Exploring the C Coding Language: A Comprehensive Overview
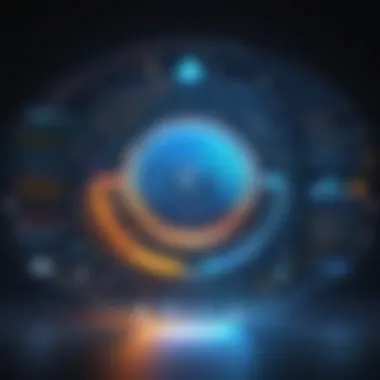
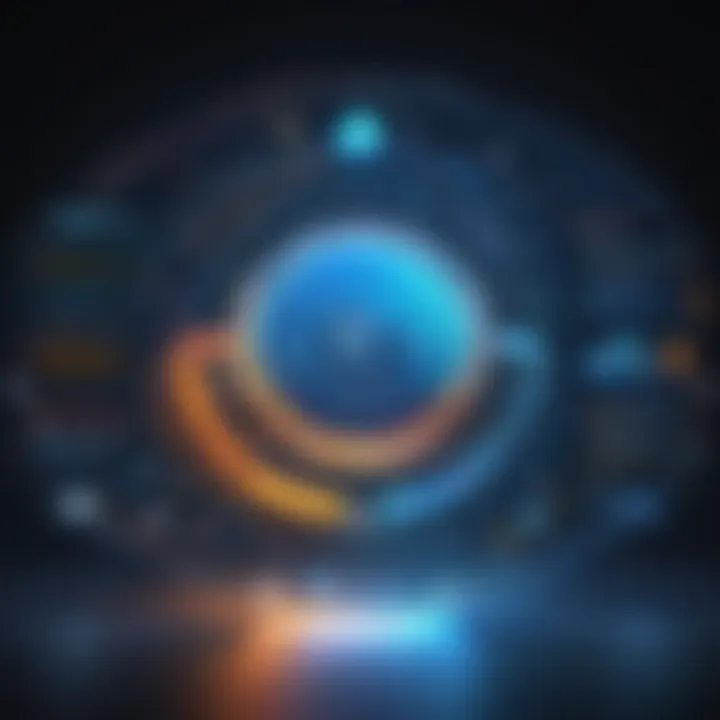
Intro
The C programming language has been a cornerstone of software development since its inception in the early 1970s. Created by Dennis Ritchie at Bell Labs, C has laid the foundation for many modern programming languages. Its simplicity, efficiency, and versatility make it relevant in various domains, from system software to application development.
This article aims to provide a thorough exploration of C, emphasizing its history, fundamental concepts, and current relevance. It is essential for developers to understand not only the language itself but also the programming constructs and practices it encompasses. By dissecting its structure and syntax, we will unveil the numerous applications and best practices associated with C programming. Let's embark on this journey together.
Foreword to Coding Language
The C programming language is often regarded as one of the most influential and foundational languages in the field of computer science. Understanding C is crucial for anyone venturing into software development or system programming. This segment presents an in-depth introduction to the C coding language, highlighting its historical roots and contemporary significance.
Historical Context and Evolution
C was developed in the early 1970s by Dennis Ritchie at Bell Labs. Its origin can be traced back to the B programming language, which itself was derived from an earlier language called BCPL. C was designed to address the needs of system programming, particularly for developing the Unix operating system, which further propelled its adoption and evolution.
Over the years, C has undergone several enhancements, leading to the ANSI C standard in 1989 and the ISO C standard in 1999. Each of these updates reflected the changing needs of the computing landscape. The language has not only retained its relevance but also served as a stepping stone for many modern programming languages, including C++, C#, and Objective-C. The evolution of C mirrors the growth of computing technology, cementing its status as a timeless resource for learners and practitioners.
Significance in Computer Science
C plays an indispensable role in computer science and programming education. It serves as the groundwork for understanding more complex languages and concepts. Notably, many classic algorithms and data structures are implemented in C, offering learners a clear view of how programming works at a low level.
Key reasons for C's significance include:
- Efficiency: C is known for its performance and reliability. The language provides low-level access to memory, allowing developers to write programs that are both fast and efficient.
- Portability: Code written in C can be compiled on various platforms with minimal changes, making it versatile across different types of systems.
- Control: It gives programmers control over system resources, which is especially important in systems programming and embedded systems.
C not only educates programmers in the basics of coding but also fosters skills essential for higher-level programming.
In summary, the introduction to the C programming language lays the foundation for a comprehensive exploration into its core concepts, structures, and applications. By delving into its history and enduring relevance, this article aims to equip software developers, IT professionals, and tech enthusiasts with valuable insights into mastering C.
Fundamental Concepts of
The fundamental concepts of the C programming language lay the groundwork for understanding its capabilities and applications. These core elements form the basis of C and highlight the language's power and flexibility. A solid grasp of these concepts is essential for any programmer aiming to write efficient, maintainable code.
Variables and Data Types
Primitive Data Types
Primitive data types serve as the building blocks of data manipulation in C. They include types like , , , and . Each type has specific memory allocation and range, which is vital for optimization. The key characteristic of primitive data types is their simplicity and efficiency in representing basic data values. This makes them a favored choice in performance-sensitive applications.
The unique feature of primitive data types is their direct representation in memory. This allows developers to create highly performant programs, as operations on these types generally require fewer resources. However, programmers should be mindful of type limits, as overflowing these can lead to undefined behavior.
User-Defined Data Types
User-defined data types allow for greater complexity and organization in C programming. By combining primitive types, developers can create structures, enums, and unions that better represent real-world entities. The key characteristic of user-defined data types is their flexibility, providing a method to group and manage data logically and effectively.
The unique feature of user-defined types lies in their ability to encapsulate data. This can improve code readability and maintainability, as programmers can define tailored structures for specific needs. However, the drawback is that misuse or improper understanding can lead to complex and less efficient code.
Operators and Expressions
Arithmetic Operators
Arithmetic operators are essential for performing mathematical calculations in C. They include , , , , and . The key characteristic of arithmetic operators is their ability to execute fundamental operations. This makes them a critical choice for countless applications, from simple calculations to complex algorithms.
The unique feature of arithmetic operators is their operation on numeric values to yield another numeric result. This simplicity is advantageous, but care must be taken to manage division by zero, which can cause runtime errors.
Logical Operators
Logical operators, such as , , and , are used to combine conditional statements. Their main purpose is to control the flow of a program based on boolean logic. The key characteristic of logical operators is their role in decision-making processes, which is crucial for implementing complex logic in applications. They are a necessary choice for situations where multiple conditions must be evaluated.
The unique feature here lies in combining or negating boolean expressions, which enhances control flow precision. Their disadvantage might arise from potential misunderstandings of operator precedence, which can lead to unintended logical errors.
Bitwise Operators
Bitwise operators manipulate data at the binary level, allowing for efficient storage and operations on data. Operators like , , , , ``, and provide powerful functionality. The key characteristic of bitwise operators is their direct manipulation of bits, which can lead to enhanced performance in scenarios requiring low-level data manipulation.
This unique feature allows for operations like masking, shifting, and setting bits. While this offers flexibility and efficiency, it also demands a comprehensive understanding of binary representation, which can intimidate some developers.
Control Flow Statements
Conditional Statements
Conditional statements are the backbone of flow control in C. They include the , , and constructs. The key characteristic of conditional statements is their ability to direct the execution flow based on specific conditions. This makes them a foundational aspect of C programming.
What stands out in conditional statements is their simplicity. By evaluating conditions, they execute different code blocks based on the result. However, overusing them without clear structure can lead to cluttered and hard-to-read code.
Loops (for, while, do-while)
Loops are used to execute a block of code repeatedly based on a condition. The , , and loops allow programmers to iterate efficiently. The key characteristic of loops is their capacity for repetition, which is essential for tasks that require multiple executions.
The unique feature of loops is their ability to reduce code redundancy. By using loops, developers can minimize the potential for errors. Nevertheless, care must be taken to avoid infinite loops, which can cause programs to hang.
Switch Statement
The switch statement offers an alternative to long chains of conditional statements. It provides a way to execute different code blocks based on the value of a variable. The key characteristic of the switch statement is its clarity and organization in handling multiple conditions. This makes it a preferred choice when dealing with numerous possible cases.
What is notable about the switch statement is its ability to improve code readability. However, it may not be suitable for all scenarios, especially those requiring complex conditions, which can lead to limitations in its application.
Programming Constructs in
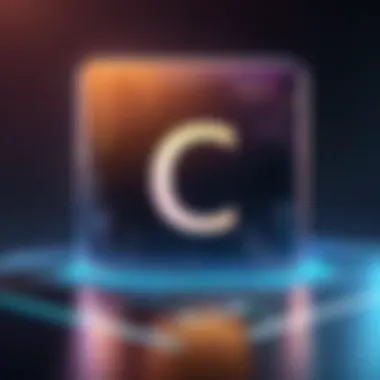
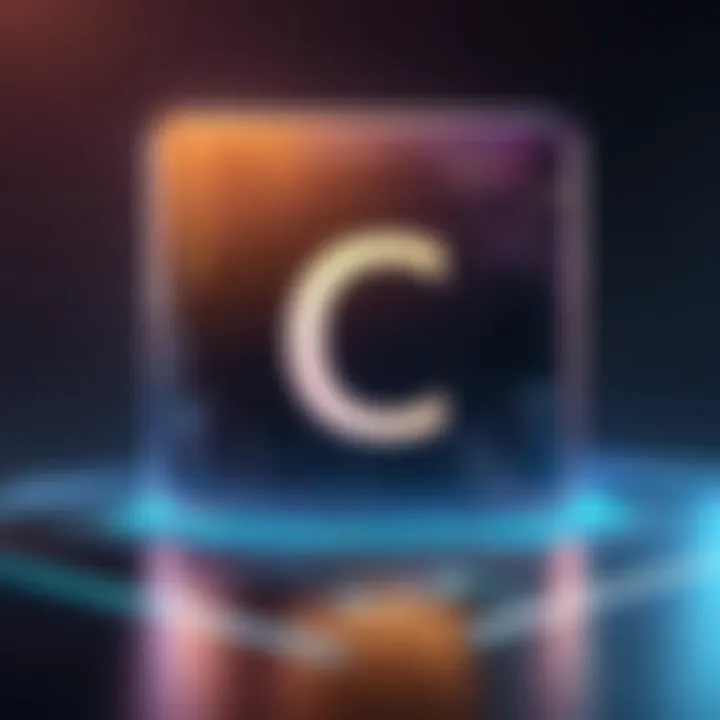
Programming constructs in C are fundamental building blocks that allow developers to write efficient, understandable, and modular code. Understanding these constructs is essential for anyone looking to harness the power of C programming effectively. This section will explore various constructs such as functions, pointers, and structures, highlighting their importance, unique features, and the role they play in advancing the C coding language.
Functions and Modular Programming
Defining Functions
Defining functions is a core aspect of C programming. A function is a block of code that performs a specific task. This approach promotes modularity, making it easier to manage and debug code. The key characteristic is its ability to encapsulate behavior, allowing code reusability. It is a beneficial choice for this article because it simplifies complex tasks into smaller, more manageable parts.
The unique feature of defining functions is their ability to take inputs and produce outputs. When programmers use functions, they can reduce redundancy in code, although overuse may lead to unwieldy function calls that complicate understanding.
Function Parameters
Function parameters enhance the flexibility of functions. Parameters allow functions to accept data inputs, enabling more dynamic code execution. A significant reason for their popularity is their capacity to handle various data inputs, supporting generalized programming. This gives programmers the ability to write more versatile code.
Function parameters have unique characteristics. They can be of different data types, including integers, floats, or even user-defined types. However, they can complicate function signatures, which may lead to miscommunication regarding the expected input types.
Return Types
Return types specify what type of value a function will return to its caller. This aspect is crucial for ensuring that functions are used correctly in a program. The defining characteristic is that it clearly indicates the output type, aiding in type safety and reducing runtime errors.
The uniqueness of return types lies in their ability to enforce return value checking, which enhances the behavior of the code. However, not defining a return type (defaulting to ) can lead to unintended consequences and errors, making proper declaration essential.
Pointers and Memory Management
Pointer Basics
Pointers are a powerful feature of C programming. They store memory addresses instead of actual data. A key characteristic is that they provide direct access to memory, enabling efficient data manipulation and resource management. This makes pointers a beneficial topic for this article as they play a vital role in dynamic data structures.
One unique feature of pointers is their ability to create complex data relationships, such as linked lists or trees. However, they also pose risks, such as invalid memory access, which can lead to application crashes or security vulnerabilities.
Dynamic Memory Allocation
Dynamic memory allocation allows programmers to request memory at runtime. This flexibility is essential for handling varying sizes of data. The main characteristic is that memory can be allocated and freed as needed, enhancing resource management and adaptability in applications.
Dynamic memory allocation's unique feature is the ability to allocate large chunks of memory efficiently, especially for data structures whose size is not known at compile time. However, it can lead to memory leaks if not managed correctly, posing significant challenges in applications.
Structures and Unions
Defining Structures
Defining structures allows programmers to group related variables under a single name, creating complex data types. This is crucial for organizing and managing data effectively. The significant characteristic is the ability to encapsulate various data types within a single entity, making it easier to handle related information.
Structures are beneficial in this article because they enhance code readability and maintainability as they represent real-world entities. However, they can introduce complexity in terms of memory usage and management, especially when dealing with large structures.
Using Unions
Unions are similar to structures but allow different data types to occupy the same memory space. A key characteristic is memory efficiency since they use less memory than structures by allocating space for the largest member only. This is particularly beneficial when dealing with large data comprehensive systems.
The unique feature is their ability to store one of several types at any given time. This can lead to easier representation of data models but also increases the risk of data corruption if not carefully managed.
"Programming constructs like functions, pointers, and structures are not just essential for coding in C but are also crucial in developing effective programming skills that translate into better software solutions."
Understanding these programming constructs equips developers to write cleaner, more efficient, and modular code. By mastering functions, pointers, structures, and unions, programmers can significantly enhance their coding practices and contribute to more stable software development.
Advanced Topics in
Advanced topics in C are essential for understanding the full capability and scope of the language. These topics address various complex programming practices that elevate a developer's ability to write efficient and maintainable code. By delving into advanced features, one can expand their programming toolkit, allowing for enhanced functionality and optimization in C projects. Each subheading below focuses on key aspects of advanced C programming.
File Handling in
File handling is a fundamental concept in C that allows the program to read from and write to files on the system. This capability is crucial in many applications where data persistence is needed.
Reading from Files
Reading from files in C is a straightforward but vital operation. It enables programs to access existing data, making it a common feature for applications needing to process large datasets.
One key characteristic of reading from files in C is the use of file pointers, which track the current location in the file being read. This method allows for efficient navigation and retrieval of information. The function is typically the starting point for opening a file, while functions like , , and are used for actual data retrieval.
The ability to read from files is advantageous because it allows developers to handle large amounts of data without hard-coding values directly into the program. However, care must be taken to manage file pointers and buffer sizes to avoid unexpected behaviors or crashes. Failure to read data correctly can lead to issues in the application's logic, which may hinder user experience.
Writing to Files
Similar to reading, writing to files is a crucial aspect of file handling. It allows programs to store data for later retrieval, which is especially important in scenarios that require logging information or saving user progress.
The primary operations for writing to files involve the , , and functions. These allow structured and unstructured data to be saved effectively. One of the notable features of writing to files is that it can replace existing data or append to it, offering flexibility in how data is stored.
In this article, writing to files presents the benefit of data persistence, enabling applications to maintain state between runs. However, developers must ensure that they handle write operations carefully to prevent data loss. Improperly closing files or overwriting important information can create significant issues in applications.
Preprocessor Directives
Preprocessor directives play an integral role in C programming. They enable conditional compilation, macro definitions, and file inclusion, which help in creating more modular and adaptable code.
Macros
Macros are a powerful feature in C that allows developers to define repetitive code segments or constants with a simple keyword. The directive declares these macros, simplifying code and enhancing readability.
The primary advantage of macros is the reduction of redundancy within the code. For instance, defining mathematical constants as macros means they can be used throughout the application simply by referring to their name rather than rewriting their value.
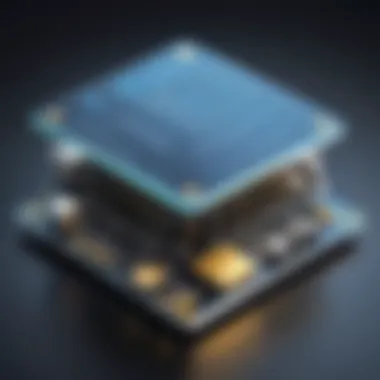
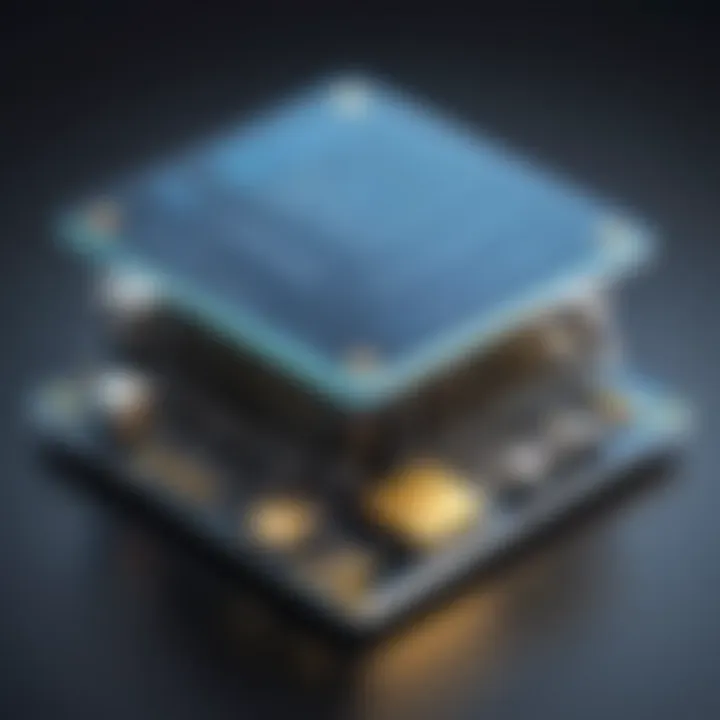
However, macros can also complicate debugging, as they may obscure the code flow. If not carefully used, they can introduce unexpected behaviors or errors, especially when there are naming conflicts or unintended expansions. Thus, while macros provide benefits, they ought to be used judiciously.
Conditional Compilation
Conditional compilation allows different sections of code to be included or excluded during the compilation process based on defined conditions. This is particularly useful for cross-platform development or creating debugging versus release versions of software.
Using directives like , , and , developers can control what parts of the code are compiled. Its main benefit is the ability to create flexible and portable code that works across various environments or configurations.
However, overusing conditional compilation can lead to cluttered code and can hinder maintenance as complexity increases. It’s essential to balance its use with clarity in the codebase.
Error Handling and Debugging Techniques
Error handling and debugging are essential aspects of software development that ensure programs run smoothly and efficiently.
Common Errors
Common errors in C programming can stem from various sources, such as syntax issues, logical mistakes, or memory management failures. Knowing these common pitfalls is vital for any developer.
For instance, memory leaks occur when a program allocates memory without deallocating it correctly, leading to inefficient use of system resources. Debugging tools and practices, like using , can help identify these memory issues during program execution.
Awareness of common errors aids developers in writing better code and improving overall reliability. Ignoring these issues, however, can result in unpredictable program behavior, making software harder to maintain.
Debugging Tools
Debugging tools are instrumental in the development process, allowing for tracking variable states, monitoring memory use, and stepping through code execution.
Tools like , the GNU Debugger, enable developers to inspect the operation of their program in real-time. Going through the code line-by-line can help isolate problems that would otherwise be hard to detect. The key advantage of using debugging tools is that they provide insights into the program's running state, which is invaluable during troubleshooting.
On the downside, there is a learning curve associated with these tools, and improper usage may lead to confusion if developers are not familiar with their features. Nevertheless, effectively using debugging tools is vital for any serious C programmer.
in Modern Software Development
C programming continues to hold significant importance in the landscape of modern software development. Its design principles and efficiency make it an ideal choice for various applications, from system-level programming to embedded systems. Many developers appreciate C for its performance and resource management, allowing them to write code that interacts closely with hardware. This section explores the applications of C in different fields and provides a comparison with other programming languages.
Applications of Language
Operating Systems
The role of C in developing operating systems cannot be overstated. Most operating systems, including Unix and Linux, are written primarily in C. One of the key characteristics of operating systems that utilize C is their ability to execute efficiently on limited resources. C’s low-level access to memory and straightforward syntax help in crafting robust kernels and drivers.
Some unique features of C in operating systems include its straightforward handling of hardware interrupts and system calls. Operating systems written in C are often notable for their performance but could face challenges related to portability across different hardware. Despite this, C remains a beneficial choice for systems programming due to its balance of power and simplicity.
Embedded Systems
C programming excels in embedded systems, where resources are limited, and efficiency is critical. These systems often require direct hardware manipulation, which C effectively supports. The key characteristic of embedded systems is their reliance on real-time performance. C allows developers to write programs that can run efficiently with minimal overhead.
A unique feature of C in embedded systems is its predictability in resource usage, which is crucial for performance-sensitive applications. However, working with C can introduce complexities, particularly regarding memory management. Developers must be cautious about memory usage to avoid issues like leaks, impacting the device's performance.
Software Development Tools
C language has a strong presence in the development of various software tools. Many compilers and interpreters for other programming languages are developed using C, showcasing its versatility. The key characteristic that sets these software development tools apart is their performance during code compilation and execution. C offers advantages in creating diagnostics and testing tools due to its support for low-level operations.
However, there are some disadvantages when using C for software tools, mainly associated with its complexity and steep learning curve for newcomers. Despite these challenges, C remains a popular and beneficial choice in software development due to its foundational role in the programming ecosystem.
vs. Other Programming Languages
Comparison with ++
C++ builds on C by adding features such as object-oriented programming. This characteristic allows developers to create more complex and maintainable code structures. Being a superset of C, C++ retains all C functionalities while expanding on them. This can be a beneficial trait when maintaining compatibility with existing C codebases.
One unique feature of C++ is its standard template library, which simplifies many programming tasks. However, this added complexity can lead to longer learning times, making straightforward C often preferable for simpler applications. In contexts where performance and simplicity are key, C still stands strong against C++.
Comparison with Python
Python is often seen as a user-friendly language that allows rapid application development. The key characteristic distinguishing Python from C is its high-level nature, making it easier to write and read but sacrificing some performance benefits intrinsic to C. For applications where execution speed is crucial, C is favored. However, for scripting and automation tasks, many choose Python due to its simplicity and extensive libraries.
Although Python excels in speed of development, the trade-off is a potential performance hit. C provides precision control over system resources, which is a valuable asset in environments where efficiency is critical. Each language has its merits, and the choice often depends on project requirements.
Best Practices in Programming
Best practices in C programming are essential for creating maintainable and efficient code. Following these guidelines can significantly enhance the reliability and readability of a program. They provide a framework that not only decreases the likelihood of bugs but also improves collaboration among developers. Adhering to best practices fosters a culture of programming that emphasizes clarity and organization.
Code Organization and Documentation
File Structure
The file structure for a C project plays a significant role in maintaining clarity and ease of navigation. A well-defined file structure usually separates source files, header files, and documentation. This organization allows developers to easily locate and modify code as needed.
A key characteristic of a good file structure is the use of directories to categorize files based on functionality. For instance, placing all utility functions in a dedicated folder enables swift identification and access. This approach is beneficial because it reduces the time spent searching for files, allowing developers to focus on coding rather than navigating through chaos.
However, a potential disadvantage of complex file structures is the initial time investment required to design it. Developers need to establish clear conventions from the outset. If done poorly, it may lead to confusion later on, especially for new team members joining a project.
Commenting Techniques
Commenting techniques are vital for enhancing code clarity and preserving intent. Effective comments explain why a certain approach was taken, making it easier understand the logic behind complex segments. Instead of commenting on what the code does, which is often evident, focusing on why it exists provides deeper insights.
One prominent feature of good commenting practices is the usage of meaningful comments. Simply stating the function of a code block does not provide any value. Instead, comments should convey the reasoning and the thought process behind decisions, which is beneficial for future reference.
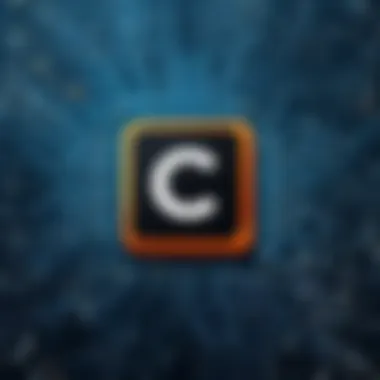
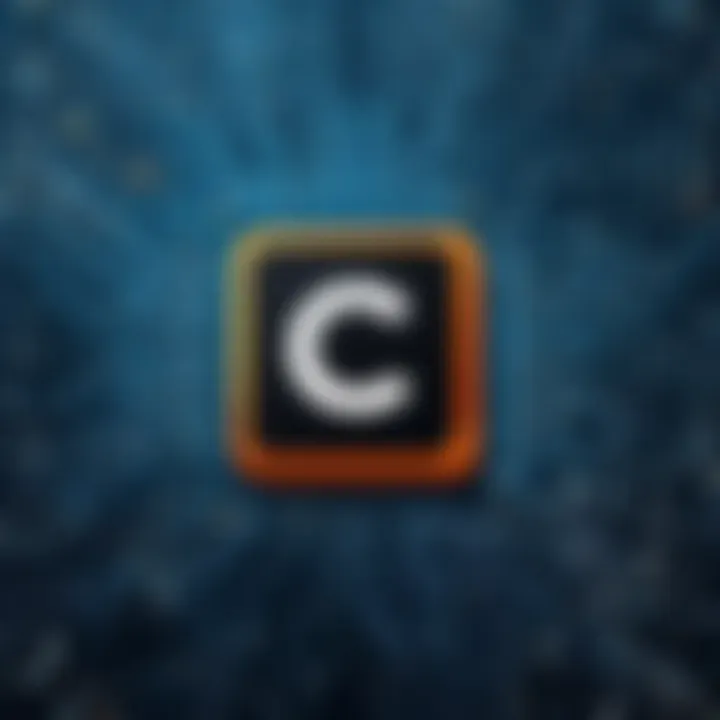
A potential drawback is over-commenting. It can clutter the code and make it harder to read. Striking the right balance between adequate and excessive detail is essential. Clear and concise comments are the ultimate goal.
Optimizing Code
Profiling Tools
Profiling tools are essential for identifying performance bottlenecks in code. They allow developers to analyze where time and resources are spent during execution. This aspect is crucial in understanding how to optimize and improve program speed.
The key characteristic of profiling tools is their ability to provide detailed reports about function calls and execution time. This data helps prioritize areas that need improvement. These tools are highly beneficial because they offer empirical evidence rather than assumptions, guiding developers toward more informed decisions about optimization.
On the downside, using profiling tools requires some learning curve. Developers must understand tool outputs and may need to adjust their coding habits based on insights. However, the long-term benefits often outweigh this initial challenge.
Algorithm Efficiency
Algorithm efficiency is a critical measure of a program’s performance. It describes the optimal way to solve a problem while minimizing resource consumption. Focusing on algorithm efficiency can drastically improve both speed and memory usage in applications.
A defining feature of algorithm efficiency is the trade-off between time complexity and space complexity. Understanding when to prioritize one over the other is essential for developers. This practice enhances program performance without unnecessary resource overhead, making it a necessary focus for serious C programmers.
Despite its advantages, improving algorithm efficiency can sometimes lead to less-readable code. More complex solutions may be optimal but difficult to trace for someone unfamiliar with the code. Striking a balance between clarity and efficiency is a continual challenge in software development.
Following these best practices in C programming leads to cleaner, more efficient, and maintainable code.
Challenges in Programming
C programming language, while powerful and efficient, presents various challenges that programmers frequently encounter. Understanding these challenges is vital for improving programming proficiency and maintaining software quality. Acknowledging potential pitfalls and difficulties helps developers minimize errors and write more secure code. Key areas of concern include memory management, error handling, and the general need for rigorous attention to detail.
Common Pitfalls
Memory Leaks
Memory leaks occur when a program allocates memory but does not properly release it after use. Such leaks result in reduced performance and can eventually exhaust available memory, leading to application crashes. This issue is particularly significant in long-running applications where memory usage accumulates over time.
The importance of understanding memory leaks lies in their pervasive nature in C programming. Developers should adopt practices that involve thorough management of memory allocation and deallocation through functions such as and .
Key characteristics of memory leaks include:
- Unreleased memory remains allocated, consuming resources.
- Difficult to detect in large codebases.
Consequently, memory leaks are detrimental for performance and reliability. A unique feature of handling this issue is the use of tools like Valgrind, which can detect memory leaks during the development process. Thus, effectively managing memory not only prevents leakages but also enhances the overall stability and performance of applications.
Pointer Dereferencing Errors
Pointer dereferencing errors happen when a program tries to access an invalid or uninitialized pointer. This scenario can lead to segmentation faults, making programs unreliable. Such errors often occur as a result of mishandling memory addresses or failing to check pointer validity before access.
The gravity of pointer dereferencing errors is evident in that they can introduce severe bugs and vulnerabilities in software. An essential characteristic of these errors is their connection to C's manual memory management, which places the onus on developers to ensure pointer integrity.
Advantages of addressing pointer dereferencing errors include:
- Improvement in code reliability.
- Prevention of potential security risks associated with accessing invalid memory locations.
Best practices to mitigate these risks involve employing defensive programming techniques, like initializing pointers before use and checking pointers against . By prioritizing pointer safety, programmers can ensure robust applications that stand the test of time.
Future of Language
The future of the C language appears promising, driven by ongoing industry trends and emerging applications. Adaptations within tech landscapes ensure that C remains relevant and continues to be a fundamental language.
Industry Trends
Current trends reflect a growing interest in low-level programming, making C increasingly relevant in systems programming, embedded systems, and IoT devices. The language's efficiency in resource management meets the demands for performance-centric applications.
A distinct feature of these trends is the renewal of interest in C for performance-critical software, such as operating systems and drivers. Industry professionals appreciate C for its ability to bridge hardware and high-level programming. This reliability ensures a bright future, as C is likely to remain in demand for applications that prioritize efficiency and control.
Emerging Applications
Emerging applications in fields like artificial intelligence and machine learning involve C due to its speed and low-level operations. C's compatibility with hardware allows developers to optimize algorithms essential for these evolving areas.
The prominent aspect of emerging applications is their reliance on C for specific performance requirements. Unique features include:
- Ability to interface closely with hardware.
- Flexibility in deploying on various platforms.
Understanding these aspects of C's evolving landscape is crucial for developers looking to leverage its strengths. As technology continues to progress, so too will the relevance of C in software development, affirming its role as a crucial language in the modern era.
Closure
The conclusion serves as a crucial component in this comprehensive overview of the C coding language. It synthesizes key themes presented throughout the article and reinforces the significance of C in both historical and modern contexts. Understanding the conclusion allows readers to reflect on their learning journey and appreciate the interconnectedness of C's various aspects.
Recapitulation of ’s Importance
C has been fundamental in shaping the field of programming. Its design principles emphasize efficiency and control, making it a preferred choice for system-level programming. Many modern programming languages have borrowed concepts from C, which speaks volumes about its lasting influence.
Some key points about C's importance include:
- Performance: C provides a low-level access to memory, which enhances performance.
- Portability: C code can be compiled on different platforms, leading to widespread application.
- Foundation for Other Languages: The structure of C laid the groundwork for languages such as C++, Java, and Python.
Thus, knowledge of C allows developers to understand and work with a variety of other programming languages more effectively.
Encouraging Further Learning
While this article covers a plethora of topics surrounding the C coding language, it serves as just a starting point. The field of programming is vast. To deepen understanding, individuals are encouraged to explore further learning opportunities.
Here are a few suggestions for continued education:
- Books: Seek out books focused on C programming, such as "The C Programming Language" by Brian W. Kernighan and Dennis M. Ritchie.
- Online Courses: Platforms like Coursera and Udacity offer detailed courses in C programming.
- Join Communities: Engaging with forums like Reddit or tech groups on Facebook can provide practical insights and mentorship opportunities.