Unveiling the Intricacies of Data Validation in JavaScript
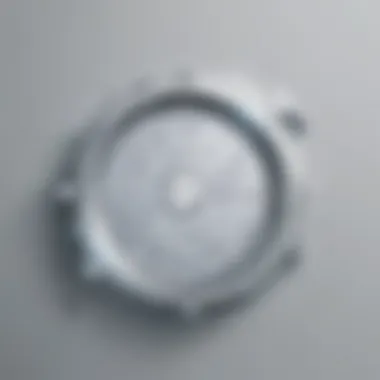
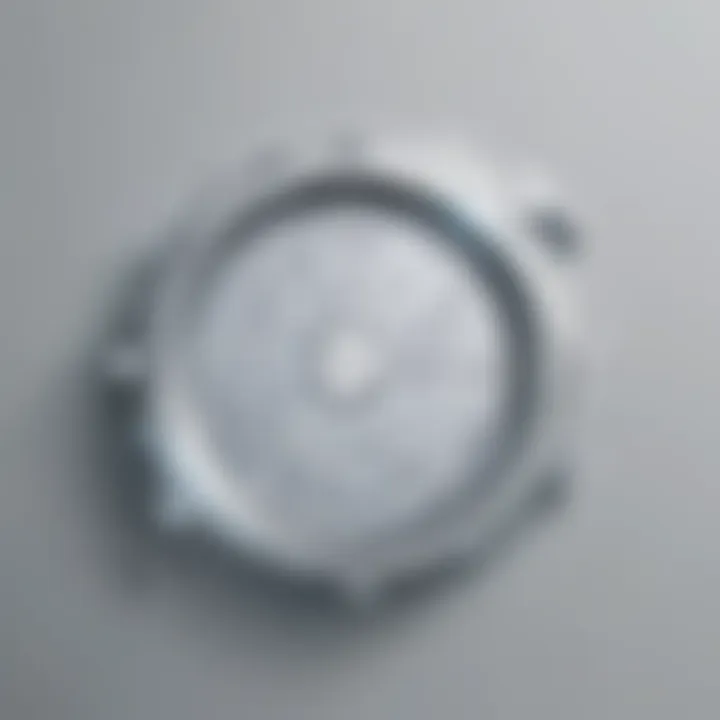
Overview of Data Validation in JavaScript
Data validation in JavaScript plays a crucial role in ensuring the integrity and security of web applications. By effectively validating user input, errors and malicious data can be prevented, leading to a more robust and reliable system. Methods utilized include both client-side and server-side validation to cover a wide range of scenarios where data validation is essential.
In the realm of software development, JavaScript serves as a cornerstone for frontend and backend web development. Its adaptability and flexibility make it a popular choice for creating dynamic and interactive web applications. Within this context, data validation acts as a safeguard against potentially harmful or erroneous input, bolstering the overall reliability of the application.
Best Practices for Data Validation in JavaScript
When implementing data validation in JavaScript, adhering to industry best practices is essential. Validating data at both the client-side and server-side, using appropriate libraries or frameworks, and implementing whitelist validation techniques are key strategies for ensuring data integrity. Furthermore, maintaining input sanitization practices and implementing secure communication protocols are vital for data security.
To maximize efficiency and productivity in data validation, developers should prioritize code readability and consistency. Utilizing descriptive variable names, comments, and documentation can aid in enhancing the maintainability of the validation process. Additionally, conducting thorough testing and validation of edge cases can help uncover potential vulnerabilities and ensure comprehensive data validation.
Common pitfalls to avoid in data validation include incomplete validation checks, inadequate error handling, and over-reliance on client-side validation. By addressing these challenges proactively, developers can create a more robust and secure data validation process.
Case Studies in Data Validation
Exploring real-world examples of successful data validation implementations provides valuable insights into effective strategies and outcomes. Case studies highlighting the impact of robust data validation practices in preventing data breaches or errors offer practical learning experiences. Learning from industry experts and their experiences can inform best practices and approaches for data validation.
Lessons learned from case studies underscore the importance of proactive validation, error detection, and secure data handling. By examining successful implementations, developers can gain inspiration and guidance for enhancing their own data validation processes.
Latest Trends and Updates in Data Validation
Staying abreast of upcoming advancements, industry trends, and innovations in data validation is crucial for harnessing cutting-edge techniques. The emergence of AI-driven validation tools, blockchain integration for secure data validation, and automated testing solutions are shaping the future of data validation practices.
Data validation tools that offer enhanced customization, scalability, and integration capabilities are gaining traction in the industry. By embracing the latest trends and updates in data validation, developers can stay ahead of the curve and optimize their validation processes for enhanced efficiency and reliability.
How-To Guides and Tutorials for Data Validation in JavaScript
For beginners and advanced users alike, step-by-step guides and hands-on tutorials on data validation in JavaScript offer practical insights and actionable knowledge. These resources cover various aspects of data validation, from basic validation techniques to advanced validation methods tailored to complex web applications.
Practical tips and tricks for effective data validation can help developers streamline their validation processes and address common challenges. By following comprehensive how-to guides and tutorials, professionals can enhance their expertise in data validation and navigate intricacies with confidence.
Introduction to Data Validation

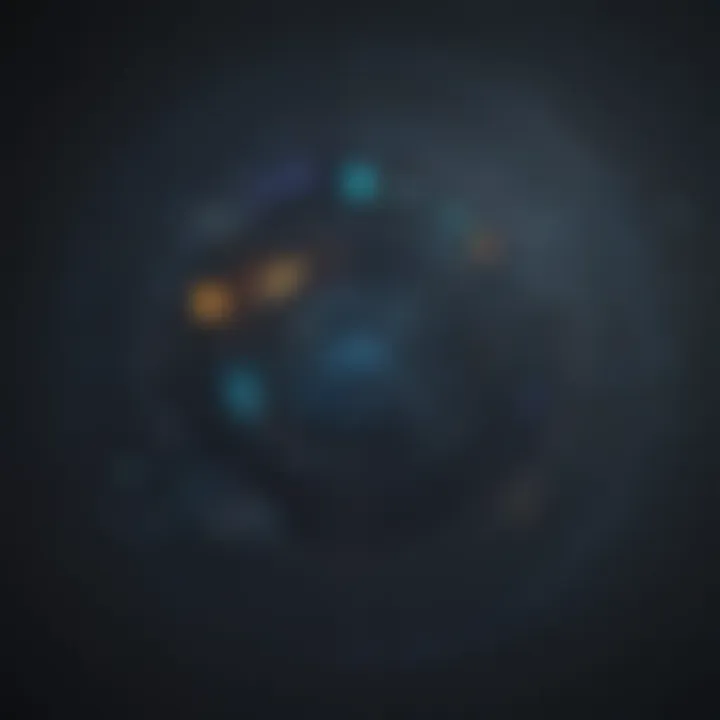
Data validation in JavaScript is a critical aspect of web development. It plays a pivotal role in ensuring the accuracy and integrity of data input by users, thereby enhancing the overall quality of web applications. By implementing robust data validation techniques, developers can prevent invalid data entry and mitigate potential security risks. This article aims to delve into the intricacies of data validation in JavaScript, providing insights into the significance, methods, and best practices to uphold data integrity and security.
Understanding the Significance
The role of data validation in web development
Data validation is essential in web development as it serves as a filter for incoming data, helping maintain consistency and validity. By validating user inputs, developers can ensure that the data meets predefined criteria, such as format and range, before processing it further. This helps prevent errors and enhances the overall reliability of the application. The role of data validation in web development is instrumental in guaranteeing data accuracy and minimizing vulnerabilities that could be exploited by malicious entities.
Importance of maintaining data integrity
Maintaining data integrity is crucial for the seamless functioning of web applications. Data integrity signifies the accuracy and consistency of data throughout its lifecycle. By incorporating data validation mechanisms, developers can uphold data integrity by detecting and correcting errors or anomalies in the input data. This ensures that the information stored in the application remains reliable and secure, fostering trust among users and safeguarding against data corruption or unauthorized access.
Impact on User Experience
Enhancing user interaction through validation
Validating user inputs not only ensures data accuracy but also contributes to a positive user experience. By providing real-time feedback on input fields, users can instantly identify and correct errors, enhancing user interaction. This interactive validation process improves usability and guides users towards completing forms accurately, reducing frustration and enhancing overall satisfaction.
Reducing input errors and enhancing usability
One of the primary benefits of data validation is its ability to minimize input errors, improving data quality and usability. By enforcing validation rules such as required field checks and input format validations, developers can streamline the data entry process and reduce the likelihood of erroneous submissions. This not only enhances the efficiency of the application but also increases user confidence in the data being submitted, leading to a smoother and more user-friendly experience.
Client-Side Data Validation
Client-side data validation is a crucial aspect when it comes to developing robust web applications in JavaScript. In this article, we will delve into the significance of implementing validation logic at the client-side to enhance user experience and ensure data integrity. By validating user inputs before sending them to the server, client-side validation helps in reducing errors and improving the overall usability of the application.
Implementing Validation Logic
Using JavaScript frameworks for client-side validation
One key aspect of client-side data validation is the utilization of JavaScript frameworks. These frameworks offer pre-built functions and methods that streamline the validation process, making it easier for developers to implement robust validation logic. The choice to use JavaScript frameworks for client-side validation is popular due to the time-saving nature of these tools, enabling developers to validate user inputs effectively and efficiently. Incorporating JavaScript frameworks for client-side validation in this article showcases a modern and efficient approach towards ensuring data integrity.
Validating user input in real-time
Validating user input in real-time is another critical component of client-side data validation. Real-time validation provides instant feedback to users as they fill out forms, alerting them to any errors or invalid entries promptly. This feature enhances user experience by improving usability and reducing the likelihood of input errors. The advantage of real-time validation lies in its proactive nature, allowing users to correct mistakes immediately without needing to wait for form submission. While real-time validation offers significant benefits, it is essential to consider factors such as performance implications and user feedback mechanisms in the context of this article.
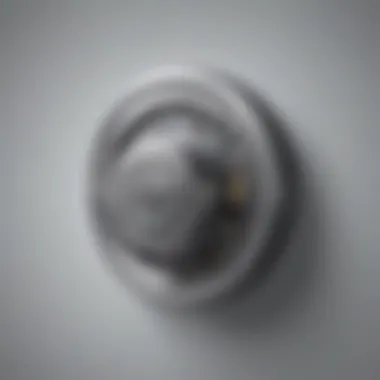
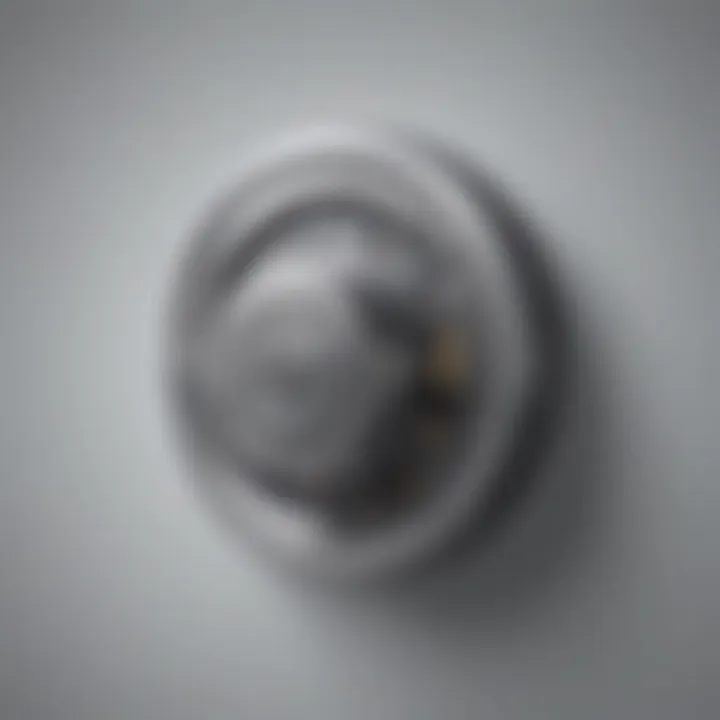
Common Validation Techniques
Required field validation
One common validation technique is ensuring that all required fields are filled out before submitting a form. Required field validation plays a fundamental role in data validation by ensuring that essential information is provided by users. By highlighting missing required fields, this technique helps in enhancing data integrity and preventing incomplete submissions. The benefit of required field validation lies in its ability to enforce data completeness, ultimately contributing to improved data quality. However, developers must carefully balance the strictness of validation to avoid frustrating users with unnecessary constraints.
Pattern matching and input format validation
Another significant validation technique involves pattern matching and validating input formats. By defining specific patterns that user inputs must adhere to, developers can ensure data consistency and accuracy. Pattern matching enables the validation of complex input formats, such as email addresses or phone numbers, enhancing the reliability of collected data. While pattern matching and input format validation offer precise control over data quality, developers need to consider the adaptability of these patterns to varying user inputs. Striking a balance between strict validation and user-friendly input requirements is key when implementing pattern matching and input format validation in this article.
Server-Side Data Validation
In the realm of JavaScript development, Server-Side Data Validation holds a paramount position in upholding data integrity and fortifying web application security. By validating data on the server, the risk of malicious input infiltrating the system is significantly minimized, ensuring that only legitimate and authorized data is processed. This crucial aspect of Server-Side Data Validation acts as a robust defense mechanism against potential cyber threats, safeguarding sensitive information from unauthorized access or manipulation. Implementing Server-Side Data Validation serves as a proactive measure to maintain data accuracy and reliability, promoting a secure digital ecosystem where user trust is preserved.
Ensuring Data Security
Validating data on the server to prevent malicious input
Validating data on the server to prevent malicious input is a foundational practice in data validation strategies. By implementing robust validation mechanisms at the server level, the integrity of the system is fortified, minimizing the risk of cyber attacks such as SQL injection or cross-site scripting. This aspect of data validation focuses on scrutinizing incoming data, verifying its authenticity, format, and permissions before allowing it to interact with the application's database or resources. The significance of this process lies in its ability to act as a gatekeeper, filtering out potentially harmful or unauthorized data, thereby preserving the confidentiality and integrity of the system.
Implementing server-side validation rules
Implementing server-side validation rules involves defining a set of criteria and constraints that incoming data must adhere to before being processed further. These rules act as benchmarks for data validity, ensuring that only conforming data is accepted and manipulated within the application environment. By establishing clear and stringent validation rules on the server side, developers can enforce data governance policies, standardize input formats, and prevent data corruption or manipulation. This systematic approach to data validation enhances the overall reliability and trustworthiness of the application, laying a solid foundation for secure and efficient data processing.
Handling Validation Errors
Displaying error messages to users
Displaying error messages to users is a critical component of the validation process, providing real-time feedback on the accuracy and completeness of input data. When validation rules are breached, informative error messages serve as guiding beacons for users, highlighting the specific issues that need to be addressed. By conveying clear and concise error feedback, users are empowered to rectify their input errors promptly, improving overall data quality and user experience. Effective error message display enhances user satisfaction and interaction with the application, fostering a seamless and intuitive data input process.
Logging and tracking validation failures
Logging and tracking validation failures play a pivotal role in error management and system maintenance. By recording instances of validation errors, developers can gain valuable insights into common pitfalls, trends in erroneous data input, and areas for improvement in validation protocols. Tracking validation failures facilitates proactive problem-solving, enabling developers to identify and address potential vulnerabilities or loopholes in the data validation process. Through systematic log management and analysis, developers can refine validation rules, optimize error-handling mechanisms, and enhance the overall robustness of the application's validation framework. The diligent tracking of validation failures contributes to continuous quality assurance and promotes a culture of data accuracy and reliability within the development environment.
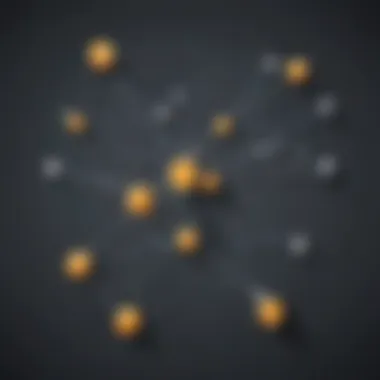
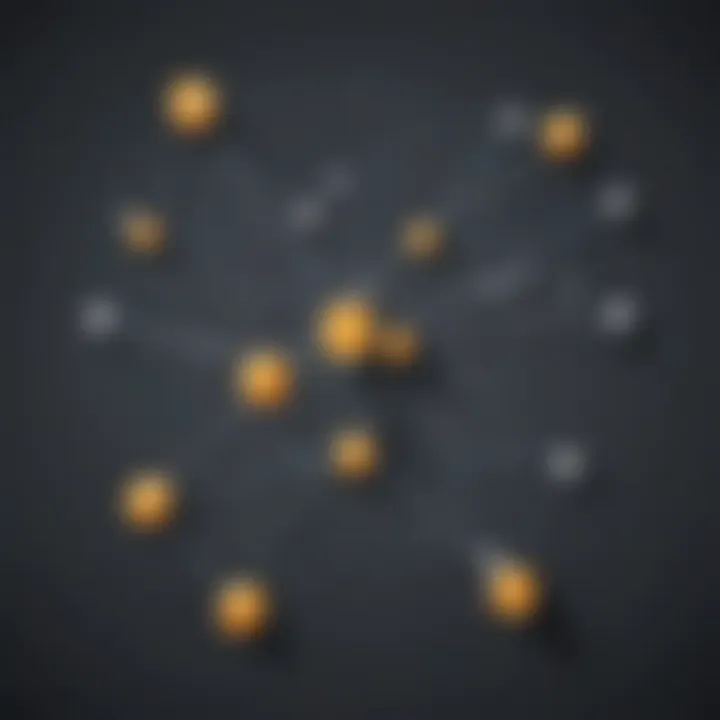
Best Practices for Data Validation
In the realm of web development, data validation stands as a crucial element to uphold data integrity and security. Implementing best practices for data validation ensures that web applications function reliably and securely. Embracing robust validation techniques not only enhances the user experience but also prevents potential vulnerabilities. By utilizing validation libraries and tools effectively, developers can streamline the validation process and maintain data accuracy consistently. It is imperative to adhere to best practices to mitigate errors and safeguard sensitive information effectively.
Validation Libraries and Tools
Utilizing libraries like Validator.js
When delving into data validation, leveraging libraries like Validator.js emerges as a pivotal strategy. Validator.js offers a comprehensive suite of validation functions, simplifying the validation of various data types. Its versatility and ease of integration make it a popular choice among developers for maintaining data accuracy. The distinct feature of Validator.js lies in its extensive range of validation methods, covering a wide array of validation requirements. While its utility in this article is unquestionable, it is vital to assess the trade-offs such as possible dependencies or performance implications.
Automating validation processes
Automation plays a significant role in optimizing validation processes. By automating validation procedures, developers can streamline the validation workflow, reducing manual intervention and ensuring consistent validation standards. The key advantage of automating validation processes lies in its efficiency and accuracy, saving time and minimizing human errors. This automated approach enhances the overall validation framework, promoting a more systematic and error-free validation process. However, careful consideration of the automation strategy is essential to address any potential limitations or complexities within the validation workflow.
Regular Expression Validation
Applying regex patterns for data validation
In data validation practices, applying regular expression (regex) patterns is a powerful technique that offers a flexible and robust solution. Regex patterns enable developers to define specific validation rules, such as data formats or constraints, with precision. The key attribute of regex lies in its ability to create custom validation patterns tailored to the application's unique requirements. By incorporating regex patterns, developers can enhance validation accuracy and address complex data validation scenarios effectively. Despite its advantages, the intricacies of regex patterns may pose a learning curve and require careful testing to ensure optimal functionality.
Customizing validation rules
Customizing validation rules allows developers to tailor validation criteria to suit specific application needs. By defining custom validation rules, developers can enforce domain-specific validation constraints and enhance validation precision. The critical aspect of customizing validation rules is its adaptability to diverse validation scenarios, providing a bespoke approach to data validation. While customization offers unparalleled control over validation logic, it necessitates thorough understanding and maintenance to ensure consistent and reliable validation outcomes. Careful consideration of the trade-offs between customization and standard validation techniques is essential to determine the most effective approach for data validation.
Conclusion
Wrapping Up Insights
Importance of robust data validation practices
The bedrock of any data validation framework lies in the robustness of its validation practices. In the context of this article, the emphasis on upholding robust data validation practices underscores a meticulous approach towards fortifying web applications against data anomalies and vulnerabilities. The key characteristic that distinguishes robust data validation practices is their proactive stance in preempting data quality issues before they propagate within the system. This proactive nature not only mitigates potential risks but also instills a sense of reliability and trust in the data processes underpinning web applications.
Moreover, robust data validation practices serve as a linchpin for preempting security breaches and ensuring compliance with data integrity standards. By adhering to stringent validation protocols, developers can engender a culture of data responsibility and reliability within their applications, fostering user confidence and loyalty. While the meticulous nature of robust data validation practices may require additional development time and resources, the long-term benefits in terms of reduced errors, enhanced user experience, and fortified security posture make them a prudent investment for any web development endeavor.
Ensuring secure and reliable web applications
The mantle of ensuring secure and reliable web applications is a critical responsibility that stems directly from implementing effective data validation mechanisms. In the context of this article, the focus on this aspect underscores the integral link between data integrity, validation practices, and the overall security posture of web applications. The key characteristic that defines secure and reliable web applications is their resilience in the face of cyber threats, data breaches, and integrity compromises.
By integrating stringent validation rules, encryption protocols, and error handling mechanisms, developers can proactively fortify their applications against a myriad of security vulnerabilities, ranging from SQL injection attacks to cross-site scripting exploits. This proactive approach not only safeguards sensitive data but also fosters a culture of user trust and loyalty, essential for the sustained success of any web-based platform.
However, it is crucial to strike a balance between robust validation practices and user experience, as overly stringent validation rules may impede usability and deter users from engaging with the application. Therefore, ensuring secure and reliable web applications necessitates a nuanced approach that prioritizes data security without compromising on user accessibility and functionality.
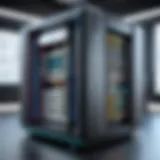
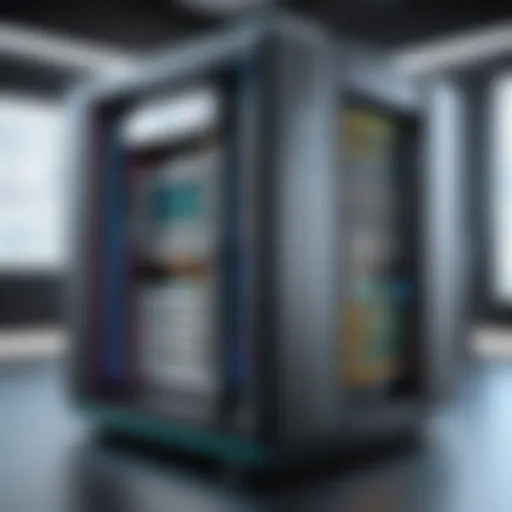