the 'enumerate' function is a pivotal aspect of efficient Python coding. This subheading delves into the nuances of how this technique contributes to the overall goal of seamless iteration over sequences. The key characteristic of accessing index and value simultaneously lies in its ability to streamline the coding process by providing direct access to both elements within a sequence. This approach proves to be a popular choice in this article due to its effectiveness in handling complex data structures and simplifying the iteration process. The unique feature of accessing index and value simultaneously is its ability to eliminate the need for manual index tracking, enhancing readability and reducing the risk of errors. While this technique offers significant advantages in terms of readability and efficiency, it may introduce slight overhead due to the additional functionality it provides.
Iterating Over Dictionaries
Accessing Keys and Values
An essential aspect of Python programming involves iterating over dictionaries, a task meticulously explored in this section. Accessing keys and values is a critical operation that furthers the overall objective of effective iteration over dictionary data structures. The key characteristic of this process lies in its ability to provide direct access to key-value pairs within a dictionary, facilitating seamless manipulation and analysis of data. This technique stands out as a popular choice in this article due to its significance in handling key-based data structures and extracting valuable information from dictionaries. The unique feature of accessing keys and values is its versatility in accommodating diverse dictionary structures and simplifying data retrieval operations. While this technique offers substantial benefits in terms of data manipulation and processing efficiency, it may require additional handling for nested dictionaries or complex key structures.
Nested For Loops
Handling Multidimensional Data
Navigating the intricacies of nested for loops opens up a world of possibilities in managing multidimensional data effectively. This section delves into the specific aspect of handling multidimensional data through nested for loops and its crucial contribution to achieving the overarching goal of efficient data processing. The key characteristic of this approach is its ability to traverse multiple dimensions of data structures, enabling comprehensive analysis and manipulation of complex datasets. Nested for loops emerge as a beneficial choice in this article due to their versatility in addressing multilayered data representations and performing intricate computations. The unique feature of handling multidimensional data through nested for loops lies in its inherent scalability and adaptability to diverse data formats, enhancing the flexibility of data processing operations. While this technique offers significant advantages in terms of data exploration and computational efficiency, it may introduce complexity in logic flow and debugging processes.
Optimization Tips
As we navigate through the intricate landscape of Python's for loop syntax, the section on Optimization Tips emerges as a beacon of significance. In this expansive guide, we place a poised emphasis on optimizing the efficiency of our code, ensuring that each iteration operates with finesse and effectiveness. Optimization Tips engenders a strategic approach to code execution, elevating the performance of our scripts to new heights. By delving into the nuances of optimization, we unearth a treasure trove of techniques that not only streamline the execution process but also enhance the overall functionality of our Python programs.
Improving Efficiency
Minimizing Loops
Within the realm of Optimization Tips lies the pivotal concept of Minimizing Loops. This facet encapsulates the art of condensing our iterative processes, consolidating redundant loops, and refining the efficiency of our codebase. Minimizing Loops stands as a beacon of efficiency, allowing us to achieve more in less time. By strategically reducing the number of loops within our iterations, we optimize the performance of our scripts, crafting leaner and swifter solutions for our programming challenges. The essence of Minimizing Loops lies in its ability to trim unnecessary repetition, promoting clean and concise code structures that resonate with the ethos of high-quality programming.
Avoiding Unnecessary Operations
Further augmenting our quest for optimization within the for loop syntax is the imperative strategy of Avoiding Unnecessary Operations. This component underlines the importance of judiciously crafting our code to sidestep superfluous operations that burden our scripts. Avoiding Unnecessary Operations catalyzes the efficiency of our programs by eliminating redundant tasks, enhancing the speed and fluidity of our iterations. By consciously steering clear of unnecessary operations, we refine the execution of our code, amplifying its performance while upholding the tenets of elegance and precision in programming. The hallmark of this strategy rests in its ability to declutter our codebase, fostering a streamlined and harmonious coding environment that speaks volumes about our commitment to excellence.