Exploring Data Structures: Key Concepts and Applications
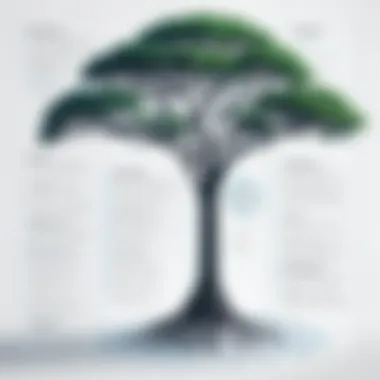
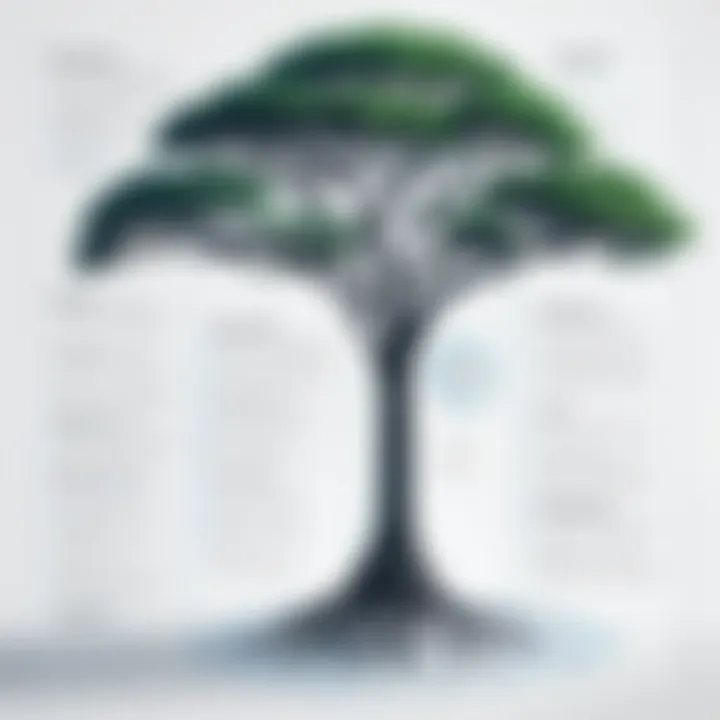
Intro
Data structures arenât just a nod to the technical; they form the backbone of effective software development. If you consider software development akin to constructing a building, then data structures represent the bricks and mortar. They allow developers to store, organize, and manipulate data efficiently. Whether one is working on a simple script or a complex application, an understanding of data structures elevates both performance and scalability.
Whatâs most fascinating is how these structures can adapt across various technologies, be it cloud computing or machine learning. Cloud systems depend heavily on data structures for organizing vast datasets across distributed networks, while machine learning algorithms thrive on efficient data manipulation. It's these intricate relationships that illustrate why mastering data structures is absolutely crucial.
Grasping the essence of these structures enables developers to make informed decisions. For instance, having a solid understanding of array versus linked lists could dictate the efficiency of the entire codebase. This leads us to explore key concepts that are indispensable for everyone, from the novice coder to the seasoned developer.
Overview of Data Structures
When we talk about data structures, itâs crucial to grasp their types. Below are the primary categories:
- Primitive Data Structures: These are the basic building blocks like integers, booleans, and characters.
- Non-Primitive Data Structures: These include more complex structures such as arrays, lists, and trees.
- Composite Data Structures: These are composed of two or more data types, such as records or structs.
Understanding these categories helps demystify the foundational aspects of data storage and manipulation. Knowing the right structure to use can not only increase performance but also enhance the maintainability of code.
"A data structure is only as good as the problem it's trying to solve."
In the forthcoming sections, we will delve deeper into each type, exploring their distinct features, functionalities, and the advantages they bring to the table. Let's take a closer look at the practical implications of these data structures in various domains of technology.
Prolusion to Data Structures
Data structures serve as the backbone of computer science, enabling the efficient organization, management, and retrieval of data. Think of them as different kinds of containers that hold information in a way that facilitates various operations. From a simple array to complex trees and graphs, each data structure has its own particular strengths and weaknesses. When choosing the right structure, developers can significantly improve the performance of any application, whether it's for searching algorithms, compiling giant datasets, or managing user information in real time.
The Importance of Data Structures in Computing
In the grand scheme of computing, data structures play an essential role in optimizing efficiency. Just consider this: if you're developing an application that requires fast access to data, the right data structure can make all the difference. For example, using a hash table can drastically reduce lookup times compared to arrays when handling large amounts of data. Moreover, the design and performance of algorithms are often closely tied to the choice of data structures, affecting everything from memory usage to execution speed.
Efficiency aside, data structures are also critical in coding practices. Utilizing them effectively contributes to maintainable and scalable code, which is vital for software that needs to adapt over time. When developers implement clear and effective data structures, they often find that it leads to cleaner code, making it easier for teams to collaborate and for future maintenance tasks to occur with minimal headaches.
"The structure of data not only impacts the outcome of programs but also shapes the way developers think about problem-solving in general."
Overview of Basic Concepts
Before diving into specific data structures, itâs crucial to grasp the basic concepts that underpin them. At the heart of all data structures are two fundamental categories: primitive and non-primitive. Primitive data structures include the basic types like integers, floats, and characters, which are the building blocks for more complex structures. Non-primitive data structures, on the other hand, encompass collections such as arrays, linked lists, and trees.
Understanding these concepts involves recognizing how data is stored in memory and how various structures manipulate that data. Hereâs a quick rundown:
- Primitive Data Structures: Data types that serve as the basic building blocks. They are typically defined by the programming language.
- Non-Primitive Data Structures: These structures combine primitive data types to form more complex models, allowing for more sophisticated data manipulation and organization.
When a developer is well-versed in these foundational concepts, they gain a better grasp of how to apply data structures effectively for various programming challenges. This foundation sets the stage for exploring more advanced data structures, which will be covered in the coming sections.
Fundamental Types of Data Structures
When diving into the realm of data structures, it can be likened to stepping into a vast library filled with various genres of information. Each section plays a critical role, but none is as foundational as the one comprising the fundamental types of data structures. These are the building blocks upon which more complex forms are built. Understanding these basics lays the groundwork for effective programming and data management, allowing developers to make informed decisions that optimize performance and resource utilization.
In this section, we will examine both primitive and non-primitive data structures, recognizing their unique traits and applications. Each type serves different purposes, and knowing when to apply the correct structure can make or break an applicationâs efficiency.
Primitive Data Structures
Prolusion to Basic Data Types
Basic data types are the simplest forms of data structures and act as the foundation in programming languages. Think of them like the letters of an alphabet â each letter has its own significance, and when combined, they create words and sentences. The most common types include integers, floats, characters, and booleans. One might argue their huge benefit comes from their efficiency, generally using minimal memory. In this guide, they represent a starting point that helps beginners grasp more complex concepts.
However, these basic types come with limitations. They are immutable in many languages, meaning once created, they cannot be changed. This can be a double-edged sword, as it provides safety from accidental modification but also poses restrictions in more dynamic applications.
Understanding Arrays
Arrays are a step up from basic data types. They enable the storage of multiple items of the same type in a contiguous block of memory. This feature provides fast access times, which is a key characteristic. When accessing an element in an array, itâs generally a simple calculation based on its index, making it a popular choice for many developers.
However, a notable drawback of arrays is their fixed size. Once an array is defined, its size cannot be altered without creating a new array. This becomes cumbersome when the size of the data is uncertain. Additionally, inserting or removing elements can be less efficient as it may require shifting other elements.
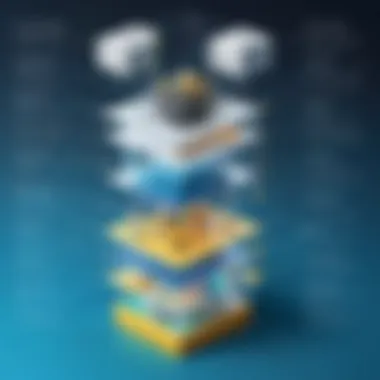
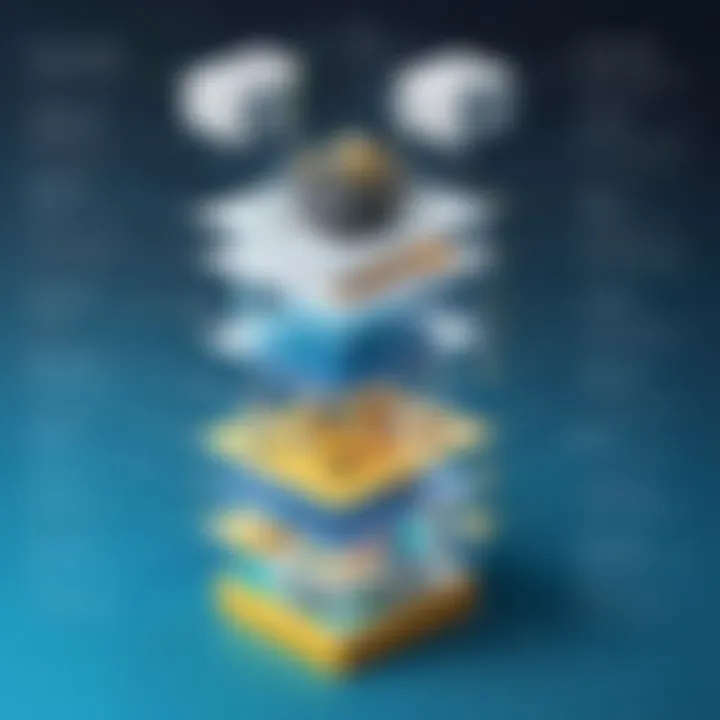
Exploring Strings
Strings, often considered a specialized array of characters, are invaluable for handling text data. They allow not only the storage but also manipulation of textual information. The flexibility of strings comes from their ability to grow and shrink dynamically, making them a handy tool for developers dealing with variable-length text.
Yet, with this flexibility also comes some challenges. Strings can consume a fair amount more memory compared to their character array counterparts, particularly in languages where strings are stored as objects. This can lead to performance issues if not managed properly.
Non-Primitive Data Structures
Understanding Structures and Unions
Structures provide a way to group multiple data types under one name. This feature allows for more complex data representation. For instance, in programming a simple address, one might combine strings and integers representing street name, house number, and zip code into a single structure. This packaging acts as a robust method to manage related data cohesively.
On the flip side, unions serve a similar purpose but with a twist. They allow storing different data types in the same memory location but only one at a time. This unique feature makes unions memory efficient, as they take up the size of the largest member only. However, it also means you must carefully manage what type of data is currently stored to avoid data corruption.
Exploring Linked Lists
Linked lists offer an alternative to arrays, especially in scenarios where dynamic memory allocation is necessary. Each element, called a node, contains a value and a reference to the next node in the sequence. Linked lists provide substantial benefit in terms of insertion and deletion operations, allowing for manipulations without the need to shift elements.
However, the key characteristic that can be seen as a downside is the increased memory usage. Each node adds additional overhead for storing pointer information, leading to less memory efficiency compared to arrays, particularly when storing a small number of items.
Preface to Stacks and Queues
Stacks and queues provide structured access to data, each employing a different approach. A stack follows Last In First Out (LIFO) principles, making it useful in scenarios like function call management in programming languages. This structure allows for quick access to the last element added, but it also limits external access to only this top element.
In contrast, queues operate on a First In First Out (FIFO) basis. This method suits situations where the order of processing is vital, similar to how people queue in a grocery store. Both structures are easy to implement and wield significant utility in both simple and complex applications. Nonetheless, as with any structured approach, careful consideration must be given to their limitations in access and flexibility.
Understanding these fundamental types of data structures is crucial for anyone looking to become proficient in programming and data management. They govern how data is organized, accessed, and manipulated.
In summary, the fundamental types of data structures establish the initial pathway into the more intricate landscapes of computer science. Their impact stretches beyond mere storage, permeating through the logic and efficiency of algorithms and applications.
Advanced Data Structures
Understanding advanced data structures is essential for anyone looking to go beyond the basics of programming and data management. These structures, like trees and graphs, offer unique capabilities that serve a variety of computing needs. They optimize data storage, enhance performance in algorithms, and enable more complex data interactions. By grasping the nuances of advanced data structures, practitioners can craft more efficient programs, making the most of both time and memory resources. With software applications becoming increasingly complex, the tools provided by these data organization methods are more relevant than ever.
Trees
Binary Trees Explained
Binary trees are a fundamental concept in computer science, representing a structure where each node has at most two children, referred to as the left and right children. The simplicity of this arrangement is a key characteristic, making binary trees a popular choice for various algorithms. One standout feature of binary trees is their ability to facilitate quick searches, as the structure allows for log(n) time complexity in balanced scenarios. This efficiency is exceptionally advantageous in applications like database systems and hierarchical data representation, leading to speedier data retrieval processes.
However, ideal performance relies on balancing, as unbalanced trees can devolve into linked lists, severely compromising efficiency. Debugging and maintaining balance, although somewhat problematic, is vital for leveraging the potential of binary trees optimally.
Balanced Trees: AVL and Red-Black Trees
Balanced trees, such as AVL and Red-Black trees, build on the concepts of binary trees by enforcing rules that maintain height balance, allowing for more predictable performance. The defining characteristic of these trees is their self-balancing nature, which guarantees that operations like insertion, deletion, and lookup run in O(log n) time, improving efficiency.
AVL trees are particularly strict about balance, maintaining a difference of at most one between the heights of subtrees. This rigidity provides faster lookups at the cost of more involved insertion and deletion operations, reflecting a necessity to maintain balance.
On the flip side, Red-Black Trees are less constraining and allow for more flexibility in balancing. This adaptability comes with the trade-off of potentially slower lookups but offers faster insertion and deletion, which can be beneficial in dynamic systems requiring frequent updates.
Applications of Trees in Computing
The applications of trees are extensive and quite vital in computing environments. One primary use is in representing hierarchical data, such as file systems or organizational structures, enabling intuitive navigation and management. Furthermore, many algorithms rely on trees for efficient sorting and searching functionalities.
For instance, Trie structures are implemented in applications like auto-suggestions and spell checkers. These trees, designed for storing strings efficiently, allow quick retrieval based on prefixes. Another practical application is in Binary Search Trees which facilitate quick data lookup by maintaining a sorted order among the elements. However, the effectiveness of trees significantly depends on maintaining balance to avoid degradation in performance.
Graphs
Preamble to Graph Theory
Graph theory lays the groundwork for understanding complex relationships between entities represented as nodes connected by edges. This area of study has wide relevance in computer science for modeling various systems, from social networks to network routing protocols. The key characteristic of graph theory is its ability to demonstrate how items relate and influence one another, offering insights into the structural dynamics of interconnected systems.
Using this approach helps elucidate concepts like connectivity and pathfinding, valuable in scenarios like logistics, route planning, and resource management. Yet, with increased complexity, such as cycles and diverse edge weights, graph algorithms often require nuanced, sophisticated methods to effectively navigate the intricacies of the network.
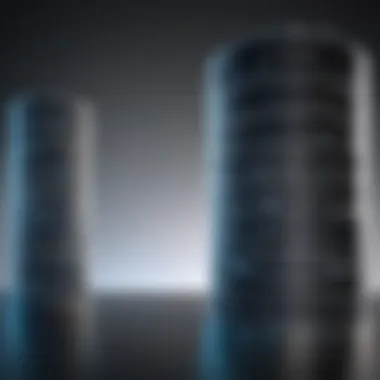
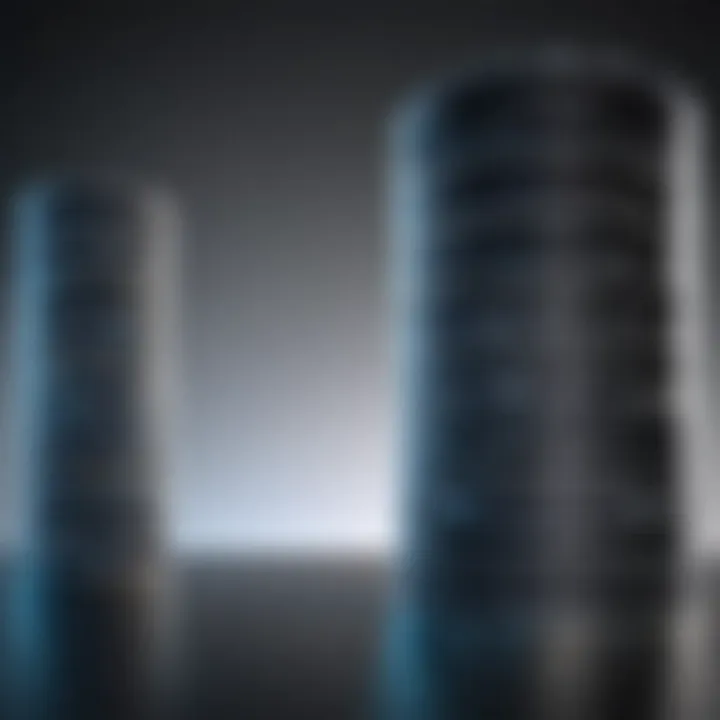
Types of Graphs
Various types of graphs exist, ranging from directed graphs to undirected graphs, each suited to different use cases. Directed graphs (digraphs) involve edges with orientation, encapsulating flows like one-way streets or social media interactions. Meanwhile, undirected graphs denote mutual relationships, useful in understanding bi-directional connections.
Another variant, weighted graphs, assigns weights to edges, reflecting costs or distances. This feature becomes critical in pathfinding algorithms like Dijkstra's, efficiently identifying the shortest route amid complexity. The choice of graph type is often dictated by the specific problem being solved and the relationships being modeled, serving as a roadmap to effective solutions.
Graph Traversal Techniques
Graph traversal techniques like Depth-First Search (DFS) and Breadth-First Search (BFS) play a crucial role in exploring nodes and edges systematically. The key characteristic of these techniques is their strategy for visiting nodes: DFS dives deep into the graph's structure, while BFS broadens the search layer by layer.
Both techniques exhibit distinct advantages depending on the goals. For example, DFS is memory-efficient for large graphs and can readily uncover deeply nested structures. On the other hand, BFS is instrumental in finding shortest paths in unweighted graphs, showcasing its utility in basic networking issues.
Ultimately, grasping these graph traversal methods enriches one's understanding of how to optimize data flow and analyze complex relationships across varied applications.
Choosing the Right Data Structure
Selecting the appropriate data structure is much like choosing the right tool for a job. It is a crucial step that significantly impacts the efficiency and performance of your algorithms. Whether you are developing sophisticated applications or processing vast datasets, making the right choice can streamline your processes, reduce resource consumption, and enhance overall productivity. This section delves into the elements that guide this selection, exploring the benefits and considerations involved in picking the most suitable data structure for different scenarios.
Factors Influencing Data Structure Selection
Time Complexity Considerations
Time complexity measures the amount of time an algorithm takes to complete as a function of the length of the input. It fundamentally influences how performant a data structure can be in practice. When you're choosing a data structure, you want to consider not just the average case, but the worst-case performance as well. The key characteristic of time complexity is its use of Big O notation. This notational system gives you a way to describe the upper limits of efficiency, letting you know how an algorithm will scale.
For instance, using a hash table allows for average-case constant time complexity for lookups, making it a popular choice when speed is essential. On the downside, if the load factor gets too high, performance may degrade, leading it to approach linear time complexity in the worst case. This unique feature of hash tables, coupled with the need to handle collisions, is something to consider when making your choice.
"The biggest misconception is that all data structures work equally well for all scenarios. You need to think about how you use data on a granular level."
Space Complexity and Memory Management
Space complexity, on the other hand, deals with the amount of memory a data structure requires relative to the size of its input. This aspect is just as critical as time complexity because memory efficiency can be the difference between a responsive application and one that lags or crashes. Generally, understanding how your data structures utilize memory can save you a headache further down the line.
For example, an array is a fixed-size data structure and can lead to significant waste of memory if not utilized effectively. On the contrary, linked lists allow dynamic memory allocation, but they come with their trade-offs, like higher overhead due to pointer storage. The unique feature of linked lists provides flexibility while potentially introducing performance overhead, making them not universally suitable for every application.
Common Use Cases
Data Storage Systems
In modern computing, data storage systems are the backbone of how applications function. Choosing the right data structure for data storage can lead to optimal retrieval times and efficiencies in storage. The key characteristic here is how certain structures such as trees or databases are designed to minimize time spent searching for data, maximizing the speed of access.
For instance, B-trees are excellent for database storage as they allow for efficient insertion, deletion, and lookups, especially with large datasets. This makes them a favorable choice when working with disk storage as their design minimizes the number of reads and writes to disk, an expensive operation in terms of time. Still, the complexity tied to maintaining balance in B-trees can be a hurdle when implementing them.
Algorithms and Processing Techniques
Algorithms and processing techniques are inexorably linked to the data structures they're built upon. The selection often depends on what you need to accomplish. For example, if you're often processing sequential data, a queue might be an ideal fit due to its FIFO (First In First Out) behavior, making it a natural choice in scenarios like task scheduling or print spooling.
An additional consideration is the type of data manipulations required; some structures are more suited for frequent insertions, while others excel in retrieval. A good understanding of these operational characteristics will guide you toward more efficient algorithm implementation, saving precious computational resources in the long run.
As you can see, the decision-making process in selecting the right data structure is both nuanced and vital. Don't take it lightly; the right data structure can make a world of difference.
Best Practices in Data Structure Implementation
When it comes to implementing data structures, navigating the forest of choices requires not just clarity but a strategic vision. Best practices in this domain are paramount, serving as guiding principles that foster efficiency, maintainability, and scalability. These practices help ensure that developers don't just throw code together haphazardly but construct strong, resilient frameworks that stand the test of time. Ignoring these methodologies can lead to messy, inefficient code and barriers in communication among development teams.
Code Efficiency and Readability
Following Coding Standards
Following coding standards is like laying down the law and establishing a set of rules everyone in a team agrees on. These standards guide the writing and formatting of code, ensuring that it remains consistent across different parts of a project. This approach makes it easier for developers to read and understand each otherâs work. Adopting established coding standards reduces cognitive load, making it easier to spot errors, collaborate effectively, and onboard new team members without much ado.
One key characteristic of following coding standards is their role in fostering collaboration. By adhering to conventions, team members can quickly familiarize themselves with the codebase. A popular choice among developers is the use of style guides like Googleâs or Airbnbâs JavaScript Style Guide, which offer structured examples of consistent coding practices.
However, a potential drawback might arise from becoming overly rigid with adherence to standards. At times, such strict adherence may stifle creativity or lead to unnecessary complexity. Just as itâs vital to stay within the bounds of conventions, remaining adaptable to how standards evolve is equally important.
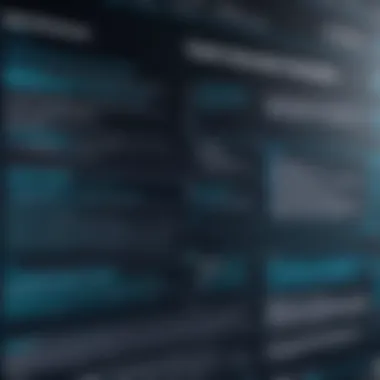
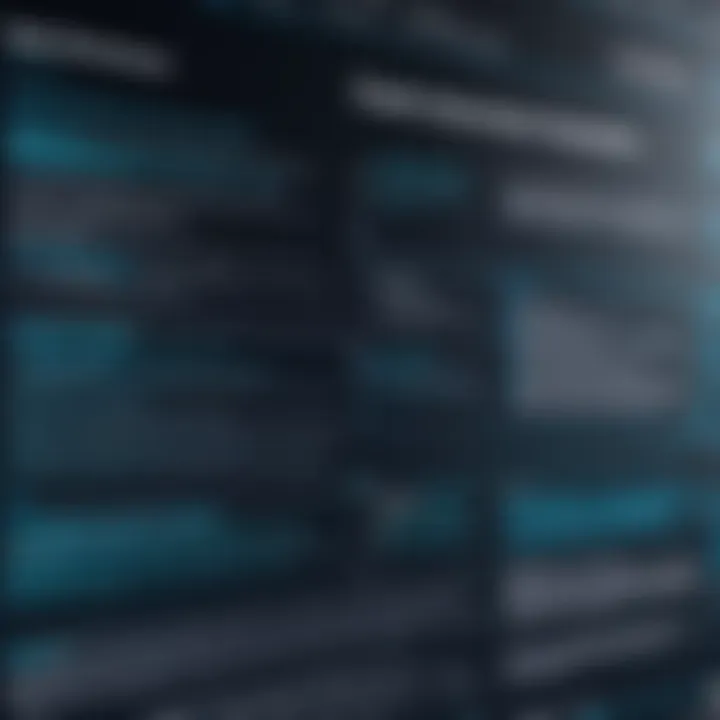
Commenting and Documenting Code
Commenting and documenting code act as the safety net during development. This practice involves adding explanatory notes to code, describing the intent behind complex algorithms or even simple lines of code. Documentation is particularly invaluable when revisiting code after a lengthy hiatus. It acts as a roadmap for the mind, allowing easier navigation through complex thoughts.
One major advantage of this practice is that it facilitates knowledge transfer among team members. When developers comment their code adequately, it becomes smoother for others to pick up where someone else left off and avoids countless hours of deciphering cryptic logical paths.
On the flip side, excessive or unclear comments can clutter the code and lead to confusion rather than clarity. Striking the right balanceâoffering sufficient insight without bogging down the reading experienceâis crucial.
Testing and Validation
Unit Testing for Data Structures
Testing acts as the guardian of reliability in software development, and unit testing specifically scrutinizes individual components of data structures. This focused approach ensures that each part functions as intended, independent of the rest of the codebase. By proactively identifying bugs within a controlled scope, developers can avoid those slippery little problems that might wreak havoc later down the road.
A notable feature of unit testing for data structures is its ability to promote confidence in code changes. Developers can make modifications without trepidation, knowing that the tests they write will catch any unintended effects. This methodology sets a foundation for better collaboration, as team members can work on different areas without inadvertently stepping on each other's toes.
Unfortunately, while testing provides immense benefits, it also requires a throw of dedication and time investment. Quality unit tests must be written alongside or after the code, and neglecting to prioritize them can lead to a jeans-rolling-up-the-ankles mess later in the project.
Benchmarking Performance
Benchmarking performance is akin to taking a car out for a spin and measuring its speed against a standard track. When implementing data structures, benchmarking helps assess their efficiency under certain conditions. This process often involves measuring parameters like bit usage, speed of access, or the time taken to modify data. By establishing baseline metrics, developers can make informed choices regarding which data structures are ideal for a given application.
One of its key characteristics lies in its ability to surface issues that may not be visible during regular code review. Developers can pinpoint bottlenecks that stand to slow down application performance, allowing targeted optimization.
On the downside, benchmarking requires time and expertise. It can also lead to a misunderstanding if developers focus too narrowly on performance at the expense of clarity and maintainability. Focusing on the balance among efficiency, clarity, and maintainability is fundamental in this aspect.
By adhering to best practices, you arm yourself with the tools necessary to craft strong, effective data structures, making a realm of difference in both individual projects and collaborative environments.
Future Trends in Data Structures
The realm of data structures is evolving at a rapid pace. With the advancement of technology and the growing amounts of data generated daily, it's crucial to stay informed about the trends that shape the future of data management. Not only do these trends influence how we design and implement data structures, but they also underscore the necessity for adaptability in our approaches to data handling.
Emerging Technologies
Emerging technologies are currently revolutionizing the way data structures are organized and utilized. Consider, for example, the rise of quantum computing. This new frontier isn't just a sci-fi dream anymore; itâs becoming a reality with profound implications for data structures. Quantum computers utilize quantum bits, or qubits, that can hold multiple states simultaneously, making traditional data structures less efficient for processing such complex data. As a result, new data structures specifically designed to take advantage of quantum propertiesâlike quantum trees or quantum hash tablesâare becoming essential.
Moreover, developments in blockchain technology are creating new paradigms for data structures. Traditional database architectures often don't translate well to decentralized systems where data integrity and transparency are paramount. In blockchain, data structures such as Merkle trees and Directed Acyclic Graphs (DAGs) serve to ensure secure and efficient data storage and retrieval.
By embracing these technologies, you're not just keeping up with the changes; you're positioning yourself to leverage these innovations for greater computational efficiency and integrity.
The Role of Data Structures in Machine Learning
In the world of machine learning, data structures play a cornerstone role. As algorithms digest large datasets, the choice of data structures can significantly affect performance and the quality of outcomes. TensorFlow and PyTorch are prime examples of frameworks that rely heavily on efficient data structures to handle tensorsâmulti-dimensional arrays that can encapsulate vast amounts of data needed for machine learning tasks.
When developing machine learning applications, the efficiency of data access and processing can be enhanced by using the right data structure. For example, utilizing k-d trees for organizing points in a k-dimensional space helps streamline nearest neighbor searches, a common operation in pattern recognition tasks.
Often overlooked, feature engineering methodologies greatly depend on the underlying data structures. For instance, if your data is nested or hierarchical, structured data representations (like trees or graphs) can facilitate the extraction of features more efficiently compared to flat structures. Enhancing the quality of your data inputs translates directly to better-performing models, thus highlighting how intertwined data structure choice and model performance really are.
"Selecting the appropriate data structure is not just a technical choice; it's a crucial strategy that influences the efficacy of machine learning outcomes."
As the demand for advanced analytical capabilities continues to grow, understanding these trends and their practical implications equips software developers, IT professionals, and data scientists with the knowledge needed to adapt and thrive in a constantly changing landscape. In this era of information chaos, being adept in the use of modern data structures can set you apart from the crowd.
Ending
Wrapping it all up, this article stirred up a whole lot of ground regarding data structures. It's not just a bunch of abstract concepts; understanding data structures means unlocking a crucial part of computer science. They form the backbone for algorithms, influence performance, and make managing data more effective. Each type of data structure, whether itâs trees, graphs, or linked lists, has its unique quirks and use cases. Choosing the right one can save time and effort down the road. When developers know how data structures operate, they can write better code that stands the test of time.
Recap of Key Points
Looking back, here's a simple rundown of what weâve covered:
- Importance in Computing: Data structures are essential for efficient data management and algorithm implementation.
- Types of Structures: We looked into basic varieties, like arrays and strings, as well as more complex constructs such as trees and graphs.
- Choosing Wisely: The choice between different data structures boils down to time and space considerations.
- Best Practices: Following code efficiency and proper testing methods ensures implementation stays robust.
- Future Trends: The emerging tech realm sees data structures shaping the machinery of machine learning and big data analytics.
Encouragement for Further Study
If youâre feeling sparked by all this, thatâs fantastic! Data structures hold endless depths waiting to be explored. Advancing your knowledge in this area not only benefits your current work but also broadens your career opportunities in software development and data science. Consider diving into specialized resources, such as online courses or interactive coding challenges, that focus on data structures in memory management or system performance.
"Learning is a treasure that will follow its owner everywhere.â
Explore communities online, like helpful forums on Reddit or specialized groups on Facebook, where you can ask questions and share experiences with other tech enthusiasts. Engaging with the broader community can provide insights that textbooks often miss. Keep pushing forwardâevery bit of knowledge counts in the fascinating world of data structures!