Exploring Java Algorithms: A Comprehensive Guide
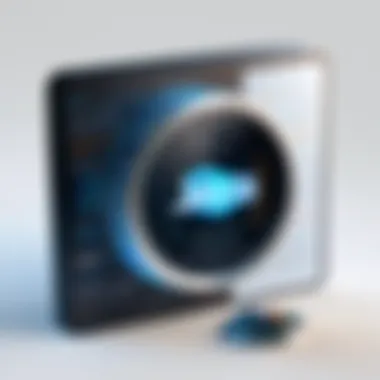
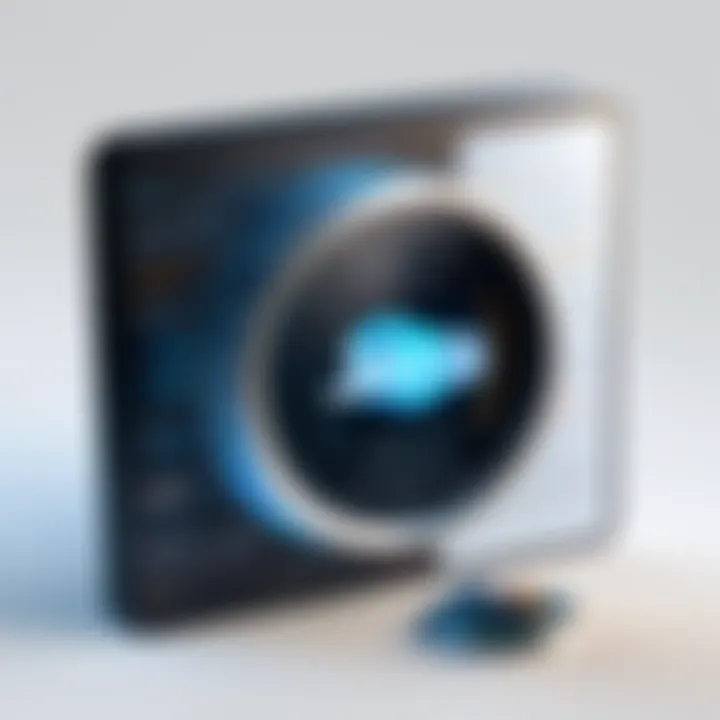
Intro
In the realm of software development, algorithms serve as the backbone of efficient programming practices. This guide aims to systematically unravel the complexities of Java algorithms. Covering key concepts, practical implementations, and advanced techniques, it caters to both novice programmers and seasoned developers.
Algorithms Not only dictate how data is processed, but also impact performance and scalability within applications. Understanding algorithms helps improve the efficiency of code and enhances the overall performance of software products. In particular, Java, as a widely-used programming language, offers a plethora of algorithmic solutions that can be leveraged effectively.
This guide will detail the relevance of Java algorithms in various aspects of programming. We will explore fundamental topics such as sorting and searching algorithms, and delve into data structures that utilize these algorithms. Furthermore, we will examine best practices for algorithm design, and offer step-by-step tutorials that will empower users to apply these concepts in real-world scenarios.
Through this journey, the objective is to provide clarity on the importance of algorithms within Java development and to equip readers with the knowledge required to optimize their programming skills.
Prologue to Java Algorithms
Understanding algorithms is crucial for any developer working in Java. Algorithms dictate how data is processed, analyzed, and transformed into useful information. In this section, we will explore the definition of algorithms specifically in the context of Java and discuss their significance in software development.
Defining Algorithms in Java
In Java, an algorithm can be defined as a step-by-step process or set of rules that leads to the solution of a problem. Each algorithm needs to be unambiguous, effective, and terminate after a finite number of steps. Java offers a robust platform for implementing various algorithms due to its object-oriented nature. For example, common algorithms like sorting or searching can be implemented through methods within classes, allowing for code reusability and organization.
By defining algorithms clearly, developers can enhance the efficiency of their applications. This can include anything from optimizing the way data is retrieved from a database to improving user interface responsiveness. Essentially, a well-crafted algorithm avoids unnecessary processing and streamlines performance.
Importance of Algorithms in Software Development
Algorithms form the backbone of software development. Their importance cannot be overstated as they directly influence the performance and scalability of applications. Effective algorithms lead to optimized resource management, which can significantly reduce the operational costs associated with running software.
Moreover, algorithms enhance problem-solving capabilities. Developers often encounter complex problems that require effective solutions. Employing the right algorithm enables them to devise strategies that efficiently solve these challenges.
Effective algorithms can enhance both the stability and reliability of software.
As technological demands evolve, the complexity of algorithms also increases. Understanding this evolution allows developers to keep pace with industry changes. Continuous learning about algorithms ensures that software maintains competitive performance in a rapidly advancing field.
Types of Algorithms
Understanding the types of algorithms is crucial for any developer working with Java. Algorithms lay the groundwork for problem-solving in programming. Different types serve specific purposes and improve efficiency in various tasks. This section will explore sorting, searching, and graph algorithms, focusing on their characteristics, advantages, and shortcomings. Each type serves as a building block in software development, impacting performance and scalability. By knowing the distinct types of algorithms, developers can choose the right one for the tasks at hand.
Sorting Algorithms
Sorting algorithms are essential for organizing data in a specific order. They greatly influence the speed of data retrieval and analysis. Efficient sorting is particularly important because it can significantly reduce the time complexity of subsequent searches. Below are some common sorting algorithms:
Bubble Sort
Bubble Sort is a straightforward sorting technique. It works by repeatedly stepping through the list, comparing adjacent elements, and swapping them if they are in the wrong order. This process continues until no swaps are needed, indicating that the list is sorted.
Key Characteristic: The method is known for its simplicity and ease of implementation.
Why It’s Beneficial: It’s often used for educational purposes to teach sorting concepts.
Unique Feature: The algorithm's time complexity is O(n^2) in the worst-case scenario, which makes it inefficient for large lists. However, its simplicity can make it useful for small datasets or when simplicity is preferable to efficiency.
Quick Sort
Quick Sort is a widely used efficient sorting algorithm. It uses a divide-and-conquer approach to sort elements. The algorithm selects a 'pivot' element and partitions the other elements into two sub-arrays according to whether they are less than or greater than the pivot.
Key Characteristic: Its average-case time complexity is O(n log n), making it much faster than bubble sort for larger datasets.
Why It’s Beneficial: It’s popular in various applications due to its efficiency.
Unique Feature: Quick Sort is in-place, which means that it requires only a small amount of additional memory space. However, its worst-case performance is O(n^2) if the pivot is not well chosen, though this can often be mitigated by using randomization.
Merge Sort
Merge Sort is another divide-and-conquer algorithm that divides the array into sub-arrays and then merges them back together in a sorted manner. This process of splitting and merging continues until the final sorted array is obtained.
Key Characteristic: Merge Sort has a consistent time complexity of O(n log n), making it reliable in different scenarios.
Why It’s Beneficial: It is particularly effective for large data sets and can handle linked lists efficiently.
Unique Feature: Merge Sort requires additional memory for the temporary arrays used during the merging process, which can be a drawback for memory-limited environments.
Searching Algorithms
Searching algorithms are critical for finding specific information within data structures. Choosing the right search method can greatly enhance the program's efficiency.
Linear Search
Linear Search is perhaps the simplest searching technique. It checks each element in a list sequentially until the desired element is found or the list ends.
Key Characteristic: There is no need for the data to be sorted.
Why It’s Beneficial: Linear search is easy to implement and understand.
Unique Feature: Its time complexity is O(n), which makes it less efficient for large datasets. It’s mostly suited for small or unsorted collections.
Binary Search
Binary Search is much more efficient than linear search but requires the data to be sorted first. It works by dividing the search space in half with each comparison until the desired element is found.
Key Characteristic: Its time complexity is O(log n), which makes it highly effective for large, sorted arrays.
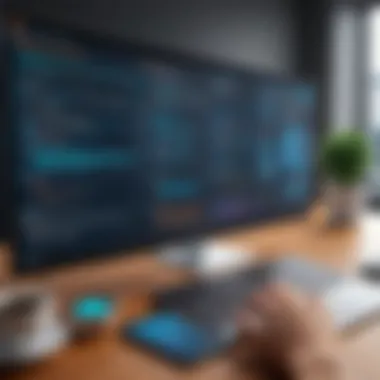
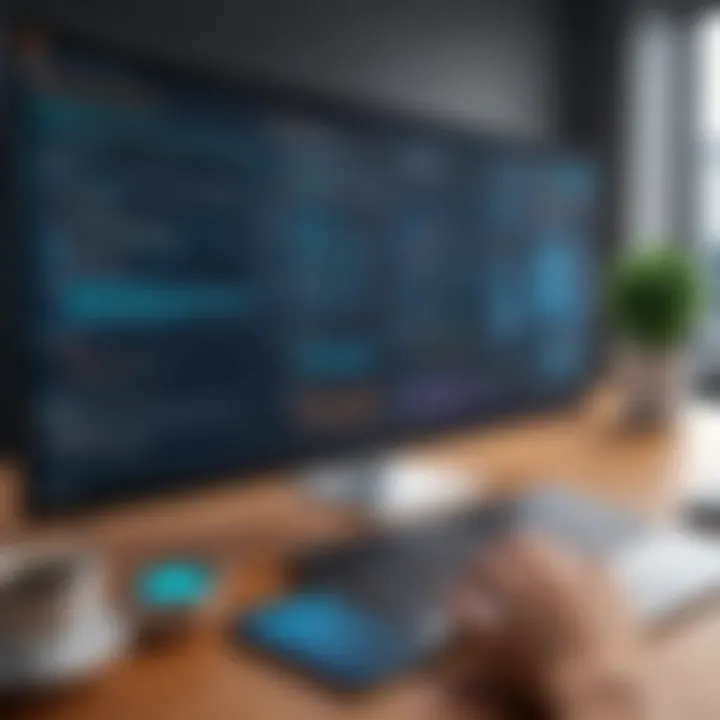
Why It’s Beneficial: It significantly reduces the search time compared to linear search.
Unique Feature: While the initial requirement of sorted data can be seen as a disadvantage, the efficiency gained in large datasets often outweighs this drawback.
Graph Algorithms
Graph algorithms are vital for managing and analyzing relationships between connected data points. They have broad applications in various fields such as networking, robotics, and social media analysis.
Dijkstra's Algorithm
Dijkstra’s algorithm finds the shortest path between nodes in a graph. It works optimally with graphs that have non-negative edge weights. The method systematically explores nodes based on the shortest discovered distance from the starting node.
Key Characteristic: It guarantees the shortest path in weighted graphs.
Why It’s Beneficial: It’s widely used in GPS systems and network routing.
Unique Feature: While efficient, Dijkstra's algorithm can be slow with densely connected graphs, as it requires prioritizing nodes dynamically.
A Search Algorithm
The A* Search Algorithm intelligently finds the shortest path using heuristics. It uses both the cost to reach the node and an estimation of the cost from the node to the goal.
Key Characteristic: It is versatile and can be tailored through different heuristics for specific use cases.
Why It’s Beneficial: Its efficiency and flexibility make it popular in game development and artificial intelligence.
Unique Feature: While it’s generally faster than other algorithms for pathfinding, its performance can be heavily influenced by the choice of heuristic.
Implementation of Java Algorithms
Implementing algorithms in Java is a crucial aspect that bridges theory with practical application. It involves translating algorithmic logic into a functional Java program. Understanding these implementations not only helps in learning Java but also cultivates problem-solving skills. One must consider various factors such as code efficiency, structure, and reusability when implementing algorithms. This section emphasizes the importance of a proper approach to algorithm implementation, which directly affects software performance and maintainability.
Java Code Structure
Classes and Methods
Classes and methods form the backbone of any Java program, especially in algorithm implementation. Classes enable the organization of code into reusable components, allowing for better management and scalability. Each class can represent a distinct algorithm or conceptual unit, encapsulating relevant data and behaviors. Methods, on the other hand, are more focused. They define actions that an object can perform.
The key characteristic of using classes and methods is modularity. This trait allows developers to break down complex algorithms into simpler, manageable parts. When an algorithm is well-structured this way, debugging and enhancements become considerably easier. For instance, a sorting algorithm can be implemented as a class with methods for various sorting techniques.
However, one unique feature of classes and methods is the method overloading, where multiple methods can have the same name but differ in parameters. This aids in increasing readability and usability of the code. Such features can be very beneficial in developing more intuitive code but might also lead to confusion if not documented properly.
Packages and Imports
Packages and imports in Java are essential for organizing classes into namespaces, allowing for a clean separation of functionalities. They help prevent naming conflicts and enable a better development organization. Utilizing packages becomes important when working on large projects where various algorithms can be compartmentalized into their respective packages.
A key characteristic of packages is encapsulation, promoting code security and functionality isolation. This is particularly beneficial in algorithm implementation, as algorithms from different domains can coexist without conflict. For instance, a package for sorting algorithms can be kept separate from one for searching algorithms.
A unique feature of packages is their ability to define a clear structure in a project. This organization allows developers to easily locate and understand sections of the code. However, excessive package nesting can complicate imports and accessibility. This may occasionally counter the benefits of using packages if not handled properly.
Setting Up Your Development Environment
A proper development environment enhances productivity and efficiency when implementing Java algorithms. One needs several components to set up effectively: an Integrated Development Environment (IDE), Java Development Kit (JDK), and build tools like Maven or Gradle. IDEs such as IntelliJ IDEA or Eclipse simplify coding with features like syntax highlighting and debugging tools. The JDK provides essential libraries and handles compilation. Familiarity with these tools can greatly speed up the coding process, making it easier to focus on implementing and testing algorithms.
Common Libraries and Tools
Java Collections Framework
The Java Collections Framework (JCF) is a fundamental part of Java that provides data structures and algorithms to store and manipulate data efficiently. This framework includes classes like List, Set, and Map, each serving different purposes in data organization. The main contribution of JCF to algorithm implementation lies in its ability to simplify operations through predefined methods and data structures.
A key characteristic here is its performance. JCF is optimized for both speed and memory usage. For algorithm developers, this framework is popular because it allows easy manipulation of data without needing to create custom data structures from scratch.
A noteworthy advantage of using JCF is that it adheres to interfaces, thus promoting a more uniform approach to handling data across various algorithms. However, one disadvantage might be the learning curve associated with mastering the collections and understanding how they interact with different algorithms.
Apache Commons
Apache Commons is a set of reusable Java components that can streamline many common programming tasks. It encompasses various libraries that empower developers to implement functions quickly without reinventing the wheel. This versatility allows developers to focus more on solving core problems rather than dealing with boilerplate code.
The key characteristic of Apache Commons is its extensive library, which addresses multiple common issues faced in programming. Its popularity stems from the wide range of functionalities provided, which cover everything from string manipulation to logging.
A unique feature of using Apache Commons is its robustness and community support. This can significantly enhance the implementation of Java algorithms by providing reliable utilities. However, dependency on external libraries can lead to version control issues, and it may be overkill for simpler tasks.
Data Structures Relevant to Algorithms
Understanding data structures is crucial for any developer working with algorithms. Data structures provide a means to store, organize, and manage data efficiently. They are the backbone of algorithm design and implementation, influencing both performance and ease of use. Selecting the right data structure can optimize an algorithm’s efficiency, affect memory consumption, and ultimately determine the success of a software application.
Data structures vary in complexity and purpose. Their choice often depends on the specific needs of the application and the operations required. Here, we will discuss different types of data structures, focusing on arrays, linked lists, stacks, queues, trees, and graphs. Each of these structures provides unique properties and functionalities that cater to various algorithmic scenarios.
Arrays and Linked Lists
Arrays serve as a fundamental data structure in Java. They allow for static data storage, making access via index very efficient. However, their size is fixed, which can lead to waste of space if not fully utilized. In contrast, linked lists offer dynamic sizing. This key characteristic allows for flexible memory use, as elements can be added or removed without reallocating the entire structure. Linked lists are particularly useful when the number of elements changes frequently.
Pros of Arrays:
- Fast access to elements via index.
- Simple structure that is easy to implement.
Cons of Arrays:
- Fixed size may lead to inefficient memory use.
- Shifting elements can be costly when inserting or deleting data.
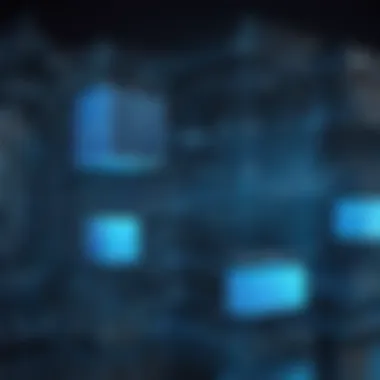
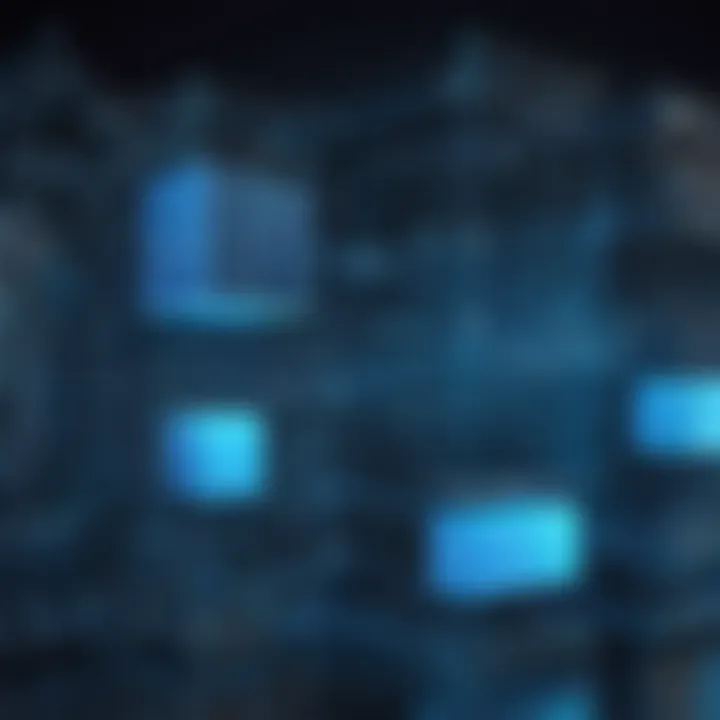
Pros of Linked Lists:
- Dynamic size allows for efficient memory utilization.
- Easier to insert and delete nodes compared to arrays.
Cons of Linked Lists:
- More memory overhead due to node pointers.
- Slower access time compared to arrays since traversal is needed.
Stacks and Queues
Stacks and queues are both specialized data structures that manage data in specific orders. A stack operates in a Last In First Out (LIFO) manner, meaning the last element added is the first to be removed. This is useful for scenarios like function calls in programming. Conversely, a queue functions on a First In First Out (FIFO) basis, where the first element added is the first to be removed. Queues are commonly used in scenarios like task scheduling and resource management.
Key Characteristics:
- Stacks: Useful for backtracking algorithms, such as undo mechanisms in text editors.
- Queues: Essential for implementing algorithms related to breadth-first search in graphs.
Trees and Graphs
Trees and graphs are more complex data structures used to represent hierarchical and interconnected data.
Binary Trees
Binary trees are tree structures with at most two children for each node. They provide a means for efficient searching, insertion, and deletion operations, making them foundational in various algorithms. One key characteristic is their balanced nature, which can enhance search performance. Binary trees are popular due to their simplicity and versatility.
Unique Feature:
A significant advantage of binary trees is their ability to facilitate ordered data, allowing for in-order, pre-order, and post-order traversals. This can be beneficial in scenarios where sorted data is crucial. However, unbalanced trees may lead to degraded performance similar to that of a linked list.
Binary Search Trees
Binary search trees extend the properties of binary trees by maintaining an ordered structure, where the left subtree contains values less than the node, and the right subtree contains values greater. They are efficient for searching, with an average time complexity of O(log n). This makes binary search trees particularly popular in applications requiring frequent search operations.
Unique Feature:
The self-balancing properties of red-black trees and AVL trees are notable. They ensure that the tree remains balanced, thus maintaining efficient operations. However, maintaining balance can introduce additional complications during insertion and deletion.
Directed and Undirected Graphs
Graphs are non-linear data structures that represent relationships between pairs of objects. In directed graphs, relationships have a specific direction, while undirected graphs lack this restriction. This distinction impacts the algorithms that can be applied effectively.
Key Characteristic:
Graphs excel in representing complex networks such as social connections or transport systems. They are crucial for pathfinding algorithms, like Dijkstra’s, which find the shortest path in weighted graphs. One unique feature is the ability to perform cycles in directed graphs, which may complicate certain algorithms. However, undirected graphs often allow for simpler traversals such as depth-first and breadth-first search, but might not accurately depict relationships with direction.
Algorithm Complexity
Understanding algorithm complexity is crucial when engaging with Java algorithms. It evaluates how efficiently an algorithm performs, both in terms of time and memory usage. As software developers, recognizing these complexities allows you to assess the scalability of your solutions in real-world applications. This topic aids in making informed choices about algorithms based on constraints and requirements, which is pivotal for creating efficient software.
Time Complexity
Time complexity refers to the amount of time an algorithm takes to complete as a function of the length of the input. This assessment allows developers to predict the performance and efficiency of an algorithm in situations with varying input sizes.
Big O Notation
Big O notation is a mathematical representation that describes the upper bound of time complexity. It provides a clear and consistent way to express how an algorithm's run time grows as the input size increases. A key characteristic of Big O notation is its ability to abstract non-dominant terms and constants, focusing solely on the highest order term, which dictates growth in terms of input size.
The main advantage of Big O notation lies in its simplicity and clarity. It allows for a quick comparison among different algorithms, guiding developers in selecting the most appropriate one for their needs. However, one unique feature to note is its disregard for constant factors, which can occasionally misrepresent an algorithm's practical performance. Thus, while Big O serves as a foundational tool in algorithm analysis, it should be used in conjunction with empirical testing for comprehensive insights.
Analyzing Time Complexity
Analyzing time complexity involves evaluating an algorithm to determine its efficiency accurately. This process combines theoretical analysis with practical benchmarking, yielding insights into both best-case and worst-case scenarios. A primary characteristic of analyzing time complexity is its capacity to provide a more complete view of an algorithm's performance across various situations.
This consideration is beneficial in both academic and industrial contexts. It enables software engineers to identify bottlenecks and optimize performance effectively. Furthermore, a unique aspect of analyzing time complexity is its ability to inform decisions about data structures suited for specific algorithms, depending on their performance profile. However, this process can become intricate and may require a deep understanding of underlying theories, which can be a disadvantage for beginners in the field.
Space Complexity
Space complexity measures the total memory space an algorithm uses relative to the input size. It dives into the allocation of temporary space during execution, which is essential for developers to consider. Balancing time and space complexity ensures that an algorithm operates efficiently without over-consuming resources, particularly in environments with limited memory.
Best Practices for Writing Java Algorithms
Writing algorithms in Java is a multifaceted task that demands a good balance of performance, clarity, and maintainability. Best practices in this area not only enhance the efficiency of the code, but they also create a more organized way for developers to collaborate and build complex systems. These practices encompass various elements, each contributing to the overall quality of the code, which is crucial in professional software development environments.
Code Efficiency
Code efficiency is a primary concern when writing algorithms. Efficient algorithms can drastically reduce runtime and resource consumption. There are various ways to improve efficiency:
- Choosing the right data structures: Different data structures come with unique properties and performance characteristics. Using an appropriate one, such as choosing a HashMap instead of an ArrayList for frequent lookups, can significantly elevate performance.
- Avoiding redundant calculations: Implementing caching techniques to store the results of expensive function calls can minimize computation time. Memoization is a common approach applied in recursive algorithms, like computing Fibonacci numbers.
- Optimizing loops: Always keep an eye on loop complexity. For instance, using a nested loop can lead to exponential time complexity, which should be avoided if possible. A well-structured loop minimizes iterations, enhancing overall execution speed.
Using tools like Java Performance Tuner can aid in identifying bottlenecks in the code. This is especially useful when dealing with large datasets or high-throughtput applications. Efficient algorithms not only help in meeting performance benchmarks but also improve user experience.
Readability and Maintainability
Readability and maintainability of code are just as important as its efficiency. Code that is clear and easy to read encourages collaboration and makes it easier for others (or oneself in the future) to understand and extend the logic. Some essential strategies include:
- Adopting clear naming conventions: Variable and method names should convey purpose. For example, a method named is instantly clear compared to .
- Commenting wisely: Use comments to explain the rationale behind complex logic, rather than stating the obvious. This helps keep the code clean while providing necessary context.
- Structuring code logically: Organizing code into modules or methods enhances clarity. Group related functions and stick to single responsibility principles, ensuring that each method does one task well.
Readable code contributes to maintainability. When code is maintainable, it saves time and reduces the risk of introducing bugs when changes are made. A team can work more effectively with clean, understandable codebases.
Testing and Debugging
In software development, testing and debugging are critical phases of writing reliable algorithms. Proper testing frameworks can dramatically streamline this process. Here are some tips:
- Writing unit tests: Tools like JUnit can be very helpful in verifying individual units of code. By writing tests as you develop, you can ensure that each part of your algorithm performs as expected.
- Implementing integration tests: This is essential to validate how different parts of the code interact with each other. Integration tests can catch issues that unit tests might miss.
- Debugging tools: Java provides various debugging tools and IDE features that allow you to step through the code. This can help isolate the root causes of issues when your algorithm does not work as intended.
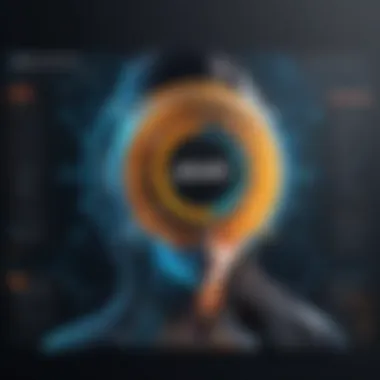
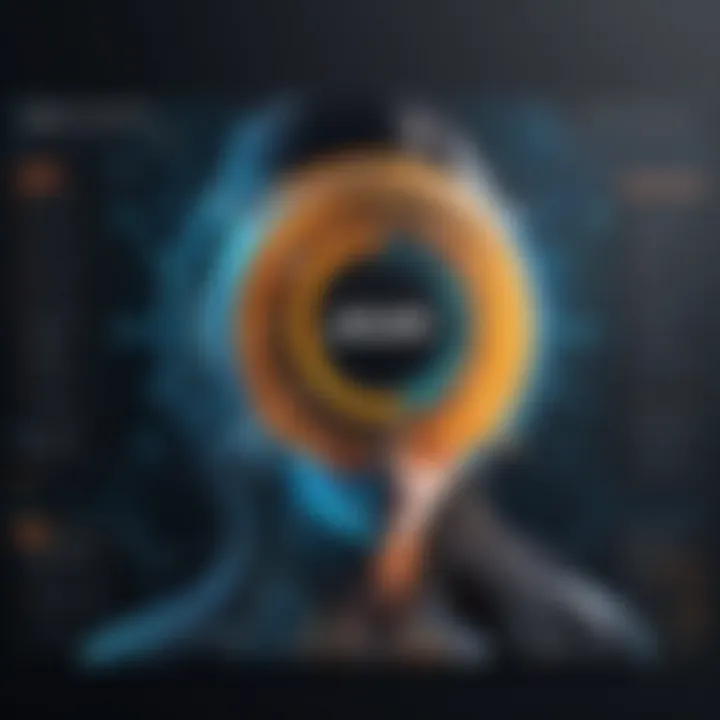
Unit testing frequently leads to fewer bugs in production. The practice of regularly testing algorithms also cultivates good coding habits and proactive problem-solving approaches.
Always remember: Good algorithms not just run well; they read well. A balance between efficiency and clarity is key to writing high-quality code.
By adhering to these best practices, you can create Java algorithms that not only perform proficiently but are also easy to read, modify, and test. This comprehensive approach yields long-term benefits in productivity and code quality.
Real-World Applications of Java Algorithms
Algorithms are essential in various domains, and their applications in real-world scenarios highlight their importance in Java programming. Understanding how these algorithms operate, along with their practical implications, can enhance a programmer's ability to solve complex problems effectively. The significance of this section lies in showcasing how Java algorithms function within real-world systems, detailing their benefits and the factors to consider when implementing them.
Algorithms in Data Analysis
In the realm of data analysis, Java algorithms play a vital role. They help in processing large volumes of data efficiently. Algorithms such as sorting and searching enable analysts to condense information into manageable outputs. For instance, using Quick Sort can significantly reduce the time it takes to organize datasets based on specific parameters.
Moreover, Java’s capabilities allow for integration with libraries like Java Data Analysis Library (JDAL) or Apache Commons Math, which provide various statistical utilities. This makes Java a suitable language for handling data mining tasks in fields like finance and healthcare.
"Data analysis algorithms can turn raw data into insights that drive decision-making processes."
Data analysis also involves critical tasks such as data cleaning and anomaly detection. Algorithms designed for these activities must not only be efficient but also scalable to handle increasing data loads. Understanding how to apply these algorithms can drastically improve the decision-making process in businesses.
Machine Learning Algorithms in Java
Machine learning is another significant area where Java algorithms are extensively utilized. Many developers prefer Java for machine learning projects due to its robust libraries and scalability. Libraries such as Weka, Deeplearning4j, and MOA (Massive Online Analysis) provide frameworks to build and train models effectively.
Java’s runtime performance ensures that machine learning algorithms, including regression models, decision trees, and neural networks, can operate on large datasets without severe latency. Furthermore, the language's ease of integration with big data tools like Apache Hadoop makes it a prominent choice for developing machine learning applications.
The flexibility of Java also allows developers to tune their algorithms by modifying parameters or implementing ensemble methods for better accuracy. Each of these aspects contributes to the growing importance of machine learning algorithms written in Java.
Challenges and Optimization
In software development, especially when working with algorithms, addressing challenges and finding optimization routes are paramount. Algorithms are not merely lines of code; they can define the efficiency and effectiveness of an application. As the complexity of the data and requirements increases, so do the challenges developers face. Recognizing these challenges allows developers to enhance their skills and create more robust applications.
Algorithm challenges can arise from various fronts, including but not limited to inefficient processing, high memory usage, and the inability to handle edge cases. Optimizing algorithms can lead to significant performance boosts. It is critical to understand that optimization does not just mean making code faster; it means making it more efficient in terms of resources utilized and responsiveness.
Common Algorithmic Challenges
There are numerous algorithmic challenges that developers encounter regularly. Here are some notable ones:
- Scalability: As data grows, algorithms may struggle to maintain performance. It is essential to design scalable algorithms that can handle increased data loads without a degradation in performance.
- Time Complexity: Writing an algorithm that executes quickly is essential. An algorithm with high time complexity can slow down applications significantly, particularly in data-intensive tasks.
- Space Complexity: Besides time, an algorithm's space usage is crucial. Improper management of resources can lead to crashes or slow response times in applications.
- Edge Cases: Many algorithms fail to consider all potential inputs. Handling edge cases is essential to ensure that the algorithm operates reliably under unusual or unexpected conditions.
By recognizing these common challenges, developers can proactively refine their approaches and implement solutions that address these issues effectively.
Techniques for Optimization
Once developers identify challenges, they can move toward optimization techniques that will enhance performance. Here are some effective strategies:
- Choosing the Right Data Structure: The choice of data structure can drastically improve algorithm efficiency. For instance, using hash tables for quick access is generally faster than arrays for search operations.
- Algorithmic Improvements: Many algorithms can be improved. For instance, modifying a brute-force solution into a divide-and-conquer approach can often reduce time complexity considerably.
- Caching Strategies: Implementing caching can improve speed by storing results of expensive function calls. When the same inputs occur again, the results can be retrieved from the cache rather than recalculated.
- Parallel Processing: Leveraging multi-threaded processing can break tasks into smaller independent units. This allows the algorithm to utilize multiple cores or processors, speeding up operations significantly.
- Profiling and Benchmarking: Profiling code helps in identifying bottlenecks. By benchmarking different implementations against each other, developers gain insights on what works best under various scenarios.
"Optimization is not a one-size-fits-all solution. It requires understanding the context and specific use-case to implement the most effective techniques."
These techniques, when applied judiciously, can lead to significantly enhanced application performance. Addressing challenges proactively allows developers to create more resilient systems, capable of adapting to changing demands.
Understanding both challenges and optimization in algorithm design not only empowers developers but also enhances the overall functionality of their software products.
Emerging Trends in Java Algorithms
In the fast-evolving field of software development, understanding the emerging trends in Java algorithms is crucial. This section explores modern techniques and methodologies that not only improve performance but also enhance scalability and sustainability of software solutions. Keeping abreast of these trends allows developers to harness the power of current technology while preparing for future advancements.
Parallel and Distributed Algorithms
Parallel and distributed algorithms have gained significant traction due to the growth in multi-core processors and networked systems. These algorithms break tasks into smaller sub-tasks, distributing them across multiple processors or machines.** This approach allows for simultaneous execution, which can dramatically reduce computation time.**
When implementing parallel algorithms in Java, developers often leverage libraries like the Fork/Join framework or the Java Concurrency API. These tools provide flexibility and efficiency, enabling improved utilization of resources. For instance, tasks that require heavy computations can be divided among several threads, each executing concurrently. The overall efficiency improves markedly as opposed to a single-threaded approach.
Considerations for Parallel Programming
- Data Dependency: Be mindful of variables that threads may access. Shared data can lead to issues if not managed correctly.
- Overhead: There is always some overhead when managing multiple threads. Ensure that the performance gain outweighs this cost.
- Testing and Debugging: Concurrent programs are often harder to test and debug due to non-deterministic behavior. Adopting best practices in coding helps mitigate such issues.
Ultimately, parallel algorithms represent a shift toward more efficient computing, crucial for applications requiring quick processing time, such as real-time analytics and high-performance computing .
Algorithmic Improvements with Cloud Computing
Cloud computing has transformed how applications are built and deployed. With its ability to scale resources dynamically, developers can implement algorithms that are not only powerful but also adaptable to varying workloads.** Cloud environments allow for the execution of algorithmic processes across numerous nodes, facilitating both horizontal and vertical scaling effortlessly.**
Java developers can exploit cloud platforms, such as Amazon Web Services (AWS) or Microsoft Azure, to leverage serverless computing models. This offers an opportunity to run Java algorithms without the concern of infrastructure management. Through these cloud services, algorithms can be executed in containers or as serverless functions, enabling a true focus on code rather than on servers.
Benefits of Cloud Computing in Algorithms
- Cost Efficiency: Pay only for what you use, minimizing operational costs.
- Elasticity: Scale resources based on demand, ensuring optimal performance.
- Global Accessibility: Cloud solutions provide accessibility from anywhere, promoting collaborative development.
Finale
In this article, the discussion around the conclusion provides a vital overview of the key findings related to Java algorithms. It is important to emphasize the role algorithms play in shaping software development. Developers rely on algorithms not only for efficiency but also for structuring logical solutions to complex problems. Algorithms can impact the performance of applications significantly, which is crucial in today's fast-paced technology landscape.
Review of Key Points
As we summarize the intricate aspects of Java algorithms, several key points emerge. Firstly, the understanding of various types of algorithms such as sorting, searching, and graph algorithms lays a foundation for effective coding practices. These facets are not merely academic but possess practical applications in solving real-world problems. Secondly, recognizing the complexities of algorithms, namely time complexity and space complexity, is necessary for optimizing code. This ensures not only functionality but also performance efficiency. Additionally, best practices in algorithm development, including code maintainability and testing, were highlighted. Each point reinforces the value of sophisticated algorithm design in the reliability of software products.
Future Directions in Java Algorithms
Looking forward, the landscape of Java algorithms is continuously evolving. Emerging trends like parallel and distributed algorithms are of increasing importance, especially as applications demand more speed and efficiency. With the workload growing, developers must adopt a scalable approach. Furthermore, the integration of cloud computing with algorithmic learning has opened pathways for more innovative solutions. This advancement will likely lead to greater collaboration among data scientists and software developers, enhancing the way algorithms are utilized in fields like machine learning and data analysis.
"The future of Java algorithms is bright, driven by the need for efficiency and adaptability in an ever-changing digital environment."
In summary, this exploration of Java algorithms has not only equipped developers with necessary knowledge but also emphasized the importance of adapting to new innovations. As these technologies progress, continuous learning and adaptation will be the keys to success in the field.