Exploring Java Interfaces: A Detailed Guide
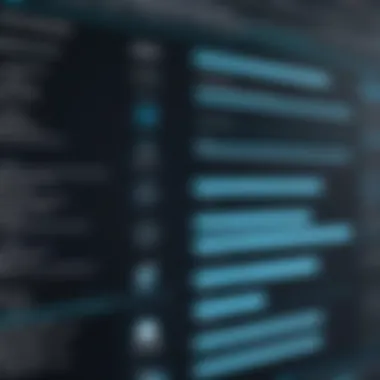
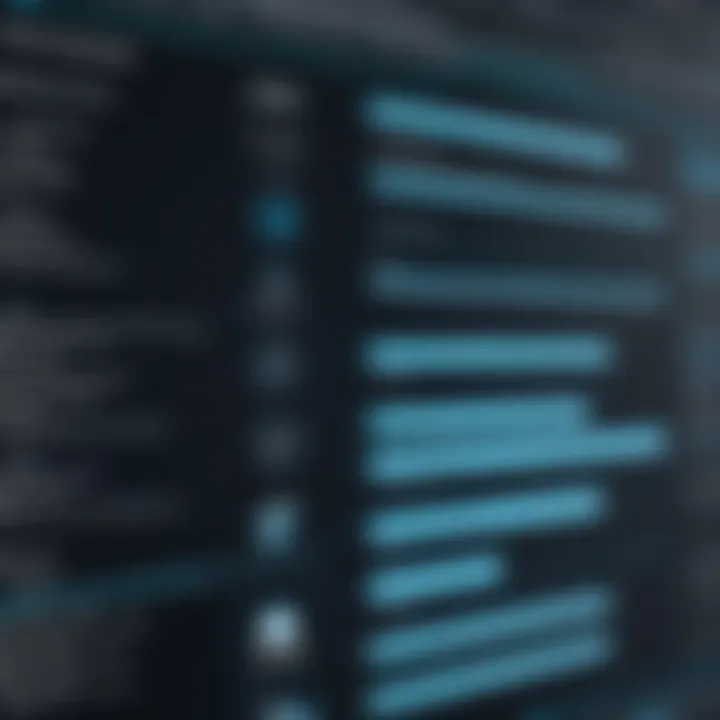
Intro
The world of software development is often seen as a complex web of languages, frameworks, and tools, each playing a vital role in bringing solutions to life. Among these, Java stands tall, characterized by its versatility and strong community support. In this ecosystem, interfaces serve as a cornerstone, shaping how developers approach object-oriented programming. They act as a contract, ensuring that certain methods are implemented while giving freedom for diverse implementations.
This exploration will clarify what interfaces are, why they matter in Java, and how they can enhance coding practices. From novice programmers finding their footing to seasoned developers looking to refine their skills, this comprehensive overview aims to provide everyone with valuable insights into Java's interface functionalities.
The Relevance of Interfaces
In simpler terms, interfaces define a method signature without implementing it, essentially giving developers a blueprint to follow. They foster a clean separation of concerns, allowing different components of software to communicate without stepping on each other's toes. Moreover, with the introduction of functional interfaces in Java 8, the landscape became richer, enabling even more powerful programming paradigms such as lambda expressions.
With these concepts in mind, let’s dive into the various dimensions of Java interfaces, addressing their definition, structural framework, real-world applications, and advanced topics that can optimize the way we write code.
Keep your eyes peeled as we unpack best practices and case studies that illuminate the innovative uses of interfaces in Java. After all, understanding Java interfaces is not just about enhancing your coding arsenal; it’s about embracing a mindset that values modularity and versatility in programming.
Prelims to Java Interfaces
Java interfaces are crucial elements in the landscape of object-oriented programming. They serve as a contract, specifying the methods that implementing classes must provide. The vitality of interfaces lies in their ability to facilitate polymorphism, allowing disparate objects to be treated uniformly. This is particularly valuable in large systems, where maintaining a common interface can greatly enhance code maintainability and clarity. When developers understand interfaces thoroughly, they open the door to cleaner designs, increased testability, and a clearer path to managing complexities in their code.
Definition and Purpose
At its core, an interface in Java is a reference type, similar to a class, that can contain constants, method signatures, default methods, static methods, and nested types. However, it cannot contain instance fields or constructors. The reason for this strict structure lies in the essence of what an interface is meant to achieve: it outlines a behavior that classes can promise to implement.
Consider the following elements of its purpose:
- Defining behavior: Interfaces establish a set of methods that various classes can implement. This ensures a level of abstraction that makes it easier for developers to work with multiple classes interchangeably.
- Enabling multiple inheritance: Unlike classes, Java interfaces allow a form of multiple inheritance, meaning a class can implement multiple interfaces, thus inheriting behavior from various sources.
- Encouraging flexibility: When systems rely heavily on interfaces, swapping implementations becomes a breeze. This gives developers the flexibility they need to adapt or expand system functionality without major rework.
"An interface is not just a tool for achieving abstraction; it’s a strategy for higher quality and more sustainable coding practices."
Historical Context
The concept of interfaces isn't unique to Java; it finds its roots in many programming languages, evolving alongside the need for clear specifications in software design. However, interfaces in Java were introduced in Java 1.0, laying the groundwork for building robust and scalable applications. Prior to interfaces, languages leaned towards using abstract classes to handle similar responsibilities, but these could only indicate one inheritance level, limiting developers.
The introduction of interfaces marked a paradigm shift. It allowed designers to focus on what a class should do rather than how it does it, reinforcing the principles of abstraction and separation of concerns. As Java itself evolved, so did its interface capabilities, notably with features like default methods introduced in Java 8. This allowed interfaces not just to declare methods, but to also provide default implementations, further enhancing their utility in modern programming practice.
In summary, Java interfaces are far more than mere declarations—they are essential game-changers that bolster good design, encourage adherence to programming principles, and significantly enhance the coder's toolkit.
Core Characteristics of Interfaces
Understanding the core characteristics of Java interfaces is paramount for any developer aiming to leverage the full potential of the language. Interfaces act as a contract within object-oriented programming, allowing different classes to work together without knowing their internal workings. This section will highlight three main characteristics that form the backbone of interfaces: abstract methods, default methods, and static methods. Each plays a critical role in how interfaces are designed and utilized in Java projects.
Abstract Methods
Abstract methods are the most fundamental feature of interfaces. Essentially, an abstract method is one that does not contain any implementation. This means when a class implements an interface, it must provide the actual code for these methods. This approach allows for flexibility and ensures that any implementing class adheres to a specific contract laid out by the interface.
In a way, abstract methods set the stage for polymorphism, a pillar of object-oriented programming. This means you can call the same method on different objects, allowing for varied behaviors based on the class of the object. For instance, consider methods in an interface named :
A class like can implement to bark, while a class might meow. The beauty lies in the interchangeability of objects without sacrificing the integrity of the code.
Default Methods
Introduced in Java 8, default methods allow developers to add new methods to interfaces without breaking the classes that already implement those interfaces. This feature is especially important for maintaining compatibility as software evolves. Default methods come with a body—meaning, they can contain implementation details, which is a departure from the traditional abstract methods.
By integrating default methods, the Java developmental community managed to enhance interface functionality while protecting legacy code. As a real-world example, consider an interface called that would have a default method for :
Here, any class implementing now has a honking capability without needing to implement that method. This promotes code reusability and cleaner design.
Static Methods
Static methods are a lesser-known yet crucial characteristic of interfaces in Java. Unlike instance methods, static methods belong to the class itself rather than to instances of the class. This means they can be called without creating an instance of the interface.
Having static methods in interfaces introduces a way to group utility or helper methods relevant to the interface's purpose. For instance:
You can call without instantiating a class—a quick, smart alternative for utility functions. This encapsulates related functionalities directly within the interface.
Ending
The core characteristics of Java interfaces—abstract methods, default methods, and static methods—each contribute significantly to robust and flexible software design. As developers harness these features, they can facilitate better code management, enhance reusability, and ensure that their applications can evolve without disruption. Understanding these elements is essential for maximizing the power of interfaces in Java programming.
Creating and Implementing Interfaces
Creating and implementing interfaces form the backbone of how we structure our Java applications. This topic holds substantial weight as interfaces not only define the contracts that classes must follow but also promote a clean separation of concerns, making code easier to read, maintain, and test. Understanding the nuances of interfaces can significantly enhance a developer’s ability to write modular and reusable code.
Defining an Interface
Defining an interface in Java is quite straightforward. At its core, an interface is like a blueprint for classes. It specifies what methods a class must implement without dictating how those methods should be defined. This provides the flexibility to implement various behaviors while adhering to a common contract.
A sample definition might look like this:
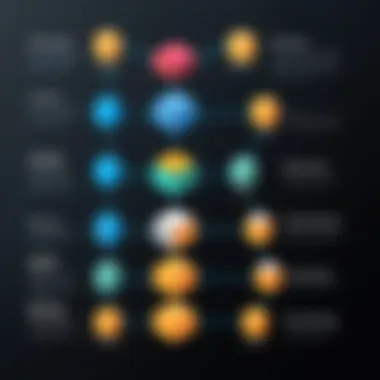
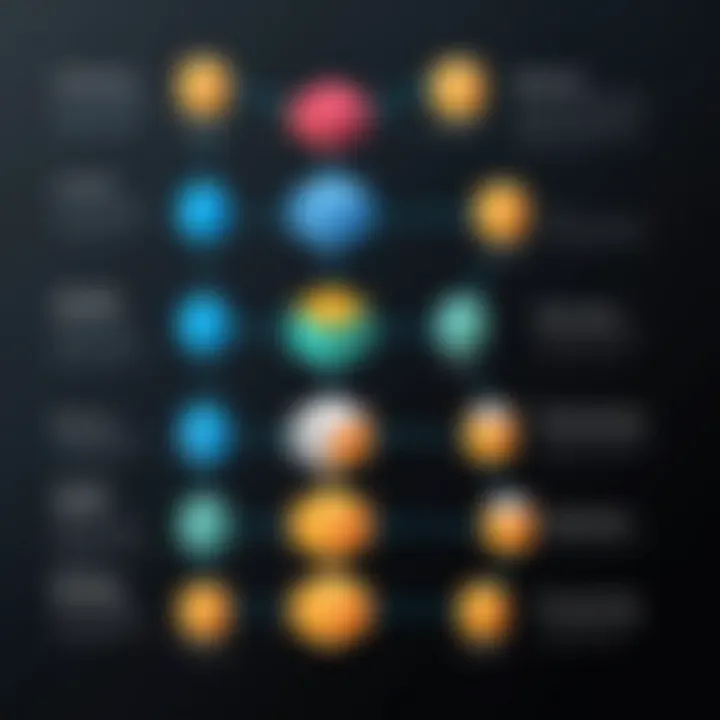
In this example, the interface states that any class implementing it must have a method. This type of definition clarifies expectations for developers, setting a clear guideline on what functionalities are necessary.
Implementation Syntax
Implementing an interface is where the promise of the contract is fulfilled. When a class implements an interface, it lays down the specific behavior of the methods declared in the interface. Take a look at this implementation:
Here, the class is implementing the interface. The annotation indicates that the method in is providing the specific behavior for the method in the interface. This clear-cut syntax reinforces the contract and ensures that developers adhere to the established rules.
Multiple Interfaces Implementation
One of the standout features of Java interfaces is the ability to implement multiple interfaces in a single class. This capability lets developers create versatile classes that embody diverse behaviors. Here’s how this can be done:
In this scenario, the class implements both the and interfaces. This dual interface implementation allows the to provide functionality for both behaving as an animal and as a pet, showcasing the flexibility offered by interfaces. By harnessing this functionality wisely, developers can create rich, polymorphic applications that align closely with real-world behaviors.
"Interfaces in Java are like contracts. They ensure that classes commit to certain behaviors, enabling a clean and flexible architecture."
In summary, understanding how to create and implement interfaces is critical for software developers aiming to build robust and maintainable applications. Interfaces empower developers by providing clarity and structure. Properly utilized, they can lead to code that is not only more readable but also more adaptive to future changes.
Interfaces and Object-Oriented Principles
The relationship between interfaces and object-oriented principles in Java is fundamental to understanding how software can be designed and structured for optimal efficiency and flexibility. When one delves into this topic, it becomes clear that interfaces don’t merely play a supporting role; rather, they act as essential building blocks that enhance the way classes can interact, share functionality, and deliver concrete implementations. This section will discuss three main concepts: polymorphism, abstraction, and encapsulation, each demonstrating how interfaces enrich object-oriented design.
Polymorphism
Polymorphism is a hallmark of object-oriented programming, allowing methods to do different things based on the objects that it is operating upon. In the context of Java interfaces, polymorphism emerges quite naturally. When a class implements multiple interfaces, it can be treated as an instance of any interface; this creates a scenario where the same method can invoke different behaviors across various objects.
For example, consider a simple interface called :
Using this interface, different classes can implement their own versions of the method:
In this setup, both and can be referred to as . Thus, calling on a reference can yield various outputs depending on the actual object that reference points to. This capability promotes flexibility within code. When designing polymorphic systems, think of how different implementations can reduce redundancy, allowing for a cleaner, more efficient code base.
Abstraction
Abstraction is another principle that interfaces excel at facilitating. By defining an interface, a developer creates a contract of methods that implementing classes must follow, while hiding the complex underlying implementation details. This leads to a situation where the focus can remain on what a class can do, rather than how it does it.
Consider a banking application where various payment methods can be used. An interface might be designed as follows:
Concrete classes like , , and can then provide their own implementations of the method:
Each payment method is abstracted from the details of how payments are processed. Consequently, when a client wants to use a payment method, they interact with the , knowing only that a payment will occur regardless of the specifics. This separation of concerns not only makes the code cleaner but also allows for easier testing and maintainability.
Encapsulation
Encapsulation limits access to certain components of an object and ensures that changes internal to the object don't directly affect the other parts of the program. Although one might usually think of this concept relating more to classes and their data, interfaces also lend themselves well to the practice of encapsulation.
In Java, by keeping certain methods or properties in the interface while implementing the logic in classes, developers can control which methods are exposed to the outer world. For example, let’s say you have an interface designed to expose the method but keep the details about internal calculations private:
The implementing class could possess private methods that are not available to the outside world:
Here, the details of or are well-encapsulated within , ensuring other classes or modules do not tamper with these important attributes directly. It promotes the integrity of the object’s state while allowing the necessary interaction through the interface methods.
This not only simplifies client-side code that consumes these objects but also protects the internal state from unintended modifications.
By mastering the interplay between interfaces and these object-oriented principles, developers can leverage sophisticated code structures that promote reusability and maintainability. As Java continues to evolve, understanding these nuances only becomes increasingly crucial for crafting robust software.
Functional Interfaces and Lambda Expressions
In the realm of modern Java, Functional Interfaces coupled with Lambda Expressions stand out as transformative components that amplify the efficiency and elegance of code writing. The evolution brought forth by Java 8 introduced a paradigm shift that resonates across software development, enabling developers to write cleaner, more concise code. This section elucidates the significance of these concepts, emphasizing their benefits and the practical considerations programmers must bear in mind.
Understanding Functional Interfaces
A functional interface, by definition, is an interface that contains exactly one abstract method. This singular focus allows it to be utilized as the assignment target for a lambda expression. Examples of functional interfaces include , , and , commonly employed in multi-thread programming and sorting operations.
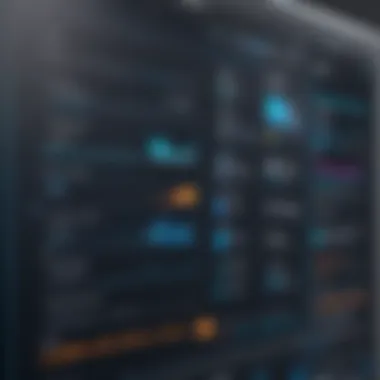
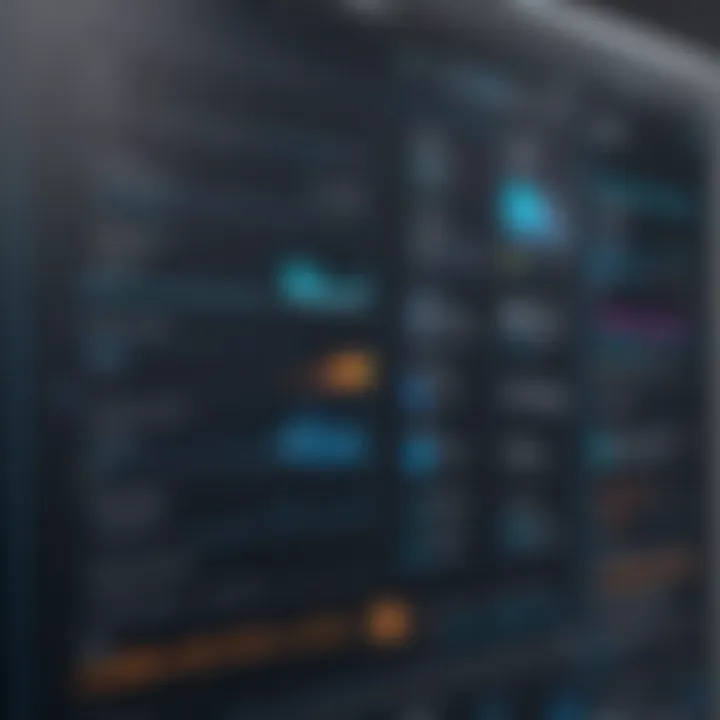
The implications of functional interfaces extend into the realm of style and clarity. They facilitate a functional programming approach in Java, which can lead to more readable and maintainable code. Consider the following example:
This simple definition sets the stage for a lambda expression that can implement the method. A notable strength of functional interfaces is their ability to promote the use of expressions rather than statements, thereby encouraging conciseness.
Benefits of Functional Interfaces
- Simplicity: Because they require only one abstract method, functional interfaces tend to be easier to implement and maintain.
- Higher Readability: They help in writing less boilerplate code, hence improving the readability of the codebase.
- Support for Lambda Expressions: They create a natural synergy that allows developers to express instances of single-method interfaces as more succinct and expressive forms.
Using Lambda Expressions
Lambda expressions are one of the most actionable features that arose with Java 8. They allow developers to create instances of functional interfaces in a more straightforward manner, without the need for formal classes. The basic syntax is simple and intuitive, resembling that of a mathematical expression.
In the above code, the lambda expression effectively implements the interface’s method in a few lines rather than requiring a full class. This showcases how lambda expressions can eliminate unnecessary verbosity, making the codebase leaner.
Moreover, lambda expressions can be passed as parameters to methods, thereby enhancing the utility of collections and streams. For instance, when paired with Java's Stream API, they enable operations such as filtering, mapping, and reducing data sets effectively. A practical example with a List would look like this:
In the snippet above, the method takes a lambda expression that allows the collection of names to be iterated over seamlessly.
"With functional interfaces and lambda expressions, Java has embraced a functional programming ethos that encourages developers to think differently about how they write code."
This shift not only enhances productivity but also aligns Java with modern programming practices. Though the concepts may appear deceptively simple, they carry profound implications on how interfaces are utilized within programming, especially in large systems where maintainability and performance become crucial.
In summary, functional interfaces and lambda expressions present powerful tools in the Java developer's kit. They break down barriers of complexity, rendering code more approachable, while fostering a deeper appreciation for the functional programming paradigm. As such, understanding and employing these features is essential for any developer looking to harness the full potential of Java.
Best Practices for Java Interfaces
When venturing into the realm of Java interfaces, it’s crucial to understand that crafting an effective interface goes beyond mere syntax. Best practices provide a roadmap to ensure that interfaces remain clear, concise, and maintainable. These practices not only enhance code readability but also simplify collaboration among developers. Below are the key practices that every programmer should internalize.
Clear Naming Conventions
A well-structured interface begins with a clear naming convention. Naming is not just about being creative; it's about clarity and intuitiveness. Here are some elements to consider:
- Descriptive Names: The names of the interfaces should reflect their purpose. For instance, using to represent sorting behavior is much clearer than something generic like .
- Avoiding Prefixes: Prefixing interface names with (like ) may be a habit from other languages but isn't necessary in Java. This unneeded prefix may even lead to confusion.
- Consistent Style: Stick to a consistent naming style across all interfaces. For instance, all interface names can be in camel case – this helps in quickly identifying what’s what.
These practices, while simple, lead to a significant improvement in code quality. A developer picking up your code should immediately grasp what an interface does just by looking at its name.
Keeping Interfaces Focused
In the world of software development, focus is a golden rule. An effective interface should serve a singular purpose. Here’s why maintaining focus is vital:
- Single Responsibility Principle (SRP): Every interface should be responsible for just one thing. This makes your interfaces much easier to implement and understand. For instance, rather than combining logging and data retrieval methods into one interface, separate them into and interfaces.
- Simplicity: A focused interface leads to simpler implementations. If an interface grows beyond its necessity, implementing classes can become overly complicated. Too much responsibility means more chances for bugs.
- Ease of Testing: Focused interfaces allow for better unit testing since you can test the implementation in isolation.
By keeping interfaces focused, developers can create cleaner, more manageable code bases. It’s like building a well-organized toolbox; you know where everything is without having to dig through a mess.
Leveraging Inheritance
Inheritance isn’t just for classes; it has a significant role in interfaces as well. Understanding how to use inheritance can enhance your interface design:
- Base Interfaces: Define base interfaces that capture common methods. For instance, if you have and , they can inherit from a base interface ; this centralization boosts consistency and reuse.
- Default Methods: Java 8 introduced default methods, allowing interfaces to provide a standard implementation. Use this feature to define default behaviors that all implementing classes can utilize or override as needed. This reduces code duplication immensely.
- Extensibility: By leveraging inheritance, you create a solid foundation for future extensions. New features can be added without altering existing code structure, encapsulating change and encouraging scalability.
In summary, inheritance in interfaces, when done correctly, can contribute to a more modular and flexible design, allowing for the addition of features without creating chaos.
Interfaces in Frameworks and Libraries
Java interfaces play a crucial role in various frameworks and libraries. They serve as a contract between classes, enabling a flexible structure that encourages cleaner and more maintainable code. This adaptability is particularly prominent in large applications where interfaces help manage interactions among different components. By defining what methods a class must implement without specifying how, developers can create interchangeable parts which can greatly simplify testing and enhance code reusability.
The design philosophy supported by interfaces ensures that developers enjoy various benefits, including easier refactoring, improved collaboration among teams, and clearer separation of concerns. Additionally, frameworks can standardize designs which helps speed up development processes. When working with frameworks like Spring and JDBC, understanding how interfaces are leveraged can elevate one’s ability to build scalable applications effectively.
Spring Framework Utilization
Spring Framework is a prime example of how interfaces can be utilized to streamline the development of Java applications. It operates on a set of core principles that revolve around dependency injection and aspect-oriented programming. Interfaces in Spring serve multiple purposes:
- Decoupling: Through the use of interfaces, Spring enables a loose coupling between different components. This means that changes to one part of the system can occur without forcing alterations to other sections, maintaining the integrity of the entire application.
- Testability: Interfaces commonly allow for easier unit testing. Developers can create mock implementations of interfaces to test class behaviors in isolation, ensuring that each piece functions properly before they are integrated together.
- Flexibility: Spring promotes the use of interfaces as a means to alter behaviors dynamically through configuration. This can save time by reusing existing code rather than writing new classes, which ultimately boosts productivity.
In AA's configuration, you might define an interface like this:
Such an interface can then be implemented by various classes providing different greeting styles—perhaps one for formal greetings and another for casual ones. The Spring framework can then inject the correct implementation at runtime based on configuration, making it a breeze to handle changes without rewriting code.
JDBC and Its Interfaces
Jumping into the JDBC (Java Database Connectivity), we see yet another significant application of interfaces in Java. JDBC is vital for interacting with databases and involves several key interfaces that define how Java applications connect and execute SQL statements against a database.
- Connection Interface: This establishes a connection to the database, controlling session and transaction handling. A class can implement this to manage how connections are made, provided that the underlying functionality remains consistent with how connection management is expected.
- Statement and PreparedStatement Interfaces: These interfaces facilitate executing SQL queries against a database. Differences between a regular statement and a prepared statement come down to performance and security, with the latter aiding in preventing SQL injection attacks.
- ResultSet Interface: This is key in allowing developers to handle the result sets from queries efficiently. An application can manipulate data fetched from a database conveniently using its defined methods, showcasing how a clear interface can streamline data retrieval processes.
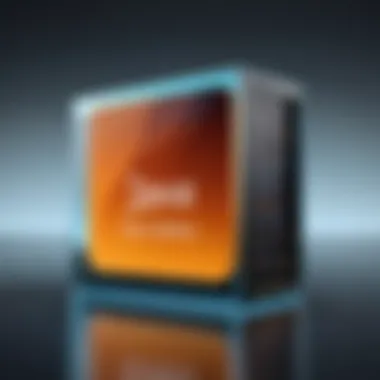
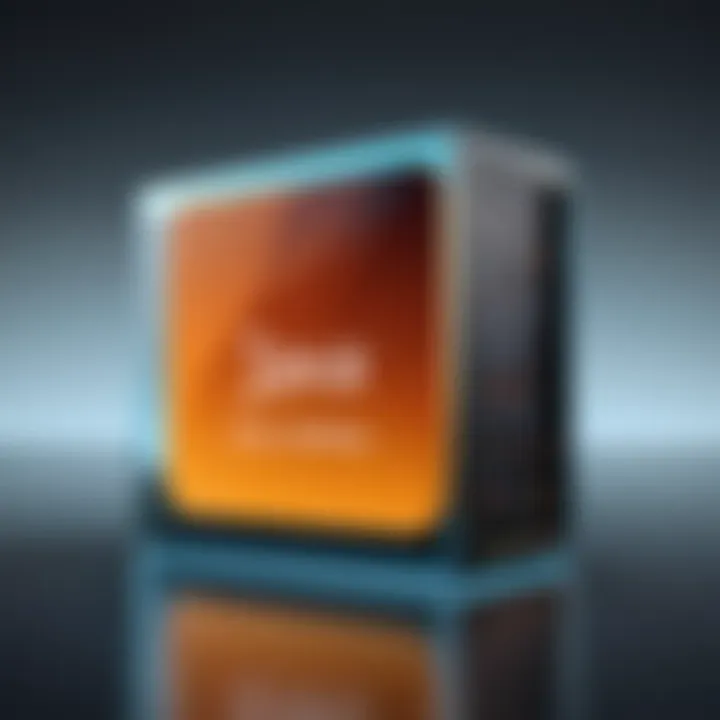
To illustrate, initializing a database connection through JDBC might look like this:
Here, the interface-based design allows any developer familiar with JDBC to navigate database interactions without deeply diving into implementation details. This makes it easy to switch out database connectors, thereby promoting flexibility and adaptability.
In summary, whether through frameworks like Spring or essential utilities such as JDBC, the impactful role of interfaces in Java cannot be overstated. They empower developers to build maintainable, testable, and scalable applications, keeping challenges at bay as systems evolve.
Real-world Applications of Java Interfaces
Understanding the real-world applications of Java interfaces is crucial for any developer looking to harness the full potential of object-oriented programming in practical scenarios. Interfaces not only dictate how classes should interact but also allow for a flexible architecture that can adapt and scale in complex applications. They serve as blueprints for software design, facilitating loose coupling and enhancing maintainability.
In environments where code reusability and modularity are paramount, interfaces become indispensable. They provide a means to enforce certain behaviors in different classes without tightly integrating those classes. This separation allows for cleaner code and a more organized approach to software development.
Design Patterns Using Interfaces
One of the fundamental ways to leverage Java interfaces in real-world applications is through established design patterns. Two popular design patterns that employ interfaces effectively are the Strategy Pattern and the Observer Pattern, each offering unique advantages and capabilities.
Strategy Pattern
The Strategy Pattern embodies a powerful approach to defining a family of algorithms or behaviors and making them interchangeable. By utilizing interfaces, developers can define a common protocol for the algorithms without tying them to specific implementations. This decoupling allows for easier changes and enhancements since new algorithms can be introduced without modifying existing code.
- Key characteristic: The primary feature of the Strategy Pattern is its ability to encapsulate algorithms.
- Why it stands out: This pattern excels in situations where multiple algorithms need to be employed interchangeably, making it a favored choice in scenarios requiring diverse functionality.
- Unique feature and considerations: The main advantage of this pattern is flexibility. However, a disadvantage is the increased number of classes that may result, which could introduce complexity in smaller applications. It's vital to balance between clarity and the number of classes used when applying this pattern.
Observer Pattern
The Observer Pattern provides a mechanism to notify multiple objects about changes in the state of another object. It’s particularly useful in scenarios where a one-to-many dependency needs to exist between objects. Interfaces in this pattern define the observer's role and behavior, allowing for dynamic relationships between the subject and its observers.
- Key characteristic: Its defining trait is the loose coupling between the subject and the observer, where the subject merely maintains a list of observers and notifies them of state changes.
- Advantages and why it’s popular: This pattern helps create a dynamic and responsive application architecture. It is especially beneficial in UI frameworks where multiple interface components need to react to changes in the underlying data. A potential disadvantage is that excessive notifications can lead to performance issues if not managed properly.
Microservices Architecture
As software architecture evolves, Java interfaces find themselves at center stage in microservices development. In a microservices architecture, the application is broken down into smaller, independent components that can be developed, deployed, and scaled independently. Interfaces enable clear contracts between these components, ensuring that they can communicate effectively despite being developed in isolation.
- Importance of interfaces: They define the methods that must be available for interaction, allowing different services to work together without needing internal knowledge of each other’s workings. This aligns perfectly with the principles of microservices, where each service can evolve independently as long as it adheres to the defined interfaces.
- Example scenarios: For instance, a user management service might implement an interface that allows other services, such as authentication and billing, to interact with user data without needing to know how that data is managed internally.
Comparative Analysis with Other Programming Paradigms
In a rapidly evolving landscape of programming languages, understanding how Java interfaces align with similar constructs in different languages can offer valuable insights. This section sheds light on the comparative strengths and weaknesses of interfaces in Java against the backdrop of languages like C# and Python. This comparison not only provides clarity on design choices but also demonstrates how various programming paradigms handle common challenges in software development.
Interfaces in
C# is a language that shares a lot of similarities with Java, especially when it comes to object-oriented principles. Interfaces in C serve as contracts, defining the capabilities that implementing classes must guarantee. Unlike Java, C allows for default implementations in interfaces, which was introduced to ease the process of evolving existing APIs without breaking backward compatibility. Here are some distinguishing features and considerations about C interfaces:
- Multiple Interface Inheritance: C# supports multiple interface inheritance directly. This enables developers to create classes that can implement numerous interfaces without the complications associated with implementing multiple classes.
- Explicit Interface Implementation: This feature allows a class to implement members of an interface in such a way that they can only be accessed through the interface type, providing a way to handle naming conflicts between interfaces.
- Nullable Reference Types: C# supports nullable reference types, enhancing type safety in the usage of interfaces by allowing for better annotations about nullability.
The syntax also strays slightly, using keyword similarly, but showcasing distinct enhancements. For example, an interface in C# can look like this:
This conveys what any animal must do, showing a neat and succinct contract.
Interfaces in Python
Python's approach to interfaces is a bit different, as it is a dynamically typed language, emphasizing duck typing over static typing. In Python, the concept of interfaces isn't enforced through a specific keyword like in Java or C#. Instead, one can define an interface through abstract base classes (ABCs) using the module. While Python does not have formal interfaces, the following aspects are noteworthy:
- Duck Typing: This principle asserts that an object's suitability for use is determined by the presence of methods and properties rather than its actual type. Thus, if an object can perform the required operations, it can be considered as implementing the interface.
- Flexible Implementation: Python allows a more flexible implementation of the interface since there's no strict enforcement. Adhering to a protocol is by convention, fostering creativity at the expense of potentially less predictability.
- Simplicity in Syntax: Python’s syntax is arguably more intuitive, leading to less boilerplate. An interface-like behavior can be created quickly using simple class definitions without the rigid structure seen in compiled languages.
Here’s a quick example in Python illustrating how one might create an interface-like structure:
This enforces that any subclass must implement the method, thus mimicking the interface concept.
Understanding these differences illuminates how each programming paradigm handles interfaces. This cross-language analysis not only reveals unique strengths and potential weaknesses but also underscores the broader impact of design choices on development. Herein, we find that while Java’s implementation emphasizes stability and structure, languages like C# and Python offer traits aimed at enhancing usability and flexibility.
Future Trends in Java Interfaces
The realm of Java interfaces is evolving at a pace not to be underestimated. As the programming landscape continues to change rapidly, so too does the way developers interact with interfaces in their projects. This section will paint a picture of the future trends that are shaping Java interfaces and why they matter for software developers, IT professionals, and tech enthusiasts alike.
Evolution of Interfaces
Throughout the past decade, we have witnessed a significant transformation in how interfaces are conceptualized and utilized. In the earlier days of Java, interfaces primarily served a simplistic role—the mere definition of a contract for classes. However, with the introduction of default and static methods in Java 8, interfaces have become more robust, allowing for greater flexibility in code implementation.
This evolution can be broken down into several key elements:
- Interfaces as a Design Tool: Developers now leverage interfaces to enforce design contracts more than ever before. They facilitate cleaner architecture and better abstraction, leading to software that is easier to maintain and extend.
- Incorporation of Functional Programming Concepts: As programming practices continue to converge, Java interfaces embrace functional programming elements, allowing for behavior to be represented as data, and simplifying the development of complex systems.
- Integration with Modern Frameworks: Java interfaces are increasingly integrated with contemporary frameworks like Spring and Hibernate, influencing how applications handle dependencies, transactions, and configurations.
These elements not only reflect changing patterns in software development but also suggest a future where interfaces will support more dynamic and adaptable coding practices.
Potential Enhancements in Upcoming Versions
As Java progresses, there's substantial conversation surrounding what enhancements will grace future versions. Here are a few speculations that could widely alter the landscape of interfaces:
- Advanced Type Inference: There’s an ongoing discussion on improving type inference within interfaces. This could enable developers to write less boilerplate code, enhancing readability and decreasing the chances of errors.
- Improved Static Methods: While static methods currently exist in interfaces, further enhancements might allow a more refined approach to how these methods interplay with instance methods, potentially leading to more cohesive designs.
- Dynamic Interfaces: Considering the rise of microservices and cloud-based applications, Java could introduce dynamic interfaces. This would support real-time updates to interface methods without needing to recompile the entire codebase, a massive boon for hot-swapping implementations.
As technology advances, staying updated on these trends is crucial for developers. Embracing the future of Java interfaces can foster innovation and creativity in software design, empowering programmers to build robust and adaptable solutions.